Tizen Native API
5.0
|
Here are grouped together functions used to create and manipulate image objects. They are available to whichever occasion one needs complex imagery on a GUI that could not be achieved by the other Evas' primitive object types, or to make image manipulations.
Evas will support whatever image file types it was compiled with support to (its image loaders) -- check your software packager for that information and see evas_object_image_extension_can_load_get().
Image object basics
The most common use of image objects -- to display an image on the canvas -- is achieved by a common function triplet:
img = evas_object_image_add(canvas); evas_object_image_file_set(img, "path/to/img", NULL); evas_object_image_fill_set(img, 0, 0, w, h);
The first function, naturally, is creating the image object. Then, one must set a source file on it, so that it knows where to fetch image data from. Next, one must set how to fill the image object's area with that given pixel data. One could use just a sub-region of the original image or even have it tiled repeatedly on the image object. For the common case of having the whole source image to be displayed on the image object, stretched to the destination's size, there's also a function helper, to be used instead of evas_object_image_fill_set():
evas_object_image_filled_set(img, EINA_TRUE);
See those functions' documentation for more details.
Scale and resizing
Resizing of image objects will scale their respective source images to their areas, if they are set to "fill" the object's area (evas_object_image_filled_set()). If the user wants any control on the aspect ratio of an image for different sizes, he/she has to take care of that themselves. There are functions to make images to get loaded scaled (up or down) in memory, already, if the user is going to use them at pre-determined sizes and wants to save computations.
Evas has even a scale cache that will take care of caching scaled versions of images with more often usage/hits. Finally, one can have images being rescaled smoothly by Evas (more computationally expensive) or not.
Performance hints
When dealing with image objects, there are some tricks to boost the performance of your application, if it does intense image loading and/or manipulations, as in animations on a UI.
Load hints
In image viewer applications, for example, the user will be looking at a given image, at full size, and will desire that the navigation to the adjacent images on his/her album be fluid and fast. Thus, while displaying a given image, the program can be in the background loading the next and previous images already, so that displaying them in sequence is just a matter of repainting the screen (and not decoding image data).
Evas addresses this issue with image pre-loading. The code for the situation above would be something like the following:
prev = evas_object_image_filled_add(canvas); evas_object_image_file_set(prev, "/path/to/prev", NULL); evas_object_image_preload(prev, EINA_TRUE); next = evas_object_image_filled_add(canvas); evas_object_image_file_set(next, "/path/to/next", NULL); evas_object_image_preload(next, EINA_TRUE);
If you're loading images that are too big, consider setting previously it's loading size to something smaller, in case you won't expose them in real size. It may speed up the loading considerably:
//to load a scaled down version of the image in memory, if that's //the size you'll be displaying it anyway evas_object_image_load_scale_down_set(img, zoom); //optional: if you know you'll be showing a sub-set of the image's //pixels, you can avoid loading the complementary data evas_object_image_load_region_set(img, x, y, w, h);
Refer to Elementary's Photocam widget for a high level (smart) object that does lots of loading speed-ups for you.
Animation hints
If you want to animate image objects on a UI (what you'd get by concomitant usage of other libraries, like Ecore and Edje), there are also some tips on how to boost the performance of your application. If the animation involves resizing of an image (thus, re-scaling), you'd better turn off smooth scaling on it during the animation, turning it back on afterwards, for less computations. Also, in this case you'd better flag the image object in question not to cache scaled versions of it:
evas_object_image_scale_hint_set(wd->img, EVAS_IMAGE_SCALE_HINT_DYNAMIC);
// resizing takes place in between
evas_object_image_scale_hint_set(wd->img, EVAS_IMAGE_SCALE_HINT_STATIC);
Finally, movement of opaque images through the canvas is less expensive than of translucid ones, because of blending computations.
