Tizen Native API
7.0
|
The Recorder API provides functions for audio and video recording.
Required Header
#include <recorder.h>
Overview
The Recorder API provides functions to control the recording of a multimedia content. Simple audio and audio/video are supported. Recording operations operate as a state machine, described below.
In addition, the API contains functions to configure the recording process, or find details about it, such as getting/setting the filename for the recording, the file format and the video codec. Some of these interfaces are listed in the Recorder Attributes API.
Additional functions allow registering notifications via callback functions for various state change events.
State Diagram
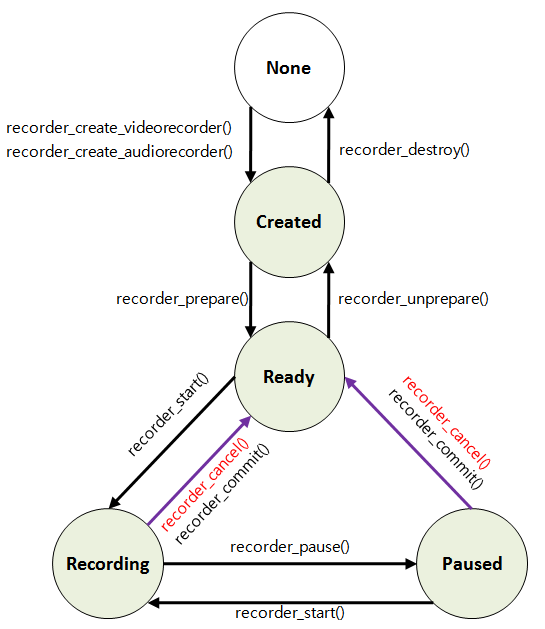
State Transitions
FUNCTION | PRE-STATE | POST-STATE | SYNC TYPE |
---|---|---|---|
recorder_create_videorecorder() recorder_create_audiorecorder() | NONE | CREATED | SYNC |
recorder_destroy() | CREATED | NONE | SYNC |
recorder_prepare() | CREATED | READY | SYNC |
recorder_unprepare() | READY | CREATED | SYNC |
recorder_start() | READY / PAUSED | RECORDING | SYNC |
recorder_pause() | RECORDING | PAUSED | SYNC |
recorder_cancel() recorder_commit() | RECORDING / PAUSED | READY | SYNC |
Callback(Event) Operations
The callback mechanism is used to notify the application about significant recorder events.
REGISTER | UNREGISTER | CALLBACK | DESCRIPTION |
---|---|---|---|
recorder_set_recording_limit_reached_cb() | recorder_unset_recording_limit_reached_cb() | recorder_recording_limit_reached_cb() | This callback is called when recording limitation error has occurred during recording. |
recorder_set_recording_status_cb() | recorder_unset_recording_status_cb() | recorder_recording_status_cb() | This callback is used to notify the recording status. |
recorder_set_state_changed_cb() | recorder_unset_state_changed_cb() | recorder_state_changed_cb() | This callback is used to notify the change of recorder's state. |
Foreach Operations
FOREACH | CALLBACK | DESCRIPTION |
---|---|---|
recorder_foreach_supported_file_format() | recorder_supported_file_format_cb() | Supported file format |
recorder_foreach_supported_audio_encoder() | recorder_supported_audio_encoder_cb() | Supported audio encoder |
recorder_foreach_supported_video_encoder() | recorder_supported_video_encoder_cb() | Supported video encoder |
Related Features
This API is related with the following features:
- http://tizen.org/feature/media.audio_recording
- http://tizen.org/feature/media.video_recording
It is recommended to design feature related codes in your application for reliability.
You can check if a device supports the related features for this API by using System Information, thereby controlling the procedure of your application.
To ensure your application is only running on the device with specific features, please define the features in your manifest file using the manifest editor in the SDK.
More details on featuring your application can be found from Feature Element.
