Tizen Native API
3.0
|
ItemView is a scrollable layout container. More...
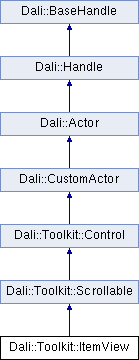
Classes | |
struct | Property |
Enumeration for the instance of properties belonging to the ScrollView class. More... | |
Public Types | |
enum | PropertyRange |
Enumeration for the start and end property ranges for this control. More... | |
Public Member Functions | |
ItemView () | |
Creates an uninitialized ItemView; this can be initialized with ItemView::New(). | |
ItemView (const ItemView &itemView) | |
Copy constructor. | |
ItemView & | operator= (const ItemView &itemView) |
Assignment operator. | |
~ItemView () | |
Destructor. | |
unsigned int | GetLayoutCount () const |
Queries the number of layouts. | |
void | AddLayout (ItemLayout &layout) |
Adds a layout. | |
void | RemoveLayout (unsigned int layoutIndex) |
Removes a layout. | |
ItemLayoutPtr | GetLayout (unsigned int layoutIndex) const |
Retrieves a layout. | |
ItemLayoutPtr | GetActiveLayout () const |
Retrieves the currently active layout, if any. | |
float | GetCurrentLayoutPosition (ItemId itemId) const |
Retrieves the current layout-position of an item in the ItemView. | |
void | ActivateLayout (unsigned int layoutIndex, Vector3 targetSize, float durationSeconds) |
Activates one of the layouts; this will resize the ItemView & relayout actors within the ItemView. | |
void | DeactivateCurrentLayout () |
Deactivates the current layout, if any. | |
void | SetMinimumSwipeSpeed (float speed) |
Sets the minimum swipe speed in pixels per second; A pan gesture must exceed this to trigger a swipe. | |
float | GetMinimumSwipeSpeed () const |
Gets the minimum swipe speed in pixels per second. | |
void | SetMinimumSwipeDistance (float distance) |
Sets the minimum swipe distance in actor coordinates; A pan gesture must exceed this to trigger a swipe. | |
float | GetMinimumSwipeDistance () const |
Gets the minimum swipe distance in actor coordinates. | |
void | SetWheelScrollDistanceStep (float step) |
Set the step of scroll distance in actor coordinates for each wheel event received. | |
float | GetWheelScrollDistanceStep () const |
Get the step of scroll distance in actor coordinates for each wheel event received. | |
void | SetAnchoring (bool enabled) |
Set whether to enable the animation for the layout to scroll to its anchor position after dragging or swiping. | |
bool | GetAnchoring () const |
Get whether the anchor animation is enabled or not. | |
void | SetAnchoringDuration (float durationSeconds) |
Set the duration of the anchor animation in seconds. | |
float | GetAnchoringDuration () const |
Get the duration of the anchor animation in seconds. | |
void | ScrollToItem (ItemId itemId, float durationSeconds) |
Scroll the current layout to a particular item. | |
void | SetRefreshInterval (float intervalLayoutPositions) |
Set the interval between refreshes. | |
float | GetRefreshInterval () const |
Get the interval between refreshes in layout position. | |
void | Refresh () |
Do a refresh of the item view. | |
Actor | GetItem (ItemId itemId) const |
Given the Item ID, this returns the accompanying actor. | |
ItemId | GetItemId (Actor actor) const |
Returns the Item ID of the specified actor. | |
void | InsertItem (Item newItem, float durationSeconds) |
Insert an item. | |
void | InsertItems (const ItemContainer &newItems, float durationSeconds) |
Insert a set of items; this is more efficient than calling InsertItem() repeatedly. | |
void | RemoveItem (ItemId itemId, float durationSeconds) |
Removes an item with the given ID. | |
void | RemoveItems (const ItemIdContainer &itemIds, float durationSeconds) |
Remove a set of items; this is more efficient than calling RemoveItem() repeatedly. | |
void | ReplaceItem (Item replacementItem, float durationSeconds) |
Replace an item. | |
void | ReplaceItems (const ItemContainer &replacementItems, float durationSeconds) |
Replace a set of items. | |
void | SetItemsParentOrigin (const Vector3 &parentOrigin) |
Set the parent origin of the items. | |
Vector3 | GetItemsParentOrigin () const |
Get the parent origin of the items. | |
void | SetItemsAnchorPoint (const Vector3 &anchorPoint) |
Set the anchor point of the items. | |
Vector3 | GetItemsAnchorPoint () const |
Get the anchor point of the items. | |
void | GetItemsRange (ItemRange &range) |
Get the range of items that are currently in ItemView. | |
ItemView::LayoutActivatedSignalType & | LayoutActivatedSignal () |
Signal emitted when layout activation is finished. | |
Static Public Member Functions | |
static ItemView | New (ItemFactory &factory) |
Creates an initialized ItemView. | |
static ItemView | DownCast (BaseHandle handle) |
Downcasts a handle to ItemView handle. |
Detailed Description
ItemView is a scrollable layout container.
