Tizen Native API
7.0
|
These functions provide array management.
The Array data type in Eina is designed to have very fast access to its data (compared to the Eina List). On the other hand, data can be added or removed only at the end of the array. To insert data at arbitrary positions, the Eina List is the correct container to use.
To use the array data type, eina_init() must be called before any other array functions. When no more eina array functions are used, eina_shutdown() must be called to free all the resources.
An array must be created with eina_array_new(). It allocates all the necessary data for an array. When not needed anymore, an array is freed with eina_array_free(). This frees the memory used by the Eina_Array itself, but does not free any memory used to store the data of each element. To free that memory you must iterate over the array and free each data element individually. A convenient way to do that is by using EINA_ARRAY_ITER_NEXT. An example of that pattern is given in the description of EINA_ARRAY_ITER_NEXT.
- Warning:
- Functions do not check if the used array is valid or not. It's up to the user to be sure of that. It is designed like that for performance reasons.
The usual features of an array are classic ones: to append an element, use eina_array_push() and to remove the last element, use eina_array_pop(). To retrieve the element at a given position, use eina_array_data_get(). The number of elements can be retrieved with eina_array_count().
An Eina_Array differs most notably from a conventional C array in that it can grow and shrink dynamically as elements are added and removed. Since allocating memory is expensive, when the array needs to grow it adds enough memory to hold step
additional elements, not just the element currently being added. Similarly when elements are removed, it won't deallocate until step
elements are removed.
The following image illustrates how an Eina_Array grows:
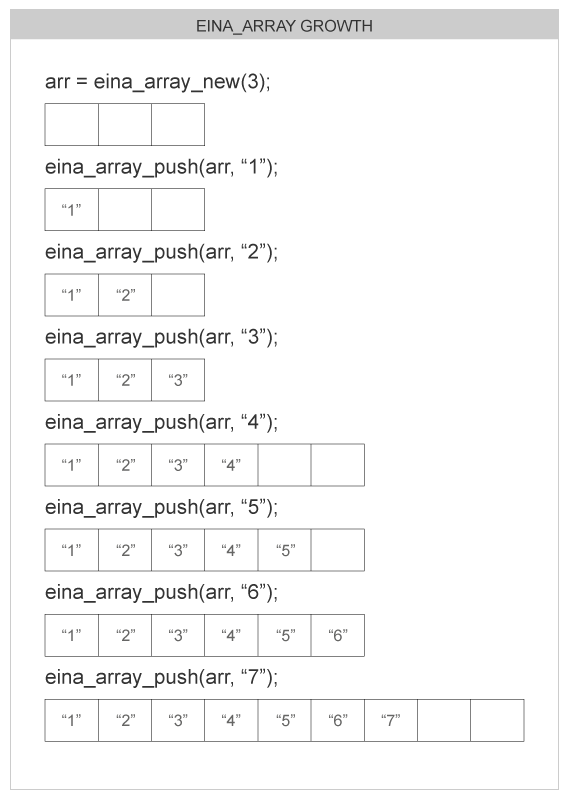
Eina_Array only stores pointers but it can store data of any type in the form of void pointers.
See here some examples:
Functions | |
Eina_Array * | eina_array_new (unsigned int step) |
Creates a new array. | |
void | eina_array_free (Eina_Array *array) |
Frees an array. | |
void | eina_array_step_set (Eina_Array *array, unsigned int sizeof_eina_array, unsigned int step) |
Sets the step of an array. | |
static void | eina_array_clean (Eina_Array *array) |
Clears an array of its elements, without deallocating memory. | |
void | eina_array_flush (Eina_Array *array) |
Clears an array's elements and deallocates the memory. | |
Eina_Bool | eina_array_remove (Eina_Array *array, Eina_Bool(*keep)(void *data, void *gdata), void *gdata) 2) |
Rebuilds an array by specifying the data to keep. | |
static Eina_Bool | eina_array_push (Eina_Array *array, const void *data) 2) |
Appends a data item to an array. | |
static void * | eina_array_pop (Eina_Array *array) |
Removes the last data item in an array. | |
static void * | eina_array_data_get (const Eina_Array *array, unsigned int idx) |
Returns the data at a given position in an array. | |
static void | eina_array_data_set (const Eina_Array *array, unsigned int idx, const void *data) |
Sets the data at a given position in an array. | |
static unsigned int | eina_array_count_get (const Eina_Array *array) |
Returns the number of elements in an array. | |
static unsigned int | eina_array_count (const Eina_Array *array) |
Returns the number of elements in an array. | |
static Eina_Bool | eina_array_find (const Eina_Array *array, const void *data, unsigned int *out_idx) |
Search for the given data in an array. | |
Eina_Iterator * | eina_array_iterator_new (const Eina_Array *array) |
Gets a new iterator associated with an array. | |
Eina_Accessor * | eina_array_accessor_new (const Eina_Array *array) |
Gets a new accessor associated with an array. | |
static Eina_Bool | eina_array_foreach (Eina_Array *array, Eina_Each_Cb cb, void *fdata) |
Iterates over an array using a callback function. | |
Typedefs | |
typedef struct _Eina_Array | Eina_Array |
typedef void ** | Eina_Array_Iterator |
Defines | |
#define | EINA_ARRAY_ITER_NEXT(array, index, item, iterator) |
Iterates through an array's elements. |
Define Documentation
#define EINA_ARRAY_ITER_NEXT | ( | array, | |
index, | |||
item, | |||
iterator | |||
) |
for (index = 0, iterator = (array)->data; \ (index < eina_array_count(array)) && ((item = *((iterator)++))); \ ++(index))
Iterates through an array's elements.
