Tizen Native API
7.0
|
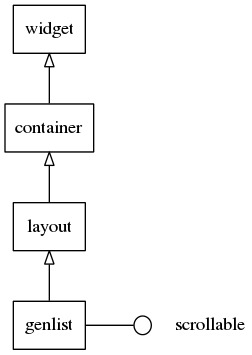
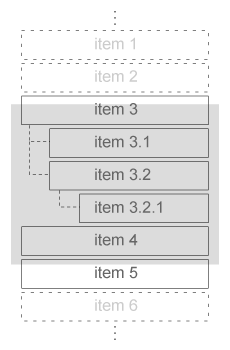
This widget aims to have more expansive list than the simple list in Elementary that could have more flexible items and allow many more entries while still being fast and low on memory usage. At the same time it was also made to be able to do tree structures. But the price to pay is more complexity when it comes to usage. If all you want is a simple list with icons and a single text, use the normal List object.
Genlist has a fairly large API, mostly because it's relatively complex, trying to be both expansive, powerful and efficient. First we will begin an overview on the theory behind genlist.
This widget inherits from the Layout one, so that all the functions acting on it also work for genlist objects.
This widget implements the elm-scrollable-interface interface, so that all (non-deprecated) functions for the base Scroller widget also work for genlists.
Some calls on the genlist's API are marked as deprecated, as they just wrap the scrollable widgets counterpart functions. Use the ones we point you to, for each case of deprecation here, instead -- eventually the deprecated ones will be discarded (next major release).
Genlist item classes - creating items
In order to have the ability to add and delete items on the fly, genlist implements a class (callback) system where the application provides a structure with information about that type of item (genlist may contain multiple different items with different classes, states and styles). Genlist will call the functions in this struct (methods) when an item is "realized" (i.e., created dynamically, while the user is scrolling the list). All objects will simply be deleted when no longer needed with evas_object_del(). The #Elm_Genlist_Item_Class structure contains the following members:
item_style
- This is a constant string and simply defines the name of the item style. It must be specified and the default should be"default"
.decorate_item_style
- This is a constant string and simply defines the name of the decorate mode item style. It is used to specify decorate mode item style. It can be used when you call elm_genlist_item_decorate_mode_set().decorate_all_item_style
- This is a constant string and simply defines the name of the decorate all item style. It is used to specify decorate all item style. It can be used to set selection, checking and deletion mode. This is used when you call elm_genlist_decorate_mode_set().func
- A struct with pointers to functions that will be called when an item is going to be actually created. All of them receive adata
parameter that will point to the same data passed to elm_genlist_item_append() and related item creation functions, and anobj
parameter that points to the genlist object itself.
The function pointers inside func
are text_get
, content_get
, state_get
and del
. The 3 first functions also receive a part
parameter described below. A brief description of these functions follows:
text_get
- Thepart
parameter is the name string of one of the existing text parts in the Edje group implementing the item's theme. This function must return a strdup'()ed string, as the caller will free() it when done. See #Elm_Genlist_Item_Text_Get_Cb.content_get
- Thepart
parameter is the name string of one of the existing (content) swallow parts in the Edje group implementing the item's theme. It must returnNULL
, when no content is desired, or a valid object handle, otherwise. The object will be deleted by the genlist on its deletion or when the item is "unrealized". See #Elm_Genlist_Item_Content_Get_Cb.func.state_get
- Thepart
parameter is the name string of one of the state parts in the Edje group implementing the item's theme. ReturnEINA_FALSE
for false/off orEINA_TRUE
for true/on. Genlists will emit a signal to its theming Edje object with"elm,state,xxx,active"
and"elm"
as "emission" and "source" arguments, respectively, when the state is true (the default is false), wherexxx
is the name of the (state) part. See #Elm_Genlist_Item_State_Get_Cb.func.del
- This is intended for use when genlist items are deleted, so any data attached to the item (e.g. its data parameter on creation) can be deleted. See #Elm_Genlist_Item_Del_Cb.
available item styles:
- default
- default_style - The text part is a textblock
- double_label
- icon_top_text_bottom
- group_index
- one_icon - Only 1 icon (left) (since 1.7)
- end_icon - Only 1 icon (at end/right) (since 1.7)
- no_icon - No icon (at end/right) (since 1.7)
- full - Only 1 icon, elm.swallow.content, which consumes whole area of genlist item (since 1.7)
If one wants to use more icons and texts than are offered in theme, there are two solutions. One is to use 'full' style that has one big swallow part. You can swallow anything there. The other solution is to customize genlist item style in application side by using elm_theme_extension_add() and its own edc. Please refer Theme - Using extensions for that.
Structure of items
An item in a genlist can have 0 or more texts (they can be regular text or textblock Evas objects - that's up to the style to determine), 0 or more contents (which are simply objects swallowed into the genlist item's theming Edje object) and 0 or more boolean states, which have the behavior left to the user to define. The Edje part names for each of these properties will be looked up, in the theme file for the genlist, under the Edje (string) data items named "labels"
, "contents"
and "states"
, respectively. For each of those properties, if more than one part is provided, they must have names listed separated by spaces in the data fields. For the default genlist item theme, we have one text part ("elm.text"
), two content parts ("elm.swallow.icon"
and "elm.swallow.end"
) and no state parts.
A genlist item may be at one of several styles. Elementary provides one by default - "default", but this can be extended by system or application custom themes/overlays/extensions (see themes for more details).
Editing and Navigating
Items can be added by several calls. All of them return a Elm_Object_Item handle that is an internal member inside the genlist. They all take a data parameter that is meant to be used for a handle to the applications internal data (eg. the struct with the original item data). The parent parameter is the parent genlist item this belongs to if it is a tree or an indexed group, and NULL if there is no parent. The flags can be a bitmask of ELM_GENLIST_ITEM_NONE, ELM_GENLIST_ITEM_TREE and ELM_GENLIST_ITEM_GROUP. If ELM_GENLIST_ITEM_TREE is set then this item is displayed as an item that is able to expand and have child items. If ELM_GENLIST_ITEM_GROUP is set then this item is group index item that is displayed at the top until the next group comes. The func parameter is a convenience callback that is called when the item is selected and the data parameter will be the func_data parameter, obj
be the genlist object and event_info will be the genlist item.
elm_genlist_item_append() adds an item to the end of the list, or if there is a parent, to the end of all the child items of the parent. elm_genlist_item_prepend() is the same but adds to the beginning of the list or children list. elm_genlist_item_insert_before() inserts at item before another item and elm_genlist_item_insert_after() inserts after the indicated item.
The application can clear the list with elm_genlist_clear() which deletes all the items in the list and elm_object_item_del() will delete a specific item. elm_genlist_item_subitems_clear() will clear all items that are children of the indicated parent item.
