Tizen Native API
7.0
|
Here are grouped together functions used to create and manipulate image objects. They are available to whichever occasion one needs complex imagery on a GUI that could not be achieved by the other Evas' primitive object types, or to make image manipulations.
Evas will support whatever image file types it was compiled with support to (its image loaders) -- check your software packager for that information and see evas_object_image_extension_can_load_get().
Image object basics
The most common use of image objects -- to display an image on the canvas -- is achieved by a common function triplet:
img = evas_object_image_add(canvas); evas_object_image_file_set(img, "path/to/img", NULL); evas_object_image_fill_set(img, 0, 0, w, h);
The first function, naturally, is creating the image object. Then, one must set a source file on it, so that it knows where to fetch image data from. Next, one must set how to fill the image object's area with that given pixel data. One could use just a sub-region of the original image or even have it tiled repeatedly on the image object. For the common case of having the whole source image to be displayed on the image object, stretched to the destination's size, there's also a function helper, to be used instead of evas_object_image_fill_set():
See those functions' documentation for more details.
Scale and resizing
Resizing of image objects will scale their respective source images to their areas, if they are set to "fill" the object's area (evas_object_image_filled_set()). If the user wants any control on the aspect ratio of an image for different sizes, he/she has to take care of that themselves. There are functions to make images to get loaded scaled (up or down) in memory, already, if the user is going to use them at pre-determined sizes and wants to save computations.
Evas has even a scale cache that will take care of caching scaled versions of images with more often usage/hits. Finally, one can have images being rescaled smoothly by Evas (more computationally expensive) or not.
Performance hints
When dealing with image objects, there are some tricks to boost the performance of your application, if it does intense image loading and/or manipulations, as in animations on a UI.
Load hints
In image viewer applications, for example, the user will be looking at a given image, at full size, and will desire that the navigation to the adjacent images on his/her album be fluid and fast. Thus, while displaying a given image, the program can be in the background loading the next and previous images already, so that displaying them in sequence is just a matter of repainting the screen (and not decoding image data).
Evas addresses this issue with image pre-loading. The code for the situation above would be something like the following:
prev = evas_object_image_filled_add(canvas); evas_object_image_file_set(prev, "/path/to/prev", NULL); evas_object_image_preload(prev, EINA_TRUE); next = evas_object_image_filled_add(canvas); evas_object_image_file_set(next, "/path/to/next", NULL); evas_object_image_preload(next, EINA_TRUE);
If you're loading images that are too big, consider setting previously it's loading size to something smaller, in case you won't expose them in real size. It may speed up the loading considerably:
//to load a scaled down version of the image in memory, if that's //the size you'll be displaying it anyway evas_object_image_load_scale_down_set(img, zoom); //optional: if you know you'll be showing a sub-set of the image's //pixels, you can avoid loading the complementary data evas_object_image_load_region_set(img, x, y, w, h);
Refer to Elementary's Photocam widget for a high level (smart) object that does lots of loading speed-ups for you.
Animation hints
If you want to animate image objects on a UI (what you'd get by concomitant usage of other libraries, like Ecore and Edje), there are also some tips on how to boost the performance of your application. If the animation involves resizing of an image (thus, re-scaling), you'd better turn off smooth scaling on it during the animation, turning it back on afterwards, for less computations. Also, in this case you'd better flag the image object in question not to cache scaled versions of it:
evas_object_image_scale_hint_set(wd->img, EVAS_IMAGE_SCALE_HINT_DYNAMIC); // resizing takes place in between evas_object_image_scale_hint_set(wd->img, EVAS_IMAGE_SCALE_HINT_STATIC);
Finally, movement of opaque images through the canvas is less expensive than of translucid ones, because of blending computations.
Borders
Evas provides facilities for one to specify an image's region to be treated specially -- as "borders". This will make those regions be treated specially on resizing scales, by keeping their aspect. This makes setting frames around other objects on UIs easy. See the following figures for a visual explanation:
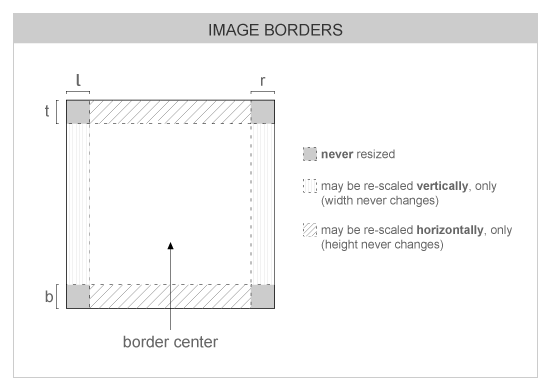
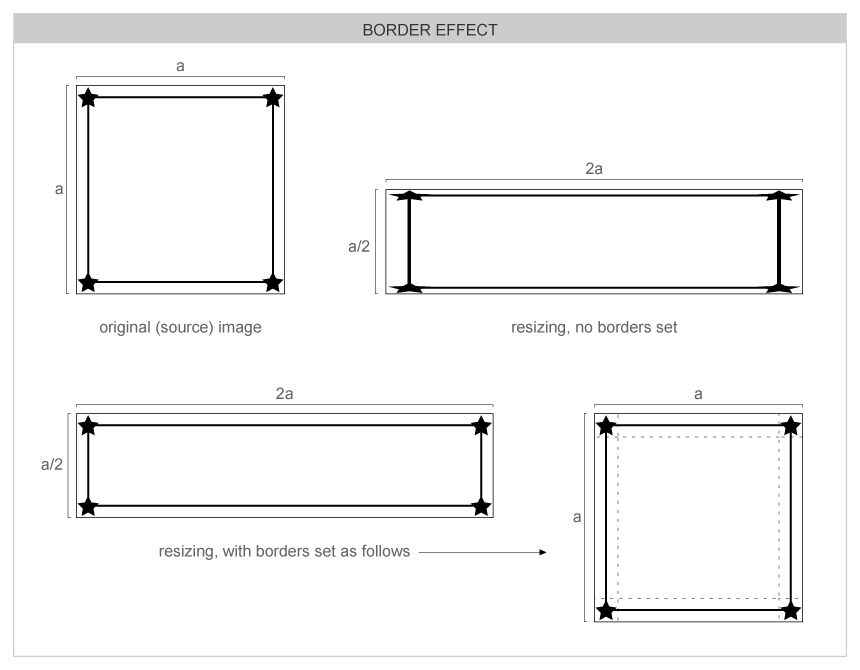
Manipulating pixels
Evas image objects can be used to manipulate raw pixels in many ways. The meaning of the data in the pixel arrays will depend on the image's color space, be warned (see next section). You can set your own data as an image's pixel data, fetch an image's pixel data for saving/altering, convert images between different color spaces and even advanced operations like setting a native surface as image objects' data.
Color spaces
Image objects may return or accept "image data" in multiple formats. This is based on the color space of an object. Here is a rundown on formats:
- #EVAS_COLORSPACE_ARGB8888: This pixel format is a linear block of pixels, starting at the top-left row by row until the bottom right of the image or pixel region. All pixels are 32-bit unsigned int's with the high-byte being alpha and the low byte being blue in the format ARGB. Alpha may or may not be used by evas depending on the alpha flag of the image, but if not used, should be set to 0xff anyway.
This colorspace uses premultiplied alpha. That means that R, G and B cannot exceed A in value. The conversion from non-premultiplied colorspace is:
R = (r * a) / 255; G = (g * a) / 255; B = (b * a) / 255;
So 50% transparent blue will be: 0x80000080. This will not be "dark" - just 50% transparent. Values are 0 == black, 255 == solid or full red, green or blue.
- #EVAS_COLORSPACE_YCBCR422P601_PL: This is a pointer-list indirected set of YUV (YCbCr) pixel data. This means that the data returned or set is not actual pixel data, but pointers TO lines of pixel data. The list of pointers will first be N rows of pointers to the Y plane - pointing to the first pixel at the start of each row in the Y plane. N is the height of the image data in pixels. Each pixel in the Y, U and V planes is 1 byte exactly, packed. The next N / 2 pointers will point to rows in the U plane, and the next N / 2 pointers will point to the V plane rows. U and V planes are half the horizontal and vertical resolution of the Y plane.