- Parameters:
-
[in] array The array to iterate over. [out] index The integer number that is increased while iterating. [out] item The data [in,out] iterator The Eina_Array_Iterator.
This macro iterates over array
in order, increasing index
from the first to last element and setting item
to each element's data item in turn.
This macro can be used for freeing the data of an array, such as the following example:
Eina_Array *array; char *item; Eina_Array_Iterator iterator; unsigned int i; // array is already filled, // its elements are just duplicated strings, // EINA_ARRAY_ITER_NEXT will be used to free those strings EINA_ARRAY_ITER_NEXT(array, i, item, iterator) free(item);
- Examples:
- eina_array_02.c.
Typedef Documentation
Type for a generic one-dimensional linear data structure.
Type for an iterator on arrays, used with EINA_ARRAY_ITER_NEXT.
Function Documentation
Eina_Accessor* eina_array_accessor_new | ( | const Eina_Array * | array | ) |
Gets a new accessor associated with an array.
- Parameters:
-
[in] array The array.
- Returns:
- A new accessor, or
NULL
ifarray
isNULL
or has no items, or if memory could not be allocated.
This function returns a newly allocated accessor associated with array
. Accessors differ from iterators in that they permit random access.
- See also:
- Accessor Functions
- Since :
- 2.3
- Examples:
- eina_accessor_01.c.
static void eina_array_clean | ( | Eina_Array * | array | ) | [static] |
Clears an array of its elements, without deallocating memory.
- Parameters:
-
[in,out] array The array to clean.
This function sets the array's
member count to 0 without freeing memory. This facilitates emptying an array and quickly refilling it with new elements. For performance reasons, there is no check of array
. If it is NULL
or invalid, the program may crash.
- Examples:
- eina_array_02.c.
static unsigned int eina_array_count | ( | const Eina_Array * | array | ) | [static] |
Returns the number of elements in an array.
- Parameters:
-
[in] array The array.
- Returns:
- The number of elements.
This function returns the number of elements in array
(array->count). For performance reasons, there is no check of array
, so if it is NULL
or invalid, the program may crash.
- Examples:
- bg_cxx_example_02.cc, and eina_array_01.c.
static unsigned int eina_array_count_get | ( | const Eina_Array * | array | ) | [static] |
Returns the number of elements in an array.
- Parameters:
-
[in] array The array.
- Returns:
- The number of elements.
This function returns the number of elements in array
(array->count). For performance reasons, there is no check of array
, so if it is NULL
or invalid, the program may crash.
static void* eina_array_data_get | ( | const Eina_Array * | array, |
unsigned int | idx | ||
) | [static] |
Returns the data at a given position in an array.
- Parameters:
-
[in] array The array. [in] idx The position of the data to retrieve.
- Returns:
- The retrieved data.
This function returns the data at the position idx
in array
. For performance reasons, there is no check of array
or idx
. If array
is NULL
or invalid, or if idx
is larger than the array's size, the program may crash.
- Examples:
- bg_cxx_example_02.cc, and eina_array_01.c.
static void eina_array_data_set | ( | const Eina_Array * | array, |
unsigned int | idx, | ||
const void * | data | ||
) | [static] |
Sets the data at a given position in an array.
- Parameters:
-
[in] array The array. [in] idx The position of the data to set. [in] data The data to set.
This function sets the data at the position idx
in array
to data
, this effectively replaces the previously held data, you must therefore get a pointer to it first if you need to free it. For performance reasons, there is no check of array
or idx
. If array
is NULL
or invalid, or if idx
is larger than the array's size, the program may crash.
- Examples:
- eina_array_02.c.
static Eina_Bool eina_array_find | ( | const Eina_Array * | array, |
const void * | data, | ||
unsigned int * | out_idx | ||
) | [static] |
Search for the given data in an array.
- Parameters:
-
[in] array The array. [in] data need to be found. [out] out_idx The position of the data in the array if found.
- Returns:
- EINA_TRUE if found otherwise returns EINA_FALSE.