To help inspect list items you can jump to the item at the top of the list with elm_genlist_first_item_get() which will return the item pointer, and similarly elm_genlist_last_item_get() gets the item at the end of the list. elm_genlist_item_next_get() and elm_genlist_item_prev_get() get the next and previous items respectively relative to the indicated item. Using these calls you can walk the entire item list/tree. Note that as a tree the items are flattened in the list, so elm_genlist_item_parent_get() will let you know which item is the parent (and thus know how to skip them if wanted).
Multi-selection
If the application wants multiple items to be able to be selected, elm_genlist_multi_select_set() can enable this. If the list is single-selection only (the default), then elm_genlist_selected_item_get() will return the selected item, if any, or NULL if none is selected. If the list is multi-select then elm_genlist_selected_items_get() will return a list (that is only valid as long as no items are modified (added, deleted, selected or unselected)).
Usage hints
There are also convenience functions. elm_object_item_widget_get() will return the genlist object the item belongs to. elm_genlist_item_show() will make the scroller scroll to show that specific item so its visible. elm_object_item_data_get() returns the data pointer set by the item creation functions.
If an item changes (state of boolean changes, text or contents change), then use elm_genlist_item_update() to have genlist update the item with the new state. Genlist will re-realize the item and thus call the functions in the _Elm_Genlist_Item_Class for that item.
To programmatically (un)select an item use elm_genlist_item_selected_set(). To get its selected state use elm_genlist_item_selected_get(). Similarly to expand/contract an item and get its expanded state, use elm_genlist_item_expanded_set() and elm_genlist_item_expanded_get(). And again to make an item disabled (unable to be selected and appear differently) use elm_object_item_disabled_set() to set this and elm_object_item_disabled_get() to get the disabled state.
In general to indicate how the genlist should expand items horizontally to fill the list area, use elm_genlist_mode_set(). Valid modes are ELM_LIST_LIMIT, ELM_LIST_COMPRESS and ELM_LIST_SCROLL. The default is ELM_LIST_SCROLL. This mode means that if items are too wide to fit, the scroller will scroll horizontally. Otherwise items are expanded to fill the width of the viewport of the scroller. If it is ELM_LIST_LIMIT, items will be expanded to the viewport width if larger than the item, but genlist widget width is limited to the largest item. Do not use ELM_LIST_LIMIT mode with homogeneous mode turned on. ELM_LIST_COMPRESS can be combined with a different style that uses edjes' ellipsis feature (cutting text off like this: "tex...").
Items will call their selection func and callback only once when first becoming selected. Any further clicks will do nothing, unless you enable always select with elm_genlist_select_mode_set() as ELM_OBJECT_SELECT_MODE_ALWAYS. This means even if selected, every click will make the selected callbacks be called. elm_genlist_select_mode_set() as ELM_OBJECT_SELECT_MODE_NONE will turn off the ability to select items entirely and they will neither appear selected nor call selected callback functions.
Remember that you can create new styles and add your own theme augmentation per application with elm_theme_extension_add(). If you absolutely must have a specific style that overrides any theme the user or system sets up you can use elm_theme_overlay_add() to add such a file.
Implementation
Evas tracks every object you create. Every time it processes an event (mouse move, down, up etc.) it needs to walk through objects and find out what event that affects. Even worse every time it renders display updates, in order to just calculate what to re-draw, it needs to walk through many many many objects. Thus, the more objects you keep active, the more overhead Evas has in just doing its work. It is advisable to keep your active objects to the minimum working set you need. Also remember that object creation and deletion carries an overhead, so there is a middle-ground, which is not easily determined. But don't keep massive lists of objects you can't see or use. Genlist does this with list objects. It creates and destroys them dynamically as you scroll around. It groups them into blocks so it can determine the visibility etc. of a whole block at once as opposed to having to walk the whole list. This 2-level list allows for very large numbers of items to be in the list (tests have used up to 2,000,000 items). Also genlist employs a queue for adding items. As items may be different sizes, every item added needs to be calculated as to its size and thus this presents a lot of overhead on populating the list, this genlist employs a queue. Any item added is queued and spooled off over time, actually appearing some time later, so if your list has many members you may find it takes a while for them to all appear, with your process consuming a lot of CPU while it is busy spooling.
Genlist also implements a tree structure for items, but it does so with callbacks to the application, with the application filling in tree structures when requested (allowing for efficient building of a very deep tree that could even be used for file-management). See the above smart signal callbacks for details.
Genlist smart events
This widget emits the following signals, besides the ones sent from Layout :
"activated"
- The user has double-clicked or pressed (enter|return|spacebar) on an item. Theevent_info
parameter is the item that was activated."pressed"
- The user pressed an item. Theevent_info
parameter is the item that was pressed."released"
- The user released an item. Theevent_info
parameter is the item that was released."clicked,double"
- The user has double-clicked an item. Theevent_info
parameter is the item that was double-clicked."clicked,right"
- The user has right-clicked an item. Theevent_info
parameter is the item that was right-clicked. (since 1.13)"selected"
- This is called when a user has made an item selected. The event_info parameter is the genlist item that was selected."unselected"
- This is called when a user has made an item unselected. The event_info parameter is the genlist item that was unselected."expanded"
- This is called when elm_genlist_item_expanded_set() is called and the item is now meant to be expanded. The event_info parameter is the genlist item that was indicated to expand. It is the job of this callback to then fill in the child items."contracted"
- This is called when elm_genlist_item_expanded_set() is called and the item is now meant to be contracted. The event_info parameter is the genlist item that was indicated to contract. It is the job of this callback to then delete the child items."expand,request"
- This is called when a user has indicated they want to expand a tree branch item. The callback should decide if the item can expand (has any children) and then call elm_genlist_item_expanded_set() appropriately to set the state. The event_info parameter is the genlist item that was indicated to expand."contract,request"
