Tizen Native API
7.0
|
The App Common API provides functions for getting information about the application.
Required Header
#include <app_common.h>
Overview
The App common API provides common APIs that can be used at UI application and Service application. This API provides interfaces for getting information about the application.
Application Package
The Tizen native application consists of structured directories to manage the application executable file, library files, resource files, and data. When you build the application, the Tizen SDK packages those as an application package for distribution.
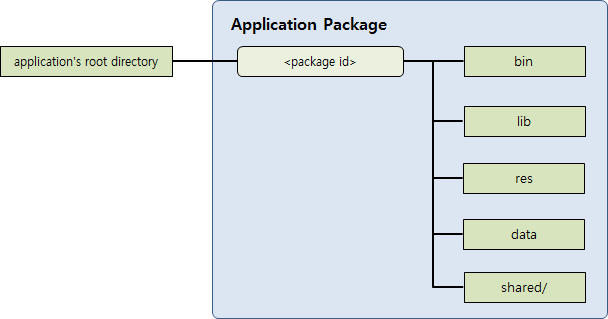
Directories | Description |
---|---|
<package id> | The fully qualified name of an application (such as org.tizen.calculator). |
bin | The executable file of the application. |
lib | The application library files |
res | The root directory in which all resource files are located. The application cannot write and modify any resource files |
data | The root directory in which an application's private data is located. The application can read and write its own data files in the application's data directory. |
shared/ | The shared directory for sharing with other applications. |
Getting Information About the Application
The API provides functions for obtaining an application's package name and absolute path to specified resources like Image, Sound, Video, UI layout (EDJ), and so on. It also provides functions to:
- Get the current orientation of the device
- Get the Internal/External root folders which are shared among all applications
Functions | |
int | app_event_get_low_memory_status (app_event_info_h event_info, app_event_low_memory_status_e *status) |
Gets the low memory status from the given event info. | |
int | app_event_get_low_battery_status (app_event_info_h event_info, app_event_low_battery_status_e *status) |
Gets the low battery status from given event info. | |
int | app_event_get_language (app_event_info_h event_info, char **lang) |
Gets the language from the given event info. | |
int | app_event_get_region_format (app_event_info_h event_info, char **region) |
Gets the region format from the given event info. | |
int | app_event_get_device_orientation (app_event_info_h event_info, app_device_orientation_e *orientation) |
Gets the device orientation from the given event info. | |
int | app_event_get_suspended_state (app_event_info_h event_info, app_suspended_state_e *state) |
Gets the suspended state of the application from the given event info. | |
int | app_get_id (char **id) |
Gets the ID of the application. | |
int | app_get_name (char **name) |
Gets the localized name of the application. | |
int | app_get_version (char **version) |
Gets the version of the application package. | |
char * | app_get_data_path (void) |
Gets the absolute path to the application's data directory which is used to store private data of the application. | |
char * | app_get_cache_path (void) |
Gets the absolute path to the application's cache directory which is used to store temporary data of the application. | |
char * | app_get_resource_path (void) |
Gets the absolute path to the application resource directory. The resource files are delivered with the application package. | |
char * | app_get_shared_data_path (void) |
Gets the absolute path to the application's shared data directory which is used to share data with other applications. | |
char * | app_get_shared_resource_path (void) |
Gets the absolute path to the application's shared resource directory which is used to share resources with other applications. | |
char * | app_get_shared_trusted_path (void) |
Gets the absolute path to the application's shared trusted directory which is used to share data with a family of trusted applications. | |
char * | app_get_external_data_path (void) |
Gets the absolute path to the application's external data directory which is used to store data of the application. | |
char * | app_get_external_cache_path (void) |
Gets the absolute path to the application's external cache directory which is used to store temporary data of the application. | |
char * | app_get_external_shared_data_path (void) TIZEN_DEPRECATED_API |
Gets the absolute path to the application's external shared data directory which is used to share data with other applications. | |
char * | app_get_tep_resource_path (void) |
Gets the absolute path to the application's TEP (Tizen Expansion Package) directory. The resource files are delivered with the expansion package. | |
int | app_get_display_state (app_display_state_e *display_state) |
Gets the display state. | |
int | app_watchdog_timer_enable (void) |
Sends the enable request to activate the watchdog timer. | |
int | app_watchdog_timer_disable (void) |
Sends the disable request to deactivate the watchdog timer. | |
int | app_watchdog_timer_kick (void) |
Sends the kick request to reset the watchdog timer. | |
Typedefs | |
typedef struct app_event_handler * | app_event_handler_h |
The event handler that returned from add event handler function to handle. | |
typedef struct app_event_info * | app_event_info_h |
The system event information handle. | |
typedef void(* | app_event_cb )(app_event_info_h event_info, void *user_data) |
The system event callback function. |
Typedef Documentation
typedef void(* app_event_cb)(app_event_info_h event_info, void *user_data) |
The system event callback function.
