Tizen Native API
3.0
|
Actor is the primary object with which Dali applications interact. More...
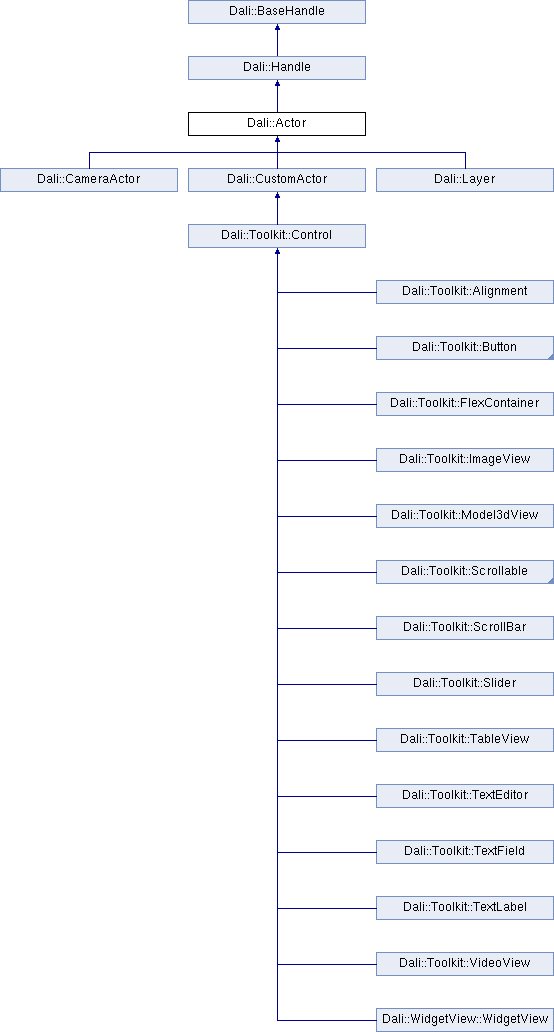
Classes | |
struct | Property |
Enumeration for the instance of properties belonging to the Actor class. More... | |
Public Types | |
typedef Signal< bool(Actor, const TouchEvent &) > | TouchSignalType |
Touch signal type. | |
typedef Signal< bool(Actor, const TouchData &) > | TouchDataSignalType |
Touch signal type. | |
typedef Signal< bool(Actor, const HoverEvent &) > | HoverSignalType |
Hover signal type. | |
typedef Signal< bool(Actor, const WheelEvent &) > | WheelEventSignalType |
Wheel signal type. | |
typedef Signal< void(Actor) > | OnStageSignalType |
Stage connection signal type. | |
typedef Signal< void(Actor) > | OffStageSignalType |
Stage disconnection signal type. | |
typedef Signal< void(Actor) > | OnRelayoutSignalType |
Called when the actor is relaid out. | |
Public Member Functions | |
Actor () | |
Creates an uninitialized Actor; this can be initialized with Actor::New(). | |
~Actor () | |
Dali::Actor is intended as a base class. | |
Actor (const Actor ©) | |
Copy constructor. | |
Actor & | operator= (const Actor &rhs) |
Assignment operator. | |
const std::string & | GetName () const |
Retrieves the Actor's name. | |
void | SetName (const std::string &name) |
Sets the Actor's name. | |
unsigned int | GetId () const |
Retrieves the unique ID of the actor. | |
bool | IsRoot () const |
Queries whether an actor is the root actor, which is owned by the Stage. | |
bool | OnStage () const |
Queries whether the actor is connected to the Stage. | |
bool | IsLayer () const |
Queries whether the actor is of class Dali::Layer. | |
Layer | GetLayer () |
Gets the layer in which the actor is present. | |
void | Add (Actor child) |
Adds a child Actor to this Actor. | |
void | Remove (Actor child) |
Removes a child Actor from this Actor. | |
void | Unparent () |
Removes an actor from its parent. | |
unsigned int | GetChildCount () const |
Retrieves the number of children held by the actor. | |
Actor | GetChildAt (unsigned int index) const |
Retrieve and child actor by index. | |
Actor | FindChildByName (const std::string &actorName) |
Search through this actor's hierarchy for an actor with the given name. | |
Actor | FindChildById (const unsigned int id) |
Search through this actor's hierarchy for an actor with the given unique ID. | |
Actor | GetParent () const |
Retrieves the actor's parent. | |
void | SetParentOrigin (const Vector3 &origin) |
Sets the origin of an actor, within its parent's area. | |
Vector3 | GetCurrentParentOrigin () const |
Retrieves the parent-origin of an actor. | |
void | SetAnchorPoint (const Vector3 &anchorPoint) |
Sets the anchor-point of an actor. | |
Vector3 | GetCurrentAnchorPoint () const |
Retrieves the anchor-point of an actor. | |
void | SetSize (float width, float height) |
Sets the size of an actor. | |
void | SetSize (float width, float height, float depth) |
Sets the size of an actor. | |
void | SetSize (const Vector2 &size) |
Sets the size of an actor. | |
void | SetSize (const Vector3 &size) |
Sets the size of an actor. | |
Vector3 | GetTargetSize () const |
Retrieves the actor's size. | |
Vector3 | GetCurrentSize () const |
Retrieves the actor's size. | |
Vector3 | GetNaturalSize () const |
Returns the natural size of the actor. | |
void | SetPosition (float x, float y) |
Sets the position of the actor. | |
void | SetPosition (float x, float y, float z) |
Sets the position of the Actor. | |
void | SetPosition (const Vector3 &position) |
Sets the position of the Actor. | |
void | SetX (float x) |
Sets the position of an actor along the X-axis. | |
void | SetY (float y) |
Sets the position of an actor along the Y-axis. | |
void | SetZ (float z) |
Sets the position of an actor along the Z-axis. | |
void | TranslateBy (const Vector3 &distance) |
Translates an actor relative to its existing position. | |
Vector3 | GetCurrentPosition () const |
Retrieves the position of the Actor. | |
Vector3 | GetCurrentWorldPosition () const |
Retrieves the world-position of the Actor. | |
void | SetPositionInheritanceMode (PositionInheritanceMode mode) DALI_DEPRECATED_API |
Sets the actors position inheritance mode. | |
void | SetInheritPosition (bool inherit) |
Sets whether a child actor inherits it's parent's position. | |
PositionInheritanceMode | GetPositionInheritanceMode () const DALI_DEPRECATED_API |
Returns the actors position inheritance mode. | |
bool | IsPositionInherited () const |
Returns whether the actor inherits its parent's position. | |
void | SetOrientation (const Degree &angle, const Vector3 &axis) |
Sets the orientation of the Actor. | |
void | SetOrientation (const Radian &angle, const Vector3 &axis) |
Sets the orientation of the Actor. | |
void | SetOrientation (const Quaternion &orientation) |
Sets the orientation of the Actor. | |
void | RotateBy (const Degree &angle, const Vector3 &axis) |
Applies a relative rotation to an actor. | |
void | RotateBy (const Radian &angle, const Vector3 &axis) |
Applies a relative rotation to an actor. | |
void | RotateBy (const Quaternion &relativeRotation) |
Applies a relative rotation to an actor. | |
Quaternion | GetCurrentOrientation () const |
Retrieves the Actor's orientation. | |
void | SetInheritOrientation (bool inherit) |
Sets whether a child actor inherits it's parent's orientation. | |
bool | IsOrientationInherited () const |
Returns whether the actor inherits its parent's orientation. | |
Quaternion | GetCurrentWorldOrientation () const |
Retrieves the world-orientation of the Actor. | |
void | SetScale (float scale) |
Sets the scale factor applied to an actor. | |
void | SetScale (float scaleX, float scaleY, float scaleZ) |
