Tizen Native API
7.0
|
Widget to display text, with simple html-like markup.
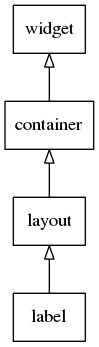
The Label widget doesn't allow text to overflow its boundaries, if the text doesn't fit the geometry of the label it will be ellipsized or be cut. Elementary provides several styles for this widget:
- default - No animation
- marker - Centers the text in the label and makes it bold by default
- slide_long - The entire text appears from the right of the screen and slides until it disappears in the left of the screen(reappearing on the right again).
- slide_short - The text appears in the left of the label and slides to the right to show the overflow. When all of the text has been shown the position is reset.
- slide_bounce - The text appears in the left of the label and slides to the right to show the overflow. When all of the text has been shown the animation reverses, moving the text to the left.
Custom themes can of course invent new markup tags and style them any way they like.
This widget inherits from the Layout one, so that all the functions acting on it also work for label objects.
This widget emits the following signals, besides the ones sent from Layout :
"language,changed"
: The program's language changed."slide,end"
: The slide is end.
See Label example for a demonstration of how to use a label widget.
Functions | |
void | elm_label_wrap_width_set (Elm_Label *obj, int w) |
Control wrap width of the label. | |
int | elm_label_wrap_width_get (const Elm_Label *obj) |
Control wrap width of the label. | |
void | elm_label_slide_speed_set (Elm_Label *obj, double speed) |
Control the slide speed of the label. | |
double | elm_label_slide_speed_get (const Elm_Label *obj) |
Control the slide speed of the label. | |
void | elm_label_slide_mode_set (Elm_Label *obj, Elm_Label_Slide_Mode mode) |
Control the slide mode of the label widget. | |
Elm_Label_Slide_Mode | elm_label_slide_mode_get (const Elm_Label *obj) |
Control the slide mode of the label widget. | |
void | elm_label_slide_duration_set (Elm_Label *obj, double duration) |
Control the slide duration of the label. | |
double | elm_label_slide_duration_get (const Elm_Label *obj) |
Control the slide duration of the label. | |
void | elm_label_line_wrap_set (Elm_Label *obj, Elm_Wrap_Type wrap) |
Control the wrapping behavior of the label. | |
Elm_Wrap_Type | elm_label_line_wrap_get (const Elm_Label *obj) |
Control the wrapping behavior of the label. | |
void | elm_label_ellipsis_set (Elm_Label *obj, Eina_Bool ellipsis) |
Control the ellipsis behavior of the label. | |
Eina_Bool | elm_label_ellipsis_get (const Elm_Label *obj) |
Control the ellipsis behavior of the label. | |
void | elm_label_slide_go (Elm_Label *obj) |
Start slide effect. | |
Evas_Object * | elm_label_add (Evas_Object *parent) |
Add a new label to the parent. |
Function Documentation
Evas_Object* elm_label_add | ( | Evas_Object * | parent | ) |
Add a new label to the parent.
- Parameters:
-
parent The parent object
- Returns:
- The new object or NULL if it cannot be created
- Since :
- 2.3.1
- Examples:
- bubble_example_01.c, fileselector_button_example.c, inwin_example.c, label_example_01.c, map_example_02.c, notify_example_01.c, panel_example_01.c, popup_example_01.c, prefs_example_01.c, prefs_example_02.c, progressbar_example.c, scroller_example_01.c, table_example_01.c, and win_example.c.
Eina_Bool elm_label_ellipsis_get | ( | const Elm_Label * | obj | ) |
Control the ellipsis behavior of the label.
If set to true and the text doesn't fit in the label an ellipsis("...") will be shown at the end of the widget.
Warning This doesn't work with slide(elm_label_slide_set()) or if the chosen wrap method was ELM_WRAP_WORD
.
- Parameters:
-
[in] obj The object.
- Returns:
- To ellipsis text or not
- Since :
- 2.3.1
void elm_label_ellipsis_set | ( | Elm_Label * | obj, |
Eina_Bool | ellipsis | ||
) |
Control the ellipsis behavior of the label.
If set to true and the text doesn't fit in the label an ellipsis("...") will be shown at the end of the widget.
Warning This doesn't work with slide(elm_label_slide_set()) or if the chosen wrap method was ELM_WRAP_WORD
.
- Parameters:
-
[in] obj The object. [in] ellipsis To ellipsis text or not
- Since :
- 2.3.1
- Examples:
- label_example_01.c.
Elm_Wrap_Type elm_label_line_wrap_get | ( | const Elm_Label * | obj | ) |
Control the wrapping behavior of the label.
By default no wrapping is done. Possible values for wrap
are: ELM_WRAP_NONE
- No wrapping ELM_WRAP_CHAR
- wrap between characters ELM_WRAP_WORD
- wrap between words ELM_WRAP_MIXED
- Word wrap, and if that fails, char wrap
- Parameters:
-
[in] obj The object.
- Returns:
- To wrap text or not
- Since :
- 2.3.1
void elm_label_line_wrap_set | ( | Elm_Label * | obj, |
Elm_Wrap_Type | wrap | ||
) |
Control the wrapping behavior of the label.
