Tizen Native API
3.0
|
CustomActorImpl is an abstract base class for custom control implementations. More...
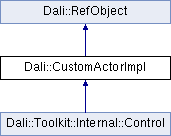
Public Member Functions | |
CustomActor | Self () const |
Used by derived CustomActorImpl instances, to access the public Actor interface. | |
virtual void | OnStageConnection (int depth)=0 |
Called after the actor has been connected to the stage. | |
virtual void | OnStageDisconnection ()=0 |
Called after the actor has been disconnected from Stage. | |
virtual void | OnChildAdd (Actor &child)=0 |
Called after a child has been added to the owning actor. | |
virtual void | OnChildRemove (Actor &child)=0 |
Called after the owning actor has attempted to remove a child (regardless of whether it succeeded or not). | |
virtual void | OnPropertySet (Property::Index index, Property::Value propertyValue) |
Called when the owning actor property is set. | |
virtual void | OnSizeSet (const Vector3 &targetSize)=0 |
Called when the owning actor's size is set e.g. using Actor::SetSize(). | |
virtual void | OnSizeAnimation (Animation &animation, const Vector3 &targetSize)=0 |
Called when the owning actor's size is animated e.g. using Animation::AnimateTo( Property( actor, Actor::Property::SIZE ), ... ). | |
virtual bool | OnTouchEvent (const TouchEvent &event) DALI_DEPRECATED_API=0 |
Called after a touch-event is received by the owning actor. | |
virtual bool | OnHoverEvent (const HoverEvent &event)=0 |
Called after a hover-event is received by the owning actor. | |
virtual bool | OnKeyEvent (const KeyEvent &event)=0 |
Called after a key-event is received by the actor that has had its focus set. | |
virtual bool | OnWheelEvent (const WheelEvent &event)=0 |
Called after a wheel-event is received by the owning actor. | |
virtual void | OnRelayout (const Vector2 &size, RelayoutContainer &container)=0 |
Called after the size negotiation has been finished for this control. | |
virtual void | OnSetResizePolicy (ResizePolicy::Type policy, Dimension::Type dimension)=0 |
Notification for deriving classes. | |
virtual Vector3 | GetNaturalSize ()=0 |
Returns the natural size of the actor. | |
virtual float | CalculateChildSize (const Dali::Actor &child, Dimension::Type dimension)=0 |
Calculates the size for a child. | |
virtual float | GetHeightForWidth (float width)=0 |
This method is called during size negotiation when a height is required for a given width. | |
virtual float | GetWidthForHeight (float height)=0 |
This method is called during size negotiation when a width is required for a given height. | |
virtual bool | RelayoutDependentOnChildren (Dimension::Type dimension=Dimension::ALL_DIMENSIONS)=0 |
Determines if this actor is dependent on its children for relayout. | |
virtual void | OnCalculateRelayoutSize (Dimension::Type dimension)=0 |
Virtual method to notify deriving classes that relayout dependencies have been met and the size for this object is about to be calculated for the given dimension. | |
virtual void | OnLayoutNegotiated (float size, Dimension::Type dimension)=0 |
Virtual method to notify deriving classes that the size for a dimension has just been negotiated. | |
virtual Extension * | GetExtension () |
Retrieves the extension for this control. | |
void | Initialize (Internal::CustomActor &owner) |
Initializes a CustomActor. | |
Internal::CustomActor * | GetOwner () const |
Gets the owner. | |
bool | RequiresTouchEvents () const |
Returns whether the OnTouchEvent() callback is required. | |
bool | RequiresHoverEvents () const |
Returns whether the OnHoverEvent() callback is required. | |
bool | RequiresWheelEvents () const |
Returns whether the OnWheelEvent() callback is required. | |
bool | IsRelayoutEnabled () const |
Returns whether relayout is enabled. | |
Protected Types | |
enum | ActorFlags |
Enumeration for the constructor flags. More... | |
Protected Member Functions | |
virtual | ~CustomActorImpl () |
Forward declare future extension interface. | |
CustomActorImpl (ActorFlags flags) | |
Creates a CustomActorImpl. | |
void | RelayoutRequest () |
Requests a relayout, which means performing a size negotiation on this actor, its parent and children (and potentially whole scene). | |
float | GetHeightForWidthBase (float width) |
Provides the Actor implementation of GetHeightForWidth. | |
float | GetWidthForHeightBase (float height) |
Provides the Actor implementation of GetWidthForHeight. | |
float | CalculateChildSizeBase (const Dali::Actor &child, Dimension::Type dimension) |
Calculates the size for a child using the base actor object. | |
bool | RelayoutDependentOnChildrenBase (Dimension::Type dimension=Dimension::ALL_DIMENSIONS) |
Determines if this actor is dependent on its children for relayout from the base class. | |
Static Protected Attributes | |
static const int | ACTOR_FLAG_COUNT = Log< LAST_ACTOR_FLAG - 1 >::value + 1 |
Value for deriving classes to continue on the flag enum. |
Detailed Description
CustomActorImpl is an abstract base class for custom control implementations.