Row order is top to bottom and row pixels are stored left to right.
There is a limitation that these images MUST be a multiple of 2 pixels in size horizontally or vertically. This is due to the U and V planes being half resolution. Also note that this assumes the itu601 YUV colorspace specification. This is defined for standard television and mpeg streams. HDTV may use the itu709 specification.
Values are 0 to 255, indicating full or no signal in that plane respectively.
- #EVAS_COLORSPACE_YCBCR422P709_PL: Not implemented yet.
- #EVAS_COLORSPACE_RGB565_A5P: In the process of being implemented in 1 engine only. This may change.
This is a pointer to image data for 16-bit half-word pixel data in 16bpp RGB 565 format (5 bits red, 6 bits green, 5 bits blue), with the high-byte containing red and the low byte containing blue, per pixel. This data is packed row by row from the top-left to the bottom right.
If the image has an alpha channel enabled there will be an extra alpha plane after the color pixel plane. If not, then this data will not exist and should not be accessed in any way. This plane is a set of pixels with 1 byte per pixel defining the alpha values of all pixels in the image from the top-left to the bottom right of the image, row by row. Even though the values of the alpha pixels can be 0 to 255, only values 0 through to 32 are used, 32 being solid and 0 being transparent.
RGB values can be 0 to 31 for red and blue and 0 to 63 for green, with 0 being black and 31 or 63 being full red, green or blue respectively. This colorspace is also pre-multiplied like EVAS_COLORSPACE_ARGB8888 so:
R = (r * a) / 32; G = (g * a) / 32; B = (b * a) / 32;
- #EVAS_COLORSPACE_GRY8: The image is just an alpha mask (8 bit's per pixel). This is used for alpha masking.
- Warning:
- We don't guarantee any proper results if you create an Image object without setting the evas engine.
Some examples of this group of functions can be found here.
Functions | |
Eina_Bool | evas_object_image_extension_can_load_get (const char *file) |
Eina_Bool | evas_object_image_extension_can_load_fast_get (const char *file) |
Evas_Object * | evas_object_image_add (Evas *e) |
Evas_Object * | evas_object_image_filled_add (Evas *e) |
void | evas_object_image_memfile_set (Evas_Object *obj, void *data, int size, char *format, char *key) |
void | evas_object_image_native_surface_set (Evas_Object *obj, Evas_Native_Surface *surf) |
Evas_Native_Surface * | evas_object_image_native_surface_get (const Evas_Object *obj) |
Get the native surface of a given image of the canvas. | |
void | evas_object_image_preload (Evas_Object *obj, Eina_Bool cancel) |
Eina_Bool | evas_object_image_source_unset (Evas_Object *obj) |
void | evas_object_image_file_set (Eo *obj, const char *file, const char *key) |
void | evas_object_image_file_get (const Eo *obj, const char **file, const char **key) |
void | evas_object_image_mmap_set (Eo *obj, const Eina_File *f, const char *key) |
void | evas_object_image_mmap_get (const Eo *obj, const Eina_File **f, const char **key) |
Eina_Bool | evas_object_image_save (const Eo *obj, const char *file, const char *key, const char *flags) |
Eina_Bool | evas_object_image_animated_get (const Eo *obj) |
void | evas_object_image_animated_frame_set (Evas_Object *obj, int frame_index) |
Set the frame to current frame of an image object. | |
int | evas_object_image_animated_frame_get (Evas_Object *obj) |
Get the frame to current frame of an image object. | |
int | evas_object_image_animated_frame_count_get (const Evas_Object *obj) |
Get the total number of frames of the image object. | |
Evas_Image_Animated_Loop_Hint | evas_object_image_animated_loop_type_get (const Evas_Object *obj) |
Get the kind of looping the image object does. | |
int | evas_object_image_animated_loop_count_get (const Evas_Object *obj) |
Get the number times the animation of the object loops. | |
double | evas_object_image_animated_frame_duration_get (const Evas_Object *obj, int start_frame, int frame_num) |
Get the duration of a sequence of frames. | |
void | evas_object_image_load_dpi_set (Evas_Object *obj, double dpi) |
Set the DPI resolution of an image object's source image. | |
double | evas_object_image_load_dpi_get (const Evas_Object *obj) |
Get the DPI resolution of a loaded image object in the canvas. | |
void | evas_object_image_load_size_set (Eo *obj, int w, int h) |
void | evas_object_image_load_size_get (const Eo *obj, int *w, int *h) |
void | evas_object_image_load_region_set (Evas_Object *obj, int x, int y, int w, int h) |
Inform a given image object to load a selective region of its source image. | |
void | evas_object_image_load_region_get (const Evas_Object *obj, int *x, int *y, int *w, int *h) |
Retrieve the coordinates of a given image object's selective (source image) load region. | |
void | evas_object_image_load_orientation_set (Evas_Object *obj, Eina_Bool enable) |
Define if the orientation information in the image file should be honored. | |
Eina_Bool | evas_object_image_load_orientation_get (const Evas_Object *obj) |
Get if the orientation information in the image file should be honored. | |
void | evas_object_image_load_scale_down_set (Evas_Object *obj, int scale_down) |
Set the scale down factor of a given image object's source image, when loading it. | |
int | evas_object_image_load_scale_down_get (const Evas_Object *obj) |
Get the scale down factor of a given image object's source image, when loading it. | |
void | evas_object_image_load_head_skip_set (Evas_Object *obj, Eina_Bool skip) |
Set a load option to skip initial header load and defer to preload. | |
Eina_Bool | evas_object_image_load_head_skip_get (const Evas_Object *obj) |
Get the load option to skip header loads before preload. | |
Evas_Load_Error | evas_object_image_load_error_get (const Evas_Object *obj) |
Retrieves a number representing any error that occurred during the last loading of the given image object's source image. | |
void | evas_object_image_smooth_scale_set (Eo *obj, Eina_Bool smooth_scale) |
Eina_Bool | evas_object_image_smooth_scale_get (const Eo *obj) |
void | evas_object_image_fill_spread_set (Evas_Object *obj, Evas_Fill_Spread spread) EINA_DEPRECATED |
Evas_Fill_Spread | evas_object_image_fill_spread_get (const Evas_Object *obj) EINA_DEPRECATED |
void | evas_object_image_fill_set (Evas_Object *obj, Evas_Coord x, Evas_Coord y, Evas_Coord w, Evas_Coord h) |
void | evas_object_image_fill_get (const Evas_Object *obj, Evas_Coord *x, Evas_Coord *y, Evas_Coord *w, Evas_Coord *h) |
void | evas_object_image_filled_set (Evas_Object *obj, Eina_Bool filled) |
Set whether the image object's fill property should track the object's size. | |
Eina_Bool | evas_object_image_filled_get (const Evas_Object *obj) |
Retrieve whether the image object's fill property should track the object's size. | |
void | evas_object_image_alpha_set (Evas_Object *obj, Eina_Bool alpha) |
Enable or disable alpha channel usage on the given image object. | |
void | evas_object_image_border_set (Evas_Object *obj, int l, int r, int t, int b) |
Dimensions of this image's border, a region that does not scale with the center area. | |
void | evas_object_image_border_get (const Evas_Object *obj, int *l, int *r, int *t, int *b) |
Dimensions of this image's border, a region that does not scale with the center area. | |
void | evas_object_image_border_scale_set (Evas_Object *obj, double scale) |
Scaling factor applied to the image borders. | |
double | evas_object_image_border_scale_get (const Evas_Object *obj) |
Scaling factor applied to the image borders. | |
void | evas_object_image_border_center_fill_set (Evas_Object *obj, Evas_Border_Fill_Mode fill) |
Specifies how the center part of the object (not the borders) should be drawn when EFL is rendering it. | |
