Tizen Native API
5.5
|
Layers provide a mechanism for overlaying groups of actors on top of each other. More...
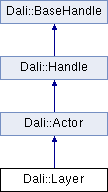
Classes | |
struct | Property |
Enumeration for the instance of properties belonging to the Layer class. More... | |
Public Types | |
enum | Behavior |
Enumeration for the behavior of the layer. More... | |
enum | TreeDepthMultiplier |
Enumeration for TREE_DEPTH_MULTIPLIER is used by the rendering sorting algorithm to decide which actors to render first. More... | |
typedef float(* | SortFunctionType )(const Vector3 &position) |
The sort function type. | |
Public Member Functions | |
Layer () | |
Creates an empty Layer handle. | |
~Layer () | |
Destructor. | |
Layer (const Layer ©) | |
Copy constructor. | |
Layer & | operator= (const Layer &rhs) |
Assignment operator. | |
uint32_t | GetDepth () const |
Queries the depth of the layer. | |
void | Raise () |
Increments the depth of the layer. | |
void | Lower () |
Decrements the depth of the layer. | |
void | RaiseAbove (Layer target) |
Ensures the layers depth is greater than the target layer. | |
void | LowerBelow (Layer target) |
Ensures the layers depth is less than the target layer. | |
void | RaiseToTop () |
Raises the layer to the top. | |
void | LowerToBottom () |
Lowers the layer to the bottom. | |
void | MoveAbove (Layer target) |
Moves the layer directly above the given layer. | |
void | MoveBelow (Layer target) |
Moves the layer directly below the given layer. | |
void | SetBehavior (Behavior behavior) |
Sets the behavior of the layer. | |
Behavior | GetBehavior () const |
Gets the behavior of the layer. | |
void | SetClipping (bool enabled) |
Sets whether clipping is enabled for a layer. | |
bool | IsClipping () const |
Queries whether clipping is enabled for a layer. | |
void | SetClippingBox (int32_t x, int32_t y, int32_t width, int32_t height) |
Sets the clipping box of a layer, in window coordinates. | |
void | SetClippingBox (ClippingBox box) |
Sets the clipping box of a layer in window coordinates. | |
ClippingBox | GetClippingBox () const |
Retrieves the clipping box of a layer in window coordinates. | |
void | SetDepthTestDisabled (bool disable) |
Whether to disable the depth test. | |
bool | IsDepthTestDisabled () const |
Retrieves whether depth test is disabled. | |
void | SetSortFunction (SortFunctionType function) |
This allows the user to specify the sort function that the layer should use. | |
void | SetTouchConsumed (bool consume) |
This allows the user to specify whether this layer should consume touch (including gestures). | |
bool | IsTouchConsumed () const |
Retrieves whether the layer consumes touch (including gestures). | |
void | SetHoverConsumed (bool consume) |
This allows the user to specify whether this layer should consume hover. | |
bool | IsHoverConsumed () const |
Retrieves whether the layer consumes hover. | |
Static Public Member Functions | |
static Layer | New () |
Creates a Layer object. | |
static Layer | DownCast (BaseHandle handle) |
Downcasts a handle to Layer handle. |
Detailed Description
Layers provide a mechanism for overlaying groups of actors on top of each other.
When added to the stage, a layer can be ordered relative to other layers. The bottom layer is at depth zero. The stage provides a default layer for it's children (see Stage::GetRootLayer()).
Layered actors inherit position etc. as normal, but are drawn in an order determined by the layers. In case of LAYER_3D, the depth buffer is cleared before each layer is rendered unless depth test is disabled or there's no need for it based on the layer's contents; actors in lower layers cannot obscure actors in higher layers.
A layer has either LAYER_UI or LAYER_3D mode. LAYER_UI has better performance, the depth test is disabled, and a child actor hides its parent actor. LAYER_3D uses the depth test, thus a close actor hides a farther one. LAYER_UI is the default mode and recommended for general cases. See Layer::Behavior and SetBehavior() for more information.
Layer is a type of Actor, thus can have parent or children actors. A layer influences rendering of its all descendant actors, until another layer appears in the actor tree and manages its own subtree.
If depth test is disabled, there is no performance overhead from clearing the depth buffer.
Actions | Action Name | Layer method called | |-----------------|----------------------| | raise | Raise() | | lower | Lower() | | raiseToTop | RaiseToTop() | | lowerToBottom | LowerToBottom() |
- Since:
- 3.0, DALi version 1.0.0
Member Typedef Documentation
typedef float(* Dali::Layer::SortFunctionType)(const Vector3 &position) |
The sort function type.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] position This is the actor translation from camera
Member Enumeration Documentation
Enumeration for the behavior of the layer.
