Tizen Native API
3.0
|
RenderTasks describe how the Dali scene should be rendered. More...
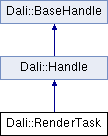
Classes | |
struct | Property |
Enumeration for instances of properties belonging to the RenderTask class. More... | |
Public Types | |
enum | RefreshRate |
Enumeration for the refresh-rate of the RenderTask. More... | |
typedef Signal< void(RenderTask &source) > | RenderTaskSignalType |
Typedef for signals sent by this class. | |
typedef bool(* | ScreenToFrameBufferFunction )(Vector2 &coordinates) |
A pointer to a function for converting screen to frame-buffer coordinates. | |
typedef bool(*const | ConstScreenToFrameBufferFunction )(Vector2 &coordinates) |
A pointer to a function for converting screen to frame-buffer coordinates. | |
Public Member Functions | |
RenderTask () | |
Creates an empty RenderTask handle. | |
~RenderTask () | |
Destructor. | |
RenderTask (const RenderTask &handle) | |
This copy constructor is required for (smart) pointer semantics. | |
RenderTask & | operator= (const RenderTask &rhs) |
This assignment operator is required for (smart) pointer semantics. | |
void | SetSourceActor (Actor actor) |
Sets the actors to be rendered. | |
Actor | GetSourceActor () const |
Retrieves the actors to be rendered. | |
void | SetExclusive (bool exclusive) |
Sets whether the RenderTask has exclusive access to the source actors; the default is false. | |
bool | IsExclusive () const |
Queries whether the RenderTask has exclusive access to the source actors. | |
void | SetInputEnabled (bool enabled) |
Sets whether the render-task should be considered for input handling; the default is true. | |
bool | GetInputEnabled () const |
Queries whether the render-task should be considered for input handling. | |
void | SetCameraActor (CameraActor cameraActor) |
Sets the actor from which the scene is viewed. | |
CameraActor | GetCameraActor () const |
Retrieves the actor from which the scene is viewed. | |
void | SetTargetFrameBuffer (FrameBufferImage frameBuffer) |
Sets the frame-buffer used as a render target. | |
FrameBufferImage | GetTargetFrameBuffer () const |
Retrieves the frame-buffer used as a render target. | |
void | SetFrameBuffer (FrameBuffer frameBuffer) |
Sets the frame-buffer used as a render target. | |
FrameBuffer | GetFrameBuffer () const |
Retrieves the frame-buffer used as a render target. | |
void | SetScreenToFrameBufferFunction (ScreenToFrameBufferFunction conversionFunction) |
Sets the function used to convert screen coordinates to frame-buffer coordinates. | |
ScreenToFrameBufferFunction | GetScreenToFrameBufferFunction () const |
Retrieves the function used to convert screen coordinates to frame-buffer coordinates. | |
void | SetScreenToFrameBufferMappingActor (Actor mappingActor) |
Sets the actor used to convert screen coordinates to frame-buffer coordinates. | |
Actor | GetScreenToFrameBufferMappingActor () const |
Retrieves the actor used to convert screen coordinates to frame-buffer coordinates. | |
void | SetViewportPosition (Vector2 position) |
Sets the GL viewport position used when rendering. | |
Vector2 | GetCurrentViewportPosition () const |
Retrieves the GL viewport position used when rendering. | |
void | SetViewportSize (Vector2 size) |
Sets the GL viewport size used when rendering. | |
Vector2 | GetCurrentViewportSize () const |
Retrieves the GL viewport size used when rendering. | |
void | SetViewport (Viewport viewport) |
Sets the GL viewport used when rendering. | |
Viewport | GetViewport () const |
Retrieves the GL viewport used when rendering. | |
void | SetClearColor (const Vector4 &color) |
Sets the clear color used when SetClearEnabled(true) is used. | |
Vector4 | GetClearColor () const |
Retrieves the clear color used when SetClearEnabled(true) is used. | |
void | SetClearEnabled (bool enabled) |
Sets whether the render-task will clear the results of previous render-tasks. | |
bool | GetClearEnabled () const |
Queries whether the render-task will clear the results of previous render-tasks. | |
void | SetCullMode (bool cullMode) |
Sets whether the render task will cull the actors to the camera's view frustum. | |
bool | GetCullMode () const |
Gets the cull mode. | |
void | SetRefreshRate (unsigned int refreshRate) |
Sets the refresh-rate of the RenderTask. | |