Evas_Border_Fill_Mode | evas_object_image_border_center_fill_get (const Evas_Object *obj) |
Specifies how the center part of the object (not the borders) should be drawn when EFL is rendering it. | |
void | evas_object_image_content_hint_set (Evas_Object *obj, Evas_Image_Content_Hint hint) |
Set the content hint setting of a given image object of the canvas. | |
Evas_Image_Content_Hint | evas_object_image_content_hint_get (const Evas_Object *obj) |
Get the content hint setting of a given image object of the canvas. | |
void | evas_object_image_size_set (Evas_Object *obj, int w, int h) |
void | evas_object_image_size_get (const Evas_Object *obj, int *w, int *h) |
void | evas_object_image_data_update_add (Evas_Object *obj, int x, int y, int w, int h) |
Mark a sub-region of the given image object to be redrawn. | |
void | evas_object_image_snapshot_set (Evas_Object *obj, Eina_Bool s) |
The content below the Evas_Object_Image will be rendered inside it and you can reuse it as a source for any kind of effect. | |
Eina_Bool | evas_object_image_snapshot_get (const Evas_Object *obj) |
Determine whether the Evas_Object_Image replicate the content of the canvas below. | |
Eina_Bool | evas_object_image_source_set (Evas_Object *obj, Evas_Object *src) |
Set the source object on an image object to used as a proxy. | |
Evas_Object * | evas_object_image_source_get (const Evas_Object *obj) |
Get the current source object of an image object. | |
void | evas_object_image_source_clip_set (Evas_Object *obj, Eina_Bool source_clip) |
Clip the proxy object with the source object's clipper. | |
Eina_Bool | evas_object_image_source_clip_get (const Evas_Object *obj) |
Determine whether an object is clipped by source object's clipper. | |
void | evas_object_image_source_events_set (Evas_Object *obj, Eina_Bool repeat) |
Set whether an Evas object is to source events. | |
Eina_Bool | evas_object_image_source_events_get (const Evas_Object *obj) |
Determine whether an object is set to source events. | |
void | evas_object_image_source_visible_set (Evas_Object *obj, Eina_Bool visible) |
Set the source object to be visible or not. | |
Eina_Bool | evas_object_image_source_visible_get (const Evas_Object *obj) |
Get the state of the source object visibility. | |
void | evas_object_image_pixels_dirty_set (Evas_Object *obj, Eina_Bool dirty) |
Mark whether the given image object is dirty and needs to request its pixels. | |
Eina_Bool | evas_object_image_pixels_dirty_get (const Evas_Object *obj) |
Retrieves whether the given image object is dirty (needs to be redrawn). | |
void | evas_object_image_pixels_get_callback_set (Evas_Object *obj, Evas_Object_Image_Pixels_Get_Cb func, void *data) |
Set the callback function to get pixels from a canvas' image. | |
void | evas_object_image_video_surface_set (Evas_Object *obj, Evas_Video_Surface *surf) |
Set the video surface linked to a given image of the canvas. | |
const Evas_Video_Surface * | evas_object_image_video_surface_get (const Evas_Object *obj) |
Get the video surface linked to a given image of the canvas. | |
void | evas_object_image_video_surface_caps_set (Evas_Object *obj, unsigned int caps) |
Set the video surface capabilities to a given image of the canvas. | |
unsigned int | evas_object_image_video_surface_caps_get (const Evas_Object *obj) |
void * | evas_object_image_data_convert (Evas_Object *obj, Evas_Colorspace to_cspace) EINA_DEPRECATED |
Eina_Bool | evas_object_image_pixels_import (Evas_Object *obj, Evas_Pixel_Import_Source *pixels) EINA_DEPRECATED |
void | evas_object_image_reload (Evas_Object *obj) EINA_DEPRECATED |
void | evas_object_image_alpha_mask_set (Evas_Object *obj, Eina_Bool ismask) EINA_DEPRECATED |
Typedefs | |
typedef void(* | Evas_Object_Image_Pixels_Get_Cb )(void *data, Evas_Object *o) |
typedef enum _Evas_Native_Surface_Type | Evas_Native_Surface_Type |
typedef enum _Evas_Native_Surface_Status | Evas_Native_Surface_Status |
typedef struct _Evas_Native_Surface | Evas_Native_Surface |
A generic datatype for engine specific native surface information. | |
typedef struct _Evas_Video_Surface | Evas_Video_Surface |
typedef void(* | Evas_Video_Cb )(void *data, Evas_Object *obj, const Evas_Video_Surface *surface) |
typedef void(* | Evas_Video_Coord_Cb )(void *data, Evas_Object *obj, const Evas_Video_Surface *surface, Evas_Coord a, Evas_Coord b) |
typedef enum _Evas_Video_Surface_Caps | Evas_Video_Surface_Caps |
typedef Emile_Colorspace | Evas_Colorspace |
Defines | |
#define | EVAS_NATIVE_SURFACE_VERSION 5 |
#define | EVAS_VIDEO_SURFACE_VERSION 1 |
Define Documentation
#define EVAS_NATIVE_SURFACE_VERSION 5 |
Magic version number to know what the native surface struct looks like
#define EVAS_VIDEO_SURFACE_VERSION 1 |
Magic version number to know what the video surf struct looks like
- Since (EFL) :
- 1.1
Typedef Documentation
typedef Emile_Colorspace Evas_Colorspace |
Colorspaces for pixel data supported by Evas Colorspaces for pixel data supported by Evas
typedef struct _Evas_Native_Surface Evas_Native_Surface |
A generic datatype for engine specific native surface information.
Please fill up Evas_Native_Surface fields that regarded with current surface type. If you want to set the native surface type to EVAS_NATIVE_SURFACE_X11, you need to set union data with x11.visual or x11.pixmap. If you need to set the native surface as EVAS_NATIVE_SURFACE_OPENGL, on the other hand, you need to set union data with opengl.texture_id or opengl.framebuffer_id and so on. If you need to set the native surface as EVAS_NATIVE_SURFACE_WL, you need to set union data with wl.legacy_buffer. If you need to set the native surface as EVAS_NATIVE_SURFACE_TBM, you need to set union data with tbm surface. The version field should be set with EVAS_NATIVE_SURFACE_VERSION in order to check abi break in your application on the different efl library versions.
- Warning:
- Native surface types totally depend on the system. Please be aware that the types are supported on your system before using them.
- Note:
- The information stored in an
Evas_Native_Surface
returned by evas_gl_native_surface_get() is not meant to be used by applications except for passing it to evas_object_image_native_surface_set().
typedef enum _Evas_Native_Surface_Status Evas_Native_Surface_Status |
Native surface types that image object supports
typedef enum _Evas_Native_Surface_Type Evas_Native_Surface_Type |
Native surface types that image object supports
typedef void(* Evas_Object_Image_Pixels_Get_Cb)(void *data, Evas_Object *o) |
Function signature for the evas object pixels get callback function
- See also:
- evas_object_image_pixels_get()
By data it will be passed the private data. By o it will be passed the Evas_Object image on which the pixels are requested.
typedef void(* Evas_Video_Cb)(void *data, Evas_Object *obj, const Evas_Video_Surface *surface) |
Evas video callback function signature
typedef void(* Evas_Video_Coord_Cb)(void *data, Evas_Object *obj, const Evas_Video_Surface *surface, Evas_Coord a, Evas_Coord b) |
Evas video coordinates callback function signature
A generic datatype for video specific surface information
- Since (EFL) :
- 1.1
typedef enum _Evas_Video_Surface_Caps Evas_Video_Surface_Caps |
Enum values for the Video surface capabilities
Enumeration Type Documentation
enum _Efl_Gfx_Fill_Spread |
- Enumerator:
Native surface types that image object supports
Native surface types that image object supports
- Enumerator:
Enum values for the Video surface capabilities
How an image's center region (the complement to the border region) should be rendered by Evas
- Enumerator:
enum Evas_Image_Orient |
Possible orientation options for evas_object_image_orient_set().
- Since (EFL) :
- 1.14
- Enumerator:
Function Documentation
Evas_Object* evas_object_image_add | ( | Evas * | e | ) |
Creates a new image object on the given Evas e
canvas.
