Tizen Native API
6.5
|
The Application API provides functions to manage the main event loop, the application's state change events, basic system events, and get information about the application.
Required Header
#include <app.h>
Overview
The Application API handles an application state change or system events and provides mechanisms that launch other applications. The Tizen native application is similar to a conventional Linux application, but has some additional features optimized for mobile devices, which may have constraints such as relatively small screen sizes and lack of system resources compared to a larger system. For example, for power management reasons, the application may wish to take actions to reduce usage when it finds out that it had its display window covered over by another application's window. State change events are delivered so that this is possible. The Application API provides interfaces for the following three categories:
- Starting or exiting the main event loop (mandatory for every Tizen native application)
- Registering callbacks for application state change events
- Registering callbacks for basic system events
Event Loop
For an application to operate successfully, it must receive events from the platform. For this, it needs to start the main event loop - this is mandatory for all Tizen native applications. The ui_app_main() function is used to start the event loop. Before calling this function, you should set up the ui_app_lifecycle_callback_s structure, which is passed to the function (see the following sections).
Registering Callbacks for Application State Change Events
The application state change events include the following:
State | Description |
---|---|
app_create_cb() | Hook to take necessary actions before the main event loop starts. Your UI generation code should be placed here so that you do not miss any events from your application UI. |
app_pause_cb() | Hook to take necessary actions when an application becomes invisible. For example, you might wish to release memory resources so other applications can use these resources. It is important not to starve the application in front, which is interacting with the user. |
app_resume_cb() | Hook to take necessary actions when an application becomes visible. If anything is relinquished in app_pause_cb() but is necessary to resume the application, it must be re-allocated here. |
app_terminate_cb() | Hook to take necessary actions when your application is terminating. Your application should release all resources, especially any allocations and shared resources must be freed here, so other running applications can fully use these shared resources. |
app_control_cb() | Hook to take necessary actions when your application called by another application. When the application gets launch request, this callback function is called. The application can get information about what is to be performed by using App Control API from app_control handle. |
For further explanation of application state changes, see the The Application States and Transitions section.
Registering Callbacks for System Events
The system event callbacks can be registered using ui_app_add_event_handler(). Multiple system event callbacks can be registered. The basic system event includes the following:
System event types | Description |
---|---|
APP_EVENT_LOW_MEMORY | The system memory is running low. Recommended actions are as follows:
|
APP_EVENT_LOW_BATTERY | The battery power is running low. Recommended actions are as follows:
|
APP_EVENT_DEVICE_ORIENT | The orientation of device is changed. Recommended action is as follows: change display orientation to match the display orientation |
APP_EVANG_LANGUAGE_CHANGED | The language setting is changed. Recommended action: refresh the display into the new language |
APP_EVENT_REGION_FORMAT_CHANGED | The region format setting changed. Region change means a different time zone; the application UI may need to update the time to reflect the time zone change. |
