Tizen Native API
7.0
|
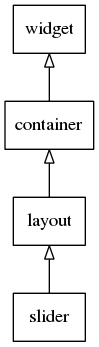
The slider adds a draggable “slider” widget for selecting the value of something within a range.
A slider can be horizontal or vertical. It can contain an Icon and has a primary label as well as a units label (that is formatted with floating point values and thus accepts a printf-style format string, like “%1.2f units”. There is also an indicator string that may be somewhere else (like on the slider itself) that also accepts a format string like units. Label, Icon Unit and Indicator strings/objects are optional.
A slider may be inverted which means values invert, with high vales being on the left or top and low values on the right or bottom (as opposed to normally being low on the left or top and high on the bottom and right).
The slider should have its minimum and maximum values set by the application with elm_slider_min_max_set() and value should also be set by the application before use with elm_slider_value_set(). The span of the slider is its length (horizontally or vertically). This will be scaled by the object or applications scaling factor. At any point code can query the slider for its value with elm_slider_value_get().
This widget inherits from the Layout one, so that all the functions acting on it also work for slider objects.
This widget emits the following signals, besides the ones sent from Layout :
"changed"
- Whenever the slider value is changed by the user."slider,drag,start"
- dragging the slider indicator around has started."slider,drag,stop"
- dragging the slider indicator around has stopped."delay,changed"
- A short time after the value is changed by the user. This will be called only when the user stops dragging for a very short period or when they release their finger/mouse, so it avoids possibly expensive reactions to the value change."focused"
- When the slider has received focus. (since 1.8)"unfocused"
- When the slider has lost focus. (since 1.8)"language,changed"
- the program's language changed (since 1.9)
Available styles for it:
"default"
Default content parts of the slider widget that you can use for are:
- "icon" - An icon of the slider
- "end" - A end part content of the slider
Default text parts of the slider widget that you can use for are:
- "default" - A label of the slider
Supported elm_object common APIs.
- elm_object_disabled_set
- elm_object_disabled_get
- elm_object_part_text_set
- elm_object_part_text_get
- elm_object_part_content_set
- elm_object_part_content_get
- elm_object_part_content_unset
Here is an example on its usage:
Functions | |
void | elm_config_slider_indicator_visible_mode_set (Elm_Slider_Indicator_Visible_Mode mode) |
Elm_Slider_Indicator_Visible_Mode | elm_config_slider_indicator_visible_mode_get (void) |
Evas_Object * | elm_slider_add (Evas_Object *parent) |
void | elm_slider_horizontal_set (Evas_Object *obj, Eina_Bool horizontal) |
Set the orientation of a given slider widget. | |
Eina_Bool | elm_slider_horizontal_get (const Evas_Object *obj) |
void | elm_slider_value_set (Evas_Object *obj, double val) |
Set the value the slider displays. | |
double | elm_slider_value_get (const Evas_Object *obj) |
Get the value displayed by the slider. | |
void | elm_slider_inverted_set (Evas_Object *obj, Eina_Bool inverted) |
Invert a given slider widget's displaying values order. | |
Eina_Bool | elm_slider_inverted_get (const Evas_Object *obj) |
Get whether a given slider widget's displaying values are inverted or not. | |
void | elm_slider_span_size_set (Evas_Object *obj, Evas_Coord size) |
Set the (exact) length of the bar region of a given slider widget. | |
Evas_Coord | elm_slider_span_size_get (const Evas_Object *obj) |
Get the length set for the bar region of a given slider widget. | |
void | elm_slider_unit_format_set (Evas_Object *obj, const char *units) |
Set the format string for the unit label. | |
const char * | elm_slider_unit_format_get (const Evas_Object *obj) |
Get the unit label format of the slider. | |
void | elm_slider_units_format_function_set (Evas_Object *obj, slider_func_type func, slider_freefunc_type free_func) |
