Tizen Native API
7.0
|
The Push API provides functions to receive push notifications.
Required Header
#include <push-service.h>
Overview
The Push API allows your application to receive push notifications from a push server. The push service is a client daemon that maintains a permanent connection between your device and the push server, in order to process your registration/deregistration requests and deliver push notifications to your application. If the application is connected, the push service passes the notification data over the connection. Otherwise, the push service posts a UI notification with the data. It will be delivered when a user launches the application by selecting the posting.
To receive push notifications, you need to follow the steps below:
- Connecting to the push service
- Registering your application
- Getting notification data
Push notification helps your application server send data to the application on devices over an IP network even if the application is not running. Push notification may reduce battery consumption over other applications keeping its own connection to its remote application server.
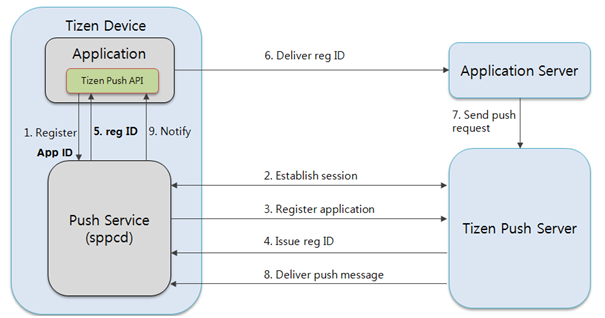
Service Limitation
- Payload of a push message is limited to 4096 bytes.
- Administrative permission is needed for your application and server to use the push service.
- Push service does not guarantee delivery and order of push messages.
- A device should have a valid IMEI number.
Functions | |
int | push_service_connect (const char *push_app_id, push_service_state_cb state_callback, push_service_notify_cb notify_callback, void *user_data, push_service_connection_h *connection) |
Connects to the push service and sets callback functions. | |
void | push_service_disconnect (push_service_connection_h connection) |
. Closes the connection and releases all its resources | |
int | push_service_register (push_service_connection_h connection, push_service_result_cb result_callback, void *user_data) |
Registers an application to the push server. | |
int | push_service_deregister (push_service_connection_h connection, push_service_result_cb result_callback, void *user_data) |
Deregisters an application from the Push server. | |
char * | push_service_app_control_to_noti_data (app_control_h app_control, char *operation) |
Retrieves the payload data of a notification that forcibly launched the app. | |
int | push_service_app_control_to_notification (app_control_h app_control, char *operation, push_service_notification_h *noti) |
Retrieves the notification that forcibly launched the app. | |
int | push_service_get_notification_data (push_service_notification_h notification, char **data) |
Gets notification data sent by the server. | |
int | push_service_get_notification_message (push_service_notification_h notification, char **msg) |
Gets the notification message sent by the server. | |
int | push_service_get_notification_time (push_service_notification_h notification, long long int *received_time) |
Gets the received time of the notification message. | |
int | push_service_get_notification_sender (push_service_notification_h notification, char **sender) |
Gets the sender of the notification. | |
int | push_service_get_notification_session_info (push_service_notification_h notification, char **session_info) |
Gets the session ID of the notification. | |
int | push_service_get_notification_request_id (push_service_notification_h notification, char **request_id) |
Gets the request ID assigned by the sender. | |
int | push_service_get_notification_type (push_service_notification_h notification, int *type) |
. Gets the value in the type field of the notification | |
int | push_service_get_unread_notification (push_service_connection_h connection, push_service_notification_h *noti) |
Gets an unread notification message from the push server. | |
int | push_service_request_unread_notification (push_service_connection_h connection) |
Requests unread notification messages to the push server. | |
int | push_service_get_registration_id (push_service_connection_h connection, char **reg_id) |
Gets the registration ID in the PUSH_SERVICE_STATE_REGISTERED state. | |
void | push_service_free_notification (push_service_notification_h noti) |
Frees the notification handle. | |
Typedefs | |
typedef struct push_connection_s * | push_service_connection_h |
Connection to the push service handle. | |
typedef struct push_notification_s * | push_service_notification_h |
Notification delivered from the push server handle. | |
typedef void(* | push_service_state_cb )(push_service_state_e state, const char *err, void *user_data) |
Called when the registration state is refreshed. | |
typedef void(* | push_service_notify_cb )(push_service_notification_h noti, void *user_data) |
Called to handle a notification. | |
typedef void(* | push_service_result_cb )(push_service_result_e result, const char *msg, void *user_data) |
Called with the result of a registration/deregistration. | |
Defines | |
#define | APP_CONTROL_DATA_PUSH_LAUNCH_TYPE "http://tizen.org/appcontrol/data/push/launch_type" |
This is key string for getting extra data of App Control response. |
Define Documentation
#define APP_CONTROL_DATA_PUSH_LAUNCH_TYPE "http://tizen.org/appcontrol/data/push/launch_type" |
This is key string for getting extra data of App Control response.
