Tizen Native API
5.5
|
This is the internal base class for all controls. More...
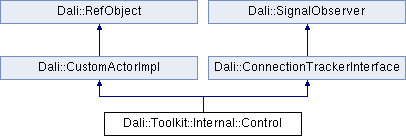
Public Member Functions | |
void | SetStyleName (const std::string &styleName) |
const std::string & | GetStyleName () const |
void | SetBackgroundColor (const Vector4 &color) |
void | SetBackground (const Property::Map &map) |
Sets the background with a property map. | |
void | ClearBackground () |
void | EnableGestureDetection (Gesture::Type type) |
Allows deriving classes to enable any of the gesture detectors that are available. | |
void | DisableGestureDetection (Gesture::Type type) |
Allows deriving classes to disable any of the gesture detectors. | |
PinchGestureDetector | GetPinchGestureDetector () const |
If deriving classes wish to fine tune pinch gesture detection, then they can access the gesture detector through this API and modify the detection. | |
PanGestureDetector | GetPanGestureDetector () const |
If deriving classes wish to fine tune pan gesture detection, then they can access the gesture detector through this API and modify the detection. | |
TapGestureDetector | GetTapGestureDetector () const |
If deriving classes wish to fine tune tap gesture detection, then they can access the gesture detector through this API and modify the detection. | |
LongPressGestureDetector | GetLongPressGestureDetector () const |
If deriving classes wish to fine tune long press gesture detection, then they can access the gesture detector through this API and modify the detection. | |
void | SetKeyboardNavigationSupport (bool isSupported) |
Sets whether this control supports two dimensional keyboard navigation (i.e. whether it knows how to handle the keyboard focus movement between its child actors). | |
bool | IsKeyboardNavigationSupported () |
Gets whether this control supports two dimensional keyboard navigation. | |
void | SetKeyInputFocus () |
bool | HasKeyInputFocus () |
void | ClearKeyInputFocus () |
void | SetAsKeyboardFocusGroup (bool isFocusGroup) |
Sets whether this control is a focus group for keyboard navigation. | |
bool | IsKeyboardFocusGroup () |
Gets whether this control is a focus group for keyboard navigation. | |
Toolkit::Control::KeyEventSignalType & | KeyEventSignal () |
Toolkit::Control::KeyInputFocusSignalType & | KeyInputFocusGainedSignal () |
Toolkit::Control::KeyInputFocusSignalType & | KeyInputFocusLostSignal () |
virtual void | OnInitialize () |
This method is called after the Control has been initialized. | |
virtual void | OnControlChildAdd (Actor &child) DALI_DEPRECATED_API |
Called whenever an Actor is added to the control. | |
virtual void | OnControlChildRemove (Actor &child) DALI_DEPRECATED_API |
Called whenever an Actor is removed from the control. | |
virtual void | OnStyleChange (Toolkit::StyleManager styleManager, StyleChange::Type change) |
This method should be overridden by deriving classes requiring notifications when the style changes. | |
virtual bool | OnAccessibilityActivated () |
This method is called when the control is accessibility activated. | |
virtual bool | OnAccessibilityPan (PanGesture gesture) |
This method should be overridden by deriving classes when they wish to respond the accessibility pan gesture. | |
virtual bool | OnAccessibilityTouch (const TouchEvent &touchEvent) |
This method should be overridden by deriving classes when they wish to respond the accessibility touch event. | |
virtual bool | OnAccessibilityValueChange (bool isIncrease) |
This method should be overridden by deriving classes when they wish to respond the accessibility up and down action (i.e. value change of slider control). | |
virtual bool | OnAccessibilityZoom () |
This method should be overridden by deriving classes when they wish to respond the accessibility zoom action. | |
virtual void | OnKeyInputFocusGained () |