unsigned int | GetRefreshRate () const |
Queries the refresh-rate of the RenderTask. | |
RenderTaskSignalType & | FinishedSignal () |
If the refresh rate is REFRESH_ONCE, connect to this signal to be notified when a RenderTask has finished. | |
Static Public Member Functions | |
static RenderTask | DownCast (BaseHandle handle) |
Downcasts a handle to RenderTask handle. | |
Static Public Attributes | |
static ConstScreenToFrameBufferFunction | DEFAULT_SCREEN_TO_FRAMEBUFFER_FUNCTION |
The default conversion function returns false for any screen coordinates. | |
static ConstScreenToFrameBufferFunction | FULLSCREEN_FRAMEBUFFER_FUNCTION |
This conversion function outputs the (unmodified) screen coordinates as frame-buffer coordinates. | |
static const bool | DEFAULT_EXCLUSIVE |
false | |
static const bool | DEFAULT_INPUT_ENABLED |
true | |
static const Vector4 | DEFAULT_CLEAR_COLOR |
Color::BLACK. | |
static const bool | DEFAULT_CLEAR_ENABLED |
false | |
static const bool | DEFAULT_CULL_MODE |
true | |
static const unsigned int | DEFAULT_REFRESH_RATE |
REFRESH_ALWAYS. |
Detailed Description
RenderTasks describe how the Dali scene should be rendered.
The Stage::GetRenderTaskList() method provides access to an ordered list of render-tasks.
Each RenderTask must specify the source actors to be rendered, and a camera actor from which the scene is viewed.
RenderTasks may optionally target a frame-buffer, otherwise the default GL surface is used; typically this is a window provided by the native system.
By default Dali provides a single RenderTask, which renders the entire actor hierachy using a default camera actor and GL surface. If stereoscopic rendering is enabled, Dali will create two additional render tasks, on for each eye. Each render task will have its own camera parented to the default camera actor.
The first RenderTask used for input handling will be the last one rendered, which also has input enabled, and has a valid source & camera actor; see SetInputEnabled().
If none of the actors are hit in the last RenderTask rendered, then input handling will continue with the second last RenderTask rendered, and so on.
All RenderTasks which target a frame-buffer (i.e. off screen) will be rendered before all RenderTasks which target the default GL surface. This allows the user to render intermediate targets which are used later when targeting the screen.
A RenderTask targeting a frame-buffer can still be hit-tested, provided that the screen->frame-buffer coordinate conversion is successful; see SetScreenToFrameBufferFunction().
If the refresh rate id REFRESH_ONCE and a "Finish" signal is connected, it will be emitted when the RenderTask is completed. Note that all connected signals must be disconnected before the object is destroyed. This is typically done in the object destructor, and requires either the Dali::Connection object or Dali::RenderTask handle to be stored.
Signals | Signal Name | Method | |--------------|-----------------------| | finished | FinishedSignal() |
- Since:
- 2.4, DALi version 1.0.0
Member Typedef Documentation
typedef bool(* const Dali::RenderTask::ConstScreenToFrameBufferFunction)(Vector2 &coordinates) |
A pointer to a function for converting screen to frame-buffer coordinates.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in,out] coordinates The screen coordinates to convert where (0,0) is the top-left of the screen
- Returns:
- True if the conversion was successful, otherwise coordinates should be unmodified
typedef Signal< void (RenderTask& source) > Dali::RenderTask::RenderTaskSignalType |
Typedef for signals sent by this class.
- Since:
- 2.4, DALi version 1.0.0
typedef bool(* Dali::RenderTask::ScreenToFrameBufferFunction)(Vector2 &coordinates) |
A pointer to a function for converting screen to frame-buffer coordinates.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in,out] coordinates The screen coordinates to convert where (0,0) is the top-left of the screen
- Returns:
- True if the conversion was successful, otherwise coordinates should be unmodified
Member Enumeration Documentation
Enumeration for the refresh-rate of the RenderTask.
- Since:
- 2.4, DALi version 1.0.0
Constructor & Destructor Documentation
Creates an empty RenderTask handle.
This can be initialised with RenderTaskList::CreateRenderTask().