Check each value to see how it affects the layer.
- Since:
- 3.0, DALi version 1.0.0
- Enumerator:
LAYER_UI UI control rendering mode (default mode).
This mode is designed for UI controls that can overlap. In this mode renderer order will be respective to the tree hierarchy of Actors.
The rendering order is depth first, so for the following actor tree, A will be drawn first, then B, D, E, then C, F. This ensures that overlapping actors are drawn as expected (whereas, with breadth first traversal, the actors would interleave).
Layer1 (parent) | A / \ B C / \ \ D E F
To change the order of sibling actors, use the Actor::Raise and Actor::Lower APIs. Within an actor, the Renderer depth index dictates the order the renderers are drawn.
- Since:
- 3.0, DALi version 1.1.45
LAYER_3D Layer will use depth test.
This mode is designed for a 3 dimensional scene where actors in front of other actors will obscure them, i.e. the actors are sorted by the distance from the camera.
When using this mode, a depth test will be used. A depth clear will happen for each layer, which means actors in a layer "above" other layers will be rendered in front of actors in those layers regardless of their Z positions (see Layer::Raise() and Layer::Lower()).
Opaque renderers are drawn first and write to the depth buffer. Then transparent renderers are drawn with depth test enabled but depth write switched off. Transparent renderers are drawn based on their distance from the camera. A renderer's DEPTH_INDEX property is used to offset the distance to the camera when ordering transparent renderers.
This is useful if you want to define the draw order of two or more transparent renderers that are equal distance from the camera. Unlike LAYER_UI, parent-child relationship does not affect rendering order at all.
- Since:
- 3.0, DALi version 1.0.0
Enumeration for TREE_DEPTH_MULTIPLIER is used by the rendering sorting algorithm to decide which actors to render first.
- Deprecated:
- Deprecated since 4.0, DALi version 1.2.26. Not intended for application use.
- Since:
- 3.0, DALi version 1.0.0
Constructor & Destructor Documentation
Creates an empty Layer handle.
This can be initialized with Layer::New(...).
- Since:
- 3.0, DALi version 1.0.0
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 3.0, DALi version 1.0.0
Dali::Layer::Layer | ( | const Layer & | copy | ) |
Copy constructor.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] copy The actor to copy
Member Function Documentation
static Layer Dali::Layer::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcasts a handle to Layer handle.
If handle points to a Layer, the downcast produces valid handle. If not, the returned handle is left uninitialized.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] handle Handle to an object
Reimplemented from Dali::Actor.
Behavior Dali::Layer::GetBehavior | ( | ) | const |
Gets the behavior of the layer.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The behavior of the layer
ClippingBox Dali::Layer::GetClippingBox | ( | ) | const |
Retrieves the clipping box of a layer in window coordinates.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The clipping box
uint32_t Dali::Layer::GetDepth | ( | ) | const |
Queries the depth of the layer.
0 is the bottom most layer, higher number is on top.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The current depth of the layer
- Precondition:
- Layer is on the stage. If layer is not added to the stage, returns 0.
bool Dali::Layer::IsClipping | ( | ) | const |
Queries whether clipping is enabled for a layer.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- True if clipping is enabled
bool Dali::Layer::IsDepthTestDisabled | ( | ) | const |
Retrieves whether depth test is disabled.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- True if depth test is disabled
bool Dali::Layer::IsHoverConsumed | ( | ) | const |
Retrieves whether the layer consumes hover.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
True
if consuming hover,false
otherwise
bool Dali::Layer::IsTouchConsumed | ( | ) | const |
Retrieves whether the layer consumes touch (including gestures).
- Since:
- 3.0, DALi version 1.0.0
- Returns:
True
if consuming touch,false
otherwise
void Dali::Layer::Lower | ( | ) |
Decrements the depth of the layer.
- Since:
- 3.0, DALi version 1.0.0
- Precondition:
- Layer is on the stage.
Reimplemented from Dali::Actor.
void Dali::Layer::LowerBelow | ( | Layer | target | ) |
Ensures the layers depth is less than the target layer.
If the layer already is below the target layer, its depth is not changed. If the layer was above target, its new depth will be immediately below target.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
target Layer to get below of
- Precondition:
- Layer is on the stage.
- Target layer is on the stage.
- Note:
- All layers between this layer and target get new depth values.
void Dali::Layer::LowerToBottom | ( | ) |
Lowers the layer to the bottom.