Called when the control gains key input focus. | |
virtual void | OnKeyInputFocusLost () |
Called when the control loses key input focus. | |
virtual Actor | GetNextKeyboardFocusableActor (Actor currentFocusedActor, Toolkit::Control::KeyboardFocus::Direction direction, bool loopEnabled) |
Gets the next keyboard focusable actor in this control towards the given direction. | |
virtual void | OnKeyboardFocusChangeCommitted (Actor commitedFocusableActor) |
Informs this control that its chosen focusable actor will be focused. | |
virtual bool | OnKeyboardEnter () |
This method is called when the control has enter pressed on it. | |
virtual void | OnPinch (const PinchGesture &pinch) |
Called whenever a pinch gesture is detected on this control. | |
virtual void | OnPan (const PanGesture &pan) |
Called whenever a pan gesture is detected on this control. | |
virtual void | OnTap (const TapGesture &tap) |
Called whenever a tap gesture is detected on this control. | |
virtual void | OnLongPress (const LongPressGesture &longPress) |
Called whenever a long press gesture is detected on this control. | |
virtual void | SignalConnected (SlotObserver *slotObserver, CallbackBase *callback) |
virtual void | SignalDisconnected (SlotObserver *slotObserver, CallbackBase *callback) |
virtual Extension * | GetControlExtension () |
Retrieves the extension for this control. | |
Static Public Member Functions | |
static Toolkit::Control | New () |
Forward declare future extension interface. | |
Protected Types | |
enum | ControlBehaviour |
Flags for the constructor. More... | |
Protected Member Functions | |
virtual | ~Control () |
Virtual destructor. | |
void | EmitKeyInputFocusSignal (bool focusGained) |
Emits KeyInputFocusGained signal if true else emits KeyInputFocusLost signal. | |
virtual void | OnStageConnection (int depth) |
virtual void | OnStageDisconnection () |
virtual void | OnChildAdd (Actor &child) |
virtual void | OnChildRemove (Actor &child) |
virtual void | OnPropertySet (Property::Index index, Property::Value propertyValue) |
virtual void | OnSizeSet (const Vector3 &targetSize) |
virtual void | OnSizeAnimation (Animation &animation, const Vector3 &targetSize) |
virtual bool | OnTouchEvent (const TouchEvent &event) |
virtual bool | OnHoverEvent (const HoverEvent &event) |
virtual bool | OnKeyEvent (const KeyEvent &event) |
virtual bool | OnWheelEvent (const WheelEvent &event) |
virtual void | OnRelayout (const Vector2 &size, RelayoutContainer &container) |
virtual void | OnSetResizePolicy (ResizePolicy::Type policy, Dimension::Type dimension) |
virtual Vector3 | GetNaturalSize () |
virtual float | CalculateChildSize (const Dali::Actor &child, Dimension::Type dimension) |
virtual float | GetHeightForWidth (float width) |
virtual float | GetWidthForHeight (float height) |
virtual bool | RelayoutDependentOnChildren (Dimension::Type dimension=Dimension::ALL_DIMENSIONS) |
virtual void | OnCalculateRelayoutSize (Dimension::Type dimension) |
virtual void | OnLayoutNegotiated (float size, Dimension::Type dimension) |
Control (ControlBehaviour behaviourFlags) | |
Control constructor. | |
void | Initialize () |
Second phase initialization. | |
Static Protected Attributes | |
static const int | CONTROL_BEHAVIOUR_FLAG_COUNT = Log< LAST_CONTROL_BEHAVIOUR_FLAG - 1 >::value + 1 |
Total count of flags. |
Detailed Description
This is the internal base class for all controls.
It will provide some common functionality required by all controls. Implements ConnectionTrackerInterface so that signals (typically connected to member functions) will be disconnected automatically when the control is destroyed.
- Since:
- 3.0, DALi version 1.0.0
Member Enumeration Documentation
enum Dali::Toolkit::Internal::Control::ControlBehaviour [protected] |
Flags for the constructor.