- Since:
- 2.4, DALi version 1.0.0
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 2.4, DALi version 1.0.0
Dali::RenderTask::RenderTask | ( | const RenderTask & | handle | ) |
This copy constructor is required for (smart) pointer semantics.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle A reference to the copied handle
Member Function Documentation
static RenderTask Dali::RenderTask::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcasts a handle to RenderTask handle.
If handle points to a RenderTask, the downcast produces valid handle. If not, the returned handle is left uninitialized.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle A handle to an object
- Returns:
- A handle to a RenderTask or an uninitialized handle
Reimplemented from Dali::Handle.
If the refresh rate is REFRESH_ONCE, connect to this signal to be notified when a RenderTask has finished.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to
CameraActor Dali::RenderTask::GetCameraActor | ( | ) | const |
Retrieves the actor from which the scene is viewed.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The scene is viewed from the perspective of this actor
Vector4 Dali::RenderTask::GetClearColor | ( | ) | const |
Retrieves the clear color used when SetClearEnabled(true) is used.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The clear color
- Note:
- This property can be animated; the return value may not match the value written with SetClearColor().
bool Dali::RenderTask::GetClearEnabled | ( | ) | const |
Queries whether the render-task will clear the results of previous render-tasks.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- True if the render-task should clear
bool Dali::RenderTask::GetCullMode | ( | ) | const |
Gets the cull mode.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- True if the render task should cull the actors to the camera's view frustum
Retrieves the GL viewport position used when rendering.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The viewport
Retrieves the GL viewport size used when rendering.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The viewport
FrameBuffer Dali::RenderTask::GetFrameBuffer | ( | ) | const |
Retrieves the frame-buffer used as a render target.
- Since:
- 3.0, DALi version 1.1.38
- Returns:
- The framebuffer
bool Dali::RenderTask::GetInputEnabled | ( | ) | const |
Queries whether the render-task should be considered for input handling.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- True if the render-task should be considered for input handling
unsigned int Dali::RenderTask::GetRefreshRate | ( | ) | const |
Retrieves the function used to convert screen coordinates to frame-buffer coordinates.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The conversion function
Retrieves the actor used to convert screen coordinates to frame-buffer coordinates.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The actor used for conversion
Actor Dali::RenderTask::GetSourceActor | ( | ) | const |
Retrieves the actors to be rendered.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- This actor and its children will be rendered
Retrieves the frame-buffer used as a render target.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- A valid frame-buffer handle, or an uninitialised handle if off-screen rendering is disabled
Viewport Dali::RenderTask::GetViewport | ( | ) | const |
Retrieves the GL viewport used when rendering.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The viewport
bool Dali::RenderTask::IsExclusive | ( | ) | const |
Queries whether the RenderTask has exclusive access to the source actors.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- True if the source actors will only be rendered by this render-task
RenderTask& Dali::RenderTask::operator= | ( | const RenderTask & | rhs | ) |
This assignment operator is required for (smart) pointer semantics.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] rhs A reference to the copied handle
- Returns:
- A reference to this
void Dali::RenderTask::SetCameraActor | ( | CameraActor | cameraActor | ) |
Sets the actor from which the scene is viewed.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] cameraActor The scene is viewed from the perspective of this actor
void Dali::RenderTask::SetClearColor | ( | const Vector4 & | color | ) |
Sets the clear color used when SetClearEnabled(true) is used.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] color The new clear color
void Dali::RenderTask::SetClearEnabled | ( | bool | enabled | ) |
Sets whether the render-task will clear the results of previous render-tasks.
The default is false.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] enabled True if the render-task should clear
- Note:
- The default GL surface is cleared automatically at the beginning of each frame; this setting is only useful when 2+ render-tasks are used, and the result of the first task needs to be (partially) cleared before rendering the second.
void Dali::RenderTask::SetCullMode | ( | bool | cullMode | ) |
Sets whether the render task will cull the actors to the camera's view frustum.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] cullMode True if the renderers should be culled
- Note:
- The default mode is to cull actors.
- If the shader uses Shader::Hint::MODIFIES_GEOMETRY then culling optimizations are disabled.
