Tizen Native API
7.0
|
Watch application API.
Required Header
#include <watch_app.h> #include <watch_app_efl.h>
Overview
The Watch Application API provides functions for handling Tizen watch application state changes or system events. Tizen watch application can be shown in the idle screen of the wearable device. This API also provides time utility functions for developing Tizen watch applications. You can develop a watch application that shows exact time using these time utility functions. For low powered wearable device, Tizen watch application supports a special mode that is named 'ambient'. When the device enters ambient mode, Tizen watch application that is shown in the idle screen can show limited UI and receives only ambient tick event at each minute to reduce power consumption. The limitation of UI that can be drawn in the ambient mode depends on the device. Usually, you should draw black and white UI only, and you should use below 20% of the pixels of the screen. If you don't want to draw your own ambient mode UI, you can set the 'ambient-support' attribute of the application as 'false' in the tizen-manifest.xml. Then, the platform will show proper default ambient mode UI. This API provides interfaces for the following categories:
- Starting or exiting the main event loop.
- Registering callbacks for application state change events including timetick events.
- Registering callbacks for basic system events.
- Time related utility APIs for watch applications.
Related Features
This API is related with the following features:
- http://tizen.org/feature/watch_app It is recommended to design feature related codes in your application for reliability. You can check if a device supports the related features for this API by using System Information, thereby controlling the procedure of your application. To ensure your application is only running on the device with specific features, please define the features in your manifest file using the manifest editor in the SDK. More details on featuring your application can be found from Feature Element.
Registering Callbacks for Application State Change Events
The state change events for Tizen watch application is similar to the Tizen UI applications. See the Application. In Tizen watch application, an ambient changed event is added to support ambient mode. Time tick related events are also added to provide an exact time tick for the watch application.
Callback | Description |
---|---|
watch_app_create_cb() | Hook to take necessary actions before the main event loop starts. Your UI generation code should be placed here so that you do not miss any events from your application UI. |
watch_app_control_cb() | Hook to take necessary actions when your application called by another application. When the application gets launch request, this callback function is called. The application can get information about what is to be performed by using App Control API from app_control handle. |
watch_app_resume_cb() | Hook to take necessary actions when an application becomes visible. If anything is relinquished in app_pause_cb() but is necessary to resume the application, it must be re-allocated here. |
watch_app_pause_cb() | Hook to take necessary actions when an application becomes invisible. For example, you might wish to release memory resources so other applications can use these resources. It is important not to starve the application in front, which is interacting with the user. |
watch_app_terminate_cb() | Hook to take necessary actions when your application is terminating. Your application should release all resources, especially any allocations and shared resources must be freed here so other running applications can fully use these shared resources. |
watch_app_ambient_changed_cb() | Hook to take necessary actions when the device enters ambient mode. Your application needs to adopt its UI to be compatibile with the ambient mode. Note that, you only can use very limited colors and pixels of the screen when the device is in the ambient mode. Usually, you should use only black and white to draw the ambient mode UI and use below 20% of the pixels of the screen. If you don't want to draw your own ambient mode UI, you can set the 'ambient-support' attribute of the application as 'false' in the tizen-manifest.xml. Then, the platform will show proper default ambient mode UI. |
watch_app_time_tick_cb() | This callback is called at each second when your application is visible. This callback is not called when your application is not visible or the device is in ambient mode. You can use this tick to update the time that is being displayed by your watch application. |
watch_app_ambient_tick_cb() | This callback is called at each minute when the device is ambient mode. You can use this tick to update the time that is being displayed by your watch application while the device is in ambient mode. You should not do a job that takes long time in this callback. You should update the UI as fast as possible in this callback. The platform might make the device to sleep in short time after the ambient tick expires. |
Refer to the following state diagram to see the possible transitions and callbacks that are called while transition.