- Since:
- 3.0, DALi version 1.0.0
- Enumerator:
CONTROL_BEHAVIOUR_DEFAULT Default behaviour: Size negotiation is enabled & listens to Style Change signal, but doesn't receive event callbacks.
- Since:
- 3.0, DALi version 1.2.10
REQUIRES_STYLE_CHANGE_SIGNALS True if needs to monitor style change signals such as theme/font change.
- Since:
- 3.0, DALi version 1.0.0
- Deprecated:
- Deprecated since 3.0, DALi version 1.2.10
REQUIRES_KEYBOARD_NAVIGATION_SUPPORT True if needs to support keyboard navigation.
- Since:
- 3.0, DALi version 1.0.0
DISABLE_STYLE_CHANGE_SIGNALS True if control should not monitor style change signals.
- Since:
- 3.0, DALi version 1.2.10
Constructor & Destructor Documentation
virtual Dali::Toolkit::Internal::Control::~Control | ( | ) | [protected, virtual] |
Virtual destructor.
- Since:
- 3.0, DALi version 1.0.0
Dali::Toolkit::Internal::Control::Control | ( | ControlBehaviour | behaviourFlags | ) | [protected] |
Control constructor.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] behaviourFlags Behavioural flags from ControlBehaviour enum
Member Function Documentation
virtual float Dali::Toolkit::Internal::Control::CalculateChildSize | ( | const Dali::Actor & | child, |
Dimension::Type | dimension | ||
) | [protected, virtual] |
Calculates the size for a child.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] child The child actor to calculate the size for [in] dimension The dimension to calculate the size for. E.g. width or height
- Returns:
- Return the calculated size for the given dimension
Implements Dali::CustomActorImpl.
Clears the background.
- Since:
- 3.0, DALi version 1.0.0
Once an actor is Set to receive key input focus this function is called to stop it receiving key events.
A check is performed to ensure it was previously set, if this check fails then nothing is done.
- Since:
- 3.0, DALi version 1.0.0
- Precondition:
- The Actor has been initialized.
Allows deriving classes to disable any of the gesture detectors.
Like EnableGestureDetection, this can also be called using bitwise or.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] type The gesture type(s) to disable
- See also:
- EnableGetureDetection
void Dali::Toolkit::Internal::Control::EmitKeyInputFocusSignal | ( | bool | focusGained | ) | [protected] |
Emits KeyInputFocusGained signal if true else emits KeyInputFocusLost signal.
Should be called last by the control after it acts on the Input Focus change.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] focusGained True if gained, False if lost
Allows deriving classes to enable any of the gesture detectors that are available.
Gesture detection can be enabled one at a time or in bitwise format as shown:
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] type The gesture type(s) to enable
virtual Extension* Dali::Toolkit::Internal::Control::GetControlExtension | ( | ) | [virtual] |
Retrieves the extension for this control.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The extension if available, NULL otherwise
virtual float Dali::Toolkit::Internal::Control::GetHeightForWidth | ( | float | width | ) | [protected, virtual] |
This method is called during size negotiation when a height is required for a given width.
Derived classes should override this if they wish to customize the height returned.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] width Width to use
- Returns:
- The height based on the width
Implements Dali::CustomActorImpl.
If deriving classes wish to fine tune long press gesture detection, then they can access the gesture detector through this API and modify the detection.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The long press gesture detector
- Precondition:
- Long press detection should have been enabled via EnableGestureDetection().
- See also:
- EnableGestureDetection
virtual Vector3 Dali::Toolkit::Internal::Control::GetNaturalSize | ( | ) | [protected, virtual] |
Returns the natural size of the actor.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The actor's natural size
Implements Dali::CustomActorImpl.
virtual Actor Dali::Toolkit::Internal::Control::GetNextKeyboardFocusableActor | ( | Actor | currentFocusedActor, |
Toolkit::Control::KeyboardFocus::Direction | direction, | ||
bool | loopEnabled | ||
) | [virtual] |
Gets the next keyboard focusable actor in this control towards the given direction.