Functions | |
int | recorder_create_videorecorder (camera_h camera, recorder_h *recorder) |
Creates a recorder handle to record a video. | |
int | recorder_create_audiorecorder (recorder_h *recorder) |
Creates a recorder handle to record an audio. | |
int | recorder_destroy (recorder_h recorder) |
Destroys the recorder handle. | |
int | recorder_prepare (recorder_h recorder) |
Prepares the media recorder for recording. | |
int | recorder_unprepare (recorder_h recorder) |
Resets the media recorder. | |
int | recorder_start (recorder_h recorder) |
Starts the recording. | |
int | recorder_pause (recorder_h recorder) |
Pauses the recording. | |
int | recorder_commit (recorder_h recorder) |
Stops recording and saves the result. | |
int | recorder_cancel (recorder_h recorder) |
Cancels the recording. | |
int | recorder_get_state (recorder_h recorder, recorder_state_e *state) |
Gets the recorder's current state. | |
int | recorder_get_audio_level (recorder_h recorder, double *dB) |
Gets the peak audio input level that was sampled since the last call to this function. | |
int | recorder_set_filename (recorder_h recorder, const char *path) |
Sets the file path to record. | |
int | recorder_get_filename (recorder_h recorder, char **path) |
Gets the file path to record. | |
int | recorder_set_file_format (recorder_h recorder, recorder_file_format_e format) |
Sets the file format for recording media stream. | |
int | recorder_get_file_format (recorder_h recorder, recorder_file_format_e *format) |
Gets the file format for recording media stream. | |
int | recorder_set_sound_stream_info (recorder_h recorder, sound_stream_info_h stream_info) |
Sets the recorder's sound manager stream information. | |
int | recorder_set_audio_encoder (recorder_h recorder, recorder_audio_codec_e codec) |
Sets the audio codec for encoding an audio stream. | |
int | recorder_get_audio_encoder (recorder_h recorder, recorder_audio_codec_e *codec) |
Gets the audio codec for encoding an audio stream. | |
int | recorder_set_video_resolution (recorder_h recorder, int width, int height) |
Sets the resolution of the video recording. | |
int | recorder_get_video_resolution (recorder_h recorder, int *width, int *height) |
Gets the resolution of the video recording. | |
int | recorder_set_video_encoder (recorder_h recorder, recorder_video_codec_e codec) |
Sets the video codec for encoding video stream. | |
int | recorder_get_video_encoder (recorder_h recorder, recorder_video_codec_e *codec) |
Gets the video codec for encoding video stream. | |
int | recorder_set_state_changed_cb (recorder_h recorder, recorder_state_changed_cb callback, void *user_data) |
Registers the callback function that will be invoked when the recorder state changes. | |
int | recorder_unset_state_changed_cb (recorder_h recorder) |
Unregisters the callback function. | |
int | recorder_set_interrupted_cb (recorder_h recorder, recorder_interrupted_cb callback, void *user_data) |
Registers a callback function to be called when the media recorder is interrupted according to a policy. | |
int | recorder_unset_interrupted_cb (recorder_h recorder) |
Unregisters the callback function. | |
int | recorder_set_interrupt_started_cb (recorder_h recorder, recorder_interrupt_started_cb callback, void *user_data) |
Registers a callback function to be called when the media recorder interrupt is started according to a policy. | |
int | recorder_unset_interrupt_started_cb (recorder_h recorder) |
Unregisters the callback function. | |
int | recorder_set_audio_stream_cb (recorder_h recorder, recorder_audio_stream_cb callback, void *user_data) |
Registers a callback function to be called when audio stream data is being delivered. | |
int | recorder_unset_audio_stream_cb (recorder_h recorder) |
Unregisters the callback function. | |
int | recorder_set_muxed_stream_cb (recorder_h recorder, recorder_muxed_stream_cb callback, void *user_data) |
Registers a callback function to be called when muxed stream data is delivered. | |
int | recorder_unset_muxed_stream_cb (recorder_h recorder) |
Unregisters the callback function. | |
int | recorder_set_video_encode_decision_cb (recorder_h recorder, recorder_video_encode_decision_cb callback, void *user_data) |
Registers a callback function to be called when each video frame is delivered before encoding. | |
int | recorder_unset_video_encode_decision_cb (recorder_h recorder) |
Unregisters the callback function. | |
int | recorder_set_recording_status_cb (recorder_h recorder, recorder_recording_status_cb callback, void *user_data) |
Registers a callback function to be invoked when the recording information changes. | |
int | recorder_unset_recording_status_cb (recorder_h recorder) |
Unregisters the callback function. | |
int | recorder_set_recording_limit_reached_cb (recorder_h recorder, recorder_recording_limit_reached_cb callback, void *user_data) |
Registers the callback function to be run when reached the recording limit. | |
int | recorder_unset_recording_limit_reached_cb (recorder_h recorder) |
Unregisters the callback function. | |