Set the format function pointer for the units label. | |
void | elm_slider_min_max_set (Evas_Object *obj, double min, double max) |
Set the minimum and maximum values for the slider. | |
void | elm_slider_min_max_get (const Evas_Object *obj, double *min, double *max) |
Get the minimum and maximum values of the slider. | |
void | elm_slider_indicator_format_set (Evas_Object *obj, const char *indicator) |
Set the format string for the indicator label. | |
const char * | elm_slider_indicator_format_get (const Evas_Object *obj) |
Get the indicator label format of the slider. | |
void | elm_slider_indicator_format_function_set (Evas_Object *obj, slider_func_type func, slider_freefunc_type free_func) |
Set the format function pointer for the indicator label. | |
void | elm_slider_indicator_show_on_focus_set (Evas_Object *obj, Eina_Bool flag) |
Show the indicator of slider on focus. | |
Eina_Bool | elm_slider_indicator_show_on_focus_get (const Evas_Object *obj) |
Get whether the indicator of the slider is set or not. | |
void | elm_slider_indicator_show_set (Evas_Object *obj, Eina_Bool show) |
Set whether to enlarge slider indicator (augmented knob) or not. | |
Eina_Bool | elm_slider_indicator_show_get (const Evas_Object *obj) |
Get whether a given slider widget's enlarging indicator or not. | |
void | elm_slider_indicator_visible_mode_set (Evas_Object *obj, Elm_Slider_Indicator_Visible_Mode indicator_visible_mode) |
Set/Get the visible mode of indicator. | |
Elm_Slider_Indicator_Visible_Mode | elm_slider_indicator_visible_mode_get (const Evas_Object *obj) |
Set/Get the visible mode of indicator. | |
double | elm_slider_step_get (const Evas_Object *obj) |
Get the step by which slider indicator moves. | |
void | elm_slider_step_set (Evas_Object *obj, double step) |
Set the step by which slider indicator will move. |
Function Documentation
Get the slider's indicator visible mode.
- Returns:
ELM_SLIDER_INDICATOR_VISIBLE_MODE_DEFAULT
if not set anything by the user.ELM_SLIDER_INDICATOR_VISIBLE_MODE_ALWAYS
, ELM_SLIDER_INDICATOR_VISIBLE_MODE_ON_FOCUS, ELM_SLIDER_INDICATOR_VISIBLE_MODE_NONE if any of the above is set by user.
- Since (EFL) :
- 1.13
- Since :
- 3.0
Sets the slider's indicator visible mode.
- Parameters:
-
mode Elm_Slider_Indicator_Visible_Mode. viewport.
- Since (EFL) :
- 1.13
- Since :
- 3.0
Evas_Object* elm_slider_add | ( | Evas_Object * | parent | ) |
Add a new slider widget to the given parent Elementary (container) object.
- Parameters:
-
parent The parent object.
- Returns:
- a new slider widget handle or
NULL
, on errors.
This function inserts a new slider widget on the canvas.
- Since :
- 2.3.1
- Examples:
- general_funcs_example.c, gengrid_example.c, photocam_example_01.c, and slider_example.c.
Eina_Bool elm_slider_horizontal_get | ( | const Evas_Object * | obj | ) |
Get the orientation of a given slider widget.
- Since :
- 2.3.1
void elm_slider_horizontal_set | ( | Evas_Object * | obj, |
Eina_Bool | horizontal | ||
) |
Set the orientation of a given slider widget.
Use this function to change how your slider is to be disposed: vertically or horizontally.
By default it's displayed horizontally.
- Parameters:
-
[in] horizontal
- Since :
- 2.3.1
- Examples:
- slider_example.c.
void elm_slider_indicator_format_function_set | ( | Evas_Object * | obj, |
slider_func_type | func, | ||
slider_freefunc_type | free_func | ||
) |
Set the format function pointer for the indicator label.
Set the callback function to format the indicator string.
- Parameters:
-
[in] obj The object. [in] func The indicator format function. [in] free_func The freeing function for the format string.
- Since :
- 2.3.1
- Examples:
- slider_example.c.
const char* elm_slider_indicator_format_get | ( | const Evas_Object * | obj | ) |
Get the indicator label format of the slider.
The slider may display its value somewhere else then unit label, for example, above the slider knob that is dragged around. This function gets the format string used for this.
- Parameters:
-
[in] obj The object.