A control needs to override this function in order to support two dimensional keyboard navigation.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] currentFocusedActor The current focused actor [in] direction The direction to move the focus towards [in] loopEnabled Whether the focus movement should be looped within the control
- Returns:
- The next keyboard focusable actor in this control or an empty handle if no actor can be focused
If deriving classes wish to fine tune pan gesture detection, then they can access the gesture detector through this API and modify the detection.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The pan gesture detector
- Precondition:
- Pan detection should have been enabled via EnableGestureDetection().
- See also:
- EnableGestureDetection
If deriving classes wish to fine tune pinch gesture detection, then they can access the gesture detector through this API and modify the detection.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The pinch gesture detector
- Precondition:
- Pinch detection should have been enabled via EnableGestureDetection().
- See also:
- EnableGestureDetection
const std::string& Dali::Toolkit::Internal::Control::GetStyleName | ( | ) | const |
Retrieves the name of the style to be applied to the control (if any).
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- A string matching a style, or an empty string
If deriving classes wish to fine tune tap gesture detection, then they can access the gesture detector through this API and modify the detection.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The tap gesture detector
- Precondition:
- Tap detection should have been enabled via EnableGestureDetection().
- See also:
- EnableGestureDetection
virtual float Dali::Toolkit::Internal::Control::GetWidthForHeight | ( | float | height | ) | [protected, virtual] |
This method is called during size negotiation when a width is required for a given height.
Derived classes should override this if they wish to customize the width returned.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] height Height to use
- Returns:
- The width based on the width
Implements Dali::CustomActorImpl.
Quries whether the control has key input focus.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- true if this control has keyboard input focus
- Precondition:
- The Control has been initialized.
- The Control should be on the stage before setting keyboard focus.
- Note:
- The control can be set to have the focus and still not receive all the key events if another control has over ridden it. As the key input focus mechanism works like a stack, the top most control receives all the key events, and passes on the unhandled events to the controls below in the stack. A control in the stack will regain key input focus when there are no more controls above it in the focus stack. To query for the control which is on top of the focus stack use Dali::Toolkit::KeyInputFocusManager::GetCurrentKeyboardFocusActor().
void Dali::Toolkit::Internal::Control::Initialize | ( | ) | [protected] |
Second phase initialization.
- Since:
- 3.0, DALi version 1.0.0
Gets whether this control is a focus group for keyboard navigation.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- true if this control is set as a focus group for keyboard navigation
Gets whether this control supports two dimensional keyboard navigation.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- true if this control supports two dimensional keyboard navigation
This signal is emitted when key event is received.
A callback of the following type may be connected:
bool YourCallbackName(Control control, const KeyEvent& event);
The return value of True, indicates that the event should be consumed. Otherwise the signal will be emitted on the next parent of the actor.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
- Precondition:
- The Control has been initialized.
Toolkit::Control::KeyInputFocusSignalType& Dali::Toolkit::Internal::Control::KeyInputFocusGainedSignal | ( | ) |
This signal is emitted when the control gets Key Input Focus.
A callback of the following type may be connected:
bool YourCallbackName( Control control );
The return value of True, indicates that the event should be consumed. Otherwise the signal will be emitted on the next parent of the actor.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
- Precondition:
- The Control has been initialized.
Toolkit::Control::KeyInputFocusSignalType& Dali::Toolkit::Internal::Control::KeyInputFocusLostSignal | ( | ) |
This signal is emitted when the control loses Key Input Focus.
This could be due to it being gained by another Control or Actor or just cleared from this control as no longer required.
A callback of the following type may be connected:
bool YourCallbackName( Control control );
The return value of True, indicates that the event should be consumed. Otherwise the signal will be emitted on the next parent of the actor.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- The signal to connect to
- Precondition:
- The Control has been initialized.
static Toolkit::Control Dali::Toolkit::Internal::Control::New | ( | ) | [static] |
Forward declare future extension interface.