This provides a series of pure virtual methods, which are called when actor-specific events occur. And CustomActorImpl is typically owned by a single CustomActor instance; see also CustomActor::CustomActor( CustomActorImpl &implementation ).
- Since:
- 2.4, DALi version 1.0.0
Member Enumeration Documentation
enum Dali::CustomActorImpl::ActorFlags [protected] |
Enumeration for the constructor flags.
- Since:
- 2.4, DALi version 1.0.0
- Enumerator:
ACTOR_BEHAVIOUR_NONE Use if no change to default behaviour is needed.
- Deprecated:
- Deprecated since 3.0, DALi version 1.2.10
ACTOR_BEHAVIOUR_DEFAULT Use to provide default behaviour (size negotiation is on, event callbacks are not called).
- Since:
- 3.0, DALi version 1.2.10
DISABLE_SIZE_NEGOTIATION True if control does not need size negotiation, i.e. it can be skipped in the algorithm.
- Since:
- 2.4, DALi version 1.0.0
REQUIRES_TOUCH_EVENTS True if the OnTouchEvent() callback is required.
- Since:
- 2.4, DALi version 1.0.0
REQUIRES_HOVER_EVENTS True if the OnHoverEvent() callback is required.
- Since:
- 2.4, DALi version 1.0.0
REQUIRES_WHEEL_EVENTS True if the OnWheelEvent() callback is required.
- Since:
- 2.4, DALi version 1.0.0
LAST_ACTOR_FLAG Special marker for last actor flag.
- Since:
- 2.4, DALi version 1.0.0
Constructor & Destructor Documentation
virtual Dali::CustomActorImpl::~CustomActorImpl | ( | ) | [protected, virtual] |
Forward declare future extension interface.
Virtual destructor
- Since:
- 2.4, DALi version 1.0.0
Dali::CustomActorImpl::CustomActorImpl | ( | ActorFlags | flags | ) | [protected] |
Creates a CustomActorImpl.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] flags Bitfield of ActorFlags to define behaviour
Member Function Documentation
virtual float Dali::CustomActorImpl::CalculateChildSize | ( | const Dali::Actor & | child, |
Dimension::Type | dimension | ||
) | [pure virtual] |
Calculates the size for a child.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] child The child actor to calculate the size for [in] dimension The dimension to calculate the size for. E.g. width or height
- Returns:
- Return the calculated size for the given dimension
Implemented in Dali::Toolkit::Internal::Control.
float Dali::CustomActorImpl::CalculateChildSizeBase | ( | const Dali::Actor & | child, |
Dimension::Type | dimension | ||
) | [protected] |
Calculates the size for a child using the base actor object.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] child The child actor to calculate the size for [in] dimension The dimension to calculate the size for. E.g. width or height
- Returns:
- Return the calculated size for the given dimension. If more than one dimension is requested, just return the first one found
virtual Extension* Dali::CustomActorImpl::GetExtension | ( | ) | [virtual] |
Retrieves the extension for this control.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The extension if available, NULL otherwise
virtual float Dali::CustomActorImpl::GetHeightForWidth | ( | float | width | ) | [pure virtual] |
This method is called during size negotiation when a height is required for a given width.
Derived classes should override this if they wish to customize the height returned.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] width Width to use
- Returns:
- The height based on the width
Implemented in Dali::Toolkit::Internal::Control.
float Dali::CustomActorImpl::GetHeightForWidthBase | ( | float | width | ) | [protected] |
Provides the Actor implementation of GetHeightForWidth.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] width Width to use
- Returns:
- The height based on the width
virtual Vector3 Dali::CustomActorImpl::GetNaturalSize | ( | ) | [pure virtual] |
Returns the natural size of the actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The actor's natural size
Implemented in Dali::Toolkit::Internal::Control.
Internal::CustomActor* Dali::CustomActorImpl::GetOwner | ( | ) | const |
Gets the owner.
This method is needed when creating additional handle objects to existing objects. Owner is the Dali::Internal::CustomActor that owns the implementation of the custom actor inside core. Creation of a handle to Dali public API Actor requires this pointer.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A pointer to the Actor implementation that owns this custom actor implementation
virtual float Dali::CustomActorImpl::GetWidthForHeight | ( | float | height | ) | [pure virtual] |
This method is called during size negotiation when a width is required for a given height.
