Tizen Native API
5.5
|
A PushButton changes its appearance when is pressed and returns to its original when is released. More...
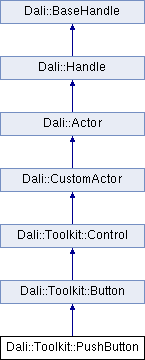
Classes | |
struct | Property |
Enumeration for the instance of properties belonging to the PushButton class. More... | |
Public Types | |
enum | PropertyRange |
Enumeration for the start and end property ranges for this control. More... | |
Public Member Functions | |
PushButton () | |
Creates an uninitialized PushButton; this can be initialized with PushButton::New(). | |
PushButton (const PushButton &pushButton) | |
Copy constructor. | |
PushButton & | operator= (const PushButton &pushButton) |
Assignment operator. | |
~PushButton () | |
Destructor. | |
Static Public Member Functions | |
static PushButton | New () |
Creates an initialized PushButton. | |
static PushButton | DownCast (BaseHandle handle) |
Downcasts a handle to PushButton handle. |
Detailed Description
A PushButton changes its appearance when is pressed and returns to its original when is released.
By default, a PushButton emits a Button::PressedSignal() signal when the button is pressed, a Button::ClickedSignal() signal when it's clicked. and a Button::ReleasedSignal() signal when it's released or having pressed it, the touch point leaves the boundary of the button.
Usage example: -
// in Creating a DALi Application void HelloWorldExample::Create( Application& application ) { PushButton button = PushButton::New(); button.SetParentOrigin( ParentOrigin::CENTER ); button.SetProperty( Button::Property::LABEL, "Press" ); Stage::GetCurrent().Add( button ); // Connect to button signals emitted by the button button.ClickedSignal().Connect( this, &HelloWorldExample::OnButtonClicked ); button.PressedSignal().Connect( this, &HelloWorldExample::OnButtonPressed ); button.ReleasedSignal().Connect( this, &HelloWorldExample::OnButtonReleased ); } bool HelloWorldExample::OnButtonClicked( Button button ) { // Do something when the button is clicked return true; } bool HelloWorldExample::OnButtonPressed( Button button ) { // Do something when the button is pressed return true; } bool HelloWorldExample::OnButtonReleased( Button button ) { // Do something when the button is released return true; }
See Button for more details on signals and modifying appearance via properties.
- Since:
- 3.0, DALi version 1.0.0
Member Enumeration Documentation
Enumeration for the start and end property ranges for this control.
- Since:
- 3.0, DALi version 1.0.0
- Enumerator:
PROPERTY_START_INDEX - Since:
- 3.0, DALi version 1.0.0
PROPERTY_END_INDEX Reserving 1000 property indices.
- Since:
- 3.0, DALi version 1.0.0
Reimplemented from Dali::Toolkit::Button.
Constructor & Destructor Documentation
Creates an uninitialized PushButton; this can be initialized with PushButton::New().
Calling member functions with an uninitialized Dali::Object is not allowed.
- Since:
- 3.0, DALi version 1.0.0
Dali::Toolkit::PushButton::PushButton | ( | const PushButton & | pushButton | ) |
Destructor.
This is non-virtual since derived Handle types must not contain data or virtual methods.
- Since:
- 3.0, DALi version 1.0.0
Member Function Documentation
static PushButton Dali::Toolkit::PushButton::DownCast | ( | BaseHandle | handle | ) | [static] |
Downcasts a handle to PushButton handle.
If handle points to a PushButton, the downcast produces valid handle. If not, the returned handle is left uninitialized.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] handle Handle to an object
- Returns:
- handle to a PushButton or an uninitialized handle
Reimplemented from Dali::Toolkit::Button.
static PushButton Dali::Toolkit::PushButton::New | ( | ) | [static] |
Creates an initialized PushButton.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- A handle to a newly allocated Dali resource
Reimplemented from Dali::Toolkit::Control.
PushButton& Dali::Toolkit::PushButton::operator= | ( | const PushButton & | pushButton | ) |
Assignment operator.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] pushButton Handle to an object
- Returns:
- A reference to this