- Parameters:
-
e The given canvas.
- Returns:
- The created image object handle.
- Note:
- If you intend to display an image somehow in a GUI, besides binding it to a real image file/source (with evas_object_image_file_set(), for example), you'll have to tell this image object how to fill its space with the pixels it can get from the source. See evas_object_image_filled_add(), for a helper on the common case of scaling up an image source to the whole area of the image object.
- See also:
- evas_object_image_fill_set()
Example:
img = evas_object_image_add(canvas); evas_object_image_file_set(img, "/path/to/img", NULL);
- Since :
- 2.3.1
- Examples:
- evas-images.c, evas-images2.c, and map_example_02.c.
void evas_object_image_alpha_mask_set | ( | Evas_Object * | obj, |
Eina_Bool | ismask | ||
) |
- Deprecated:
- This function has never been implemented. Please use evas_object_clip_set() with an alpha or RGBA image instead of setting this flag.
- Since :
- 2.3.1
void evas_object_image_alpha_set | ( | Evas_Object * | obj, |
Eina_Bool | alpha | ||
) |
Enable or disable alpha channel usage on the given image object.
This function sets a flag on an image object indicating whether or not to use alpha channel data. A value of true
makes it use alpha channel data, and false
makes it ignore that data. Note that this has nothing to do with an object's color as manipulated by evas_object_color_set.
- Parameters:
-
[in] obj The object [in] alpha Whether to use alpha channel ($true) data or not ($false).
- Since :
- 2.3.1
- Examples:
- efl_thread_6.c, and evas-images.c.
int evas_object_image_animated_frame_count_get | ( | const Evas_Object * | obj | ) |
Get the total number of frames of the image object.
This returns total number of frames the image object supports (if animated).
See also evas_object_image_animated_get, evas_object_image_animated_loop_type_get, evas_object_image_animated_loop_count_get, evas_object_image_animated_frame_duration_get.
- Returns:
- The number of frames.
- Since (EFL) :
- 1.1
- Since :
- 2.3.1
double evas_object_image_animated_frame_duration_get | ( | const Evas_Object * | obj, |
int | start_frame, | ||
int | frame_num | ||
) |
Get the duration of a sequence of frames.
This returns total duration that the specified sequence of frames should take in seconds.
If you set start_frame to 1 and frame_num 0, you get frame 1's duration. If you set start_frame to 1 and frame_num 1, you get frame 1's duration + frame2's duration.
See also evas_object_image_animated_get, evas_object_image_animated_frame_count_get, evas_object_image_animated_loop_type_get, evas_object_image_animated_loop_count_get,
- Parameters:
-
[in] obj The object [in] frame_num Number of frames in the sequence.
- Since (EFL) :
- 1.1
- Since :
- 2.3.1
int evas_object_image_animated_frame_get | ( | Evas_Object * | obj | ) |
Get the frame to current frame of an image object.
This returns image object's current frame.
See also evas_object_image_animated_get, evas_object_image_animated_frame_count_get, evas_object_image_animated_loop_type_get, evas_object_image_animated_loop_count_get, evas_object_image_animated_frame_duration_get. evas_object_image_animated_frame_set.
- Parameters:
-
[in] obj The object
- Returns:
- The index of current frame.
- Since (EFL) :
- 1.24
void evas_object_image_animated_frame_set | ( | Evas_Object * | obj, |
int | frame_index | ||
) |
Set the frame to current frame of an image object.
This set image object's current frame to frame_num with 1 being the first frame.
See also evas_object_image_animated_get, evas_object_image_animated_frame_count_get, evas_object_image_animated_loop_type_get, evas_object_image_animated_loop_count_get, evas_object_image_animated_frame_duration_get. evas_object_image_animated_frame_get.
- Parameters:
-
[in] obj The object [in] frame_index The index of current frame.
- Since (EFL) :
- 1.1
- Since :
- 2.3.1
Eina_Bool evas_object_image_animated_get | ( | const Eo * | obj | ) |
Check if an image object can be animated (have multiple frames)
- Parameters:
-
[in] obj The object
- Returns:
- whether obj support animation
This returns if the image file of an image object is capable of animation such as an animated gif file might. This is only useful to be called once the image object file has been set.
Example:
extern Evas_Object *obj; if (evas_object_image_animated_get(obj)) { int frame_count; int loop_count; Evas_Image_Animated_Loop_Hint loop_type; double duration; frame_count = evas_object_image_animated_frame_count_get(obj); printf("This image has %d frames\n",frame_count); duration = evas_object_image_animated_frame_duration_get(obj,1,0); printf("Frame 1's duration is %f. You had better set object's frame to 2 after this duration using timer\n"); loop_count = evas_object_image_animated_loop_count_get(obj); printf("loop count is %d. You had better run loop %d times\n",loop_count,loop_count); loop_type = evas_object_image_animated_loop_type_get(obj); if (loop_type == EVAS_IMAGE_ANIMATED_HINT_LOOP) printf("You had better set frame like 1->2->3->1->2->3...\n"); else if (loop_type == EVAS_IMAGE_ANIMATED_HINT_PINGPONG) printf("You had better set frame like 1->2->3->2->1->2...\n"); else printf("Unknown loop type\n"); evas_object_image_animated_frame_set(obj,1); printf("You set image object's frame to 1. You can see frame 1\n"); }
- See also:
- evas_object_image_animated_get()
- evas_object_image_animated_frame_count_get()
- evas_object_image_animated_loop_type_get()
- evas_object_image_animated_loop_count_get()
- evas_object_image_animated_frame_duration_get()
- evas_object_image_animated_frame_set()
- Since (EFL) :
- 1.1
- Since :
- 2.3.1
int evas_object_image_animated_loop_count_get | ( | const Evas_Object * | obj | ) |
Get the number times the animation of the object loops.
This returns loop count of image. The loop count is the number of times the animation will play fully from first to last frame until the animation should stop (at the final frame).
If 0 is returned, then looping should happen indefinitely (no limit to the number of times it loops).
See also evas_object_image_animated_get, evas_object_image_animated_frame_count_get, evas_object_image_animated_loop_type_get, evas_object_image_animated_frame_duration_get.
- Parameters:
-
[in] obj The object
- Returns:
- The number of loop of an animated image object.
- Since (EFL) :
- 1.1
- Since :
- 2.3.1
Evas_Image_Animated_Loop_Hint evas_object_image_animated_loop_type_get | ( | const Evas_Object * | obj | ) |
Get the kind of looping the image object does.
This returns the kind of looping the image object wants to do.
If it returns EVAS_IMAGE_ANIMATED_HINT_LOOP, you should display frames in a sequence like: 1->2->3->1->2->3->1...
If it returns EVAS_IMAGE_ANIMATED_HINT_PINGPONG, it is better to display frames in a sequence like: 1->2->3->2->1->2->3->1...
The default type is EVAS_IMAGE_ANIMATED_HINT_LOOP.
See also evas_object_image_animated_get, evas_object_image_animated_frame_count_get, evas_object_image_animated_loop_count_get, evas_object_image_animated_frame_duration_get.
- Parameters:
-
[in] obj The object
- Returns:
- Loop type of the image object.
- Since (EFL) :
- 1.1
- Since :
- 2.3.1
Specifies how the center part of the object (not the borders) should be drawn when EFL is rendering it.
This function sets how the center part of the image object's source image is to be drawn, which must be one of the values in Evas_Border_Fill_Mode. By center we mean the complementary part of that defined by evas_object_image_border_set. This one is very useful for making frames and decorations.
The default value is
- Parameters:
-
[in] obj The object
- Returns:
- Fill mode of the center region of
obj
(a value in Evas_Border_Fill_Mode).
- Since :
- 2.3.1
void evas_object_image_border_center_fill_set | ( | Evas_Object * | obj, |
Evas_Border_Fill_Mode | fill | ||
) |
Specifies how the center part of the object (not the borders) should be drawn when EFL is rendering it.
This function sets how the center part of the image object's source image is to be drawn, which must be one of the values in Evas_Border_Fill_Mode. By center we mean the complementary part of that defined by evas_object_image_border_set. This one is very useful for making frames and decorations.