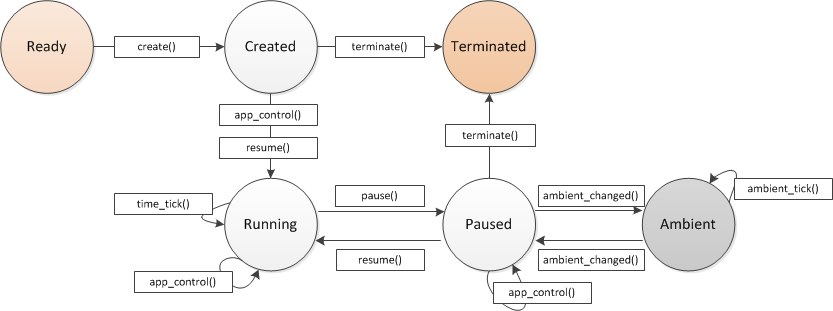
It is almost same as the Tizen UI application. Here are some remarks:
- When your application is in running state, if the device enters the ambient mode, watch_app_pause_cb() will be called before the watch_app_ambient_changed_cb() is called. It is because you need to draw new UI for the ambient mode and release unnecessary resources in the ambient mode.
- When the device returns from the ambient mode, the watch_app_resume_cb() will be called if your application is visible after the device returns from the ambient mode.
- watch_app_time_tick() is only called while your application is visible.
- watch_app_ambient_tick() is only called while the device is in the ambient mode.
Registering Callbacks for System Events
Tizen watch applications can receive system events with watch_app_add_event_handler() API. The type of system events that can be received are same as Tizen UI applications. See Application.
Functions | |
int | watch_app_add_event_handler (app_event_handler_h *handler, app_event_type_e event_type, app_event_cb callback, void *user_data) |
Adds the system event handler. | |
int | watch_app_remove_event_handler (app_event_handler_h event_handler) |
Removes registered event handler. | |
int | watch_app_main (int argc, char **argv, watch_app_lifecycle_callback_s *callback, void *user_data) |
Runs the main loop of the application until watch_app_exit() is called. | |
void | watch_app_exit (void) |
Exits the main loop of the application. | |
int | watch_app_set_time_tick_frequency (int ticks, watch_app_time_tick_resolution_e type) |
Sets the frequency of watch_app_time_tick_cb() calls. | |
int | watch_app_get_time_tick_frequency (int *ticks, watch_app_time_tick_resolution_e *type) |
Gets the frequency of watch_app_time_tick_cb() calls. | |
int | watch_app_set_ambient_tick_type (watch_app_ambient_tick_type_e type) |
Sets the type of periodic ambient tick. | |
int | watch_app_get_ambient_tick_type (watch_app_ambient_tick_type_e *type) |
Gets the type of periodic ambient tick. | |
int | watch_time_get_current_time (watch_time_h *watch_time) |
Gets the current time. | |
int | watch_time_delete (watch_time_h watch_time) |
Deletes the watch time handle and releases all its resources. | |
int | watch_time_get_year (watch_time_h watch_time, int *year) |
Gets the year info. | |
int | watch_time_get_month (watch_time_h watch_time, int *month) |
Gets the month info. | |
int | watch_time_get_day (watch_time_h watch_time, int *day) |
Gets the day info. | |
int | watch_time_get_day_of_week (watch_time_h watch_time, int *day_of_week) |
Gets the day of week info. | |
int | watch_time_get_hour (watch_time_h watch_time, int *hour) |
Gets the hour info. | |
int | watch_time_get_hour24 (watch_time_h watch_time, int *hour24) |
Gets the hour info in 24-hour presentation. | |
int | watch_time_get_minute (watch_time_h watch_time, int *minute) |
Gets the minute info. | |
int | watch_time_get_second (watch_time_h watch_time, int *second) |
Gets the second info. | |
int | watch_time_get_millisecond (watch_time_h watch_time, int *millisecond) |
Gets the millisecond info. | |
int | watch_time_get_utc_time (watch_time_h watch_time, struct tm *utc_time) |
Gets the UTC time. | |
int | watch_time_get_utc_timestamp (watch_time_h watch_time, time_t *utc_timestamp) |
Gets the UTC timestamp. | |
int | watch_time_get_time_zone (watch_time_h watch_time, char **time_zone_id) |
Gets the ID of timezone for the watch_time handle. | |
int | watch_time_get_dst_status (watch_time_h watch_time, bool *status) |
Gets the daylight saving time status. | |
int | watch_app_get_elm_win (Evas_Object **win) |
Gets Evas_Object for a Elementary window of watch application. You must use this window to draw watch UI on the idle screen. | |
Typedefs | |
typedef struct _watch_time_s * | watch_time_h |
watch_time_h watch_time handle. | |
typedef bool(* | watch_app_create_cb )(int width, int height, void *user_data) |