Sets the scale factor applied to an actor. | |
void | SetScale (const Vector3 &scale) |
Sets the scale factor applied to an actor. | |
void | ScaleBy (const Vector3 &relativeScale) |
Applies a relative scale to an actor. | |
Vector3 | GetCurrentScale () const |
Retrieves the scale factor applied to an actor. | |
Vector3 | GetCurrentWorldScale () const |
Retrieves the world-scale of the Actor. | |
void | SetInheritScale (bool inherit) |
Sets whether a child actor inherits it's parent's scale. | |
bool | IsScaleInherited () const |
Returns whether the actor inherits its parent's scale. | |
Matrix | GetCurrentWorldMatrix () const |
Retrieves the world-matrix of the actor. | |
void | SetVisible (bool visible) |
Sets the visibility flag of an actor. | |
bool | IsVisible () const |
Retrieves the visibility flag of an actor. | |
void | SetOpacity (float opacity) |
Sets the opacity of an actor. | |
float | GetCurrentOpacity () const |
Retrieves the actor's opacity. | |
void | SetColor (const Vector4 &color) |
Sets the actor's color; this is an RGBA value. | |
Vector4 | GetCurrentColor () const |
Retrieves the actor's color. | |
void | SetColorMode (ColorMode colorMode) |
Sets the actor's color mode. | |
ColorMode | GetColorMode () const |
Returns the actor's color mode. | |
Vector4 | GetCurrentWorldColor () const |
Retrieves the world-color of the Actor, where each component is clamped within the 0->1 range. | |
void | SetDrawMode (DrawMode::Type drawMode) |
Sets how the actor and its children should be drawn. | |
DrawMode::Type | GetDrawMode () const |
Queries how the actor and its children will be drawn. | |
void | SetSensitive (bool sensitive) |
Sets whether an actor should emit touch or hover signals. | |
bool | IsSensitive () const |
Queries whether an actor emits touch or hover event signals. | |
bool | ScreenToLocal (float &localX, float &localY, float screenX, float screenY) const |
Converts screen coordinates into the actor's coordinate system using the default camera. | |
void | SetLeaveRequired (bool required) |
Sets whether the actor should receive a notification when touch or hover motion events leave the boundary of the actor. | |
bool | GetLeaveRequired () const |
This returns whether the actor requires touch or hover events whenever touch or hover motion events leave the boundary of the actor. | |
void | SetKeyboardFocusable (bool focusable) |
Sets whether the actor should be focusable by keyboard navigation. | |
bool | IsKeyboardFocusable () const |
Returns whether the actor is focusable by keyboard navigation. | |
void | SetResizePolicy (ResizePolicy::Type policy, Dimension::Type dimension) |
Sets the resize policy to be used for the given dimension(s). | |
ResizePolicy::Type | GetResizePolicy (Dimension::Type dimension) const |
Returns the resize policy used for a single dimension. | |
void | SetSizeScalePolicy (SizeScalePolicy::Type policy) |
Sets the policy to use when setting size with size negotiation. Defaults to SizeScalePolicy::USE_SIZE_SET. | |
SizeScalePolicy::Type | GetSizeScalePolicy () const |
Returns the size scale policy in use. | |
void | SetSizeModeFactor (const Vector3 &factor) |
Sets the relative to parent size factor of the actor. | |
Vector3 | GetSizeModeFactor () const |
Retrieves the relative to parent size factor of the actor. | |
float | GetHeightForWidth (float width) |
Calculates the height of the actor given a width. | |
float | GetWidthForHeight (float height) |
Calculates the width of the actor given a height. | |
float | GetRelayoutSize (Dimension::Type dimension) const |
Returns the value of negotiated dimension for the given dimension. | |
void | SetPadding (const Padding &padding) |
Sets the padding for use in layout. | |
void | GetPadding (Padding &paddingOut) const |
Returns the value of the padding. | |
void | SetMinimumSize (const Vector2 &size) |
Sets the minimum size an actor can be assigned in size negotiation. | |
Vector2 | GetMinimumSize () |
Returns the minimum relayout size. | |
void | SetMaximumSize (const Vector2 &size) |
Sets the maximum size an actor can be assigned in size negotiation. | |
Vector2 | GetMaximumSize () |
Returns the maximum relayout size. | |
int | GetHierarchyDepth () |
Gets depth in the hierarchy for the actor. | |
unsigned int | AddRenderer (Renderer &renderer) |
Adds a renderer to this actor. | |
unsigned int | GetRendererCount () const |
Gets the number of renderers on this actor. | |
Renderer | GetRendererAt (unsigned int index) |
Gets a Renderer by index. | |
void | RemoveRenderer (Renderer &renderer) |
Removes a renderer from the actor. | |
void | RemoveRenderer (unsigned int index) |
Removes a renderer from the actor by index. | |
TouchSignalType & | TouchedSignal () DALI_DEPRECATED_API |
This signal is emitted when touch input is received. | |
TouchDataSignalType & | TouchSignal () |
This signal is emitted when touch input is received. | |
HoverSignalType & | HoveredSignal () |
This signal is emitted when hover input is received. | |
WheelEventSignalType & | WheelEventSignal () |
This signal is emitted when wheel event is received. | |
OnStageSignalType & | OnStageSignal () |
This signal is emitted after the actor has been connected to the stage. | |
OffStageSignalType & | OffStageSignal () |
This signal is emitted after the actor has been disconnected from the stage. | |
OnRelayoutSignalType & | OnRelayoutSignal () |
This signal is emitted after the size has been set on the actor during relayout. | |
Static Public Member Functions | |
static Actor | New () |
Creates an initialized Actor. | |
static Actor | DownCast (BaseHandle handle) |
Downcasts a handle to Actor handle. |
Detailed Description
Actor is the primary object with which Dali applications interact.
