Tizen Native API
7.0
|
Creating an Eina_List and adding elements to it is very easy and can be understood from an example: First thing is always to include Eina.h, for this example we also include stdio.h so we can use printf.
#include <stdio.h> #include <Eina.h>
Just some boilerplate code, declaring some variables and initializing eina.
int main(int argc, char **argv) { (void)argc; (void)argv; Eina_List *list = NULL; Eina_List *l; void *list_data; eina_init();
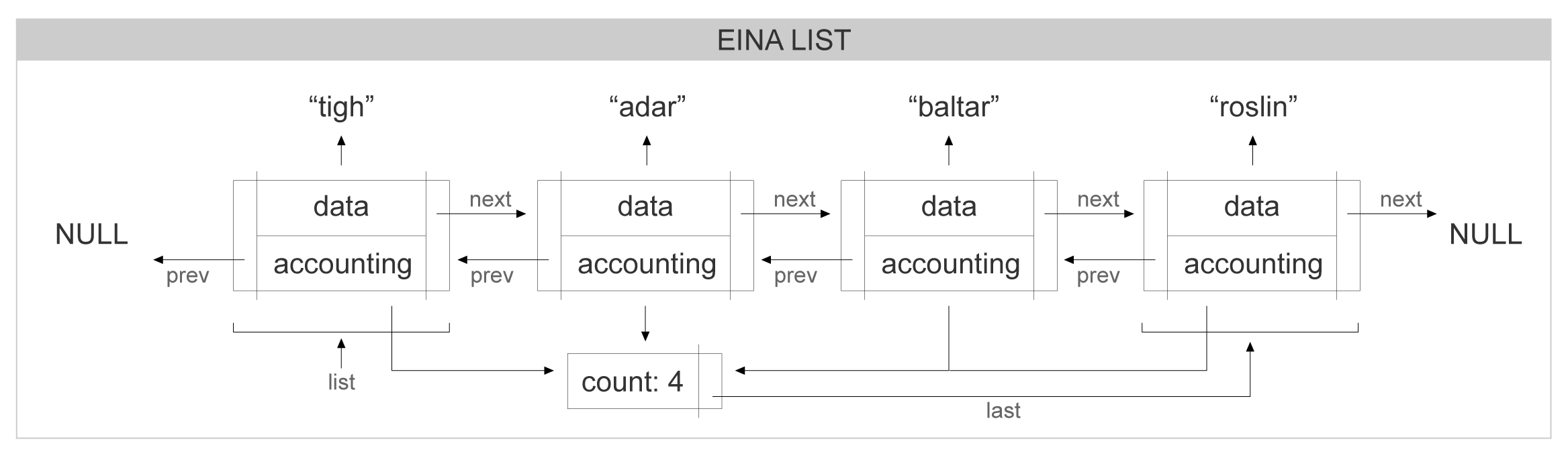
list = eina_list_append(list, "tigh"); list = eina_list_append(list, "adar"); list = eina_list_append(list, "baltar"); list = eina_list_append(list, "roslin");
Now that we have a list with some elements in it we can look at its contents.
EINA_LIST_FOREACH(list, l, list_data) printf("%s\n", (char*)list_data);
There are many ways of accessing elements in the list, including by its index:
printf("\n"); l = eina_list_nth_list(list, 1);
- Note:
- It should be noted that the index starts at 0.
eina_list_append() is not the only way to add elements to a a list. A common requirement is to add an element in a specific position this can be accomplished using eina_list_append_relative() and eina_list_append_relative_list():
list = eina_list_append_relative_list(list, "cain", l); list = eina_list_append_relative(list, "zarek", "cain");
Eina_List also has prepend analogs to append functions we have used so far:
list = eina_list_prepend(list, "adama"); list = eina_list_prepend_relative(list, "gaeta", "cain"); list = eina_list_prepend_relative_list(list, "lampkin", l);

Once done using the list it needs to be freed, and since we are done with eina that also need to be shutdown:
EINA_LIST_FOREACH(list, l, list_data) printf("%s\n", (char*)list_data); eina_list_free(list); eina_shutdown(); return 0; }
The full source code can be found on the examples folder on the eina_list_01.c file.