Tizen Native API
5.5
|
This class looks for panning (or dragging) gestures. More...
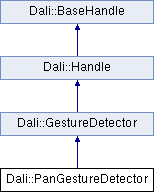
Classes | |
struct | Property |
Enumeration for the instance of properties belonging to the PanGestureDetector class. More... | |
Public Types | |
typedef Signal< void(Actor, const PanGesture &) > | DetectedSignalType |
Pan gesture detected signal type. | |
typedef std::pair< Radian, Radian > | AngleThresholdPair |
Range of angles for a direction. | |
Public Member Functions | |
PanGestureDetector () | |
Creates an uninitialized PanGestureDetector; this can be initialized with PanGestureDetector::New(). | |
~PanGestureDetector () | |
Destructor. | |
PanGestureDetector (const PanGestureDetector &handle) | |
This copy constructor is required for (smart) pointer semantics. | |
PanGestureDetector & | operator= (const PanGestureDetector &rhs) |
This assignment operator is required for (smart) pointer semantics. | |
void | SetMinimumTouchesRequired (uint32_t minimum) |
This is the minimum number of touches required for the pan gesture to be detected. | |
void | SetMaximumTouchesRequired (uint32_t maximum) |
This is the maximum number of touches required for the pan gesture to be detected. | |
uint32_t | GetMinimumTouchesRequired () const |
Retrieves the minimum number of touches required for the pan gesture to be detected. | |
uint32_t | GetMaximumTouchesRequired () const |
Retrieves the maximum number of touches required for the pan gesture to be detected. | |
void | AddAngle (Radian angle, Radian threshold=DEFAULT_THRESHOLD) |
The pan gesture is only emitted if the pan occurs in the direction specified by this method with a +/- threshold allowance. | |
void | AddDirection (Radian direction, Radian threshold=DEFAULT_THRESHOLD) |
A helper method for adding bi-directional angles where the pan should take place. | |
size_t | GetAngleCount () const |
Returns the count of angles that this pan gesture detector emits a signal. | |
AngleThresholdPair | GetAngle (size_t index) const |
Returns the angle by index that this pan gesture detector emits a signal. | |
void | ClearAngles () |
Clears any directional angles that are used by the gesture detector. | |
void | RemoveAngle (Radian angle) |
Removes the angle specified from the container. | |
void | RemoveDirection (Radian direction) |
Removes the two angles that make up the direction from the container. | |
DetectedSignalType & | DetectedSignal () |
This signal is emitted when the pan gesture is detected on the attached actor. | |
Static Public Member Functions | |
static PanGestureDetector | New () |
Creates an initialized PanGestureDetector. | |
static PanGestureDetector | DownCast (BaseHandle handle) |
Downcasts a handle to PanGestureDetector handle. | |
static void | SetPanGestureProperties (const PanGesture &pan) |
Allows setting of the pan properties that are returned in constraints. | |
Static Public Attributes | |
static const Radian | DIRECTION_LEFT |
For a left pan (-PI Radians). | |
static const Radian | DIRECTION_RIGHT |
For a right pan (0 Radians). | |
static const Radian | DIRECTION_UP |
For an up pan (-0.5 * PI Radians). | |
static const Radian | DIRECTION_DOWN |
For a down pan (0.5 * PI Radians). | |
static const Radian | DIRECTION_HORIZONTAL |
For a left and right pan (PI Radians). Useful for AddDirection(). | |
static const Radian | DIRECTION_VERTICAL |
For an up and down pan (-0.5 * PI Radians). Useful for AddDirection(). | |
static const Radian | DEFAULT_THRESHOLD |
The default threshold is PI * 0.25 radians (or 45 degrees). |
Detailed Description
This class looks for panning (or dragging) gestures.
The user will be pressing one or more fingers on an actor while they pan it.
The application programmer can use this gesture detector as follows:
PanGestureDetector detector = PanGestureDetector::New(); detector.Attach(myActor); detector.DetectedSignal().Connect(this, &MyApplication::OnPan); // Detect pan gesture for single and double touch. detector.SetMaximumTouchesRequired(2);
- Since:
- 3.0, DALi version 1.0.0
- See also:
- PanGesture
Signals | Signal Name | Method | |--------------|-----------------------| | panDetected | DetectedSignal() |
Member Typedef Documentation
typedef std::pair< Radian, Radian > Dali::PanGestureDetector::AngleThresholdPair |
Range of angles for a direction.
- Since:
- 3.0, DALi version 1.0.0
typedef Signal< void ( Actor, const PanGesture& ) > Dali::PanGestureDetector::DetectedSignalType |
Pan gesture detected signal type.
- Since:
- 3.0, DALi version 1.0.0
Constructor & Destructor Documentation
Creates an uninitialized PanGestureDetector; this can be initialized with PanGestureDetector::New().
Calling member functions with an uninitialized PanGestureDetector handle is not allowed.
- Since:
- 3.0, DALi version 1.0.0
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 3.0, DALi version 1.0.0
Dali::PanGestureDetector::PanGestureDetector | ( | const PanGestureDetector & | handle | ) |
This copy constructor is required for (smart) pointer semantics.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] handle A reference to the copied handle
Member Function Documentation
void Dali::PanGestureDetector::AddAngle | ( | Radian | angle, |
Radian | threshold = DEFAULT_THRESHOLD |
||
) |
The pan gesture is only emitted if the pan occurs in the direction specified by this method with a +/- threshold allowance.
