Tizen Native API
5.5
|
PropertyBuffer is a handle to an object that contains a buffer of structured properties. More...
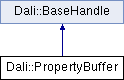
Public Member Functions | |
PropertyBuffer () | |
Default constructor, creates an empty handle. | |
~PropertyBuffer () | |
Destructor. | |
PropertyBuffer (const PropertyBuffer &handle) | |
Copy constructor, creates a new handle to the same object. | |
PropertyBuffer & | operator= (const PropertyBuffer &handle) |
Assignment operator, changes this handle to point at the same object. | |
void | SetData (const void *data, std::size_t size) |
Updates the whole buffer information. | |
std::size_t | GetSize () const |
Gets the number of elements in the buffer. | |
PropertyBuffer (Internal::PropertyBuffer *pointer) | |
The constructor. | |
Static Public Member Functions | |
static PropertyBuffer | New (Dali::Property::Map &bufferFormat) |
Creates a PropertyBuffer. Static property buffers use less memory. | |
static PropertyBuffer | DownCast (BaseHandle handle) |
Downcasts to a property buffer handle. If not, a property buffer the returned property buffer handle is left uninitialized. |
Detailed Description
PropertyBuffer is a handle to an object that contains a buffer of structured properties.
PropertyBuffers can be used to provide data to Geometry objects.
Example:
const float halfQuadSize = .5f; struct TexturedQuadVertex { Vector2 position; Vector2 textureCoordinates; }; TexturedQuadVertex texturedQuadVertexData[4] = { { Vector2(-halfQuadSize, -halfQuadSize), Vector2(0.f, 0.f) }, { Vector2( halfQuadSize, -halfQuadSize), Vector2(1.f, 0.f) }, { Vector2(-halfQuadSize, halfQuadSize), Vector2(0.f, 1.f) }, { Vector2( halfQuadSize, halfQuadSize), Vector2(1.f, 1.f) } };
Property::Map texturedQuadVertexFormat; texturedQuadVertexFormat["aPosition"] = Property::VECTOR2; texturedQuadVertexFormat["aTexCoord"] = Property::VECTOR2; PropertyBuffer texturedQuadVertices = PropertyBuffer::New( texturedQuadVertexFormat ); texturedQuadVertices.SetData( texturedQuadVertexData, 4 );
// Create indices uint32_t indexData[6] = { 0, 3, 1, 0, 2, 3 };
// Create the geometry object Geometry texturedQuadGeometry = Geometry::New(); texturedQuadGeometry.AddVertexBuffer( texturedQuadVertices ); texturedQuadGeometry.SetIndexBuffer( indexData, sizeof(indexData)/sizeof(indexData[0] );
- Since:
- 3.0, DALi version 1.1.43
Constructor & Destructor Documentation
Default constructor, creates an empty handle.
- Since:
- 3.0, DALi version 1.1.43
Destructor.
- Since:
- 3.0, DALi version 1.1.43
Dali::PropertyBuffer::PropertyBuffer | ( | const PropertyBuffer & | handle | ) |
Copy constructor, creates a new handle to the same object.
- Since:
- 3.0, DALi version 1.1.43
- Parameters:
-
[in] handle Handle to an object
Dali::PropertyBuffer::PropertyBuffer | ( | Internal::PropertyBuffer * | pointer | ) | [explicit] |
The constructor.
- Note:
- Not intended for application developers.
- Since:
- 3.0, DALi version 1.1.43
- Parameters:
-
[in] pointer A pointer to a newly allocated PropertyBuffer
Member Function Documentation
static PropertyBuffer Dali::PropertyBuffer::DownCast | ( | BaseHandle | handle | ) | [static] |
std::size_t Dali::PropertyBuffer::GetSize | ( | ) | const |
Gets the number of elements in the buffer.
- Since:
- 3.0, DALi version 1.1.43
- Returns:
- Number of elements to expand or contract the buffer
static PropertyBuffer Dali::PropertyBuffer::New | ( | Dali::Property::Map & | bufferFormat | ) | [static] |
Creates a PropertyBuffer. Static property buffers use less memory.
- Since:
- 3.0, DALi version 1.1.43
- Parameters:
-
[in] bufferFormat Map of names and types that describes the components of the buffer
- Returns:
- Handle to a newly allocated PropertyBuffer
PropertyBuffer& Dali::PropertyBuffer::operator= | ( | const PropertyBuffer & | handle | ) |
Assignment operator, changes this handle to point at the same object.
- Since:
- 3.0, DALi version 1.1.43
- Parameters:
-
[in] handle Handle to an object
- Returns:
- Reference to the assigned object
void Dali::PropertyBuffer::SetData | ( | const void * | data, |
std::size_t | size | ||
) |
Updates the whole buffer information.
This function expects a pointer to an array of structures with the same format that was given in the construction, and the number of elements to be the same as the size of the buffer.
If the initial structure was: { { "position", VECTOR3}, { "uv", VECTOR2 } } and a size of 10 elements, this function should be called with a pointer equivalent to:
struct Vertex { Dali::Vector3 position; Dali::Vector2 uv; }; Vertex vertices[ 10 ] = { ... }; propertyBuffer.SetData( vertices );
- Since:
- 3.0, DALi version 1.1.43
- Parameters:
-
[in] data A pointer to the data that will be copied to the buffer [in] size Number of elements to expand or contract the buffer