Tizen Native API
7.0
|
These functions provide double linked list management.
Eina_List is a doubly linked list. It can store data of any type in the form of void pointers. It has convenience functions to do all the common operations which means it should rarely if ever be necessary to directly access the struct's fields. Nevertheless it can be useful to understand the inner workings of the data structure being used.
Eina_List nodes keep references to the previous node, the next node, its data and to an accounting structure.
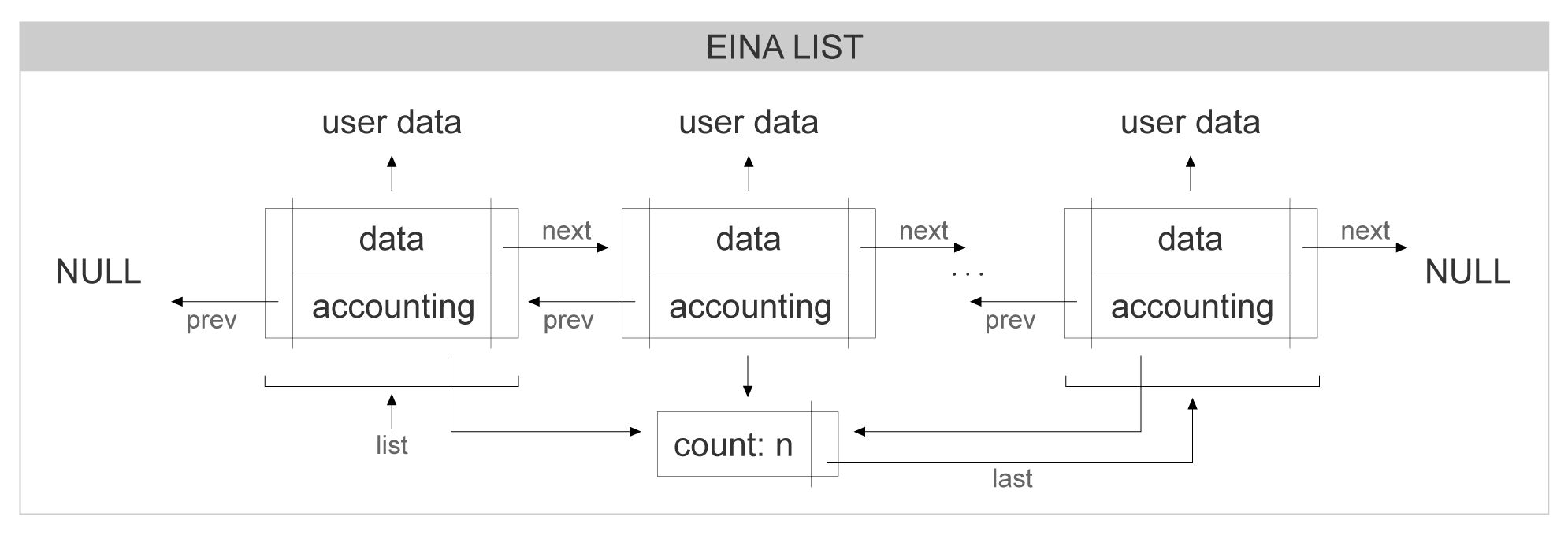
Eina_List_Accounting is used to improve the performance of some functions. It is private and should not be modified. It contains a reference to the end of the list and the number of elements in the list.
- Note:
- Every function that modifies the contents of the list returns a pointer to the head of the list and it is essential that this be pointer be used in any future references to the list.
Most functions have two versions that have the same effect but operate on different arguments, the plain functions operate over data(e.g..: eina_list_append_relative, eina_list_remove, eina_list_data_find), the list versions of these functions operate on Eina_List nodes.
- Warning:
- You must always use the pointer to the first element of the list as the list!
- You must never use a pointer to an element in the middle of the list as the list!
Here are some examples of Eina_List usage:
- Adding elements to Eina_List
- Sorting Eina_List elements
- Reordering Eina_List elements
- Eina_List and memory allocation
Functions | |
Eina_List * | eina_list_append (Eina_List *list, const void *data) |
Appends the given data to the given linked list. | |
Eina_List * | eina_list_prepend (Eina_List *list, const void *data) |
Prepends the given data to the given linked list. | |
Eina_List * | eina_list_append_relative (Eina_List *list, const void *data, const void *relative) |
Inserts the given data into the given linked list after the specified data. | |
Eina_List * | eina_list_append_relative_list (Eina_List *list, const void *data, Eina_List *relative) |
Appends a list node to a linked list after the specified member. | |
Eina_List * | eina_list_prepend_relative (Eina_List *list, const void *data, const void *relative) |
Prepends a data pointer to a linked list before the specified member. | |
Eina_List * | eina_list_prepend_relative_list (Eina_List *list, const void *data, Eina_List *relative) |
Prepends a list node to a linked list before the specified member. | |
Eina_List * | eina_list_sorted_insert (Eina_List *list, Eina_Compare_Cb func, const void *data) |
Inserts a new node into a sorted list. | |
Eina_List * | eina_list_remove (Eina_List *list, const void *data) |
Removes the first instance of the specified data from the given list. | |
Eina_List * | eina_list_remove_list (Eina_List *list, Eina_List *remove_list) |
Removes the specified list node. | |
Eina_List * | eina_list_promote_list (Eina_List *list, Eina_List *move_list) |
Moves the specified data to the head of the list. | |
Eina_List * | eina_list_demote_list (Eina_List *list, Eina_List *move_list) |
Moves the specified data to the tail of the list. | |
void * | eina_list_data_find (const Eina_List *list, const void *data) |
Finds a member of a list and returns it. | |
Eina_List * | eina_list_data_find_list (const Eina_List *list, const void *data) |
Finds a member of a list and returns it as a list node. | |
Eina_Bool | eina_list_move (Eina_List **to, Eina_List **from, void *data) |
Moves a data pointer from one list to another. | |
Eina_Bool | eina_list_move_list (Eina_List **to, Eina_List **from, Eina_List *data) |
Moves a list node from one list to another. | |
Eina_List * | eina_list_free (Eina_List *list) |
Frees an entire list and all the nodes, ignoring the data contained. | |
void * | eina_list_nth (const Eina_List *list, unsigned int n) |
Gets the nth member's data pointer in a list, or NULL on error. | |
Eina_List * | eina_list_nth_list (const Eina_List *list, unsigned int n) |
Gets the nth member's list node in a list. | |
Eina_List * | eina_list_reverse (Eina_List *list) |
Reverses all the elements in the list. | |
Eina_List * | eina_list_reverse_clone (const Eina_List *list) |
Clones (copies) all the elements in the list in reverse order. | |
Eina_List * | eina_list_clone (const Eina_List *list) |
Clones (copies) all the elements in the list in exactly same order. | |
Eina_List * | eina_list_sort (Eina_List *list, unsigned int limit, Eina_Compare_Cb func) |
Sorts a list according to the ordering func will return. | |
Eina_List * | eina_list_shuffle (Eina_List *list, Eina_Random_Cb func) |
Shuffles list. | |
Eina_List * | eina_list_merge (Eina_List *left, Eina_List *right) |
Merges two list. | |
Eina_List * | eina_list_sorted_merge (Eina_List *left, Eina_List *right, Eina_Compare_Cb func) |
Merges two sorted list according to the ordering func will return. | |
Eina_List * | eina_list_split_list (Eina_List *list, Eina_List *relative, Eina_List **right) |
Splits a list into 2 lists. | |
Eina_List * | eina_list_search_sorted_near_list (const Eina_List *list, Eina_Compare_Cb func, const void *data, int *result_cmp) |
Returns node nearest to data from the sorted list. | |
Eina_List * | eina_list_search_sorted_list (const Eina_List *list, Eina_Compare_Cb func, const void *data) |
Returns node if data is in the sorted list. | |
void * | eina_list_search_sorted (const Eina_List *list, Eina_Compare_Cb func, const void *data) |
Returns node data if it is in the sorted list. | |
Eina_List * | eina_list_search_unsorted_list (const Eina_List *list, Eina_Compare_Cb func, const void *data) |
Returns node if data is in the unsorted list. | |
void * | eina_list_search_unsorted (const Eina_List *list, Eina_Compare_Cb func, const void *data) |
Returns node data if it is in the unsorted list. | |
static Eina_List * | eina_list_last (const Eina_List *list) |
Gets the last list node in the list. | |
static Eina_List * | eina_list_next (const Eina_List *list) |
Gets the next list node after the specified list node. | |
static Eina_List * | eina_list_prev (const Eina_List *list) |
Gets the list node prior to the specified list node. | |
static void * | eina_list_data_get (const Eina_List *list) |
Gets the list node data member. | |
static void * | eina_list_data_set (Eina_List *list, const void *data) |
Sets the list node data member. | |
static unsigned int | eina_list_count (const Eina_List *list) |
Gets the count of the number of items in a list. | |
static void * | eina_list_last_data_get (const Eina_List *list) |
Returns the last list node's data. | |
Eina_Iterator * | eina_list_iterator_new (const Eina_List *list) |
Returns a new iterator associated with a list. | |
Eina_Iterator * | eina_list_iterator_reversed_new (const Eina_List *list) |
Returns a new reversed iterator associated with a list. | |
Eina_Accessor * | eina_list_accessor_new (const Eina_List *list) |
Returns a new accessor associated with a list. | |
int | eina_list_data_idx (const Eina_List *list, void *data) |
Finds the member of the list and returns the index. | |
Typedefs | |
typedef struct _Eina_List | Eina_List |
typedef struct _Eina_List_Accounting | Eina_List_Accounting |
Defines | |
#define | EINA_LIST_FOREACH(list, l, data) |
Definition for the macro to iterate over a list. | |
#define | EINA_LIST_REVERSE_FOREACH(list, l, _data) |
Definition for the macro to iterate over a list in the reverse order. | |
#define | EINA_LIST_FOREACH_SAFE(list, l, l_next, data) |
Definition for the macro to iterate over a list with support for node deletion. | |
#define | EINA_LIST_REVERSE_FOREACH_SAFE(list, l, l_prev, data) |
Definition for the macro to iterate over a list in the reverse order with support for deletion. | |
#define | EINA_LIST_FREE(list, data) |
Definition for the macro to remove each list node while having access to each node's data. |
Define Documentation
#define EINA_LIST_FOREACH | ( | list, | |
l, | |||
data | |||
) |
for (l = list, \ data = eina_list_data_get(l); \ l; \ l = eina_list_next(l), \ data = eina_list_data_get(l))
Definition for the macro to iterate over a list.