int | recorder_set_error_cb (recorder_h recorder, recorder_error_cb callback, void *user_data) |
Registers a callback function to be called when an asynchronous operation error occurred. | |
int | recorder_unset_error_cb (recorder_h recorder) |
Unregisters the callback function. | |
int | recorder_get_device_state (recorder_type_e type, recorder_device_state_e *state) |
Gets the state of recorder device. | |
int | recorder_add_device_state_changed_cb (recorder_device_state_changed_cb callback, void *user_data, int *cb_id) |
Registers a callback function to be called when the recorder device state changes. | |
int | recorder_remove_device_state_changed_cb (int cb_id) |
Unregisters a callback function. | |
Typedefs | |
typedef struct recorder_s * | recorder_h |
The Media recorder handle. | |
typedef camera_preview_data_s | recorder_video_data_s |
The structure type to contain video stream data. | |
typedef void(* | recorder_recording_limit_reached_cb )(recorder_recording_limit_type_e type, void *user_data) |
Called when limitation error occurs while recording. | |
typedef void(* | recorder_recording_status_cb )(unsigned long long elapsed_time, unsigned long long file_size, void *user_data) |
Called to indicate the recording status. | |
typedef void(* | recorder_state_changed_cb )(recorder_state_e previous, recorder_state_e current, bool by_policy, void *user_data) |
Called when the record state is changed. | |
typedef void(* | recorder_device_state_changed_cb )(recorder_type_e type, recorder_device_state_e state, void *user_data) |
Called when the recorder device state is changed. | |
typedef void(* | recorder_interrupted_cb )(recorder_policy_e policy, recorder_state_e previous, recorder_state_e current, void *user_data) |
Called when the recorder is interrupted by a policy. | |
typedef void(* | recorder_interrupt_started_cb )(recorder_policy_e policy, recorder_state_e state, void *user_data) |
Called when the recorder interrupt is started by a policy. | |
typedef void(* | recorder_audio_stream_cb )(void *stream, int size, audio_sample_type_e format, int channel, unsigned int timestamp, void *user_data) |
Called when audio stream data was being delivered just before storing in the recorded file. | |
typedef void(* | recorder_muxed_stream_cb )(void *stream, int size, unsigned long long offset, void *user_data) |
Called when muxed stream data is delivered just before writing to the file. | |
typedef bool(* | recorder_video_encode_decision_cb )(recorder_video_data_s *frame, void *user_data) |
Called when each video frame is delivered before encoding, and it will be encoded if the application returns true , otherwise dropped. | |
typedef bool(* | recorder_supported_video_resolution_cb )(int width, int height, void *user_data) |
Called once for each supported video resolution. | |
typedef void(* | recorder_error_cb )(recorder_error_e error, recorder_state_e current_state, void *user_data) |
Called when the error occurred. |
Typedef Documentation
typedef void(* recorder_audio_stream_cb)(void *stream, int size, audio_sample_type_e format, int channel, unsigned int timestamp, void *user_data) |
Called when audio stream data was being delivered just before storing in the recorded file.
- Since :
- 2.3.1
- Remarks:
- The callback function holds the same buffer that will be recorded.
So if the user changes the buffer, the result file will contain the buffer. - The callback is called via internal thread of Frameworks, therefore do not invoke UI API, recorder_unprepare(), recorder_commit() and recorder_cancel() in callback.
- Parameters:
-
[in] stream The audio stream data [in] size The size of the stream data [in] format The audio format [in] channel The number of the channel [in] timestamp The timestamp of the stream buffer (in msec) [in] user_data The user data passed from the callback registration function
- See also:
- recorder_set_audio_stream_cb()
typedef void(* recorder_device_state_changed_cb)(recorder_type_e type, recorder_device_state_e state, void *user_data) |
Called when the recorder device state is changed.
- Since :
- 3.0
- Parameters:
-
[in] type The recorder type [in] state The state of the recorder device [in] user_data The user data passed from the callback registration function
typedef void(* recorder_error_cb)(recorder_error_e error, recorder_state_e current_state, void *user_data) |
Called when the error occurred.
- Since :
- 2.3.1
- Remarks:
- This callback informs about the critical error situation.
When being invoked, user should release the resource and terminate the application.
This error code will be reported. RECORDER_ERROR_DEVICE
RECORDER_ERROR_INVALID_OPERATION
RECORDER_ERROR_OUT_OF_MEMORY.
- Parameters:
-
[in] error The error code [in] current_state The current state of the recorder [in] user_data The user data passed from the callback registration function
- Precondition:
- This callback function is invoked if you register this callback using recorder_set_error_cb().
typedef struct recorder_s* recorder_h |
The Media recorder handle.