- This is called when a user has indicated they want to contract a tree branch item. The callback should decide if the item can contract (has any children) and then call elm_genlist_item_expanded_set() appropriately to set the state. The event_info parameter is the genlist item that was indicated to contract."realized"
- This is called when the item in the list is created as a real evas object. event_info parameter is the genlist item that was created."unrealized"
- This is called just before an item is unrealized. After this call content objects provided will be deleted and the item object itself delete or be put into a floating cache."drag,start,up"
- This is called when the item in the list has been dragged (not scrolled) up."drag,start,down"
- This is called when the item in the list has been dragged (not scrolled) down."drag,start,left"
- This is called when the item in the list has been dragged (not scrolled) left."drag,start,right"
- This is called when the item in the list has been dragged (not scrolled) right."drag,stop"
- This is called when the item in the list has stopped being dragged."drag"
- This is called when the item in the list is being dragged."longpressed"
- This is called when the item is pressed for a certain amount of time. By default it's 1 second. The event_info parameter is the longpressed genlist item."scroll"
- the content has been scrolled (moved) (since 1.13)"scroll,anim,start"
- This is called when scrolling animation has started."scroll,anim,stop"
- This is called when scrolling animation has stopped."scroll,drag,start"
- This is called when dragging the content has started."scroll,drag,stop"
- This is called when dragging the content has stopped."edge,top"
- This is called when the genlist is scrolled until the top edge."edge,bottom"
- This is called when the genlist is scrolled until the bottom edge."edge,left"
- This is called when the genlist is scrolled until the left edge."edge,right"
- This is called when the genlist is scrolled until the right edge."multi,swipe,left"
- This is called when the genlist is multi-touch swiped left."multi,swipe,right"
- This is called when the genlist is multi-touch swiped right."multi,swipe,up"
- This is called when the genlist is multi-touch swiped up."multi,swipe,down"
- This is called when the genlist is multi-touch swiped down."multi,pinch,out"
- This is called when the genlist is multi-touch pinched out."multi,pinch,in"
- This is called when the genlist is multi-touch pinched in."swipe"
- This is called when the genlist is swiped."moved"
- This is called when a genlist item is moved by a user interaction in a reorder mode. Theevent_info
parameter is the item that was moved."moved,after"
- This is called when a genlist item is moved after another item in reorder mode. The event_info parameter is the reordered item. To get the relative previous item, use elm_genlist_item_prev_get(). This signal is called along with "moved" signal."moved,before"
- This is called when a genlist item is moved before another item in reorder mode. The event_info parameter is the reordered item. To get the relative previous item, use elm_genlist_item_next_get(). This signal is called along with "moved" signal."index,update"
- This is called when a genlist item index is changed. Note that this callback is called while each item is being realized."language,changed"
- This is called when the program's language is changed. Call the elm_genlist_realized_items_update() if items text should be translated."tree,effect,finished"
- This is called when a genlist tree effect is finished."highlighted"
- an item in the list is highlighted. This is called when the user presses an item or keyboard selection is done so the item is physically highlighted. Theevent_info
parameter is the item that was highlighted."unhighlighted"
- an item in the list is unhighlighted. This is called when the user releases an item or keyboard selection is moved so the item is physically unhighlighted. Theevent_info
parameter is the item that was unhighlighted."focused"
- When the genlist has received focus. (since 1.8)"unfocused"
- When the genlist has lost focus. (since 1.8)"item,focused"
- When the genlist item has received focus. (since 1.10)"item,unfocused"
- When the genlist item has lost focus. (since 1.10)
Supported elm_object_item common APIs
- elm_object_item_part_content_get
- elm_object_item_part_text_get
- elm_object_item_disabled_set
- elm_object_item_disabled_get
- elm_object_item_signal_emit
Unsupported elm_object_item common APIs due to the genlist concept. Genlist fills content/text according to the appropriate callback functions. Please use elm_genlist_item_update() or elm_genlist_item_fields_update() instead.
Examples
Here is a list of examples that use the genlist, trying to show some of its capabilities:
- Genlist - basic usage
- Genlist - list setup functions
- Genlist - different width options
- Genlist - items manipulation
- Genlist - working with subitems
Functions | |
Elm_Genlist_Item_Class * | elm_genlist_item_class_new (void) |
void | elm_genlist_item_class_free (Elm_Genlist_Item_Class *itc) |
void | elm_genlist_item_class_ref (Elm_Genlist_Item_Class *itc) |
void | elm_genlist_item_class_unref (Elm_Genlist_Item_Class *itc) |
void | elm_genlist_item_tooltip_text_set (Elm_Object_Item *it, const char *text) |
void | elm_genlist_item_tooltip_content_cb_set (Elm_Object_Item *it, Elm_Tooltip_Item_Content_Cb func, const void *data, Evas_Smart_Cb del_cb) |
void | elm_genlist_item_tooltip_unset (Elm_Object_Item *it) |
void | elm_genlist_item_tooltip_style_set (Elm_Object_Item *it, const char *style) |
const char * | elm_genlist_item_tooltip_style_get (const Elm_Object_Item *it) |
void | elm_genlist_item_cursor_set (Elm_Object_Item *it, const char *cursor) |
const char * | elm_genlist_item_cursor_get (const Elm_Object_Item *it) |
void | elm_genlist_item_cursor_unset (Elm_Object_Item *it) |
void | elm_genlist_item_cursor_style_set (Elm_Object_Item *it, const char *style) |
const char * | elm_genlist_item_cursor_style_get (const Elm_Object_Item *it) |
void | elm_genlist_item_cursor_engine_only_set (Elm_Object_Item *it, Eina_Bool engine_only) |
Eina_Bool | elm_genlist_item_cursor_engine_only_get (const Elm_Object_Item *it) |
void | elm_genlist_homogeneous_set (Elm_Genlist *obj, Eina_Bool homogeneous) |
Enable/disable homogeneous mode. | |
Eina_Bool | elm_genlist_homogeneous_get (const Elm_Genlist *obj) |
Get whether the homogeneous mode is enabled. | |
void | elm_genlist_select_mode_set (Elm_Genlist *obj, Elm_Object_Select_Mode mode) |
Set the genlist select mode. | |
Elm_Object_Select_Mode | elm_genlist_select_mode_get (const Elm_Genlist *obj) |
Get the genlist select mode. | |
void | elm_genlist_focus_on_selection_set (Elm_Genlist *obj, Eina_Bool enabled) |
Set focus upon items selection mode. | |
Eina_Bool | elm_genlist_focus_on_selection_get (const Elm_Genlist *obj) |
Get whether focus upon item's selection mode is enabled. | |
void | elm_genlist_longpress_timeout_set (Elm_Genlist *obj, double timeout) |
Set the timeout in seconds for the longpress event. | |
double | elm_genlist_longpress_timeout_get (const Elm_Genlist *obj) |
Get the timeout in seconds for the longpress event. | |
void | elm_genlist_multi_select_set (Elm_Genlist *obj, Eina_Bool multi) |