- Parameters:
-
[in] list The list to iterate over. [out] l A list that is used as an iterator and points to the current node. [out] _data Current item's data.
This macro iterates over list
from the first element to the last. data
is the data related to the current element. l
is an Eina_List used as the list iterator.
The following diagram illustrates this macro iterating over a list of four elements("one", "two", "three" and "four"):
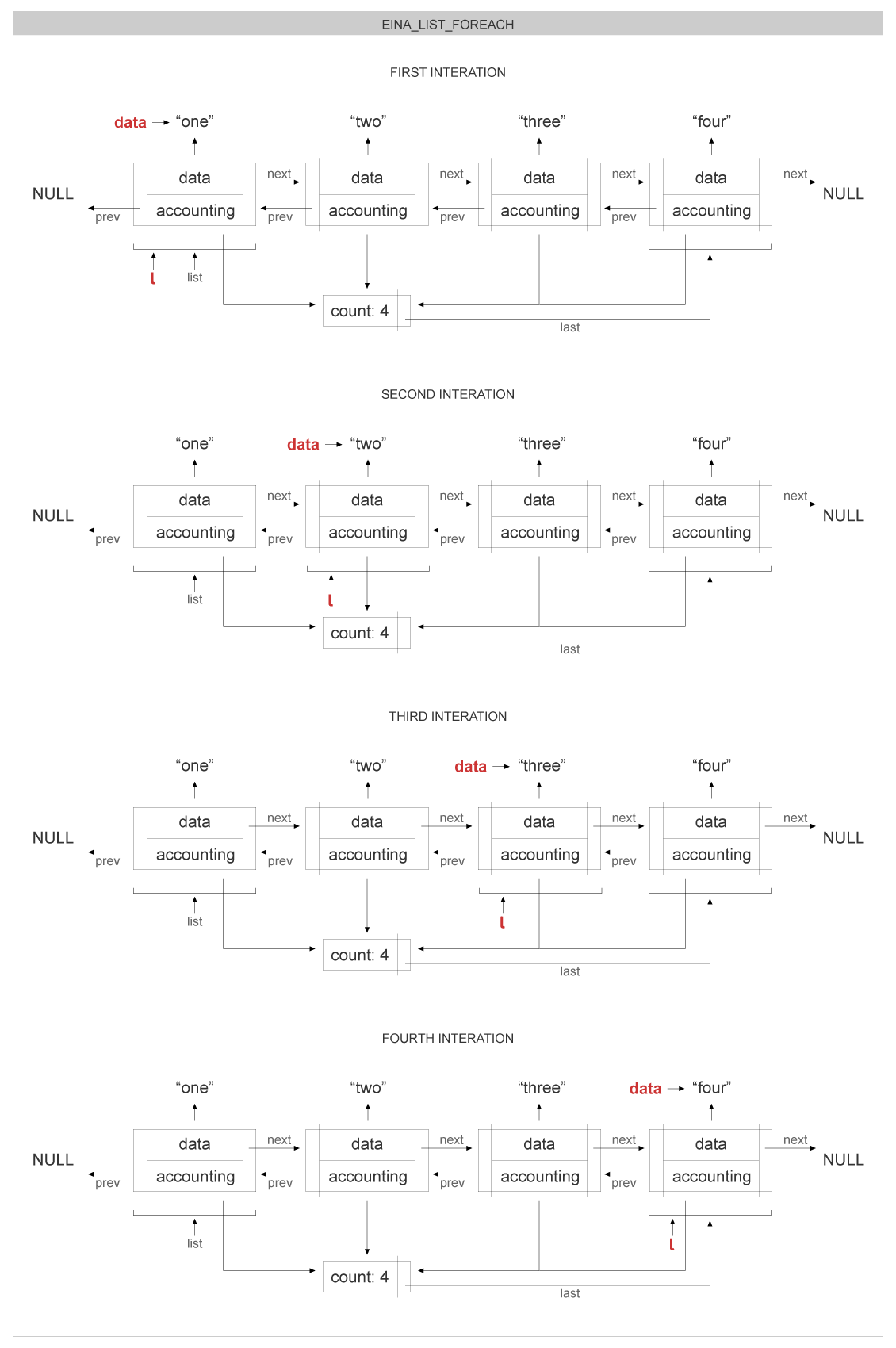
It can be used to free list data, as in the following example:
Eina_List *list; Eina_List *l; char *data; // list is already filled, // its elements are just duplicated strings, // EINA_LIST_FOREACH will be used to free those strings EINA_LIST_FOREACH(list, l, data) free(data); eina_list_free(list);
- Note:
- This is not the optimal way to release memory allocated to a list, since it iterates over the list twice. For an optimized algorithm, use EINA_LIST_FREE().
- Warning:
list
must be a pointer to the first element of the list.- Be careful when deleting list nodes. If you remove the current node and continue iterating, the code will fail because the macro will not be able to get the next node. Notice that it's OK to remove any node if you stop the loop after that. For destructive operations such as this, consider using EINA_LIST_FOREACH_SAFE().
- Examples:
- diskselector_example_02.c, ecore_con_server_simple_example.c, ecore_con_url_cookies_example.c, ecore_con_url_headers_example.c, ecore_evas_basics_example.c, ecore_imf_example.c, edje-box2.c, eina_inlist_02.c, eina_list_01.c, entry_example.c, evas-box.c, evas-buffer-simple.c, evas-events.c, evas-init-shutdown.c, gengrid_example.c, genlist_example_02.c, genlist_example_05.c, hoversel_example_01.c, list_example_03.c, slideshow_example.c, and transit_example_04.c.
#define EINA_LIST_FOREACH_SAFE | ( | list, | |
l, | |||
l_next, | |||
data | |||
) |
for (l = list, \ l_next = eina_list_next(l), \ EINA_PREFETCH(l_next), \ data = eina_list_data_get(l); \ EINA_PREFETCH(data), \ l; \ l = l_next, \ l_next = eina_list_next(l), \ EINA_PREFETCH(l_next), \ data = eina_list_data_get(l), \ EINA_PREFETCH(data))
Definition for the macro to iterate over a list with support for node deletion.
- Parameters:
-
[in] list The list to iterate over. [out] l A list that is used as an iterator and points to the current node. [out] l_next A list that is used as an iterator and points to the next node. [out] data Current item's data.