Called when the application starts. | |
typedef void(* | watch_app_control_cb )(app_control_h app_control, void *user_data) |
Called when another application sends a launch request to the application. | |
typedef void(* | watch_app_pause_cb )(void *user_data) |
Called when the application is completely obscured by another application and becomes invisible. | |
typedef void(* | watch_app_resume_cb )(void *user_data) |
Called when the application becomes visible. | |
typedef void(* | watch_app_terminate_cb )(void *user_data) |
Called when the application's main loop exits. | |
typedef void(* | watch_app_time_tick_cb )(watch_time_h watch_time, void *user_data) |
Called with the frequency set with watch_app_set_time_tick_frequency(). | |
typedef void(* | watch_app_ambient_tick_cb )(watch_time_h watch_time, void *user_data) |
Called with the frequency set with watch_app_set_ambient_tick_type() if the device is in the ambient mode. | |
typedef void(* | watch_app_ambient_changed_cb )(bool ambient_mode, void *user_data) |
Called when the device enters or exits the ambient mode. |
Typedef Documentation
typedef void(* watch_app_ambient_changed_cb)(bool ambient_mode, void *user_data) |
Called when the device enters or exits the ambient mode.
- Since :
- 2.3.1
- Remarks:
- For low powered wearable device, Tizen watch application supports a special mode that is named 'ambient'. When the device enters ambient mode, Tizen watch application that is shown in the idle screen can show limited UI and receives only ambient tick event at each minute to reduce power consumption. The limitation of UI that can be drawn in the ambient mode depends on the device. Usually, you should draw black and white UI only, and you should use below 20% of the pixels of the screen. If you don't want to draw your own ambient mode UI, you can set the 'ambient-support' attribute of the application as 'false' in the tizen-manifest.xml. Then, the platform will show proper default ambient mode UI.
- Parameters:
-
[in] ambient_mode If true
the device enters the ambient mode, otherwisefalse
[in] user_data The user data to be passed to the callback functions
typedef void(* watch_app_ambient_tick_cb)(watch_time_h watch_time, void *user_data) |
Called with the frequency set with watch_app_set_ambient_tick_type() if the device is in the ambient mode.
- Since :
- 2.3.1
- Remarks:
- You should not do a job that takes long time in this callback. You should update the UI as fast as possible in this callback. The platform might make the device to sleep in short time after the ambient tick expires. Since 2.3.2, you can control when this callback is called by watch_app_set_ambient_tick_type()
- Parameters:
-
[in] watch_time The watch time handle. watch_time will not be available after returning this callback. It will be freed by the framework. [in] user_data The user data to be passed to the callback functions
- See also:
- watch_app_set_ambient_tick_type()
typedef void(* watch_app_control_cb)(app_control_h app_control, void *user_data) |
Called when another application sends a launch request to the application.
When the application is launched, this callback function is called after the main loop of the application starts up. The passed app_control handle describes the launch request and contains the information about why the application is launched. If the launch request is sent to the application in the running or pause state, this callback function can be called again to notify that the application has been asked to launch. The application is responsible for handling each launch request and responding appropriately. Using the App Control API, the application can get information about what is to be performed. The app_control handle may include only the default operation (APP_CONTROL_OPERATION_DEFAULT) without any data. For more information, see The App Control API description.
