Tizen Native API
|
Functions | |
void | elm_image_editable_set (Elm_Image *obj, Eina_Bool set) |
Make the image 'editable'. | |
Eina_Bool | elm_image_editable_get (const Elm_Image *obj) |
Check if the image is 'editable'. | |
void | elm_image_animated_play_set (Elm_Image *obj, Eina_Bool play) |
Start or stop an image object's animation. | |
Eina_Bool | elm_image_animated_play_get (const Elm_Image *obj) |
Get whether an image object is under animation or not. | |
void | elm_image_smooth_set (Elm_Image *obj, Eina_Bool smooth) |
Set the smooth effect for an image. | |
Eina_Bool | elm_image_smooth_get (const Elm_Image *obj) |
Get the smooth effect for an image. | |
void | elm_image_no_scale_set (Elm_Image *obj, Eina_Bool no_scale) |
Disable scaling of this object. | |
Eina_Bool | elm_image_no_scale_get (const Elm_Image *obj) |
Get whether scaling is disabled on the object. | |
void | elm_image_animated_set (Elm_Image *obj, Eina_Bool anim) |
Set whether an image object (which supports animation) is to animate itself or not. | |
Eina_Bool | elm_image_animated_get (const Elm_Image *obj) |
Get whether an image object has animation enabled or not. | |
void | elm_image_aspect_fixed_set (Elm_Image *obj, Eina_Bool fixed) |
Set whether the original aspect ratio of the image should be kept on resize. | |
Eina_Bool | elm_image_aspect_fixed_get (const Elm_Image *obj) |
Get if the object retains the original aspect ratio. | |
void | elm_image_orient_set (Elm_Image *obj, Elm_Image_Orient orient) |
Set the image orientation. | |
Elm_Image_Orient | elm_image_orient_get (const Elm_Image *obj) |
Get the image orientation. | |
void | elm_image_fill_outside_set (Elm_Image *obj, Eina_Bool fill_outside) |
Set if the image fills the entire object area, when keeping the aspect ratio. | |
Eina_Bool | elm_image_fill_outside_get (const Elm_Image *obj) |
Get if the object is filled outside. | |
void | elm_image_resizable_set (Elm_Image *obj, Eina_Bool up, Eina_Bool down) |
Set if the object is (up/down) resizable. | |
void | elm_image_resizable_get (const Elm_Image *obj, Eina_Bool *up, Eina_Bool *down) |
Get if the object is (up/down) resizable. | |
void | elm_image_preload_disabled_set (Elm_Image *obj, Eina_Bool disabled) |
Enable or disable preloading of the image. | |
Eina_Bool | elm_image_memfile_set (Elm_Image *obj, const void *img, size_t size, const char *format, const char *key) |
Set a location in memory to be used as an image object's source bitmap. | |
Eina_Bool | elm_image_animated_available_get (const Elm_Image *obj) |
Get whether an image object supports animation or not. | |
Evas_Object * | elm_image_object_get (const Elm_Image *obj) |
Get the inlined image object of the image widget. | |
void | elm_image_object_size_get (const Elm_Image *obj, int *w, int *h) |
Get the current size of the image. | |
Evas_Object * | elm_image_add (Evas_Object *parent) |
Add a new image to the parent. | |
Eina_Bool | elm_image_file_set (Evas_Object *obj, const char *file, const char *group) |
Set the file that will be used as the image's source. | |
void | elm_image_file_get (const Eo *obj, const char **file, const char **group) |
Get the file that will be used as image. | |
void | elm_image_prescale_set (Evas_Object *obj, int size) |
Set the prescale size for the image. | |
int | elm_image_prescale_get (const Evas_Object *obj) |
Get the prescale size for the image. | |
Typedefs | |
typedef struct _Elm_Image_Progress | Elm_Image_Progress |
typedef struct _Elm_Image_Error | Elm_Image_Error |
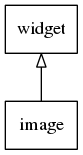
An Elementary image object is a direct realization of elm-image-class, and it allows one to load and display an image file on it, be it from a disk file or from a memory region. Exceptionally, one may also load an Edje group as the contents of the image. In this case, though, most of the functions of the image API will act as a no-op.
