Tizen Native API
7.0
|
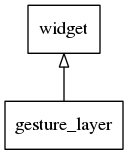
Gesture Layer Usage:
Use Gesture Layer to detect gestures. The advantage is that you don't have to implement gesture detection, just set callbacks of gesture state. By using gesture layer we make standard interface.
In order to use Gesture Layer you start with elm_gesture_layer_add with a parent object parameter. Next 'activate' gesture layer with a elm_gesture_layer_attach call. Usually with same object as target (2nd parameter).
Now you need to tell gesture layer what gestures you follow. This is done with elm_gesture_layer_cb_set call. By setting the callback you actually saying to gesture layer: I would like to know when the gesture Elm_Gesture_Type switches to state Elm_Gesture_State.
Next, you need to implement the actual action that follows the input in your callback.
Note that if you like to stop being reported about a gesture, just set all callbacks referring this gesture to NULL. (again with elm_gesture_layer_cb_set)
The information reported by gesture layer to your callback is depending on Elm_Gesture_Type : Elm_Gesture_Taps_Info is the info reported for tap gestures: ELM_GESTURE_N_TAPS, ELM_GESTURE_N_LONG_TAPS, ELM_GESTURE_N_DOUBLE_TAPS, ELM_GESTURE_N_TRIPLE_TAPS.
Elm_Gesture_Momentum_Info is info reported for momentum gestures: ELM_GESTURE_MOMENTUM.
Elm_Gesture_Line_Info is the info reported for line gestures: (this also contains Elm_Gesture_Momentum_Info internal structure) ELM_GESTURE_N_LINES, ELM_GESTURE_N_FLICKS. Note that we consider a flick as a line-gesture that should be completed in flick-time-limit as defined in Config.
Elm_Gesture_Zoom_Info is the info reported for ELM_GESTURE_ZOOM gesture.
Elm_Gesture_Rotate_Info is the info reported for ELM_GESTURE_ROTATE gesture.
Gesture Layer Tweaks:
Note that line, flick, gestures can start without the need to remove fingers from surface. When user fingers rests on same-spot gesture is ended and starts again when fingers moved.
Setting glayer_continues_enable to false in Config will change this behavior so gesture starts when user touches (a *DOWN event) touch-surface and ends when no fingers touches surface (a *UP event).
Supported elm_object common APIs.
Functions | |
double | elm_config_glayer_long_tap_start_timeout_get (void) |
void | elm_config_glayer_long_tap_start_timeout_set (double long_tap_timeout) |
double | elm_config_glayer_double_tap_timeout_get (void) |
void | elm_config_glayer_double_tap_timeout_set (double double_tap_timeout) |
void | elm_gesture_layer_line_min_length_set (Evas_Object *obj, int line_min_length) |
int | elm_gesture_layer_line_min_length_get (const Evas_Object *obj) |
void | elm_gesture_layer_zoom_distance_tolerance_set (Evas_Object *obj, Evas_Coord zoom_distance_tolerance) |
Evas_Coord | elm_gesture_layer_zoom_distance_tolerance_get (const Evas_Object *obj) |
void | elm_gesture_layer_line_distance_tolerance_set (Evas_Object *obj, Evas_Coord line_distance_tolerance) |
Evas_Coord | elm_gesture_layer_line_distance_tolerance_get (const Evas_Object *obj) |
void | elm_gesture_layer_line_angular_tolerance_set (Evas_Object *obj, double line_angular_tolerance) |
double | elm_gesture_layer_line_angular_tolerance_get (const Evas_Object *obj) |
void | elm_gesture_layer_zoom_wheel_factor_set (Evas_Object *obj, double zoom_wheel_factor) |
double | elm_gesture_layer_zoom_wheel_factor_get (const Evas_Object *obj) |
void | elm_gesture_layer_zoom_finger_factor_set (Evas_Object *obj, double zoom_finger_factor) |
double | elm_gesture_layer_zoom_finger_factor_get (const Evas_Object *obj) |
void | elm_gesture_layer_rotate_angular_tolerance_set (Evas_Object *obj, double rotate_angular_tolerance) |
double | elm_gesture_layer_rotate_angular_tolerance_get (const Evas_Object *obj) |
