Tizen Native API
|
The Stage is a top-level object used for displaying a tree of Actors. More...
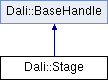
Public Types | |
typedef Signal< void(const KeyEvent &)> | KeyEventSignalType |
Key event signal type. | |
typedef Signal< void() > | EventProcessingFinishedSignalType |
Event Processing finished signal type. | |
typedef Signal< void(const TouchEvent &)> | TouchedSignalType |
Touched signal type. | |
typedef Signal< void(const WheelEvent &)> | WheelEventSignalType |
Touched signal type. | |
typedef Signal< void() > | ContextStatusSignal |
Context status signal type. | |
typedef Signal< void() > | SceneCreatedSignalType |
Scene created signal type. | |
Public Member Functions | |
Stage () | |
Allows the creation of an empty stage handle. | |
~Stage () | |
Destructor. | |
Stage (const Stage &handle) | |
This copy constructor is required for (smart) pointer semantics. | |
Stage & | operator= (const Stage &rhs) |
This assignment operator is required for (smart) pointer semantics. | |
void | Add (Actor &actor) |
Adds a child Actor to the Stage. | |
void | Remove (Actor &actor) |
Removes a child Actor from the Stage. | |
Vector2 | GetSize () const |
Returns the size of the Stage in pixels as a Vector. | |
RenderTaskList | GetRenderTaskList () const |
Retrieve the list of render-tasks. | |
unsigned int | GetLayerCount () const |
Query the number of on-stage layers. | |
Layer | GetLayer (unsigned int depth) const |
Retrieve the layer at a specified depth. | |
Layer | GetRootLayer () const |
Returns the Stage's Root Layer. | |
void | SetBackgroundColor (Vector4 color) |
Set the background color of the stage. | |
Vector4 | GetBackgroundColor () const |
Retrieve the background color of the stage. | |
Vector2 | GetDpi () const |
Retrieve the DPI of the display device to which the stage is connected. | |
ObjectRegistry | GetObjectRegistry () const |
Get the Object registry. | |
void | KeepRendering (float durationSeconds) |
Keep rendering for at least the given amount of time. | |
KeyEventSignalType & | KeyEventSignal () |
This signal is emitted when key event is received. | |
EventProcessingFinishedSignalType & | EventProcessingFinishedSignal () |
This signal is emitted just after the event processing is finished. | |
TouchedSignalType & | TouchedSignal () |
This signal is emitted when the screen is touched and when the touch ends (i.e. the down & up touch events only). | |
WheelEventSignalType & | WheelEventSignal () |
This signal is emitted when wheel event is received. | |
ContextStatusSignal & | ContextLostSignal () |
This signal is emitted when the GL context is lost (Platform specific behaviour). | |
ContextStatusSignal & | ContextRegainedSignal () |
This signal is emitted when the GL context is regained (Platform specific behaviour). | |
SceneCreatedSignalType & | SceneCreatedSignal () |
This signal is emitted after the initial scene is created. | |
Static Public Member Functions | |
static Stage | GetCurrent () |
Get the current Stage. | |
static bool | IsInstalled () |
Query whether the Stage exists; this should only return false during or after destruction of Dali core. | |
Static Public Attributes | |
static const Vector4 | DEFAULT_BACKGROUND_COLOR |
Default black background. | |
static const Vector4 | DEBUG_BACKGROUND_COLOR |
Green background, useful when debugging. |
Detailed Description
The Stage is a top-level object used for displaying a tree of Actors.
Multiple stage/window support is not currently provided.
- Since :
- 2.4
Member Typedef Documentation
typedef Signal< void () > Dali::Stage::ContextStatusSignal |
Context status signal type.
- Since :
- 2.4
typedef Signal< void () > Dali::Stage::EventProcessingFinishedSignalType |
Event Processing finished signal type.
- Since :
- 2.4
typedef Signal< void (const KeyEvent&)> Dali::Stage::KeyEventSignalType |
Key event signal type.
- Since :
- 2.4
typedef Signal< void () > Dali::Stage::SceneCreatedSignalType |
Scene created signal type.
- Since :
- 2.4
typedef Signal< void (const TouchEvent&)> Dali::Stage::TouchedSignalType |
Touched signal type.
- Since :
- 2.4
typedef Signal< void (const WheelEvent&)> Dali::Stage::WheelEventSignalType |
Touched signal type.
- Since :
- 2.4
Constructor & Destructor Documentation
Allows the creation of an empty stage handle.
To retrieve the current stage, this handle can be set using Stage::GetCurrent().
