Tizen Native API
|
Button is a base class for different kind of buttons. More...
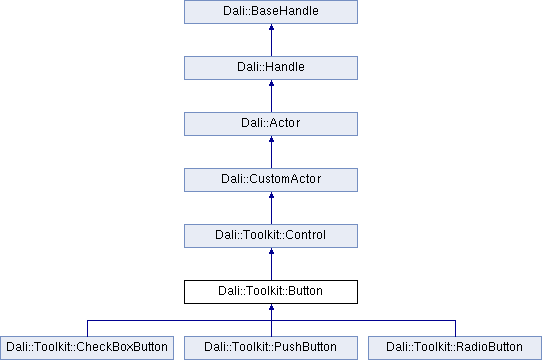
Classes | |
struct | Property |
An enumeration of properties belonging to the Button class. More... | |
Public Types | |
enum | PropertyRange |
The start and end property ranges for this control. More... | |
typedef Signal< bool(Button) > | ButtonSignalType |
Button signal type. | |
Public Member Functions | |
Button () | |
Create an uninitialized Button. | |
Button (const Button &button) | |
Copy constructor. | |
Button & | operator= (const Button &button) |
Assignment operator. | |
~Button () | |
Destructor. | |
void | SetDisabled (bool disabled) |
Sets the button as disabled. | |
bool | IsDisabled () const |
Returns if the button is disabled. | |
void | SetAutoRepeating (bool autoRepeating) |
Sets the autorepeating property. | |
bool | IsAutoRepeating () const |
Returns if the autorepeating property is set. | |
void | SetInitialAutoRepeatingDelay (float initialAutoRepeatingDelay) |
Sets the initial autorepeating delay. | |
float | GetInitialAutoRepeatingDelay () const |
Gets the initial autorepeating delay in seconds. | |
void | SetNextAutoRepeatingDelay (float nextAutoRepeatingDelay) |
Sets the next autorepeating delay. | |
float | GetNextAutoRepeatingDelay () const |
Gets the next autorepeating delay in seconds. | |
void | SetTogglableButton (bool togglable) |
Sets the togglable property. | |
bool | IsTogglableButton () const |
Returns if the togglable property is set. | |
void | SetSelected (bool selected) |
Sets the button as selected or unselected. | |
bool | IsSelected () const |
Returns if the selected property is set and the button is togglable. | |
void | SetAnimationTime (float animationTime) |
Sets the animation time. | |
float | GetAnimationTime () const |
Retrieves button's animation time. | |
void | SetLabelText (const std::string &label) |
Sets the button's label. | |
std::string | GetLabelText () const |
Gets the label. | |
void | SetUnselectedImage (const std::string &filename) |
Sets the unselected button image. | |
void | SetBackgroundImage (const std::string &filename) |
Sets the background image. | |
void | SetSelectedImage (const std::string &filename) |
Sets the selected image. | |
void | SetSelectedBackgroundImage (const std::string &filename) |
Sets the selected background image. | |
void | SetDisabledBackgroundImage (const std::string &filename) |
Sets the disabled background image. | |
void | SetDisabledImage (const std::string &filename) |
Sets the disabled button image. | |
void | SetDisabledSelectedImage (const std::string &filename) |
Sets the disabled selected button image. | |
void | SetLabel (Actor label) |
Sets the label with an actor. | |
void | SetButtonImage (Image image) |
Sets the button image. | |
void | SetSelectedImage (Image image) |
Sets the selected image. | |
Actor | GetButtonImage () const |
Gets the button image. | |
Actor | GetSelectedImage () const |
Gets the selected image. | |
ButtonSignalType & | PressedSignal () |
This signal is emitted when the button is touched. | |
ButtonSignalType & | ReleasedSignal () |
This signal is emitted when the button is touched and the touch point leaves the boundary of the button. | |
ButtonSignalType & | ClickedSignal () |
This signal is emitted when the button is touched and the touch point doesn't leave the boundary of the button. | |
ButtonSignalType & | StateChangedSignal () |
This signal is emitted when the button's state is changed. | |
Static Public Member Functions | |
static Button | DownCast (BaseHandle handle) |
Downcast a handle to Button handle. |
Detailed Description
Button is a base class for different kind of buttons.
This class provides the disabled property and the clicked signal.
A ClickedSignal() is emitted when the button is touched and the touch point doesn't leave the boundary of the button.
When the disabled property is set to true, no signal is emitted.
Button provides the following properties which modify the signals emitted:
-
autorepeating When autorepeating is set to true, a Button::PressedSignal(), Button::ReleasedSignal() and Button::ClickedSignal() signals are emitted at regular intervals while the button is touched. The intervals could be modified with the Button::SetInitialAutoRepeatingDelay and Button::SetNextAutoRepeatingDelay methods.