This macro iterates over list
from the first element to the last. data
is the data related to the current element. l
is an Eina_List used as the list iterator.
Since this macro stores a pointer to the next list node in l_next
, deleting the current node and continuing looping is safe.
The following diagram illustrates this macro iterating over a list of four elements ("one", "two", "three" and "four"):
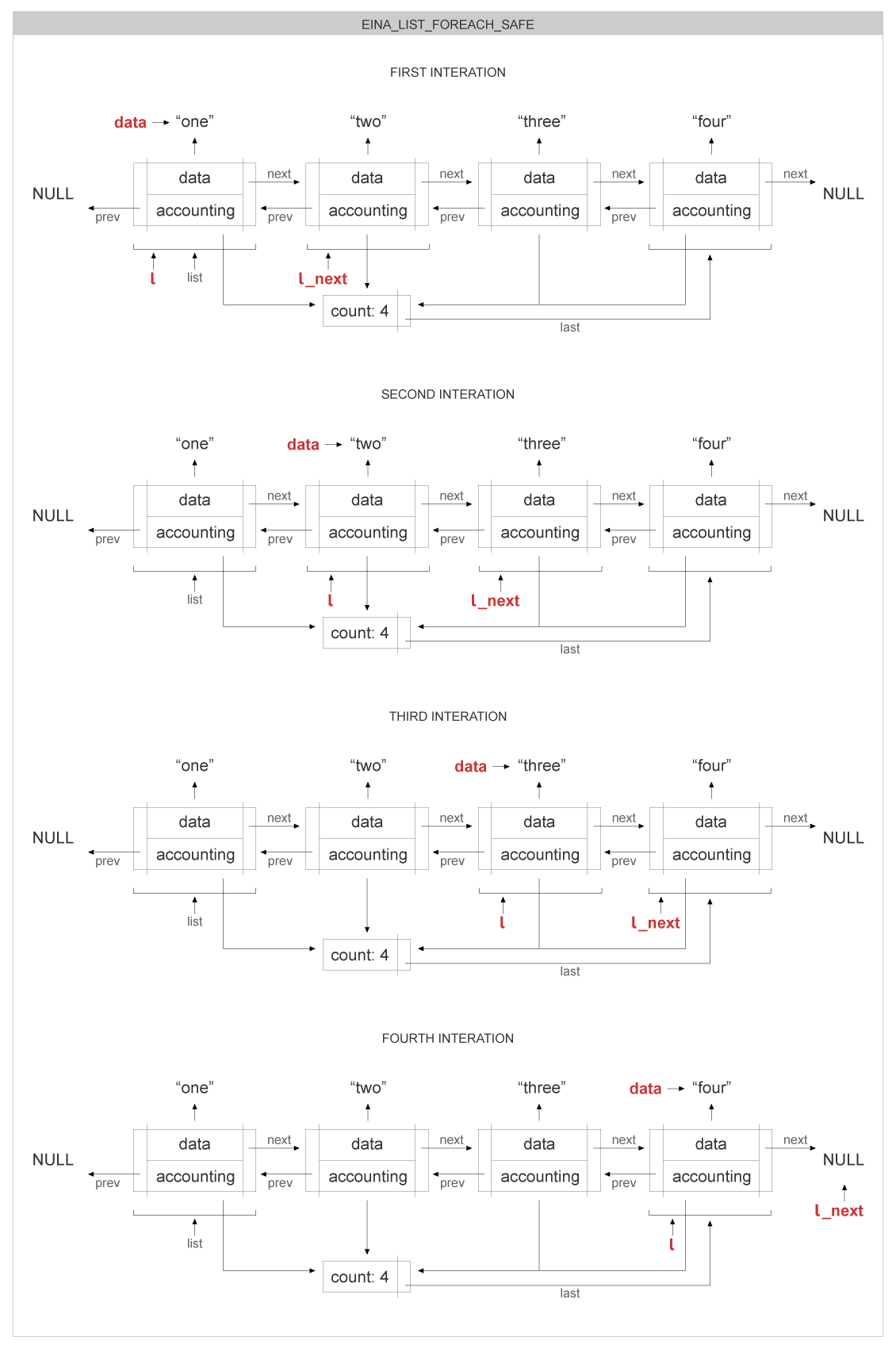
This macro can be used to free list nodes, as in the following example:
Eina_List *list; Eina_List *l; Eina_List *l_next; char *data; // list is already filled, // its elements are just duplicated strings, // EINA_LIST_FOREACH_SAFE will be used to free elements that match "key". EINA_LIST_FOREACH_SAFE(list, l, l_next, data) if (strcmp(data, "key") == 0) { free(data); list = eina_list_remove_list(list, l); }
- Warning:
list
must be a pointer to the first element of the list.
- Examples:
- eina_inlist_02.c.
#define EINA_LIST_FREE | ( | list, | |
data | |||
) |
for (data = eina_list_data_get(list), \ list ? EINA_PREFETCH(((Eina_List *)list)->next) : EINA_PREFETCH(list); \ list; \ list = eina_list_remove_list(list, list), \ list ? EINA_PREFETCH(((Eina_List *)list)->next) : EINA_PREFETCH(list), \ data = eina_list_data_get(list))
Definition for the macro to remove each list node while having access to each node's data.
- Parameters:
-
[in,out] list The list that will be cleared. [out] data Current node's data.
This macro will call eina_list_remove_list for each list node, and store the data contained in the current node in data
.
The following diagram illustrates this macro iterating over a list of four elements ("one", "two", "three" and "four"):
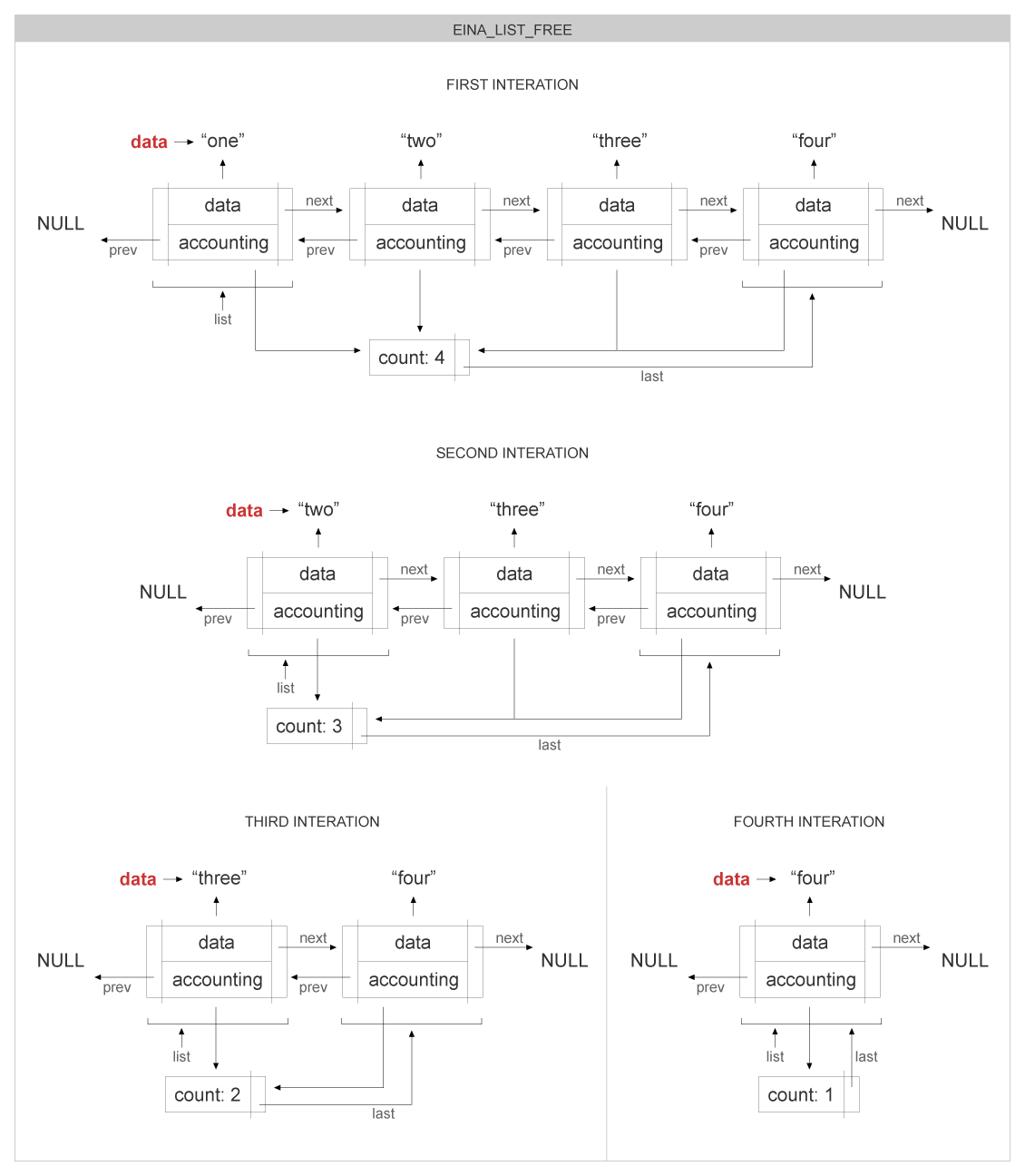
If you do not need to release node data, it is easier to call eina_list_free().