- Since :
- 2.3.1
- Parameters:
-
[in] app_control The handle to the app_control [in] user_data The user data passed from the callback registration function
- See also:
- watch_app_main()
- watch_app_lifecycle_callback_s
- App Control API
typedef bool(* watch_app_create_cb)(int width, int height, void *user_data) |
Called when the application starts.
The callback function is called before the main loop of the application starts. In this callback, you can initialize application resources like window creation, data structure, and so on. After this callback function returns true
, the main loop starts up and watch_app_control_cb() is subsequently called. If this callback function returns false
, the main loop doesn't start and watch_app_terminate_cb() is subsequently called.
- Since :
- 2.3.1
- Parameters:
-
[in] width The width of the window of idle screen that will show the watch UI [in] height The height of the window of idle screen that will show the watch UI [in] user_data The user data passed from the callback registration function
- Returns:
true
on success, otherwisefalse
typedef void(* watch_app_pause_cb)(void *user_data) |
Called when the application is completely obscured by another application and becomes invisible.
The application is not terminated and still running in the paused state.
- Since :
- 2.3.1
- Parameters:
-
[in] user_data The user data passed from the callback registration function
typedef void(* watch_app_resume_cb)(void *user_data) |
Called when the application becomes visible.
- Since :
- 2.3.1
- Remarks:
- This callback function is not called when the application moves from the created state to the running state.
- Parameters:
-
[in] user_data The user data passed from the callback registration function
typedef void(* watch_app_terminate_cb)(void *user_data) |
Called when the application's main loop exits.
You should release the application's resources in this function.
- Since :
- 2.3.1
- Parameters:
-
[in] user_data The user data passed from the callback registration function
typedef void(* watch_app_time_tick_cb)(watch_time_h watch_time, void *user_data) |
Called with the frequency set with watch_app_set_time_tick_frequency().
This callback is not called while the app is paused or the device is in ambient mode.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch time handle. watch_time will not be available after returning this callback. It will be freed by the framework. [in] user_data The user data to be passed to the callback functions
typedef struct _watch_time_s* watch_time_h |
watch_time_h watch_time handle.
- Since :
- 2.3.1
Enumeration Type Documentation
Enumeration for periodic ambient tick type.
- Since :
- 2.3.2
- Enumerator:
WATCH_APP_AMBIENT_TICK_NO_TICK No periodic ambient tick. watch_app_ambient_tick_cb() will be called once when it enters the ambient mode.
WATCH_APP_AMBIENT_TICK_EVERY_MINUTE watch_app_ambient_tick_cb() will be called every minute.
WATCH_APP_AMBIENT_TICK_EVERY_FIVE_MINUTES watch_app_ambient_tick_cb() will be called every 5 minutes. It will be called at 12:00 AM, 12:05 AM, 12:10 AM ...
WATCH_APP_AMBIENT_TICK_EVERY_FIFTEEN_MINUTES watch_app_ambient_tick_cb() will be called every 15 minutes. It will be called at 12:00 AM, 12:15 AM, 12:30 AM ...
WATCH_APP_AMBIENT_TICK_EVERY_THIRTY_MINUTES watch_app_ambient_tick_cb() will be called every 30 minutes. It will be called at 12:00 AM, 12:30 AM, 1:00 AM ...
WATCH_APP_AMBIENT_TICK_EVERY_HOUR watch_app_ambient_tick_cb() will be called every hour. It will be called at 12:00 AM, 1:00 AM, 2:00 AM ...
WATCH_APP_AMBIENT_TICK_EVERY_THREE_HOURS watch_app_ambient_tick_cb() will be called every 3 hours. It will be called at 12:00 AM, 3:00 AM, 6:00 AM ...