A togglable button can't be autorepeating. If the autorepeating property is set to true, then the togglable property is set to false but no signal is emitted.
- togglable When togglable is set to true, a Button::StateChangedSignal() signal is emitted, with the selected state.
The button's appearance can be modified by setting properties for the various image filenames.
The background is always shown and doesn't change if the button is pressed or released. The button image is shown over the background image when the button is not pressed and is replaced by the selected image when the button is pressed. The text label is placed always on the top of all images.
When the button is disabled, background, button and selected images are replaced by their disabled images.
Is not mandatory set all images. A button could be defined only by setting its background image or by setting its background and selected images.
- Since :
- 2.4
Member Typedef Documentation
typedef Signal< bool ( Button ) > Dali::Toolkit::Button::ButtonSignalType |
Button signal type.
- Since :
- 2.4
Member Enumeration Documentation
The start and end property ranges for this control.
- Since :
- 2.4
Reimplemented from Dali::Toolkit::Control.
Reimplemented in Dali::Toolkit::PushButton.
Constructor & Destructor Documentation
Create an uninitialized Button.
Only derived versions can be instantiated. Calling member functions with an uninitialized Dali::Object is not allowed.
- Since :
- 2.4
Dali::Toolkit::Button::Button | ( | const Button & | button | ) |
Copy constructor.
- Since :
- 2.4
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since :
- 2.4
Member Function Documentation
This signal is emitted when the button is touched and the touch point doesn't leave the boundary of the button.
A callback of the following type may be connected:
bool YourCallbackName( Button button );
- Since :
- 2.4
- Returns:
- The signal to connect to
static Button Dali::Toolkit::Button::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcast a handle to Button handle.
If handle points to a Button the downcast produces valid handle. If not the returned handle is left uninitialized.
- Since :
- 2.4
- Parameters:
-
[in] handle Handle to an object
- Returns:
- A handle to a Button or an uninitialized handle
Reimplemented from Dali::Toolkit::Control.
Reimplemented in Dali::Toolkit::RadioButton, Dali::Toolkit::PushButton, and Dali::Toolkit::CheckBoxButton.
float Dali::Toolkit::Button::GetAnimationTime | ( | ) | const |
Retrieves button's animation time.
- Since :
- 2.4
- Returns:
- The animation time in seconds
Actor Dali::Toolkit::Button::GetButtonImage | ( | ) | const |
Gets the button image.
- Since :
- 2.4
- Remarks:
- Avoid using this method as it's a legacy code.
- Returns:
- An actor with the button image
float Dali::Toolkit::Button::GetInitialAutoRepeatingDelay | ( | ) | const |
Gets the initial autorepeating delay in seconds.
- Since :
- 2.4
- Returns:
- The initial autorepeating delay in seconds
std::string Dali::Toolkit::Button::GetLabelText | ( | ) | const |
Gets the label.
- Since :
- 2.4
- Returns:
- The label text
float Dali::Toolkit::Button::GetNextAutoRepeatingDelay | ( | ) | const |
Gets the next autorepeating delay in seconds.
- Since :
- 2.4
- Returns:
- The next autorepeating delay in seconds
Actor Dali::Toolkit::Button::GetSelectedImage | ( | ) | const |
Gets the selected image.
- Since :
- 2.4
- Remarks:
- Avoid using this method as it's a legacy code.
- Returns:
- An actor with the selected image
bool Dali::Toolkit::Button::IsAutoRepeating | ( | ) | const |
Returns if the autorepeating property is set.
- Since :
- 2.4
- Returns:
- True if the autorepeating property is set.
bool Dali::Toolkit::Button::IsDisabled | ( | ) | const |
Returns if the button is disabled.
- Since :
- 2.4
- Returns:
- True if the button is disabled.
bool Dali::Toolkit::Button::IsSelected | ( | ) | const |
Returns if the selected property is set and the button is togglable.
- Since :
- 2.4
- Returns:
- True if the button is selected.
bool Dali::Toolkit::Button::IsTogglableButton | ( | ) | const |
Returns if the togglable property is set.
- Since :
- 2.4
- Returns:
- True if the togglable property is set.
This signal is emitted when the button is touched.
A callback of the following type may be connected:
bool YourCallbackName( Button button );
- Since :
- 2.4
- Returns:
- The signal to connect to
This signal is emitted when the button is touched and the touch point leaves the boundary of the button.
A callback of the following type may be connected:
bool YourCallbackName( Button button );
- Since :
- 2.4
- Returns:
- The signal to connect to
void Dali::Toolkit::Button::SetAnimationTime | ( | float | animationTime | ) |
Sets the animation time.