- Parameters:
-
[in] obj The object [in] fill Fill mode of the center region of obj
(a value in Evas_Border_Fill_Mode).
- Since :
- 2.3.1
void evas_object_image_border_get | ( | const Evas_Object * | obj, |
int * | l, | ||
int * | r, | ||
int * | t, | ||
int * | b | ||
) |
Dimensions of this image's border, a region that does not scale with the center area.
When EFL renders an image, its source may be scaled to fit the size of the object. This function sets an area from the borders of the image inwards which is not to be scaled. This function is useful for making frames and for widget theming, where, for example, buttons may be of varying sizes, but their border size must remain constant.
The units used for l
, r
, t
and b
are canvas units (pixels).
- Note:
- The border region itself may be scaled by the evas_object_image_border_scale_set function.
-
By default, image objects have no borders set, i.e.
l
,r
,t
andb
start as 0. - Similar to the concepts of 9-patch images or cap insets.
- Parameters:
-
[in] obj The object [out] l The border's left width. [out] r The border's right width. [out] t The border's top height. [out] b The border's bottom height.
- Since :
- 2.3.1
- Examples:
- evas-images.c.
double evas_object_image_border_scale_get | ( | const Evas_Object * | obj | ) |
Scaling factor applied to the image borders.
This value multiplies the size of the evas_object_image_border_get when scaling an object.
Default value is 1.0 (no scaling).
- Parameters:
-
[in] obj The object
- Returns:
- The scale factor.
- Since :
- 2.3.1
- Examples:
- evas-images.c.
void evas_object_image_border_scale_set | ( | Evas_Object * | obj, |
double | scale | ||
) |
Scaling factor applied to the image borders.
This value multiplies the size of the evas_object_image_border_get when scaling an object.
Default value is 1.0 (no scaling).
- Parameters:
-
[in] obj The object [in] scale The scale factor.
- Since :
- 2.3.1
- Examples:
- evas-images.c.
void evas_object_image_border_set | ( | Evas_Object * | obj, |
int | l, | ||
int | r, | ||
int | t, | ||
int | b | ||
) |
Dimensions of this image's border, a region that does not scale with the center area.
When EFL renders an image, its source may be scaled to fit the size of the object. This function sets an area from the borders of the image inwards which is not to be scaled. This function is useful for making frames and for widget theming, where, for example, buttons may be of varying sizes, but their border size must remain constant.
The units used for l
, r
, t
and b
are canvas units (pixels).
- Note:
- The border region itself may be scaled by the evas_object_image_border_scale_set function.
-
By default, image objects have no borders set, i.e.
l
,r
,t
andb
start as 0. - Similar to the concepts of 9-patch images or cap insets.
- Parameters:
-
[in] obj The object [in] l The border's left width. [in] r The border's right width. [in] t The border's top height. [in] b The border's bottom height.
- Since :
- 2.3.1
Evas_Image_Content_Hint evas_object_image_content_hint_get | ( | const Evas_Object * | obj | ) |
Get the content hint setting of a given image object of the canvas.
This returns #EVAS_IMAGE_CONTENT_HINT_NONE on error.
- Parameters:
-
[in] obj The object
- Returns:
- The content hint value, one of the Evas_Image_Content_Hint ones.
- Since :
- 2.3.1
void evas_object_image_content_hint_set | ( | Evas_Object * | obj, |
Evas_Image_Content_Hint | hint | ||
) |
Set the content hint setting of a given image object of the canvas.
This function sets the content hint value of the given image of the canvas. For example, if you're on the GL engine and your driver implementation supports it, setting this hint to #EVAS_IMAGE_CONTENT_HINT_DYNAMIC will make it need zero copies at texture upload time, which is an "expensive" operation.
- Parameters:
-
[in] obj The object [in] hint The content hint value, one of the Evas_Image_Content_Hint ones.
- Since :
- 2.3.1
void* evas_object_image_data_convert | ( | Evas_Object * | obj, |
Evas_Colorspace | to_cspace | ||
) |
- Deprecated:
- evas_object_image_data_convert
- Since :
- 2.3.1
void evas_object_image_data_update_add | ( | Evas_Object * | obj, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h | ||
) |
Mark a sub-region of the given image object to be redrawn.
This function schedules a particular rectangular region of an image object to be updated (redrawn) at the next rendering cycle.
- Parameters:
-
[in] obj The object [in] y Y-offset of the region to be updated. [in] w Width of the region to be updated. [in] h Height of the region to be updated.
- Since :
- 2.3.1
Eina_Bool evas_object_image_extension_can_load_fast_get | ( | const char * | file | ) |
Check if a file extension may be supported by Image Object Functions.
- Parameters:
-
file The file to check, it should be an Eina_Stringshare.
- Returns:
EINA_TRUE
if we may be able to open it,EINA_FALSE
if it's unlikely.
- Since (EFL) :
- 1.1
This functions is threadsafe.
- Since :
- 2.3.1
Eina_Bool evas_object_image_extension_can_load_get | ( | const char * | file | ) |
Check if a file extension may be supported by Image Object Functions.
- Parameters:
-
file The file to check
- Returns:
EINA_TRUE
if we may be able to open it,EINA_FALSE
if it's unlikely.
- Since (EFL) :
- 1.1
If file is an Eina_Stringshare, use directly evas_object_image_extension_can_load_fast_get.
This functions is threadsafe.
- Since :
- 2.3.1
void evas_object_image_file_get | ( | const Eo * | obj, |
const char ** | file, | ||
const char ** | key | ||
) |
Retrieve the source file from where an image object is to fetch the real image data (it may be an Eet file, besides pure image ones).
You must not modify the strings on the returned pointers.
- Note:
- Use
NULL
pointers on the file components you're not interested in: they'll be ignored by the function.
- Parameters:
-
[in] obj The object [out] file The image file path. [out] key The image key in file
(if its an Eet one), orNULL
, otherwise.
- Since :
- 2.3.1
void evas_object_image_file_set | ( | Eo * | obj, |
const char * | file, | ||
const char * | key | ||
) |
Set the source file from where an image object must fetch the real image data (it may be an Eet file, besides pure image ones).
If the file supports multiple data stored in it (as Eet files do), you can specify the key to be used as the index of the image in this file.
Example:
img = evas_object_image_add(canvas); evas_object_image_file_set(img, "/path/to/img", NULL); err = evas_object_image_load_error_get(img); if (err != EVAS_LOAD_ERROR_NONE) { fprintf(stderr, "could not load image '%s'. error string is \"%s\"\n", valid_path, evas_load_error_str(err)); } else { evas_object_image_fill_set(img, 0, 0, w, h); evas_object_resize(img, w, h); evas_object_show(img); }
- Parameters:
-
[in] obj The object [in] file The image file path. [in] key The image key in file
(if its an Eet one), orNULL
, otherwise.
- Since :
- 2.3.1
void evas_object_image_fill_get | ( | const Evas_Object * | obj, |
Evas_Coord * | x, | ||
Evas_Coord * | y, | ||
Evas_Coord * | w, | ||
Evas_Coord * | h | ||
) |
Retrieve how an image object is to fill its drawing rectangle, given the (real) image bound to it.
- Note:
- Use
NULL
pointers on the fill components you're not interested in: they'll be ignored by the function.
See evas_object_image_fill_set() for more details.
- Parameters:
-
[in] obj The object [out] x The x coordinate (from the top left corner of the bound image) to start drawing from. [out] y The y coordinate (from the top left corner of the bound image) to start drawing from. [out] w The width the bound image will be displayed at. [out] h The height the bound image will be displayed at.
- Since :
- 2.3.1
- Examples:
- evas-images.c.
void evas_object_image_fill_set | ( | Evas_Object * | obj, |
Evas_Coord | x, | ||
Evas_Coord | y, | ||
Evas_Coord | w, | ||
Evas_Coord | h | ||
) |
Set how to fill an image object's drawing rectangle given the (real) image bound to it.
