Tizen Native API
7.0
|
The STT API provides functions to recognize the speech.
Required Header
#include <stt.h>
Overview
A main function of Speech-To-Text (below STT) API recognizes sound data recorded by users. After choosing a language, applications will start recording and recognizing. After recording, the applications will receive the recognized result. To use of STT, use the following steps:
1. Create a handle
2. Register callback functions for notifications
3. Prepare stt-service asynchronously
4. Start recording for recognition
5. Stop recording
6. Get result after processing
7. Destroy a handle
The STT has a client-server for the service of multi-applications. The STT service always works in the background as a server. If the service is not working, client library will invoke it and client will communicate with it. The service has engines and the recorder so client does not have the recorder itself. Only the client request commands to the STT service for using STT.
State Diagram
The following diagram shows the life cycle and the states of the STT.
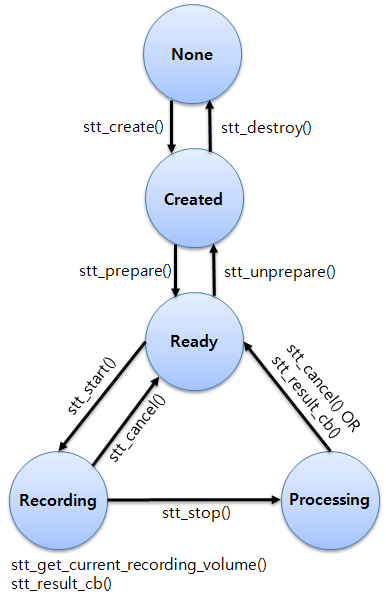
State Transitions
FUNCTION | PRE-STATE | POST-STATE | SYNC TYPE |
---|---|---|---|
stt_prepare() | Created | Ready | ASYNC |
stt_start() | Ready | Recording | ASYNC |
stt_stop() | Recording | Processing | ASYNC |
stt_cancel() | Recording or Processing | Ready | ASYNC |
State Dependent Function Calls
The following table shows state-dependent function calls. It is forbidden to call functions listed below in wrong states. Violation of this rule may result in an unpredictable behavior.
Related Features
This API is related with the following features:
- http://tizen.org/feature/speech.recognition
- http://tizen.org/feature/microphone
It is recommended to design feature related codes in your application for reliability.
You can check if a device supports the related features for this API by using System Information, thereby controlling the procedure of your application.
To ensure your application is only running on the device with specific features, please define the features in your manifest file using the manifest editor in the SDK.
More details on featuring your application can be found from Feature Element.
Functions | |
int | stt_create (stt_h *stt) |
Creates a STT handle. | |
int | stt_destroy (stt_h stt) |
Destroys a STT handle. | |
int | stt_foreach_supported_engines (stt_h stt, stt_supported_engine_cb callback, void *user_data) |
Retrieves supported engine information using a callback function. | |
int | stt_get_engine (stt_h stt, char **engine_id) |
Gets the current engine ID. | |
int | stt_set_engine (stt_h stt, const char *engine_id) |
Sets the engine ID. | |
int | stt_set_credential (stt_h stt, const char *credential) |
Sets the app credential. | |
int | stt_set_private_data (stt_h stt, const char *key, const char *data) |
Sets the private data to stt engine. | |
int | stt_get_private_data (stt_h stt, const char *key, char **data) |
Gets the private data from stt engine. | |
int | stt_prepare (stt_h stt) |
Connects the STT service asynchronously. | |
int | stt_unprepare (stt_h stt) |
Disconnects the STT service. | |
int | stt_foreach_supported_languages (stt_h stt, stt_supported_language_cb callback, void *user_data) |
Retrieves all supported languages of current engine using callback function. | |
int | stt_get_default_language (stt_h stt, char **language) |
Gets the default language set by the user. | |
int | stt_get_state (stt_h stt, stt_state_e *state) |
Gets the current STT state. | |
int | stt_get_error_message (stt_h stt, char **err_msg) |
Gets the current error message. | |