One can tune various properties of the image, like:
- pre-scaling,
- smooth scaling,
- orientation,
- aspect ratio during resizes, etc.
An image object may also be made valid source and destination for drag and drop actions, through the elm_image_editable_set() call.
Signals that you can add callbacks for are:
"drop"
- This is called when a user has dropped an image typed object onto the object in question -- the event info argument is the path to that image file"clicked"
- This is called when a user has clicked the image
An example of usage for this API follows:
Typedef Documentation
typedef struct _Elm_Image_Error Elm_Image_Error |
Structre associated with smart callback 'download,error'
- Since (EFL) :
- 1.8
typedef struct _Elm_Image_Progress Elm_Image_Progress |
Structure associated with smart callback 'download,progress'.
- Since (EFL) :
- 1.8
Enumeration Type Documentation
enum Elm_Image_Orient |
Possible orientation options for elm_image_orient_set().
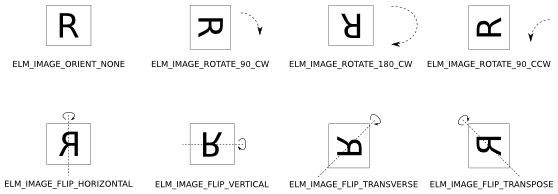
- Enumerator:
Function Documentation
Evas_Object* elm_image_add | ( | Evas_Object * | parent | ) |
Add a new image to the parent.
- Since :
- 2.3
- Parameters:
-
[in] parent The parent object
- Returns:
- The new object or NULL if it cannot be created
- See also:
- elm_image_file_set()
Eina_Bool elm_image_animated_available_get | ( | const Elm_Image * | obj | ) |
Get whether an image object supports animation or not.
- Since :
- 2.3
- Returns:
EINA_TRUE
if the image supports animation,EINA_FALSE
otherwise.
- Remarks:
- This function returns if this Elementary image object's internal image can be animated. Currently Evas only supports GIF animation. If the return value is EINA_FALSE, other
elm_image_animated_xxx
API calls won't work.
- See also:
- elm_image_animated_set()
- Since (EFL) :
- 1.7
- Parameters:
-
[in] obj The elm image object
Eina_Bool elm_image_animated_get | ( | const Elm_Image * | obj | ) |
Get whether an image object has animation enabled or not.
- Since :
- 2.3
- Returns:
EINA_TRUE
if the image has animation enabled,EINA_FALSE
otherwise.
- See also:
- elm_image_animated_set()
- Since (EFL) :
- 1.7
- Parameters:
-
[in] obj The elm image object
Eina_Bool elm_image_animated_play_get | ( | const Elm_Image * | obj | ) |
Get whether an image object is under animation or not.
- Since :
- 2.3
- Returns:
EINA_TRUE
, if the image is being animated,EINA_FALSE
otherwise.
- See also:
- elm_image_animated_play_get()
- Since (EFL) :
- 1.7
- Parameters:
-
[in] obj The elm image object
void elm_image_animated_play_set | ( | Elm_Image * | obj, |
Eina_Bool | play | ||
) |
Start or stop an image object's animation.
- Since :
- 2.3
- Remarks:
- To actually start playing any image object's animation, if it supports it, one must do something like:
if (elm_image_animated_available_get(img)) { elm_image_animated_set(img, EINA_TRUE); elm_image_animated_play_set(img, EINA_TRUE); }
- Remarks:
- elm_image_animated_set() will enable animation on the image, but not start it yet. This is the function one uses to start and stop animations on image objects.
- Since (EFL) :
- 1.7
- Parameters:
-
[in] obj The elm image object [in] play EINA_TRUE
to start the animation,EINA_FALSE
otherwise. Default isEINA_FALSE
.
void elm_image_animated_set | ( | Elm_Image * | obj, |
Eina_Bool | anim | ||
) |
Set whether an image object (which supports animation) is to animate itself or not.