Eina_List *list; char *data; // list is already filled, // its elements are just duplicated strings, EINA_LIST_FREE(list, data) free(data);
- Warning:
list
must be a pointer to the first element of the list.
- See also:
- eina_list_free()
#define EINA_LIST_REVERSE_FOREACH | ( | list, | |
l, | |||
_data | |||
) |
for (l = eina_list_last(list), \ _data = eina_list_data_get(l), \ l ? (EINA_PREFETCH(((Eina_List *)l)->prev), EINA_PREFETCH(_data)) : EINA_PREFETCH(l); \ l; \ l = eina_list_prev(l), \ _data = eina_list_data_get(l), \ l ? (EINA_PREFETCH(((Eina_List *)l)->prev), EINA_PREFETCH(_data)) : EINA_PREFETCH(l))
Definition for the macro to iterate over a list in the reverse order.
- Parameters:
-
[in] list The list to iterate over. [out] l A list that is used as an iterator and points to the current node. [out] _data Current item's data.
This macro works like EINA_LIST_FOREACH, but iterates from the last element of a list to the first. data
is the data related to the current element, while l
is an Eina_List that is used as the list iterator.
The following diagram illustrates this macro iterating over a list of four elements("one", "two", "three" and "four"):
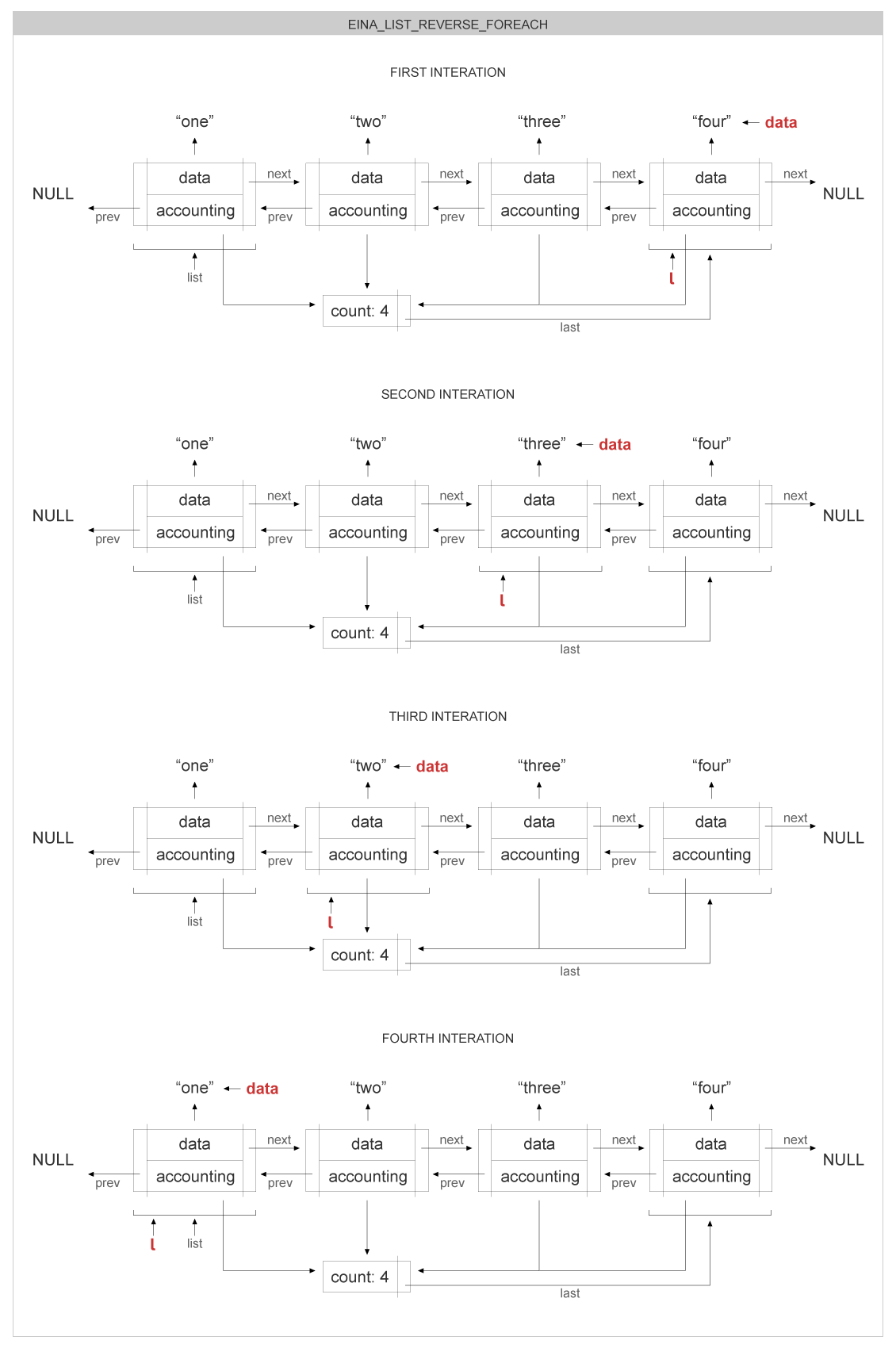
It can be used to free list data, as in the following example:
Eina_List *list; Eina_List *l; char *data; // list is already filled, // its elements are just duplicated strings, // EINA_LIST_REVERSE_FOREACH will be used to free those strings EINA_LIST_REVERSE_FOREACH(list, l, data) free(data); eina_list_free(list);
- Note:
- This is not the optimal way to release memory allocated to a list, since it iterates over the list twice. For an optimized algorithm, use EINA_LIST_FREE().
- Warning:
list
must be a pointer to the first element of the list.- Be careful when deleting list nodes. If you remove the current node and continue iterating, the code will fail because the macro will not be able to get the next node. Notice that it's OK to remove any node if you stop the loop after that. For destructive operations such as this, consider using EINA_LIST_REVERSE_FOREACH_SAFE().
#define EINA_LIST_REVERSE_FOREACH_SAFE | ( | list, | |
l, | |||
l_prev, | |||
data | |||
) |
for (l = eina_list_last(list), \ l_prev = eina_list_prev(l), \ EINA_PREFETCH(l_prev), \ data = eina_list_data_get(l); \ EINA_PREFETCH(data), \ l; \ l = l_prev, \ l_prev = eina_list_prev(l), \ EINA_PREFETCH(l_prev), \ data = eina_list_data_get(l), \ EINA_PREFETCH(data))
Definition for the macro to iterate over a list in the reverse order with support for deletion.
- Parameters:
-
[in] list The list to iterate over. [out] l A list that is used as an iterator and points to the current node. [out] l_prev A list that is used as an iterator and points to the previous node. [out] data Current item's data.
This macro works like EINA_LIST_FOREACH_SAFE, but iterates from the last element of a list to the first. data
is the data related to the current element, while l
is an Eina_List that is used as the list iterator.
Since this macro stores a pointer to the previous list node in l_prev
, deleting the current node and continuing looping is safe.