int | stt_is_recognition_type_supported (stt_h stt, const char *type, bool *support) |
Checks whether the recognition type is supported. | |
int | stt_set_silence_detection (stt_h stt, stt_option_silence_detection_e type) |
Sets the silence detection. | |
int | stt_set_start_sound (stt_h stt, const char *filename) |
Sets the sound to start recording. | |
int | stt_unset_start_sound (stt_h stt) |
Unsets the sound to start recording. | |
int | stt_set_stop_sound (stt_h stt, const char *filename) |
Sets the sound to stop recording. | |
int | stt_unset_stop_sound (stt_h stt) |
Unsets the sound to stop recording. | |
int | stt_start (stt_h stt, const char *language, const char *type) |
Starts recording and recognition asynchronously. | |
int | stt_stop (stt_h stt) |
Finishes the recording and starts recognition processing in engine asynchronously. | |
int | stt_cancel (stt_h stt) |
Cancels processing recognition and recording asynchronously. | |
int | stt_get_recording_volume (stt_h stt, float *volume) |
Gets the microphone volume during recording. | |
int | stt_foreach_detailed_result (stt_h stt, stt_result_time_cb callback, void *user_data) |
Retrieves the time stamp of the current recognition result using the callback function. | |
int | stt_set_recognition_result_cb (stt_h stt, stt_recognition_result_cb callback, void *user_data) |
Sets a callback function to get the recognition result. | |
int | stt_unset_recognition_result_cb (stt_h stt) |
Unsets the callback function. | |
int | stt_set_state_changed_cb (stt_h stt, stt_state_changed_cb callback, void *user_data) |
Sets a callback function to be called when STT state changes. | |
int | stt_unset_state_changed_cb (stt_h stt) |
Unsets the callback function. | |
int | stt_set_error_cb (stt_h stt, stt_error_cb callback, void *user_data) |
Sets a callback function to be called when an error occurred. | |
int | stt_unset_error_cb (stt_h stt) |
Unsets the callback function. | |
int | stt_set_default_language_changed_cb (stt_h stt, stt_default_language_changed_cb callback, void *user_data) |
Sets a callback function to detect the default language change. | |
int | stt_unset_default_language_changed_cb (stt_h stt) |
Unsets the callback function. | |
int | stt_set_engine_changed_cb (stt_h stt, stt_engine_changed_cb callback, void *user_data) |
Sets a callback function to detect the engine change. | |
int | stt_unset_engine_changed_cb (stt_h stt) |
Unsets the callback function. | |
int | stt_set_speech_status_cb (stt_h stt, stt_speech_status_cb callback, void *user_data) |
Sets a callback function to detect the speech status is changed. | |
int | stt_unset_speech_status_cb (stt_h stt) |
Unsets the callback function to detect the speech status is changed. | |
Typedefs | |
typedef struct stt_s * | stt_h |
A structure of STT handle. | |
typedef bool(* | stt_supported_engine_cb )(stt_h stt, const char *engine_id, const char *engine_name, void *user_data) |
Called to get the engine information. | |
typedef void(* | stt_recognition_result_cb )(stt_h stt, stt_result_event_e event, const char **data, int data_count, const char *msg, void *user_data) |
Called when STT gets the recognition result from the engine. | |
typedef bool(* | stt_result_time_cb )(stt_h stt, int index, stt_result_time_event_e event, const char *text, long start_time, long end_time, void *user_data) |
Called when STT get the result time stamp in free partial type. | |
typedef void(* | stt_state_changed_cb )(stt_h stt, stt_state_e previous, stt_state_e current, void *user_data) |
Called when the state of STT is changed. | |
typedef void(* | stt_error_cb )(stt_h stt, stt_error_e reason, void *user_data) |