Creates a new ControlImpl instance that does not require touch by default.
If touch is required, then the user can connect to this class' touch signal.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- A handle to the ControlImpl instance
virtual bool Dali::Toolkit::Internal::Control::OnAccessibilityActivated | ( | ) | [virtual] |
This method is called when the control is accessibility activated.
Derived classes should override this to perform custom accessibility activation.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- true if this control can perform accessibility activation
virtual bool Dali::Toolkit::Internal::Control::OnAccessibilityPan | ( | PanGesture | gesture | ) | [virtual] |
This method should be overridden by deriving classes when they wish to respond the accessibility pan gesture.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] gesture The pan gesture
- Returns:
- true if the pan gesture has been consumed by this control
virtual bool Dali::Toolkit::Internal::Control::OnAccessibilityTouch | ( | const TouchEvent & | touchEvent | ) | [virtual] |
This method should be overridden by deriving classes when they wish to respond the accessibility touch event.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] touchEvent The touch event
- Returns:
- true if the touch event has been consumed by this control
virtual bool Dali::Toolkit::Internal::Control::OnAccessibilityValueChange | ( | bool | isIncrease | ) | [virtual] |
This method should be overridden by deriving classes when they wish to respond the accessibility up and down action (i.e. value change of slider control).
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] isIncrease Whether the value should be increased or decreased
- Returns:
- true if the value changed action has been consumed by this control
virtual bool Dali::Toolkit::Internal::Control::OnAccessibilityZoom | ( | ) | [virtual] |
This method should be overridden by deriving classes when they wish to respond the accessibility zoom action.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- true if the zoom action has been consumed by this control
virtual void Dali::Toolkit::Internal::Control::OnCalculateRelayoutSize | ( | Dimension::Type | dimension | ) | [protected, virtual] |
Virtual method to notify deriving classes that relayout dependencies have been met and the size for this object is about to be calculated for the given dimension.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] dimension The dimension that is about to be calculated
Implements Dali::CustomActorImpl.
virtual void Dali::Toolkit::Internal::Control::OnChildAdd | ( | Actor & | child | ) | [protected, virtual] |
Called after a child has been added to the owning actor.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] child The child which has been added
- Note:
- If overridden, then an up-call to Control::OnChildAdd MUST be made at the end.
Implements Dali::CustomActorImpl.
virtual void Dali::Toolkit::Internal::Control::OnChildRemove | ( | Actor & | child | ) | [protected, virtual] |
Called after the owning actor has attempted to remove a child (regardless of whether it succeeded or not).
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] child The child being removed
- Note:
- If overridden, then an up-call to Control::OnChildRemove MUST be made at the end.
Implements Dali::CustomActorImpl.
virtual void Dali::Toolkit::Internal::Control::OnControlChildAdd | ( | Actor & | child | ) | [virtual] |
Called whenever an Actor is added to the control.
- Deprecated:
- Deprecated since 3.0, DALi version 1.1.30. Override OnChildAdd instead.
Could be overridden by derived classes.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] child The added actor
virtual void Dali::Toolkit::Internal::Control::OnControlChildRemove | ( | Actor & | child | ) | [virtual] |
Called whenever an Actor is removed from the control.
- Deprecated:
- Deprecated since 3.0, DALi version 1.1.30. Override OnChildRemove instead.
Could be overridden by derived classes.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] child The removed actor
virtual bool Dali::Toolkit::Internal::Control::OnHoverEvent | ( | const HoverEvent & | event | ) | [protected, virtual] |
Called after a hover-event is received by the owning actor.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] event The hover event
- Returns:
- True if the event should be consumed
- Note:
- CustomActorImpl::REQUIRES_HOVER_EVENTS must be enabled during construction. See CustomActorImpl::CustomActorImpl( ActorFlags flags ).