The following diagram illustrates this macro iterating over a list of four elements ("one", "two", "three" and "four"):
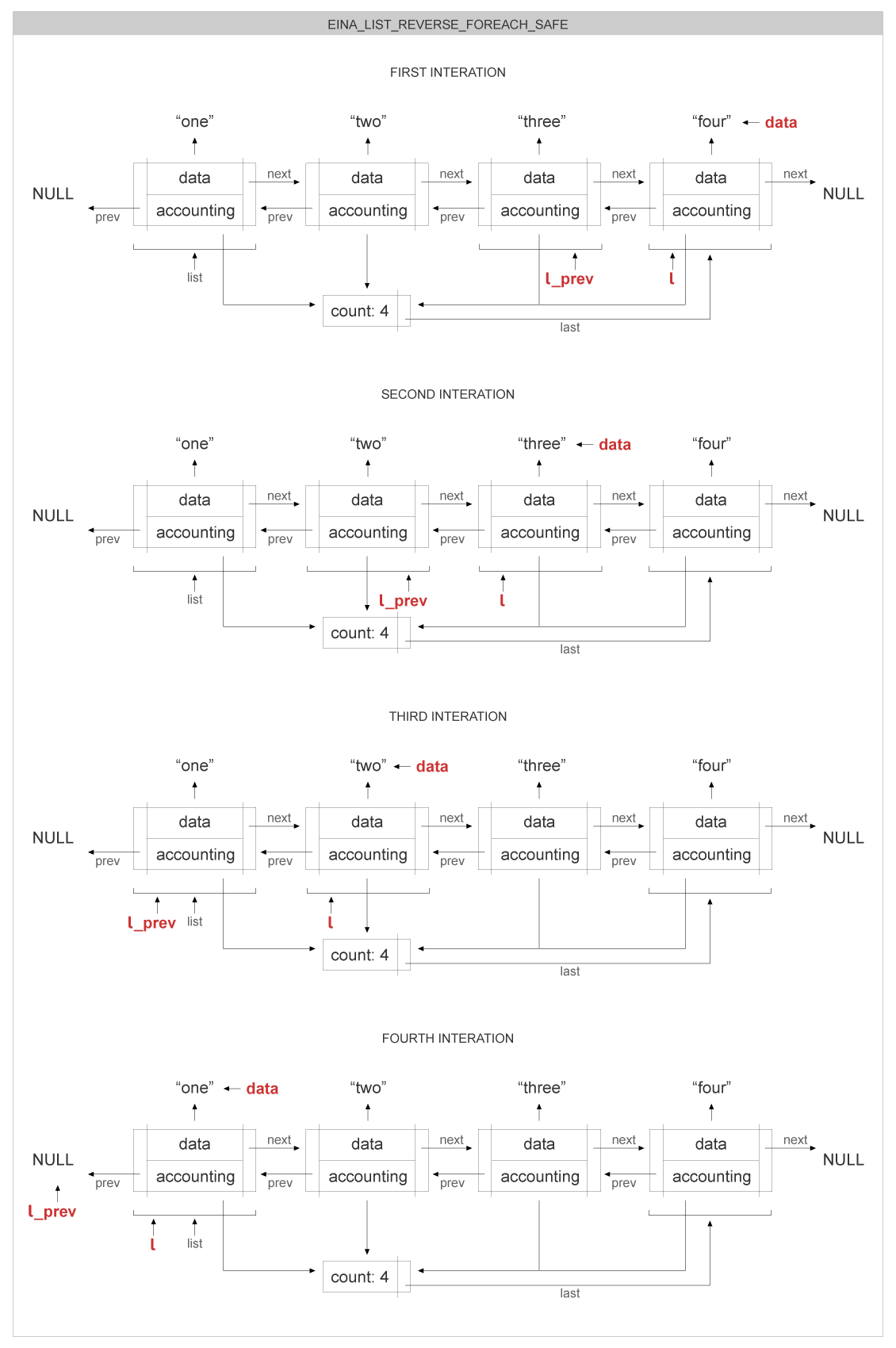
This macro can be used to free list nodes, as in the following example:
Eina_List *list; Eina_List *l; Eina_List *l_prev; char *data; // list is already filled, // its elements are just duplicated strings, // EINA_LIST_REVERSE_FOREACH_SAFE will be used to free elements that match "key". EINA_LIST_REVERSE_FOREACH_SAFE(list, l, l_prev, data) if (strcmp(data, "key") == 0) { free(data); list = eina_list_remove_list(list, l); }
- Warning:
list
must be a pointer to the first element of the list.
Typedef Documentation
Type for a generic double linked list.
Cache used to store the last element of a list and the number of elements, for fast access.
Function Documentation
Eina_Accessor* eina_list_accessor_new | ( | const Eina_List * | list | ) |
Returns a new accessor associated with a list.
- Parameters:
-
[in] list The list.
- Returns:
- A new accessor, or
NULL
on error.
This function returns a newly allocated accessor associated with list
. If list
is NULL
or the count member of list
is less or equal than 0, this function returns NULL
. If the memory can not be allocated, NULL
is returned; otherwise, a valid accessor is returned.
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_accessor_01.c.
Eina_List* eina_list_append | ( | Eina_List * | list, |
const void * | data | ||
) |
Appends the given data to the given linked list.
- Parameters:
-
[in,out] list The given list. [in] data The data to append.
- Returns:
- A list pointer, or
NULL
on error.
This function appends data
to list
. If list
is NULL
, a new list is returned. On success, a new list pointer that should be used in place of the one given to this function is returned. Otherwise, the old pointer is returned.
The following example code demonstrates how to ensure that the given data has been successfully appended.
Eina_List *list = NULL; extern void *my_data; list = eina_list_append(list, my_data);
- Warning:
list
must be a pointer to the first element of the list(or NULL).
- Since :
- 2.3.1
Eina_List* eina_list_append_relative | ( | Eina_List * | list, |
const void * | data, | ||
const void * | relative | ||
) |
Inserts the given data into the given linked list after the specified data.
- Parameters:
-
[in,out] list The given linked list. [in] data The data to insert. [in] relative The data to insert after.
- Returns:
- A list pointer, or
NULL
on error.
This function inserts data
into list
after relative
. If relative
is not in the list, data
is appended to the list. If list
is NULL
, a new list is returned. If there are multiple instances of relative
in the list, data
is inserted after the first instance. On success, a new list pointer that should be used in place of the one given to this function is returned. Otherwise, the old pointer is returned.
The following example code demonstrates how to ensure that the given data has been successfully inserted.
Eina_List *list = NULL; extern void *my_data; extern void *relative_member; list = eina_list_append(list, relative_member); list = eina_list_append_relative(list, my_data, relative_member);
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_01.c.
Eina_List* eina_list_append_relative_list | ( | Eina_List * | list, |
const void * | data, | ||
Eina_List * | relative | ||
) |
Appends a list node to a linked list after the specified member.
- Parameters:
-
[in,out] list The given linked list. [in] data The data to insert. [in] relative The list node to insert after.
- Returns:
- A list pointer, or
NULL
on error.
This function inserts data
into list
after the list node relative
. If list
or relative
are NULL
, data
is just appended to list
using eina_list_append(). If list
is NULL
, a new list is returned. If there are multiple instances of relative
in the list, data
is inserted after the first instance. On success, a new list pointer that should be used in place of the one given to this function is returned. Otherwise, the old pointer is returned.
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_01.c, and eina_list_02.c.
Eina_List* eina_list_clone | ( | const Eina_List * | list | ) |
Clones (copies) all the elements in the list in exactly same order.
- Parameters:
-
[in] list The list to clone.
- Returns:
- The new list that has been cloned, or
NULL
on error.
This function clone in order of all elements in list
. If list
is NULL
, this function returns NULL
. This returns a copy of the given list.
- Note:
- copy: this will copy the list and you should then eina_list_free() when it is not required anymore.
- Warning:
list
must be a pointer to the first element of the list.
- See also:
- eina_list_reverse_clone()
- Since :
- 2.3.1
static unsigned int eina_list_count | ( | const Eina_List * | list | ) | [static] |
Gets the count of the number of items in a list.
- Parameters:
-
[in] list The list whose count to return.
- Returns:
- The number of members in the list.
This function returns the quantity of members that list
contains. If the list is NULL
, 0
is returned.
NB: This is an order-1 operation and takes the same time regardless of the length of the list.
- Warning:
list
must be a pointer to the first element of the list.
void* eina_list_data_find | ( | const Eina_List * | list, |
const void * | data | ||
) |
Finds a member of a list and returns it.
- Parameters:
-
[in] list The linked list to search. [in] data The data member to find in the list.
- Returns:
- The data member pointer if found, or
NULL
otherwise.
This function searches in list
from beginning to end for the first member whose data pointer is data
. If it is found, data
will be returned, otherwise NULL
will be returned.
Example:
extern Eina_List *list; extern void *my_data; if (eina_list_data_find(list, my_data) == my_data) { printf("Found member %p\n", my_data); }
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
Eina_List* eina_list_data_find_list | ( | const Eina_List * | list, |
const void * | data | ||
) |
Finds a member of a list and returns it as a list node.