Called when an error occurs. | |
typedef bool(* | stt_supported_language_cb )(stt_h stt, const char *language, void *user_data) |
Called to retrieve the supported languages. | |
typedef void(* | stt_default_language_changed_cb )(stt_h stt, const char *previous_language, const char *current_language, void *user_data) |
Called when the default language is changed. | |
typedef void(* | stt_engine_changed_cb )(stt_h stt, const char *engine_id, const char *language, bool support_silence, bool need_credential, void *user_data) |
Called when the engine is changed. | |
typedef void(* | stt_speech_status_cb )(stt_h stt, stt_speech_status_e status, void *user_data) |
Called when STT engine detects the beginning or end of the speech. | |
Defines | |
#define | STT_RECOGNITION_TYPE_FREE "stt.recognition.type.FREE" |
Definition for free form dictation and default type. | |
#define | STT_RECOGNITION_TYPE_FREE_PARTIAL "stt.recognition.type.FREE.PARTIAL" |
Definition for continuous free dictation. | |
#define | STT_RECOGNITION_TYPE_SEARCH "stt.recognition.type.SEARCH" |
Definition for search. | |
#define | STT_RECOGNITION_TYPE_WEB_SEARCH "stt.recognition.type.WEB_SEARCH" |
Definition for web search. | |
#define | STT_RECOGNITION_TYPE_MAP "stt.recognition.type.MAP" |
Definition for map. | |
#define | STT_RESULT_MESSAGE_NONE "stt.result.message.none" |
Definition for none message. | |
#define | STT_RESULT_MESSAGE_ERROR_TOO_SOON "stt.result.message.error.too.soon" |
Definition for failed recognition because the speech started too soon. | |
#define | STT_RESULT_MESSAGE_ERROR_TOO_SHORT "stt.result.message.error.too.short" |
Definition for failed recognition because the speech is too short. | |
#define | STT_RESULT_MESSAGE_ERROR_TOO_LONG "stt.result.message.error.too.long" |
Definition for failed recognition because the speech is too long. | |
#define | STT_RESULT_MESSAGE_ERROR_TOO_QUIET "stt.result.message.error.too.quiet" |
Definition for failed recognition because the speech is too quiet to listen. | |
#define | STT_RESULT_MESSAGE_ERROR_TOO_LOUD "stt.result.message.error.too.loud" |
Definition for failed recognition because the speech is too loud to listen. | |
#define | STT_RESULT_MESSAGE_ERROR_TOO_FAST "stt.result.message.error.too.fast" |
Definition for failed recognition because the speech is too fast to listen. |
Define Documentation
#define STT_RECOGNITION_TYPE_FREE "stt.recognition.type.FREE" |
Definition for free form dictation and default type.
- Since :
- 2.3.1
#define STT_RECOGNITION_TYPE_FREE_PARTIAL "stt.recognition.type.FREE.PARTIAL" |
Definition for continuous free dictation.
- Since :
- 2.3.1
#define STT_RECOGNITION_TYPE_MAP "stt.recognition.type.MAP" |
Definition for map.
- Since :
- 2.3.1
#define STT_RECOGNITION_TYPE_SEARCH "stt.recognition.type.SEARCH" |
Definition for search.
- Since :
- 2.3.1
#define STT_RECOGNITION_TYPE_WEB_SEARCH "stt.recognition.type.WEB_SEARCH" |
Definition for web search.
- Since :
- 2.3.1
#define STT_RESULT_MESSAGE_ERROR_TOO_FAST "stt.result.message.error.too.fast" |
Definition for failed recognition because the speech is too fast to listen.
- Since :
- 2.3.1
#define STT_RESULT_MESSAGE_ERROR_TOO_LONG "stt.result.message.error.too.long" |
Definition for failed recognition because the speech is too long.
- Since :
- 2.3.1
#define STT_RESULT_MESSAGE_ERROR_TOO_LOUD "stt.result.message.error.too.loud" |
Definition for failed recognition because the speech is too loud to listen.
- Since :
- 2.3.1
#define STT_RESULT_MESSAGE_ERROR_TOO_QUIET "stt.result.message.error.too.quiet" |
Definition for failed recognition because the speech is too quiet to listen.