- Since :
- 2.3
- Remarks:
- If the given event_info has APP_SUSPENDED_STATE_WILL_ENTER value, the application should not call any asynchronous operations in this callback. After the callback returns, process of the application will be changed to suspended state immediately. Thus, asynchronous operations may work incorrectly. (since 2.4)
- Parameters:
-
[in] event_info The system event information [in] user_data The user data passed from the add event handler function
- See also:
- ui_app_add_event_handler
- app_event_info_h
typedef struct app_event_handler* app_event_handler_h |
The event handler that returned from add event handler function to handle.
- Since :
- 2.3
typedef struct app_event_info* app_event_info_h |
The system event information handle.
- Since :
- 2.3
Enumeration Type Documentation
Enumeration for device orientation.
- Since :
- 2.3
enum app_display_state_e |
enum app_event_type_e |
Enumeration for system events.
- Since :
- 2.3
- Enumerator:
APP_EVENT_LOW_MEMORY The low memory event
APP_EVENT_LOW_BATTERY The low battery event
APP_EVENT_LANGUAGE_CHANGED The system language changed event
APP_EVENT_DEVICE_ORIENTATION_CHANGED The device orientation changed event
APP_EVENT_REGION_FORMAT_CHANGED The region format changed event
APP_EVENT_SUSPENDED_STATE_CHANGED The suspended state changed event of the application (since 2.4)
- See also:
- app_event_get_suspended_state()
APP_EVENT_UPDATE_REQUESTED The update requested event (Since 3.0) This event can occur when an app needs to be updated. It is dependent on target devices.
Function Documentation
int app_event_get_device_orientation | ( | app_event_info_h | event_info, |
app_device_orientation_e * | orientation | ||
) |
Gets the device orientation from the given event info.
- Since :
- 2.3
- Parameters:
-
[in] event_info The system event info [out] orientation The device orientation changed
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_INVALID_CONTEXT Invalid event context
- See also:
- app_event_info_h
- app_device_orientation_e
int app_event_get_language | ( | app_event_info_h | event_info, |
char ** | lang | ||
) |
Gets the language from the given event info.
- Since :
- 2.3
- Remarks:
- lang must be released using free().
- Parameters:
-
[in] event_info The system event info [out] lang The language changed
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_INVALID_CONTEXT Invalid event context
- See also:
- app_event_info_h
int app_event_get_low_battery_status | ( | app_event_info_h | event_info, |
app_event_low_battery_status_e * | status | ||
) |
Gets the low battery status from given event info.
- Since :
- 2.3
- Parameters:
-
[in] event_info The system event info [out] status The low battery status
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_INVALID_CONTEXT Invalid event context
int app_event_get_low_memory_status | ( | app_event_info_h | event_info, |
app_event_low_memory_status_e * | status | ||
) |
Gets the low memory status from the given event info.
- Since :
- 2.3
- Parameters:
-
[in] event_info The system event info [out] status The low memory status
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_INVALID_CONTEXT Invalid event context
int app_event_get_region_format | ( | app_event_info_h | event_info, |
char ** | region | ||
) |
Gets the region format from the given event info.
- Since :
- 2.3
- Remarks:
- region must be released using free().
- Parameters:
-
[in] event_info The system event info [out] region The region format changed
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_INVALID_CONTEXT Invalid event context
- See also:
- app_event_info_h
int app_event_get_suspended_state | ( | app_event_info_h | event_info, |
app_suspended_state_e * | state | ||
) |
Gets the suspended state of the application from the given event info.
