Tizen Native API
5.5
|
The TypeRegistry allows registration of type instance creation functions. More...
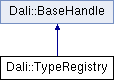
Public Member Functions | |
TypeRegistry () | |
Allows the creation of an empty typeRegistry handle. | |
~TypeRegistry () | |
Destructor. | |
TypeRegistry (const TypeRegistry &handle) | |
This copy constructor is required for (smart) pointer semantics. | |
TypeRegistry & | operator= (const TypeRegistry &rhs) |
This assignment operator is required for (smart) pointer semantics. | |
TypeInfo | GetTypeInfo (const std::string &uniqueTypeName) |
Gets TypeInfo for a registered type. | |
TypeInfo | GetTypeInfo (const std::type_info ®isterType) |
Gets TypeInfo for a registered type. | |
size_t | GetTypeNameCount () const |
Gets type name count. | |
std::string | GetTypeName (size_t index) const |
Gets type names by index. | |
Static Public Member Functions | |
static TypeRegistry | Get () |
Gets Type Registry handle. |
Detailed Description
The TypeRegistry allows registration of type instance creation functions.
These can then be created later by name and down cast to the appropriate type.
Usage: (Registering)
In my-actor.cpp
// Note: object construction in namespace scope is defined in a translation unit as being // in appearance order (C++ standard 3.6/2). So TypeRegistration is declared first in // the cpp file below. Signal, action and property declarations follow in any order. namespace { TypeRegistration myActorType(typeid(MyActor), typeid(Actor), CreateMyActor ); SignalConnectorType( myActorType, "highlighted", ConnectSignalForMyActor ); TypeAction( myActorType, "open", DoMyActorAction ); TypeAction( myActorType, "close", DoMyActorAction ); PropertyRegistration( myActorType, "status", PropertyRegistration::START_INDEX, Property::BOOLEAN, SetPropertyFunction, GetPropertyFunction ); }
Usage: (Creation)
TypeRegistry::TypeInfo type = TypeRegistry::Get().GetTypeInfo("MyActor"); MyActor a = MyActor::DownCast(type.CreateInstance()); if(a) { ... }
Actors that inherit from the CustomActor framework must ensure the implementation class has an identical name to the Actor class. This is to ensure the class can be found at runtime for signals and actions. Otherwise these will silently fail.
As namespaces are discarded the convention is to use a namespace ie
'class MyActor {}; namespace Internal { class MyActor {}; }'
Warning: this arrangement will silently fail
'class MyActor {}; class MyActorImpl {};'
Naming Conventions
Signal and action names follow properties and are by convention lower case hyphen separated ie 'next-page'. This maintains consistency with the scripted interface.
- Since:
- 3.0, DALi version 1.0.0
Constructor & Destructor Documentation
Allows the creation of an empty typeRegistry handle.
- Since:
- 3.0, DALi version 1.0.0
Destructor.
- Since:
- 3.0, DALi version 1.0.0
Dali::TypeRegistry::TypeRegistry | ( | const TypeRegistry & | handle | ) |
This copy constructor is required for (smart) pointer semantics.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] handle A reference to the copied handle
Member Function Documentation
static TypeRegistry Dali::TypeRegistry::Get | ( | ) | [static] |
TypeInfo Dali::TypeRegistry::GetTypeInfo | ( | const std::string & | uniqueTypeName | ) |
TypeInfo Dali::TypeRegistry::GetTypeInfo | ( | const std::type_info & | registerType | ) |
std::string Dali::TypeRegistry::GetTypeName | ( | size_t | index | ) | const |
Gets type names by index.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] index The index to get the type name
- Returns:
- The type name or an empty string when index is not valid
size_t Dali::TypeRegistry::GetTypeNameCount | ( | ) | const |
Gets type name count.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The count
TypeRegistry& Dali::TypeRegistry::operator= | ( | const TypeRegistry & | rhs | ) |
This assignment operator is required for (smart) pointer semantics.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] rhs A reference to the copied handle
- Returns:
- A reference to this