This function searches for the data pointer data
inside array
, returning EINA_TRUE
if found. The exact position where the pointer is found can be retrieved through out_idx
. Please note that only the pointer is compared, not the actual data pointed by it.
- Since (EFL) :
- 1.23
void eina_array_flush | ( | Eina_Array * | array | ) |
Clears an array's elements and deallocates the memory.
- Parameters:
-
[in,out] array The array to flush.
This function sets the count and total members of array
to 0, and frees its data member and sets it to NULL. For performance reasons, there is no check of array
. If it is NULL
or invalid, the program may crash.
- Since :
- 2.3
static Eina_Bool eina_array_foreach | ( | Eina_Array * | array, |
Eina_Each_Cb | cb, | ||
void * | fdata | ||
) | [static] |
Iterates over an array using a callback function.
- Parameters:
-
[in] array The array to iterate over. [in] cb The callback to invoke for each item. [in] fdata The user data to pass to the callback.
- Returns:
- EINA_TRUE if it successfully iterated all items of the array.
This function iterates over an array in order, calling cb
for each item. cb
should return EINA_TRUE if the loop should continue, or EINA_FALSE to exit the loop, in which case eina_array_foreach() will return EINA_FALSE.
- Examples:
- eina_array_01.c, and eina_simple_xml_parser_01.c.
void eina_array_free | ( | Eina_Array * | array | ) |
Frees an array.
- Parameters:
-
[in] array The array to free.
This function finalizes array
by flushing (see eina_array_flush()), and then freeing the memory of the pointer. It does not free the memory allocated for the elements of array
. To free them, walk the array with EINA_ARRAY_ITER_NEXT.
- Since :
- 2.3
Eina_Iterator* eina_array_iterator_new | ( | const Eina_Array * | array | ) |
Gets a new iterator associated with an array.
- Parameters:
-
[in] array The array.
- Returns:
- A new iterator, or
NULL
ifarray
isNULL
or has no items, or if memory could not be allocated.
This function allocates a new iterator associated with array
. Use EINA_ARRAY_ITER_NEXT() to iterate through the array's data items in order of entry.
- See also:
- Iterator Functions
- Since :
- 2.3
- Examples:
- eina_iterator_01.c.
Eina_Array* eina_array_new | ( | unsigned int | step | ) |
Creates a new array.
- Parameters:
-
[in] step The count of pointers to add when increasing the array size.
- Returns:
NULL
on failure, nonNULL
otherwise.
This function creates a new array. When adding an element, the array allocates step
elements. When that buffer is full, adding another element will increase the buffer by step
elements again.
This function return a valid array on success, or NULL
if memory allocation fails.
- Since :
- 2.3
static void* eina_array_pop | ( | Eina_Array * | array | ) | [static] |
Removes the last data item in an array.
- Parameters:
-
[in,out] array The array.
- Returns:
- The retrieved data, or
NULL
if there are no remaining items.
This function removes the last data item from array
, decreases the length of array
and returns the data item. For performance reasons, there is no check of array
, so if it is NULL
or invalid, the program may crash.
- Examples:
- eina_array_01.c.
static Eina_Bool eina_array_push | ( | Eina_Array * | array, |
const void * | data | ||
) | [static] |
Appends a data item to an array.
- Parameters:
-
[in,out] array The array. [in] data The data to add.
- Returns:
- EINA_TRUE on success, EINA_FALSE if allocation is necessary and fails or if
data
isNULL
.
This function appends data
to array
. For performance reasons, there is no check of array
. If it is NULL
or invalid, the program may crash.
Eina_Bool eina_array_remove | ( | Eina_Array * | array, |
Eina_Bool(*)(void *data, void *gdata) | keep, | ||
void * | gdata | ||
) |
Rebuilds an array by specifying the data to keep.
- Parameters:
-
[in,out] array The array. [in] keep The functions which selects the data to keep. [in] gdata The data to pass to the function keep.
- Returns:
- EINA_TRUE on success, EINA_FALSE otherwise.
This function rebuilds array
by specifying the elements to keep with the function keep
. No empty/invalid fields are left in the array. gdata
is an additional data to pass to keep
. For performance reasons, there is no check of array
. If it is NULL
or invalid, the program may crash.
If it wasn't able to remove items due to an allocation failure, it will return EINA_FALSE.
- Since :
- 2.3
- Examples:
- eina_array_02.c.
void eina_array_step_set | ( | Eina_Array * | array, |
unsigned int | sizeof_eina_array, | ||
unsigned int | step | ||
) |
Sets the step of an array.
- Parameters:
-
[in,out] array The array. [in] sizeof_eina_array Should be the value returned by sizeof(Eina_Array). [in] step The count of pointers to add when increasing the array size.
This function sets the step of array
to step
. For performance reasons, there is no check of array
. If it is NULL
or invalid, the program may crash.
- Warning:
- This function can only be called on uninitialized arrays.
- Since :
- 2.3
- Examples:
- eina_array_01.c.