void | elm_gesture_layer_flick_time_limit_ms_set (Evas_Object *obj, unsigned int flick_time_limit_ms) |
unsigned int | elm_gesture_layer_flick_time_limit_ms_get (const Evas_Object *obj) |
void | elm_gesture_layer_long_tap_start_timeout_set (Evas_Object *obj, double long_tap_start_timeout) |
double | elm_gesture_layer_long_tap_start_timeout_get (const Evas_Object *obj) |
void | elm_gesture_layer_continues_enable_set (Evas_Object *obj, Eina_Bool continues_enable) |
Eina_Bool | elm_gesture_layer_continues_enable_get (const Evas_Object *obj) |
void | elm_gesture_layer_double_tap_timeout_set (Evas_Object *obj, double double_tap_timeout) |
double | elm_gesture_layer_double_tap_timeout_get (const Evas_Object *obj) |
void | elm_gesture_layer_tap_finger_size_set (Evas_Object *obj, Evas_Coord sz) |
Evas_Coord | elm_gesture_layer_tap_finger_size_get (const Evas_Object *obj) |
void | elm_gesture_layer_zoom_step_set (Elm_Gesture_Layer *obj, double step) |
Control step value for zoom action. | |
double | elm_gesture_layer_zoom_step_get (const Elm_Gesture_Layer *obj) |
Control step value for zoom action. | |
void | elm_gesture_layer_tap_finger_size_set (Elm_Gesture_Layer *obj, int sz) |
This function sets the gesture layer finger-size for taps. | |
int | elm_gesture_layer_tap_finger_size_get (const Elm_Gesture_Layer *obj) |
This function returns the gesture layer finger-size for taps. | |
void | elm_gesture_layer_hold_events_set (Elm_Gesture_Layer *obj, Eina_Bool hold_events) |
This function makes gesture-layer repeat events. | |
Eina_Bool | elm_gesture_layer_hold_events_get (const Elm_Gesture_Layer *obj) |
Get the repeat-events setting. | |
void | elm_gesture_layer_rotate_step_set (Elm_Gesture_Layer *obj, double step) |
This function returns step-value for rotate action. | |
double | elm_gesture_layer_rotate_step_get (const Elm_Gesture_Layer *obj) |
This function returns step-value for rotate action. | |
void | elm_gesture_layer_cb_set (Elm_Gesture_Layer *obj, Elm_Gesture_Type idx, Elm_Gesture_State cb_type, Elm_Gesture_Event_Cb cb, void *data) |
Set the gesture state change callback. | |
Eina_Bool | elm_gesture_layer_attach (Elm_Gesture_Layer *obj, Efl_Canvas_Object *target) |
Attach a gesture layer widget to an Evas object (setting the widget's target). | |
void | elm_gesture_layer_cb_del (Elm_Gesture_Layer *obj, Elm_Gesture_Type idx, Elm_Gesture_State cb_type, Elm_Gesture_Event_Cb cb, void *data) |
Remove a gesture callback. | |
void | elm_gesture_layer_cb_add (Elm_Gesture_Layer *obj, Elm_Gesture_Type idx, Elm_Gesture_State cb_type, Elm_Gesture_Event_Cb cb, void *data) |
Add a gesture state change callback. | |
Evas_Object * | elm_gesture_layer_add (Evas_Object *parent) |
void | elm_gesture_layer_tap_longpress_cb_add (Evas_Object *obj, Elm_Gesture_State state, Elm_Gesture_Event_Cb cb, void *data) |
void | elm_gesture_layer_tap_longpress_cb_del (Evas_Object *obj, Elm_Gesture_State state, Elm_Gesture_Event_Cb cb, void *data) |
Typedefs | |
typedef struct _Elm_Gesture_Taps_Info | Elm_Gesture_Taps_Info |
typedef struct _Elm_Gesture_Momentum_Info | Elm_Gesture_Momentum_Info |
typedef struct _Elm_Gesture_Line_Info | Elm_Gesture_Line_Info |
typedef struct _Elm_Gesture_Zoom_Info | Elm_Gesture_Zoom_Info |
typedef struct _Elm_Gesture_Rotate_Info | Elm_Gesture_Rotate_Info |
typedef Evas_Event_Flags(* | Elm_Gesture_Event_Cb )(void *data, void *event_info) |
Typedef Documentation
typedef Evas_Event_Flags(* Elm_Gesture_Event_Cb)(void *data, void *event_info) |
User callback used to stream gesture info from gesture layer
- Parameters:
-
data user data event_info gesture report info Returns a flag field to be applied on the causing event. You should probably return EVAS_EVENT_FLAG_ON_HOLD if your widget acted upon the event, in an irreversible way.