- Since :
- 2.4
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since :
- 2.4
Dali::Stage::Stage | ( | const Stage & | handle | ) |
This copy constructor is required for (smart) pointer semantics.
- Since :
- 2.4
- Parameters:
-
[in] handle A reference to the copied handle
Member Function Documentation
void Dali::Stage::Add | ( | Actor & | actor | ) |
This signal is emitted when the GL context is lost (Platform specific behaviour).
If the application is responsible for handling context loss, it should listen to this signal and tear down UI components when recieved.
- Since :
- 2.4
- Returns:
- The context lost signal to connect to.
This signal is emitted when the GL context is regained (Platform specific behaviour).
If the application is responsible for handling context loss, it should listen to this signal and rebuild UI components on receipt.
- Since :
- 2.4
- Returns:
- The context regained signal to connect to.
This signal is emitted just after the event processing is finished.
- Since :
- 2.4
- Returns:
- The signal to connect to.
Vector4 Dali::Stage::GetBackgroundColor | ( | ) | const |
Retrieve the background color of the stage.
- Since :
- 2.4
- Returns:
- The background color.
static Stage Dali::Stage::GetCurrent | ( | ) | [static] |
Get the current Stage.
- Since :
- 2.4
- Returns:
- The current stage or an empty handle if the internal core has not been created or has been already destroyed.
Vector2 Dali::Stage::GetDpi | ( | ) | const |
Retrieve the DPI of the display device to which the stage is connected.
- Since :
- 2.4
- Returns:
- The horizontal and vertical DPI
Layer Dali::Stage::GetLayer | ( | unsigned int | depth | ) | const |
Retrieve the layer at a specified depth.
- Since :
- 2.4
- Parameters:
-
[in] depth The depth.
- Returns:
- The layer found at the given depth.
- Precondition:
- Depth is less than layer count; see GetLayerCount().
unsigned int Dali::Stage::GetLayerCount | ( | ) | const |
Query the number of on-stage layers.
Note that a default layer is always provided (count >= 1).
- Since :
- 2.4
- Returns:
- The number of layers.
ObjectRegistry Dali::Stage::GetObjectRegistry | ( | ) | const |
Get the Object registry.
- Since :
- 2.4
- Returns:
- The object registry.
RenderTaskList Dali::Stage::GetRenderTaskList | ( | ) | const |
Layer Dali::Stage::GetRootLayer | ( | ) | const |
Vector2 Dali::Stage::GetSize | ( | ) | const |
static bool Dali::Stage::IsInstalled | ( | ) | [static] |
Query whether the Stage exists; this should only return false during or after destruction of Dali core.
- Since :
- 2.4
- Returns:
- True when it's safe to call Stage::GetCurrent().
void Dali::Stage::KeepRendering | ( | float | durationSeconds | ) |
Keep rendering for at least the given amount of time.
By default Dali will stop rendering when no Actor positions are being set, and when no animations are running etc. This method is useful to force screen refreshes e.g. when updating a NativeImage.
- Since :
- 2.4
- Parameters:
-
i] durationSeconds Time to keep rendering, 0 means render at least one more frame
This signal is emitted when key event is received.
A callback of the following type may be connected:
void YourCallbackName(const KeyEvent& event);
- Since :
- 2.4
- Returns:
- The signal to connect to.
This assignment operator is required for (smart) pointer semantics.
- Since :
- 2.4
- Parameters:
-
[in] rhs A reference to the copied handle
- Returns:
- A reference to this
void Dali::Stage::Remove | ( | Actor & | actor | ) |
This signal is emitted after the initial scene is created.
It will be triggered after the application init signal.
A callback of the following type may be connected:
void YourCallbackName();
- Since :
- 2.4
- Returns:
- The signal to connect to.
void Dali::Stage::SetBackgroundColor | ( | Vector4 | color | ) |
Set the background color of the stage.
- Since :
- 2.4
- Parameters:
-
[in] color The new background color.
This signal is emitted when the screen is touched and when the touch ends (i.e. the down & up touch events only).
If there are multiple touch points, then this will be emitted when the first touch occurs and then when the last finger is lifted. An interrupted event will also be emitted. A callback of the following type may be connected:
void YourCallbackName(const TouchEvent& event);
- Since :
- 2.4
- Returns:
- The touch signal to connect to.
- Note:
- Motion events are not emitted.
This signal is emitted when wheel event is received.
A callback of the following type may be connected:
void YourCallbackName(const WheelEvent& event);
- Since :
- 2.4
- Returns:
- The signal to connect to.