- Since :
- 2.4
- Parameters:
-
[in] animationTime The animation time in seconds
void Dali::Toolkit::Button::SetAutoRepeating | ( | bool | autoRepeating | ) |
Sets the autorepeating property.
If the autorepeating property is set to true, then the togglable property is set to false but no signal is emitted.
- Since :
- 2.4
- Parameters:
-
[in] autoRepeating autorepeating property.
void Dali::Toolkit::Button::SetBackgroundImage | ( | const std::string & | filename | ) |
Sets the background image.
- Since :
- 2.4
- Parameters:
-
[in] filename The background image
void Dali::Toolkit::Button::SetButtonImage | ( | Image | image | ) |
Sets the button image.
- Since :
- 2.4
- Remarks:
- Avoid using this method as it's a legacy code. Instead, use SetUnselectedImage.
- Parameters:
-
[in] image The button image
void Dali::Toolkit::Button::SetDisabled | ( | bool | disabled | ) |
Sets the button as disabled.
No signals are emitted when the disabled property is set.
- Since :
- 2.4
- Parameters:
-
[in] disabled property
void Dali::Toolkit::Button::SetDisabledBackgroundImage | ( | const std::string & | filename | ) |
Sets the disabled background image.
- Since :
- 2.4
- Parameters:
-
[in] filename The disabled background image
void Dali::Toolkit::Button::SetDisabledImage | ( | const std::string & | filename | ) |
Sets the disabled button image.
- Since :
- 2.4
- Parameters:
-
[in] filename The disabled button image
void Dali::Toolkit::Button::SetDisabledSelectedImage | ( | const std::string & | filename | ) |
Sets the disabled selected button image.
- Since :
- 2.4
- Parameters:
-
[in] filename The disabled selected button image
void Dali::Toolkit::Button::SetInitialAutoRepeatingDelay | ( | float | initialAutoRepeatingDelay | ) |
Sets the initial autorepeating delay.
By default this value is set to 0.15 seconds.
- Since :
- 2.4
- Parameters:
-
[in] initialAutoRepeatingDelay in seconds.
- Precondition:
- initialAutoRepeatingDelay must be greater than zero.
void Dali::Toolkit::Button::SetLabel | ( | Actor | label | ) |
Sets the label with an actor.
- Since :
- 2.4
- Remarks:
- Avoid using this method as it's a legacy code. Instead, use SetLabelText.
- Parameters:
-
[in] label The actor to use as a label
void Dali::Toolkit::Button::SetLabelText | ( | const std::string & | label | ) |
Sets the button's label.
- Since :
- 2.4
- Parameters:
-
[in] label The label text
void Dali::Toolkit::Button::SetNextAutoRepeatingDelay | ( | float | nextAutoRepeatingDelay | ) |
Sets the next autorepeating delay.
By default this value is set to 0.05 seconds.
- Since :
- 2.4
- Parameters:
-
[in] nextAutoRepeatingDelay in seconds.
- Precondition:
- nextAutoRepeatingDelay must be greater than zero.
void Dali::Toolkit::Button::SetSelected | ( | bool | selected | ) |
Sets the button as selected or unselected.
togglable property must be set to true.
Emits a Button::StateChangedSignal() signal.
- Since :
- 2.4
- Parameters:
-
[in] selected property
void Dali::Toolkit::Button::SetSelectedBackgroundImage | ( | const std::string & | filename | ) |
Sets the selected background image.
- Since :
- 2.4
- Parameters:
-
[in] filename The selected background image
void Dali::Toolkit::Button::SetSelectedImage | ( | const std::string & | filename | ) |
Sets the selected image.
- Since :
- 2.4
- Parameters:
-
[in] filename The selected image
void Dali::Toolkit::Button::SetSelectedImage | ( | Image | image | ) |
Sets the selected image.
- Since :
- 2.4
- Remarks:
- Avoid using this method as it's a legacy code. Instead, use SetSelectedImage( const std::string& filename ).
- Parameters:
-
[in] image The selected image
void Dali::Toolkit::Button::SetTogglableButton | ( | bool | togglable | ) |
Sets the togglable property.
If the togglable property is set to true, then the autorepeating property is set to false.
- Since :
- 2.4
- Parameters:
-
[in] togglable property
void Dali::Toolkit::Button::SetUnselectedImage | ( | const std::string & | filename | ) |
Sets the unselected button image.
- Since :
- 2.4
- Parameters:
-
[in] filename The button image
This signal is emitted when the button's state is changed.
The application can get the state by calling IsSelected().
A callback of the following type may be connected:
bool YourCallbackName( Button button );
- Since :
- 2.4
- Returns:
- The signal to connect to