- Since :
- 2.3.1
typedef void(* recorder_interrupt_started_cb)(recorder_policy_e policy, recorder_state_e state, void *user_data) |
Called when the recorder interrupt is started by a policy.
- Since :
- 4.0
- Remarks:
- This callback is called before interrupt handling is started.
- Parameters:
-
[in] policy The policy that is interrupting the recorder [in] state The current state of the recorder [in] user_data The user data passed from the callback registration function
- See also:
- recorder_set_interrupt_started_cb()
typedef void(* recorder_interrupted_cb)(recorder_policy_e policy, recorder_state_e previous, recorder_state_e current, void *user_data) |
Called when the recorder is interrupted by a policy.
- Since :
- 2.3.1
- Remarks:
- This callback is called after interrupt handling is completed.
- Parameters:
-
[in] policy The policy that interrupted the recorder [in] previous The previous state of the recorder [in] current The current state of the recorder [in] user_data The user data passed from the callback registration function
- See also:
- recorder_set_interrupted_cb()
typedef void(* recorder_muxed_stream_cb)(void *stream, int size, unsigned long long offset, void *user_data) |
Called when muxed stream data is delivered just before writing to the file.
- Since :
- 4.0
- Remarks:
- This callback receives the data that will be recorded,
but any changes to this data will not affect the recorded file.
The stream should not be freed and it's valid only in the callback. To use outside the callback, make a copy.
- Parameters:
-
[in] stream The muxed stream data [in] size The size of the stream data [in] offset The offset of the stream data [in] user_data The user data passed from the callback registration function
- See also:
- recorder_set_muxed_stream_cb()
typedef void(* recorder_recording_limit_reached_cb)(recorder_recording_limit_type_e type, void *user_data) |
Called when limitation error occurs while recording.
The callback function is possible to receive three types of limits: time, size and no-space.
- Since :
- 2.3.1
- Remarks:
- After being called, recording data is discarded and not written in the recording file. Also the state of recorder is not changed.
- Parameters:
-
[in] type The imitation type [in] user_data The user data passed from the callback registration function
- Precondition:
- You have to register a callback using recorder_set_recording_limit_reached_cb().
typedef void(* recorder_recording_status_cb)(unsigned long long elapsed_time, unsigned long long file_size, void *user_data) |
Called to indicate the recording status.
- Since :
- 2.3.1
- Remarks:
- This callback function is repeatedly invoked during the RECORDER_STATE_RECORDING state.
- Parameters:
-
[in] elapsed_time The time of the recording (milliseconds) [in] file_size The size of the recording file (KB) [in] user_data The user data passed from the callback registration function
- Precondition:
- recorder_start() will invoke this callback if you register it using recorder_set_recording_status_cb().
typedef void(* recorder_state_changed_cb)(recorder_state_e previous, recorder_state_e current, bool by_policy, void *user_data) |
Called when the record state is changed.
- Since :
- 2.3.1
- Parameters:
-
[in] previous The previous state of the recorder [in] current The current state of the recorder [in] by_policy true
if the state is changed by policy, otherwisefalse
if the state is not changed[in] user_data The user data passed from the callback registration function
- Precondition:
- This function is required to register a callback using recorder_set_state_changed_cb().
typedef bool(* recorder_supported_video_resolution_cb)(int width, int height, void *user_data) |
Called once for each supported video resolution.
- Since :
- 2.3.1
- Parameters:
-
[in] width The video image width [in] height The video image height [in] user_data The user data passed from the foreach function
- Returns:
true
to continue with the next iteration of the loop,
otherwisefalse
to break out of the loop
- Precondition:
- recorder_foreach_supported_video_resolution() will invoke this callback.
The structure type to contain video stream data.
- Since :
- 6.0
typedef bool(* recorder_video_encode_decision_cb)(recorder_video_data_s *frame, void *user_data) |
Called when each video frame is delivered before encoding,
and it will be encoded if the application returns true
, otherwise dropped.
- Since :
- 6.0
- Remarks:
- This function is issued in the context of internal framework so the UI update code should not be directly invoked.
- The frame should not be released and it's available until the callback returns.
- Parameters:
-
[in] frame The reference pointer to video stream data [in] user_data The user data passed from the callback registration function
Enumeration Type Documentation
enum recorder_error_e |
Enumeration for error code of the media recorder.
- Since :
- 2.3.1
- Enumerator:
Enumeration for the file container format.
- Since :
- 2.3.1
- Enumerator:
enum recorder_policy_e |
enum recorder_rotation_e |
enum recorder_state_e |
Enumeration for recorder states.