typedef struct _Elm_Gesture_Line_Info Elm_Gesture_Line_Info |
Holds line info for user
typedef struct _Elm_Gesture_Momentum_Info Elm_Gesture_Momentum_Info |
holds momentum info for user
typedef struct _Elm_Gesture_Rotate_Info Elm_Gesture_Rotate_Info |
Holds rotation info for user
typedef struct _Elm_Gesture_Taps_Info Elm_Gesture_Taps_Info |
Holds taps info for user
typedef struct _Elm_Gesture_Zoom_Info Elm_Gesture_Zoom_Info |
Holds zoom info for user
Function Documentation
double elm_config_glayer_double_tap_timeout_get | ( | void | ) |
Get the duration for occurring double tap event of gesture layer.
- Returns:
- Timeout for double tap event of gesture layer.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_config_glayer_double_tap_timeout_set | ( | double | double_tap_timeout | ) |
Set the duration for occurring double tap event of gesture layer.
- Parameters:
-
double_tap_timeout Timeout for double tap event of gesture layer.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
double elm_config_glayer_long_tap_start_timeout_get | ( | void | ) |
Get the duration for occurring long tap event of gesture layer.
- Returns:
- Timeout for long tap event of gesture layer.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_config_glayer_long_tap_start_timeout_set | ( | double | long_tap_timeout | ) |
Set the duration for occurring long tap event of gesture layer.
- Parameters:
-
long_tap_timeout Timeout for long tap event of gesture layer.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
Evas_Object* elm_gesture_layer_add | ( | Evas_Object * | parent | ) |
Call this function to construct a new gesture-layer object.
- Parameters:
-
parent The gesture layer's parent widget.
- Returns:
- A new gesture layer object.
This does not activate the gesture layer. You have to call elm_gesture_layer_attach() in order to 'activate' gesture-layer.
- Since :
- 2.3.1
Eina_Bool elm_gesture_layer_attach | ( | Elm_Gesture_Layer * | obj, |
Efl_Canvas_Object * | target | ||
) |
Attach a gesture layer widget to an Evas object (setting the widget's target).
A gesture layer's target may be any Evas object. This object will be used to listen to mouse and key events.
- Parameters:
-
[in] obj The object. [in] target The object to attach.
- Returns:
true
on success,false
otherwise.
- Since :
- 2.3.1
void elm_gesture_layer_cb_add | ( | Elm_Gesture_Layer * | obj, |
Elm_Gesture_Type | idx, | ||
Elm_Gesture_State | cb_type, | ||
Elm_Gesture_Event_Cb | cb, | ||
void * | data | ||
) |
Add a gesture state change callback.
When all callbacks for the gesture are set to null
, it means this gesture is disabled.
If a function was already set for this gesture/type/state, it will be replaced by the new one. For ABI compat, callbacks added by elm_gesture_layer_cb_add will be removed. It is recommended to use only one of these functions for a gesture object.
- Parameters:
-
[in] obj The object. [in] idx The gesture you want to track state of. [in] cb_type The event the callback tracks (START, MOVE, END, ABORT). [in] cb The callback itself. [in] data Custom data to be passed.
- Since :
- 3.0
void elm_gesture_layer_cb_del | ( | Elm_Gesture_Layer * | obj, |
Elm_Gesture_Type | idx, | ||
Elm_Gesture_State | cb_type, | ||
Elm_Gesture_Event_Cb | cb, | ||
void * | data | ||
) |
Remove a gesture callback.