Enable or disable multi-selection in the genlist. | |
Eina_Bool | elm_genlist_multi_select_get (const Elm_Genlist *obj) |
Get if multi-selection in genlist is enabled or disabled. | |
void | elm_genlist_reorder_mode_set (Elm_Genlist *obj, Eina_Bool reorder_mode) |
Set reorder mode. | |
Eina_Bool | elm_genlist_reorder_mode_get (const Elm_Genlist *obj) |
Get the reorder mode. | |
void | elm_genlist_multi_select_mode_set (Elm_Genlist *obj, Elm_Object_Multi_Select_Mode mode) |
Set the genlist multi select mode. | |
Elm_Object_Multi_Select_Mode | elm_genlist_multi_select_mode_get (const Elm_Genlist *obj) |
Get the genlist multi select mode. | |
void | elm_genlist_block_count_set (Elm_Genlist *obj, int count) |
Set the maximum number of items within an item block. | |
int | elm_genlist_block_count_get (const Elm_Genlist *obj) |
Get the maximum number of items within an item block. | |
void | elm_genlist_tree_effect_enabled_set (Elm_Genlist *obj, Eina_Bool enabled) |
Control genlist tree effect. | |
Eina_Bool | elm_genlist_tree_effect_enabled_get (const Elm_Genlist *obj) |
Control genlist tree effect. | |
void | elm_genlist_highlight_mode_set (Elm_Genlist *obj, Eina_Bool highlight) |
Set whether the genlist items should be highlighted on item selection. | |
Eina_Bool | elm_genlist_highlight_mode_get (const Elm_Genlist *obj) |
Get whether the genlist items' should be highlighted when item selected. | |
void | elm_genlist_mode_set (Elm_Genlist *obj, Elm_List_Mode mode) |
This sets the horizontal stretching mode. | |
Elm_List_Mode | elm_genlist_mode_get (const Elm_Genlist *obj) |
Get the horizontal stretching mode. | |
Elm_Widget_Item * | elm_genlist_selected_item_get (const Elm_Genlist *obj) |
Get the selected item in the genlist. | |
Elm_Widget_Item * | elm_genlist_first_item_get (const Elm_Genlist *obj) |
Get the first item in the genlist. | |
Eina_List * | elm_genlist_realized_items_get (const Elm_Genlist *obj) |
Get a list of realized items in genlist. | |
const Eina_List * | elm_genlist_selected_items_get (const Elm_Genlist *obj) |
Get a list of selected items in the genlist. | |
Elm_Widget_Item * | elm_genlist_last_item_get (const Elm_Genlist *obj) |
Get the last item in the genlist. | |
Elm_Widget_Item * | elm_genlist_item_insert_before (Elm_Genlist *obj, const Elm_Genlist_Item_Class *itc, const void *data, Elm_Widget_Item *parent, Elm_Widget_Item *before_it, Elm_Genlist_Item_Type type, Evas_Smart_Cb func, const void *func_data) |
Insert an item before another in a genlist widget. | |
void | elm_genlist_realized_items_update (Elm_Genlist *obj) |
Update the contents of all realized items. | |
Elm_Widget_Item * | elm_genlist_item_insert_after (Elm_Genlist *obj, const Elm_Genlist_Item_Class *itc, const void *data, Elm_Widget_Item *parent, Elm_Widget_Item *after_it, Elm_Genlist_Item_Type type, Evas_Smart_Cb func, const void *func_data) |
Insert an item after another in a genlist widget. | |
Elm_Widget_Item * | elm_genlist_at_xy_item_get (const Elm_Genlist *obj, int x, int y, int *posret) |
Get the item that is at the x, y canvas coords. | |
void | elm_genlist_filter_set (Elm_Genlist *obj, void *key) |
Set filter mode with key. | |
Eina_Iterator * | elm_genlist_filter_iterator_new (Elm_Genlist *obj) |
Returns an iterator over the list of filtered items. | |
unsigned int | elm_genlist_filtered_items_count (const Elm_Genlist *obj) |
Return how many items have passed the filter currently. | |
unsigned int | elm_genlist_items_count (const Elm_Genlist *obj) |
Return how many items are currently in a list. | |
Elm_Widget_Item * | elm_genlist_item_prepend (Elm_Genlist *obj, const Elm_Genlist_Item_Class *itc, const void *data, Elm_Widget_Item *parent, Elm_Genlist_Item_Type type, Evas_Smart_Cb func, const void *func_data) |
Prepend a new item in a given genlist widget. | |
void | elm_genlist_clear (Elm_Genlist *obj) |
Remove all items from a given genlist widget. | |
Elm_Widget_Item * | elm_genlist_item_append (Elm_Genlist *obj, const Elm_Genlist_Item_Class *itc, const void *data, Elm_Widget_Item *parent, Elm_Genlist_Item_Type type, Evas_Smart_Cb func, const void *func_data) |
Append a new item in a given genlist widget. | |
Elm_Widget_Item * | elm_genlist_item_sorted_insert (Elm_Genlist *obj, const Elm_Genlist_Item_Class *itc, const void *data, Elm_Widget_Item *parent, Elm_Genlist_Item_Type type, Eina_Compare_Cb comp, Evas_Smart_Cb func, const void *func_data) |
Insert a new item into the sorted genlist object. | |
Elm_Widget_Item * | elm_genlist_search_by_text_item_get (Elm_Genlist *obj, Elm_Widget_Item *item_to_search_from, const char *part_name, const char *pattern, Elm_Glob_Match_Flags flags) |
Get genlist item by given string. | |
Evas_Object * | elm_genlist_add (Evas_Object *parent) |
Elm_Object_Item * | elm_genlist_nth_item_get (const Evas_Object *obj, unsigned int nth) |
Function Documentation
Evas_Object* elm_genlist_add | ( | Evas_Object * | parent | ) |
Add a new genlist widget to the given parent Elementary (container) object
- Parameters:
-
parent The parent object
- Returns:
- a new genlist widget handle or
NULL
, on errors
This function inserts a new genlist widget on the canvas.
- Since :
- 2.3.1
Elm_Widget_Item* elm_genlist_at_xy_item_get | ( | const Elm_Genlist * | obj, |
int | x, | ||
int | y, | ||
int * | posret | ||
) |
Get the item that is at the x, y canvas coords.
This returns the item at the given coordinates (which are canvas relative, not object-relative). If an item is at that coordinate, that item handle is returned, and if posret
is not NULL, the integer pointed to is set to a value of -1, 0 or 1, depending if the coordinate is on the upper portion of that item (-1), on the middle section (0) or on the lower part (1). If NULL is returned as an item (no item found there), then posret may indicate -1 or 1 based if the coordinate is above or below all items respectively in the genlist.
- Parameters:
-
[in] obj The object. [in] x The input x coordinate. [in] y The input y coordinate. [out] posret The position relative to the item returned here.
- Returns:
- Item at position
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
int elm_genlist_block_count_get | ( | const Elm_Genlist * | obj | ) |
Get the maximum number of items within an item block.
- Parameters:
-
[in] obj The object.
- Returns:
- Maximum number of items within an item block. Default is 32.
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
void elm_genlist_block_count_set | ( | Elm_Genlist * | obj, |
int | count | ||
) |
Set the maximum number of items within an item block.
This will configure the block count to tune to the target with particular performance matrix.
A block of objects will be used to reduce the number of operations due to many objects in the screen. It can determine the visibility, or if the object has changed, it theme needs to be updated, etc. doing this kind of calculation to the entire block, instead of per object.
The default value for the block count is enough for most lists, so unless you know you will have a lot of objects visible in the screen at the same time, don't try to change this.
- Parameters:
-
[in] obj The object. [in] count Maximum number of items within an item block. Default is 32.