- Since :
- 2.3.1
- Enumerator:
enum recorder_type_e |
Function Documentation
int recorder_add_device_state_changed_cb | ( | recorder_device_state_changed_cb | callback, |
void * | user_data, | ||
int * | cb_id | ||
) |
Registers a callback function to be called when the recorder device state changes.
- Since :
- 3.0
- Parameters:
-
[in] callback The callback function to register [in] user_data The user data to be passed to the callback function [out] cb_id The id of the registered callback
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_OUT_OF_MEMORY Out of memory RECORDER_ERROR_NOT_SUPPORTED The feature is not supported
- Postcondition:
- This function will invoke recorder_device_state_changed_cb() when the recorder device's state changes.
int recorder_cancel | ( | recorder_h | recorder | ) |
Cancels the recording.
The recording data is discarded and not written in the recording file.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- When you want to record audio or video file, you need to add privilege according to rules below additionally.
If you want to save contents to internal storage, you should add mediastorage privilege.
If you want to save contents to external storage, you should add externalstorage privilege.
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state must be RECORDER_STATE_RECORDING set by recorder_start() or RECORDER_STATE_PAUSED by recorder_pause().
- Postcondition:
- The recorder state will be RECORDER_STATE_READY.
int recorder_commit | ( | recorder_h | recorder | ) |
Stops recording and saves the result.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- When you want to record audio or video file, you need to add privilege according to rules below additionally.
If you want to save contents to internal storage, you should add mediastorage privilege.
If you want to save contents to external storage, you should add externalstorage privilege.
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state must be RECORDER_STATE_RECORDING set by recorder_start() or RECORDER_STATE_PAUSED by recorder_pause().
- Postcondition:
- The recorder state will be RECORDER_STATE_READY.
int recorder_create_audiorecorder | ( | recorder_h * | recorder | ) |
Creates a recorder handle to record an audio.
- Since :
- 2.3.1
- Remarks:
- You must release recorder using recorder_destroy().
-
The privilege http://tizen.org/privilege/recorder is not required since 4.0,
but it is required in all earlier versions. -
Since 4.0, It's related to the following feature: http://tizen.org/feature/media.audio_recording
instead of using http://tizen.org/feature/microphone
- Parameters:
-
[out] recorder A handle to the recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_OUT_OF_MEMORY Out of memory RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported
- Postcondition:
- The recorder state will be RECORDER_STATE_CREATED.
- See also:
- recorder_destroy()
int recorder_create_videorecorder | ( | camera_h | camera, |
recorder_h * | recorder | ||
) |
Creates a recorder handle to record a video.
- Since :
- 2.3.1
- Remarks:
- You must release recorder using recorder_destroy().
The camera handle also could be used for capturing images.
If the camera state was CAMERA_STATE_CREATED, the preview format will be changed to the recommended preview format for recording. -
The created recorder state will be different according to camera state :
CAMERA_STATE_CREATED -> RECORDER_STATE_CREATED
CAMERA_STATE_PREVIEW -> RECORDER_STATE_READY
CAMERA_STATE_CAPTURED -> RECORDER_STATE_READY -
The privilege http://tizen.org/privilege/recorder is not required since 4.0,
but it is required in all earlier versions. -
Since 4.0, It's related to the following feature: http://tizen.org/feature/media.video_recording
instead of using http://tizen.org/feature/microphone
- Parameters:
-
[in] camera The handle to the camera [out] recorder A handle to the recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_OUT_OF_MEMORY Out of memory RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported
int recorder_destroy | ( | recorder_h | recorder | ) |
Destroys the recorder handle.
- Since :
- 2.3.1
- Remarks:
- The video recorder's camera handle is not released by this function.
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported
- Precondition:
- The recorder state should be RECORDER_STATE_CREATED.
- Postcondition:
- The recorder state will be RECORDER_STATE_NONE.
int recorder_get_audio_encoder | ( | recorder_h | recorder, |
recorder_audio_codec_e * | codec | ||
) |
Gets the audio codec for encoding an audio stream.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder [out] codec The audio codec
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int recorder_get_audio_level | ( | recorder_h | recorder, |
double * | dB | ||
) |
Gets the peak audio input level that was sampled since the last call to this function.
- Since :
- 2.3.1
- Remarks:
0
dB indicates maximum input level,-300
dB indicates minimum input level.