UI controls can be built by combining multiple actors.
Multi-Touch Events:
Touch or hover events are received via signals; see Actor::TouchedSignal() and Actor::HoveredSignal() for more details.
Hit Testing Rules Summary:
- An actor is only hittable if the actor's touch or hover signal has a connection.
- An actor is only hittable when it is between the camera's near and far planes.
- If an actor is made insensitive, then the actor and its children are not hittable; see IsSensitive().
- If an actor's visibility flag is unset, then none of its children are hittable either; see IsVisible().
- To be hittable, an actor must have a non-zero size.
- If an actor's world color is fully transparent, then it is not hittable; see GetCurrentWorldColor().
Hit Test Algorithm:
- Stage
- Gets the first down and the last up touch events to the screen, regardless of actor touch event consumption.
- Stage's root layer can be used to catch unconsumed touch events.
- RenderTasks
- Hit testing is dependent on the camera used, which is specific to each RenderTask.
- Layers
- For each RenderTask, hit testing starts from the top-most layer and we go through all the layers until we have a hit or there are none left.
- Before we perform a hit test within a layer, we check if all the layer's parents are visible and sensitive.
- If they are not, we skip hit testing the actors in that layer altogether.
- If a layer is set to consume all touch, then we do not check any layers behind this layer.
- Actors
- The final part of hit testing is performed by walking through the actor tree within a layer.
- The following pseudocode shows the algorithm used:
HIT-TEST-WITHIN-LAYER( ACTOR ) { // Only hit-test the actor and its children if it is sensitive and visible IF ( ACTOR-IS-SENSITIVE && ACTOR-IS-VISIBLE && ACTOR-IS-ON-STAGE ) { // Depth-first traversal within current layer, visiting parent first // Check whether current actor should be hit-tested. IF ( ( TOUCH-SIGNAL-NOT-EMPTY || HOVER-SIGNAL-NOT-EMPTY ) && ACTOR-HAS-NON-ZERO-SIZE && ACTOR-WORLD-COLOR-IS-NOT-TRANSPARENT ) { // Hit-test current actor IF ( ACTOR-HIT ) { IF ( ACTOR-IS-OVERLAY || ( DISTANCE-TO-ACTOR < DISTANCE-TO-LAST-HIT-ACTOR ) ) { // The current actor is the closest actor that was underneath the touch. LAST-HIT-ACTOR = CURRENT-ACTOR } } } // Keep checking children, in case we hit something closer. FOR-EACH CHILD (in order) { IF ( CHILD-IS-NOT-A-LAYER ) { // Continue traversal for this child's sub-tree HIT-TEST-WITHIN-LAYER ( CHILD ) } // else we skip hit-testing the child's sub-tree altogether. } } }
- Overlays always take priority (i.e. they're considered closer) regardless of distance. The overlay children take priority over their parents, and overlay siblings take priority over their previous siblings (i.e. reverse of rendering order): For more information, see SetDrawMode().
1 / \ / \ 2 5 / \ \ / \ \ 3 4 6 Hit Priority of above Actor tree (all overlays): 1 - Lowest. 6 - Highest.
Touch or hover Event Delivery:
- Delivery
- The hit actor's touch or hover signal is emitted first; if it is not consumed by any of the listeners, the parent's touch or hover signal is emitted, and so on.
- The following pseudocode shows the delivery mechanism:
EMIT-TOUCH-SIGNAL( ACTOR ) { IF ( TOUCH-SIGNAL-NOT-EMPTY ) { // Only do the emission if touch signal of actor has connections. CONSUMED = TOUCHED-SIGNAL( TOUCH-EVENT ) } IF ( NOT-CONSUMED ) { // If event is not consumed then deliver it to the parent unless we reach the root actor IF ( ACTOR-PARENT ) { EMIT-TOUCH-SIGNAL( ACTOR-PARENT ) } } } EMIT-HOVER-SIGNAL( ACTOR ) { IF ( HOVER-SIGNAL-NOT-EMPTY ) { // Only do the emission if hover signal of actor has connections. CONSUMED = HOVERED-SIGNAL( HOVER-EVENT ) } IF ( NOT-CONSUMED ) { // If event is not consumed then deliver it to the parent unless we reach the root actor. IF ( ACTOR-PARENT ) { EMIT-HOVER-SIGNAL( ACTOR-PARENT ) } } }
- If there are several touch points, then the delivery is only to the first touch point's hit actor (and its parents). There will be NO touch or hover signal delivery for the hit actors of the other touch points.
- The local coordinates are from the top-left (0.0f, 0.0f, 0.5f) of the hit actor.
- Leave State
- A "Leave" state is set when the first point exits the bounds of the previous first point's hit actor (primary hit actor).
- When this happens, the last primary hit actor's touch or hover signal is emitted with a "Leave" state (only if it requires leave signals); see SetLeaveRequired().
- Interrupted State
- If a system event occurs which interrupts the touch or hover processing, then the last primary hit actor's touch or hover signals are emitted with an "Interrupted" state.
- If the last primary hit actor, or one of its parents, is no longer touchable or hoverable, then its touch or hover signals are also emitted with an "Interrupted" state.
- If the consumed actor on touch-down is not the same as the consumed actor on touch-up, then touch signals are also emitted from the touch-down actor with an "Interrupted" state.