- Returns:
- The format string for the indicator display.
- Since :
- 2.3.1
void elm_slider_indicator_format_set | ( | Evas_Object * | obj, |
const char * | indicator | ||
) |
Set the format string for the indicator label.
The slider may display its value somewhere else then unit label, for example, above the slider knob that is dragged around. This function sets the format string used for this.
If null
, indicator label won't be visible. If not it sets the format string for the label text. To the label text is provided a floating point value, so the label text can display up to 1 floating point value. Note that this is optional.
Use a format string such as "%1.2f meters" for example, and it will display values like: "3.14 meters" for a value equal to 3.14159.
Default is indicator label disabled.
- Parameters:
-
[in] obj The object. [in] indicator The format string for the indicator display.
- Since :
- 2.3.1
- Examples:
- general_funcs_example.c, gengrid_example.c, and slider_example.c.
Eina_Bool elm_slider_indicator_show_get | ( | const Evas_Object * | obj | ) |
Get whether a given slider widget's enlarging indicator or not.
- Parameters:
-
[in] obj The object.
- Returns:
true
will make it enlarge,false
will let the knob always at default size.
- Since :
- 2.3.1
Eina_Bool elm_slider_indicator_show_on_focus_get | ( | const Evas_Object * | obj | ) |
Get whether the indicator of the slider is set or not.
- Parameters:
-
[in] obj The object.
- Returns:
true
if indicator is shown on focus,false
otherwise
- Since :
- 3.0
void elm_slider_indicator_show_on_focus_set | ( | Evas_Object * | obj, |
Eina_Bool | flag | ||
) |
Show the indicator of slider on focus.
- Parameters:
-
[in] obj The object. [in] flag true
if indicator is shown on focus,false
otherwise
- Since :
- 3.0
void elm_slider_indicator_show_set | ( | Evas_Object * | obj, |
Eina_Bool | show | ||
) |
Set whether to enlarge slider indicator (augmented knob) or not.
By default, indicator will be bigger while dragged by the user.
- Parameters:
-
[in] obj The object. [in] show true
will make it enlarge,false
will let the knob always at default size.
- Since :
- 2.3.1
Set/Get the visible mode of indicator.
- Parameters:
-
[in] obj The object.
- Returns:
- The indicator visible mode.
- Since :
- 3.0
void elm_slider_indicator_visible_mode_set | ( | Evas_Object * | obj, |
Elm_Slider_Indicator_Visible_Mode | indicator_visible_mode | ||
) |
Set/Get the visible mode of indicator.
- Parameters:
-
[in] obj The object. [in] indicator_visible_mode The indicator visible mode.
- Since :
- 3.0
Eina_Bool elm_slider_inverted_get | ( | const Evas_Object * | obj | ) |
Get whether a given slider widget's displaying values are inverted or not.
- Returns:
- Use
true
to makeobj
inverted,false
to bring it back to default, non-inverted values.
- Since :
- 2.3.1
void elm_slider_inverted_set | ( | Evas_Object * | obj, |
Eina_Bool | inverted | ||
) |
Invert a given slider widget's displaying values order.
A slider may be inverted, in which state it gets its values inverted, with high vales being on the left or top and low values on the right or bottom, as opposed to normally have the low values on the former and high values on the latter, respectively, for horizontal and vertical modes.
- Parameters:
-
[in] inverted Use true
to makeobj
inverted,false
to bring it back to default, non-inverted values.
- Since :
- 2.3.1
- Examples:
- slider_example.c.
void elm_slider_min_max_get | ( | const Evas_Object * | obj, |
double * | min, | ||
double * | max | ||
) |
Get the minimum and maximum values of the slider.
- Note:
- If only one value is needed, the other pointer can be passed as
null
.
- Parameters:
-
[out] min The minimum value. [out] max The maximum value.
- Since :
- 2.3.1
void elm_slider_min_max_set | ( | Evas_Object * | obj, |
double | min, | ||
double | max | ||
) |
Set the minimum and maximum values for the slider.
Define the allowed range of values to be selected by the user.