- Since :
- 2.3.1
#define STT_RESULT_MESSAGE_ERROR_TOO_SHORT "stt.result.message.error.too.short" |
Definition for failed recognition because the speech is too short.
- Since :
- 2.3.1
#define STT_RESULT_MESSAGE_ERROR_TOO_SOON "stt.result.message.error.too.soon" |
Definition for failed recognition because the speech started too soon.
- Since :
- 2.3.1
#define STT_RESULT_MESSAGE_NONE "stt.result.message.none" |
Definition for none message.
- Since :
- 2.3.1
Typedef Documentation
typedef void(* stt_default_language_changed_cb)(stt_h stt, const char *previous_language, const char *current_language, void *user_data) |
Called when the default language is changed.
- Since :
- 2.3.1
- Parameters:
-
[in] stt The STT handle [in] previous_language A previous language [in] current_language A current language [in] user_data The user data passed from the callback registration function
typedef void(* stt_engine_changed_cb)(stt_h stt, const char *engine_id, const char *language, bool support_silence, bool need_credential, void *user_data) |
Called when the engine is changed.
- Since :
- 2.3.2
- Remarks:
- The language is specified as an ISO 3166 alpha-2 two letter country-code followed by ISO 639-1 for the two-letter language code. For example, "ko_KR" for Korean, "en_US" for American English.
- Parameters:
-
[in] stt The STT handle [in] engine_id Engine ID [in] language The default language [in] support_silence Whether the silence detection is supported or not [in] need_credential The necessity of credential [in] user_data The user data passed from the callback registration function
- See also:
- stt_set_engine_changed_cb()
typedef void(* stt_error_cb)(stt_h stt, stt_error_e reason, void *user_data) |
Called when an error occurs.
- Since :
- 2.3.1
- Parameters:
-
[in] stt The STT handle [in] reason The error type (e.g. STT_ERROR_OUT_OF_NETWORK, STT_ERROR_IO_ERROR) [in] user_data The user data passed from the callback registration function
- Precondition:
- An application registers this callback using stt_set_error_cb() to detect error.
- See also:
- stt_set_error_cb()
- stt_unset_error_cb()
typedef struct stt_s* stt_h |
A structure of STT handle.
- Since :
- 2.3.1
typedef void(* stt_recognition_result_cb)(stt_h stt, stt_result_event_e event, const char **data, int data_count, const char *msg, void *user_data) |
Called when STT gets the recognition result from the engine.
- Since :
- 2.3.1
- Remarks:
- After stt_stop() is called, silence is detected from recording, or partial result is occurred, this function is called.
- Parameters:
-
[in] stt The STT handle [in] event The result event [in] data Result texts [in] data_count Result text count [in] msg Engine message (e.g. STT_RESULT_MESSAGE_NONE, STT_RESULT_MESSAGE_ERROR_TOO_SHORT) [in] user_data The user data passed from the callback registration function
- Precondition:
- stt_stop() will invoke this callback if you register it using stt_set_result_cb().
- Postcondition:
- If this function is called and event is STT_RESULT_EVENT_FINAL_RESULT, the STT state will be STT_STATE_READY.
typedef bool(* stt_result_time_cb)(stt_h stt, int index, stt_result_time_event_e event, const char *text, long start_time, long end_time, void *user_data) |
Called when STT get the result time stamp in free partial type.
- Since :
- 2.3.1
- Parameters:
-
[in] stt The STT handle [in] index The result index [in] event The token event [in] text The result text [in] start_time The start time of result text [in] end_time The end time of result text [in] user_data The user data passed from the foreach function
- Returns:
true
to continue with the next iteration of the loop otherwisefalse
to break out of the loop
- Precondition:
- stt_recognition_result_cb() should be called.
- See also:
- stt_recognition_result_cb()
typedef void(* stt_speech_status_cb)(stt_h stt, stt_speech_status_e status, void *user_data) |
Called when STT engine detects the beginning or end of the speech.