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
void elm_genlist_clear | ( | Elm_Genlist * | obj | ) |
Remove all items from a given genlist widget.
This removes (and deletes) all items in obj
, leaving it empty.
- Parameters:
-
[in] obj The object.
- Since :
- 2.3.1
Eina_Iterator* elm_genlist_filter_iterator_new | ( | Elm_Genlist * | obj | ) |
Returns an iterator over the list of filtered items.
Return NULL if filter is not set. Application must take care of the case while calling the API. Must be freed after use.
- Parameters:
-
[in] obj The object.
- Returns:
- Iterator on genlist
void elm_genlist_filter_set | ( | Elm_Genlist * | obj, |
void * | key | ||
) |
Set filter mode with key.
This initiates the filter mode of genlist with user/application provided key. If key is NULL, the filter mode is turned off.
The filter data passed has to be managed by application itself and should not be deleted before genlist is deleted(or while filtering is not done.
- Parameters:
-
[in] obj The object. [in] key Filter key
unsigned int elm_genlist_filtered_items_count | ( | const Elm_Genlist * | obj | ) |
Return how many items have passed the filter currently.
This behaviour is O(1) and returns the count of items which are currently passed by the filter. After "filter,done", the call returns total count of the filtered items.
- Parameters:
-
[in] obj The object.
- Returns:
- Count of items passing the filter
- Since (EFL) :
- 1.18
Elm_Widget_Item* elm_genlist_first_item_get | ( | const Elm_Genlist * | obj | ) |
Get the first item in the genlist.
This returns the first item in the list.
If filter is set on genlist, it returns the first filtered item in the list.
- Parameters:
-
[in] obj The object.
- Returns:
- The first item or
null
.
- Since :
- 2.3.1
- Examples:
- genlist_example_04.c.
Eina_Bool elm_genlist_focus_on_selection_get | ( | const Elm_Genlist * | obj | ) |
Get whether focus upon item's selection mode is enabled.
- Parameters:
-
[in] obj The object.
- Returns:
- The tree effect status.
void elm_genlist_focus_on_selection_set | ( | Elm_Genlist * | obj, |
Eina_Bool | enabled | ||
) |
Set focus upon items selection mode.
When enabled, every selection of an item inside the genlist will automatically set focus to its first focusable widget from the left. This is true of course if the selection was made by clicking an unfocusable area in an item or selecting it with a key movement. Clicking on a focusable widget inside an item will cause this particular item to get focus as usual.
- Parameters:
-
[in] obj The object. [in] enabled The tree effect status.
Eina_Bool elm_genlist_highlight_mode_get | ( | const Elm_Genlist * | obj | ) |
Get whether the genlist items' should be highlighted when item selected.
- Parameters:
-
[in] obj The object.
- Returns:
true
to enable highlighting orfalse
to disable it.
- Since :
- 2.3.1
void elm_genlist_highlight_mode_set | ( | Elm_Genlist * | obj, |
Eina_Bool | highlight | ||
) |
Set whether the genlist items should be highlighted on item selection.
This will turn on/off the highlight effect on item selection. The selected and clicked callback functions will still be called.
Highlight is enabled by default.
- Parameters:
-
[in] obj The object. [in] highlight true
to enable highlighting orfalse
to disable it.
- Since :
- 2.3.1
Eina_Bool elm_genlist_homogeneous_get | ( | const Elm_Genlist * | obj | ) |
Get whether the homogeneous mode is enabled.
- Parameters:
-
[in] obj The object.
- Returns:
- Assume the items within the genlist are of the same height and width. Default is
false
.
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
void elm_genlist_homogeneous_set | ( | Elm_Genlist * | obj, |
Eina_Bool | homogeneous | ||
) |
Enable/disable homogeneous mode.
This will enable the homogeneous mode where items are of the same height and width so that genlist may do the lazy-loading at its maximum (which increases the performance for scrolling the list). In the normal mode, genlist will pre-calculate all the items' sizes even though they are not in use. So items' callbacks are called many times than expected. But homogeneous mode will skip the item size pre-calculation process so items' callbacks are called only when the item is needed.
- Note:
- This also works well with group index.
- Parameters:
-
[in] obj The object. [in] homogeneous Assume the items within the genlist are of the same height and width. Default is false
.
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
Elm_Widget_Item* elm_genlist_item_append | ( | Elm_Genlist * | obj, |
const Elm_Genlist_Item_Class * | itc, | ||
const void * | data, | ||
Elm_Widget_Item * | parent, | ||
Elm_Genlist_Item_Type | type, | ||
Evas_Smart_Cb | func, | ||
const void * | func_data | ||
) |
Append a new item in a given genlist widget.
This adds the given item to the end of the list or the end of the children list if the parent
is given.
- Parameters:
-
[in] obj The object. [in] itc The item class for the item. [in] data The item data. [in] parent The parent item, or null
if none.[in] type Item type. [in] func Convenience function called when the item is selected. [in] func_data Data passed to func
above.
- Returns:
- Handle to appended item
- Since :
- 2.3.1
void elm_genlist_item_class_free | ( | Elm_Genlist_Item_Class * | itc | ) |
Remove an item class in a given genlist widget.
- Parameters:
-
itc The itc to be removed.
This removes item class from the genlist widget. Whenever it has no more references to it, item class is going to be freed. Otherwise it just decreases its reference count.
- Since :
- 2.3.1
Create a new genlist item class in a given genlist widget.
- Returns:
- New allocated genlist item class.
This adds genlist item class for the genlist widget. When adding an item, genlist_item_{append, prepend, insert} function needs item class of the item. Given callback parameters are used at retrieving {text, content} of added item. Set as NULL if it's not used. If there's no available memory, return can be NULL.
- Since :
- 2.3.1
void elm_genlist_item_class_ref | ( | Elm_Genlist_Item_Class * | itc | ) |
Increments object reference count for the item class.
- Parameters:
-
itc The given item class object to reference
This API just increases its reference count for item class management.
- See also:
- elm_genlist_item_class_unref()
- Since :
- 2.3.1
void elm_genlist_item_class_unref | ( | Elm_Genlist_Item_Class * | itc | ) |
Decrements object reference count for the item class.
- Parameters:
-
itc The given item class object to reference
This API just decreases its reference count for item class management. Reference count can't be less than 0.
- Since :
- 2.3.1
Eina_Bool elm_genlist_item_cursor_engine_only_get | ( | const Elm_Object_Item * | it | ) |
Get if the (custom) cursor for a given genlist item is being searched in its theme, also, or is only relying on the rendering engine.
- Parameters:
-
it a genlist item
- Returns:
EINA_TRUE
, if cursors are being looked for only on those provided by the rendering engine,EINA_FALSE
if they are being searched on the widget's theme, as well.