Implements Dali::CustomActorImpl.
virtual void Dali::Toolkit::Internal::Control::OnInitialize | ( | ) | [virtual] |
This method is called after the Control has been initialized.
Derived classes should do any second phase initialization by overriding this method.
- Since:
- 3.0, DALi version 1.0.0
virtual bool Dali::Toolkit::Internal::Control::OnKeyboardEnter | ( | ) | [virtual] |
This method is called when the control has enter pressed on it.
Derived classes should override this to perform custom actions.
- Since:
- 3.0, DALi version 1.0.0
- Returns:
- true if this control supported this action
virtual void Dali::Toolkit::Internal::Control::OnKeyboardFocusChangeCommitted | ( | Actor | commitedFocusableActor | ) | [virtual] |
Informs this control that its chosen focusable actor will be focused.
This allows the application to perform any actions if wishes before the focus is actually moved to the chosen actor.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] commitedFocusableActor The commited focusable actor
virtual bool Dali::Toolkit::Internal::Control::OnKeyEvent | ( | const KeyEvent & | event | ) | [protected, virtual] |
Called after a key-event is received by the actor that has had its focus set.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] event The Key Event
- Returns:
- True if the event should be consumed
Implements Dali::CustomActorImpl.
virtual void Dali::Toolkit::Internal::Control::OnKeyInputFocusGained | ( | ) | [virtual] |
Called when the control gains key input focus.
Should be overridden by derived classes if they need to customize what happens when focus is gained.
- Since:
- 3.0, DALi version 1.0.0
virtual void Dali::Toolkit::Internal::Control::OnKeyInputFocusLost | ( | ) | [virtual] |
Called when the control loses key input focus.
Should be overridden by derived classes if they need to customize what happens when focus is lost.
- Since:
- 3.0, DALi version 1.0.0
virtual void Dali::Toolkit::Internal::Control::OnLayoutNegotiated | ( | float | size, |
Dimension::Type | dimension | ||
) | [protected, virtual] |
Virtual method to notify deriving classes that the size for a dimension has just been negotiated.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] size The new size for the given dimension [in] dimension The dimension that was just negotiated
Implements Dali::CustomActorImpl.
virtual void Dali::Toolkit::Internal::Control::OnLongPress | ( | const LongPressGesture & | longPress | ) | [virtual] |
Called whenever a long press gesture is detected on this control.
This should be overridden by deriving classes when long press detection is enabled.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] longPress The long press gesture
- Note:
- There is no default behaviour associated with a long press.
- Long press detection should be enabled via EnableGestureDetection().
- See also:
- EnableGestureDetection
virtual void Dali::Toolkit::Internal::Control::OnPan | ( | const PanGesture & | pan | ) | [virtual] |
Called whenever a pan gesture is detected on this control.
This should be overridden by deriving classes when pan detection is enabled.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] pan The pan gesture
- Note:
- There is no default behavior with panning.
- Pan detection should be enabled via EnableGestureDetection().
- See also:
- EnableGestureDetection
virtual void Dali::Toolkit::Internal::Control::OnPinch | ( | const PinchGesture & | pinch | ) | [virtual] |
Called whenever a pinch gesture is detected on this control.
This can be overridden by deriving classes when pinch detection is enabled. The default behaviour is to scale the control by the pinch scale.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] pinch The pinch gesture
- Note:
- If overridden, then the default behavior will not occur.
- Pinch detection should be enabled via EnableGestureDetection().
- See also:
- EnableGestureDetection
virtual void Dali::Toolkit::Internal::Control::OnPropertySet | ( | Property::Index | index, |
Property::Value | propertyValue | ||
) | [protected, virtual] |
Called when the owning actor property is set.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] index The Property index that was set [in] propertyValue The value to set
- Note:
- If overridden, then an up-call to Control::OnChildRemove MUST be made at the end.
