Tizen Native API
5.0
|
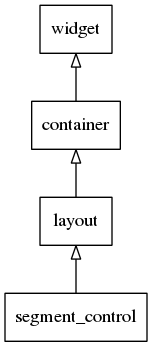

Segment control widget is a horizontal control made of multiple segment items, each segment item functioning similar to discrete two state button. A segment control groups the items together and provides compact single button with multiple equal size segments.
Segment item size is determined by base widget size and the number of items added. Only one segment item can be at selected state. A segment item can display combination of Text and any Evas_Object like Images or other widget.
This widget inherits from the Layout one, so that all the functions acting on it also work for segment control objects.
This widget emits the following signals, besides the ones sent from Layout:
"changed"
- When the user clicks on a segment item which is not previously selected and get selected. The event_info parameter is the segment item pointer."language,changed"
- the program's language changed (since 1.9)
Available styles for it:
"default"
Default content parts of the segment control items that you can use for are:
- "icon" - An icon in a segment control item
Default text parts of the segment control items that you can use for are:
- "default" - A title label in a segment control item
Supported elm_object common APIs.
- elm_object_disabled_set
- elm_object_disabled_get
Supported elm_object_item common APIs.
- elm_object_item_del
- elm_object_item_part_text_set
- elm_object_item_part_text_get
- elm_object_item_part_content_set
- elm_object_item_part_content_get
Here is an example on its usage:
Functions | |
int | elm_segment_control_item_count_get (const Elm_Segment_Control *obj) |
Get the Segment items count from segment control. | |
Elm_Widget_Item * | elm_segment_control_item_selected_get (const Elm_Segment_Control *obj) |
Get the selected item. | |
const char * | elm_segment_control_item_label_get (const Elm_Segment_Control *obj, int idx) |
Get the label of item. | |
Elm_Widget_Item * | elm_segment_control_item_insert_at (Elm_Segment_Control *obj, Efl_Canvas_Object *icon, const char *label, int idx) |
Insert a new item to the segment control object at specified position. | |
Elm_Widget_Item * | elm_segment_control_item_get (const Elm_Segment_Control *obj, int idx) |
Get the item placed at specified index. | |
void | elm_segment_control_item_del_at (Elm_Segment_Control *obj, int idx) |
Remove a segment control item at given index from its parent, deleting it. | |
Elm_Widget_Item * | elm_segment_control_item_add (Elm_Segment_Control *obj, Efl_Canvas_Object *icon, const char *label) |
Append a new item to the segment control object. | |
Efl_Canvas_Object * | elm_segment_control_item_icon_get (const Elm_Segment_Control *obj, int idx) |
Get the icon associated to the item. | |
Evas_Object * | elm_segment_control_add (Evas_Object *parent) |
Function Documentation
Evas_Object* elm_segment_control_add | ( | Evas_Object * | parent | ) |
Add a new segment control widget to the given parent Elementary (container) object.
- Parameters:
-
parent The parent object.
- Returns:
- a new segment control widget handle or
NULL
, on errors.
This function inserts a new segment control widget on the canvas.
- Since :
- 2.3
- Examples:
- segment_control_example.c.
Elm_Widget_Item* elm_segment_control_item_add | ( | Elm_Segment_Control * | obj, |
Efl_Canvas_Object * | icon, | ||
const char * | label | ||
) |
Append a new item to the segment control object.
A new item will be created and appended to the segment control, i.e., will be set as last item.
If it should be inserted at another position, elm_segment_control_item_insert_at() should be used instead.
Items created with this function can be deleted with function elm_object_item_del() or elm_object_item_del_at().
- Note:
label
set toNULL
is different from empty string "". If an item only has icon, it will be displayed bigger and centered. If it has icon and label, even that an empty string, icon will be smaller and positioned at left.
- Parameters:
-
[in] obj The object. [in] icon The icon object to use for the left side of the item. An icon can be any Evas object, but usually it is an icon created with elm_icon_add(). [in] label The label of the item. Note that, NULL is different from empty string "".