- See also:
- elm_genlist_item_cursor_engine_only_set(), for more details
- Since :
- 2.3.1
void elm_genlist_item_cursor_engine_only_set | ( | Elm_Object_Item * | it, |
Eina_Bool | engine_only | ||
) |
Set if the (custom) cursor for a given genlist item should be searched in its theme, also, or should only rely on the rendering engine.
- Parameters:
-
it item with custom (custom) cursor already set on engine_only Use EINA_TRUE
to have cursors looked for only on those provided by the rendering engine,EINA_FALSE
to have them searched on the widget's theme, as well.
- Note:
- This call is of use only if you've set a custom cursor for genlist items, with elm_genlist_item_cursor_set().
- By default, cursors will only be looked for between those provided by the rendering engine.
- Since :
- 2.3.1
const char* elm_genlist_item_cursor_get | ( | const Elm_Object_Item * | it | ) |
Get the type of mouse pointer/cursor decoration set to be shown, when the mouse pointer is over the given genlist widget item
- Parameters:
-
it genlist item with custom cursor set
- Returns:
- the cursor type's name or
NULL
, if no custom cursors were set toitem
(and on errors)
- See also:
- elm_object_cursor_get()
- elm_genlist_item_cursor_set() for more details
- elm_genlist_item_cursor_unset()
- Since :
- 2.3.1
void elm_genlist_item_cursor_set | ( | Elm_Object_Item * | it, |
const char * | cursor | ||
) |
Set the type of mouse pointer/cursor decoration to be shown, when the mouse pointer is over the given genlist widget item
- Parameters:
-
it genlist item to customize cursor on cursor the cursor type's name
This function works analogously as elm_object_cursor_set(), but here the cursor's changing area is restricted to the item's area, and not the whole widget's. Note that that item cursors have precedence over widget cursors, so that a mouse over item
will always show cursor type
.
If this function is called twice for an object, a previously set cursor will be unset on the second call.
- Since :
- 2.3.1
const char* elm_genlist_item_cursor_style_get | ( | const Elm_Object_Item * | it | ) |
Get the current style set for a given genlist item's custom cursor
- Parameters:
-
it genlist item with custom cursor set.
- Returns:
- style the cursor style in use. If the object does not have a cursor set, then
NULL
is returned.
- See also:
- elm_genlist_item_cursor_style_set() for more details
- Since :
- 2.3.1
void elm_genlist_item_cursor_style_set | ( | Elm_Object_Item * | it, |
const char * | style | ||
) |
Set a different style for a given custom cursor set for a genlist item.
- Parameters:
-
it genlist item with custom cursor set style the theme style to use (e.g. "default"
,"transparent"
, etc)
This function only makes sense when one is using custom mouse cursor decorations defined in a theme file , which can have, given a cursor name/type, alternate styles on it. It works analogously as elm_object_cursor_style_set(), but here applied only to genlist item objects.
- Warning:
- Before you set a cursor style you should have defined a custom cursor previously on the item, with elm_genlist_item_cursor_set()
- Since :
- 2.3.1
void elm_genlist_item_cursor_unset | ( | Elm_Object_Item * | it | ) |
Unset any custom mouse pointer/cursor decoration set to be shown, when the mouse pointer is over the given genlist widget item, thus making it show the default cursor again.
- Parameters:
-
it a genlist item
Use this call to undo any custom settings on this item's cursor decoration, bringing it back to defaults (no custom style set).
- See also:
- elm_object_cursor_unset()
- elm_genlist_item_cursor_set() for more details
- Since :
- 2.3.1
Elm_Widget_Item* elm_genlist_item_insert_after | ( | Elm_Genlist * | obj, |
const Elm_Genlist_Item_Class * | itc, | ||
const void * | data, | ||
Elm_Widget_Item * | parent, | ||
Elm_Widget_Item * | after_it, | ||
Elm_Genlist_Item_Type | type, | ||
Evas_Smart_Cb | func, | ||
const void * | func_data | ||
) |
Insert an item after another in a genlist widget.
This inserts an item after another in the list. It will be in the same tree level or group as the item it is inserted after.
- Parameters:
-
[in] obj The object. [in] itc The item class for the item. [in] data The item data. [in] parent The parent item, or null
if none.[in] after_it The item to place this new one after. [in] type Item type. [in] func Convenience function called when the item is selected. [in] func_data Data passed to func
above.
- Returns:
- Handle to inserted item
- Since :
- 2.3.1
- Examples:
- genlist_example_04.c, and genlist_example_05.c.
Elm_Widget_Item* elm_genlist_item_insert_before | ( | Elm_Genlist * | obj, |
const Elm_Genlist_Item_Class * | itc, | ||
const void * | data, | ||
Elm_Widget_Item * | parent, | ||
Elm_Widget_Item * | before_it, | ||
Elm_Genlist_Item_Type | type, | ||
Evas_Smart_Cb | func, | ||
const void * | func_data | ||
) |
Insert an item before another in a genlist widget.
This inserts an item before another in the list. It will be in the same tree level or group as the item it is inserted before.
- Parameters:
-
[in] obj The object. [in] itc The item class for the item. [in] data The item data. [in] parent The parent item, or null
if none.[in] before_it The item to place this new one before. [in] type Item type. [in] func Convenience function called when the item is selected. [in] func_data Data passed to func
above.
- Returns:
- Handle to inserted item
- Since :
- 2.3.1
- Examples:
- genlist_example_04.c.
Elm_Widget_Item* elm_genlist_item_prepend | ( | Elm_Genlist * | obj, |
const Elm_Genlist_Item_Class * | itc, | ||
const void * | data, | ||
Elm_Widget_Item * | parent, | ||
Elm_Genlist_Item_Type | type, | ||
Evas_Smart_Cb | func, | ||
const void * | func_data | ||
) |
Prepend a new item in a given genlist widget.
This adds an item to the beginning of the list or beginning of the children of the parent if given.
- Parameters:
-
[in] obj The object. [in] itc The item class for the item. [in] data The item data. [in] parent The parent item, or null
if none.[in] type Item type. [in] func Convenience function called when the item is selected. [in] func_data Data passed to func
above.
- Returns:
- Handle to prepended item
- Since :
- 2.3.1
- Examples:
- genlist_example_04.c, and genlist_example_05.c.
Elm_Widget_Item* elm_genlist_item_sorted_insert | ( | Elm_Genlist * | obj, |
const Elm_Genlist_Item_Class * | itc, | ||
const void * | data, | ||
Elm_Widget_Item * | parent, | ||
Elm_Genlist_Item_Type | type, | ||
Eina_Compare_Cb | comp, | ||
Evas_Smart_Cb | func, | ||
const void * | func_data | ||
) |
Insert a new item into the sorted genlist object.
This inserts an item in the genlist based on user defined comparison function. The two arguments passed to the function func
are genlist item handles to compare.
