Tizen Native API
5.5
|
A WatchApplication class object should be created by every watch application that wishes to use Dali. More...
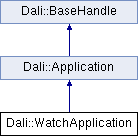
Public Types | |
typedef Signal< void(Application &, const WatchTime &) > | WatchTimeSignal |
Watch pointer signal callback type. | |
typedef Signal< void(Application &, bool) > | WatchBoolSignal |
Watch bool signal callback type. | |
Public Member Functions | |
WatchApplication () | |
Construct an empty handle. | |
WatchApplication (const WatchApplication &implementation) | |
Copy Constructor. | |
WatchApplication & | operator= (const WatchApplication &application) |
Assignment operator. | |
~WatchApplication () | |
Destructor. | |
WatchTimeSignal & | TimeTickSignal () |
This signal is emitted at every second A callback of the following type may be connected: | |
WatchTimeSignal & | AmbientTickSignal () |
This signal is emitted at each minute in ambient mode A callback of the following type may be connected: | |
WatchBoolSignal & | AmbientChangedSignal () |
This signal is emitted when the device enters or exits ambient mode A callback of the following type may be connected: | |
Static Public Member Functions | |
static WatchApplication | New () |
This is the constructor for applications without an argument list. | |
static WatchApplication | New (int *argc, char **argv[]) |
This is the constructor for applications. | |
static WatchApplication | New (int *argc, char **argv[], const std::string &stylesheet) |
This is the constructor for applications with a name. |
Detailed Description
A WatchApplication class object should be created by every watch application that wishes to use Dali.
It provides a means for initialising the resources required by the Dali::Core. Like Application class, the WatchApplication class manages Tizen watch application life cycle.
The WatchApplication class emits additional signals which are availalble only in the watch application (TimeTick, AmbientTick, AmbientChanged)
This feature is supported in wearable applications only.
WatchApplication should follow the example below:
class ExampleController: public ConnectionTracker { public: ExampleController( WatchApplication& application ) : mApplication( application ) { mApplication.InitSignal().Connect( this, &ExampleController::Create ); } void Create( Application& application ) { // Create Dali components... } ... private: WatchApplication& mApplication; }; int DALI_ADAPTOR_API main (int argc, char **argv) { WatchApplication app = WatchApplication::New(&argc, &argv); ExampleController example( app ); app.MainLoop(); }
If required, you can also connect class member functions to a signal:
MyApplication app; app.ResumeSignal().Connect(&app, &MyApplication::Resume);
When the above options are found, they are stripped from argv, and argc is updated appropriately.
- Since:
- 3.0, DALi version 1.1.37
Member Typedef Documentation
typedef Signal< void (Application&, bool) > Dali::WatchApplication::WatchBoolSignal |
Watch bool signal callback type.
- Since:
- 3.0, DALi version 1.1.37
typedef Signal< void (Application&, const WatchTime&) > Dali::WatchApplication::WatchTimeSignal |
Watch pointer signal callback type.
- Since:
- 3.0, DALi version 1.1.37
Constructor & Destructor Documentation
Construct an empty handle.
- Since:
- 3.0, DALi version 1.1.37
Dali::WatchApplication::WatchApplication | ( | const WatchApplication & | implementation | ) |
Copy Constructor.
- Since:
- 3.0, DALi version 1.1.37
- Parameters:
-
[in] implementation The WatchApplication implementation
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 3.0, DALi version 1.1.37
Member Function Documentation
This signal is emitted when the device enters or exits ambient mode A callback of the following type may be connected:
void YourCallbackName(Application& application, bool ambient);
ambient_mode If true the device enters the ambient mode, otherwise false
- Since:
- 3.0, DALi version 1.1.37
- Returns:
- The signal to connect to
This signal is emitted at each minute in ambient mode A callback of the following type may be connected:
void YourCallbackName(Application& application, const WatchTime& time);
time(watch time handle) will not be available after returning this callback. It will be freed by the framework.
- Since:
- 3.0, DALi version 1.1.37
- Remarks:
- http://tizen.org/privilege/alarm.set privilege is needed to receive ambient ticks at each minute. The AmbientTickSignal() will be ignored if your app doesn't have the privilege
- Returns:
- The signal to connect to
static WatchApplication Dali::WatchApplication::New | ( | ) | [static] |
This is the constructor for applications without an argument list.
- Since:
- 3.0, DALi version 1.1.37
- Returns:
- A handle to the WatchApplication
Reimplemented from Dali::Application.
static WatchApplication Dali::WatchApplication::New | ( | int * | argc, |
char ** | argv[] | ||
) | [static] |
This is the constructor for applications.
- Since:
- 3.0, DALi version 1.1.37
- Parameters:
-
[in,out] argc A pointer to the number of arguments [in,out] argv A pointer the the argument list
- Returns:
- A handle to the WatchApplication
Reimplemented from Dali::Application.
static WatchApplication Dali::WatchApplication::New | ( | int * | argc, |
char ** | argv[], | ||
const std::string & | stylesheet | ||
) | [static] |
This is the constructor for applications with a name.
- Since:
- 3.0, DALi version 1.1.37
- Parameters:
-
[in,out] argc A pointer to the number of arguments [in,out] argv A pointer the the argument list [in] stylesheet The path to user defined theme file
- Returns:
- A handle to the WatchApplication
Reimplemented from Dali::Application.
WatchApplication& Dali::WatchApplication::operator= | ( | const WatchApplication & | application | ) |
Assignment operator.
- Since:
- 3.0, DALi version 1.1.37
- Parameters:
-
[in] application Handle to an object
- Returns:
- A reference to this
This signal is emitted at every second A callback of the following type may be connected:
void YourCallbackName(Application& application, const WatchTime& time);
time(watch time handle) will not be available after returning this callback. It will be freed by the framework.
- Since:
- 3.0, DALi version 1.1.37
- Returns:
- The signal to connect to