Tizen Native API
7.0
|
Following the same idea as the Ecore_Con - Creating a server , this example will demonstrate how to create a client that connects to a specified server through a TCP port. You can see the full source code at ecore_con_client_simple_example::c.
Starting from the main
function, after reading the command line argument list and initializing the libraries, we try to connect to the server:
main(int argc, const char *argv[]) { Ecore_Con_Server *svr; const char *address; int port = 8080; if (argc < 2) { printf("wrong usage. Command syntax is:\n"); printf("\tecore_con_client_simple_example <address> [port]\n"); exit(1); } address = argv[1]; if (argc > 2) port = atoi(argv[2]); eina_init(); ecore_init(); ecore_con_init(); if (!(svr = ecore_con_server_connect(ECORE_CON_REMOTE_TCP, address, port, NULL))) { printf("could not connect to the server: %s, port %d.\n", address, port); exit(2); }
After doing this, everything else in main
is setting up callbacks for the client events, starting the main loop and shutting down the libraries after it.
Now let's go to the callbacks. These callbacks are very similar to the server callbacks (our implementation for this example is very simple). On the _add
callback, we just set a data structure to the server, print some information about the server, and send a welcome message to it:
Eina_Bool _add(void *data EINA_UNUSED, int type EINA_UNUSED, Ecore_Con_Event_Server_Add *ev) { char welcome[] = "hello! - sent from the client"; struct _Server *server = malloc(sizeof(*server)); server->sdata = 0; ecore_con_server_data_set(ev->server, server); printf("Server with ip %s, name %s, port %d, connected = %d!\n", ecore_con_server_ip_get(ev->server), ecore_con_server_name_get(ev->server), ecore_con_server_port_get(ev->server), ecore_con_server_connected_get(ev->server)); ecore_con_server_send(ev->server, welcome, sizeof(welcome)); ecore_con_server_flush(ev->server); return ECORE_CALLBACK_RENEW; }
The _del
callback is as simple as the previous one. We free the data associated with the server, print the uptime of this client, and quit the main loop (since there's nothing to do once we disconnect):
Eina_Bool _del(void *data EINA_UNUSED, int type EINA_UNUSED, Ecore_Con_Event_Server_Del *ev) { if (!ev->server) { printf("Failed to establish connection to the server.\nExiting.\n"); ecore_main_loop_quit(); return ECORE_CALLBACK_RENEW; }
The _data
callback is also similar to the server data callback. it will print any received data, and increase the data counter in the structure associated with this server:
Eina_Bool _data(void *data EINA_UNUSED, int type EINA_UNUSED, Ecore_Con_Event_Server_Data *ev) { char fmt[128]; struct _Server *server = ecore_con_server_data_get(ev->server); snprintf(fmt, sizeof(fmt), "Received %i bytes from server:\n" ">>>>>\n" "%%.%is\n" ">>>>>\n", ev->size, ev->size); printf(fmt, ev->data); server->sdata += ev->size; return ECORE_CALLBACK_RENEW; }
You can see the server counterpart functions of the ones used in this example in the Ecore_Con - Creating a server.
This example will connect to the server and start comunicating with it, as demonstrated in the following diagram:
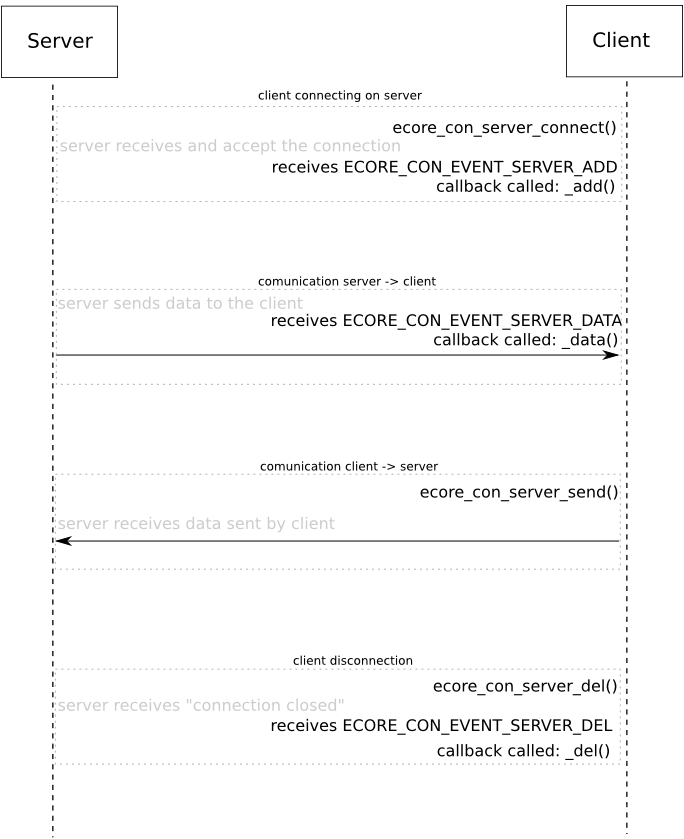
- Note:
- This example contains a serious security flaw: it doesn't check for the size of data being received, thus allowing to the string to be exploited in some way. However, it is left like this to make the code simpler and just demonstrate the API usage.