- Parameters:
-
[in] list The list to search for data. [in] data The data pointer to find in the list.
- Returns:
- A list node containing the data member on success,
NULL
otherwise.
This function searches list
from beginning to end for the first member whose data pointer is data
. If it is found, the list node containing the specified member is returned, otherwise NULL
is returned.
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_03.c.
static void* eina_list_data_get | ( | const Eina_List * | list | ) | [static] |
Gets the list node data member.
- Parameters:
-
[in] list The list node to get the data member of.
- Returns:
- The data member from the list node.
This function returns the data member of the specified list node list
. It is equivalent to list->data. If list
is NULL
, this function returns NULL
.
- Warning:
list
must be a pointer to the first element of the list.
- Examples:
- box_example_02.c, eina_list_04.c, menu_example_01.c, and slideshow_example.c.
int eina_list_data_idx | ( | const Eina_List * | list, |
void * | data | ||
) |
Finds the member of the list and returns the index.
- Parameters:
-
[in] list The list. [in] data The data member.
- Returns:
- The index of data member if found,
-1
otherwise.
This function searches in list
from beginning to end for the first member whose data pointer is data
. If it is found, the index of the data will be returned, otherwise -1
will be returned.
- Warning:
list
must be a pointer to the first element of the list.
- Since (EFL) :
- 1.14
- Since :
- 3.0
static void* eina_list_data_set | ( | Eina_List * | list, |
const void * | data | ||
) | [static] |
Sets the list node data member.
- Parameters:
-
[in,out] list The list node to set the data member of. [in] data The data to be set.
- Returns:
- The previous data value.
This function sets the data member data
of the specified list node list
. It returns the previous data of the node. If list
is NULL
, this function returns NULL
.
- Warning:
list
must be a pointer to the first element of the list.
- Examples:
- eina_list_03.c.
Eina_List* eina_list_demote_list | ( | Eina_List * | list, |
Eina_List * | move_list | ||
) |
Moves the specified data to the tail of the list.
- Parameters:
-
[in,out] list The given linked list. [in] move_list The list node to move.
- Returns:
- A new list handle to replace the old one, or
NULL
on error.
This function move move_list
to the end of list
. If list is NULL
, NULL
is returned. If move_list
is NULL
, list
is returned. Otherwise, a new list pointer that should be used in place of the one passed to this function is returned.
Example:
extern Eina_List *list; Eina_List *l; extern void *my_data; void *data; EINA_LIST_FOREACH(list, l, data) { if (data == my_data) { list = eina_list_demote_list(list, l); break; } }
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- box_example_02.c, and eina_list_03.c.
Eina_List* eina_list_free | ( | Eina_List * | list | ) |
Frees an entire list and all the nodes, ignoring the data contained.
- Parameters:
-
[in,out] list The list to free.
- Returns:
- A
NULL
pointer.
This function frees all the nodes of list
. It does not free the data of the nodes. To free them, use EINA_LIST_FREE.
- Since :
- 2.3.1
Eina_Iterator* eina_list_iterator_new | ( | const Eina_List * | list | ) |
Returns a new iterator associated with a list.
- Parameters:
-
[in] list The list.
- Returns:
- A new iterator, or
NULL
on memory allocation failure.
This function returns a newly allocated iterator associated with list
. If list
is NULL
or the count member of list
is less than or equal to 0, this function still returns a valid iterator that will always return false on eina_iterator_next(), thus keeping API sane.
- Warning:
list
must be a pointer to the first element of the list.- if the list structure changes then the iterator becomes invalid! That is, if you add or remove nodes this iterator behavior is undefined and your program may crash!
- Since :
- 2.3.1
- Examples:
- eina_iterator_01.c, and eina_list_03.c.
Eina_Iterator* eina_list_iterator_reversed_new | ( | const Eina_List * | list | ) |
Returns a new reversed iterator associated with a list.
- Parameters:
-
[in] list The list.
- Returns:
- A new iterator, or
NULL
on memory allocation failure.
This function returns a newly allocated iterator associated with list
. If list
is NULL
or the count member of list
is less than or equal to 0, this function still returns a valid iterator that will always return false on eina_iterator_next(), thus keeping API sane.
Unlike eina_list_iterator_new(), this will walk the list backwards.
- Warning:
list
must be a pointer to the first element of the list.- if the list structure changes then the iterator becomes invalid! That is, if you add or remove nodes this iterator behavior is undefined and your program may crash!
- Since :
- 2.3.1
static Eina_List* eina_list_last | ( | const Eina_List * | list | ) | [static] |
Gets the last list node in the list.
- Parameters:
-
[in] list The list to get the last list node from.
- Returns:
- The last list node in the list.
This function returns the last list node in the list list
. If list
is NULL
or empty, NULL
is returned.
This is a order-1 operation (it takes the same short time regardless of the length of the list).
- Warning:
list
must be a pointer to the first element of the list.
- Examples:
- eina_list_04.c.
static void* eina_list_last_data_get | ( | const Eina_List * | list | ) | [static] |
Returns the last list node's data.
- Parameters:
-
[in] list The list.
- Returns:
- The node's data, or
NULL
on being passed aNULL
pointer
This function is a shortcut for typing eina_list_data_get(eina_list_last())
- Since (EFL) :
- 1.8
Eina_List* eina_list_merge | ( | Eina_List * | left, |
Eina_List * | right | ||
) |
Merges two list.
- Parameters:
-
[in] left Head list to merge. [in] right Tail list to merge.
- Returns:
- A new merged list.
This function puts right at the end of left and returns the head.
Both left and right do not exist anymore after the merge.
- Note:
- Merge cost is O(n), being n the size of the smallest list. This is due the need to fix accounting of that segment, making count and last access O(1).
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
Eina_Bool eina_list_move | ( | Eina_List ** | to, |
Eina_List ** | from, | ||
void * | data | ||
) |
Moves a data pointer from one list to another.
- Parameters:
-
[in,out] to The list to move the data to. [in,out] from The list to move from. [in] data The data member to move.
- Returns:
- EINA_TRUE on success, EINA_FALSE otherwise.
This function is a shortcut for doing the following: to = eina_list_append(to, data); from = eina_list_remove(from, data);
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
Eina_Bool eina_list_move_list | ( | Eina_List ** | to, |
Eina_List ** | from, | ||
Eina_List * | data | ||
) |
Moves a list node from one list to another.
- Parameters:
-
[in,out] to The list to move the data to. [in,out] from The list to move from. [in] data The list node containing the data to move.
- Returns:
- EINA_TRUE on success, EINA_FALSE otherwise.
This function is a shortcut for doing the following: to = eina_list_append(to, data->data); from = eina_list_remove_list(from, data);
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
static Eina_List* eina_list_next | ( | const Eina_List * | list | ) | [static] |
Gets the next list node after the specified list node.
- Parameters:
-
[in] list The list node to get the next list node from
- Returns:
- The next list node on success,
NULL
otherwise.
This function returns the next list node after the current one in list
. It is equivalent to list->next. If list
is NULL
or if no next list node exists, it returns NULL
.
- Warning:
list
must be a pointer to the first element of the list.
- Examples:
- eina_list_04.c.
void* eina_list_nth | ( | const Eina_List * | list, |
unsigned int | n | ||
) |
Gets the nth member's data pointer in a list, or NULL
on error.
- Parameters:
-
[in] list The list to get the specified member number from. [in] n The number of the element (0 being the first).
- Returns:
- The data pointer stored in the specified element.
This function returns the data pointer of element number n
, in the list
. The first element in the array is element number 0. If the element number n
does not exist, NULL
is returned. Otherwise, the data of the found element is returned.
- Note:
- Worst case is O(n).
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- evas-box.c.
Eina_List* eina_list_nth_list | ( | const Eina_List * | list, |
unsigned int | n | ||
) |
Gets the nth member's list node in a list.
- Parameters:
-
[in] list The list to get the specified member number from. [in] n The number of the element (0 being the first).
- Returns:
- The list node stored in the numbered element, or
NULL
on error.
This function returns the list node of element number n
, in list
. The first element in the array is element number 0. If the element number n
does not exist or list
is NULL
or n
is greater than the count of elements in list
minus 1, NULL
is returned. Otherwise the list node stored in the numbered element is returned.
- Note:
- Worst case is O(n).
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_01.c, and eina_list_03.c.