Multiple ItemLayouts may be provided to determine the logical position of each item layout. Actors are provided from an external ItemFactory to display the currently visible items.
Signals | Signal Name | Method | |---------------------------------|--------------------------------------------| | layoutActivated | LayoutActivatedSignal() |
- Since:
- 2.4, DALi version 1.0.0
Actions | Action Name | Attributes | Description | |---------------|-------------------------|-------------------------------------------------| | stopScrolling | Doesn't have attributes | Stops the scroll animation. See DoAction() |
- Since:
- 3.0, DALi version 1.1.33
Member Enumeration Documentation
Enumeration for the start and end property ranges for this control.
- Since:
- 3.0, DALi version 1.1.18
- Enumerator:
Reimplemented from Dali::Toolkit::Scrollable.
Constructor & Destructor Documentation
Creates an uninitialized ItemView; this can be initialized with ItemView::New().
Calling member functions with an uninitialized Dali::Object is not allowed.
- Since:
- 2.4, DALi version 1.0.0
Dali::Toolkit::ItemView::ItemView | ( | const ItemView & | itemView | ) |
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 2.4, DALi version 1.0.0
Member Function Documentation
void Dali::Toolkit::ItemView::ActivateLayout | ( | unsigned int | layoutIndex, |
Vector3 | targetSize, | ||
float | durationSeconds | ||
) |
Activates one of the layouts; this will resize the ItemView & relayout actors within the ItemView.
This is done by applying constraints from the new layout, and removing constraints from the previous layout.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] layoutIndex The index of one of the ItemView layouts [in] targetSize The target ItemView & layout size [in] durationSeconds The time taken to relayout in seconds (zero for immediate)
- Precondition:
- layoutIndex is less than GetLayoutCount().
- durationSeconds is greater or equal to zero.
void Dali::Toolkit::ItemView::AddLayout | ( | ItemLayout & | layout | ) |
Adds a layout.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] layout The layout
Deactivates the current layout, if any.
The constraints applied by the layout will be removed.
- Since:
- 2.4, DALi version 1.0.0
static ItemView Dali::Toolkit::ItemView::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcasts a handle to ItemView handle.
If handle points to a ItemView, the downcast produces valid handle. If not, the returned handle is left uninitialized.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] handle Handle to an object
- Returns:
- A handle to a ItemView or an uninitialized handle
Reimplemented from Dali::Toolkit::Scrollable.
Retrieves the currently active layout, if any.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The layout, or an uninitialized pointer if no layout is active
bool Dali::Toolkit::ItemView::GetAnchoring | ( | ) | const |
Get whether the anchor animation is enabled or not.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- Whether the anchor animation is enabled or not.
float Dali::Toolkit::ItemView::GetAnchoringDuration | ( | ) | const |
Get the duration of the anchor animation in seconds.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The duration of the anchor animation
float Dali::Toolkit::ItemView::GetCurrentLayoutPosition | ( | ItemId | itemId | ) | const |
Retrieves the current layout-position of an item in the ItemView.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] itemId The item identifier
- Returns:
- The current layout-position
Actor Dali::Toolkit::ItemView::GetItem | ( | ItemId | itemId | ) | const |
Given the Item ID, this returns the accompanying actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] itemId The Item ID of the actor required.
- Returns:
- The Actor corresponding to the Item ID.
