Tizen Native API
5.0
|
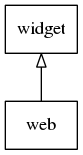
A web widget is used for displaying web pages (HTML/CSS/JS) using WebKit-EFL. You must have compiled Elementary with ewebkit support.
Signals that you can add callbacks for are:
- "download,request": A file download has been requested. Event info is a pointer to a Elm_Web_Download
- "editorclient,contents,changed": Editor client's contents changed
- "editorclient,selection,changed": Editor client's selection changed
- "frame,created": A new frame was created. Event info is an Evas_Object which can be handled with WebKit's ewk_frame API
- "icon,received": An icon was received by the main frame
- "inputmethod,changed": Input method changed. Event info is an Eina_Bool indicating whether it's enabled or not
- "js,windowobject,clear": JS window object has been cleared
- "link,hover,in": Mouse cursor is hovering over a link. Event info is a char *link[2], where the first string contains the URL the link points to, and the second one the title of the link
- "link,hover,out": Mouse cursor left the link
- "load,document,finished": Loading of a document finished. Event info is the frame that finished loading
- "load,error": Load failed. Event info is a pointer to Elm_Web_Frame_Load_Error
- "load,finished": Load finished. Event info is NULL on success, on error it's a pointer to Elm_Web_Frame_Load_Error
- "load,newwindow,show": A new window was created and is ready to be shown
- "load,progress": Overall load progress. Event info is a pointer to a double containing a value between 0.0 and 1.0
- "load,provisional": Started provisional load
- "load,started": Loading of a document started
- "menubar,visible,get": Queries if the menubar is visible. Event info is a pointer to Eina_Bool where the callback should set
EINA_TRUE
if the menubar is visible, orEINA_FALSE
in case it's not - "menubar,visible,set": Informs menubar visibility. Event info is an Eina_Bool indicating the visibility
- "popup,created": A dropdown widget was activated, requesting its popup menu to be created. Event info is a pointer to Elm_Web_Menu
- "popup,willdelete": The web object is ready to destroy the popup object created. Event info is a pointer to Elm_Web_Menu
- "ready": Page is fully loaded
- "scrollbars,visible,get": Queries visibility of scrollbars. Event info is a pointer to Eina_Bool where the visibility state should be set
- "scrollbars,visible,set": Informs scrollbars visibility. Event info is an Eina_Bool with the visibility state set
- "statusbar,text,set": Text of the statusbar changed. Even info is a string with the new text
- "statusbar,visible,get": Queries visibility of the status bar. Event info is a pointer to Eina_Bool where the visibility state should be set.
- "statusbar,visible,set": Informs statusbar visibility. Event info is an Eina_Bool with the visibility value
- "title,changed": Title of the main frame changed. Event info is a string with the new title
- "toolbars,visible,get": Queries visibility of toolbars. Event info is a pointer to Eina_Bool where the visibility state should be set
- "toolbars,visible,set": Informs the visibility of toolbars. Event info is an Eina_Bool with the visibility state
- "tooltip,text,set": Show and set text of a tooltip. Event info is a string with the text to show
- "uri,changed": URI of the main frame changed. Event info is a string (deprecated. use "url,changed" instead)
- "url,changed": URL of the main frame changed. Event info is a string with the new URI
- "view,resized": The web object internal's view changed sized
- "windows,close,request": A JavaScript request to close the current window was requested
- "zoom,animated,end": Animated zoom finished
- "focused" : When the web has received focus. (since 1.8)
- "unfocused" : When the web has lost focus. (since 1.8)
available styles:
- default
An example of use of web:
- web_example_01
- Web - Simple example
Typedefs | |
typedef struct _Elm_Web_Frame_Load_Error | Elm_Web_Frame_Load_Error |
typedef struct _Elm_Web_Menu_Item | Elm_Web_Menu_Item |
typedef struct _Elm_Web_Menu | Elm_Web_Menu |
typedef struct _Elm_Web_Window_Features | Elm_Web_Window_Features |
typedef Evas_Object *(* | Elm_Web_Window_Open )(void *data, Evas_Object *obj, Eina_Bool js, const Elm_Web_Window_Features *window_features) |
typedef Evas_Object *(* | Elm_Web_Dialog_Alert )(void *data, Evas_Object *obj, const char *message) |
typedef Evas_Object *(* | Elm_Web_Dialog_Confirm )(void *data, Evas_Object *obj, const char *message, Eina_Bool *ret) |
typedef Evas_Object *(* | Elm_Web_Dialog_Prompt )(void *data, Evas_Object *obj, const char *message, const char *def_value, const char **value, Eina_Bool *ret) |
typedef Evas_Object *(* | Elm_Web_Dialog_File_Selector )(void *data, Evas_Object *obj, Eina_Bool allows_multiple, Eina_List *accept_types, Eina_List **selected, Eina_Bool *ret) |
typedef void(* | Elm_Web_Console_Message )(void *data, Evas_Object *obj, const char *message, unsigned int line_number, const char *source_id) |
Typedef Documentation
typedef void(* Elm_Web_Console_Message)(void *data, Evas_Object *obj, const char *message, unsigned int line_number, const char *source_id) |
Callback type for the JS console message hook.
When a console message is added from JavaScript, any set function to the console message hook will be called for the user to handle. There is no default implementation of this hook.
- Parameters:
-
data User data pointer set when setting the hook function. obj The elm_web object that originated the message. message The message sent. line_number The line number. source_id Source id.