- Parameters:
-
[in] obj The object. [in] idx The gesture you want to track state of. [in] cb_type The event the callback tracks (START, MOVE, END, ABORT). [in] cb The callback itself. [in] data Custom callback data.
- Since :
- 3.0
void elm_gesture_layer_cb_set | ( | Elm_Gesture_Layer * | obj, |
Elm_Gesture_Type | idx, | ||
Elm_Gesture_State | cb_type, | ||
Elm_Gesture_Event_Cb | cb, | ||
void * | data | ||
) |
Set the gesture state change callback.
When all callbacks for the gesture are set to null
, it means this gesture is disabled.
- Parameters:
-
[in] obj The object. [in] idx The gesture you want to track state of. [in] cb_type The event the callback tracks (START, MOVE, END, ABORT). [in] cb The callback itself. [in] data Custom data to be passed.
- Since :
- 2.3.1
Eina_Bool elm_gesture_layer_continues_enable_get | ( | const Evas_Object * | obj | ) |
This function returns the gesture layer continues enable of an object
- Parameters:
-
obj gesture-layer.
- Returns:
- continues enable
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_continues_enable_set | ( | Evas_Object * | obj, |
Eina_Bool | continues_enable | ||
) |
This function sets the gesture layer continues enable of an object
An option that allows user to start a gesture even when user is in touch move state.
- Parameters:
-
obj gesture-layer. continues_enable continues enable
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
double elm_gesture_layer_double_tap_timeout_get | ( | const Evas_Object * | obj | ) |
this function returns the gesture layer double tap timeout of an object
- Parameters:
-
obj gesture-layer.
- Returns:
- double tap timeout
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_double_tap_timeout_set | ( | Evas_Object * | obj, |
double | double_tap_timeout | ||
) |
This function sets the gesture layer double tap timeout of an object
When the time difference between two tabs is less than the double_tap_timeout
value, it is recognized as a double tab, if set negative value, timeout will be 0.
- Parameters:
-
obj gesture-layer. double_tap_timeout double tap timeout
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
unsigned int elm_gesture_layer_flick_time_limit_ms_get | ( | const Evas_Object * | obj | ) |
This function returns the gesture layer flick time limit (in ms) of an object
- Parameters:
-
obj gesture-layer.
- Returns:
- flick time limit (in ms)
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_flick_time_limit_ms_set | ( | Evas_Object * | obj, |
unsigned int | flick_time_limit_ms | ||
) |
This function sets the gesture layer flick time limit (in ms) of an object
Recognize as a flick when a gesture occurs over flick_time_limit_ms
.
- Parameters:
-
obj gesture-layer. flick_time_limit_ms flick time limit (in ms)
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
Eina_Bool elm_gesture_layer_hold_events_get | ( | const Elm_Gesture_Layer * | obj | ) |
Get the repeat-events setting.
- Parameters:
-
[in] obj The object.
- Returns:
- If
true
get events only if gesture was not detected,false
otherwise
- Since :
- 2.3.1
void elm_gesture_layer_hold_events_set | ( | Elm_Gesture_Layer * | obj, |
Eina_Bool | hold_events | ||
) |
This function makes gesture-layer repeat events.
Set this if you like to get the raw events only if gestures were not detected.
Clear this if you like gesture layer to forward events as testing gestures.
- Parameters:
-
[in] obj The object. [in] hold_events If true
get events only if gesture was not detected,false
otherwise
- Since :
- 2.3.1
double elm_gesture_layer_line_angular_tolerance_get | ( | const Evas_Object * | obj | ) |
This function returns the gesture layer line angular tolerance of an object
- Parameters:
-
obj gesture-layer.
- Returns:
- line angular tolerance
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_line_angular_tolerance_set | ( | Evas_Object * | obj, |
double | line_angular_tolerance | ||
) |
This function sets the gesture layer line angular tolerance of an object
Sets the minimum line_angular_tolerance
angle for the gesture to be recognized as a line.