- Since:
- 3.0, DALi version 1.0.0
- Precondition:
- layer is on the stage.
Reimplemented from Dali::Actor.
void Dali::Layer::MoveAbove | ( | Layer | target | ) |
Moves the layer directly above the given layer.
After the call, this layers depth will be immediately above target.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
target Layer to get on top of
- Precondition:
- Layer is on the stage.
- Target layer is on the stage.
- Note:
- All layers between this layer and target get new depth values.
void Dali::Layer::MoveBelow | ( | Layer | target | ) |
Moves the layer directly below the given layer.
After the call, this layers depth will be immediately below target.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
target Layer to get below of
- Precondition:
- Layer is on the stage.
- Target layer is on the stage.
- Note:
- All layers between this layer and target get new depth values.
static Layer Dali::Layer::New | ( | ) | [static] |
Creates a Layer object.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- A handle to a newly allocated Layer
Reimplemented from Dali::Actor.
Assignment operator.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] rhs The actor to copy
- Returns:
- A reference to this
void Dali::Layer::Raise | ( | ) |
Increments the depth of the layer.
- Since:
- 3.0, DALi version 1.0.0
- Precondition:
- Layer is on the stage.
Reimplemented from Dali::Actor.
void Dali::Layer::RaiseAbove | ( | Layer | target | ) |
Ensures the layers depth is greater than the target layer.
If the layer already is above the target layer, its depth is not changed. If the layer was below target, its new depth will be immediately above target.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
target Layer to get above of
- Precondition:
- Layer is on the stage.
- Target layer is on the stage.
- Note:
- All layers between this layer and target get new depth values.
void Dali::Layer::RaiseToTop | ( | ) |
Raises the layer to the top.
- Since:
- 3.0, DALi version 1.0.0
- Precondition:
- Layer is on the stage.
Reimplemented from Dali::Actor.
void Dali::Layer::SetBehavior | ( | Behavior | behavior | ) |
Sets the behavior of the layer.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] behavior The desired behavior
void Dali::Layer::SetClipping | ( | bool | enabled | ) |
Sets whether clipping is enabled for a layer.
Clipping is initially disabled; see also SetClippingBox().
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] enabled True if clipping is enabled
- Note:
- When clipping is enabled, the default clipping box is empty (0,0,0,0), which means everything is clipped.
void Dali::Layer::SetClippingBox | ( | int32_t | x, |
int32_t | y, | ||
int32_t | width, | ||
int32_t | height | ||
) |
Sets the clipping box of a layer, in window coordinates.
The contents of the layer will not be visible outside this box, when clipping is enabled. The default clipping box is empty (0,0,0,0) which means everything is clipped. You can only do rectangular clipping using this API in window coordinates.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] x The X-coordinate of the top-left corner of the box [in] y The Y-coordinate of the top-left corner of the box [in] width The width of the box [in] height The height of the box
void Dali::Layer::SetClippingBox | ( | ClippingBox | box | ) |
Sets the clipping box of a layer in window coordinates.
The contents of the layer will not be visible outside this box when clipping is enabled. The default clipping box is empty (0,0,0,0).
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] box The clipping box
void Dali::Layer::SetDepthTestDisabled | ( | bool | disable | ) |
Whether to disable the depth test.
By default a layer enables depth test if there is more than one opaque actor or if there is one opaque actor and one, or more, transparent actors in LAYER_3D mode. However, it's possible to disable the depth test by calling this method.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] disable True disables depth test. false sets the default behavior
void Dali::Layer::SetHoverConsumed | ( | bool | consume | ) |
This allows the user to specify whether this layer should consume hover.
If set, any layers behind this layer will not be hit-test.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] consume Whether the layer should consume hover
void Dali::Layer::SetSortFunction | ( | SortFunctionType | function | ) |
This allows the user to specify the sort function that the layer should use.
The sort function is used to determine the order in which the actors are drawn and input is processed on the actors in the layer.
A function of the following type should be used:
float YourSortFunction(const Vector3& position);
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] function The sort function pointer
- Note:
- If the sort function returns a low number, the actor with the data will be drawn in front of an actor whose data yields a high value from the sort function.
- All child layers use the same sort function. If a child layer is added to this layer, then the sort function used by the child layer will also be the same.
void Dali::Layer::SetTouchConsumed | ( | bool | consume | ) |
This allows the user to specify whether this layer should consume touch (including gestures).
If set, any layers behind this layer will not be hit-test.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] consume Whether the layer should consume touch (including gestures)