Borders
Evas provides facilities for one to specify an image's region to be treated specially -- as "borders". This will make those regions be treated specially on resizing scales, by keeping their aspect. This makes setting frames around other objects on UIs easy. See the following figures for a visual explanation:
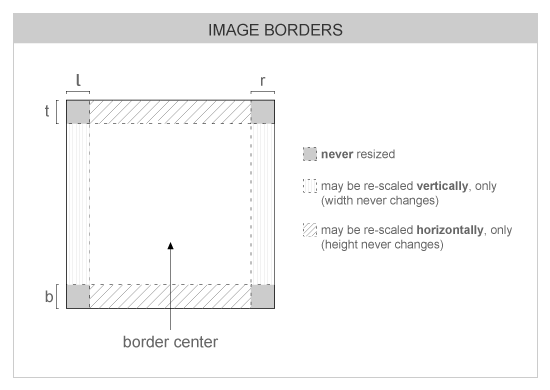
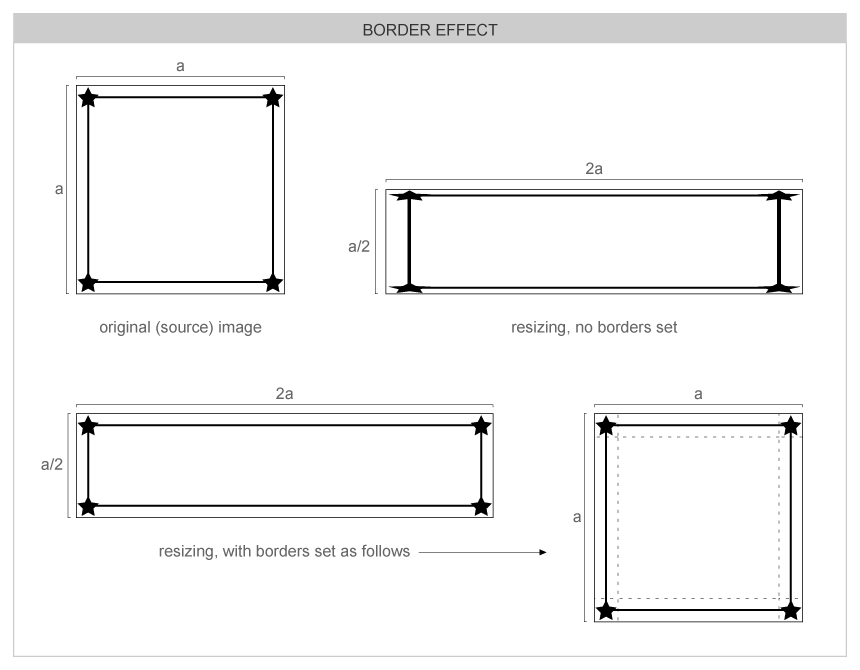
Manipulating pixels
Evas image objects can be used to manipulate raw pixels in many ways. The meaning of the data in the pixel arrays will depend on the image's color space, be warned (see next section). You can set your own data as an image's pixel data, fetch an image's pixel data for saving/altering, convert images between different color spaces and even advanced operations like setting a native surface as image objects' data.
Color spaces
Image objects may return or accept "image data" in multiple formats. This is based on the color space of an object. Here is a rundown on formats:
- #EVAS_COLORSPACE_ARGB8888: This pixel format is a linear block of pixels, starting at the top-left row by row until the bottom right of the image or pixel region. All pixels are 32-bit unsigned int's with the high-byte being alpha and the low byte being blue in the format ARGB. Alpha may or may not be used by evas depending on the alpha flag of the image, but if not used, should be set to 0xff anyway.
This colorspace uses premultiplied alpha. That means that R, G and B cannot exceed A in value. The conversion from non-premultiplied colorspace is:
R = (r * a) / 255; G = (g * a) / 255; B = (b * a) / 255;
So 50% transparent blue will be: 0x80000080. This will not be "dark" - just 50% transparent. Values are 0 == black, 255 == solid or full red, green or blue.
- #EVAS_COLORSPACE_YCBCR422P601_PL: This is a pointer-list indirected set of YUV (YCbCr) pixel data. This means that the data returned or set is not actual pixel data, but pointers TO lines of pixel data. The list of pointers will first be N rows of pointers to the Y plane - pointing to the first pixel at the start of each row in the Y plane. N is the height of the image data in pixels. Each pixel in the Y, U and V planes is 1 byte exactly, packed. The next N / 2 pointers will point to rows in the U plane, and the next N / 2 pointers will point to the V plane rows. U and V planes are half the horizontal and vertical resolution of the Y plane.
Row order is top to bottom and row pixels are stored left to right.
