Tizen Native API
|
TableView is a layout container for aligning child actors in a grid like layout. More...
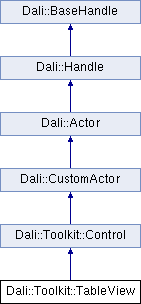
Classes | |
struct | CellPosition |
Structure to specify layout position for child actor. More... | |
struct | Property |
An enumeration of properties belonging to the TableView class. More... | |
Public Types | |
enum | PropertyRange |
The start and end property ranges for this control. More... | |
enum | LayoutPolicy |
Describes how the size of a row / column been set. More... | |
Public Member Functions | |
TableView () | |
Create a TableView handle; this can be initialised with TableView::New() Calling member functions with an uninitialised handle is not allowed. | |
TableView (const TableView &handle) | |
Copy constructor. Creates another handle that points to the same real object. | |
TableView & | operator= (const TableView &handle) |
Assignment operator. Changes this handle to point to another real object. | |
~TableView () | |
Destructor. | |
bool | AddChild (Actor child, CellPosition position) |
Adds a child to the table If the row or column index is outside the table, the table gets resized bigger. | |
Actor | GetChildAt (CellPosition position) |
Returns a child from the given layout position. | |
Actor | RemoveChildAt (CellPosition position) |
Removes a child from the given layout position. | |
bool | FindChildPosition (Actor child, CellPosition &position) |
Finds the childs layout position. | |
void | InsertRow (unsigned int rowIndex) |
Insert a new row to given index. | |
void | DeleteRow (unsigned int rowIndex) |
Delete a row from given index Removed elements are deleted. | |
void | DeleteRow (unsigned int rowIndex, std::vector< Actor > &removed) |
Delete a row from given index. | |
void | InsertColumn (unsigned int columnIndex) |
Insert a new column to given index. | |
void | DeleteColumn (unsigned int columnIndex) |
Delete a column from given index. Removed elements are deleted. | |
void | DeleteColumn (unsigned int columnIndex, std::vector< Actor > &removed) |
Delete a column from given index. | |
void | Resize (unsigned int rows, unsigned int columns) |
Resize the TableView. | |
void | Resize (unsigned int rows, unsigned int columns, std::vector< Actor > &removed) |
Resize the TableView. | |
void | SetCellPadding (Size padding) |
Set horizontal and vertical padding between cells. | |
Size | GetCellPadding () |
Get the current padding as width and height. | |
void | SetFitHeight (unsigned int rowIndex) |
Specify this row as fitting its height to its children. | |
bool | IsFitHeight (unsigned int rowIndex) const |
Is the row a fit row. | |
void | SetFitWidth (unsigned int columnIndex) |
Specify this column as fitting its width to its children. | |
bool | IsFitWidth (unsigned int columnIndex) const |
Is the column a fit column. | |
void | SetFixedHeight (unsigned int rowIndex, float height) |
Sets a row to have fixed height Setting a fixed height of 0 has no effect. | |
float | GetFixedHeight (unsigned int rowIndex) const |
Gets a row's fixed height. | |
void | SetRelativeHeight (unsigned int rowIndex, float heightPercentage) |
Sets a row to have relative height. Relative height means percentage of the remainder of the table height after subtracting Padding and Fixed height rows Setting a relative height of 0 has no effect. | |
float | GetRelativeHeight (unsigned int rowIndex) const |
Gets a row's relative height. | |
void | SetFixedWidth (unsigned int columnIndex, float width) |
Sets a column to have fixed width Setting a fixed width of 0 has no effect. | |
float | GetFixedWidth (unsigned int columnIndex) const |
Gets a column's fixed width. | |
void | SetRelativeWidth (unsigned int columnIndex, float widthPercentage) |
Sets a column to have relative width. Relative width means percentage of the remainder of table width after subtracting Padding and Fixed width columns Setting a relative width of 0 has no effect. | |
float | GetRelativeWidth (unsigned int columnIndex) const |
Gets a column's relative width. | |
unsigned int | GetRows () |
Gets the amount of rows in the table. | |
unsigned int | GetColumns () |
Gets the amount of columns in the table. | |
void | SetCellAlignment (CellPosition position, HorizontalAlignment::Type horizontal, VerticalAlignment::Type vertical) |
Set the alignment on a cell. | |
Static Public Member Functions | |
static TableView | New (unsigned int initialRows, unsigned int initialColumns) |
Create the TableView control. | |
static TableView | DownCast (BaseHandle handle) |
Downcast a handle to TableView handle. |
Detailed Description
TableView is a layout container for aligning child actors in a grid like layout.
TableView constrains the x and y position and width and height of the child actors. z position and depth are left intact so that 3D model actors can also be laid out in a grid without loosing their depth scaling.
Per-child Custom properties for script supporting:
When an actor is add to the tableView through Actor::Add() instead of TableView::AddChild, the following custom properties of the actor are checked to decide the actor position inside the table.
These properties are registered dynamically to the child and is non-animatable.