By default no wrapping is done. Possible values for wrap
are: ELM_WRAP_NONE
- No wrapping ELM_WRAP_CHAR
- wrap between characters ELM_WRAP_WORD
- wrap between words ELM_WRAP_MIXED
- Word wrap, and if that fails, char wrap
- Parameters:
-
[in] obj The object. [in] wrap To wrap text or not
- Since :
- 2.3.1
- Examples:
- label_example_01.c.
double elm_label_slide_duration_get | ( | const Elm_Label * | obj | ) |
Control the slide duration of the label.
- Note:
- If you set the speed of the slide using elm_label_slide_speed_set you cannot get the correct duration using this function until the label is actually rendered and resized.
- Parameters:
-
[in] obj The object.
- Returns:
- The duration in seconds in moving text from slide begin position to slide end position
- Since :
- 2.3.1
void elm_label_slide_duration_set | ( | Elm_Label * | obj, |
double | duration | ||
) |
Control the slide duration of the label.
- Note:
- If you set the speed of the slide using elm_label_slide_speed_set you cannot get the correct duration using this function until the label is actually rendered and resized.
- Parameters:
-
[in] obj The object. [in] duration The duration in seconds in moving text from slide begin position to slide end position
- Since :
- 2.3.1
- Examples:
- label_example_01.c.
void elm_label_slide_go | ( | Elm_Label * | obj | ) |
Elm_Label_Slide_Mode elm_label_slide_mode_get | ( | const Elm_Label * | obj | ) |
Control the slide mode of the label widget.
By default, slide mode is none. Possible values for mode
are: ELM_LABEL_SLIDE_MODE_NONE - no slide effect ELM_LABEL_SLIDE_MODE_AUTO - slide only if the label area is bigger than the text width length ELM_LABEL_SLIDE_MODE_ALWAYS - slide always
- Warning:
- ELM_LABEL_SLIDE_MODE_AUTO, ELM_LABEL_SLIDE_MODE_ALWAYS only work with the themes "slide_short", "slide_long" and "slide_bounce". Warning: ELM_LABEL_SLIDE_MODE_AUTO, ELM_LABEL_SLIDE_MODE_ALWAYS don't work if the line wrap(elm_label_line_wrap_set()) or ellipsis(elm_label_ellipsis_set()) is set.
- Parameters:
-
[in] obj The object.
- Returns:
- The slide mode
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_label_slide_mode_set | ( | Elm_Label * | obj, |
Elm_Label_Slide_Mode | mode | ||
) |
Control the slide mode of the label widget.
By default, slide mode is none. Possible values for mode
are: ELM_LABEL_SLIDE_MODE_NONE - no slide effect ELM_LABEL_SLIDE_MODE_AUTO - slide only if the label area is bigger than the text width length ELM_LABEL_SLIDE_MODE_ALWAYS - slide always
- Warning:
- ELM_LABEL_SLIDE_MODE_AUTO, ELM_LABEL_SLIDE_MODE_ALWAYS only work with the themes "slide_short", "slide_long" and "slide_bounce". Warning: ELM_LABEL_SLIDE_MODE_AUTO, ELM_LABEL_SLIDE_MODE_ALWAYS don't work if the line wrap(elm_label_line_wrap_set()) or ellipsis(elm_label_ellipsis_set()) is set.
- Parameters:
-
[in] obj The object. [in] mode The slide mode
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Examples:
- label_example_01.c.
double elm_label_slide_speed_get | ( | const Elm_Label * | obj | ) |
Control the slide speed of the label.
- Note:
- If you set the duration of the slide using elm_label_slide_duration_set you cannot get the correct speed using this function until the label is actually rendered and resized.
See elm_label_slide_duration_set
- Parameters:
-
[in] obj The object.
- Returns:
- The speed of the slide animation in px per seconds
- Since :
- 3.0
void elm_label_slide_speed_set | ( | Elm_Label * | obj, |
double | speed | ||
) |
Control the slide speed of the label.
- Note:
- If you set the duration of the slide using elm_label_slide_duration_set you cannot get the correct speed using this function until the label is actually rendered and resized.
See elm_label_slide_duration_set
- Parameters:
-
[in] obj The object. [in] speed The speed of the slide animation in px per seconds
- Since :
- 3.0
int elm_label_wrap_width_get | ( | const Elm_Label * | obj | ) |
Control wrap width of the label.
This function sets the maximum width size hint of the label.
- Warning:
- This is only relevant if the label is inside a container.
- Parameters:
-
[in] obj The object.
- Returns:
- The wrap width in pixels at a minimum where words need to wrap
- Since :
- 2.3.1
void elm_label_wrap_width_set | ( | Elm_Label * | obj, |
int | w | ||
) |
Control wrap width of the label.
This function sets the maximum width size hint of the label.
- Warning:
- This is only relevant if the label is inside a container.
- Parameters:
-
[in] obj The object. [in] w The wrap width in pixels at a minimum where words need to wrap
- Since :
- 2.3.1