- Since :
- 3.0
- Remarks:
- Your application could be launched in the background by push service.
If you want to know what push operation makes your application wake up, use app_control_get_extra_data() with below key.
In the case that your application is launched by push notification with "LAUNCH" option, you can see a value, "notification" in App Control callback.
On the other hand, when the state of registration changed, you can find the other value, "registration_change".
- See also:
- app_control_get_extra_data()
Typedef Documentation
typedef struct push_connection_s* push_service_connection_h |
Connection to the push service handle.
- Since :
- 2.3.1
typedef struct push_notification_s* push_service_notification_h |
Notification delivered from the push server handle.
- Since :
- 2.3.1
typedef void(* push_service_notify_cb)(push_service_notification_h noti, void *user_data) |
Called to handle a notification.
- Since :
- 2.3.1
- Parameters:
-
[in] noti A handle of the notification containing its payload
The handle is available inside this callback only.[in] user_data The user data passed to this callback
typedef void(* push_service_result_cb)(push_service_result_e result, const char *msg, void *user_data) |
Called with the result of a registration/deregistration.
- Since :
- 2.3.1
- Parameters:
-
[in] result The registration/deregistration result [in] msg The result message from the push server, otherwise NULL
[in] user_data The user data passed to this callback
typedef void(* push_service_state_cb)(push_service_state_e state, const char *err, void *user_data) |
Called when the registration state is refreshed.
- Since :
- 2.3.1
- Remarks:
- This callback will be invoked when the registration state is refreshed.
If the registration or deregistration has succeeded, then this state callback must be called.
In addition, the state can be changed if the push server deregisters the application.
- Parameters:
-
[in] state The registration state [in] err The error message [in] user_data The user data passed to this callback
- See also:
- push_service_connect()
Enumeration Type Documentation
enum push_service_error_e |
Enumerations of error codes for push API.
- Since :
- 2.3.1
- Enumerator:
enum push_service_state_e |
Enumeration of registration states.
- Since :
- 2.3.1
Function Documentation
char* push_service_app_control_to_noti_data | ( | app_control_h | app_control, |
char * | operation | ||
) |
Retrieves the payload data of a notification that forcibly launched the app.
When a notification arrives at the device with the "LAUNCH"
option or a user clicks a notification in the quick panel,
the push daemon forcibly launches the app and delivers the
notification to the app as a bundle. This function returns
the payload data in the notification.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/push
- Remarks:
- This function must be called in the app control callback function.
You must release the payload data using free().
push_service_app_control_to_notification() is preferred to this API.
The specific error code can be obtained using the get_last_result()
Error codes are described in the exception section.
- Parameters:
-
[in] app_control The app control handle that is handed over in the
app control callback function[in] operation The operation in the app control handle retrieved by
app_control_get_operation()
- Returns:
- The payload data (appData) in the notification
NULL if the app is not launched by a push notification.
- Exceptions:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_NO_DATA Not launched by a notification PUSH_SERVICE_ERROR_OPERATION_FAILED Operation fail PUSH_SERVICE_ERROR_OUT_OF_MEMORY Out of memory PUSH_SERVICE_ERROR_NOT_CONNECTED Connection to the daemon failed PUSH_SERVICE_ERROR_PERMISSION_DENIED No push privilege PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
- See also:
- app_control_get_operation()
int push_service_app_control_to_notification | ( | app_control_h | app_control, |
char * | operation, | ||
push_service_notification_h * | noti | ||
) |
Retrieves the notification that forcibly launched the app.
When a notification arrives at the device with the "LAUNCH"
option or a user clicks a notification in the quick panel,
the push daemon forcibly launches the app and delivers the
notification to the app as a bundle. This function returns
the notification from the bundle.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/push
- Remarks:
- This function must be called in the app control callback function.
You must release the notification using push_service_free_notification().