Derived classes should override this if they wish to customize the width returned.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] height Height to use
- Returns:
- The width based on the width
Implemented in Dali::Toolkit::Internal::Control.
float Dali::CustomActorImpl::GetWidthForHeightBase | ( | float | height | ) | [protected] |
Provides the Actor implementation of GetWidthForHeight.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] height Height to use
- Returns:
- The width based on the height
void Dali::CustomActorImpl::Initialize | ( | Internal::CustomActor & | owner | ) |
Initializes a CustomActor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] owner The owning object
- Precondition:
- The CustomActorImpl is not already owned.
- Note:
- Called when ownership of the CustomActorImpl is passed to a CustomActor.
bool Dali::CustomActorImpl::IsRelayoutEnabled | ( | ) | const |
Returns whether relayout is enabled.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Return true if relayout is enabled on the custom actor
- Note:
- Called when ownership of the CustomActorImpl is passed to a CustomActor.
virtual void Dali::CustomActorImpl::OnCalculateRelayoutSize | ( | Dimension::Type | dimension | ) | [pure virtual] |
Virtual method to notify deriving classes that relayout dependencies have been met and the size for this object is about to be calculated for the given dimension.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] dimension The dimension that is about to be calculated
Implemented in Dali::Toolkit::Internal::Control.
virtual void Dali::CustomActorImpl::OnChildAdd | ( | Actor & | child | ) | [pure virtual] |
Called after a child has been added to the owning actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] child The child which has been added
Implemented in Dali::Toolkit::Internal::Control.
virtual void Dali::CustomActorImpl::OnChildRemove | ( | Actor & | child | ) | [pure virtual] |
Called after the owning actor has attempted to remove a child (regardless of whether it succeeded or not).
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] child The child being removed
Implemented in Dali::Toolkit::Internal::Control.
virtual bool Dali::CustomActorImpl::OnHoverEvent | ( | const HoverEvent & | event | ) | [pure virtual] |
Called after a hover-event is received by the owning actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] event The hover event
- Returns:
- True if the event should be consumed
- Note:
- CustomActorImpl::REQUIRES_HOVER_EVENTS must be enabled during construction. See CustomActorImpl::CustomActorImpl( ActorFlags flags ).
Implemented in Dali::Toolkit::Internal::Control.
virtual bool Dali::CustomActorImpl::OnKeyEvent | ( | const KeyEvent & | event | ) | [pure virtual] |
Called after a key-event is received by the actor that has had its focus set.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] event The Key Event
- Returns:
- True if the event should be consumed
Implemented in Dali::Toolkit::Internal::Control.
virtual void Dali::CustomActorImpl::OnLayoutNegotiated | ( | float | size, |
Dimension::Type | dimension | ||
) | [pure virtual] |
Virtual method to notify deriving classes that the size for a dimension has just been negotiated.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] size The new size for the given dimension [in] dimension The dimension that was just negotiated
Implemented in Dali::Toolkit::Internal::Control.
virtual void Dali::CustomActorImpl::OnPropertySet | ( | Property::Index | index, |
Property::Value | propertyValue | ||
) | [virtual] |
Called when the owning actor property is set.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The Property index that was set [in] propertyValue The value to set
virtual void Dali::CustomActorImpl::OnRelayout | ( | const Vector2 & | size, |
RelayoutContainer & | container | ||
) | [pure virtual] |
Called after the size negotiation has been finished for this control.
The control is expected to assign this given size to itself/its children.
Should be overridden by derived classes if they need to layout actors differently after certain operations like add or remove actors, resize or after changing specific properties.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] size The allocated size [in,out] container The control should add actors to this container that it is not able to allocate a size for
- Note:
- As this function is called from inside the size negotiation algorithm, you cannot call RequestRelayout (the call would just be ignored).
Implemented in Dali::Toolkit::Internal::Control.
virtual void Dali::CustomActorImpl::OnSetResizePolicy | ( | ResizePolicy::Type | policy, |
Dimension::Type | dimension | ||
) | [pure virtual] |
Notification for deriving classes.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] policy The policy being set [in] dimension The dimension the policy is being set for
Implemented in Dali::Toolkit::Internal::Control.
virtual void Dali::CustomActorImpl::OnSizeAnimation | ( | Animation & | animation, |
const Vector3 & | targetSize | ||
) | [pure virtual] |
Called when the owning actor's size is animated e.g. using Animation::AnimateTo( Property( actor, Actor::Property::SIZE ), ... ).
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] animation The object which is animating the owning actor [in] targetSize The target size. Note that this target size may not match the size returned via Actor::GetTargetSize
Implemented in Dali::Toolkit::Internal::Control.
virtual void Dali::CustomActorImpl::OnSizeSet | ( | const Vector3 & | targetSize | ) | [pure virtual] |
Called when the owning actor's size is set e.g. using Actor::SetSize().