- If the consumed actor on hover-start is not the same as the consumed actor on hover-finished, then hover signals are also emitted from the hover-started actor with an "Interrupted" state.
Key Events:
Key events are received by an actor once set to grab key events, only one actor can be set as focused.
Signals | Signal Name | Method | |-------------------|------------------------------| | touched | TouchedSignal() | | hovered | HoveredSignal() | | wheelEvent | WheelEventSignal() | | onStage | OnStageSignal() | | offStage | OffStageSignal() | | onRelayout | OnRelayoutSignal() |
Actions | Action Name | Actor method called | |-------------------|------------------------------| | show | SetVisible( true ) | | hide | SetVisible( false ) |
- Since:
- 2.4, DALi version 1.0.0
Member Typedef Documentation
typedef Signal< bool (Actor, const HoverEvent&) > Dali::Actor::HoverSignalType |
Hover signal type.
- Since:
- 2.4, DALi version 1.0.0
typedef Signal< void (Actor) > Dali::Actor::OffStageSignalType |
Stage disconnection signal type.
- Since:
- 2.4, DALi version 1.0.0
typedef Signal< void (Actor) > Dali::Actor::OnRelayoutSignalType |
Called when the actor is relaid out.
- Since:
- 2.4, DALi version 1.0.0
typedef Signal< void (Actor) > Dali::Actor::OnStageSignalType |
Stage connection signal type.
- Since:
- 2.4, DALi version 1.0.0
typedef Signal< bool (Actor, const TouchData&) > Dali::Actor::TouchDataSignalType |
Touch signal type.
- Since:
- 3.0, DALi version 1.1.37
typedef Signal< bool (Actor, const TouchEvent&) > Dali::Actor::TouchSignalType |
Touch signal type.
- Deprecated:
- Deprecated since 3.0, DALi version 1.1.37
- Since:
- 2.4, DALi version 1.0.0
typedef Signal< bool (Actor, const WheelEvent&) > Dali::Actor::WheelEventSignalType |
Wheel signal type.
- Since:
- 2.4, DALi version 1.0.0
Constructor & Destructor Documentation
Creates an uninitialized Actor; this can be initialized with Actor::New().
Calling member functions with an uninitialized Actor handle is not allowed.
- Since:
- 2.4, DALi version 1.0.0
Dali::Actor is intended as a base class.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 2.4, DALi version 1.0.0
Dali::Actor::Actor | ( | const Actor & | copy | ) |
Copy constructor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] copy The actor to copy
Member Function Documentation
void Dali::Actor::Add | ( | Actor | child | ) |
Adds a child Actor to this Actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] child The child
- Precondition:
- This Actor (the parent) has been initialized.
- The child actor has been initialized.
- The child actor is not the same as the parent actor.
- The actor is not the Root actor.
- Postcondition:
- The child will be referenced by its parent. This means that the child will be kept alive, even if the handle passed into this method is reset or destroyed.
- Note:
- If the child already has a parent, it will be removed from old parent and reparented to this actor. This may change child's position, color, scale etc as it now inherits them from this actor.
unsigned int Dali::Actor::AddRenderer | ( | Renderer & | renderer | ) |
static Actor Dali::Actor::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcasts a handle to Actor handle.
If handle points to an Actor object, the downcast produces valid handle. If not, the returned handle is left uninitialized.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle to An object
- Returns:
- handle to a Actor object or an uninitialized handle
Reimplemented from Dali::Handle.
Reimplemented in Dali::Toolkit::ScrollView, Dali::Toolkit::TextLabel, Dali::Toolkit::FlexContainer, Dali::Toolkit::TableView, Dali::Toolkit::TextField, Dali::Toolkit::Slider, Dali::Toolkit::Control, Dali::Toolkit::ScrollBar, Dali::Layer, Dali::Toolkit::ImageView, Dali::Toolkit::TextEditor, Dali::Toolkit::PushButton, Dali::Toolkit::VideoView, Dali::WidgetView::WidgetView, Dali::Toolkit::ItemView, Dali::Toolkit::Alignment, Dali::Toolkit::Button, Dali::CameraActor, Dali::Toolkit::Scrollable, Dali::Toolkit::Model3dView, Dali::Toolkit::RadioButton, Dali::Toolkit::CheckBoxButton, and Dali::CustomActor.
Actor Dali::Actor::FindChildById | ( | const unsigned int | id | ) |
Search through this actor's hierarchy for an actor with the given unique ID.
The actor itself is also considered in the search.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] id The ID of the actor to find
- Returns:
- A handle to the actor if found, or an empty handle if not
- Precondition:
- The Actor has been initialized.
Actor Dali::Actor::FindChildByName | ( | const std::string & | actorName | ) |
Search through this actor's hierarchy for an actor with the given name.
The actor itself is also considered in the search.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] actorName The name of the actor to find
- Returns:
- A handle to the actor if found, or an empty handle if not
- Precondition:
- The Actor has been initialized.
Actor Dali::Actor::GetChildAt | ( | unsigned int | index | ) | const |
Retrieve and child actor by index.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the child to retrieve
- Returns:
- The actor for the given index or empty handle if children not initialized
- Precondition:
- The Actor has been initialized.
unsigned int Dali::Actor::GetChildCount | ( | ) | const |
Retrieves the number of children held by the actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The number of children
- Precondition:
- The Actor has been initialized.
ColorMode Dali::Actor::GetColorMode | ( | ) | const |
Returns the actor's color mode.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Currently used colorMode
- Precondition:
- The Actor has been initialized.
Vector3 Dali::Actor::GetCurrentAnchorPoint | ( | ) | const |
Retrieves the anchor-point of an actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The current anchor-point
- Precondition:
- The Actor has been initialized.
- Note:
- This property can be animated; the return value may not match the value written with SetAnchorPoint().
Vector4 Dali::Actor::GetCurrentColor | ( | ) | const |
Retrieves the actor's color.
Actor's own color is not clamped.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The color
- Precondition:
- The Actor has been initialized.
- Note:
- This property can be animated; the return value may not match the value written with SetColor().
float Dali::Actor::GetCurrentOpacity | ( | ) | const |
Retrieves the actor's opacity.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The actor's opacity
- Precondition:
- The actor has been initialized.