Reimplemented from Dali::CustomActorImpl.
virtual void Dali::Toolkit::Internal::Control::OnRelayout | ( | const Vector2 & | size, |
RelayoutContainer & | container | ||
) | [protected, virtual] |
Called after the size negotiation has been finished for this control.
The control is expected to assign this given size to itself/its children.
Should be overridden by derived classes if they need to layout actors differently after certain operations like add or remove actors, resize or after changing specific properties.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] size The allocated size [in,out] container The control should add actors to this container that it is not able to allocate a size for
- Note:
- As this function is called from inside the size negotiation algorithm, you cannot call RequestRelayout (the call would just be ignored).
Implements Dali::CustomActorImpl.
virtual void Dali::Toolkit::Internal::Control::OnSetResizePolicy | ( | ResizePolicy::Type | policy, |
Dimension::Type | dimension | ||
) | [protected, virtual] |
Notification for deriving classes.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] policy The policy being set [in] dimension The dimension the policy is being set for
Implements Dali::CustomActorImpl.
virtual void Dali::Toolkit::Internal::Control::OnSizeAnimation | ( | Animation & | animation, |
const Vector3 & | targetSize | ||
) | [protected, virtual] |
Called when the owning actor's size is animated e.g. using Animation::AnimateTo( Property( actor, Actor::Property::SIZE ), ... ).
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] animation The object which is animating the owning actor [in] targetSize The target size. Note that this target size may not match the size returned via Actor::GetTargetSize
- Note:
- If overridden, then an up-call to Control::OnSizeAnimation MUST be made at the end.
Implements Dali::CustomActorImpl.
virtual void Dali::Toolkit::Internal::Control::OnSizeSet | ( | const Vector3 & | targetSize | ) | [protected, virtual] |
Called when the owning actor's size is set e.g. using Actor::SetSize().
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] targetSize The target size. Note that this target size may not match the size returned via Actor::GetTargetSize
- Note:
- If overridden, then an up-call to Control::OnSizeSet MUST be made at the end.
Implements Dali::CustomActorImpl.
virtual void Dali::Toolkit::Internal::Control::OnStageConnection | ( | int | depth | ) | [protected, virtual] |
Called after the actor has been connected to the stage.
When an actor is connected, it will be directly or indirectly parented to the root Actor.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] depth The depth in the hierarchy for the actor
- Note:
- The root Actor is provided automatically by Dali::Stage, and is always considered to be connected. When the parent of a set of actors is connected to the stage, then all of the children will received this callback. For the following actor tree, the callback order will be A, B, D, E, C, and finally F.
A (parent) / \ B C / \ \ D E F
- Parameters:
-
[in] depth The depth in the hierarchy for the actor
- Note:
- If overridden, then an up-call to Control::OnStageConnection MUST be made at the end.
virtual void Dali::Toolkit::Internal::Control::OnStageDisconnection | ( | ) | [protected, virtual] |
Called after the actor has been disconnected from Stage.
If an actor is disconnected, it either has no parent or is parented to a disconnected actor.
- Since:
- 3.0, DALi version 1.0.0
- Note:
- When the parent of a set of actors is disconnected to the stage, then all of the children will received this callback, starting with the leaf actors. For the following actor tree, the callback order will be D, E, B, F, C, and finally A.
A (parent) / \ B C / \ \ D E F
- Note:
- If overridden, then an up-call to Control::OnStageDisconnection MUST be made at the end.
Implements Dali::CustomActorImpl.
virtual void Dali::Toolkit::Internal::Control::OnStyleChange | ( | Toolkit::StyleManager | styleManager, |
StyleChange::Type | change | ||
) | [virtual] |
This method should be overridden by deriving classes requiring notifications when the style changes.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] styleManager The StyleManager object [in] change Information denoting what has changed
virtual void Dali::Toolkit::Internal::Control::OnTap | ( | const TapGesture & | tap | ) | [virtual] |
Called whenever a tap gesture is detected on this control.