- Parameters:
-
obj gesture-layer. line_angular_tolerance line angular tolerance
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
Evas_Coord elm_gesture_layer_line_distance_tolerance_get | ( | const Evas_Object * | obj | ) |
This function returns the gesture layer line distance tolerance of an object
- Parameters:
-
obj gesture-layer.
- Returns:
- line distance tolerance
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_line_distance_tolerance_set | ( | Evas_Object * | obj, |
Evas_Coord | line_distance_tolerance | ||
) |
This function sets the gesture layer line distance tolerance of an object
Sets the minimum line_distance_tolerance
length for the gesture to be recognized as a line.
- Parameters:
-
obj gesture-layer. line_distance_tolerance line distance tolerance
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
int elm_gesture_layer_line_min_length_get | ( | const Evas_Object * | obj | ) |
This function returns the gesture layer line min length of an object
- Parameters:
-
obj gesture-layer.
- Returns:
- the length.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_line_min_length_set | ( | Evas_Object * | obj, |
int | line_min_length | ||
) |
This function sets the gesture layer line min length of an object
Sets the minimum line length at which the user's gesture will be recognized as a line.
- Parameters:
-
obj gesture-layer. line_min_length the length.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
double elm_gesture_layer_long_tap_start_timeout_get | ( | const Evas_Object * | obj | ) |
this function returns the gesture layer long tap start timeout of an object
- Parameters:
-
obj gesture-layer.
- Returns:
- long tap start timeout
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_long_tap_start_timeout_set | ( | Evas_Object * | obj, |
double | long_tap_start_timeout | ||
) |
This function sets the gesture layer long tap start timeout of an object
Recognize as a long tap when a tab occurs over long_tap_start_timeout
, if set negative value, timeout will be 0.
- Parameters:
-
obj gesture-layer. long_tap_start_timeout long tap start timeout
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
double elm_gesture_layer_rotate_angular_tolerance_get | ( | const Evas_Object * | obj | ) |
This function returns the gesture layer rotate angular tolerance of an object
- Parameters:
-
obj gesture-layer.
- Returns:
- rotate angular tolerance
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_rotate_angular_tolerance_set | ( | Evas_Object * | obj, |
double | rotate_angular_tolerance | ||
) |
This function sets the gesture layer rotate angular tolerance of an object
Sets the minimum rotate_angular_tolerance
angle for the gesture to be recognized as a rotate. Rotate should be rotated beyond the givin rotate_angular_tolerance
to recognize the start of the rotate gesture.
- Parameters:
-
obj gesture-layer. rotate_angular_tolerance rotate angular tolerance
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
double elm_gesture_layer_rotate_step_get | ( | const Elm_Gesture_Layer * | obj | ) |
This function returns step-value for rotate action.
- Parameters:
-
[in] obj The object.
- Returns:
- New rotate step value.
- Since :
- 2.3.1
void elm_gesture_layer_rotate_step_set | ( | Elm_Gesture_Layer * | obj, |
double | step | ||
) |
This function returns step-value for rotate action.
Set to 0 to cancel step setting.
When recognizing the rotate gesture, the rotated size must be larger than step.
- Parameters:
-
[in] obj The object. [in] step New rotate step value.
- Since :
- 2.3.1
int elm_gesture_layer_tap_finger_size_get | ( | const Elm_Gesture_Layer * | obj | ) |
This function returns the gesture layer finger-size for taps.
- Parameters:
-
[in] obj The object.
- Returns:
- The finger size.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
Evas_Coord elm_gesture_layer_tap_finger_size_get | ( | const Evas_Object * | obj | ) |
This function returns the gesture layer finger-size for taps
- Parameters:
-
obj gesture-layer.
- Returns:
- Finger size that is currently used by Gesture Layer for taps.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_tap_finger_size_set | ( | Elm_Gesture_Layer * | obj, |
int | sz | ||
) |
This function sets the gesture layer finger-size for taps.
If not set, it's taken from elm_config. Set to 0 if you want GLayer to use the system finger size value (default).
- Parameters:
-
[in] obj The object. [in] sz The finger size.