APP_EVENT_SUSPENDED_STATE_CHANGED | The suspended state is changed. The application will enter the suspended state, or exit from the state. Do the necessary action before or after suspended; the application shall not respond to requests of the other modules. |
The Application States and Transitions
The Tizen native application can be in one of the several different states. Typically, the application is launched by the user from the Launcher, or by another application. As the application is starting, app_create_cb() is executed, and then the main event loop starts. After executing event loop, app_control_cb() and app_resume_cb() will be invoked. The application now normally becomes the front-most window with focus. When the application loses the front-most/focus status, the app_pause_cb() callback is invoked. There are many scenarios for your application to go into the pause state, which means your application is not terminated, but is running in the background:
- A new application is launched from the request of your application
- The user requests to go to the home screen
- A system event occurs and it causes a resident application with higher priority (e.g. a phone call is received) to become active, and hides your application temporarily
- An alarm went off for another application so it now becomes the top-most window and hides your application
Since Tizen 2.4, the application on the background goes in to a suspended state. In the suspended state, the application process is executed with limited CPU resources. In other words, the platform does not allow the running of the background applications. When your application becomes visible again, the app_resume_cb() callback is invoked. Some possible scenarios for your application to become visible are:
- Another application requests your application to run (perhaps the Task Navigator which shows all running applications and lets the user select any app to run)
- All applications on top of your application in the window stack finish
- An alarm is going off for your application so it becomes the top-most window and hides other applications When your application starts exiting, the app_pause_cb() callback is invoked if the application is visible, and then the app_terminate_cb() callback is invoked. Possible scenarios to start termination of your application are:
- Your application itself requests to exit by calling ui_app_exit() to terminate the event loop
- The Low Memory Killer is killing your application in a low memory situation. Note that a service application doesn't have UI, so the service application doesn't have Paused state. Application state changes are managed by the underlying framework. Refer to the following state diagram to see the possible transitions:
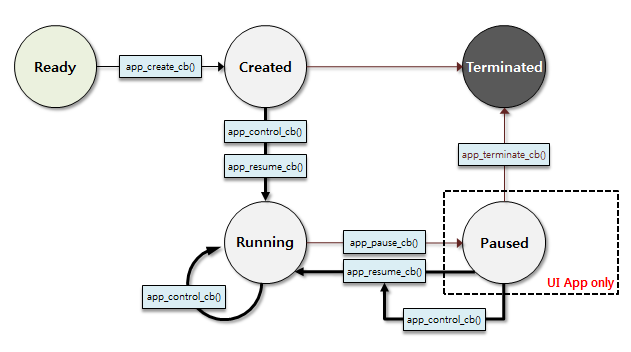
State | Description |
---|---|
READY | The application is launched. |
CREATED | The application starts the main loop. |
RUNNING | The application is running and visible to the user. |
PAUSED | The application is running but invisible to the user. |
TERMINATED | The application is terminated |
The Application API defines five states with their corresponding transition handlers. The state transition is notified through the state transition callback function, whether the application is created, is running, is paused, is resumed, or is terminated. And the application has to perform a specific task that is appropriate to those state changes.
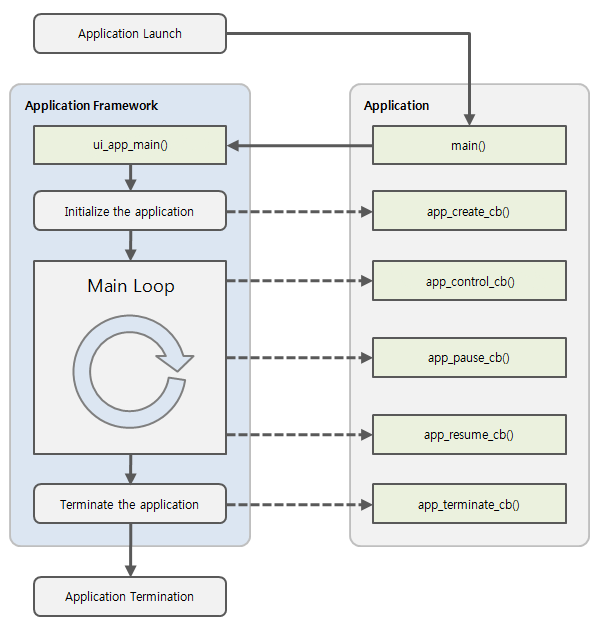
How to start the Tizen native application
An application can be launched by the user from the Launcher or by another application. Regardless of how an application is launched, the Application Framework starts an application by creating a new process and calls the entry point of the application. Like a conventional Linux application, the main function of its application is the entry point. In the Tizen application, the main task is to hand over control to the Application Framework by calling the ui_app_main() function.
