Tizen Native API
5.5
|
An abstract base class for Constraints. More...
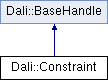
Classes | |
class | Function |
Template for the Function that is called by the Constraint system. More... | |
Public Types | |
enum | RemoveAction |
Enumeration for the action that will happen when the constraint is removed. More... | |
Public Member Functions | |
Constraint () | |
Creates an uninitialized Constraint; this can be initialized with Constraint::New(). | |
Constraint | Clone (Handle handle) |
Creates a clone of this constraint for another object. | |
~Constraint () | |
Destructor. | |
Constraint (const Constraint &constraint) | |
This copy constructor is required for (smart) pointer semantics. | |
Constraint & | operator= (const Constraint &rhs) |
This assignment operator is required for (smart) pointer semantics. | |
void | AddSource (ConstraintSource source) |
Adds a constraint source to the constraint. | |
void | Apply () |
Applies this constraint. | |
void | Remove () |
Removes this constraint. | |
Handle | GetTargetObject () |
Retrieves the object which this constraint is targeting. | |
Dali::Property::Index | GetTargetProperty () |
Retrieves the property which this constraint is targeting. | |
void | SetRemoveAction (RemoveAction action) |
Sets the remove action. Constraint::Bake will "bake" a value when fully-applied. | |
RemoveAction | GetRemoveAction () const |
Retrieves the remove action that will happen when the constraint is removed. | |
void | SetTag (const uint32_t tag) |
Sets a tag for the constraint so it can be identified later. | |
uint32_t | GetTag () const |
Gets the tag. | |
Static Public Member Functions | |
template<class P > | |
static Constraint | New (Handle handle, Property::Index targetIndex, void(*function)(P &, const PropertyInputContainer &)) |
Creates a constraint which targets a property using a function or a static class member. | |
template<class P , class T > | |
static Constraint | New (Handle handle, Property::Index targetIndex, const T &object) |
Creates a constraint which targets a property using a functor object. | |
template<class P , class T > | |
static Constraint | New (Handle handle, Property::Index targetIndex, const T &object, void(T::*memberFunction)(P &, const PropertyInputContainer &)) |
Creates a constraint which targets a property using an object method. | |
static Constraint | DownCast (BaseHandle baseHandle) |
Downcasts a handle to Constraint handle. | |
Static Public Attributes | |
static const RemoveAction | DEFAULT_REMOVE_ACTION |
Bake. |
Detailed Description
An abstract base class for Constraints.
This can be used to constrain a property of an object, after animations have been applied. Constraints are applied in the following order:
- Constraints are applied to on-stage actors in a depth-first traversal.
- For each actor, the constraints are applied in the same order as the calls to Apply().
- Constraints are not applied to off-stage actors.
Create a constraint using one of the New methods depending on the type of callback function used. Try to use a C function unless some data needs to be stored, otherwise functors and class methods are also supported.
A constraint can be applied to an object in the following manner:
Handle handle = CreateMyObject(); Constraint constraint = Constraint::New< Vector3 >( handle, CONSTRAINING_PROPERTY_INDEX, &MyFunction ); constraint.AddSource( LocalSource( INPUT_PROPERTY_INDEX ) ); constraint.Apply();
- Since:
- 3.0, DALi version 1.0.0
Member Enumeration Documentation
Enumeration for the action that will happen when the constraint is removed.
The final value may be "baked" i.e. saved permanently. Alternatively the constrained value may be discarded when the constraint is removed.
- Since:
- 3.0, DALi version 1.0.0
Constructor & Destructor Documentation
Creates an uninitialized Constraint; this can be initialized with Constraint::New().
Calling member functions with an uninitialized Constraint handle is not allowed.
- Since:
- 3.0, DALi version 1.0.0
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 3.0, DALi version 1.0.0
Dali::Constraint::Constraint | ( | const Constraint & | constraint | ) |
This copy constructor is required for (smart) pointer semantics.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] constraint A reference to the copied handle
Member Function Documentation
void Dali::Constraint::AddSource | ( | ConstraintSource | source | ) |
Adds a constraint source to the constraint.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] source The constraint source input to add
void Dali::Constraint::Apply | ( | ) |
Applies this constraint.