- Parameters:
-
[in] app_control The app control handle that is handed over in the
app control callback function[in] operation The operation in the app control handle retrieved by
app_control_get_operation()[out] noti The handle of the notification that forcibly launched the app
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_NO_DATA Not launched by a notification PUSH_SERVICE_ERROR_OPERATION_FAILED Operation fail PUSH_SERVICE_ERROR_OUT_OF_MEMORY Out of memory PUSH_SERVICE_ERROR_NOT_CONNECTED Connection to the daemon failed PUSH_SERVICE_ERROR_PERMISSION_DENIED No push privilege PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
int push_service_connect | ( | const char * | push_app_id, |
push_service_state_cb | state_callback, | ||
push_service_notify_cb | notify_callback, | ||
void * | user_data, | ||
push_service_connection_h * | connection | ||
) |
Connects to the push service and sets callback functions.
- Since :
- 2.3.1
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/push
- Remarks:
- If there is a connection between an application and the push service,
the notify callback passes the notification upon its arrival.
Otherwise, the push service posts a UI notification to alert users.
The connection should be freed with push_service_disconnect() by you.
- Parameters:
-
[in] push_app_id App id received from Tizen Push Server team [in] state_callback State callback function [in] notify_callback Notify callback function [in] user_data User data to pass to state_cb and notify_cb [out] connection The connection handle to the push service
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_OUT_OF_MEMORY Out of memory PUSH_SERVICE_ERROR_NOT_CONNECTED Connection to the daemon failed PUSH_SERVICE_ERROR_PERMISSION_DENIED No push privilege PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
- Precondition:
- There is no connection to the push service for the app_id.
- Postcondition:
- The state callback will be called to let you know the current registration state immediately.
- See also:
- push_service_disconnect()
int push_service_deregister | ( | push_service_connection_h | connection, |
push_service_result_cb | result_callback, | ||
void * | user_data | ||
) |
Deregisters an application from the Push server.
- Since :
- 2.3.1
- Parameters:
-
[in] connection The connection handle to the push service [in] result_callback Result callback function [in] user_data User data to pass to result_cb
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_OUT_OF_MEMORY Out of memory PUSH_SERVICE_ERROR_NOT_CONNECTED No connection to the push service PUSH_SERVICE_ERROR_OPERATION_FAILED Operation failed PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
- Precondition:
- The application should be connected to the push service.
- Postcondition:
- As a result, the state callback will be invoked.
- See also:
- push_service_register()
void push_service_disconnect | ( | push_service_connection_h | connection | ) |
. Closes the connection and releases all its resources
- Since :
- 2.3.1
- Remarks:
- If you call this function in the push callback functions,
it may cause your application to crash.
The specific error code can be obtained using the get_last_result()
Error codes are described in the exception section.
- Parameters:
-
[in] connection The connection handle to the push service
- Exceptions:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
- See also:
- push_service_connect()
Frees the notification handle.
- Since :
- 2.3.1
- Remarks:
- The specific error code can be obtained using the get_last_result()
Error codes are described in the exception section.
- Parameters:
-
[in] noti The notification handle
- Exceptions:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
int push_service_get_notification_data | ( | push_service_notification_h | notification, |
char ** | data | ||
) |
Gets notification data sent by the server.
- Since :
- 2.3.1
- Remarks:
- You must release data using free().
- Parameters:
-
[in] notification The notification handle [out] data The notification data
Set NULL if error but PUSH_SERVICE_ERROR_INVALID_PARAMETER
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_OUT_OF_MEMORY Out of memory PUSH_SERVICE_ERROR_NO_DATA No data available PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
int push_service_get_notification_message | ( | push_service_notification_h | notification, |
char ** | msg | ||
) |
Gets the notification message sent by the server.
- Since :
- 2.3.1
- Remarks:
- The msg must be released with free().
push_service_request_unread_notification() is
preferred to this API.
- Parameters:
-
[in] notification The notification handle [out] msg The notification message
Set NULL if error but PUSH_SERVICE_ERROR_INVALID_PARAMETER
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_OUT_OF_MEMORY Out of memory PUSH_SERVICE_ERROR_NO_DATA No data available PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
int push_service_get_notification_request_id | ( | push_service_notification_h | notification, |
char ** | request_id | ||
) |
Gets the request ID assigned by the sender.
- Since :
- 2.3.1
- Remarks:
- You must release request_id using free().
- Parameters:
-
[in] notification The notification handle [out] request_id The request ID
SetNULL
if error but PUSH_SERVICE_ERROR_INVALID_PARAMETER
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_OUT_OF_MEMORY Out of memory PUSH_SERVICE_ERROR_NO_DATA No data available PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
int push_service_get_notification_sender | ( | push_service_notification_h | notification, |
char ** | sender | ||
) |
Gets the sender of the notification.