- Since :
- 5.5
- Parameters:
-
[in] stt The STT handle [in] status The speech status [in] user_data The user data passed from the callback registration function
- See also:
- stt_set_speech_status_cb()
typedef void(* stt_state_changed_cb)(stt_h stt, stt_state_e previous, stt_state_e current, void *user_data) |
Called when the state of STT is changed.
- Since :
- 2.3.1
- Parameters:
-
[in] stt The STT handle [in] previous A previous state [in] current A current state [in] user_data The user data passed from the callback registration function
- Precondition:
- An application registers this callback using stt_set_state_changed_cb() to detect changing state.
typedef bool(* stt_supported_engine_cb)(stt_h stt, const char *engine_id, const char *engine_name, void *user_data) |
Called to get the engine information.
- Since :
- 2.3.1
- Parameters:
-
[in] stt The STT handle [in] engine_id Engine ID [in] engine_name Engine name [in] user_data User data passed from the stt_setting_foreach_supported_engines()
- Returns:
true
to continue with the next iteration of the loop, otherwisefalse
to break out of the loop
- Precondition:
- stt_foreach_supported_engines() will invoke this callback.
- See also:
- stt_foreach_supported_engines()
typedef bool(* stt_supported_language_cb)(stt_h stt, const char *language, void *user_data) |
Called to retrieve the supported languages.
- Since :
- 2.3.1
- Remarks:
- The language is specified as an ISO 3166 alpha-2 two letter country-code followed by ISO 639-1 for the two-letter language code. For example, "ko_KR" for Korean, "en_US" for American English.
- Parameters:
-
[in] stt The STT handle [in] language The language [in] user_data The user data passed from the foreach function
- Returns:
true
to continue with the next iteration of the loop,false
to break out of the loop
- Precondition:
- stt_foreach_supported_languages() will invoke this callback.
- See also:
- stt_foreach_supported_languages()
Enumeration Type Documentation
enum stt_error_e |
Enumeration for error codes.
- Since :
- 2.3.1
- Enumerator:
enum stt_result_event_e |
enum stt_speech_status_e |
enum stt_state_e |
Function Documentation
int stt_cancel | ( | stt_h | stt | ) |
Cancels processing recognition and recording asynchronously.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- This function cancels recording and engine cancels recognition processing. After successful cancel, stt_state_changed_cb() is called otherwise if error is occurred, stt_error_cb() is called.
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied STT_ERROR_IN_PROGRESS_TO_READY Progress to ready is not finished STT_ERROR_IN_PROGRESS_TO_RECORDING Progress to recording is not finished STT_ERROR_IN_PROGRESS_TO_PROCESSING Progress to processing is not finished
- Precondition:
- The state should be STT_STATE_RECORDING or STT_STATE_PROCESSING.
- Postcondition:
- It will invoke stt_state_changed_cb(), if you register a callback with stt_state_changed_cb(). If this function succeeds, the STT state will be STT_STATE_READY. If you call this function again before state changes, you will receive STT_ERROR_IN_PROGRESS_TO_READY.
- See also:
- stt_start()
- stt_stop()
- stt_state_changed_cb()
int stt_create | ( | stt_h * | stt | ) |
Creates a STT handle.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If the function succeeds, stt handle must be released with stt_destroy().
- Parameters:
-
[out] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_OUT_OF_MEMORY Out of memory STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Postcondition:
- If this function is called, the STT state will be STT_STATE_CREATED.
- See also:
- stt_destroy()
int stt_destroy | ( | stt_h | stt | ) |
Destroys a STT handle.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- See also:
- stt_create()
int stt_foreach_detailed_result | ( | stt_h | stt, |
stt_result_time_cb | callback, | ||
void * | user_data | ||
) |
Retrieves the time stamp of the current recognition result using the callback function.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- This function should be called in stt_recognition_result_cb(). After stt_recognition_result_cb(), result data is NOT valid.