bool app_create(void *user_data) { // Hook to take necessary actions before the main event loop starts // Initialize UI resources and application's data // If this function returns @c true, the main loop of the application starts // If this function returns @c false, the application is terminated return true; } void app_control(app_control_h app_control, void *user_data) { // Handle the launch request } void app_pause(void *user_data) { // Take necessary actions when the application becomes invisible } void app_resume(void *user_data) { // Take necessary actions when the application becomes visible } void app_terminate(void *user_data) { // Release all resources } int main(int argc, char *argv[]) { struct appdata ad; ui_app_lifecycle_callback_s event_callback = {0,}; event_callback.create = app_create; event_callback.terminate = app_terminate; event_callback.pause = app_pause; event_callback.resume = app_resume; event_callback.app_control = app_control; memset(&ad, 0x0, sizeof(struct appdata)); return ui_app_main(argc, argv, &event_callback, &ad); }
The ui_app_main() function initializes the application and then starts the main loop. The ui_app_main() function takes four parameters and uses them to initialize the application. The argc and argv parameters contain the values from the Application Framework, so that you should never have to change the values. The third parameter is a state transition handler that is responsible for managing the state transitions that the application goes through while it is running. The fourth parameter is the application data to be passed to each state handler. When ui_app_main() is first invoked, the application moves from the ready state to the created state. The application has to initialize itself. During this transition, the Application Framework calls the application's app_create_cb() state transition callback function just before the application enters the main loop. Within the registered app_create_cb() callback function, you should initialize the application's resources and create the main window. If the app_create_cb() callback function returns false
, the application moves to the terminated state.
If the app_create_cb() callback function returns true
, the application enters the main loop.
Handling the launch options
The Application Framework calls the application's app_control_cb() callback function just after the application enters the main loop. This callback function is passed to the app_control containing the reason due to which the application is launched. For example, the application might be launched to open a file to handle the request that has been sent by another application. In all of these cases, the application is responsible for checking the contents of the app_control and responding appropriately. The contents of the app_control can be empty in situations where the application is launched by the user from the Launcher.
static void app_control(app_control_h app_control, void *user_data) { struct appdata *ad = (struct appdata *)user_data; char *operation; char *uri; char *mime_type; app_control_get_operation(app_control, operation); if (!strcmp(operation, SERVICE_OPERATION_VIEW)) { app_control_get_uri(app_control, &uri); app_control_get_mime(app_control, &mime_type); if (uri && !strcmp(mime_type, "image/jpg")) display_image_file(ad, uri); // Display a specific image file } if (ad->win) elm_win_activate(ad->win); }
Functions | |
app_device_orientation_e | app_get_device_orientation (void) |
Gets the current device orientation. | |
int | ui_app_main (int argc, char **argv, ui_app_lifecycle_callback_s *callback, void *user_data) |
Runs the application's main loop until ui_app_exit() is called. | |
void | ui_app_exit (void) |
Exits the main loop of application. | |
int | ui_app_add_event_handler (app_event_handler_h *event_handler, app_event_type_e event_type, app_event_cb callback, void *user_data) |
Adds the system event handler. | |
int | ui_app_remove_event_handler (app_event_handler_h event_handler) |
Removes registered event handler. | |
Typedefs | |
typedef bool(* | app_create_cb )(void *user_data) |
Called when the application starts. | |
typedef void(* | app_pause_cb )(void *user_data) |
Called when the application is completely obscured by another application and becomes invisible. | |
typedef void(* | app_resume_cb )(void *user_data) |
Called when the application becomes visible. | |
typedef void(* | app_terminate_cb )(void *user_data) |
Called when the application's main loop exits. | |
typedef void(* | app_control_cb )(app_control_h app_control, void *user_data) |
Called when another application sends a launch request to the application. |
Typedef Documentation
typedef void(* app_control_cb)(app_control_h app_control, void *user_data) |
Called when another application sends a launch request to the application.
When the application is launched, this callback function is called after the main loop of the application starts up. The passed app_control handle describes the launch request and contains the information about why the application is launched. If the launch request is sent to the application in the running or pause state, this callback function can be called again to notify that the application has been asked to launch. The application could be explicitly launched by the user from the application launcher or be launched to perform the specific operation by another application. The application is responsible for handling each launch request and responding appropriately. Using the App Control API, the application can get information about what is to be performed. If the application is launched from the application launcher or explicitly launched by another application, the passed app_control handle may include only the default operation (APP_CONTROL_OPERATION_DEFAULT) without any data. For more information, see The App Control API description.