- Since :
- 2.4
Member Enumeration Documentation
Describes how the size of a row / column been set.
- Since :
- 2.4
- Enumerator:
The start and end property ranges for this control.
- Since :
- 2.4
Reimplemented from Dali::Toolkit::Control.
Constructor & Destructor Documentation
Create a TableView handle; this can be initialised with TableView::New() Calling member functions with an uninitialised handle is not allowed.
- Since :
- 2.4
Dali::Toolkit::TableView::TableView | ( | const TableView & | handle | ) |
Copy constructor. Creates another handle that points to the same real object.
- Since :
- 2.4
- Parameters:
-
handle to copy from
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since :
- 2.4
Member Function Documentation
bool Dali::Toolkit::TableView::AddChild | ( | Actor | child, |
CellPosition | position | ||
) |
Adds a child to the table If the row or column index is outside the table, the table gets resized bigger.
- Since :
- 2.4
- Parameters:
-
[in] child to add [in] position for the child
- Returns:
- true if the addition succeeded, false if the cell is already occupied
- Precondition:
- The child actor has been initialized.
void Dali::Toolkit::TableView::DeleteColumn | ( | unsigned int | columnIndex | ) |
Delete a column from given index. Removed elements are deleted.
- Since :
- 2.4
- Parameters:
-
[in] columnIndex of the column to delete
void Dali::Toolkit::TableView::DeleteColumn | ( | unsigned int | columnIndex, |
std::vector< Actor > & | removed | ||
) |
Delete a column from given index.
- Since :
- 2.4
- Parameters:
-
[in] columnIndex of the column to delete [out] removed elements
void Dali::Toolkit::TableView::DeleteRow | ( | unsigned int | rowIndex | ) |
Delete a row from given index Removed elements are deleted.
- Since :
- 2.4
- Parameters:
-
[in] rowIndex of the row to delete
void Dali::Toolkit::TableView::DeleteRow | ( | unsigned int | rowIndex, |
std::vector< Actor > & | removed | ||
) |
Delete a row from given index.
- Since :
- 2.4
- Parameters:
-
[in] rowIndex of the row to delete [out] removed elements
static TableView Dali::Toolkit::TableView::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcast a handle to TableView handle.
If handle points to a TableView the downcast produces valid handle. If not the returned handle is left uninitialized.
- Since :
- 2.4
- Parameters:
-
[in] handle Handle to an object
- Returns:
- handle to a TableView or an uninitialized handle
Reimplemented from Dali::Toolkit::Control.
bool Dali::Toolkit::TableView::FindChildPosition | ( | Actor | child, |
CellPosition & | position | ||
) |
Finds the childs layout position.
- Since :
- 2.4
- Parameters:
-
[in] child to search for [out] position for the child
- Returns:
- true if the child was included in this TableView
Get the current padding as width and height.
- Since :
- 2.4
- Returns:
- the current padding as width and height
Actor Dali::Toolkit::TableView::GetChildAt | ( | CellPosition | position | ) |
Returns a child from the given layout position.
- Since :
- 2.4
- Parameters:
-
[in] position in the table
- Returns:
- child that was in the cell or an uninitialized handle
- Note:
- if there is no child in this position this method returns an uninitialized Actor handle
unsigned int Dali::Toolkit::TableView::GetColumns | ( | ) |
Gets the amount of columns in the table.
- Since :
- 2.4
- Returns:
- the amount of columns in the table
float Dali::Toolkit::TableView::GetFixedHeight | ( | unsigned int | rowIndex | ) | const |
Gets a row's fixed height.
- Since :
- 2.4
- Returns:
- height in world coordinate units.
- Precondition:
- The row rowIndex must exist.
- Note:
- The returned value is valid if it has been set before.
float Dali::Toolkit::TableView::GetFixedWidth | ( | unsigned int | columnIndex | ) | const |
Gets a column's fixed width.
- Since :
- 2.4
- Returns:
- width in world coordinate units.
- Precondition:
- The column columnIndex must exist.
- Note:
- The returned value is valid if it has been set before.
float Dali::Toolkit::TableView::GetRelativeHeight | ( | unsigned int | rowIndex | ) | const |
Gets a row's relative height.
- Since :
- 2.4
- Returns:
- height in percentage units, between 0.0f and 1.0f.
- Precondition:
- The row rowIndex must exist.
- Note:
- The returned value is valid if it has been set before.
float Dali::Toolkit::TableView::GetRelativeWidth | ( | unsigned int | columnIndex | ) | const |
Gets a column's relative width.
- Since :
- 2.4
- Returns:
- width in percentage units, between 0.0f and 1.0f.
- Precondition:
- The column columnIndex must exist.
- Note:
- The returned value is valid if it has been set before.
unsigned int Dali::Toolkit::TableView::GetRows | ( | ) |
Gets the amount of rows in the table.
- Since :
- 2.4
- Returns:
- the amount of rows in the table
void Dali::Toolkit::TableView::InsertColumn | ( | unsigned int | columnIndex | ) |
Insert a new column to given index.