There is a limitation that these images MUST be a multiple of 2 pixels in size horizontally or vertically. This is due to the U and V planes being half resolution. Also note that this assumes the itu601 YUV colorspace specification. This is defined for standard television and mpeg streams. HDTV may use the itu709 specification.
Values are 0 to 255, indicating full or no signal in that plane respectively.
- #EVAS_COLORSPACE_YCBCR422P709_PL: Not implemented yet.
- #EVAS_COLORSPACE_RGB565_A5P: In the process of being implemented in 1 engine only. This may change.
This is a pointer to image data for 16-bit half-word pixel data in 16bpp RGB 565 format (5 bits red, 6 bits green, 5 bits blue), with the high-byte containing red and the low byte containing blue, per pixel. This data is packed row by row from the top-left to the bottom right.
If the image has an alpha channel enabled there will be an extra alpha plane after the color pixel plane. If not, then this data will not exist and should not be accessed in any way. This plane is a set of pixels with 1 byte per pixel defining the alpha values of all pixels in the image from the top-left to the bottom right of the image, row by row. Even though the values of the alpha pixels can be 0 to 255, only values 0 through to 32 are used, 32 being solid and 0 being transparent.
RGB values can be 0 to 31 for red and blue and 0 to 63 for green, with 0 being black and 31 or 63 being full red, green or blue respectively. This colorspace is also pre-multiplied like EVAS_COLORSPACE_ARGB8888 so:
R = (r * a) / 32; G = (g * a) / 32; B = (b * a) / 32;
- #EVAS_COLORSPACE_GRY8: The image is just an alpha mask (8 bit's per pixel). This is used for alpha masking.
- Warning:
- We don't guarantee any proper results if you create an Image object without setting the evas engine.
Some examples of this group of functions can be found here.
Functions | |
Eina_Bool | evas_object_image_extension_can_load_get (const char *file) |
Eina_Bool | evas_object_image_extension_can_load_fast_get (const char *file) |
Typedefs | |
typedef void(* | Evas_Object_Image_Pixels_Get_Cb )(void *data, Evas_Object *o) |
typedef Efl_Gfx_Colorspace | Evas_Colorspace |
Typedef Documentation
typedef Efl_Gfx_Colorspace Evas_Colorspace |
Colorspaces for pixel data supported by Evas Colorspaces for pixel data supported by Evas
typedef void(* Evas_Object_Image_Pixels_Get_Cb)(void *data, Evas_Object *o) |
Function signature for the evas object pixels get callback function
- See also:
- evas_object_image_pixels_get()
By data it will be passed the private data. By o it will be passed the Evas_Object image on which the pixels are requested.
Function Documentation
Evas_Object* evas_object_image_add | ( | Evas * | e | ) |
Creates a new image object on the given Evas e
canvas.
- Parameters:
-
e The given canvas.
- Returns:
- The created image object handle.
- Note:
- If you intend to display an image somehow in a GUI, besides binding it to a real image file/source (with evas_object_image_file_set(), for example), you'll have to tell this image object how to fill its space with the pixels it can get from the source. See evas_object_image_filled_add(), for a helper on the common case of scaling up an image source to the whole area of the image object.
- See also:
- evas_object_image_fill_set()
Example:
img = evas_object_image_add(canvas); evas_object_image_file_set(img, "/path/to/img", NULL);
- Since :
- 2.3.1
- Examples:
- evas-images.c, evas-images2.c, and map_example_02.c.
Eina_Bool evas_object_image_extension_can_load_fast_get | ( | const char * | file | ) |
Check if a file extension may be supported by Image Object Functions.
- Parameters:
-
file The file to check, it should be an Eina_Stringshare.
- Returns:
EINA_TRUE
if we may be able to open it,EINA_FALSE
if it's unlikely.
- Since (EFL) :
- 1.1
This functions is threadsafe.
- Since :
- 2.3.1
Eina_Bool evas_object_image_extension_can_load_get | ( | const char * | file | ) |
Check if a file extension may be supported by Image Object Functions.
- Parameters:
-
file The file to check
- Returns:
EINA_TRUE
if we may be able to open it,EINA_FALSE
if it's unlikely.
- Since (EFL) :
- 1.1
If file is an Eina_Stringshare, use directly evas_object_image_extension_can_load_fast_get.
This functions is threadsafe.
- Since :
- 2.3.1