- Note:
- This property can be animated; the return value may not match the value written with SetOpacity().
Quaternion Dali::Actor::GetCurrentOrientation | ( | ) | const |
Retrieves the Actor's orientation.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The current orientation
- Precondition:
- The Actor has been initialized.
- Note:
- This property can be animated; the return value may not match the value written with SetOrientation().
Vector3 Dali::Actor::GetCurrentParentOrigin | ( | ) | const |
Retrieves the parent-origin of an actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The current parent-origin
- Precondition:
- The Actor has been initialized.
- Note:
- This property can be animated; the return value may not match the value written with SetParentOrigin().
Vector3 Dali::Actor::GetCurrentPosition | ( | ) | const |
Retrieves the position of the Actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The Actor's current position
- Precondition:
- The Actor has been initialized.
- Note:
- This property can be animated; the return value may not match the value written with SetPosition().
Vector3 Dali::Actor::GetCurrentScale | ( | ) | const |
Retrieves the scale factor applied to an actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A vector representing the scale factor for each axis
- Precondition:
- The Actor has been initialized.
- Note:
- This property can be animated; the return value may not match the value written with SetScale().
Vector3 Dali::Actor::GetCurrentSize | ( | ) | const |
Retrieves the actor's size.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The actor's current size
- Precondition:
- The actor has been initialized.
- Note:
- This property can be animated; the return value may not match the value written with SetSize().
Vector4 Dali::Actor::GetCurrentWorldColor | ( | ) | const |
Retrieves the world-color of the Actor, where each component is clamped within the 0->1 range.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The Actor's current color in the world
- Precondition:
- The Actor has been initialized.
- Note:
- The actor will not have a world-color, unless it has previously been added to the stage.
Matrix Dali::Actor::GetCurrentWorldMatrix | ( | ) | const |
Vector3 Dali::Actor::GetCurrentWorldPosition | ( | ) | const |
Vector3 Dali::Actor::GetCurrentWorldScale | ( | ) | const |
DrawMode::Type Dali::Actor::GetDrawMode | ( | ) | const |
Queries how the actor and its children will be drawn.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Return the draw mode type
float Dali::Actor::GetHeightForWidth | ( | float | width | ) |
Calculates the height of the actor given a width.
The natural size is used for default calculation. size 0 is treated as aspect ratio 1:1.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] width Width to use
- Returns:
- Return the height based on the width
int Dali::Actor::GetHierarchyDepth | ( | ) |
Gets depth in the hierarchy for the actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The current depth in the hierarchy of the actor, or
-1
if actor is not in the hierarchy
unsigned int Dali::Actor::GetId | ( | ) | const |
Retrieves the unique ID of the actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The ID
- Precondition:
- The Actor has been initialized.
Gets the layer in which the actor is present.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The layer, which will be uninitialized if the actor is off-stage
- Precondition:
- The Actor has been initialized.
bool Dali::Actor::GetLeaveRequired | ( | ) | const |
This returns whether the actor requires touch or hover events whenever touch or hover motion events leave the boundary of the actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
true
if a Leave event is required,false
otherwise
- Precondition:
- The Actor has been initialized.
Returns the maximum relayout size.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Return the maximum size
Returns the minimum relayout size.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Return the mininmum size
const std::string& Dali::Actor::GetName | ( | ) | const |
Vector3 Dali::Actor::GetNaturalSize | ( | ) | const |
Returns the natural size of the actor.
Deriving classes stipulate the natural size and by default an actor has a ZERO natural size.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The actor's natural size
void Dali::Actor::GetPadding | ( | Padding & | paddingOut | ) | const |
Returns the value of the padding.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] paddingOut The returned padding data
Actor Dali::Actor::GetParent | ( | ) | const |
Retrieves the actor's parent.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A handle to the actor's parent. If the actor has no parent, this handle will be invalid
- Precondition:
- The actor has been initialized.
Returns the actors position inheritance mode.
- Deprecated:
- Deprecated since 3.0, DALi version 1.1.24 Use IsPositionInherited
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Return the position inheritance mode
- Precondition:
- The Actor has been initialized.
float Dali::Actor::GetRelayoutSize | ( | Dimension::Type | dimension | ) | const |
Returns the value of negotiated dimension for the given dimension.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] dimension The dimension to retrieve
- Returns:
- Return the value of the negotiated dimension. If more than one dimension is requested, just return the first one found
Renderer Dali::Actor::GetRendererAt | ( | unsigned int | index | ) |
Gets a Renderer by index.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index The index of the renderer to fetch
- Returns:
- The renderer at the specified index
- Precondition:
- The index must be between 0 and GetRendererCount()-1
unsigned int Dali::Actor::GetRendererCount | ( | ) | const |
Gets the number of renderers on this actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The number of renderers on this actor
ResizePolicy::Type Dali::Actor::GetResizePolicy | ( | Dimension::Type | dimension | ) | const |
Returns the resize policy used for a single dimension.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] dimension The dimension to get policy for
- Returns:
- Return the dimension resize policy. If more than one dimension is requested, just return the first one found
Vector3 Dali::Actor::GetSizeModeFactor | ( | ) | const |
Returns the size scale policy in use.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Return the size scale policy
Vector3 Dali::Actor::GetTargetSize | ( | ) | const |
Retrieves the actor's size.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The actor's target size
- Precondition:
- The actor has been initialized.
- Note:
- This return is the value that was set using SetSize or the target size of an animation.
float Dali::Actor::GetWidthForHeight | ( | float | height | ) |
Calculates the width of the actor given a height.
The natural size is used for default calculation. size 0 is treated as aspect ratio 1:1.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] height Height to use
- Returns:
- Return the width based on the height
This signal is emitted when hover input is received.
A callback of the following type may be connected:
bool YourCallbackName(Actor actor, const HoverEvent& event);
The return value of True, indicates that the hover event should be consumed. Otherwise the signal will be emitted on the next sensitive parent of the actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
- Precondition:
- The Actor has been initialized.
bool Dali::Actor::IsKeyboardFocusable | ( | ) | const |
Returns whether the actor is focusable by keyboard navigation.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
true
if the actor is focusable by keyboard navigation,false
if not
- Precondition:
- The Actor has been initialized.
bool Dali::Actor::IsLayer | ( | ) | const |
Queries whether the actor is of class Dali::Layer.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- True if the actor is a layer
- Precondition:
- The Actor has been initialized.
bool Dali::Actor::IsOrientationInherited | ( | ) | const |
Returns whether the actor inherits its parent's orientation.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
true
if the actor inherits its parent orientation,false
if it uses world orientation
- Precondition:
- The Actor has been initialized.
bool Dali::Actor::IsPositionInherited | ( | ) | const |
Returns whether the actor inherits its parent's position.