- Returns:
- The created item or
NULL
upon failure.
- Since :
- 2.3
- Examples:
- segment_control_example.c.
int elm_segment_control_item_count_get | ( | const Elm_Segment_Control * | obj | ) |
Get the Segment items count from segment control.
It will just return the number of items added to segment control obj
.
- Parameters:
-
[in] obj The object.
- Returns:
- Segment items count.
- Since :
- 2.3
- Examples:
- segment_control_example.c.
void elm_segment_control_item_del_at | ( | Elm_Segment_Control * | obj, |
int | idx | ||
) |
Remove a segment control item at given index from its parent, deleting it.
Items can be added with elm_segment_control_item_add() or elm_segment_control_item_insert_at().
- Parameters:
-
[in] obj The object. [in] idx The position of the segment control item to be deleted.
- Since :
- 2.3
- Examples:
- segment_control_example.c.
Elm_Widget_Item* elm_segment_control_item_get | ( | const Elm_Segment_Control * | obj, |
int | idx | ||
) |
Get the item placed at specified index.
Index is the position of an item in segment control widget. Its range is from $0 to count - 1
. Count is the number of items, that can be get with elm_segment_control_item_count_get().
- Parameters:
-
[in] obj The object. [in] idx The index of the segment item.
- Returns:
- The segment control item or
NULL
on failure.
- Since :
- 2.3
- Examples:
- segment_control_example.c.
Efl_Canvas_Object* elm_segment_control_item_icon_get | ( | const Elm_Segment_Control * | obj, |
int | idx | ||
) |
Get the icon associated to the item.
The return value is a pointer to the icon associated to the item when it was created, with function elm_segment_control_item_add(), or later with function elm_object_item_part_content_set(). If no icon was passed as argument, it will return NULL
.
- Parameters:
-
[in] obj The object. [in] idx The index of the segment item.
- Returns:
- The left side icon associated to the item at
index
.
- Since :
- 2.3
Elm_Widget_Item* elm_segment_control_item_insert_at | ( | Elm_Segment_Control * | obj, |
Efl_Canvas_Object * | icon, | ||
const char * | label, | ||
int | idx | ||
) |
Insert a new item to the segment control object at specified position.
Index values must be between $0, when item will be prepended to segment control, and items count, that can be get with elm_segment_control_item_count_get(), case when item will be appended to segment control, just like elm_segment_control_item_add().
Items created with this function can be deleted with function elm_object_item_del() or elm_segment_control_item_del_at().
- Note:
label
set toNULL
is different from empty string "". If an item only has icon, it will be displayed bigger and centered. If it has icon and label, even that an empty string, icon will be smaller and positioned at left.
- Parameters:
-
[in] obj The object. [in] icon The icon object to use for the left side of the item. An icon can be any Evas object, but usually it is an icon created with elm_icon_add(). [in] label The label of the item. [in] idx Item position. Value should be between 0 and items count.
- Returns:
- The created item or
NULL
upon failure.
- Since :
- 2.3
- Examples:
- segment_control_example.c.
const char* elm_segment_control_item_label_get | ( | const Elm_Segment_Control * | obj, |
int | idx | ||
) |
Get the label of item.
The return value is a pointer to the label associated to the item when it was created, with function elm_segment_control_item_add(), or later with function elm_object_item_text_set. If no label was passed as argument, it will return NULL
.
- Parameters:
-
[in] obj The object. [in] idx The index of the segment item.
- Returns:
- The label of the item at
index
.
- Since :
- 2.3
Elm_Widget_Item* elm_segment_control_item_selected_get | ( | const Elm_Segment_Control * | obj | ) |
Get the selected item.
The selected item can be unselected with function elm_segment_control_item_selected_set().
The selected item always will be highlighted on segment control.
- Parameters:
-
[in] obj The object.
- Returns:
- The selected item or
NULL
if none of segment items is selected.
- Since :
- 2.3
- Examples:
- segment_control_example.c.