Section Changer
The section changer component provides an application architecture, which has multiple sections on one page.
With Section changer on one page, you can scroll the <section> elements horizontally or vertically without changing current page to another.
Note |
---|
This component is supported since Tizen 2.4. |
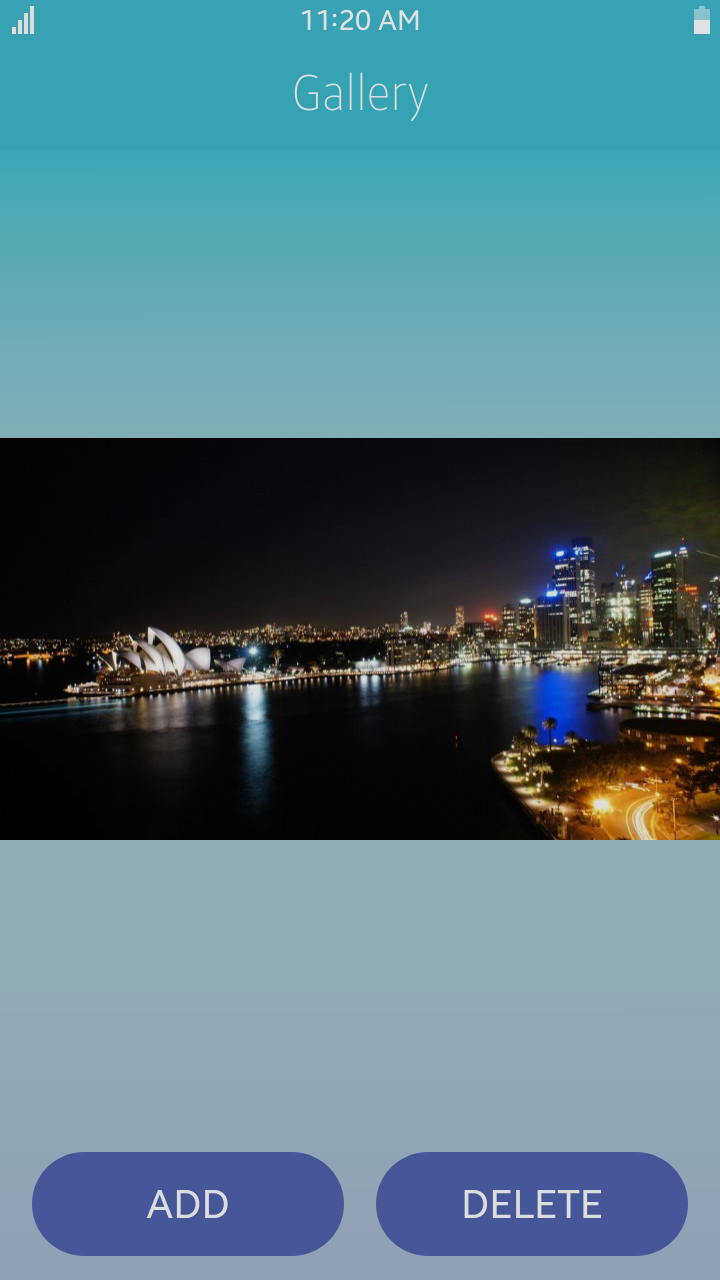
Table of Contents
Default Selectors
By default, all HTML elements with the class="ui-section-changer"
or data-role="section-changer"
attribute are displayed as SectionChanger.
HTML Example
To create a simple SectionChanger with Header.
<div id="hasSectionchangerPage" class="ui-page"> <header class="ui-header"> <h2 class="ui-title">SectionChanger</h2> </header> <div class="ui-section-changer" id="sectionchanger"> <div> <section> <h3>LEFT1 PAGE</h3> </section> <section class="ui-section-active"> <h3>MAIN PAGE</h3> </section> <section> <h3>RIGHT1 PAGE</h3> </section> </div> </div> </div>
Manual Constructor
To manually create a SectionChanger component, use the component constructor from the tau
namespace
var sectionChangerEl = document.getElementById("sectionchanger"), sectionChangerWidget = tau.widget.SectionChanger(sectionChangerEl);
Options
Option | Input type | Default value | Description |
---|---|---|---|
data-orientation | "horizontal"|"vertical" | "horizontal" | Sets the section changer orientation |
data-circular | Boolean | false | Presents the sections in a circular scroll fashion. |
data-use-bouncing-effect | Boolean | false | Shows a scroll end effect on the scroll edge. |
data-items | String | "section" | Defines the section element selector. |
data-active-class | String | "ui-section-active" | Specifies the CSS classes which define the active section element. Add the specified class (section-active) to a <section> element to indicate which section must be shown first. By default, the first section is shown first. |
data-fill-content | Boolean | true | Declares width of section tag to fill content or not. If fillContent value is set to false, you must set the width of each section tag. |
data-use-tab | Boolean | false | Defines use tab indicator on top of section changer. |
Events
Name | Description |
---|---|
sectionchange | Triggered when the section is changed. |
To use the sectionchange event, use the following code:
(function() { var changer = document.getElementById("sectionchanger"); changer.addEventListener("sectionchange", function(evt) { console.debug(evt.detail.active + " section is active."); }); })();
Methods
Summary
Method | Description |
---|---|
setActiveSection(number index) |
Changes the currently active section element (index).
|
number getActiveSectionIndex() |
Gets the currently active section element's index.
|
refresh() |
Updates the section changer form.
|
setActiveSection
-
Changes the currently active section element (index).
For smooth scrolling, the duration parameter must be in milliseconds.setActiveSection(number index)
Parameters:
Parameter Type Required / optional Default value Description index number required index which is set be active state. Return value:
No Return ValueCode example:
HTML code:
<div id="sectionChanger"></div>
JS code:
var element = document.getElementById("sectionChanger"), sectionChangerWidget = tau.widget.SectionChanger(element); sectionChangerWidget.setActiveSection(2);
getActiveSectionIndex
Gets the currently active section element's index.
The return value is a number (activeIndex).
number getActiveSectionIndex()
Return value:
Type | Description |
---|---|
number | index of active section |
Code example:
HTML code:
<div id="sectionChanger"></div>
JS code:
var element = document.getElementById("sectionChanger"), sectionChangerWidget = tau.widget.SectionChanger(element), activeSectionIndex; activeSectionIndex = sectionChangerWidget.getActiveSectionIndex();
refresh
Updates the section changer form.
When you add new section dynamically through JavaScript, you must call the refresh() method to update section information.
refresh()
Return value:
No Return ValueCode example:
HTML code:
<div id="sectionChanger"></div>
JS code:
var element = document.getElementById("sectionChanger"), sectionChangerWidget = tau.widget.SectionChanger(element); /* Do some DOM changes dynamically */ sectionChangerWidget.refresh();