- Parameters:
-
[in] stt The STT handle [in] callback The callback function to invoke [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- This function should be called in stt_recognition_result_cb().
- Postcondition:
- This function invokes stt_result_time_cb() repeatedly for getting time information.
int stt_foreach_supported_engines | ( | stt_h | stt, |
stt_supported_engine_cb | callback, | ||
void * | user_data | ||
) |
Retrieves supported engine information using a callback function.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle [in] callback The callback function to invoke [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Success STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE STT Not initialized STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_CREATED.
- Postcondition:
- This function invokes stt_supported_engine_cb() repeatedly for getting engine information.
- See also:
- stt_supported_engine_cb()
int stt_foreach_supported_languages | ( | stt_h | stt, |
stt_supported_language_cb | callback, | ||
void * | user_data | ||
) |
Retrieves all supported languages of current engine using callback function.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle [in] callback The callback function to invoke [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_OUT_OF_MEMORY Out of memory STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_ENGINE_NOT_FOUND No available engine STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Postcondition:
- This function invokes stt_supported_language_cb() repeatedly for getting languages.
int stt_get_default_language | ( | stt_h | stt, |
char ** | language | ||
) |
Gets the default language set by the user.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- The language is specified as an ISO 3166 alpha-2 two letter country-code followed by ISO 639-1 for the two-letter language code. For example, "ko_KR" for Korean, "en_US" for American English. If the function succeeds, language must be released using free() when it is no longer required.
- Parameters:
-
[in] stt The STT handle [out] language The language
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- See also:
- stt_foreach_supported_languages()
int stt_get_engine | ( | stt_h | stt, |
char ** | engine_id | ||
) |
Gets the current engine ID.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If the function is success, engine_id must be released using free().
- Parameters:
-
[in] stt The STT handle [out] engine_id Engine ID
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Success STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE STT Not initialized STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_CREATED.
- See also:
- stt_set_engine()
int stt_get_error_message | ( | stt_h | stt, |
char ** | err_msg | ||
) |
Gets the current error message.
- Since :
- 2.3.2
- Remarks:
- This function should be called during an stt error callback. If not, the error as operation failure will be returned. If the function succeeds, err_msg must be released using free() when it is no longer required.
- Parameters:
-
[in] stt The STT handle [out] err_msg The current error message
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_OPERATION_FAILED Operation failure
- See also:
- stt_set_error_cb()
- stt_unset_error_cb()
int stt_get_private_data | ( | stt_h | stt, |
const char * | key, | ||
char ** | data | ||
) |
Gets the private data from stt engine.
The private data is the information provided by the engine. Using this API, the application can get the private data which corresponds to the key from the engine.
- Since :
- 2.3.2
- Remarks:
- If the engine is replaced with the other engine, the key may be ignored.
- The data must be released using free() when it is no longer required.
- Parameters:
-
[in] stt The STT handle [in] key The field name of private data [out] data The data field of private data
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_TIMED_OUT No answer from the STT service
- Precondition:
- The state should be STT_STATE_READY.
- See also:
- stt_set_private_data()
int stt_get_recording_volume | ( | stt_h | stt, |
float * | volume | ||
) |
Gets the microphone volume during recording.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle [out] volume Recording volume
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_RECORDING.
- See also:
- stt_start()
int stt_get_state | ( | stt_h | stt, |
stt_state_e * | state | ||
) |
Gets the current STT state.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle [out] state The current STT state
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
int stt_is_recognition_type_supported | ( | stt_h | stt, |
const char * | type, | ||
bool * | support | ||
) |
Checks whether the recognition type is supported.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle [in] type The type for recognition (e.g. STT_RECOGNITION_TYPE_FREE, STT_RECOGNITION_TYPE_FREE_PARTIAL) [out] support The result status true
= supported,false
= not supported
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_READY.
int stt_prepare | ( | stt_h | stt | ) |
Connects the STT service asynchronously.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_CREATED.