- Since :
- 2.4
- Parameters:
-
[in] columnIndex of the new column
void Dali::Toolkit::TableView::InsertRow | ( | unsigned int | rowIndex | ) |
Insert a new row to given index.
- Since :
- 2.4
- Parameters:
-
[in] rowIndex of the new row
bool Dali::Toolkit::TableView::IsFitHeight | ( | unsigned int | rowIndex | ) | const |
Is the row a fit row.
- Since :
- 2.4
- Parameters:
-
[in] rowIndex The row to check
- Returns:
- Return true if the row is fit
bool Dali::Toolkit::TableView::IsFitWidth | ( | unsigned int | columnIndex | ) | const |
Is the column a fit column.
- Since :
- 2.4
- Parameters:
-
[in] columnIndex The column to check
- Returns:
- Return true if the column is fit
static TableView Dali::Toolkit::TableView::New | ( | unsigned int | initialRows, |
unsigned int | initialColumns | ||
) | [static] |
Assignment operator. Changes this handle to point to another real object.
- Since :
- 2.4
Actor Dali::Toolkit::TableView::RemoveChildAt | ( | CellPosition | position | ) |
Removes a child from the given layout position.
- Since :
- 2.4
- Parameters:
-
[in] position for the child to remove
- Returns:
- child that was removed or an uninitialized handle
- Note:
- if there is no child in this position this method does nothing
void Dali::Toolkit::TableView::Resize | ( | unsigned int | rows, |
unsigned int | columns | ||
) |
Resize the TableView.
- Since :
- 2.4
- Parameters:
-
[in] rows for the table [in] columns for the table
- Note:
- if the new size is smaller than old, superfluous actors get removed. If you want to relayout removed children, use the variant that returns the removed Actors and reinsert them into the table If an actor spans to a removed row or column it gets removed from the table
void Dali::Toolkit::TableView::Resize | ( | unsigned int | rows, |
unsigned int | columns, | ||
std::vector< Actor > & | removed | ||
) |
Resize the TableView.
- Since :
- 2.4
- Parameters:
-
[in] rows for the table [in] columns for the table [out] removed actor handles
- Note:
- if the new size is smaller than old, superfluous actors get removed. If an actor spans to a removed row or column it gets removed from the table
void Dali::Toolkit::TableView::SetCellAlignment | ( | CellPosition | position, |
HorizontalAlignment::Type | horizontal, | ||
VerticalAlignment::Type | vertical | ||
) |
Set the alignment on a cell.
Cells without calling this function have the default values of LEFT and TOP respectively.
- Since :
- 2.4
- Parameters:
-
[in] position The cell to set alignment on. [in] horizontal The horizontal alignment. [in] vertical The vertical alignment.
void Dali::Toolkit::TableView::SetCellPadding | ( | Size | padding | ) |
Set horizontal and vertical padding between cells.
- Since :
- 2.4
- Parameters:
-
[in] padding width and height
void Dali::Toolkit::TableView::SetFitHeight | ( | unsigned int | rowIndex | ) |
Specify this row as fitting its height to its children.
- Since :
- 2.4
- Parameters:
-
[in] rowIndex The row to set
void Dali::Toolkit::TableView::SetFitWidth | ( | unsigned int | columnIndex | ) |
Specify this column as fitting its width to its children.
- Since :
- 2.4
- Parameters:
-
[in] columnIndex The column to set
void Dali::Toolkit::TableView::SetFixedHeight | ( | unsigned int | rowIndex, |
float | height | ||
) |
Sets a row to have fixed height Setting a fixed height of 0 has no effect.
- Since :
- 2.4
- Parameters:
-
rowIndex for row with fixed height height in world coordinate units
- Precondition:
- The row rowIndex must exist.
void Dali::Toolkit::TableView::SetFixedWidth | ( | unsigned int | columnIndex, |
float | width | ||
) |
Sets a column to have fixed width Setting a fixed width of 0 has no effect.
- Since :
- 2.4
- Parameters:
-
columnIndex for column with fixed width width in world coordinate units
- Precondition:
- The column columnIndex must exist.
void Dali::Toolkit::TableView::SetRelativeHeight | ( | unsigned int | rowIndex, |
float | heightPercentage | ||
) |
Sets a row to have relative height. Relative height means percentage of the remainder of the table height after subtracting Padding and Fixed height rows Setting a relative height of 0 has no effect.
- Since :
- 2.4
- Parameters:
-
rowIndex for row with relative height heightPercentage between 0.0f and 1.0f
- Precondition:
- The row rowIndex must exist.
void Dali::Toolkit::TableView::SetRelativeWidth | ( | unsigned int | columnIndex, |
float | widthPercentage | ||
) |
Sets a column to have relative width. Relative width means percentage of the remainder of table width after subtracting Padding and Fixed width columns Setting a relative width of 0 has no effect.
- Since :
- 2.4
- Parameters:
-
columnIndex for column with fixed width widthPercentage between 0.0f and 1.0f
- Precondition:
- The column columnIndex must exist.