- Since :
- 2.4
- Remarks:
- The application should not use any asynchronous operations in APP_SUSPENDED_STATE_WILL_ENTER event. Because applications will be changed to suspended state just after APP_SUSPENDED_STATE_WILL_ENTER, asynchronous calls are not guaranteed to work properly.
- Parameters:
-
[in] event_info The handle for getting the suspended state [out] state The suspended state of the application
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_INVALID_CONTEXT Invalid event context
char* app_get_cache_path | ( | void | ) |
Gets the absolute path to the application's cache directory which is used to store temporary data of the application.
An application can read and write its own files in the application's cache directory.
- Since :
- 2.3
- Remarks:
- The returned value should be released using free().
The files stored in the application's cache directory can be removed by setting application or platform while the application is running.
- Returns:
- The absolute path to the application's cache directory,
otherwise a null pointer if the memory is insufficient
char* app_get_data_path | ( | void | ) |
Gets the absolute path to the application's data directory which is used to store private data of the application.
An application can read and write its own files in the application's data directory.
- Since :
- 2.3
- Remarks:
- The returned value should be released using free().
- Returns:
- The absolute path to the application's data directory,
otherwise a null pointer if the memory is insufficient
int app_get_display_state | ( | app_display_state_e * | display_state | ) |
Gets the display state.
- Since :
- 5.5
- Remarks:
- If the application is service-application, this function returns APP_ERROR_INVALID_CONTEXT. In the headless device, this function returns APP_ERROR_NOT_SUPPORTED.
- Parameters:
-
[out] display_state The display state
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_NOT_SUPPORTED Not supported APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_INVALID_CONTEXT The display is in an unknown state.
char* app_get_external_cache_path | ( | void | ) |
Gets the absolute path to the application's external cache directory which is used to store temporary data of the application.
An application can read and write its own files in the application's external cache directory.
- Since :
- 2.3
- Remarks:
- The returned value should be released using free().
The files stored in the application's external cache directory can be removed by setting application while the application is running.
The important files stored in the application's external cache directory should be encrypted because they can be exported via the external sdcard. To access the path returned by this function requires the privilege that is "http://tizen.org/privilege/externalstorage.appdata".
- Returns:
- The absolute path to the application's external cache directory,
otherwise a null pointer if the memory is insufficient
char* app_get_external_data_path | ( | void | ) |
Gets the absolute path to the application's external data directory which is used to store data of the application.
An application can read and write its own files in the application's external data directory.
- Since :
- 2.3
- Remarks:
- The returned value should be released using free().
The important files stored in the application's external data directory should be encrypted because they can be exported via the external sdcard. To access the path returned by this function requires the privilege that is "http://tizen.org/privilege/externalstorage.appdata".
- Returns:
- The absolute path to the application's external data directory,
otherwise a null pointer if the memory is insufficient
char* app_get_external_shared_data_path | ( | void | ) |
Gets the absolute path to the application's external shared data directory which is used to share data with other applications.
- Deprecated:
- Deprecated since 2.4.
An application can read and write its own files in the application's external shared data directory, and others can only read the files.
- Since :
- 2.3
- Remarks:
- The returned value should be released using free(). To access the path returned by this function requires the privilege that is "http://tizen.org/privilege/externalstorage.appdata". The function may not work as intended in certain devices due to some implementation issues.
- Returns:
- The absolute path to the application's external shared data directory,
otherwise a null pointer if the memory is insufficient
int app_get_id | ( | char ** | id | ) |
Gets the ID of the application.
- Since :
- 2.3
- Remarks:
- id must be released using free().
- Parameters:
-
[out] id The ID of the application
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_INVALID_CONTEXT The application is launched illegally, not launched by the launch system APP_ERROR_OUT_OF_MEMORY Out of memory
int app_get_name | ( | char ** | name | ) |
Gets the localized name of the application.
- Since :
- 2.3
- Remarks:
- name must be released using free().
- Parameters:
-
[out] name The name of the application
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_INVALID_CONTEXT The application is launched illegally, not launched by the launch system APP_ERROR_OUT_OF_MEMORY Out of memory
char* app_get_resource_path | ( | void | ) |
Gets the absolute path to the application resource directory. The resource files are delivered with the application package.