- See also:
- Shader::Hint
void Dali::RenderTask::SetExclusive | ( | bool | exclusive | ) |
Sets whether the RenderTask has exclusive access to the source actors; the default is false.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] exclusive True if the source actors will only be rendered by this render-task
void Dali::RenderTask::SetFrameBuffer | ( | FrameBuffer | frameBuffer | ) |
Sets the frame-buffer used as a render target.
- Since:
- 3.0, DALi version 1.1.38
- Parameters:
-
[in] frameBuffer er A valid FrameBuffer handle to enable off-screen rendering, or an uninitialized handle to disable it
void Dali::RenderTask::SetInputEnabled | ( | bool | enabled | ) |
Sets whether the render-task should be considered for input handling; the default is true.
The task used for input handling will be last task in the RenderTaskList which has input enabled, and has a valid source & camera actor. A RenderTask targetting a frame-buffer can still be hit-tested, provided that the screen->frame-buffer coordinate conversion is successful; see also SetScreenToFrameBufferFunction().
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] enabled True if the render-task should be considered for input handling
void Dali::RenderTask::SetRefreshRate | ( | unsigned int | refreshRate | ) |
Sets the refresh-rate of the RenderTask.
The default is REFRESH_ALWAYS (1), meaning that the RenderTask will be processed every frame if the scene graph is changing. It may be desirable to process less frequently. For example, SetRefreshRate(3) will process once every 3 frames if the scene graph is changing. If the scene graph is not changing, then the render task will not be rendered, regardless of this value.
The REFRESH_ONCE value means that the RenderTask will be processed once only, to take a snap-shot of the scene. Repeatedly calling SetRefreshRate(REFRESH_ONCE) will cause more snap-shots to be taken.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] refreshRate The new refresh rate
void Dali::RenderTask::SetScreenToFrameBufferFunction | ( | ScreenToFrameBufferFunction | conversionFunction | ) |
Sets the function used to convert screen coordinates to frame-buffer coordinates.
This is useful for hit-testing actors which are rendered off-screen.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] conversionFunction The conversion function
void Dali::RenderTask::SetScreenToFrameBufferMappingActor | ( | Actor | mappingActor | ) |
Sets the actor used to convert screen coordinates to frame-buffer coordinates.
The local coordinates of the actor are mapped as frame-buffer coordinates. This is useful for hit-testing actors which are rendered off-screen.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] mappingActor The actor used for conversion
- Note:
- The mapping actor needs to be rendered by the default render task to make the mapping work properly.
void Dali::RenderTask::SetSourceActor | ( | Actor | actor | ) |
Sets the actors to be rendered.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] actor This actor and its children will be rendered. If actor is an empty handle, then nothing will be rendered
void Dali::RenderTask::SetTargetFrameBuffer | ( | FrameBufferImage | frameBuffer | ) |
Sets the frame-buffer used as a render target.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] frameBuffer A valid frame-buffer handle to enable off-screen rendering, or an uninitialized handle to disable
void Dali::RenderTask::SetViewport | ( | Viewport | viewport | ) |
Sets the GL viewport used when rendering.
This specifies the transformation between normalized device coordinates and target window (or frame-buffer) coordinates. By default this will match the target window or frame-buffer size.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] viewport The new viewport
- Note:
- Unlike the glViewport method, the x & y coordinates refer to the top-left of the viewport rectangle.
void Dali::RenderTask::SetViewportPosition | ( | Vector2 | position | ) |
Sets the GL viewport position used when rendering.
This specifies the transformation between normalized device coordinates and target window (or frame-buffer) coordinates. By default this will match the target window or frame-buffer size.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] position The viewports position (x,y)
- Note:
- Unlike the glViewport method, the x & y coordinates refer to the top-left of the viewport rectangle.
void Dali::RenderTask::SetViewportSize | ( | Vector2 | size | ) |
Sets the GL viewport size used when rendering.
This specifies the transformation between normalized device coordinates and target window (or frame-buffer) coordinates. By default this will match the target window or frame-buffer size.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] size The viewports size (width,height)
Member Data Documentation
The default conversion function returns false for any screen coordinates.
This effectively disables hit-testing for RenderTasks rendering to a frame buffer. See also FULLSCREEN_FRAMEBUFFER_FUNCTION below.
This conversion function outputs the (unmodified) screen coordinates as frame-buffer coordinates.
Therefore the contents of an off-screen image is expected to be rendered "full screen".