Eina_List* eina_list_prepend | ( | Eina_List * | list, |
const void * | data | ||
) |
Prepends the given data to the given linked list.
- Parameters:
-
[in,out] list The given list. [in] data The data to prepend.
- Returns:
- A list pointer, or
NULL
on error.
This function prepends data
to list
. If list
is NULL
, a new list is returned. On success, a new list pointer that should be used in place of the one given to this function is returned. Otherwise, the old pointer is returned.
The following example code demonstrates how to ensure that the given data has been successfully prepended.
Example:
Eina_List *list = NULL; extern void *my_data; list = eina_list_prepend(list, my_data);
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_inlist_02.c, and eina_list_01.c.
Eina_List* eina_list_prepend_relative | ( | Eina_List * | list, |
const void * | data, | ||
const void * | relative | ||
) |
Prepends a data pointer to a linked list before the specified member.
- Parameters:
-
[in,out] list The given linked list. [in] data The data to insert. [in] relative The member that data will be inserted before.
- Returns:
- A list pointer, or
NULL
on error.
This function inserts data
to list
before relative
. If relative
is not in the list, data
is prepended to the list with eina_list_prepend(). If list
is NULL
, a new list is returned. If there are multiple instances of relative
in the list, data
is inserted before the first instance. On success, a new list pointer that should be used in place of the one given to this function is returned. Otherwise, the old pointer is returned.
The following code example demonstrates how to ensure that the given data has been successfully inserted.
Eina_List *list = NULL; extern void *my_data; extern void *relative_member; list = eina_list_append(list, relative_member); list = eina_list_prepend_relative(list, my_data, relative_member);
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_01.c.
Eina_List* eina_list_prepend_relative_list | ( | Eina_List * | list, |
const void * | data, | ||
Eina_List * | relative | ||
) |
Prepends a list node to a linked list before the specified member.
- Parameters:
-
[in,out] list The given linked list. [in] data The data to insert. [in] relative The list node to insert before.
- Returns:
- A list pointer, or
NULL
on error.
This function inserts data
to list
before the list node relative
. If list
or relative
are NULL
, data
is just prepended to list
using eina_list_prepend(). If list
is NULL
, a new list is returned. If there are multiple instances of relative
in the list, data
is inserted before the first instance. On success, a new list pointer that should be used in place of the one given to this function is returned. Otherwise, the old pointer is returned.
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_01.c, and eina_list_02.c.
static Eina_List* eina_list_prev | ( | const Eina_List * | list | ) | [static] |
Gets the list node prior to the specified list node.
- Parameters:
-
[in] list The list node to get the previous list node from.
- Returns:
- The previous list node on success,
NULL
otherwise.
This function returns the previous list node before the current one in list
. It is equivalent to list->prev. If list
is NULL
or if no previous list node exists, it returns NULL
.
- Warning:
list
must be a pointer to the first element of the list.
- Examples:
- eina_list_04.c.
Eina_List* eina_list_promote_list | ( | Eina_List * | list, |
Eina_List * | move_list | ||
) |
Moves the specified data to the head of the list.
- Parameters:
-
[in,out] list The given linked list. [in] move_list The list node to move.
- Returns:
- A new list handle to replace the old one, or
NULL
on error.
This function moves move_list
to the front of list
. If list is NULL
, NULL
is returned. If move_list
is NULL
, list
is returned. Otherwise, a new list pointer that should be used in place of the one passed to this function is returned.
Example:
extern Eina_List *list; Eina_List *l; extern void *my_data; void *data; EINA_LIST_FOREACH(list, l, data) { if (data == my_data) { list = eina_list_promote_list(list, l); break; } }
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_03.c.
Eina_List* eina_list_remove | ( | Eina_List * | list, |
const void * | data | ||
) |
Removes the first instance of the specified data from the given list.
- Parameters:
-
[in,out] list The given list. [in] data The specified data.
- Returns:
- A list pointer, or
NULL
on error.
This function removes the first instance of data
from list
. If data
is not in the given list or is NULL
, nothing is done and the specified list
returned. If list
is NULL
, NULL
is returned, otherwise a new list pointer that should be used in place of the one passed to this function is returned.
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- efl_thread_6.c, eina_list_03.c, and genlist_example_05.c.
Eina_List* eina_list_remove_list | ( | Eina_List * | list, |
Eina_List * | remove_list | ||
) |
Removes the specified list node.
- Parameters:
-
[in,out] list The given linked list. [in] remove_list The list node which is to be removed.
- Returns:
- A list pointer, or
NULL
on error.
This function removes the list node remove_list
from list
and frees the list node structure remove_list
. If list
is NULL
, this function returns NULL
. If remove_list
is NULL
, it returns list
; otherwise, a new list pointer that should be used in place of the one passed to this function.
The following code gives an example. (Notice we use EINA_LIST_FOREACH rather than EINA_LIST_FOREACH_SAFE because we stop the loop after removing the current node.)
extern Eina_List *list; Eina_List *l; extern void *my_data; void *data EINA_LIST_FOREACH(list, l, data) { if (data == my_data) { list = eina_list_remove_list(list, l); break; } }
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_inlist_02.c.
Eina_List* eina_list_reverse | ( | Eina_List * | list | ) |
Reverses all the elements in the list.
- Parameters:
-
[in,out] list The list to reverse.
- Returns:
- The list head after it has been reversed, or
NULL
on error.
This function reverses the order of all elements in list
, so the last member is now first, and so on. If list
is NULL
, this function returns NULL
.
- Note:
- in-place: this will change the given list, so you should now point to the new list head that is returned by this function.
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
Eina_List* eina_list_reverse_clone | ( | const Eina_List * | list | ) |
Clones (copies) all the elements in the list in reverse order.
- Parameters:
-
[in] list The list to reverse.
- Returns:
- The new list that has been reversed, or
NULL
on error.
This function reverses the order of all elements in list
, so the last member is now first, and so on. If list
is NULL
, this function returns NULL
. This returns a copy of the given list.
- Note:
- copy: this will copy the list and you should then eina_list_free() when it is not required anymore.
- Warning:
list
must be a pointer to the first element of the list.
- See also:
- eina_list_reverse()
- eina_list_clone()
- Since :
- 2.3.1
- Examples:
- eina_list_03.c.
void* eina_list_search_sorted | ( | const Eina_List * | list, |
Eina_Compare_Cb | func, | ||
const void * | data | ||
) |
Returns node data if it is in the sorted list.
- Parameters:
-
[in] list The list to search for data, must be sorted. [in] func A function pointer that can handle comparing the list data nodes. [in] data reference value to search.
- Returns:
- The node value (
node->data
) if func(node->data, data) == 0,NULL
if not found.
This can be used to check if some value is inside the list and get the existing instance in this case. It should be used when list is known to be sorted as it will do binary search for results.
Example: imagine user gives a string, you check if it's in the list before duplicating its contents.
- Note:
- O(log2(n)) average/worst case performance, for 1,000,000 elements it will do a maximum of 20 comparisons. This is much faster than the 1,000,000 comparisons made by eina_list_search_unsorted(), so depending on the number of searches and insertions, it may be worth to eina_list_sort() the list and do the searches later. As said in eina_list_search_sorted_near_list(), lists do not have O(1) access time, so walking to the correct node can be costly, consider worst case to be almost O(n) pointer dereference (list walk).
- Warning:
list
must be a pointer to the first element of the list.
- See also:
- eina_list_search_sorted_list()
- eina_list_sort()
- eina_list_sorted_merge()
- eina_list_search_unsorted_list()
- Since :
- 2.3.1
- Examples:
- eina_list_02.c.
Eina_List* eina_list_search_sorted_list | ( | const Eina_List * | list, |
Eina_Compare_Cb | func, | ||
const void * | data | ||
) |
Returns node if data is in the sorted list.
- Parameters:
-
[in] list The list to search for data, must be sorted. [in] func A function pointer that can handle comparing the list data nodes. [in] data reference value to search.
- Returns:
- The node if func(node->data, data) == 0,
NULL
if not found.
This can be used to check if some value is inside the list and get the container node in this case. It should be used when list is known to be sorted as it will do binary search for results.
Example: imagine user gives a string, you check if it's in the list before duplicating its contents.
- Note:
- O(log2(n)) average/worst case performance, for 1,000,000 elements it will do a maximum of 20 comparisons. This is much faster than the 1,000,000 comparisons made by eina_list_search_unsorted_list(), so depending on the number of searches and insertions, it may be worth to eina_list_sort() the list and do the searches later. As said in eina_list_search_sorted_near_list(), lists do not have O(1) access time, so walking to the correct node can be costly, consider worst case to be almost O(n) pointer dereference (list walk).