- Parameters:
-
[in] recorder The handle to the media recorder [out] dB The audio input level in dB
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state must be RECORDER_STATE_RECORDING or RECORDER_STATE_PAUSED.
int recorder_get_device_state | ( | recorder_type_e | type, |
recorder_device_state_e * | state | ||
) |
Gets the state of recorder device.
- Since :
- 3.0
- Parameters:
-
[in] type The recorder type [out] state The current state of the device
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_NOT_SUPPORTED The feature is not supported
int recorder_get_file_format | ( | recorder_h | recorder, |
recorder_file_format_e * | format | ||
) |
Gets the file format for recording media stream.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder [out] format The media file format
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int recorder_get_filename | ( | recorder_h | recorder, |
char ** | path | ||
) |
Gets the file path to record.
- Since :
- 2.3.1
- Remarks:
- You must release path using free().
- Parameters:
-
[in] recorder The handle to the media recorder [out] path The recording file path
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- See also:
- recorder_set_filename()
int recorder_get_state | ( | recorder_h | recorder, |
recorder_state_e * | state | ||
) |
Gets the recorder's current state.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder [out] state The current state of the recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int recorder_get_video_encoder | ( | recorder_h | recorder, |
recorder_video_codec_e * | codec | ||
) |
Gets the video codec for encoding video stream.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder [out] codec The video codec
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int recorder_get_video_resolution | ( | recorder_h | recorder, |
int * | width, | ||
int * | height | ||
) |
Gets the resolution of the video recording.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder [out] width The video width [out] height The video height
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int recorder_pause | ( | recorder_h | recorder | ) |
Pauses the recording.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- Recording can be resumed with recorder_start().
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state must be RECORDER_STATE_RECORDING.
- Postcondition:
- The recorder state will be RECORDER_STATE_PAUSED.
int recorder_prepare | ( | recorder_h | recorder | ) |
Prepares the media recorder for recording.
- Since :
- 2.3.1
- Remarks:
- Before calling the function, it is required to properly set audio encoder (recorder_set_audio_encoder()), video encoder(recorder_set_video_encoder()) and file format (recorder_set_file_format()).
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_RESOURCE_CONFLICT Resource conflict error RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state should be RECORDER_STATE_CREATED by recorder_create_videorecorder(), recorder_create_audiorecorder() or recorder_unprepare().
- Postcondition:
- The recorder state will be RECORDER_STATE_READY.
- If recorder handle is created by recorder_create_videorecorder(), the camera state will be changed to CAMERA_STATE_PREVIEW.
int recorder_remove_device_state_changed_cb | ( | int | cb_id | ) |
Unregisters a callback function.
- Since :
- 3.0
- Parameters:
-
[in] cb_id The id of the registered callback
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_NOT_SUPPORTED The feature is not supported
int recorder_set_audio_encoder | ( | recorder_h | recorder, |
recorder_audio_codec_e | codec | ||
) |
Sets the audio codec for encoding an audio stream.
- Since :
- 2.3.1
- Remarks:
- You can get available audio encoders by using recorder_foreach_supported_audio_encoder().
If set to RECORDER_AUDIO_CODEC_DISABLE, the audio track is not created in recording files.
Since 2.3.1, it could be returned RECORDER_ERROR_INVALID_OPERATION
when it's audio recorder and its state is RECORDER_STATE_READY
because of checking codec compatibility with current file format.
- Parameters:
-
[in] recorder The handle to the media recorder [in] codec The audio codec
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_INVALID_OPERATION Invalid operation (Since 2.3.1) RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state must be RECORDER_STATE_CREATED or RECORDER_STATE_READY.
int recorder_set_audio_stream_cb | ( | recorder_h | recorder, |
recorder_audio_stream_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when audio stream data is being delivered.
- Since :
- 2.3.1
- Remarks:
- This callback function holds the same buffer that will be recorded.
Therefore if an user changes the buffer, the result file will have the buffer.
-
The callback is called via internal thread of Frameworks. Therefore do not invoke UI API, recorder_unprepare(), recorder_commit() and recorder_cancel() in callback.
This callback function to be called in RECORDER_STATE_RECORDING and RECORDER_STATE_PAUSED state.
- Parameters:
-
[in] recorder The handle to the recorder [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state should be RECORDER_STATE_READY or RECORDER_STATE_CREATED.
int recorder_set_error_cb | ( | recorder_h | recorder, |
recorder_error_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when an asynchronous operation error occurred.
- Since :
- 2.3.1
- Remarks:
- This callback informs critical error situation.
When this callback is invoked, user should release the resource and terminate the application.
These error codes will occur.