- Since :
- 2.3
- Remarks:
- An image object, even if it supports animation, will be displayed by default without animation. Call this function with animated set to
EINA_TRUE
to enable its animation. To start or stop the animation, actually, use elm_image_animated_play_set().
- Since (EFL) :
- 1.7
- Parameters:
-
[in] obj The elm image object [in] anim EINA_TRUE
if the object is to animate itself,EINA_FALSE
otherwise. Default isEINA_FALSE
.
Eina_Bool elm_image_aspect_fixed_get | ( | const Elm_Image * | obj | ) |
Get if the object retains the original aspect ratio.
- Since :
- 2.3
- Returns:
EINA_TRUE
if the object keeps the original aspect,EINA_FALSE
otherwise.
- Parameters:
-
[in] obj The elm image object
void elm_image_aspect_fixed_set | ( | Elm_Image * | obj, |
Eina_Bool | fixed | ||
) |
Set whether the original aspect ratio of the image should be kept on resize.
- Since :
- 2.3
- Remarks:
- The original aspect ratio (width / height) of the image is usually distorted to match the object's size. Enabling this option will retain this original aspect, and the way that the image is fit into the object's area depends on the option set by elm_image_fill_outside_set().
- Parameters:
-
[in] obj The elm image object [in] fixed EINA_TRUE
if the image should retain the aspect,EINA_FALSE
otherwise.
Eina_Bool elm_image_editable_get | ( | const Elm_Image * | obj | ) |
Check if the image is 'editable'.
- Since :
- 2.3
- Returns:
- Editability.
- Remarks:
- A return value of EINA_TRUE means the image is a valid drag target for drag and drop, and can be cut or pasted too.
- Parameters:
-
[in] obj The elm image object
void elm_image_editable_set | ( | Elm_Image * | obj, |
Eina_Bool | set | ||
) |
Make the image 'editable'.
- Since :
- 2.3
- Remarks:
- This means the image is a valid drag target for drag and drop, and can be cut or pasted too.
- Parameters:
-
[in] obj The elm image object [in] set Turn on or off editability. Default is EINA_FALSE
.
void elm_image_file_get | ( | const Eo * | obj, |
const char ** | file, | ||
const char ** | group | ||
) |
Get the file that will be used as image.
- Since :
- 2.3
- See also:
- elm_image_file_set()
- Parameters:
-
[out] file The path to file that will be used as image source [out] group The group that the image belongs to, in case it's an EET (including Edje case) file. This can be used as a key inside evas image cache if this is a normal image file not eet file.
Eina_Bool elm_image_file_set | ( | Evas_Object * | obj, |
const char * | file, | ||
const char * | group | ||
) |
Set the file that will be used as the image's source.
- Since :
- 2.3
- Parameters:
-
[in] obj The image object [in] file The path to file that will be used as image source [in] group The group that the image belongs to, in case it's an EET (including Edje case) file. This can be used as a key inside evas image cache if this is a normal image file not eet file.
- Returns:
- (
EINA_TRUE
= success,EINA_FALSE
= error)
- See also:
- elm_image_file_get()
- Remarks:
- This function will trigger the Edje file case based on the extension of the file string (expects
".edj"
, for this case). -
If you use animated gif image and create multiple image objects with one gif image file, you should set the
group
differently for each object. Or image objects will share one evas image cache entry and you will get unwanted frames.
Eina_Bool elm_image_fill_outside_get | ( | const Elm_Image * | obj | ) |
Get if the object is filled outside.
- Since :
- 2.3
- Returns:
EINA_TRUE
if the object is filled outside,EINA_FALSE
otherwise.
- See also:
- elm_image_fill_inside_get()
- elm_image_fill_inside_set()
- elm_image_fill_outside_set()
- Parameters:
-
[in] obj The elm image object
void elm_image_fill_outside_set | ( | Elm_Image * | obj, |
Eina_Bool | fill_outside | ||
) |
Set if the image fills the entire object area, when keeping the aspect ratio.
