Tizen Native API
5.5
|
An Application class object should be created by every application that wishes to use Dali. More...
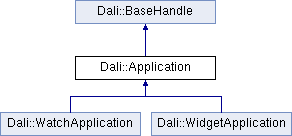
Public Types | |
enum | WINDOW_MODE |
Enumeration for deciding whether a Dali application window is opaque or transparent. More... | |
typedef Signal< void(DeviceStatus::Battery::Status) > | LowBatterySignalType |
Application device signal type. | |
typedef Signal< void(DeviceStatus::Memory::Status) > | LowMemorySignalType |
Application device signal type. | |
typedef Signal< void(Application &) > | AppSignalType |
Application lifecycle signal and system signal callback type. | |
typedef Signal< void(Application &, void *) | AppControlSignalType ) |
Application control signal callback type. | |
Public Member Functions | |
Application () | |
Constructs an empty handle. | |
Application (const Application &application) | |
Copy Constructor. | |
Application & | operator= (const Application &application) |
Assignment operator. | |
~Application () | |
Destructor. | |
void | MainLoop () |
This starts the application. | |
void | MainLoop (Configuration::ContextLoss configuration) |
This starts the application, and allows the app to choose a different configuration. | |
void | Lower () |
This lowers the application to bottom without actually quitting it. | |
void | Quit () |
This quits the application. Tizen applications should use Lower to improve re-start performance unless they need to Quit completely. | |
bool | AddIdle (CallbackBase *callback) |
Ensures that the function passed in is called from the main loop when it is idle. | |
Window | GetWindow () |
Retrieves the main window used by the Application class. | |
void | ReplaceWindow (PositionSize windowPosition, const std::string &name) DALI_DEPRECATED_API |
Replaces the current window. | |
std::string | GetRegion () const |
This is used to get region information from device. | |
std::string | GetLanguage () const |
This is used to get language information from device. | |
void | SetViewMode (ViewMode viewMode) |
Sets the viewing mode for the application. | |
ViewMode | GetViewMode () const |
Gets the current viewing mode. | |
void | SetStereoBase (float stereoBase) |
Sets the stereo base (eye separation) for Stereoscopic 3D. | |
float | GetStereoBase () const |
Gets the stereo base (eye separation) for Stereoscopic 3D. | |
AppSignalType & | InitSignal () |
The user should connect to this signal to determine when they should initialize their application. | |
AppSignalType & | TerminateSignal () |
The user should connect to this signal to determine when they should terminate their application. | |
AppSignalType & | PauseSignal () |
The user should connect to this signal if they need to perform any special activities when the application is about to be paused. | |
AppSignalType & | ResumeSignal () |
The user should connect to this signal if they need to perform any special activities when the application has resumed. | |
AppSignalType & | ResetSignal () |
This signal is sent when the system requires the user to reinitialize itself. | |
AppSignalType & | ResizeSignal () DALI_DEPRECATED_API |
This signal is emitted when the window application rendering on is resized. | |
AppControlSignalType & | AppControlSignal () |
This signal is emitted when another application sends a launch request to the application. | |
AppSignalType & | LanguageChangedSignal () |
This signal is emitted when the language is changed on the device. | |
AppSignalType & | RegionChangedSignal () |
This signal is emitted when the region of the device is changed. | |
AppSignalType & | BatteryLowSignal () DALI_DEPRECATED_API |
This signal is emitted when the battery level of the device is low. | |
AppSignalType & | MemoryLowSignal () DALI_DEPRECATED_API |
This signal is emitted when the memory level of the device is low. | |
LowBatterySignalType & | LowBatterySignal () |
This signal is emitted when the battery level of the device is low. | |
LowMemorySignalType & | LowMemorySignal () |
This signal is emitted when the memory level of the device is low. | |
Static Public Member Functions | |
static Application | New () |
This is the constructor for applications without an argument list. | |
static Application | New (int *argc, char **argv[]) |
This is the constructor for applications. | |
static Application | New (int *argc, char **argv[], const std::string &stylesheet) |
This is the constructor for applications with a name. | |
static Application | New (int *argc, char **argv[], const std::string &stylesheet, WINDOW_MODE windowMode) |
This is the constructor for applications with a name. | |
static Application | New (int *argc, char **argv[], const std::string &stylesheet, Application::WINDOW_MODE windowMode, PositionSize positionSize) |
This is the constructor for applications. | |
static std::string | GetResourcePath () |
Get path application resources are stored at. |
Detailed Description
An Application class object should be created by every application that wishes to use Dali.
It provides a means for initializing the resources required by the Dali::Core.
The Application class emits several signals which the user can connect to. The user should not create any Dali objects in the main function and instead should connect to the Init signal of the Application and create the Dali objects in the connected callback.