- Since:
- 3.0, DALi version 1.1.24
- Returns:
true
if the actor inherits its parent position,false
if it uses world position
- Precondition:
- The Actor has been initialized.
bool Dali::Actor::IsRoot | ( | ) | const |
bool Dali::Actor::IsScaleInherited | ( | ) | const |
Returns whether the actor inherits its parent's scale.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
true
if the actor inherits its parent scale,false
if it uses world scale
- Precondition:
- The Actor has been initialized.
bool Dali::Actor::IsSensitive | ( | ) | const |
Queries whether an actor emits touch or hover event signals.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
true
, if emission of touch or hover event signals is enabled,false
otherwise
- Precondition:
- The Actor has been initialized.
- Note:
- If an actor is not sensitive, then it's children will not be hittable either. This is regardless of the individual sensitivity values of the children i.e. an actor will only be hittable if all of its parents have sensitivity set to true.
bool Dali::Actor::IsVisible | ( | ) | const |
Retrieves the visibility flag of an actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The visibility flag
- Precondition:
- The actor has been initialized.
- Note:
- This property can be animated; the return value may not match the value written with SetVisible().
- If an actor is not visible, then the actor and its children will not be rendered. This is regardless of the individual visibility values of the children i.e. an actor will only be rendered if all of its parents have visibility set to true.
static Actor Dali::Actor::New | ( | ) | [static] |
Creates an initialized Actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A handle to a newly allocated Dali resource
Reimplemented from Dali::Handle.
Reimplemented in Dali::Toolkit::ScrollView, Dali::Toolkit::TextLabel, Dali::Toolkit::FlexContainer, Dali::Layer, Dali::Toolkit::TextField, Dali::Toolkit::Slider, Dali::Toolkit::Control, Dali::Toolkit::TextEditor, Dali::Toolkit::PushButton, Dali::CameraActor, Dali::Toolkit::ImageView, Dali::Toolkit::RadioButton, Dali::Toolkit::CheckBoxButton, Dali::Toolkit::VideoView, and Dali::Toolkit::Model3dView.
This signal is emitted after the actor has been disconnected from the stage.
If an actor is disconnected it either has no parent, or is parented to a disconnected actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
- Note:
- When the parent of a set of actors is disconnected to the stage, then all of the children will received this callback, starting with the leaf actors. For the following actor tree, the callback order will be D, E, B, F, C, and finally A.
A (parent) / \ B C / \ \ D E F
This signal is emitted after the size has been set on the actor during relayout.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal
bool Dali::Actor::OnStage | ( | ) | const |
Queries whether the actor is connected to the Stage.
When an actor is connected, it will be directly or indirectly parented to the root Actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- True if the actor is connected to the Stage
- Precondition:
- The Actor has been initialized.
- Note:
- The root Actor is provided automatically by Dali::Stage, and is always considered to be connected.
This signal is emitted after the actor has been connected to the stage.
When an actor is connected, it will be directly or indirectly parented to the root Actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
- Note:
- The root Actor is provided automatically by Dali::Stage, and is always considered to be connected.
- When the parent of a set of actors is connected to the stage, then all of the children will received this callback. For the following actor tree, the callback order will be A, B, D, E, C, and finally F.
A (parent) / \ B C / \ \ D E F
Assignment operator.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] rhs The actor to copy
- Returns:
- A reference to this
void Dali::Actor::Remove | ( | Actor | child | ) |
void Dali::Actor::RemoveRenderer | ( | Renderer & | renderer | ) |
Removes a renderer from the actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] renderer Handle to the renderer that is to be removed
void Dali::Actor::RemoveRenderer | ( | unsigned int | index | ) |
Removes a renderer from the actor by index.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] index Index of the renderer that is to be removed
- Precondition:
- The index must be between 0 and GetRendererCount()-1
void Dali::Actor::RotateBy | ( | const Degree & | angle, |
const Vector3 & | axis | ||
) |
Applies a relative rotation to an actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] angle The angle to the rotation to combine with the existing orientation [in] axis The axis of the rotation to combine with the existing orientation
- Precondition:
- The actor has been initialized.
void Dali::Actor::RotateBy | ( | const Radian & | angle, |
const Vector3 & | axis | ||
) |
Applies a relative rotation to an actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] angle The angle to the rotation to combine with the existing orientation [in] axis The axis of the rotation to combine with the existing orientation
- Precondition:
- The actor has been initialized.
void Dali::Actor::RotateBy | ( | const Quaternion & | relativeRotation | ) |
Applies a relative rotation to an actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] relativeRotation The rotation to combine with the existing orientation
- Precondition:
- The actor has been initialized.
void Dali::Actor::ScaleBy | ( | const Vector3 & | relativeScale | ) |
Applies a relative scale to an actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] relativeScale The scale to combine with the actor's existing scale
- Precondition:
- The actor has been initialized.
bool Dali::Actor::ScreenToLocal | ( | float & | localX, |
float & | localY, | ||
float | screenX, | ||
float | screenY | ||
) | const |
Converts screen coordinates into the actor's coordinate system using the default camera.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[out] localX On return, the X-coordinate relative to the actor [out] localY On return, the Y-coordinate relative to the actor [in] screenX The screen X-coordinate [in] screenY The screen Y-coordinate
- Returns:
- True if the conversion succeeded
- Precondition:
- The Actor has been initialized.
- Note:
- The actor coordinates are relative to the top-left (0.0, 0.0, 0.5)
void Dali::Actor::SetAnchorPoint | ( | const Vector3 & | anchorPoint | ) |
Sets the anchor-point of an actor.