- Warning:
list
must be a pointer to the first element of the list.
- See also:
- eina_list_search_sorted()
- eina_list_sort()
- eina_list_sorted_merge()
- eina_list_search_unsorted_list()
- eina_list_search_sorted_near_list()
- Since :
- 2.3.1
- Examples:
- eina_list_02.c.
Eina_List* eina_list_search_sorted_near_list | ( | const Eina_List * | list, |
Eina_Compare_Cb | func, | ||
const void * | data, | ||
int * | result_cmp | ||
) |
Returns node nearest to data from the sorted list.
- Parameters:
-
[in] list The list to search for data, must be sorted. [in] func A function pointer that can handle comparing the list data nodes. [in] data reference value to search. [out] result_cmp If provided returns the result of func(node->data, data) node being the last (returned) node. If node was found (exact match), then it is 0. If returned node is smaller than requested data, it is less than 0 and if it's bigger it's greater than 0. It is the last value returned by func().
- Returns:
- The nearest node,
NULL
if not found.
This function searches for a node containing data
as its data in list
, if such a node exists it will be returned and result_cmp
will be 0
. If the data of no node in list
is equal to data
, the node with the nearest value to that will be returned and result_cmp
will be the return value of func
with data
and the returned node's data as arguments.
This function is useful for inserting an element in the list only in case it isn't already present in the list, the naive way of doing this would be:
void *ptr = eina_list_data_find(list, "my data"); if (!ptr) eina_list_sorted_insert(list, "my data");
However this has the downside of walking through the list twice, once to check if the data is already present and another to insert the element in the correct position. This can be done more efficiently:
int cmp_result; l = eina_list_search_sorted_near_list(list, cmp_func, "my data", &cmp_result); if (cmp_result > 0) list = eina_list_prepend_relative_list(list, "my data", l); else if (cmp_result < 0) list = eina_list_append_relative_list(list, "my data", l);
If cmp_result is 0 the element is already in the list and we need not insert it, if cmp_result is greater than zero "my @a data" needs to come after l (the nearest node present), if less than zero before.
- Note:
- O(log2(n)) average/worst case performance, for 1,000,000 elements it will do a maximum of 20 comparisons. This is much faster than the 1,000,000 comparisons made naively walking the list from head to tail, so depending on the number of searches and insertions, it may be worth to eina_list_sort() the list and do the searches later. As lists do not have O(1) access time, walking to the correct node can be costly, consider worst case to be almost O(n) pointer dereference (list walk).
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_02.c.
void* eina_list_search_unsorted | ( | const Eina_List * | list, |
Eina_Compare_Cb | func, | ||
const void * | data | ||
) |
Returns node data if it is in the unsorted list.
- Parameters:
-
[in] list The list to search for data, may be unsorted. [in] func A function pointer that can handle comparing the list data nodes. [in] data reference value to search.
- Returns:
- The node value (
node->data
) if func(node->data, data) == 0,NULL
if not found.
This can be used to check if some value is inside the list and get the existing instance in this case.
Example: imagine user gives a string, you check if it's in the list before duplicating its contents.
- Note:
- this is expensive and may walk the whole list, it's order-N, that is, for 1,000,000 elements list it may walk and compare 1,000,000 nodes.
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_02.c.
Eina_List* eina_list_search_unsorted_list | ( | const Eina_List * | list, |
Eina_Compare_Cb | func, | ||
const void * | data | ||
) |
Returns node if data is in the unsorted list.
- Parameters:
-
[in] list The list to search for data, may be unsorted. [in] func A function pointer that can handle comparing the list data nodes. [in] data reference value to search.
- Returns:
- The node if func(node->data, data) == 0,
NULL
if not found.
This can be used to check if some value is inside the list and get the container node in this case.
Example: imagine user gives a string, you check if it's in the list before duplicating its contents.
- Note:
- this is expensive and may walk the whole list, it's order-N, that is for 1,000,000 elements list it may walk and compare 1,000,000 nodes.
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_02.c.
Eina_List* eina_list_shuffle | ( | Eina_List * | list, |
Eina_Random_Cb | func | ||
) |
Shuffles list.
- Parameters:
-
[in] list The list handle to shuffle. [in] func A function pointer that can return an int between 2 inclusive values
- Returns:
- The new head of list.
This function shuffles list
. func
is used to generate random list indexes within the range of unshuffled list items. If func
is NULL
, rand is used.
- Note:
- in-place: This will change the given list, so you should now point to the new list head that is returned by this function.
- Since (EFL) :
- 1.8
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 3.0
Eina_List* eina_list_sort | ( | Eina_List * | list, |
unsigned int | limit, | ||
Eina_Compare_Cb | func | ||
) |
Sorts a list according to the ordering func will return.
- Parameters:
-
[in] list The list handle to sort. [in] limit The maximum number of list elements to sort. [in] func A function pointer that can handle comparing the list data nodes.
- Returns:
- The new head of list.
This function sorts list
. If limit
is 0 or greater than the number of elements in list
, all the elements are sorted. func
is used to compare two elements of list
. If func
is NULL
, this function returns list
.
- Note:
- in-place: this will change the given list, so you should now point to the new list head that is returned by this function.
- Worst case is O(n * log2(n)) comparisons (calls to func()). That means that for 1,000,000 list sort will do 20,000,000 comparisons.
Example:
int sort_cb(const void *d1, const void *d2) { const char *txt = d1; const char *txt2 = d2; if(!txt) return(1); if(!txt2) return(-1); return(strcmp(txt, txt2)); } extern Eina_List *list; list = eina_list_sort(list, eina_list_count(list), sort_cb);
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_02.c.
Eina_List* eina_list_sorted_insert | ( | Eina_List * | list, |
Eina_Compare_Cb | func, | ||
const void * | data | ||
) |
Inserts a new node into a sorted list.
- Parameters:
-
[in,out] list The given linked list, must be sorted. [in] func The function called for the sort. [in] data The data to be inserted in sorted order.
- Returns:
- A list pointer, or
NULL
on error.
This function inserts values into a linked list assuming it was sorted and the result will be sorted. If list
is NULL
, a new list is returned. On success, a new list pointer that should be used in place of the one given to this function is returned. Otherwise, the old pointer is returned.
- Note:
- O(log2(n)) comparisons (calls to
func
) average/worst case performance as it uses eina_list_search_sorted_near_list() and thus is bounded to that. As said in eina_list_search_sorted_near_list(), lists do not have O(1) access time, so walking to the correct node can be costly, consider worst case to be almost O(n) pointer dereference (list walk).
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_02.c.
Eina_List* eina_list_sorted_merge | ( | Eina_List * | left, |
Eina_List * | right, | ||
Eina_Compare_Cb | func | ||
) |
Merges two sorted list according to the ordering func will return.
- Parameters:
-
[in] left First list to merge. [in] right Second list to merge. [in] func A function pointer that can handle comparing the list data nodes.
- Returns:
- A new sorted list.
This function compares the head of left
and right
, and choose the smallest one to be head of the returned list. It will continue this process for all entry of both list.
Both left and right lists are not valid anymore after the merge and should not be used. If func
is NULL
, it will return NULL
.
Example:
int sort_cb(void *d1, void *d2) { const char *txt = NULL; const char *txt2 = NULL; if(!d1) return(1); if(!d2) return(-1); return(strcmp((const char*)d1, (const char*)d2)); } extern Eina_List *sorted1; extern Eina_List *sorted2; list = eina_list_sorted_merge(sorted1, sorted2, sort_cb);
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_02.c.
Eina_List* eina_list_split_list | ( | Eina_List * | list, |
Eina_List * | relative, | ||
Eina_List ** | right | ||
) |
Splits a list into 2 lists.
- Parameters:
-
[in] list List to split. [in] relative The list will be split after relative
.[out] right The head of the new right list.
- Returns:
- The new left list
This function splits list
into two lists ( left and right ) after the node relative
. Relative
will become the last node of the left list. If list
or right
are NULL
list is returns. If relative
is NULL right is set to list
and NULL
is returns. If relative
is the last node of list
list is returns and right
is set to NULL
.
list does not exist anymore after the split.
- Warning:
list
must be a pointer to the first element of the list.
- Since :
- 2.3.1
- Examples:
- eina_list_02.c.