An application can only read its own files in the application's resource directory.
- Since :
- 2.3
- Remarks:
- The returned value should be released using free().
- Returns:
- The absolute path to the application's resource directory,
otherwise a null pointer if the memory is insufficient
char* app_get_shared_data_path | ( | void | ) |
Gets the absolute path to the application's shared data directory which is used to share data with other applications.
An application can read and write its own files in the application's shared data directory and others can only read the files.
- Since :
- 2.3
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/appdir.shareddata
- Remarks:
- The returned value should be released using free().
Since Tizen 3.0, an application that want to use shared/data directory must declare http://tizen.org/privilege/appdir.shareddata privilege. If the application doesn't declare the privilege, the framework will not create shared/data directory for the application. Carefully consider the privacy implications when deciding whether to use the shared/data directory, since the application cannot control access to this directory by other applications. If you want to share files with other applications, consider passing path via App Control API. The App Control API supports giving permission to other applications by passing path via app_control.
The specific error code can be obtained using the get_last_result(). Error codes are described in Exception section.
- Returns:
- The absolute path to the application's shared data directory,
otherwise a null pointer if the memory is insufficient. It will return NULL for applications with api-version 3.0 or later, and set APP_ERROR_PERMISSION_DENIED if the application does not declare the shareddata privilege.
- Exceptions:
-
APP_ERROR_NONE Success APP_ERROR_PERMISSION_DENIED Permission denied APP_ERROR_OUT_OF_MEMORY Out of memory
char* app_get_shared_resource_path | ( | void | ) |
Gets the absolute path to the application's shared resource directory which is used to share resources with other applications.
An application can read its own files in the application's shared resource directory, and others can only read the files.
- Since :
- 2.3
- Remarks:
- The returned value should be released using free().
- Returns:
- The absolute path to the application's shared resource directory,
otherwise a null pointer if the memory is insufficient
char* app_get_shared_trusted_path | ( | void | ) |
Gets the absolute path to the application's shared trusted directory which is used to share data with a family of trusted applications.
An application can read and write its own files in the application's shared trusted directory and the family applications signed with the same certificate can read and write the files in the shared trusted directory.
- Since :
- 2.3
- Remarks:
- The returned value should be released using free().
- Returns:
- The absolute path to the application's shared trusted directory,
otherwise a null pointer if the memory is insufficient
char* app_get_tep_resource_path | ( | void | ) |
Gets the absolute path to the application's TEP (Tizen Expansion Package) directory. The resource files are delivered with the expansion package.
An application can only read its own files in the application's TEP (Tizen Expansion Package) directory.
- Since :
- 2.4
- Remarks:
- The returned value should be released using free().
- Returns:
- The absolute path to the application's TEP (Tizen Expansion Package) directory,
otherwise a null pointer if the memory is insufficient
int app_get_version | ( | char ** | version | ) |
Gets the version of the application package.
- Since :
- 2.3
- Remarks:
- version must be released using free().
- Parameters:
-
[out] version The version of the application
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_INVALID_CONTEXT The application is launched illegally, not launched by the launch system APP_ERROR_OUT_OF_MEMORY Out of memory
int app_watchdog_timer_disable | ( | void | ) |
Sends the disable request to deactivate the watchdog timer.
- Since :
- 5.5
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_CONTEXT Invalid context
- See also:
- app_watchdog_timer_enable()
int app_watchdog_timer_enable | ( | void | ) |
Sends the enable request to activate the watchdog timer.
- Since :
- 5.5
- Remarks:
- After this function is called, the system detects a timeout error. If, due to a program error as ANR (Application Not Responding), the system fails to reset the watchdog, the timer will elapse and generate a signal to terminate the running application. If the running application has to process a lot of operations, the application should disable or reset the watchdog timer.
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_CONTEXT Invalid context
int app_watchdog_timer_kick | ( | void | ) |
Sends the kick request to reset the watchdog timer.
- Since :
- 5.5
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_CONTEXT Invalid context
- See also:
- app_watchdog_timer_enable()