- See also:
- elm_web_console_message_hook_set()
typedef Evas_Object*(* Elm_Web_Dialog_Alert)(void *data, Evas_Object *obj, const char *message) |
Callback type for the JS alert hook.
- Parameters:
-
data User data pointer set when setting the hook function. obj The elm_web object requesting the new window. message The message to show in the alert dialog.
- Returns:
- The object representing the alert dialog. Elm_Web will run a second main loop to handle the dialog and normal flow of the application will be restored when the object is deleted, so the user should handle the popup properly in order to delete the object when the action is finished. If the function returns
NULL
the popup will be ignored.
- See also:
- elm_web_dialog_alert_hook_set()
typedef Evas_Object*(* Elm_Web_Dialog_Confirm)(void *data, Evas_Object *obj, const char *message, Eina_Bool *ret) |
Callback type for the JS confirm hook.
- Parameters:
-
data User data pointer set when setting the hook function. obj The elm_web object requesting the new window. message The message to show in the confirm dialog. ret Pointer to store the user selection. EINA_TRUE
if the user selectedOk
,EINA_FALSE
otherwise.
- Returns:
- The object representing the confirm dialog. Elm_Web will run a second main loop to handle the dialog and normal flow of the application will be restored when the object is deleted, so the user should handle the popup properly in order to delete the object when the action is finished. If the function returns
NULL
the popup will be ignored.
- See also:
- elm_web_dialog_confirm_hook_set()
typedef Evas_Object*(* Elm_Web_Dialog_File_Selector)(void *data, Evas_Object *obj, Eina_Bool allows_multiple, Eina_List *accept_types, Eina_List **selected, Eina_Bool *ret) |
Callback type for the JS file selector hook.
- Parameters:
-
data User data pointer set when setting the hook function. obj The elm_web object requesting the new window. allows_multiple EINA_TRUE
if multiple files can be selected.accept_types Mime types accepted. selected Pointer to store the list of malloc'ed strings containing the path to each file selected. Must be NULL
if the file dialog is canceled.ret Pointer to store the user selection. EINA_TRUE
if the user selectedOk
,EINA_FALSE
otherwise.
- Returns:
- The object representing the file selector dialog. Elm_Web will run a second main loop to handle the dialog and normal flow of the application will be restored when the object is deleted, so the user should handle the popup properly in order to delete the object when the action is finished. If the function returns
NULL
the popup will be ignored.
- See also:
- elm_web_dialog_file selector_hook_set()
typedef Evas_Object*(* Elm_Web_Dialog_Prompt)(void *data, Evas_Object *obj, const char *message, const char *def_value, const char **value, Eina_Bool *ret) |
Callback type for the JS prompt hook.
- Parameters:
-
data User data pointer set when setting the hook function. obj The elm_web object requesting the new window. message The message to show in the prompt dialog. def_value The default value to present the user in the entry value Pointer to store the value given by the user. Must be a malloc'ed string or NULL
if the user canceled the popup.ret Pointer to store the user selection. EINA_TRUE
if the user selectedOk
,EINA_FALSE
otherwise.
- Returns:
- The object representing the prompt dialog. Elm_Web will run a second main loop to handle the dialog and normal flow of the application will be restored when the object is deleted, so the user should handle the popup properly in order to delete the object when the action is finished. If the function returns
NULL
the popup will be ignored.
- See also:
- elm_web_dialog_prompt_hook_set()
typedef struct _Elm_Web_Frame_Load_Error Elm_Web_Frame_Load_Error |
Structure used to report load errors.
Load errors are reported as signal by elm_web. All the strings are temporary references and should not be used after the signal callback returns. If it's required, make copies with strdup() or eina_stringshare_add() (they are not even guaranteed to be stringshared, so must use eina_stringshare_add() and not eina_stringshare_ref()).
typedef struct _Elm_Web_Menu Elm_Web_Menu |
Structure describing the menu of a popup
This structure will be passed as the event_info
for the "popup,create" signal, which is emitted when a dropdown menu is opened. Users wanting to handle these popups by themselves should listen to this signal and set the handled
property of the struct to EINA_TRUE
. Leaving this property as EINA_FALSE
means that the user will not handle the popup and the default implementation will be used.
When the popup is ready to be dismissed, a "popup,willdelete" signal will be emitted to notify the user that it can destroy any objects and free all data related to it.
- See also:
- elm_web_popup_selected_set()
- elm_web_popup_destroy()
typedef struct _Elm_Web_Menu_Item Elm_Web_Menu_Item |
Structure describing the items in a menu
typedef struct _Elm_Web_Window_Features Elm_Web_Window_Features |
Opaque handler containing the features (such as statusbar, menubar, etc) that are to be set on a newly requested window.
typedef Evas_Object*(* Elm_Web_Window_Open)(void *data, Evas_Object *obj, Eina_Bool js, const Elm_Web_Window_Features *window_features) |
Callback type for the create_window hook.
- Parameters:
-
data User data pointer set when setting the hook function. obj The elm_web object requesting the new window. js Set to EINA_TRUE
if the request was originated from JavaScript.EINA_FALSE
otherwise.window_features A pointer of Elm_Web_Window_Features indicating the features requested for the new window.
- Returns:
- The
elm_web
widget where the request will be loaded. That is, if a new window or tab is created, the elm_web widget in it should be returned, and NOT the window object. ReturningNULL
should cancel the request.
- See also:
- elm_web_window_create_hook_set()
Enumeration Type Documentation
The possibles types that the items in a menu can be
Definitions of web window features.
enum Elm_Web_Zoom_Mode |
Types of zoom available.