This is expressed in unit coordinates, such that (0.0, 0.0, 0.5) is the top-left corner of the actor, and (1.0, 1.0, 0.5) is the bottom-right corner. The default anchor point is Dali::AnchorPoint::CENTER (0.5, 0.5, 0.5). An actor position is the distance between its parent-origin, and this anchor-point. An actor's orientation is the rotation from its default orientation, the rotation is centered around its anchor-point.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] anchorPoint The new anchor-point
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentAnchorPoint().
- See also:
- Dali::AnchorPoint for predefined anchor point values
void Dali::Actor::SetColor | ( | const Vector4 & | color | ) |
Sets the actor's color; this is an RGBA value.
The final color of the actor depends on its color mode.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] color The new color
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentColor().
void Dali::Actor::SetColorMode | ( | ColorMode | colorMode | ) |
void Dali::Actor::SetDrawMode | ( | DrawMode::Type | drawMode | ) |
Sets how the actor and its children should be drawn.
Not all actors are renderable, but DrawMode can be inherited from any actor. If an object is in a 3D layer, it will be depth-tested against other objects in the world i.e. it may be obscured if other objects are in front.
If DrawMode::OVERLAY_2D is used, the actor and its children will be drawn as a 2D overlay. Overlay actors are drawn in a separate pass, after all non-overlay actors within the Layer. For overlay actors, the drawing order is with respect to tree levels of Actors, and depth-testing will not be used.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] drawMode The new draw-mode to use
- Note:
- Layers do not inherit the DrawMode from their parents.
void Dali::Actor::SetInheritOrientation | ( | bool | inherit | ) |
Sets whether a child actor inherits it's parent's orientation.
Default is to inherit. Switching this off means that using SetOrientation() sets the actor's world orientation.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] inherit - true
if the actor should inherit orientation,false
otherwise
- Precondition:
- The Actor has been initialized.
void Dali::Actor::SetInheritPosition | ( | bool | inherit | ) |
Sets whether a child actor inherits it's parent's position.
Default is to inherit. Switching this off means that using SetPosition() sets the actor's world position3
- Since:
- 3.0, DALi version 1.1.24
- Parameters:
-
[in] inherit - true
if the actor should inherit position,false
otherwise
- Precondition:
- The Actor has been initialized.
void Dali::Actor::SetInheritScale | ( | bool | inherit | ) |
Sets whether a child actor inherits it's parent's scale.
Default is to inherit. Switching this off means that using SetScale() sets the actor's world scale.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] inherit - true
if the actor should inherit scale,false
otherwise
- Precondition:
- The Actor has been initialized.
void Dali::Actor::SetKeyboardFocusable | ( | bool | focusable | ) |
Sets whether the actor should be focusable by keyboard navigation.
The default is false.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] focusable - true if the actor should be focusable by keyboard navigation, false otherwise
- Precondition:
- The Actor has been initialized.
void Dali::Actor::SetLeaveRequired | ( | bool | required | ) |
Sets whether the actor should receive a notification when touch or hover motion events leave the boundary of the actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] required Should be set to true if a Leave event is required
- Precondition:
- The Actor has been initialized.
- Note:
- By default, this is set to false as most actors do not require this.
- Need to connect to the TouchedSignal() or HoveredSignal() to actually receive this event.
void Dali::Actor::SetMaximumSize | ( | const Vector2 & | size | ) |
Sets the maximum size an actor can be assigned in size negotiation.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] size The maximum size
void Dali::Actor::SetMinimumSize | ( | const Vector2 & | size | ) |
Sets the minimum size an actor can be assigned in size negotiation.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] size The minimum size
void Dali::Actor::SetName | ( | const std::string & | name | ) |
void Dali::Actor::SetOpacity | ( | float | opacity | ) |
Sets the opacity of an actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] opacity The new opacity
- Precondition:
- The actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentOpacity().
void Dali::Actor::SetOrientation | ( | const Degree & | angle, |
const Vector3 & | axis | ||
) |
Sets the orientation of the Actor.
An actor's orientation is the rotation from its default orientation, and the rotation is centered around its anchor-point.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] angle The new orientation angle in degrees [in] axis The new axis of orientation
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentOrientation().
void Dali::Actor::SetOrientation | ( | const Radian & | angle, |
const Vector3 & | axis | ||
) |
Sets the orientation of the Actor.
An actor's orientation is the rotation from its default orientation, and the rotation is centered around its anchor-point.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] angle The new orientation angle in radians [in] axis The new axis of orientation
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentOrientation().
void Dali::Actor::SetOrientation | ( | const Quaternion & | orientation | ) |
Sets the orientation of the Actor.
An actor's orientation is the rotation from its default orientation, and the rotation is centered around its anchor-point.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] orientation The new orientation
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentOrientation().
void Dali::Actor::SetPadding | ( | const Padding & | padding | ) |
Sets the padding for use in layout.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] padding Padding for the actor
void Dali::Actor::SetParentOrigin | ( | const Vector3 & | origin | ) |
Sets the origin of an actor, within its parent's area.
This is expressed in unit coordinates, such that (0.0, 0.0, 0.5) is the top-left corner of the parent, and (1.0, 1.0, 0.5) is the bottom-right corner. The default parent-origin is Dali::ParentOrigin::TOP_LEFT (0.0, 0.0, 0.5). An actor's position is the distance between this origin, and the actor's anchor-point.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] origin The new parent-origin
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentParentOrigin().
- See also:
- Dali::ParentOrigin for predefined parent origin values
void Dali::Actor::SetPosition | ( | float | x, |
float | y | ||
) |
Sets the position of the actor.
The Actor's z position will be set to 0.0f.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] x The new x position [in] y The new y position
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentPosition().
void Dali::Actor::SetPosition | ( | float | x, |
float | y, | ||
float | z | ||
) |
Sets the position of the Actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] x The new x position [in] y The new y position [in] z The new z position
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentPosition().
void Dali::Actor::SetPosition | ( | const Vector3 & | position | ) |
Sets the position of the Actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] position The new position
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentPosition().
Sets the actors position inheritance mode.
- Deprecated:
- Deprecated since 3.0, DALi version 1.1.24 Use SetInheritPosition instead
The default is to inherit. Switching this off means that using SetPosition() sets the actor's world position.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] mode to use
- Precondition:
- The Actor has been initialized.
- See also:
- PositionInheritanceMode
void Dali::Actor::SetResizePolicy | ( | ResizePolicy::Type | policy, |
Dimension::Type | dimension | ||
) |
Sets the resize policy to be used for the given dimension(s).