RECORDER_ERROR_DEVICE
RECORDER_ERROR_INVALID_OPERATION
RECORDER_ERROR_OUT_OF_MEMORY
- Parameters:
-
[in] recorder The handle to the recorder [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Postcondition:
- This function will invoke recorder_error_cb() when an asynchronous operation error occur.
int recorder_set_file_format | ( | recorder_h | recorder, |
recorder_file_format_e | format | ||
) |
Sets the file format for recording media stream.
- Since :
- 2.3.1
- Remarks:
- Since 2.3.1, it could be returned RECORDER_ERROR_INVALID_OPERATION
when it's audio recorder and its state is RECORDER_STATE_READY
because of checking codec compatibility with current encoder.
- Parameters:
-
[in] recorder The handle to the media recorder [in] format The media file format
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_INVALID_OPERATION Invalid operation (Since 2.3.1) RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state must be RECORDER_STATE_CREATED or RECORDER_STATE_READY (for video recorder only).
Since 2.3.1, this API also works for audio recorder when its state is RECORDER_STATE_READY.
int recorder_set_filename | ( | recorder_h | recorder, |
const char * | path | ||
) |
Sets the file path to record.
This function sets file path which defines where newly recorded data should be stored.
- Since :
- 2.3.1
- Remarks:
- If the same file already exists in the file system, then old file will be overwritten.
- Parameters:
-
[in] recorder The handle to the media recorder [in] path The recording file path
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state must be RECORDER_STATE_CREATED or RECORDER_STATE_READY.
- See also:
- recorder_get_filename()
int recorder_set_interrupt_started_cb | ( | recorder_h | recorder, |
recorder_interrupt_started_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when the media recorder interrupt is started according to a policy.
- Since :
- 4.0
- Parameters:
-
[in] recorder The handle to the media recorder [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter
int recorder_set_interrupted_cb | ( | recorder_h | recorder, |
recorder_interrupted_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when the media recorder is interrupted according to a policy.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int recorder_set_muxed_stream_cb | ( | recorder_h | recorder, |
recorder_muxed_stream_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when muxed stream data is delivered.
- Since :
- 4.0
- Remarks:
- This callback receives the data that will be recorded,
but any changes to this data will not affect the recorded file.
- Parameters:
-
[in] recorder The handle to the recorder [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_INVALID_STATE Invalid state
- Precondition:
- The recorder state should be RECORDER_STATE_READY or RECORDER_STATE_CREATED.
int recorder_set_recording_limit_reached_cb | ( | recorder_h | recorder, |
recorder_recording_limit_reached_cb | callback, | ||
void * | user_data | ||
) |
Registers the callback function to be run when reached the recording limit.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to media recorder [in] callback The function pointer of user callback [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Postcondition:
- recorder_recording_limit_reached_cb() will be invoked.
int recorder_set_recording_status_cb | ( | recorder_h | recorder, |
recorder_recording_status_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be invoked when the recording information changes.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder [in] callback The function pointer of user callback [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Postcondition:
- recorder_recording_status_cb() will be invoked.
int recorder_set_sound_stream_info | ( | recorder_h | recorder, |
sound_stream_info_h | stream_info | ||
) |
Sets the recorder's sound manager stream information.
- Since :
- 3.0
- Remarks:
- You can set sound stream information including audio routing. For more details, please refer to Sound Manager
- Parameters:
-
[in] recorder The handle to the media recorder [in] stream_info The sound manager info
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_INVALID_OPERATION Invalid operation
- Precondition:
- The recorder state must be RECORDER_STATE_CREATED or RECORDER_STATE_READY
int recorder_set_state_changed_cb | ( | recorder_h | recorder, |
recorder_state_changed_cb | callback, | ||
void * | user_data | ||
) |
Registers the callback function that will be invoked when the recorder state changes.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder [in] callback The function pointer of user callback [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Postcondition:
- recorder_state_changed_cb() will be invoked.
int recorder_set_video_encode_decision_cb | ( | recorder_h | recorder, |
recorder_video_encode_decision_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when each video frame is delivered before encoding.
- Since :
- 6.0
- Remarks:
- The audio stream will be disabled by force if callback is set.
- Parameters:
-
[in] recorder The handle to the recorder [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_NOT_SUPPORTED The feature is not supported
- Precondition:
- The recorder state should be RECORDER_STATE_READY or RECORDER_STATE_CREATED.
- Postcondition:
- The callback will be invoked when each video frame is delivered before encoding,
and it will be encoded if the callback returnstrue
, otherwise dropped.
int recorder_set_video_encoder | ( | recorder_h | recorder, |
recorder_video_codec_e | codec | ||
) |
Sets the video codec for encoding video stream.