This should be overridden by deriving classes when tap detection is enabled.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] tap The tap gesture
- Note:
- There is no default behavior with a tap.
- Tap detection should be enabled via EnableGestureDetection().
- See also:
- EnableGestureDetection
virtual bool Dali::Toolkit::Internal::Control::OnTouchEvent | ( | const TouchEvent & | event | ) | [protected, virtual] |
Called after a touch-event is received by the owning actor.
- Deprecated:
- Deprecated since 3.0, DALi version 1.1.37 Connect to TouchSignal() instead.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] event The touch event
- Returns:
- True if the event should be consumed
- Note:
- CustomActorImpl::REQUIRES_TOUCH_EVENTS must be enabled during construction. See CustomActorImpl::CustomActorImpl( ActorFlags flags ).
Implements Dali::CustomActorImpl.
virtual bool Dali::Toolkit::Internal::Control::OnWheelEvent | ( | const WheelEvent & | event | ) | [protected, virtual] |
Called after a wheel-event is received by the owning actor.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] event The wheel event
- Returns:
- True if the event should be consumed
- Note:
- CustomActorImpl::REQUIRES_WHEEL_EVENTS must be enabled during construction. See CustomActorImpl::CustomActorImpl( ActorFlags flags ).
Implements Dali::CustomActorImpl.
virtual bool Dali::Toolkit::Internal::Control::RelayoutDependentOnChildren | ( | Dimension::Type | dimension = Dimension::ALL_DIMENSIONS | ) | [protected, virtual] |
Determines if this actor is dependent on its children for relayout.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] dimension The dimension(s) to check for
- Returns:
- Return if the actor is dependent on it's children
Implements Dali::CustomActorImpl.
void Dali::Toolkit::Internal::Control::SetAsKeyboardFocusGroup | ( | bool | isFocusGroup | ) |
Sets whether this control is a focus group for keyboard navigation.
(i.e. the scope of keyboard focus movement can be limited to its child actors). The control is not a focus group by default.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] isFocusGroup Whether this control is set as a focus group for keyboard navigation
void Dali::Toolkit::Internal::Control::SetBackground | ( | const Property::Map & | map | ) |
Sets the background with a property map.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] map The background property map
void Dali::Toolkit::Internal::Control::SetBackgroundColor | ( | const Vector4 & | color | ) |
Sets the background color of the control.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] color The required background color of the control
- Note:
- If SetBackgroundImage is called later, this background color is removed.
- The background color fully blends with the actor color.
void Dali::Toolkit::Internal::Control::SetKeyboardNavigationSupport | ( | bool | isSupported | ) |
Sets whether this control supports two dimensional keyboard navigation (i.e. whether it knows how to handle the keyboard focus movement between its child actors).
The control doesn't support it by default.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] isSupported Whether this control supports two dimensional keyboard navigation
void Dali::Toolkit::Internal::Control::SetStyleName | ( | const std::string & | styleName | ) |
Sets the name of the style to be applied to the control.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] styleName A string matching a style described in a stylesheet
virtual void Dali::Toolkit::Internal::Control::SignalConnected | ( | SlotObserver * | slotObserver, |
CallbackBase * | callback | ||
) | [virtual] |
Called when a signal is connected.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] slotObserver The slot observer i.e. a signal. Ownership is not passed [in] callback The call back. Ownership is not passed
Implements Dali::ConnectionTrackerInterface.
virtual void Dali::Toolkit::Internal::Control::SignalDisconnected | ( | SlotObserver * | slotObserver, |
CallbackBase * | callback | ||
) | [virtual] |
This method is called when the signal is disconnecting.
- Since:
- 3.0, DALi version 1.0.0
- Parameters:
-
[in] slotObserver The signal that has disconnected [in] callback The callback attached to the signal disconnected
Implements Dali::SignalObserver.