- Since:
- 3.0, DALi version 1.0.0
- Precondition:
- The constraint must be initialized.
- The target object must still be alive.
- The source inputs should not have been destroyed.
Constraint Dali::Constraint::Clone | ( | Handle | handle | ) |
Creates a clone of this constraint for another object.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] handle The handle to the property-owning object this constraint is to be cloned for
- Returns:
- The new constraint
static Constraint Dali::Constraint::DownCast | ( | BaseHandle | baseHandle | ) | [static] |
Downcasts a handle to Constraint handle.
If handle points to a Constraint object, the downcast produces valid handle. If not, the returned handle is left uninitialized.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] baseHandle BaseHandle to an object
- Returns:
- Handle to a Constraint object or an uninitialized handle
Retrieves the remove action that will happen when the constraint is removed.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The remove-action
uint32_t Dali::Constraint::GetTag | ( | ) | const |
Gets the tag.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The tag
Retrieves the object which this constraint is targeting.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The target object
Retrieves the property which this constraint is targeting.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The target property
static Constraint Dali::Constraint::New | ( | Handle | handle, |
Property::Index | targetIndex, | ||
void(*)(P &, const PropertyInputContainer &) | function | ||
) | [static] |
Creates a constraint which targets a property using a function or a static class member.
The expected signature, for a Vector3 type for example, of the function is:
void MyFunction( Vector3&, const PropertyInputContainer& );
Create the constraint with this function as follows:
Constraint constraint = Constraint::New< Vector3 >( handle, CONSTRAINING_PROPERTY_INDEX, &MyFunction );
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] handle The handle to the property-owning object [in] targetIndex The index of the property to constrain [in] function The function to call to set the constrained property value
- Template Parameters:
-
P The type of the property to constrain
- Returns:
- The new constraint
static Constraint Dali::Constraint::New | ( | Handle | handle, |
Property::Index | targetIndex, | ||
const T & | object | ||
) | [static] |
Creates a constraint which targets a property using a functor object.
The expected structure, for a Vector3 type for example, of the functor object is:
struct MyObject { void operator() ( Vector3&, const PropertyInputContainer& ); };
Create the constraint with this object as follows:
Constraint constraint = Constraint::New< Vector3 >( handle, CONSTRAINING_PROPERTY_INDEX, MyObject() );
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] handle The handle to the property-owning object [in] targetIndex The index of the property to constraint [in] object The functor object whose functor is called to set the constrained property value
- Template Parameters:
-
P The type of the property to constrain T The type of the object
- Returns:
- The new constraint
static Constraint Dali::Constraint::New | ( | Handle | handle, |
Property::Index | targetIndex, | ||
const T & | object, | ||
void(T::*)(P &, const PropertyInputContainer &) | memberFunction | ||
) | [static] |
Creates a constraint which targets a property using an object method.
The expected structure, for a Vector3 type for example, of the object is:
struct MyObject { void MyMethod( Vector3&, const PropertyInputContainer& ); };
Create the constraint with this object as follows:
Constraint constraint = Constraint::New< Vector3 >( handle, CONSTRAINING_PROPERTY_INDEX, MyObject(), &MyObject::MyMethod );
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] handle The handle to the property-owning object [in] targetIndex The index of the property to constraint [in] object The object whose member function is called to set the constrained property value [in] memberFunction The member function to call to set the constrained property value
- Returns:
- The new constraint
- Template Parameters:
-
P The type of the property to constrain T The type of the object
Constraint& Dali::Constraint::operator= | ( | const Constraint & | rhs | ) |
This assignment operator is required for (smart) pointer semantics.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] rhs A reference to the copied handle
- Returns:
- A reference to this
void Dali::Constraint::Remove | ( | ) |
Removes this constraint.
- Since:
- 3.0, DALi version 1.0.0
void Dali::Constraint::SetRemoveAction | ( | RemoveAction | action | ) |
Sets the remove action. Constraint::Bake will "bake" a value when fully-applied.
In case of Constraint::Discard, the constrained value will be discarded, when the constraint is removed. The default value is Constraint::Bake.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] action The remove-action
void Dali::Constraint::SetTag | ( | const uint32_t | tag | ) |
Sets a tag for the constraint so it can be identified later.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] tag An integer to identify the constraint