Note that if w
or h
are smaller than the dimensions of obj
, the displayed image will be tiled around the object's area. To have only one copy of the bound image drawn, x
and y
must be 0 and w
and h
need to be the exact width and height of the image object itself, respectively.
See the following image to better understand the effects of this call. On this diagram, both image object and original image source have a
x a
dimensions and the image itself is a circle, with empty space around it:
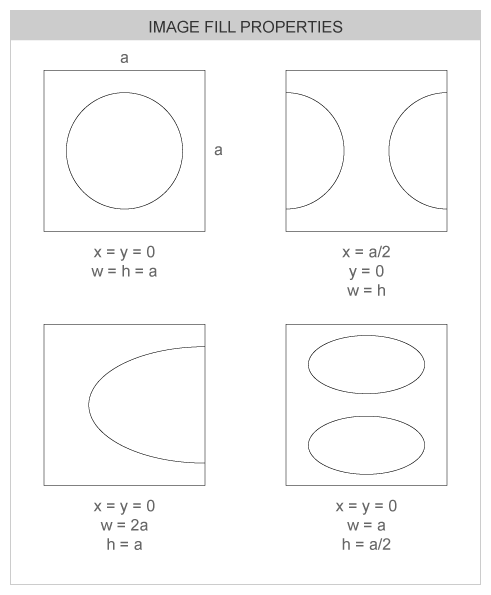
- Warning:
- The default values for the fill parameters are
x
= 0,y
= 0,w
= 0 andh
= 0. Thus, if you're not using the evas_object_image_filled_add() helper and want your image displayed, you'll have to set valid values with this function on your object.
- Note:
- evas_object_image_filled_set() is a helper function which will override the values set here automatically, for you, in a given way.
- Parameters:
-
[in] obj The object [in] x The x coordinate (from the top left corner of the bound image) to start drawing from. [in] y The y coordinate (from the top left corner of the bound image) to start drawing from. [in] w The width the bound image will be displayed at. [in] h The height the bound image will be displayed at.
- Since :
- 2.3.1
- Examples:
- evas-images.c.
Evas_Fill_Spread evas_object_image_fill_spread_get | ( | const Evas_Object * | obj | ) |
Retrieves the spread (tiling mode) for the given image object's fill.
- Parameters:
-
[in] obj The object
- Returns:
- The current spread mode of the image object.
- Since :
- 2.3.1
void evas_object_image_fill_spread_set | ( | Evas_Object * | obj, |
Evas_Fill_Spread | spread | ||
) |
Sets the tiling mode for the given evas image object's fill. EVAS_TEXTURE_RESTRICT, or EVAS_TEXTURE_PAD.
- Parameters:
-
[in] obj The object [in] spread One of EVAS_TEXTURE_REFLECT, EVAS_TEXTURE_REPEAT,
- Since :
- 2.3.1
Creates a new image object that automatically scales its bound image to the object's area, on both axis.
- Parameters:
-
e The given canvas.
- Returns:
- The created image object handle.
This is a helper function around evas_object_image_add() and evas_object_image_filled_set(). It has the same effect of applying those functions in sequence, which is a very common use case.
- Note:
- Whenever this object gets resized, the bound image will be rescaled, too.
- Since :
- 2.3.1
Eina_Bool evas_object_image_filled_get | ( | const Evas_Object * | obj | ) |
Retrieve whether the image object's fill property should track the object's size.
Returns true
if it is tracking, false
if not (and evas_object_image_fill_set must be called manually).
- Parameters:
-
[in] obj The object
- Returns:
true
to make the fill property follow object size orfalse
otherwise.
- Since :
- 2.3.1
- Examples:
- evas-images.c.
void evas_object_image_filled_set | ( | Evas_Object * | obj, |
Eina_Bool | filled | ||
) |
Set whether the image object's fill property should track the object's size.
If setting
is true
, then every evas_object_resize will automatically trigger a call to evas_object_image_fill_set with the that new size (and 0, 0 as source image's origin), so the bound image will fill the whole object's area.
- Parameters:
-
[in] obj The object [in] filled true
to make the fill property follow object size orfalse
otherwise.
- Since :
- 2.3.1
- Examples:
- ecore_evas_buffer_example_02.c, evas-images.c, evas-images2.c, and map_example_02.c.
double evas_object_image_load_dpi_get | ( | const Evas_Object * | obj | ) |
Get the DPI resolution of a loaded image object in the canvas.
This function returns the DPI resolution of the given canvas image.
- Parameters:
-
[in] obj The object
- Returns:
- The DPI resolution.
- Since :
- 2.3.1
void evas_object_image_load_dpi_set | ( | Evas_Object * | obj, |
double | dpi | ||
) |
Set the DPI resolution of an image object's source image.
This function sets the DPI resolution of a given loaded canvas image. Most useful for the SVG image loader.
- Parameters:
-
[in] obj The object [in] dpi The DPI resolution.
- Since :
- 2.3.1
Evas_Load_Error evas_object_image_load_error_get | ( | const Evas_Object * | obj | ) |
Retrieves a number representing any error that occurred during the last loading of the given image object's source image.
- Parameters:
-
[in] obj The object
- Returns:
- A value giving the last error that occurred. It should be one of the Evas_Load_Error values. EVAS_LOAD_ERROR_NONE is returned if there was no error.
- Since :
- 2.3.1
- Examples:
- evas-events.c, evas-images.c, and evas-object-manipulation.c.
Eina_Bool evas_object_image_load_head_skip_get | ( | const Evas_Object * | obj | ) |
Get the load option to skip header loads before preload.
This gets the head skip value set by evas_object_image_load_head_skip_set()
- Parameters:
-
[in] obj The object
- See also:
- evas_object_image_load_head_skip_set
- Since (EFL) :
- 1.19
void evas_object_image_load_head_skip_set | ( | Evas_Object * | obj, |
Eina_Bool | skip | ||
) |
Set a load option to skip initial header load and defer to preload.
This is meant to be used in conjunction with evas_object_image_file_set() and evas_object_image_preload() by deferring any header loading until a evas_object_image_preload() is issued making the file file set simply set up the file to refer to without any validation of its type or file existence or even inspecting the image header to get size or alpha channel flags etc. All of this will then be done as part of the preload stage.
- Parameters:
-
[in] obj The object
- Since (EFL) :
- 1.19
Eina_Bool evas_object_image_load_orientation_get | ( | const Evas_Object * | obj | ) |
Get if the orientation information in the image file should be honored.
- Parameters:
-
[in] obj The object
- Returns:
true
means that it should honor the orientation information.
- Since (EFL) :
- 1.1
- Since :
- 2.3.1
void evas_object_image_load_orientation_set | ( | Evas_Object * | obj, |
Eina_Bool | enable | ||
) |
Define if the orientation information in the image file should be honored.
- Parameters:
-
[in] obj The object [in] enable true
means that it should honor the orientation information.
- Since (EFL) :
- 1.1
- Since :
- 2.3.1
void evas_object_image_load_region_get | ( | const Evas_Object * | obj, |
int * | x, | ||
int * | y, | ||
int * | w, | ||
int * | h | ||
) |
Retrieve the coordinates of a given image object's selective (source image) load region.
- Note:
- Use
null
pointers on the coordinates you're not interested in: they'll be ignored by the function.
- Parameters:
-
[in] obj The object [out] x X-offset of the region to be loaded. [out] y Y-offset of the region to be loaded. [out] w Width of the region to be loaded. [out] h Height of the region to be loaded.
- Since :
- 2.3.1
void evas_object_image_load_region_set | ( | Evas_Object * | obj, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h | ||
) |
Inform a given image object to load a selective region of its source image.
This function is useful when one is not showing all of an image's area on its image object.
- Note:
- The image loader for the image format in question has to support selective region loading in order to this function to take effect.
- Parameters:
-
[in] obj The object [in] x X-offset of the region to be loaded. [in] y Y-offset of the region to be loaded. [in] w Width of the region to be loaded. [in] h Height of the region to be loaded.
- Since :
- 2.3.1
int evas_object_image_load_scale_down_get | ( | const Evas_Object * | obj | ) |
Get the scale down factor of a given image object's source image, when loading it.
