Slider
The slider component changes the range-type browser input to sliders.
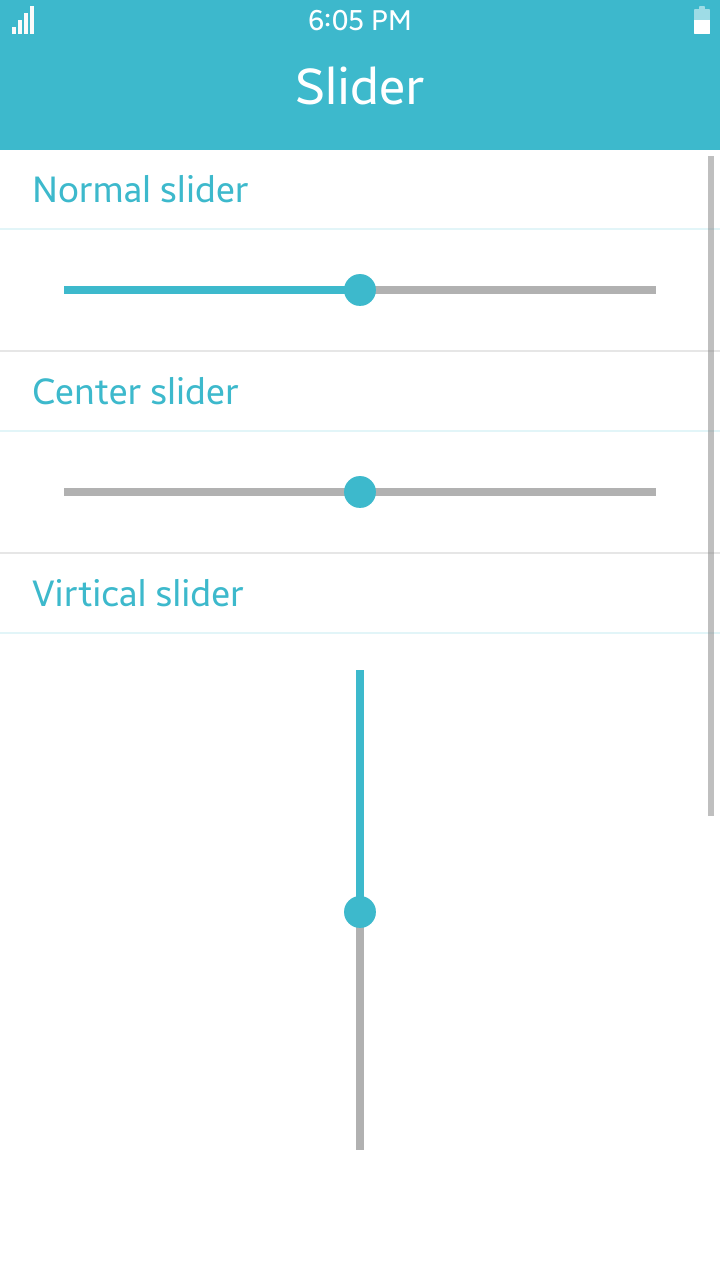
Table of Contents
Default Selectors
By default, all <input>
elements with the type="range"
attribute and data-type="range"
and data-role="slider"
are displayed as Tizen Web UI sliders.
Manual Constructor
To manually create a slider component, use the component constructor from the tau
namespace
HTML code:
<input id="slider"/>
JS code:
var sliderElement = document.getElementById("slider"), slider = tau.widget.Slider(sliderElement);
The constructor requires an HTMLElement
parameter to create the component, and you can get it with the document.getElementById()
method. The constructor can also take a second parameter, which is an object defining the configuration options for the component.
HTML Examples
To create slider input:
<input type="range" name="slider-1" id="slider" value="60" min="0" max="100"/>
Options
The following table lists the options for the slider component.
Option | Input type | Default value | Description |
---|---|---|---|
data-type | "normal" | "center" | "normal" | Sets slider style. center type is pointing to the center of the slider. |
data-orientation | "horizontal" | "vertical" | "horizontal" | Sets slider orientation. |
data-expand | boolean | false | Sets whether the slider handler is enabled. |
Methods
To call a method on the component, use one of the existing APIs:
HTML code:
<input id="slider" type="range" name="slider" value="60" min="0" max="100"/>
JS code:
var slider = document.getElementById("slider"), slider = tau.widget.Slider(slider); /* slider.methodName(methodArgument1, methodArgument2, ...); For example: */ var value = slider.value(5);
Summary
Method | Description |
---|---|
Slider disable() |
Disables the slider. |
Slider enable() |
Enables the slider. |
Slider refresh() |
Refreshes a slider markup. |
string value() |
Gets or sets a value. |
disable
-
Disables the slider.
Slider disable()
The method sets the disabled attribute for the slider and changes the look of the slider to the disabled state.
Return value:
Type Description Slider Returns this. Code example:
HTML code:
<input id="mySlider1" name="mySlider1" data-popup='false' type="range" value="5" min="0" max="10"/>
JS code:
var slider = document.getElementById("mySlider1"), sliderWidget = tau.widget.Slider(slider); sliderWidget.disable();
enable
-
Enables the slider.
Slider enable()
The method removes the disabled attribute from the slider and changes the look of the slider to the enabled state.
Return value:
Type Description Slider Returns this. Code example :
HTML code:
<input id="mySlider1" name="mySlider1" data-popup='false' type="range" value="5" min="0" max="10"/>
JS code:
var slider = document.getElementById("mySlider1"), sliderWidget = tau.widget.Slider(slider); sliderWidget.enable();
refresh
-
Refreshes a slider markup.
Slider refresh()
The method rebuilds the DOM structure of the slider component. It must be called after you manually change the HTML attributes of the component's DOM structure.
The method is called automatically after any component option is changed.
Return value:
Type Description Slider Returns this. Code example :
HTML code:
<input id="mySlider1" name="mySlider1" data-popup='false' type="range" value="5" min="0" max="10"/>
JS code:
var slider = document.getElementById("mySlider1"), sliderWidget = tau.widget.Slider(slider); sliderWidget.refresh();
value
-
Gets or sets a value.
string value()
Since: 2.3
The method returns the element value or sets the element value.
Return value:
Type Description string In the get mode, returns the element value. Code example :
HTML code:
<input id="mySlider1" name="mySlider1" data-popup='false' type="range" value="5" min="0" max="10"/>
JS code:
var slider = document.getElementById("mySlider1"), sliderWidget = tau.widget.Slider(slider); /* Value contains index of select tag */ value = sliderWidget.value(); /* Sets the index for the slider */ sliderWidget.value("1");