- Postcondition:
- If this function is successful, the STT state will be STT_STATE_READY. If this function is failed, the error callback is called. (e.g. STT_ERROR_ENGINE_NOT_FOUND)
- See also:
- stt_unprepare()
int stt_set_credential | ( | stt_h | stt, |
const char * | credential | ||
) |
Sets the app credential.
Using this API, the application can set a credential. The credential is a key to verify the authorization about using the engine. If the application sets the credential, it will be able to use functions of the engine entirely.
- Since :
- 2.3.2
- Remarks:
- The necessity of the credential depends on the engine. In case of the engine which is basically embedded in Tizen, the credential is not necessary so far. However, if the user wants to apply the 3rd party's engine, the credential may be necessary. In that case, please follow the policy provided by the corresponding engine.
- Parameters:
-
[in] stt The STT handle [in] credential The app credential
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Success STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported
- Precondition:
- The state should be STT_STATE_CREATED or STT_STATE_READY.
- See also:
- stt_start()
int stt_set_default_language_changed_cb | ( | stt_h | stt, |
stt_default_language_changed_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to detect the default language change.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_CREATED.
int stt_set_engine | ( | stt_h | stt, |
const char * | engine_id | ||
) |
Sets the engine ID.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Privilege:
- http://tizen.org/privilege/appmanager.launch
- Remarks:
- A privilege (http://tizen.org/privilege/appmanager.launch) is necessary since 3.0.
- Parameters:
-
[in] stt The STT handle [in] engine_id Engine ID
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Success STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE STT Not initialized STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_CREATED.
- See also:
- stt_get_engine()
int stt_set_engine_changed_cb | ( | stt_h | stt, |
stt_engine_changed_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to detect the engine change.
- Since :
- 2.3.2
- Parameters:
-
[in] stt The STT handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported
- Precondition:
- The state should be STT_STATE_CREATED.
int stt_set_error_cb | ( | stt_h | stt, |
stt_error_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to be called when an error occurred.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_CREATED.
- See also:
- stt_error_cb()
- stt_unset_error_cb()
int stt_set_private_data | ( | stt_h | stt, |
const char * | key, | ||
const char * | data | ||
) |
Sets the private data to stt engine.
The private data is the setting parameter for applying keys provided by the engine. Using this API, the application can set the private data and use the corresponding key of the engine. For example, if the engine provides 'partial recognition' as a recognition type, the application can set the private data as the following. int ret = stt_set_private_data(stt_h, "recognition_type", "PARTIAL");
- Since :
- 2.3.2
- Remarks:
- If the engine is replaced with the other engine, the key may be ignored.
- Parameters:
-
[in] stt The STT handle [in] key The field name of private data [in] data The data for set
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_TIMED_OUT No answer from the STT service
- Precondition:
- The state should be STT_STATE_READY.
- See also:
- stt_get_private_data()
int stt_set_recognition_result_cb | ( | stt_h | stt, |
stt_recognition_result_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to get the recognition result.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_CREATED.
int stt_set_silence_detection | ( | stt_h | stt, |
stt_option_silence_detection_e | type | ||
) |
Sets the silence detection.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle [in] type The option type
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED_FEATURE Not supported feature of current engine STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_READY.
int stt_set_speech_status_cb | ( | stt_h | stt, |
stt_speech_status_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to detect the speech status is changed.
- Since :
- 5.5
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be STT_STATE_CREATED.
int stt_set_start_sound | ( | stt_h | stt, |
const char * | filename | ||
) |
Sets the sound to start recording.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- Sound file type should be wav type.
- Parameters:
-
[in] stt The STT handle [in] filename The sound file path
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_READY.
int stt_set_state_changed_cb | ( | stt_h | stt, |
stt_state_changed_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to be called when STT state changes.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_CREATED.
int stt_set_stop_sound | ( | stt_h | stt, |
const char * | filename | ||
) |
Sets the sound to stop recording.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- Sound file type should be wav type.