ItemId Dali::Toolkit::ItemView::GetItemId | ( | Actor | actor | ) | const |
Returns the Item ID of the specified actor.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] actor The actor whose Item ID is required.
- Returns:
- The Item ID of the item.
- Precondition:
- The actor should be an item of ItemView.
Get the anchor point of the items.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The current anchor point of the items
Get the parent origin of the items.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The current parent origin of the items
void Dali::Toolkit::ItemView::GetItemsRange | ( | ItemRange & | range | ) |
Get the range of items that are currently in ItemView.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[out] range The range of items.
ItemLayoutPtr Dali::Toolkit::ItemView::GetLayout | ( | unsigned int | layoutIndex | ) | const |
Retrieves a layout.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] layoutIndex The index of the layout to retrieve
- Returns:
- The layout
- Precondition:
- layoutIndex is less than GetLayoutCount().
unsigned int Dali::Toolkit::ItemView::GetLayoutCount | ( | ) | const |
Queries the number of layouts.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The number of layouts
float Dali::Toolkit::ItemView::GetMinimumSwipeDistance | ( | ) | const |
Gets the minimum swipe distance in actor coordinates.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The minimum swipe distance
float Dali::Toolkit::ItemView::GetMinimumSwipeSpeed | ( | ) | const |
Gets the minimum swipe speed in pixels per second.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The minimum swipe speed
float Dali::Toolkit::ItemView::GetRefreshInterval | ( | ) | const |
Get the interval between refreshes in layout position.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The refresh interval
float Dali::Toolkit::ItemView::GetWheelScrollDistanceStep | ( | ) | const |
Get the step of scroll distance in actor coordinates for each wheel event received.
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The step of scroll distance(pixel)
void Dali::Toolkit::ItemView::InsertItem | ( | Item | newItem, |
float | durationSeconds | ||
) |
Insert an item.
A relayout will occur for the existing actors; for example if InsertItem(Item(2, ActorZ), 0) is called, the items with ID 2 or greater will be moved: Initial actors: After insert: ID 1 - ActorA ID 1 - ActorA ID 2 - ActorB ID 2 - ActorZ ! ID 3 - ActorC ID 3 - ActorB ID 4 - ActorC
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] newItem The item to insert. [in] durationSeconds How long the relayout takes in seconds.
- Precondition:
- durationSeconds must be zero or greater; zero means the relayout occurs instantly.
void Dali::Toolkit::ItemView::InsertItems | ( | const ItemContainer & | newItems, |
float | durationSeconds | ||
) |
Insert a set of items; this is more efficient than calling InsertItem() repeatedly.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] newItems The items to insert. [in] durationSeconds How long the relayout takes in seconds.
- Precondition:
- durationSeconds must be zero or greater; zero means the relayout occurs instantly.
Signal emitted when layout activation is finished.
A callback of the following type may be connected:
void YourCallbackName();
- Since:
- 2.4, DALi version 1.0.0
- Returns:
- The signal to connect to.
- Precondition:
- The Object has been initialized.
static ItemView Dali::Toolkit::ItemView::New | ( | ItemFactory & | factory | ) | [static] |
Assignment operator.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] itemView Handle to an object
- Returns:
- A reference to this
void Dali::Toolkit::ItemView::Refresh | ( | ) |
Do a refresh of the item view.
- Since:
- 2.4, DALi version 1.0.0
void Dali::Toolkit::ItemView::RemoveItem | ( | ItemId | itemId, |
float | durationSeconds | ||
) |
Removes an item with the given ID.
A relayout will occur for the remaining actors; for example if RemoveItem(Item(2, ActorZ), 0) is called, the items with ID 3 or greater will be moved: | Initial actors: | After remove: | |:------------------ |:-------------- | | ID 1 - ActorA | ID 1 - ActorA | | ID 2 - ActorB | ID 2 - ActorC (previously ID 3) | | ID 3 - ActorC | ID 3 - ActorB (previously ID 4) | | ID 4 - ActorD | |
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] itemId The Item ID of the item to remove. [in] durationSeconds How long the relayout takes in seconds.