- Parameters:
-
[in] obj The object
- Returns:
- The scale down factor.
- Since :
- 2.3.1
void evas_object_image_load_scale_down_set | ( | Evas_Object * | obj, |
int | scale_down | ||
) |
Set the scale down factor of a given image object's source image, when loading it.
This function sets the scale down factor of a given canvas image. Most useful for the SVG image loader.
- Parameters:
-
[in] obj The object [in] scale_down The scale down factor.
- Since :
- 2.3.1
void evas_object_image_load_size_get | ( | const Eo * | obj, |
int * | w, | ||
int * | h | ||
) |
Get the load size of a given image object's source image.
This function gets the geometry size set manually for the given canvas image.
- Note:
- Use
NULL
pointers on the size components you're not interested in: they'll be ignored by the function. -
w
andh
will be set with the image's loading size only if the image's load size is set manually: if evas_object_image_load_size_set() has not been called,w
andh
will be set with 0.
- See also:
- evas_object_image_load_size_set() for more details
- Parameters:
-
[in] obj The object [out] w The new width of the image's load size. [out] h The new height of the image's load size.
- Since :
- 2.3.1
void evas_object_image_load_size_set | ( | Eo * | obj, |
int | w, | ||
int | h | ||
) |
Set the load size of a given image object's source image.
This function sets a new geometry size for the given canvas image. The image will be loaded into memory as if it was the set size instead of the original size.
- Note:
- The size of a given image object's source image will be less than or equal to the size of
w
andh
.
- See also:
- evas_object_image_load_size_get()
- Parameters:
-
[in] obj The object [in] w The new width of the image's load size. [in] h The new height of the image's load size.
- Since :
- 2.3.1
void evas_object_image_memfile_set | ( | Evas_Object * | obj, |
void * | data, | ||
int | size, | ||
char * | format, | ||
char * | key | ||
) |
Sets the data for an image from memory to be loaded
This is the same as evas_object_image_file_set() but the file to be loaded may exist at an address in memory (the data for the file, not the filename itself). The data
at the address is copied and stored for future use, so no data
needs to be kept after this call is made. It will be managed and freed for you when no longer needed. The size
is limited to 2 gigabytes in size, and must be greater than 0. A NULL
data
pointer is also invalid. Set the filename to NULL
to reset to empty state and have the image file data freed from memory using evas_object_image_file_set().
The format
is optional (pass NULL
if you don't need/use it). It is used to help Evas guess better which loader to use for the data. It may simply be the "extension" of the file as it would normally be on disk such as "jpg" or "png" or "gif" etc.
- Parameters:
-
obj The given image object. data The image file data address size The size of the image file data in bytes format The format of the file (optional), or NULL
if not neededkey The image key in file, or NULL
.
- Since :
- 2.3.1
void evas_object_image_mmap_get | ( | const Eo * | obj, |
const Eina_File ** | f, | ||
const char ** | key | ||
) |
Get the source mmaped file from where an image object must fetch the real image data (it must be an Eina_File).
If the file supports multiple data stored in it (as Eet files do), you can get the key to be used as the index of the image in this file.
- Since (EFL) :
- 1.10
- Parameters:
-
[in] obj The object [out] f The mmaped file [out] key The image key in file
(if its an Eet one), orNULL
, otherwise.
- Since :
- 3.0
void evas_object_image_mmap_set | ( | Eo * | obj, |
const Eina_File * | f, | ||
const char * | key | ||
) |
Set the source mmaped file from where an image object must fetch the real image data (it must be an Eina_File).
If the file supports multiple data stored in it (as Eet files do), you can specify the key to be used as the index of the image in this file.
- Since (EFL) :
- 1.8
- Parameters:
-
[in] obj The object [in] f The mmaped file [in] key The image key in file
(if its an Eet one), orNULL
, otherwise.
- Since :
- 3.0
Evas_Native_Surface* evas_object_image_native_surface_get | ( | const Evas_Object * | obj | ) |
Get the native surface of a given image of the canvas.
This function returns the native surface of a given canvas image.
- Returns:
- The native surface.
- Since :
- 2.3.1
void evas_object_image_native_surface_set | ( | Evas_Object * | obj, |
Evas_Native_Surface * | surf | ||
) |
Set the native surface of a given image of the canvas
- Parameters:
-
obj The given canvas pointer. surf The new native surface.
This function sets a native surface of a given canvas image.
- Since :
- 2.3.1
Eina_Bool evas_object_image_pixels_dirty_get | ( | const Evas_Object * | obj | ) |
Retrieves whether the given image object is dirty (needs to be redrawn).
- Parameters:
-
[in] obj The object
- Returns:
- Whether the image is dirty.
- Since :
- 2.3.1
void evas_object_image_pixels_dirty_set | ( | Evas_Object * | obj, |
Eina_Bool | dirty | ||
) |
Mark whether the given image object is dirty and needs to request its pixels.
This function will only properly work if a pixels get callback has been set.
- Warning:
- Use this function if you really know what you are doing.
- Parameters:
-
[in] obj The object [in] dirty Whether the image is dirty.
- Since :
- 2.3.1
void evas_object_image_pixels_get_callback_set | ( | Evas_Object * | obj, |
Evas_Object_Image_Pixels_Get_Cb | func, | ||
void * | data | ||
) |
Set the callback function to get pixels from a canvas' image.
This functions sets a function to be the callback function that get pixels from a image of the canvas.
- Parameters:
-
[in] obj The object [in] func The callback function. [in] data The data pointer to be passed to func
.
- Since :
- 2.3.1
Eina_Bool evas_object_image_pixels_import | ( | Evas_Object * | obj, |
Evas_Pixel_Import_Source * | pixels | ||
) |
- Deprecated:
- evas_object_image_pixels_import
- Since :
- 2.3.1
void evas_object_image_preload | ( | Evas_Object * | obj, |
Eina_Bool | cancel | ||
) |
Preload an image object's image data in the background
- Parameters:
-
obj The given image object. cancel EINA_FALSE
will add it the preloading work queue,EINA_TRUE
will remove it (if it was issued before).
This function requests the preload of the data image in the background. The work is queued before being processed (because there might be other pending requests of this type).
Whenever the image data gets loaded, Evas will call #EVAS_CALLBACK_IMAGE_PRELOADED registered callbacks on obj
(what may be immediately, if the data was already preloaded before).
Use EINA_TRUE
for cancel
on scenarios where you don't need the image data preloaded anymore.
- Note:
- Any evas_object_show() call after evas_object_image_preload() will make the latter to be cancelled, with the loading process now taking place synchronously (and, thus, blocking the return of the former until the image is loaded). It is highly advisable, then, that the user preload an image with it being hidden, just to be shown on the #EVAS_CALLBACK_IMAGE_PRELOADED event's callback.
- Since :
- 2.3.1
- Examples:
- evas-images2.c.
void evas_object_image_reload | ( | Evas_Object * | obj | ) |
- Deprecated:
- evas_object_image_reload
- Since :
- 2.3.1
Eina_Bool evas_object_image_save | ( | const Eo * | obj, |
const char * | file, | ||
const char * | key, | ||
const char * | flags | ||
) |
Save the given image object's contents to an (image) file. Proxy object is image object, but it doesn't have contents. So you can't use this function for proxy objects.
The extension suffix on file
will determine which saver module Evas is to use when saving, thus the final file's format. If the file supports multiple data stored in it (Eet ones), you can specify the key to be used as the index of the image in it.
You can specify some flags when saving the image. Currently acceptable flags are quality
and compress
. Eg.: "quality=100 compress=9"
quality is hint for the quality of image,0-100. 0 means low quality and saved image size is small. 100 means high quality and saved image size is big.
compress is hint for the compression modes (Eet ones) or for the compression flags (Png one) (1 == compress, 0 = don't compress).
- Parameters:
-
[in] obj The object [in] file The filename to be used to save the image (extension obligatory). [in] key The image key in the file (if an Eet one), or NULL
, otherwise.[in] flags String containing the flags to be used ( NULL
for none).