- Since :
- 2.3
- Remarks:
- When the image should keep its aspect ratio even if resized to another aspect ratio, there are two possibilities to resize it: keep the entire image inside the limits of height and width of the object (
fill_outside
isEINA_FALSE
) or let the extra width or height go outside of the object, and the image will fill the entire object (fill_outside
isEINA_TRUE
). -
This option will have no effect if elm_image_aspect_fixed_set() is set to
EINA_FALSE
.
- See also:
- elm_image_fill_inside_get()
- elm_image_fill_inside_set()
- elm_image_fill_outside_get()
- elm_image_aspect_fixed_set()
- Parameters:
-
[in] obj The elm image object [in] fill_outside EINA_TRUE
if the object is filled outside,EINA_FALSE
otherwise. Default isEINA_FALSE
.
Eina_Bool elm_image_memfile_set | ( | Elm_Image * | obj, |
const void * | img, | ||
size_t | size, | ||
const char * | format, | ||
const char * | key | ||
) |
Set a location in memory to be used as an image object's source bitmap.
- Since :
- 2.3
- Remarks:
- This function is handy when the contents of an image file are mapped in memory, for example.
-
The
format
string should be something like"png"
,"jpg"
,"tga"
,"tiff"
,"bmp"
etc, when provided (NULL
, on the contrary). This improves the loader performance as it tries the "correct" loader first, before trying a range of other possible loaders until one succeeds.
- Returns:
- (
EINA_TRUE
= success,EINA_FALSE
= error)
- Since (EFL) :
- 1.7
- Parameters:
-
[in] obj The elm image object [in] img The binary data that will be used as image source [in] size The size of binary data blob img
[in] format (Optional) expected format of img
bytes[in] key Optional indexing key of img
to be passed to the image loader (eg. ifimg
is a memory-mapped EET file)
Eina_Bool elm_image_no_scale_get | ( | const Elm_Image * | obj | ) |
Get whether scaling is disabled on the object.
- Since :
- 2.3
- Returns:
EINA_TRUE
if scaling is disabled,EINA_FALSE
otherwise
- See also:
- elm_image_no_scale_set()
- Parameters:
-
[in] obj The elm image object
void elm_image_no_scale_set | ( | Elm_Image * | obj, |
Eina_Bool | no_scale | ||
) |
Disable scaling of this object.
- Since :
- 2.3
- Remarks:
- This function disables scaling of the elm_image widget through the function elm_object_scale_set(). However, this does not affect the widget size/resize in any way. For that effect, take a look at elm_image_resizable_set().
- Parameters:
-
[in] obj The elm image object [in] no_scale EINA_TRUE
if the object is not scalable,EINA_FALSE
otherwise. Default isEINA_FALSE
.
Evas_Object* elm_image_object_get | ( | const Elm_Image * | obj | ) |
Get the inlined image object of the image widget.
- Since :
- 2.3
- Returns:
- The inlined image object, or NULL if none exists
- Remarks:
- This function allows one to get the underlying
Evas_Object
of type Image from this elementary widget. It can be useful to do things like get the pixel data, save the image to a file, etc. - Be careful to not manipulate it, as it is under control of elementary.
- Parameters:
-
[in] obj The elm image object
void elm_image_object_size_get | ( | const Elm_Image * | obj, |
int * | w, | ||
int * | h | ||
) |
Get the current size of the image.
- Since :
- 2.3
- Remarks:
- This is the real size of the image, not the size of the object.
- Parameters:
-
[in] obj The elm image object [out] w Pointer to store width, or NULL. [out] h Pointer to store height, or NULL.
Elm_Image_Orient elm_image_orient_get | ( | const Elm_Image * | obj | ) |
Get the image orientation.
- Since :
- 2.3
- Returns:
- The image orientation Elm_Image_Orient
- See also:
- elm_image_orient_set()
- Elm_Image_Orient
- Parameters:
-
[in] obj The elm image object
void elm_image_orient_set | ( | Elm_Image * | obj, |
Elm_Image_Orient | orient | ||
) |
Set the image orientation.
- Since :
- 2.3
- Remarks:
- This function allows to rotate or flip the given image.