WATCH_APP_AMBIENT_TICK_EVERY_SIX_HOURS watch_app_ambient_tick_cb() will be called every 6 hours. It will be called at 12:00 AM, 6:00 AM, 12:00 PM, 6:00 PM ...
WATCH_APP_AMBIENT_TICK_EVERY_TWELVE_HOURS watch_app_ambient_tick_cb() will be called every 12 hours. It will be called at 12:00 AM, 12:00 PM
WATCH_APP_AMBIENT_TICK_EVERY_DAY watch_app_ambient_tick_cb() will be called at the start of the day. It will be called at 12:00 AM
Enumeration for tick resolution type.
It is one of the input parameters of the watch_app_set_time_tick_frequency() and watch_app_get_time_tick_frequency() functions.
- Since :
- 3.0
Function Documentation
int watch_app_add_event_handler | ( | app_event_handler_h * | handler, |
app_event_type_e | event_type, | ||
app_event_cb | callback, | ||
void * | user_data | ||
) |
Adds the system event handler.
- Since :
- 2.3.1
- Parameters:
-
[out] handler The event handler [in] event_type The system event type [in] callback The callback function [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_OUT_OF_MEMORY Out of memory APP_ERROR_NOT_SUPPORTED Not supported
void watch_app_exit | ( | void | ) |
Exits the main loop of the application.
The main loop of the application stops and watch_app_terminate_cb() is invoked.
- Since :
- 2.3.1
- See also:
- watch_app_main()
- watch_app_terminate_cb()
int watch_app_get_ambient_tick_type | ( | watch_app_ambient_tick_type_e * | type | ) |
Gets the type of periodic ambient tick.
- Since :
- 2.3.2
- Remarks:
- If you do not set specific tick type with watch_app_set_ambient_tick_type(), this function will set type to WATCH_APP_AMBIENT_TICK_EVERY_MINUTE.
- Parameters:
-
[out] type The type of periodic ambient tick
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_app_get_elm_win | ( | Evas_Object ** | win | ) |
Gets Evas_Object for a Elementary window of watch application. You must use this window to draw watch UI on the idle screen.
- Since :
- 2.3.1
- Parameters:
-
[out] win The pointer of Evas_Object for a Elementary window.
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_OUT_OF_MEMORY Out of memory APP_ERROR_INVALID_CONTEXT Watch app is not initialized properly APP_ERROR_NONE Successful APP_ERROR_NOT_SUPPORTED Not supported
int watch_app_get_time_tick_frequency | ( | int * | ticks, |
watch_app_time_tick_resolution_e * | type | ||
) |
Gets the frequency of watch_app_time_tick_cb() calls.
- Since :
- 3.0
- Parameters:
-
[out] ticks The number of ticks per given resolution type [out] type The resolution type
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_app_main | ( | int | argc, |
char ** | argv, | ||
watch_app_lifecycle_callback_s * | callback, | ||
void * | user_data | ||
) |
Runs the main loop of the application until watch_app_exit() is called.
- Since :
- 2.3.1
- Remarks:
- http://tizen.org/privilege/alarm.set privilege is needed to receive ambient ticks at each minute. The watch_app_ambient_tick_cb() will be ignored if your app doesn't have the privilege.
- Parameters:
-
[in] argc The argument count [in] argv The argument vector [in] callback The set of callback functions to handle application events [in] user_data The user data to be passed to the callback functions
- Returns:
0
on success, otherwise a negative error value.
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_INVALID_CONTEXT The application is illegally launched, not launched by the launch system. APP_ERROR_ALREADY_RUNNING The main loop has already started APP_ERROR_NOT_SUPPORTED Not supported
int watch_app_remove_event_handler | ( | app_event_handler_h | event_handler | ) |
Removes registered event handler.
- Since :
- 2.3.1
- Parameters:
-
[in] event_handler The event handler
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_NOT_SUPPORTED Not supported
- See also:
- watch_app_add_event_handler
Sets the type of periodic ambient tick.