- Parameters:
-
[in] stt The STT handle [in] filename The sound file path
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_READY.
Starts recording and recognition asynchronously.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- This function starts recording in the STT service and sending recording data to engine. This work continues until stt_stop(), stt_cancel() or silence detected by engine.
- Parameters:
-
[in] stt The STT handle [in] language The language selected from stt_foreach_supported_languages() [in] type The type for recognition (e.g. STT_RECOGNITION_TYPE_FREE, STT_RECOGNITION_TYPE_FREE_PARTIAL)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_RECORDER_BUSY Recorder busy STT_ERROR_INVALID_LANGUAGE Invalid language STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied STT_ERROR_IN_PROGRESS_TO_RECORDING Progress to recording is not finished
- Precondition:
- The state should be STT_STATE_READY.
- Postcondition:
- It will invoke stt_state_changed_cb(), if you register a callback with stt_state_changed_cb(). If this function succeeds, the STT state will be STT_STATE_RECORDING. If you call this function again before state changes, you will receive STT_ERROR_IN_PROGRESS_TO_RECORDING.
- See also:
- stt_stop()
- stt_cancel()
- stt_state_changed_cb()
Finishes the recording and starts recognition processing in engine asynchronously.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied STT_ERROR_IN_PROGRESS_TO_READY Progress to ready is not finished STT_ERROR_IN_PROGRESS_TO_RECORDING Progress to recording is not finished STT_ERROR_IN_PROGRESS_TO_PROCESSING Progress to processing is not finished
- Precondition:
- The state should be STT_STATE_RECORDING.
- Postcondition:
- It will invoke stt_state_changed_cb(), if you register a callback with stt_state_changed_cb(). If this function succeeds, the STT state will be STT_STATE_PROCESSING. If you call this function again before state changes, you will receive STT_ERROR_IN_PROGRESS_TO_PROCESSING. After processing of engine, stt_result_cb() is called.
int stt_unprepare | ( | stt_h | stt | ) |
Disconnects the STT service.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_READY.
- Postcondition:
- If this function is called, the STT state will be STT_STATE_CREATED.
- See also:
- stt_prepare()
int stt_unset_default_language_changed_cb | ( | stt_h | stt | ) |
Unsets the callback function.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_CREATED.
int stt_unset_engine_changed_cb | ( | stt_h | stt | ) |
Unsets the callback function.
- Since :
- 2.3.2
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported
- Precondition:
- The state should be STT_STATE_CREATED.
- See also:
- stt_set_engine_changed_cb()
int stt_unset_error_cb | ( | stt_h | stt | ) |
Unsets the callback function.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_CREATED.
- See also:
- stt_set_error_cb()
int stt_unset_recognition_result_cb | ( | stt_h | stt | ) |
Unsets the callback function.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_CREATED.
- See also:
- stt_set_recognition_result_cb()
int stt_unset_speech_status_cb | ( | stt_h | stt | ) |
Unsets the callback function to detect the speech status is changed.
- Since :
- 5.5
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be STT_STATE_CREATED.
- See also:
- stt_set_speech_status_cb()
int stt_unset_start_sound | ( | stt_h | stt | ) |
Unsets the sound to start recording.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_READY.
int stt_unset_state_changed_cb | ( | stt_h | stt | ) |
Unsets the callback function.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_CREATED.
- See also:
- stt_set_state_changed_cb()
int stt_unset_stop_sound | ( | stt_h | stt | ) |
Unsets the sound to stop recording.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Parameters:
-
[in] stt The STT handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
STT_ERROR_NONE Successful STT_ERROR_INVALID_PARAMETER Invalid parameter STT_ERROR_INVALID_STATE Invalid state STT_ERROR_OPERATION_FAILED Operation failure STT_ERROR_NOT_SUPPORTED STT NOT supported STT_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The state should be STT_STATE_READY.