- Since :
- 2.3
- Remarks:
- After this callback returns, the handle of the app_control is freed. Therefore, if you want to use the handle after returning this callback, you MUST copy it by using app_control_clone() API.
- Parameters:
-
[in] app_control The handle to the app_control [in] user_data The user data passed from the callback registration function
- See also:
- ui_app_main()
- ui_app_lifecycle_callback_s
- App Control API
typedef bool(* app_create_cb)(void *user_data) |
Called when the application starts.
The callback function is called before the main loop of the application starts. In this callback, you can initialize application resources like window creation, data structure, and so on. After this callback function returns true
, the main loop starts up and app_control_cb() is subsequently called. If this callback function returns false
, the main loop doesn't start and app_terminate_cb() is subsequently called.
- Since :
- 2.3
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- Returns:
true
on success, otherwisefalse
- Precondition:
- ui_app_main() will invoke this callback function.
- See also:
- ui_app_main()
- ui_app_lifecycle_callback_s
typedef void(* app_pause_cb)(void *user_data) |
Called when the application is completely obscured by another application and becomes invisible.
The application is not terminated and still running in the paused state.
- Since :
- 2.3
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- See also:
- ui_app_main()
- ui_app_lifecycle_callback_s
typedef void(* app_resume_cb)(void *user_data) |
Called when the application becomes visible.
- Since :
- 2.3
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- See also:
- ui_app_main()
- ui_app_lifecycle_callback_s
typedef void(* app_terminate_cb)(void *user_data) |
Called when the application's main loop exits.
You should release the application's resources in this function.
- Since :
- 2.3
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- See also:
- ui_app_main()
- ui_app_lifecycle_callback_s
Enumeration Type Documentation
enum app_error_e |
Enumeration for application error.
- Since :
- 2.3
- Enumerator:
Function Documentation
Gets the current device orientation.
- Since :
- 2.3
- Returns:
- The current device orientation
int ui_app_add_event_handler | ( | app_event_handler_h * | event_handler, |
app_event_type_e | event_type, | ||
app_event_cb | callback, | ||
void * | user_data | ||
) |
Adds the system event handler.
- Since :
- 2.3
- Parameters:
-
[out] event_handler The event handler [in] event_type The system event type [in] callback The callback function [in] user_data The user data to be passed to the callback functions
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_OUT_OF_MEMORY Out of memory
void ui_app_exit | ( | void | ) |
Exits the main loop of application.
The main loop of application stops and app_terminate_cb() is invoked.
- Since :
- 2.3
- See also:
- ui_app_main()
- app_terminate_cb()
int ui_app_main | ( | int | argc, |
char ** | argv, | ||
ui_app_lifecycle_callback_s * | callback, | ||
void * | user_data | ||
) |
Runs the application's main loop until ui_app_exit() is called.
This function is the main entry point of the Tizen application. The app_create_cb() callback function is called to initialize the application before the main loop of application starts up. After the app_create_cb() callback function returns true, the main loop starts up and the app_control_cb() callback function is subsequently called. If the app_create_cb() callback function returns false, the main loop doesn't start up and app_terminate_cb() callback function is called. This main loop supports event handling for the Ecore Main Loop.
- Since :
- 2.3
- Parameters:
-
[in] argc The argument count [in] argv The argument vector [in] callback The set of callback functions to handle application lifecycle events [in] user_data The user data to be passed to the callback functions
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter APP_ERROR_INVALID_CONTEXT The application is launched illegally, not launched by the launch system APP_ERROR_ALREADY_RUNNING The main loop already started
int ui_app_remove_event_handler | ( | app_event_handler_h | event_handler | ) |
Removes registered event handler.
- Since :
- 2.3
- Parameters:
-
[in] event_handler The event handler
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
APP_ERROR_NONE Successful APP_ERROR_INVALID_PARAMETER Invalid parameter
- See also:
- ui_app_add_event_handler()