Applications should follow the example below:
class ExampleController: public ConnectionTracker { public: ExampleController( Application& application ) : mApplication( application ) { mApplication.InitSignal().Connect( this, &ExampleController::Create ); } void Create( Application& application ) { // Create Dali components... } ... private: Application& mApplication; }; int main (int argc, char **argv) { Application app = Application::New(&argc, &argv); ExampleController example( app ); app.MainLoop(); }
If required, you can also connect class member functions to a signal:
MyApplication app; app.ResumeSignal().Connect(&app, &MyApplication::Resume);
This class accepts command line arguments as well. The following options are supported:
-w|--width Stage Width -h|--height Stage Height -d|--dpi Emulated DPI --help Help
When the above options are found, they are stripped from argv, and argc is updated appropriately.
- Since:
- 3.0, DALi version 1.0.0
Member Typedef Documentation
typedef Signal< void (Application&, void *) Dali::Application::AppControlSignalType) |
Application control signal callback type.
- Since:
- 3.0, DALi version 1.0.0
typedef Signal< void (Application&) > Dali::Application::AppSignalType |
Application lifecycle signal and system signal callback type.
- Since:
- 3.0, DALi version 1.0.0
typedef Signal< void (DeviceStatus::Battery::Status) > Dali::Application::LowBatterySignalType |
Application device signal type.
- Since:
- 4.0, DALi version 1.2.62
typedef Signal< void (DeviceStatus::Memory::Status) > Dali::Application::LowMemorySignalType |
Application device signal type.
- Since:
- 4.0, DALi version 1.2.62
Member Enumeration Documentation
Enumeration for deciding whether a Dali application window is opaque or transparent.
- Since:
- 3.0, DALi version 1.0.0
Constructor & Destructor Documentation
Constructs an empty handle.
- Since:
- 3.0, DALi version 1.0.0
Dali::Application::Application | ( | const Application & | application | ) |
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 3.0, DALi version 1.0.0
Member Function Documentation
bool Dali::Application::AddIdle | ( | CallbackBase * | callback | ) |
Ensures that the function passed in is called from the main loop when it is idle.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] callback The function to call
- Returns:
true
if added successfully,false
otherwise
- Note:
- Function must be called from main event thread only
A callback of the following type may be used:
void MyFunction();
This callback will be deleted once it is called.
- Note:
- Ownership of the callback is passed onto this class.
This signal is emitted when another application sends a launch request to the application.
When the application is launched, this signal is emitted after the main loop of the application starts up. The passed parameter describes the launch request and contains the information about why the application is launched.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
This signal is emitted when the battery level of the device is low.
- Deprecated:
- Deprecated since 4.0, DALi version 1.2.62 Use LowBatterySignal() instead.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
std::string Dali::Application::GetLanguage | ( | ) | const |
This is used to get language information from device.
- Since:
- 4.0, DALi version 1.2.62
- Returns:
- Language information
std::string Dali::Application::GetRegion | ( | ) | const |
This is used to get region information from device.
- Since:
- 4.0, DALi version 1.2.62
- Returns:
- Region information
static std::string Dali::Application::GetResourcePath | ( | ) | [static] |
Get path application resources are stored at.
- Since:
- 4.0, DALi version 1.2.2
- Returns:
- the full path of the resources
float Dali::Application::GetStereoBase | ( | ) | const |
Gets the stereo base (eye separation) for Stereoscopic 3D.
- Deprecated:
- Deprecated since 5.5, DALi version 1.3.51
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The stereo base (eye separation) for Stereoscopic 3D
ViewMode Dali::Application::GetViewMode | ( | ) | const |
Gets the current viewing mode.
- Deprecated:
- Deprecated since 5.5, DALi version 1.3.51
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The current viewing mode
Retrieves the main window used by the Application class.
The application writer can use the window to change indicator and orientation properties.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- A handle to the window
The user should connect to this signal to determine when they should initialize their application.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
This signal is emitted when the language is changed on the device.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
This signal is emitted when the battery level of the device is low.
- Since:
- 4.0, DALi version 1.2.62
- Returns:
- The signal to connect to
void Dali::Application::Lower | ( | ) |
This lowers the application to bottom without actually quitting it.
- Since:
- 3.0, DALi version 1.0.0
This signal is emitted when the memory level of the device is low.
- Since:
- 4.0, DALi version 1.2.62
- Returns:
- The signal to connect to
void Dali::Application::MainLoop | ( | ) |
This starts the application.
Choosing this form of main loop indicates that the default application configuration of APPLICATION_HANDLES_CONTEXT_LOSS is used. On platforms where context loss can occur, the application is responsible for tearing down and re-loading UI. The application should listen to Stage::ContextLostSignal and Stage::ContextRegainedSignal.
- Since:
- 3.0, DALi version 1.0.0
void Dali::Application::MainLoop | ( | Configuration::ContextLoss | configuration | ) |
This starts the application, and allows the app to choose a different configuration.
