Tizen Native API
5.5
|
Dali::Texture Class Reference
Texture represents a texture object used as input or output by shaders. More...
Inheritance diagram for Dali::Texture:
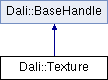
Public Member Functions | |
Texture () | |
Default constructor, creates an empty handle. | |
~Texture () | |
Destructor. | |
Texture (const Texture &handle) | |
Copy constructor, creates a new handle to the same object. | |
Texture & | operator= (const Texture &handle) |
Assignment operator, changes this handle to point at the same object. | |
bool | Upload (PixelData pixelData) |
Uploads data to the texture from a PixelData object. | |
bool | Upload (PixelData pixelData, uint32_t layer, uint32_t mipmap, uint32_t xOffset, uint32_t yOffset, uint32_t width, uint32_t height) |
Uploads data to the texture from a PixelData object. | |
void | GenerateMipmaps () |
Generates mipmaps for the texture. This will auto generate all the mipmaps for the texture based on the data in the base level. | |
uint32_t | GetWidth () const |
Returns the width of the texture. | |
uint32_t | GetHeight () const |
Returns the height of the texture. | |
Texture (Internal::Texture *pointer) | |
The constructor. | |
Static Public Member Functions | |
static Texture | New (TextureType::Type type, Pixel::Format format, uint32_t width, uint32_t height) |
Creates a new Texture object. | |
static Texture | New (NativeImageInterface &nativeImageInterface) |
Creates a new Texture object from a native image. | |
static Texture | DownCast (BaseHandle handle) |
Downcasts to a texture. If not, the returned handle is left uninitialized. |
Detailed Description
Texture represents a texture object used as input or output by shaders.
- Since:
- 3.0, DALi version 1.1.43
Constructor & Destructor Documentation
Default constructor, creates an empty handle.
- Since:
- 3.0, DALi version 1.1.43
Destructor.
- Since:
- 3.0, DALi version 1.1.43
Dali::Texture::Texture | ( | const Texture & | handle | ) |
Copy constructor, creates a new handle to the same object.
- Since:
- 3.0, DALi version 1.1.43
- Parameters:
-
[in] handle Handle to an object
Dali::Texture::Texture | ( | Internal::Texture * | pointer | ) | [explicit] |
The constructor.
- Note:
- Not intended for application developers.
- Since:
- 3.0, DALi version 1.1.43
- Parameters:
-
[in] pointer A pointer to a newly allocated Texture
Member Function Documentation
static Texture Dali::Texture::DownCast | ( | BaseHandle | handle | ) | [static] |
void Dali::Texture::GenerateMipmaps | ( | ) |
Generates mipmaps for the texture. This will auto generate all the mipmaps for the texture based on the data in the base level.
- Since:
- 3.0, DALi version 1.1.43
uint32_t Dali::Texture::GetHeight | ( | ) | const |
Returns the height of the texture.
- Since:
- 3.0, DALi version 1.1.43
- Returns:
- The height, in pixels, of the texture
uint32_t Dali::Texture::GetWidth | ( | ) | const |
Returns the width of the texture.
- Since:
- 3.0, DALi version 1.1.43
- Returns:
- The width, in pixels, of the texture
static Texture Dali::Texture::New | ( | TextureType::Type | type, |
Pixel::Format | format, | ||
uint32_t | width, | ||
uint32_t | height | ||
) | [static] |
static Texture Dali::Texture::New | ( | NativeImageInterface & | nativeImageInterface | ) | [static] |
Creates a new Texture object from a native image.
- Since:
- 3.0, DALi version 1.1.43
- Parameters:
-
[in] nativeImageInterface A native image
- Returns:
- A handle to a newly allocated Texture
- Note:
- It is not possible to upload data to textures created from a native image using Upload methods although there might be platform specific APIs to upload data to a native image.
Assignment operator, changes this handle to point at the same object.
- Since:
- 3.0, DALi version 1.1.43
- Parameters:
-
[in] handle Handle to an object
- Returns:
- Reference to the assigned object
bool Dali::Texture::Upload | ( | PixelData | pixelData | ) |
bool Dali::Texture::Upload | ( | PixelData | pixelData, |
uint32_t | layer, | ||
uint32_t | mipmap, | ||
uint32_t | xOffset, | ||
uint32_t | yOffset, | ||
uint32_t | width, | ||
uint32_t | height | ||
) |
Uploads data to the texture from a PixelData object.
- Note:
- Upload does not upsample or downsample pixel data to fit the specified rectangular area in the texture.
- Since:
- 3.0, DALi version 1.1.43
- Parameters:
-
[in] pixelData The pixelData object [in] layer Specifies the layer of a cube map or array texture (Unused for 2D textures).
- See also:
- CubeMapLayer
- Parameters:
-
[in] mipmap Specifies the level-of-detail number. Level 0 is the base image level. Level n is the nth mipmap reduction image [in] xOffset Specifies an horizontal offset of the rectangular area in the texture that will be updated [in] yOffset Specifies a vertical offset of the rectangular area in the texture that will be updated [in] width Specifies the width of the rectangular area in the texture that will be updated [in] height Specifies the height of the rectangular area in the texture that will be updated
- Returns:
- True if the PixelData object has compatible pixel format and fits in the rectangle specified, false otherwise