- Parameters:
-
[in] obj The object. [in] itc The item class for the item. [in] data The item data. [in] parent The parent item, or null
if none.[in] type Item type. [in] comp The function called for the sort. [in] func Convenience function called when the item is selected. [in] func_data Data passed to func
above.
- Returns:
- Handle to inserted item
- Since :
- 2.3.1
void elm_genlist_item_tooltip_content_cb_set | ( | Elm_Object_Item * | it, |
Elm_Tooltip_Item_Content_Cb | func, | ||
const void * | data, | ||
Evas_Smart_Cb | del_cb | ||
) |
Set the content to be shown in a given genlist item's tooltips
- Parameters:
-
it The genlist item. func The function returning the tooltip contents. data What to provide to func as callback data/context. del_cb Called when data is not needed anymore, either when another callback replaces func
, the tooltip is unset with elm_genlist_item_tooltip_unset() or the owneritem
dies. This callback receives as its first parameter the givendata
, beingevent_info
the item handle.
This call will setup the tooltip's contents to item
(analogous to elm_object_tooltip_content_cb_set(), but being item tooltips with higher precedence than object tooltips). It can have only one tooltip at a time, so any previous tooltip content will get removed. func
(with data
) will be called every time Elementary needs to show the tooltip and it should return a valid Evas object, which will be fully managed by the tooltip system, getting deleted when the tooltip is gone.
In order to set just a text as a tooltip, look at elm_genlist_item_tooltip_text_set().
- Since :
- N/A
const char* elm_genlist_item_tooltip_style_get | ( | const Elm_Object_Item * | it | ) |
Get the style set a given genlist item's tooltip.
- Parameters:
-
it genlist item with tooltip already set on.
- Returns:
- style the theme style in use, which defaults to "default". If the object does not have a tooltip set, then
NULL
is returned.
- See also:
- elm_genlist_item_tooltip_style_set() for more details
- Since :
- N/A
void elm_genlist_item_tooltip_style_set | ( | Elm_Object_Item * | it, |
const char * | style | ||
) |
Set a different style for a given genlist item's tooltip.
- Parameters:
-
it genlist item with tooltip set style the theme style to use on tooltips (e.g. "default"
,"transparent"
, etc)
Tooltips can have alternate styles to be displayed on, which are defined by the theme set on Elementary. This function works analogously as elm_object_tooltip_style_set(), but here applied only to genlist item objects. The default style for tooltips is "default"
.
- Note:
- before you set a style you should define a tooltip with elm_genlist_item_tooltip_content_cb_set() or elm_genlist_item_tooltip_text_set()
- See also:
- elm_genlist_item_tooltip_style_get()
- Since :
- N/A
void elm_genlist_item_tooltip_text_set | ( | Elm_Object_Item * | it, |
const char * | text | ||
) |
Set the text to be shown in a given genlist item's tooltips.
- Parameters:
-
it The genlist item text The text to set in the content
This call will setup the text to be used as tooltip to that item (analogous to elm_object_tooltip_text_set(), but being item tooltips with higher precedence than object tooltips). It can have only one tooltip at a time, so any previous tooltip data will get removed.
In order to set a content or something else as a tooltip, look at elm_genlist_item_tooltip_content_cb_set().
- Since :
- N/A
void elm_genlist_item_tooltip_unset | ( | Elm_Object_Item * | it | ) |
Unset a tooltip from a given genlist item
- Parameters:
-
it genlist item to remove a previously set tooltip from.
This call removes any tooltip set on item
. The callback provided as del_cb
to elm_genlist_item_tooltip_content_cb_set() will be called to notify it is not used anymore (and have resources cleaned, if need be).
- Since :
- N/A
unsigned int elm_genlist_items_count | ( | const Elm_Genlist * | obj | ) |
Return how many items are currently in a list.
This behavior is O(1) and includes items which may or may not be realized.
- Parameters:
-
[in] obj The object.
- Returns:
- Item in list
- Since :
- 2.3.1
Elm_Widget_Item* elm_genlist_last_item_get | ( | const Elm_Genlist * | obj | ) |
Get the last item in the genlist.
This returns the last item in the list.
If filter is set to genlist, it returns last filtered item in the list.
- Parameters:
-
[in] obj The object.
- Returns:
- Last item in list
- Since :
- 2.3.1
- Examples:
- genlist_example_04.c.
double elm_genlist_longpress_timeout_get | ( | const Elm_Genlist * | obj | ) |
Get the timeout in seconds for the longpress event.
- Parameters:
-
[in] obj The object.
- Returns:
- Timeout in seconds. Default is elm config value (1.0).
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
void elm_genlist_longpress_timeout_set | ( | Elm_Genlist * | obj, |
double | timeout | ||
) |
Set the timeout in seconds for the longpress event.
This option will change how long it takes to send an event "longpressed" after the mouse down signal is sent to the list. If this event occurs, no "clicked" event will be sent.
- Warning:
- If you set the longpress timeout value with this API, your genlist will not be affected by the longpress value of elementary config value later.
- Parameters:
-
[in] obj The object. [in] timeout Timeout in seconds. Default is elm config value (1.0).
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
Elm_List_Mode elm_genlist_mode_get | ( | const Elm_Genlist * | obj | ) |
Get the horizontal stretching mode.
- Parameters:
-
[in] obj The object.
- Returns:
- The mode to use (one of ELM_LIST_SCROLL or ELM_LIST_LIMIT).
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
void elm_genlist_mode_set | ( | Elm_Genlist * | obj, |
Elm_List_Mode | mode | ||
) |
This sets the horizontal stretching mode.
This sets the mode used for sizing items horizontally. Valid modes are ELM_LIST_LIMIT, ELM_LIST_SCROLL, and ELM_LIST_COMPRESS. The default is ELM_LIST_SCROLL. This mode means that if items are too wide to fit, the scroller will scroll horizontally. Otherwise items are expanded to fill the width of the viewport of the scroller. If it is ELM_LIST_LIMIT, items will be expanded to the viewport width and limited to that size. If it is ELM_LIST_COMPRESS, the item width will be fixed (restricted to a minimum of) to the list width when calculating its size in order to allow the height to be calculated based on it. This allows, for instance, text block to wrap lines if the Edje part is configured with "text.min: 0 1".
- Note:
- ELM_LIST_COMPRESS will make list resize slower as it will have to recalculate every item height again whenever the list width changes!
- Homogeneous mode gives all items in the genlist the same width/height. With ELM_LIST_COMPRESS, genlist items initialize fast, but there cannot be any sub-objects in the genlist which require on-the-fly resizing (such as TEXTBLOCK). In this case some dynamic resizable objects in the genlist might not diplay properly.
- Parameters:
-
[in] obj The object. [in] mode The mode to use (one of ELM_LIST_SCROLL or ELM_LIST_LIMIT).
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c, and genlist_example_03.c.
