Tizen Native API
3.0
|
A 3D parametric curve. More...
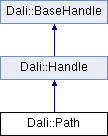
Classes | |
struct | Property |
Enumeration for the instance of properties belonging to the Path class. More... | |
Public Member Functions | |
Path () | |
Creates an uninitialized Path handle. | |
~Path () | |
Destructor. | |
Path (const Path &handle) | |
This copy constructor is required for (smart) pointer semantics. | |
Path & | operator= (const Path &rhs) |
This assignment operator is required for (smart) pointer semantics. | |
void | AddPoint (const Vector3 &point) |
Adds an interpolation point. | |
void | AddControlPoint (const Vector3 &point) |
Adds a control point. | |
void | GenerateControlPoints (float curvature) |
Automatic generation of control points. Generated control points which result in a smooth join between the splines of each segment. | |
void | Sample (float progress, Vector3 &position, Vector3 &tangent) const |
Sample path at a given progress. Calculates position and tangent at that point of the curve. | |
Vector3 & | GetPoint (size_t index) |
Accessor for the interpolation points. | |
Vector3 & | GetControlPoint (size_t index) |
Accessor for the control points. | |
size_t | GetPointCount () const |
Gets the number of interpolation points in the path. | |
Static Public Member Functions | |
static Path | New () |
Creates an initialized Path handle. | |
static Path | DownCast (BaseHandle handle) |
Downcasts a handle to Path handle. |
Detailed Description
A 3D parametric curve.
Paths can be used to animate position and orientation of actors using Dali::Animate().
- Since:
- 2.4, DALi version 1.0.0
Constructor & Destructor Documentation
Dali::Path::Path | ( | ) |
Creates an uninitialized Path handle.
This can be initialized with Path::New(). Calling member functions with an uninitialized Path handle is not allowed.
- Since:
- 2.4, DALi version 1.0.0
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 2.4, DALi version 1.0.0
Dali::Path::Path | ( | const Path & | handle | ) |
This copy constructor is required for (smart) pointer semantics.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle A reference to the copied handle
Member Function Documentation
void Dali::Path::AddControlPoint | ( | const Vector3 & | point | ) |
Adds a control point.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] point The new control point to be added
void Dali::Path::AddPoint | ( | const Vector3 & | point | ) |
Adds an interpolation point.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] point The new interpolation point to be added
static Path Dali::Path::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcasts a handle to Path handle.
If handle points to a Path object, the downcast produces valid handle. If not, the returned handle is left uninitialized.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle Handle to an object
Reimplemented from Dali::Handle.
void Dali::Path::GenerateControlPoints | ( | float | curvature | ) |
Automatic generation of control points. Generated control points which result in a smooth join between the splines of each segment.
The generating algorithm is as follows: For a given knot point K[N], find the vector that bisects K[N-1],[N] and [N],[N+1]. Calculate the tangent vector by taking the normal of this bisector. The in control point is the length of the preceding segment back along this bisector multiplied by the curvature. The out control point is the length of the succeeding segment forward along this bisector multiplied by the curvature.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] curvature The curvature of the spline. 0 gives straight lines between the knots, negative values means the spline contains loops, positive values up to 0.5 result in a smooth curve, positive values between 0.5 and 1 result in looped curves where the loops are not distinct (i.e. the curve appears to be non-continuous), positive values higher than 1 result in looped curves
- Precondition:
- There are at least two points in the path ( one segment ).
Vector3& Dali::Path::GetControlPoint | ( | size_t | index | ) |
Accessor for the control points.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the control point
- Returns:
- A reference to the control point
Vector3& Dali::Path::GetPoint | ( | size_t | index | ) |
Accessor for the interpolation points.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the interpolation point
- Returns:
- A reference to the interpolation point
size_t Dali::Path::GetPointCount | ( | ) | const |
Gets the number of interpolation points in the path.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The number of interpolation points in the path
static Path Dali::Path::New | ( | ) | [static] |
Creates an initialized Path handle.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A handle to a newly allocated Dali resource
Reimplemented from Dali::Handle.
This assignment operator is required for (smart) pointer semantics.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] rhs A reference to the copied handle
- Returns:
- A reference to this
void Dali::Path::Sample | ( | float | progress, |
Vector3 & | position, | ||
Vector3 & | tangent | ||
) | const |
Sample path at a given progress. Calculates position and tangent at that point of the curve.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] progress A floating point value between 0.0 and 1.0 [out] position The interpolated position at that progress [out] tangent The interpolated tangent at that progress