- Since :
- 2.3.1
- Examples:
- evas-images2.c.
void evas_object_image_size_get | ( | const Evas_Object * | obj, |
int * | w, | ||
int * | h | ||
) |
Retrieves the size of the given image object.
See evas_object_image_size_set() for more details.
- Parameters:
-
[in] obj The object [out] w The new width of the image. [out] h The new height of the image.
- Since :
- 2.3.1
- Examples:
- evas-map-utils.c.
void evas_object_image_size_set | ( | Evas_Object * | obj, |
int | w, | ||
int | h | ||
) |
Sets the size of the given image object.
This function will scale down or crop the image so that it is treated as if it were at the given size. If the size given is smaller than the image, it will be cropped. If the size given is larger, then the image will be treated as if it were in the upper left hand corner of a larger image that is otherwise transparent.
- Parameters:
-
[in] obj The object [in] w The new width of the image. [in] h The new height of the image.
- Since :
- 2.3.1
- Examples:
- ecore_evas_buffer_example_02.c, efl_thread_6.c, and evas-images2.c.
Eina_Bool evas_object_image_smooth_scale_get | ( | const Eo * | obj | ) |
Retrieves whether the given image object is using high-quality image scaling algorithm.
- Returns:
- Whether smooth scale is being used.
See evas_object_image_smooth_scale_set() for more details.
- Since :
- 2.3.1
- Examples:
- evas-images.c.
void evas_object_image_smooth_scale_set | ( | Eo * | obj, |
Eina_Bool | smooth_scale | ||
) |
Sets whether to use high-quality image scaling algorithm on the given image object.
When enabled, a higher quality image scaling algorithm is used when scaling images to sizes other than the source image's original one. This gives better results but is more computationally expensive.
- Note:
- Image objects get created originally with smooth scaling on.
- See also:
- evas_object_image_smooth_scale_get()
- Parameters:
-
[in] obj The object [in] smooth_scale Whether to use smooth scale or not.
- Since :
- 2.3.1
- Examples:
- evas-images.c.
Eina_Bool evas_object_image_snapshot_get | ( | const Evas_Object * | obj | ) |
Determine whether the Evas_Object_Image replicate the content of the canvas below.
- Parameters:
-
[in] obj The object
- Returns:
- Wether to put the content of the canvas below inside the Evas_Object_Image.
- Since (EFL) :
- 1.15
- Since :
- 3.0
void evas_object_image_snapshot_set | ( | Evas_Object * | obj, |
Eina_Bool | s | ||
) |
The content below the Evas_Object_Image will be rendered inside it and you can reuse it as a source for any kind of effect.
- Parameters:
-
[in] obj The object [in] s Whether to put the content of the canvas below inside the Evas_Object_Image.
- Since (EFL) :
- 1.15
- Since :
- 3.0
Eina_Bool evas_object_image_source_clip_get | ( | const Evas_Object * | obj | ) |
Determine whether an object is clipped by source object's clipper.
- Parameters:
-
[in] obj The object
- Returns:
- Whether
obj
is clipped by the source clipper ($true) or not ($false).
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void evas_object_image_source_clip_set | ( | Evas_Object * | obj, |
Eina_Bool | source_clip | ||
) |
Clip the proxy object with the source object's clipper.
- Parameters:
-
[in] obj The object [in] source_clip Whether obj
is clipped by the source clipper ($true) or not ($false).
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
Eina_Bool evas_object_image_source_events_get | ( | const Evas_Object * | obj | ) |
Determine whether an object is set to source events.
- Parameters:
-
[in] obj The object
- Returns:
- Whether
obj
is to pass events ($true) or not ($false).
- Since (EFL) :
- 1.8
- Since :
- 3.0
- Examples:
- evas-images2.c.
void evas_object_image_source_events_set | ( | Evas_Object * | obj, |
Eina_Bool | repeat | ||
) |
Set whether an Evas object is to source events.
Set whether an Evas object is to repeat events to source.
If repeat
is true
, it will make events on obj
to also be repeated for the source object (see evas_object_image_source_set). Even the obj
and source geometries are different, the event position will be transformed to the source object's space.
If repeat
is false
, events occurring on obj
will be processed only on it.
- Parameters:
-
[in] obj The object [in] repeat Whether obj
is to pass events ($true) or not ($false).
- Since (EFL) :
- 1.8
- Since :
- 3.0
- Examples:
- evas-images2.c.
Evas_Object* evas_object_image_source_get | ( | const Evas_Object * | obj | ) |
Get the current source object of an image object.
- Parameters:
-
[in] obj The object
- Returns:
- Source object to use for the proxy.
- Since :
- 2.3.1
- Examples:
- evas-images2.c.
Eina_Bool evas_object_image_source_set | ( | Evas_Object * | obj, |
Evas_Object * | src | ||
) |
Set the source object on an image object to used as a proxy.
If an image object is set to behave as a proxy, it will mirror the rendering contents of a given source object in its drawing region, without affecting that source in any way. The source must be another valid Evas object. Other effects may be applied to the proxy, such as a map (see evas_object_map_set) to create a reflection of the original object (for example).
Any existing source object on obj
will be removed after this call. Setting src
to null
clears the proxy object (not in "proxy state" anymore).
- Warning:
- You cannot set a proxy as another proxy's source.
- Parameters:
-
[in] obj The object [in] src Source object to use for the proxy.
- Since :
- 2.3.1
- Examples:
- evas-images2.c, and evas-map-utils.c.
Clear the source object on a proxy image object.
- Parameters:
-
obj Image object to clear source of.
- Returns:
EINA_TRUE
on success,EINA_FALSE
on error.
This is equivalent to calling evas_object_image_source_set() with a NULL
source.
- Since :
- 2.3.1
Eina_Bool evas_object_image_source_visible_get | ( | const Evas_Object * | obj | ) |
Get the state of the source object visibility.
- Parameters:
-
[in] obj The object
- Returns:
true
is source object to be shown,false
otherwise.
- Deprecated:
- Please use evas_object_norender_get() on the source instead.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Examples:
- evas-images2.c.
void evas_object_image_source_visible_set | ( | Evas_Object * | obj, |
Eina_Bool | visible | ||
) |
Set the source object to be visible or not.
If visible
is set to false
, the source object of the proxy ($obj) will be invisible.
This API works differently to evas_object_show and evas_object_hide. Once source object is hidden, the proxy object will be hidden as well. Actually in this case both objects are excluded from the Evas internal update circle.
By this API, instead, one can toggle the visibility of a proxy's source object remaining the proxy visibility untouched.
- Warning:
- If the all of proxies are deleted, then the source visibility of the source object will be cancelled.
- Parameters:
-
[in] obj The object [in] visible true
is source object to be shown,false
otherwise.
- Deprecated:
- Please use evas_object_norender_get() on the source instead.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
- Examples:
- evas-images2.c.
unsigned int evas_object_image_video_surface_caps_get | ( | const Evas_Object * | obj | ) |
Get the video surface capabilities to a given image of the canvas.
- Parameters:
-
[in] obj The object
- Returns:
- Surface capabilities
- Since :
- 3.0
void evas_object_image_video_surface_caps_set | ( | Evas_Object * | obj, |
unsigned int | caps | ||
) |
Set the video surface capabilities to a given image of the canvas.
- Parameters:
-
[in] obj The object [in] caps Surface capabilities
- Since :
- 3.0
const Evas_Video_Surface* evas_object_image_video_surface_get | ( | const Evas_Object * | obj | ) |
Get the video surface linked to a given image of the canvas.
- Parameters:
-
[in] obj The object
- Returns:
- The new video surface.
- Since (EFL) :
- 1.1
- Since :
- 2.3.1
void evas_object_image_video_surface_set | ( | Evas_Object * | obj, |
Evas_Video_Surface * | surf | ||
) |
Set the video surface linked to a given image of the canvas.
- Parameters:
-
[in] obj The object [in] surf The new video surface.
- Since (EFL) :
- 1.1
- Since :
- 2.3.1