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] policy The resize policy to use [in] dimension The dimension(s) to set policy for. Can be a bitfield of multiple dimensions
void Dali::Actor::SetScale | ( | float | scale | ) |
Sets the scale factor applied to an actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] scale The scale factor applied on all axes
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentScale().
void Dali::Actor::SetScale | ( | float | scaleX, |
float | scaleY, | ||
float | scaleZ | ||
) |
Sets the scale factor applied to an actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] scaleX The scale factor applied along the x-axis [in] scaleY The scale factor applied along the y-axis [in] scaleZ The scale factor applied along the z-axis
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentScale().
void Dali::Actor::SetScale | ( | const Vector3 & | scale | ) |
Sets the scale factor applied to an actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] scale A vector representing the scale factor for each axis
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentScale().
void Dali::Actor::SetSensitive | ( | bool | sensitive | ) |
Sets whether an actor should emit touch or hover signals.
An actor is sensitive by default, which means that as soon as an application connects to the SignalTouch(), the touch event signal will be emitted, and as soon as an application connects to the SignalHover(), the hover event signal will be emitted.
If the application wishes to temporarily disable the touch or hover event signal emission, then they can do so by calling:
actor.SetSensitive(false);
Then, to re-enable the touch or hover event signal emission, the application should call:
actor.SetSensitive(true);
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] sensitive true to enable emission of the touch or hover event signals, false otherwise
- Precondition:
- The Actor has been initialized.
- Note:
- If an actor's sensitivity is set to false, then it's children will not be hittable either. This is regardless of the individual sensitivity values of the children i.e. an actor will only be hittable if all of its parents have sensitivity set to true.
- See also:
- TouchedSignal() and HoveredSignal().
void Dali::Actor::SetSize | ( | float | width, |
float | height | ||
) |
Sets the size of an actor.
Geometry can be scaled to fit within this area. This does not interfere with the actors scale factor. The actors default depth is the minimum of width & height.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] width The new width [in] height The new height
- Precondition:
- The actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentSize().
void Dali::Actor::SetSize | ( | float | width, |
float | height, | ||
float | depth | ||
) |
Sets the size of an actor.
Geometry can be scaled to fit within this area. This does not interfere with the actors scale factor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] width The size of the actor along the x-axis [in] height The size of the actor along the y-axis [in] depth The size of the actor along the z-axis
- Precondition:
- The actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentSize().
void Dali::Actor::SetSize | ( | const Vector2 & | size | ) |
Sets the size of an actor.
Geometry can be scaled to fit within this area. This does not interfere with the actors scale factor. The actors default depth is the minimum of width & height.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] size The new size
- Precondition:
- The actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentSize().
void Dali::Actor::SetSize | ( | const Vector3 & | size | ) |
Sets the size of an actor.
Geometry can be scaled to fit within this area. This does not interfere with the actors scale factor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] size The new size
- Precondition:
- The actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentSize().
void Dali::Actor::SetSizeModeFactor | ( | const Vector3 & | factor | ) |
Sets the relative to parent size factor of the actor.
This factor is only used when ResizePolicy is set to either: ResizePolicy::SIZE_RELATIVE_TO_PARENT or ResizePolicy::SIZE_FIXED_OFFSET_FROM_PARENT. This actor's size is set to the actor's size multiplied by or added to this factor, depending on ResizePolicy ( See SetResizePolicy() ).
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] factor A Vector3 representing the relative factor to be applied to each axis
- Precondition:
- The Actor has been initialized.
void Dali::Actor::SetSizeScalePolicy | ( | SizeScalePolicy::Type | policy | ) |
Sets the policy to use when setting size with size negotiation. Defaults to SizeScalePolicy::USE_SIZE_SET.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] policy The policy to use for when the size is set
void Dali::Actor::SetVisible | ( | bool | visible | ) |
Sets the visibility flag of an actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] visible The new visibility flag
- Precondition:
- The actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with IsVisible().
- If an actor's visibility flag is set to false, then the actor and its children will not be rendered. This is regardless of the individual visibility values of the children i.e. an actor will only be rendered if all of its parents have visibility set to true.
void Dali::Actor::SetX | ( | float | x | ) |
Sets the position of an actor along the X-axis.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] x The new x position
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentPosition().
void Dali::Actor::SetY | ( | float | y | ) |
Sets the position of an actor along the Y-axis.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] y The new y position
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentPosition().
void Dali::Actor::SetZ | ( | float | z | ) |
Sets the position of an actor along the Z-axis.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] z The new z position
- Precondition:
- The Actor has been initialized.
- Note:
- This is an asynchronous method; the value written may not match a value subsequently read with GetCurrentPosition().
This signal is emitted when touch input is received.
- Deprecated:
- Deprecated since 3.0, DALi version 1.1.37 Use TouchSignal() instead.
A callback of the following type may be connected:
bool YourCallbackName(Actor actor, const TouchEvent& event);
The return value of True, indicates that the touch event should be consumed. Otherwise the signal will be emitted on the next sensitive parent of the actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
- Precondition:
- The Actor has been initialized.
This signal is emitted when touch input is received.
A callback of the following type may be connected:
bool YourCallbackName( Actor actor, TouchData& touch );
The return value of True, indicates that the touch event has been consumed. Otherwise the signal will be emitted on the next sensitive parent of the actor.
- Since:
- 3.0, DALi version 1.1.37
- Returns:
- The signal to connect to
- Precondition:
- The Actor has been initialized.
void Dali::Actor::TranslateBy | ( | const Vector3 & | distance | ) |
Translates an actor relative to its existing position.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] distance The actor will move by this distance
- Precondition:
- The actor has been initialized.
void Dali::Actor::Unparent | ( | ) |
Removes an actor from its parent.
If the actor has no parent, this method does nothing.
- Since:
- 2.4, DALi version 1.0.0
- Precondition:
- The (child) actor has been initialized.
This signal is emitted when wheel event is received.
A callback of the following type may be connected:
bool YourCallbackName(Actor actor, const WheelEvent& event);
The return value of True, indicates that the wheel event should be consumed. Otherwise the signal will be emitted on the next sensitive parent of the actor.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
- Precondition:
- The Actor has been initialized.