If the application plans on using the ReplaceSurface or ReplaceWindow API, then this will trigger context loss & regain. The application should listen to Stage::ContextLostSignal and Stage::ContextRegainedSignal.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] configuration The context loss configuration
This signal is emitted when the memory level of the device is low.
- Deprecated:
- Deprecated since 4.0, DALi version 1.2.62 Use LowMemorySignal() instead.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
static Application Dali::Application::New | ( | ) | [static] |
This is the constructor for applications without an argument list.
- Since:
- 3.0, DALi version 1.0.0
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/display
- Returns:
- A handle to the Application
Reimplemented in Dali::WatchApplication.
static Application Dali::Application::New | ( | int * | argc, |
char ** | argv[] | ||
) | [static] |
This is the constructor for applications.
- Since:
- 3.0, DALi version 1.0.0
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/display
- Parameters:
-
[in,out] argc A pointer to the number of arguments [in,out] argv A pointer to the argument list
- Returns:
- A handle to the Application
Reimplemented in Dali::WatchApplication.
static Application Dali::Application::New | ( | int * | argc, |
char ** | argv[], | ||
const std::string & | stylesheet | ||
) | [static] |
This is the constructor for applications with a name.
- Since:
- 3.0, DALi version 1.0.0
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/display
- Parameters:
-
[in,out] argc A pointer to the number of arguments [in,out] argv A pointer to the argument list [in] stylesheet The path to user defined theme file
- Returns:
- A handle to the Application
- Note:
- If the stylesheet is not specified, then the library's default stylesheet will not be overridden.
Reimplemented in Dali::WatchApplication, and Dali::WidgetApplication.
static Application Dali::Application::New | ( | int * | argc, |
char ** | argv[], | ||
const std::string & | stylesheet, | ||
WINDOW_MODE | windowMode | ||
) | [static] |
This is the constructor for applications with a name.
- Since:
- 3.0, DALi version 1.0.0
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/display
- Parameters:
-
[in,out] argc A pointer to the number of arguments [in,out] argv A pointer to the argument list [in] stylesheet The path to user defined theme file [in] windowMode A member of WINDOW_MODE
- Returns:
- A handle to the Application
- Note:
- If the stylesheet is not specified, then the library's default stylesheet will not be overridden.
static Application Dali::Application::New | ( | int * | argc, |
char ** | argv[], | ||
const std::string & | stylesheet, | ||
Application::WINDOW_MODE | windowMode, | ||
PositionSize | positionSize | ||
) | [static] |
This is the constructor for applications.
- Since:
- 4.0, DALi version 1.2.60
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/display
- Parameters:
-
[in,out] argc A pointer to the number of arguments [in,out] argv A pointer to the argument list [in] stylesheet The path to user defined theme file [in] windowMode A member of WINDOW_MODE [in] positionSize A position and a size of the window
- Returns:
- A handle to the Application
- Note:
- If the stylesheet is not specified, then the library's default stylesheet will not be overridden.
Application& Dali::Application::operator= | ( | const Application & | application | ) |
Assignment operator.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] application Handle to an object
- Returns:
- A reference to this
The user should connect to this signal if they need to perform any special activities when the application is about to be paused.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
void Dali::Application::Quit | ( | ) |
This quits the application. Tizen applications should use Lower to improve re-start performance unless they need to Quit completely.
- Since:
- 3.0, DALi version 1.0.0
This signal is emitted when the region of the device is changed.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
void Dali::Application::ReplaceWindow | ( | PositionSize | windowPosition, |
const std::string & | name | ||
) |
Replaces the current window.
- Deprecated:
- Deprecated since 5.5, DALi version 1.4.12
This will force context loss. If you plan on using this API in your application, then you should configure it to prevent discard behavior when handling the Init signal.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] windowPosition The position and size parameters of the new window [in] name The name of the new window
This signal is sent when the system requires the user to reinitialize itself.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
This signal is emitted when the window application rendering on is resized.
- Deprecated:
- Deprecated since 4.0, DALi version 1.2.62 Use Window::ResizedSignal() instead.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
The user should connect to this signal if they need to perform any special activities when the application has resumed.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
void Dali::Application::SetStereoBase | ( | float | stereoBase | ) |
Sets the stereo base (eye separation) for Stereoscopic 3D.
- Deprecated:
- Deprecated since 5.5, DALi version 1.3.51
The stereo base is the distance in millimetres between the eyes. Typical values are between 50mm and 70mm. The default value is 65mm.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] stereoBase The stereo base (eye separation) for Stereoscopic 3D
void Dali::Application::SetViewMode | ( | ViewMode | viewMode | ) |
Sets the viewing mode for the application.
- Deprecated:
- Deprecated since 5.5, DALi version 1.3.51
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] viewMode The new viewing mode
The user should connect to this signal to determine when they should terminate their application.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to