- Since :
- 2.3.1
- Remarks:
- You can get available video encoders by using recorder_foreach_supported_video_encoder().
- Parameters:
-
[in] recorder The handle to the media recorder [in] codec The video codec
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state must be RECORDER_STATE_CREATED or RECORDER_STATE_READY.
int recorder_set_video_resolution | ( | recorder_h | recorder, |
int | width, | ||
int | height | ||
) |
Sets the resolution of the video recording.
- Since :
- 2.3.1
- Remarks:
- This function should be called before recording (recorder_start()).
- Parameters:
-
[in] recorder The handle to the media recorder [in] width The video width [in] height The video height
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state must be RECORDER_STATE_CREATED or RECORDER_STATE_READY.
int recorder_start | ( | recorder_h | recorder | ) |
Starts the recording.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/recorder
- Remarks:
- If file path has been set to an existing file, this file is removed automatically and updated by new one.
In the video recorder, some preview format does not support record mode. It will return RECORDER_ERROR_INVALID_OPERATION error.
You should use default preview format or CAMERA_PIXEL_FORMAT_NV12 in the record mode.
When you want to record audio or video file, you need to add privilege according to rules below additionally.
If you want to save contents to internal storage, you should add mediastorage privilege.
If you want to save contents to external storage, you should add externalstorage privilege.
The filename should be set before this function is invoked.
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state must be RECORDER_STATE_READY by recorder_prepare() or RECORDER_STATE_PAUSED by recorder_pause().
The filename should be set by recorder_set_filename().
- Postcondition:
- The recorder state will be RECORDER_STATE_RECORDING.
int recorder_unprepare | ( | recorder_h | recorder | ) |
Resets the media recorder.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The recorder state should be RECORDER_STATE_READY set by recorder_prepare(), recorder_cancel() or recorder_commit().
- Postcondition:
- The recorder state will be RECORDER_STATE_CREATED.
- If the recorder handle is created by recorder_create_videorecorder(), camera state will be changed to CAMERA_STATE_CREATED.
int recorder_unset_audio_stream_cb | ( | recorder_h | recorder | ) |
Unregisters the callback function.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- See also:
- recorder_set_audio_stream_cb()
int recorder_unset_error_cb | ( | recorder_h | recorder | ) |
Unregisters the callback function.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the recorder
- Returns:
on
success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- See also:
- recorder_set_error_cb()
int recorder_unset_interrupt_started_cb | ( | recorder_h | recorder | ) |
Unregisters the callback function.
- Since :
- 4.0
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter
- See also:
- recorder_set_interrupt_started_cb()
int recorder_unset_interrupted_cb | ( | recorder_h | recorder | ) |
Unregisters the callback function.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- See also:
- recorder_set_interrupted_cb()
int recorder_unset_muxed_stream_cb | ( | recorder_h | recorder | ) |
Unregisters the callback function.
- Since :
- 4.0
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_INVALID_STATE Invalid state
- Precondition:
- The recorder state should be RECORDER_STATE_READY or RECORDER_STATE_CREATED.
- See also:
- recorder_set_muxed_stream_cb()
int recorder_unset_recording_limit_reached_cb | ( | recorder_h | recorder | ) |
Unregisters the callback function.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int recorder_unset_recording_status_cb | ( | recorder_h | recorder | ) |
Unregisters the callback function.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- See also:
- recorder_set_recording_status_cb()
int recorder_unset_state_changed_cb | ( | recorder_h | recorder | ) |
Unregisters the callback function.
- Since :
- 2.3.1
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_PERMISSION_DENIED The access to the resources can not be granted RECORDER_ERROR_NOT_SUPPORTED The feature is not supported RECORDER_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- See also:
- recorder_set_state_changed_cb()
int recorder_unset_video_encode_decision_cb | ( | recorder_h | recorder | ) |
Unregisters the callback function.
- Since :
- 6.0
- Parameters:
-
[in] recorder The handle to the media recorder
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RECORDER_ERROR_NONE Successful RECORDER_ERROR_INVALID_PARAMETER Invalid parameter RECORDER_ERROR_INVALID_OPERATION Invalid operation RECORDER_ERROR_INVALID_STATE Invalid state RECORDER_ERROR_NOT_SUPPORTED The feature is not supported
- Precondition:
- The recorder state should be RECORDER_STATE_READY or RECORDER_STATE_CREATED.