The angle is from -180 -> 0 -> 180 degrees (or -M_PI -> 0 -> M_PI in radians) i.e:
-90.0f ( -0.5f * PI ) | | 180.0f ( PI ) ------------- 0.0f ( 0.0f ) | | 90.0f ( 0.5f * PI )
If an angle of 0.0 degrees is specified and the threshold is 45 degrees then the acceptable direction range is from -45 to 45 degrees.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] angle The angle that pan should be allowed [in] threshold The threshold around that angle
- Precondition:
- The gesture detector has been initialized.
- Note:
- The angle added using this API is only checked when the gesture first starts, after that, this detector will emit the gesture regardless of what angle the pan is moving.
- The user can add as many angles as they require.
- If an angle outside the range above is given, then it is wrapped within the range, i.e. 190 degrees becomes -170 degrees and 370 degrees becomes 10 degrees.
- As long as you specify the type, you can also pass in a Dali::Degree to this method.
- If no threshold is provided, then the default threshold (PI * 0.25) is used.
- If the threshold is greater than PI, then PI will be used as the threshold.
void Dali::PanGestureDetector::AddDirection | ( | Radian | direction, |
Radian | threshold = DEFAULT_THRESHOLD |
||
) |
A helper method for adding bi-directional angles where the pan should take place.
In other words, if 0 is requested, then PI will also be added so that we have both left and right scrolling.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] direction The direction of panning required [in] threshold The threshold
- Precondition:
- The gesture detector has been initialized.
- Note:
- If a direction outside the range above is given, then it is wrapped within the range, i.e. 190 degrees becomes -170 degrees and 370 degrees becomes 10 degrees.
- If no threshold is provided, then the default threshold (PI * 0.25) is used.
- If the threshold is greater than PI, then PI will be used as the threshold.
- As long as you specify the type, you can also pass in a Dali::Degree to this method.
- See also:
- AddAngle
Clears any directional angles that are used by the gesture detector.
After this, the pan gesture will be emitted for a pan in ANY direction.
- Since:
- 3.0, DALi version 1.0.0
- Precondition:
- The gesture detector has been initialized.
This signal is emitted when the pan gesture is detected on the attached actor.
A callback of the following type may be connected:
void YourCallbackName( Actor actor, const PanGesture& gesture );
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
- Precondition:
- The gesture detector has been initialized.
static PanGestureDetector Dali::PanGestureDetector::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcasts a handle to PanGestureDetector handle.
If handle points to a PanGestureDetector object, the downcast produces valid handle. If not, the returned handle is left uninitialized.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] handle Handle to an object
- Returns:
- Handle to a PanGestureDetector object or an uninitialized handle
Reimplemented from Dali::GestureDetector.
AngleThresholdPair Dali::PanGestureDetector::GetAngle | ( | size_t | index | ) | const |
Returns the angle by index that this pan gesture detector emits a signal.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] index The angle's index
- Returns:
- An angle threshold pair, or a zero valued angle pair when index is invalid
- Precondition:
- The gesture detector has been initialized.
- The index is less than GetAngleCount()
size_t Dali::PanGestureDetector::GetAngleCount | ( | ) | const |
Returns the count of angles that this pan gesture detector emits a signal.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The count
- Precondition:
- The gesture detector has been initialized.
uint32_t Dali::PanGestureDetector::GetMaximumTouchesRequired | ( | ) | const |
Retrieves the maximum number of touches required for the pan gesture to be detected.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The maximum touches required
- Precondition:
- The gesture detector has been initialized.
uint32_t Dali::PanGestureDetector::GetMinimumTouchesRequired | ( | ) | const |
Retrieves the minimum number of touches required for the pan gesture to be detected.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The minimum touches required
- Precondition:
- The gesture detector has been initialized.
static PanGestureDetector Dali::PanGestureDetector::New | ( | ) | [static] |
Creates an initialized PanGestureDetector.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- A handle to a newly allocated Dali resource
Reimplemented from Dali::Handle.
PanGestureDetector& Dali::PanGestureDetector::operator= | ( | const PanGestureDetector & | rhs | ) |
This assignment operator is required for (smart) pointer semantics.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] rhs A reference to the copied handle
- Returns:
- A reference to this
void Dali::PanGestureDetector::RemoveAngle | ( | Radian | angle | ) |
Removes the angle specified from the container.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] angle The angle to remove
- Precondition:
- The gesture detector has been initialized.
- Note:
- This will only remove the first instance of the angle found from the container.
- If an angle outside the range in AddAngle() is given, then the value is wrapped within the range and that is removed.
void Dali::PanGestureDetector::RemoveDirection | ( | Radian | direction | ) |
Removes the two angles that make up the direction from the container.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] direction The direction to remove
- Precondition:
- The gesture detector has been initialized.
- Note:
- If a direction outside the range in AddAngle() is given, then the value is wrapped within the range and that is removed.
void Dali::PanGestureDetector::SetMaximumTouchesRequired | ( | uint32_t | maximum | ) |
This is the maximum number of touches required for the pan gesture to be detected.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] maximum Maximum touches required
- Precondition:
- The gesture detector has been initialized.
- Note:
- The default maximum is '1'.
void Dali::PanGestureDetector::SetMinimumTouchesRequired | ( | uint32_t | minimum | ) |
This is the minimum number of touches required for the pan gesture to be detected.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] minimum Minimum touches required
- Precondition:
- The gesture detector has been initialized.
- Note:
- The default minimum is '1'.
static void Dali::PanGestureDetector::SetPanGestureProperties | ( | const PanGesture & | pan | ) | [static] |
Allows setting of the pan properties that are returned in constraints.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] pan The pan gesture to set
- Note:
- If a normal pan is taking place, then any value set is ignored.