- See also:
- elm_image_orient_get()
- Elm_Image_Orient
- Parameters:
-
[in] obj The elm image object [in] orient The image orientation Elm_Image_Orient Default is ELM_IMAGE_ORIENT_NONE.
void elm_image_preload_disabled_set | ( | Elm_Image * | obj, |
Eina_Bool | disabled | ||
) |
Enable or disable preloading of the image.
- Since :
- 2.3
- Remarks:
- If the object is visible when calling this function the image data will be loaded asynchronously. If the object is invisible or the preloading is disabled, the image data will be loaded at render time.
- Parameters:
-
[in] obj The elm image object [in] disabled If EINA_TRUE, preloading will be disabled
int elm_image_prescale_get | ( | const Evas_Object * | obj | ) |
Get the prescale size for the image.
- Since :
- 2.3
- Parameters:
-
[in] obj The image object
- Returns:
- The prescale size
- See also:
- elm_image_prescale_set()
void elm_image_prescale_set | ( | Evas_Object * | obj, |
int | size | ||
) |
Set the prescale size for the image.
- Since :
- 2.3
- Parameters:
-
[in] obj The image object [in] size The prescale size. This value is used for both width and height, but is actually for the width rather than the height.
- Remarks:
- This function sets a loading size of an image. The image will be loaded into memory as if it was the requested size instead of the original size. This can save a lot of memory, and is important for scalable types like SVG, but also works JPG. It allows the image to be loaded already in the specified size, reducing the memory usage and load time when loading a big image with load size set to a smaller size.
- Aspect_ratio between width and height is relatively preserved. Ratio between original image and prescaled image is decided by width ratio. For example, if image's content size is 640X480, the prescale size is 160, then the ratio of width is 4, which means the loaded image size will be 160X120.
- It's equivalent to the elm_bg_load_size_set() function for bg.
- this is just a hint, the real size of the pixmap may differ depending on the type of image being loaded, being bigger than requested.
void elm_image_resizable_get | ( | const Elm_Image * | obj, |
Eina_Bool * | up, | ||
Eina_Bool * | down | ||
) |
Get if the object is (up/down) resizable.
- Since :
- 2.3
- See also:
- elm_image_resizable_set()
- Parameters:
-
[in] obj The elm image object [out] up A bool to set if the object is resizable up. Default is EINA_TRUE
.[out] down A bool to set if the object is resizable down. Default is EINA_TRUE
.
void elm_image_resizable_set | ( | Elm_Image * | obj, |
Eina_Bool | up, | ||
Eina_Bool | down | ||
) |
Set if the object is (up/down) resizable.
- Since :
- 2.3
- Remarks:
- This function limits the image resize ability. If
size_up
is set toEINA_FALSE
, the object can't have its height or width resized to a value higher than the original image size. Same is valid forsize_down
.
- See also:
- elm_image_resizable_get()
- Parameters:
-
[in] obj The elm image object [in] up A bool to set if the object is resizable up. Default is EINA_TRUE
.[in] down A bool to set if the object is resizable down. Default is EINA_TRUE
.
Eina_Bool elm_image_smooth_get | ( | const Elm_Image * | obj | ) |
Get the smooth effect for an image.
- Since :
- 2.3
- Returns:
EINA_TRUE
if smooth scaling is enabled,EINA_FALSE
otherwise.
- See also:
- elm_image_smooth_set()
- Parameters:
-
[in] obj The elm image object
void elm_image_smooth_set | ( | Elm_Image * | obj, |
Eina_Bool | smooth | ||
) |
Set the smooth effect for an image.
- Since :
- 2.3
- Remarks:
- Set the scaling algorithm to be used when scaling the image. Smooth scaling provides a better resulting image, but is slower.
- The smooth scaling should be disabled when making animations that change the image size, since it will be faster. Animations that don't require resizing of the image can keep the smooth scaling enabled (even if the image is already scaled, since the scaled image will be cached).
- See also:
- elm_image_smooth_get()
- Parameters:
-
[in] obj The elm image object [in] smooth EINA_TRUE
if smooth scaling should be used,EINA_FALSE
otherwise. Default isEINA_TRUE
.