Eina_Bool elm_genlist_multi_select_get | ( | const Elm_Genlist * | obj | ) |
Get if multi-selection in genlist is enabled or disabled.
- Parameters:
-
[in] obj The object.
- Returns:
- Multi-select enable/disable. Default is disabled.
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
Elm_Object_Multi_Select_Mode elm_genlist_multi_select_mode_get | ( | const Elm_Genlist * | obj | ) |
Get the genlist multi select mode.
- Parameters:
-
[in] obj The object.
- Returns:
- The multi select mode
- Since (EFL) :
- 1.8
void elm_genlist_multi_select_mode_set | ( | Elm_Genlist * | obj, |
Elm_Object_Multi_Select_Mode | mode | ||
) |
Set the genlist multi select mode.
ELM_OBJECT_MULTI_SELECT_MODE_DEFAULT means that select/unselect items whenever each item is clicked. ELM_OBJECT_MULTI_SELECT_MODE_WITH_CONTROL means that only one item will be selected although multi-selection is enabled, if clicked without pressing control key. This mode is only available with multi-selection.
- Parameters:
-
[in] obj The object. [in] mode The multi select mode
- Since (EFL) :
- 1.8
void elm_genlist_multi_select_set | ( | Elm_Genlist * | obj, |
Eina_Bool | multi | ||
) |
Enable or disable multi-selection in the genlist.
This enables ($true) or disables ($false) multi-selection in the list. This allows more than 1 item to be selected. To retrieve the list of selected items, use elm_genlist_selected_items_get.
- Parameters:
-
[in] obj The object. [in] multi Multi-select enable/disable. Default is disabled.
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
Elm_Object_Item* elm_genlist_nth_item_get | ( | const Evas_Object * | obj, |
unsigned int | nth | ||
) |
Get the nth item, in a given genlist widget, placed at position nth
, in its internal items list
- Parameters:
-
obj The genlist object nth The number of the item to grab (0 being the first)
- Returns:
- The item stored in
obj
at positionnth
orNULL
, if there's no item with that index (and on errors)
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
Eina_List* elm_genlist_realized_items_get | ( | const Elm_Genlist * | obj | ) |
Get a list of realized items in genlist.
This returns a list of the realized items in the genlist. The list contains genlist item pointers. The list must be freed by the caller when done with eina_list_free. The item pointers in the list are only valid so long as those items are not deleted or the genlist is not deleted.
- Parameters:
-
[in] obj The object.
- Returns:
- List of realized items
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
void elm_genlist_realized_items_update | ( | Elm_Genlist * | obj | ) |
Update the contents of all realized items.
This updates all realized items by calling all the item class functions again to get the contents, texts and states. Use this when the original item data has changed and the changes are desired to be reflected.
To update just one item, use elm_genlist_item_update.
- Parameters:
-
[in] obj The object.
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
Eina_Bool elm_genlist_reorder_mode_get | ( | const Elm_Genlist * | obj | ) |
Get the reorder mode.
- Parameters:
-
[in] obj The object.
- Returns:
- The reorder mode.
- Since :
- 2.3.1
void elm_genlist_reorder_mode_set | ( | Elm_Genlist * | obj, |
Eina_Bool | reorder_mode | ||
) |
Set reorder mode.
After turning on the reorder mode, longpress on normal item will trigger reordering of the item. You can move the item up and down. However, reorder does not work with group item.
- Parameters:
-
[in] obj The object. [in] reorder_mode The reorder mode.
- Since :
- 2.3.1
Elm_Widget_Item* elm_genlist_search_by_text_item_get | ( | Elm_Genlist * | obj, |
Elm_Widget_Item * | item_to_search_from, | ||
const char * | part_name, | ||
const char * | pattern, | ||
Elm_Glob_Match_Flags | flags | ||
) |
Get genlist item by given string.
It takes pointer to the genlist item that will be used to start search from it.
This function uses globs (like "*.jpg") for searching and takes search flags as last parameter That is a bitfield with values to be ored together or 0 for no flags.
- Parameters:
-
[in] obj The object. [in] item_to_search_from Pointer to item to start search from. If null
, search will be started from the first item of the genlist.[in] part_name Name of the TEXT part of genlist item to search string in. [in] pattern The search pattern. [in] flags Search flags.
- Returns:
- Searched item
- Since (EFL) :
- 1.11
Elm_Object_Select_Mode elm_genlist_select_mode_get | ( | const Elm_Genlist * | obj | ) |
Get the genlist select mode.
- Parameters:
-
[in] obj The object.
- Returns:
- The select mode.
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
void elm_genlist_select_mode_set | ( | Elm_Genlist * | obj, |
Elm_Object_Select_Mode | mode | ||
) |
Set the genlist select mode.
ELM_OBJECT_SELECT_MODE_DEFAULT means that items will call their selection func and callback once when first becoming selected. Any further clicks will do nothing, unless you set always select mode. ELM_OBJECT_SELECT_MODE_ALWAYS means that even if selected, every click will make the selected callbacks be called. ELM_OBJECT_SELECT_MODE_NONE will turn off the ability to select items entirely and they will neither appear selected nor call selected callback functions.
- Parameters:
-
[in] obj The object. [in] mode The select mode.
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
Elm_Widget_Item* elm_genlist_selected_item_get | ( | const Elm_Genlist * | obj | ) |
Get the selected item in the genlist.
This gets the selected item in the list (if multi-selection is enabled, only the item that was first selected in the list is returned - which is not very useful, so see elm_genlist_selected_items_get for when multi-selection is used).
If no item is selected, null
is returned.
- Parameters:
-
[in] obj The object.
- Returns:
- The selected item, or
null
if none is selected.
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c, genlist_example_04.c, and genlist_example_05.c.
const Eina_List* elm_genlist_selected_items_get | ( | const Elm_Genlist * | obj | ) |
Get a list of selected items in the genlist.
It returns a list of the selected items. This list pointer is only valid so long as the selection doesn't change (no items are selected or unselected, or unselected implicitl by deletion). The list contains genlist items pointers. The order of the items in this list is the order which they were selected, i.e. the first item in this list is the first item that was selected, and so on.
- Note:
- If not in multi-select mode, consider using function elm_genlist_selected_item_get instead.
- Parameters:
-
[in] obj The object.
- Returns:
- List of selected items
- Since :
- 2.3.1
- Examples:
- genlist_example_02.c.
Eina_Bool elm_genlist_tree_effect_enabled_get | ( | const Elm_Genlist * | obj | ) |
Control genlist tree effect.
- Parameters:
-
[in] obj The object.
- Returns:
- The tree effect status.
void elm_genlist_tree_effect_enabled_set | ( | Elm_Genlist * | obj, |
Eina_Bool | enabled | ||
) |
Control genlist tree effect.
- Parameters:
-
[in] obj The object. [in] enabled The tree effect status.