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_tap_finger_size_set | ( | Evas_Object * | obj, |
Evas_Coord | sz | ||
) |
This function sets the gesture layer finger-size for taps If not set, this size taken from elm_config. Set to ZERO if you want GLayer to use system finger size value (default)
- Parameters:
-
obj gesture-layer. sz Finger size
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_tap_longpress_cb_add | ( | Evas_Object * | obj, |
Elm_Gesture_State | state, | ||
Elm_Gesture_Event_Cb | cb, | ||
void * | data | ||
) |
This function adds a callback called during Tap + Long Tap sequence.
- Parameters:
-
state state for the callback to add. cb callback pointer data user data for the callback.
The callbacks will be called as followed:
- start cbs on single tap start
- move cbs on long press move
- end cbs on long press end
- abort cbs whenever in the sequence. The event info will be NULL, because it can be triggered from multiple events (timer expired, abort single/long taps).
You can remove the callbacks by using elm_gesture_layer_tap_longpress_cb_del.
- Since (EFL) :
- 1.8
- Since :
- 3.0
void elm_gesture_layer_tap_longpress_cb_del | ( | Evas_Object * | obj, |
Elm_Gesture_State | state, | ||
Elm_Gesture_Event_Cb | cb, | ||
void * | data | ||
) |
This function removes a callback called during Tap + Long Tap sequence.
- Parameters:
-
state state for the callback to add. cb callback pointer data user data for the callback.
The internal data used for the sequence will be freed ONLY when all the callbacks added via elm_gesture_layer_tap_longpress_cb_add are removed by this function.
- Since (EFL) :
- 1.8
- Since :
- 3.0
Evas_Coord elm_gesture_layer_zoom_distance_tolerance_get | ( | const Evas_Object * | obj | ) |
This function returns the gesture layer zoom distance tolerance of an object
- Parameters:
-
obj gesture-layer.
- Returns:
- zoom distance tolerance
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_zoom_distance_tolerance_set | ( | Evas_Object * | obj, |
Evas_Coord | zoom_distance_tolerance | ||
) |
This function sets the gesture layer zoom distance tolerance of an object
If the distance between the two tab events becomes larger or smaller than zoom_distance_tolerance
, it is recognized as the start of the zoom gesture.
- Parameters:
-
obj gesture-layer. zoom_distance_tolerance zoom distance tolerance
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
double elm_gesture_layer_zoom_finger_factor_get | ( | const Evas_Object * | obj | ) |
This function returns the gesture layer zoom finger factor of an object
- Parameters:
-
obj gesture-layer.
- Returns:
- zoom finger factor
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_zoom_finger_factor_set | ( | Evas_Object * | obj, |
double | zoom_finger_factor | ||
) |
This function sets the gesture layer zoom finger factor of an object
Sets the zoom size by multiplaying the zoom value by zoom_finger_factor
when zooming.
- Parameters:
-
obj gesture-layer. zoom_finger_factor zoom finger factor
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
double elm_gesture_layer_zoom_step_get | ( | const Elm_Gesture_Layer * | obj | ) |
Control step value for zoom action.
- Parameters:
-
[in] obj The object.
- Returns:
- The zoom step value.
- Since :
- 2.3.1
void elm_gesture_layer_zoom_step_set | ( | Elm_Gesture_Layer * | obj, |
double | step | ||
) |
Control step value for zoom action.
When recognizing the zoom gesture, it should be recognized as zooming larger than step.
- Parameters:
-
[in] obj The object. [in] step The zoom step value.
- Since :
- 2.3.1
double elm_gesture_layer_zoom_wheel_factor_get | ( | const Evas_Object * | obj | ) |
This function returns the gesture layer zoom wheel factor of an object
- Parameters:
-
obj gesture-layer.
- Returns:
- zoom wheel factor
- Since (EFL) :
- 1.8
- Since :
- 2.3.1
void elm_gesture_layer_zoom_wheel_factor_set | ( | Evas_Object * | obj, |
double | zoom_wheel_factor | ||
) |
This function sets the gesture layer zoom wheel factor of an object
Sets the zoom size when a mouse wheel event occurs.
- Parameters:
-
obj gesture-layer. zoom_wheel_factor zoom wheel factor
- Since (EFL) :
- 1.8
- Since :
- 2.3.1