- Since :
- 2.3.2
- Remarks:
- If you do not set specific tick type with this function, the watch_app_ambient_tick_cb() will be called every minute by default.
- Parameters:
-
[in] type The type of periodic ambient tick
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
- See also:
- watch_app_ambient_tick_cb()
int watch_app_set_time_tick_frequency | ( | int | ticks, |
watch_app_time_tick_resolution_e | type | ||
) |
Sets the frequency of watch_app_time_tick_cb() calls.
If watch_app_set_time_tick_frequency() is not called, watch_app_time_tick_cb() will be called every second.
- Since :
- 3.0
- Parameters:
-
[in] ticks The number of ticks per given resolution type [in] type The resolution type
- Returns:
- 0 on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_delete | ( | watch_time_h | watch_time | ) |
Deletes the watch time handle and releases all its resources.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch_time handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_current_time | ( | watch_time_h * | watch_time | ) |
Gets the current time.
- Since :
- 2.3.1
- Remarks:
- You must release watch_time using watch_time_delete() after using it.
- Parameters:
-
[out] watch_time The watch_time handle to be newly created on success
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_OUT_OF_MEMORY Out of Memory APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_day | ( | watch_time_h | watch_time, |
int * | day | ||
) |
Gets the day info.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch_time handle [out] day The day info
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_day_of_week | ( | watch_time_h | watch_time, |
int * | day_of_week | ||
) |
Gets the day of week info.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch_time handle [out] day_of_week The day of week info. The value returns from 1 (Sunday) to 7 (Saturday).
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_dst_status | ( | watch_time_h | watch_time, |
bool * | status | ||
) |
Gets the daylight saving time status.
- Since :
- 4.0
- Parameters:
-
[in] watch_time The watch_time handle [out] status The daylight saving time status; true
if DST is in effect,false
otherwise
- Returns:
- 0 on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_hour | ( | watch_time_h | watch_time, |
int * | hour | ||
) |
Gets the hour info.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch_time handle [out] hour The hour info
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_hour24 | ( | watch_time_h | watch_time, |
int * | hour24 | ||
) |
Gets the hour info in 24-hour presentation.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch_time handle [out] hour24 The hour info
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_millisecond | ( | watch_time_h | watch_time, |
int * | millisecond | ||
) |
Gets the millisecond info.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch_time handle [out] millisecond The millisecond info
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_minute | ( | watch_time_h | watch_time, |
int * | minute | ||
) |
Gets the minute info.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch_time handle [out] minute The minute info
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_month | ( | watch_time_h | watch_time, |
int * | month | ||
) |
Gets the month info.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch_time handle [out] month The month info
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_second | ( | watch_time_h | watch_time, |
int * | second | ||
) |
Gets the second info.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch_time handle [out] second The second info
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_time_zone | ( | watch_time_h | watch_time, |
char ** | time_zone_id | ||
) |
Gets the ID of timezone for the watch_time handle.
- Since :
- 2.3.1
- Remarks:
- You must release time_zone_id using free() after using it.
- Parameters:
-
[in] watch_time The watch_time handle [out] time_zone_id The Timezone ID, such as "America/Los_Angeles"
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_utc_time | ( | watch_time_h | watch_time, |
struct tm * | utc_time | ||
) |
Gets the UTC time.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch_time handle [out] utc_time The UTC time
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_utc_timestamp | ( | watch_time_h | watch_time, |
time_t * | utc_timestamp | ||
) |
Gets the UTC timestamp.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch_time handle [out] utc_timestamp The UTC timestamp
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported
int watch_time_get_year | ( | watch_time_h | watch_time, |
int * | year | ||
) |
Gets the year info.
- Since :
- 2.3.1
- Parameters:
-
[in] watch_time The watch_time handle [out] year The year info
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid Parameter APP_ERROR_NOT_SUPPORTED Not supported