- Since :
- 2.3.1
- Remarks:
- You must release sender using free().
- Parameters:
-
[in] notification The notification handle [out] sender The sender
SetNULL
if error but PUSH_SERVICE_ERROR_INVALID_PARAMETER
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_OUT_OF_MEMORY Out of memory PUSH_SERVICE_ERROR_NO_DATA No data available PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
int push_service_get_notification_session_info | ( | push_service_notification_h | notification, |
char ** | session_info | ||
) |
Gets the session ID of the notification.
- Since :
- 2.3.1
- Remarks:
- You must release session_info using free().
- Parameters:
-
[in] notification The notification handle [out] session_info The session ID
SetNULL
if error but PUSH_SERVICE_ERROR_INVALID_PARAMETER
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_OUT_OF_MEMORY Out of memory PUSH_SERVICE_ERROR_NO_DATA No data available PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
int push_service_get_notification_time | ( | push_service_notification_h | notification, |
long long int * | received_time | ||
) |
Gets the received time of the notification message.
- Since :
- 2.3.1
- Parameters:
-
[in] notification The notification handle [out] received_time The received time of the notification message
The received_time is based on UTC.
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_NO_DATA No data available PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
int push_service_get_notification_type | ( | push_service_notification_h | notification, |
int * | type | ||
) |
. Gets the value in the type field of the notification
- Since :
- 2.3.1
- Parameters:
-
[in] notification The notification handle [out] type The type value assigned by the sender
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_NO_DATA No data available PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
int push_service_get_registration_id | ( | push_service_connection_h | connection, |
char ** | reg_id | ||
) |
Gets the registration ID in the PUSH_SERVICE_STATE_REGISTERED state.
- Since :
- 2.3.1
- Remarks:
- You must release reg_id using free().
- Parameters:
-
[in] connection The connection handle to the push service [out] reg_id The registration ID
Set NULL if error but PUSH_SERVICE_ERROR_INVALID_PARAMETER
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_OUT_OF_MEMORY Out of memory PUSH_SERVICE_ERROR_NO_DATA No registration ID available PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
int push_service_get_unread_notification | ( | push_service_connection_h | connection, |
push_service_notification_h * | noti | ||
) |
Gets an unread notification message from the push server.
If an application receives an unread message with this method, the message is removed from the system.
This method can be called repeatedly until it returns PUSH_SERVICE_ERROR_NO_DATA
But, this method does NOT guarantee order and reliability of notification messages.
Some notification messages can be dropped when the system message queue is full.
- Since :
- 2.3.1
- Remarks:
- It is recommended to use push_service_request_unread_notification().
- You must release noti using push_service_free_notification().
- Parameters:
-
[in] connection The connection handle to the push service [out] noti The notification handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_OUT_OF_MEMORY Out of memory PUSH_SERVICE_ERROR_NO_DATA No data available PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
int push_service_register | ( | push_service_connection_h | connection, |
push_service_result_cb | result_callback, | ||
void * | user_data | ||
) |
Registers an application to the push server.
- Since :
- 2.3.1
- Parameters:
-
[in] connection The connection handle to the push service [in] result_callback Result callback function [in] user_data User data to pass to result_cb
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_OUT_OF_MEMORY Out of memory PUSH_SERVICE_ERROR_NOT_CONNECTED No connection to the push service PUSH_SERVICE_ERROR_OPERATION_FAILED Operation failed PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature
- Precondition:
- The application should be connected to the push service.
- Postcondition:
- As a result, the state callback will be invoked.
- See also:
- push_service_deregister()
int push_service_request_unread_notification | ( | push_service_connection_h | connection | ) |
Requests unread notification messages to the push server.
When the app wants to receive messages that arrived before it launched, this
method should be called. Upon receiving this request, the daemon sends messages
stored in its DB to the app. The notify_callback() method assigned in push_service_connect()
will be called when these messages arrive. No need to call this method multiple
times to receive multiple messages. This method does NOT guarantee order and
reliability of notification messages.
- Since :
- 2.3.1
- Remarks:
- This method is preferred to push_service_get_unread_notification().
- Parameters:
-
[in] connection The connection handle to the push service
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PUSH_SERVICE_ERROR_NONE Successful PUSH_SERVICE_ERROR_INVALID_PARAMETER Invalid parameter PUSH_SERVICE_ERROR_NOT_CONNECTED Not connected to the daemon PUSH_SERVICE_ERROR_OPERATION_FAILED Error when sending the request PUSH_SERVICE_ERROR_NOT_SUPPORTED Not supported feature