If actual value is less than min
, it will be updated to min
. If it is bigger then max
, will be updated to max
. Actual value can be get with Efl::Ui::Progress::progress_value::get
By default, min is equal to 0.0, and max is equal to 1.0.
- Warning:
- maximum must be greater than minimum, otherwise behavior is undefined.
- Parameters:
-
[in] min The minimum value. [in] max The maximum value.
- Since :
- 2.3.1
- Examples:
- general_funcs_example.c, and slider_example.c.
Evas_Coord elm_slider_span_size_get | ( | const Evas_Object * | obj | ) |
Get the length set for the bar region of a given slider widget.
If that size was not set previously, with elm_slider_span_size_set, this call will return $0.
- Returns:
- The length of the slider's bar region.
- Since :
- 2.3.1
void elm_slider_span_size_set | ( | Evas_Object * | obj, |
Evas_Coord | size | ||
) |
Set the (exact) length of the bar region of a given slider widget.
This sets the minimum width (when in horizontal mode) or height (when in vertical mode) of the actual bar area of the slider obj
. This in turn affects the object's minimum size. Use this when you're not setting other size hints expanding on the given direction (like weight and alignment hints) and you would like it to have a specific size.
- Note:
- Icon, end, label, indicator and unit text around
obj
will require their own space, which will makeobj
to require more thesize
, actually.
- Parameters:
-
[in] size The length of the slider's bar region.
- Since :
- 2.3.1
- Examples:
- gengrid_example.c, and slider_example.c.
double elm_slider_step_get | ( | const Evas_Object * | obj | ) |
Get the step by which slider indicator moves.
- Parameters:
-
[in] obj The object.
- Returns:
- The step value.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_slider_step_set | ( | Evas_Object * | obj, |
double | step | ||
) |
Set the step by which slider indicator will move.
This value is used when a draggable object is moved automatically such as when key events like the up/down/left/right key are pressed or in case accessibility is set and flick event is used to inc/dec slider values. By default step value is 0.05.
- Parameters:
-
[in] obj The object. [in] step The step value.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
const char* elm_slider_unit_format_get | ( | const Evas_Object * | obj | ) |
Get the unit label format of the slider.
Unit label is displayed all the time, if set, after slider's bar. In horizontal mode, at right and in vertical mode, at bottom.
- Returns:
- The format string for the unit display.
- Since :
- 2.3.1
void elm_slider_unit_format_set | ( | Evas_Object * | obj, |
const char * | units | ||
) |
Set the format string for the unit label.
Unit label is displayed all the time, if set, after slider's bar. In horizontal mode, at right and in vertical mode, at bottom.
If null
, unit label won't be visible. If not it sets the format string for the label text. To the label text is provided a floating point value, so the label text can display up to 1 floating point value. Note that this is optional.
Use a format string such as "%1.2f meters" for example, and it will display values like: "3.14 meters" for a value equal to 3.14159.
Default is unit label disabled.
- Parameters:
-
[in] units The format string for the unit display.
- Since :
- 2.3.1
- Examples:
- general_funcs_example.c, and slider_example.c.
void elm_slider_units_format_function_set | ( | Evas_Object * | obj, |
slider_func_type | func, | ||
slider_freefunc_type | free_func | ||
) |
Set the format function pointer for the units label.
Set the callback function to format the units string.
- Parameters:
-
[in] func The units format function. [in] free_func The freeing function for the format string.
- Since :
- 2.3.1
double elm_slider_value_get | ( | const Evas_Object * | obj | ) |
Get the value displayed by the slider.
- Returns:
- The value to be displayed.
- Since :
- 2.3.1
- Examples:
- general_funcs_example.c, gengrid_example.c, photocam_example_01.c, and slider_example.c.
void elm_slider_value_set | ( | Evas_Object * | obj, |
double | val | ||
) |
Set the value the slider displays.
Value will be presented on the unit label following format specified with elm_slider_unit_format_set and on indicator with elm_slider_indicator_format_set.
- Note:
- The value must to be between min and max values. This values are set by elm_slider_min_max_set.
- Parameters:
-
[in] val The value to be displayed.
- Since :
- 2.3.1
- Examples:
- gengrid_example.c, and slider_example.c.