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] targetSize The target size. Note that this target size may not match the size returned via Actor::GetTargetSize
Implemented in Dali::Toolkit::Internal::Control.
virtual void Dali::CustomActorImpl::OnStageConnection | ( | int | depth | ) | [pure virtual] |
Called after the actor has been connected to the stage.
When an actor is connected, it will be directly or indirectly parented to the root Actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] depth The depth in the hierarchy for the actor
- Note:
- The root Actor is provided automatically by Dali::Stage, and is always considered to be connected. When the parent of a set of actors is connected to the stage, then all of the children will received this callback. For the following actor tree, the callback order will be A, B, D, E, C, and finally F.
A (parent) / \ B C / \ \ D E F
- Parameters:
-
[in] depth The depth in the hierarchy for the actor
Implemented in Dali::Toolkit::Internal::Control.
virtual void Dali::CustomActorImpl::OnStageDisconnection | ( | ) | [pure virtual] |
Called after the actor has been disconnected from Stage.
If an actor is disconnected, it either has no parent or is parented to a disconnected actor.
- Since:
- 2.4, DALi version 1.0.0
- Note:
- When the parent of a set of actors is disconnected to the stage, then all of the children will received this callback, starting with the leaf actors. For the following actor tree, the callback order will be D, E, B, F, C, and finally A.
A (parent) / \ B C / \ \ D E F
Implemented in Dali::Toolkit::Internal::Control.
virtual bool Dali::CustomActorImpl::OnTouchEvent | ( | const TouchEvent & | event | ) | [pure virtual] |
Called after a touch-event is received by the owning actor.
- Deprecated:
- Deprecated since 3.0, DALi version 1.1.37 Connect to TouchSignal() instead.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] event The touch event
- Returns:
- True if the event should be consumed
- Note:
- CustomActorImpl::REQUIRES_TOUCH_EVENTS must be enabled during construction. See CustomActorImpl::CustomActorImpl( ActorFlags flags ).
Implemented in Dali::Toolkit::Internal::Control.
virtual bool Dali::CustomActorImpl::OnWheelEvent | ( | const WheelEvent & | event | ) | [pure virtual] |
Called after a wheel-event is received by the owning actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] event The wheel event
- Returns:
- True if the event should be consumed
- Note:
- CustomActorImpl::REQUIRES_WHEEL_EVENTS must be enabled during construction. See CustomActorImpl::CustomActorImpl( ActorFlags flags ).
Implemented in Dali::Toolkit::Internal::Control.
virtual bool Dali::CustomActorImpl::RelayoutDependentOnChildren | ( | Dimension::Type | dimension = Dimension::ALL_DIMENSIONS | ) | [pure virtual] |
Determines if this actor is dependent on its children for relayout.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] dimension The dimension(s) to check for
- Returns:
- Return if the actor is dependent on it's children
Implemented in Dali::Toolkit::Internal::Control.
bool Dali::CustomActorImpl::RelayoutDependentOnChildrenBase | ( | Dimension::Type | dimension = Dimension::ALL_DIMENSIONS | ) | [protected] |
Determines if this actor is dependent on its children for relayout from the base class.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] dimension The dimension(s) to check for
- Returns:
- Return if the actor is dependent on it's children
void Dali::CustomActorImpl::RelayoutRequest | ( | ) | [protected] |
Requests a relayout, which means performing a size negotiation on this actor, its parent and children (and potentially whole scene).
This method can also be called from a derived class every time it needs a different size. At the end of event processing, the relayout process starts and all controls which requested Relayout will have their sizes (re)negotiated.
- Since:
- 2.4, DALi version 1.0.0
- Note:
- RelayoutRequest() can be called multiple times; the size negotiation is still only performed once, i.e. there is no need to keep track of this in the calling side.
bool Dali::CustomActorImpl::RequiresHoverEvents | ( | ) | const |
Returns whether the OnHoverEvent() callback is required.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- True if the OnHoverEvent() callback is required
- Note:
- Called when ownership of the CustomActorImpl is passed to a CustomActor.
bool Dali::CustomActorImpl::RequiresTouchEvents | ( | ) | const |
Returns whether the OnTouchEvent() callback is required.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- True if the OnTouchEvent() callback is required
- Note:
- Called when ownership of the CustomActorImpl is passed to a CustomActor.
bool Dali::CustomActorImpl::RequiresWheelEvents | ( | ) | const |
Returns whether the OnWheelEvent() callback is required.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- True if the OnWheelEvent() callback is required
- Note:
- Called when ownership of the CustomActorImpl is passed to a CustomActor.
CustomActor Dali::CustomActorImpl::Self | ( | ) | const |
Used by derived CustomActorImpl instances, to access the public Actor interface.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A pointer to self, or an uninitialized pointer if the CustomActorImpl is not owned.