- Precondition:
- durationSeconds must be zero or greater; zero means the relayout occurs instantly.
void Dali::Toolkit::ItemView::RemoveItems | ( | const ItemIdContainer & | itemIds, |
float | durationSeconds | ||
) |
Remove a set of items; this is more efficient than calling RemoveItem() repeatedly.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] itemIds The IDs of the items to remove. [in] durationSeconds How long the relayout takes in seconds.
- Precondition:
- durationSeconds must be zero or greater; zero means the relayout occurs instantly.
void Dali::Toolkit::ItemView::RemoveLayout | ( | unsigned int | layoutIndex | ) |
Removes a layout.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] layoutIndex The index of one of the ItemView layouts
- Precondition:
- layoutIndex is less than GetLayoutCount().
void Dali::Toolkit::ItemView::ReplaceItem | ( | Item | replacementItem, |
float | durationSeconds | ||
) |
Replace an item.
A relayout will occur for the replacement item only.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] replacementItem The replacement for an existing item. [in] durationSeconds How long the relayout takes in seconds.
- Precondition:
- durationSeconds must be zero or greater; zero means the relayout occurs instantly.
void Dali::Toolkit::ItemView::ReplaceItems | ( | const ItemContainer & | replacementItems, |
float | durationSeconds | ||
) |
Replace a set of items.
A relayout will occur for the replacement items only.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] replacementItems The replacements for a set of existing items. [in] durationSeconds How long the relayout takes in seconds.
- Precondition:
- durationSeconds must be zero or greater; zero means the relayout occurs instantly.
void Dali::Toolkit::ItemView::ScrollToItem | ( | ItemId | itemId, |
float | durationSeconds | ||
) |
Scroll the current layout to a particular item.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] itemId The ID of an item in the layout. [in] durationSeconds How long the scrolling takes in seconds.
- Precondition:
- durationSeconds must be zero or greater; zero means the layout should scroll to the particular item instantly. If calling this with zero second of duration immediately after calling ActivateLayout, it might not work unless the duration of relayout animation for ActivateLayout is also set to be zero.
void Dali::Toolkit::ItemView::SetAnchoring | ( | bool | enabled | ) |
Set whether to enable the animation for the layout to scroll to its anchor position after dragging or swiping.
The anchor position is the position where all the items in the layout are aligned to their closest rounded layout positions in integer.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] enabled Whether the anchor animation is enabled or not.
void Dali::Toolkit::ItemView::SetAnchoringDuration | ( | float | durationSeconds | ) |
Set the duration of the anchor animation in seconds.
This is the time taken to reach the nearest anchor position after a drag or swipe gesture ends.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] durationSeconds The duration of the anchor animation in seconds.
- Precondition:
- durationSeconds must be greater than zero.
void Dali::Toolkit::ItemView::SetItemsAnchorPoint | ( | const Vector3 & | anchorPoint | ) |
Set the anchor point of the items.
A relayout will occur for all the items if the anchor point is different than the current one.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] anchorPoint New anchor point position vector
void Dali::Toolkit::ItemView::SetItemsParentOrigin | ( | const Vector3 & | parentOrigin | ) |
Set the parent origin of the items.
A relayout will occur for all the items if the parent origin is different than the current one.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] parentOrigin New parent origin position vector
void Dali::Toolkit::ItemView::SetMinimumSwipeDistance | ( | float | distance | ) |
Sets the minimum swipe distance in actor coordinates; A pan gesture must exceed this to trigger a swipe.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] distance The minimum swipe distance
void Dali::Toolkit::ItemView::SetMinimumSwipeSpeed | ( | float | speed | ) |
Sets the minimum swipe speed in pixels per second; A pan gesture must exceed this to trigger a swipe.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] speed The minimum swipe speed
void Dali::Toolkit::ItemView::SetRefreshInterval | ( | float | intervalLayoutPositions | ) |
Set the interval between refreshes.
When the layout-position of items is changed by this interval, new items are requested from ItemFactory.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] intervalLayoutPositions The refresh interval in layout position.
void Dali::Toolkit::ItemView::SetWheelScrollDistanceStep | ( | float | step | ) |
Set the step of scroll distance in actor coordinates for each wheel event received.
- Since:
- 2.4, DALi version 1.0.0
- Parameters:
-
[in] step The step of scroll distance(pixel).