Tizen Native API
6.5
|
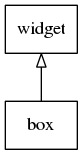
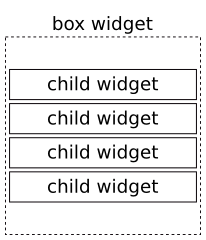
A box arranges objects in a linear fashion, governed by a layout function that defines the details of this arrangement.
By default, the box will use an internal function to set the layout to a single row, either vertical or horizontal. This layout is affected by a number of parameters, such as the homogeneous flag set by elm_box_homogeneous_set(), the values given by elm_box_padding_set() and elm_box_align_set() and the hints set to each object in the box.
For this default layout, it's possible to change the orientation with elm_box_horizontal_set(). The box will start in the vertical orientation, placing its elements ordered from top to bottom. When horizontal is set, the order will go from left to right. If the box is set to be homogeneous, every object in it will be assigned the same space, that of the largest object. Padding can be used to set some spacing between the cell given to each object. The alignment of the box, set with elm_box_align_set(), determines how the bounding box of all the elements will be placed within the space given to the box widget itself.
The size hints of each object also affect how they are placed and sized within the box. evas_object_size_hint_min_set() will give the minimum size the object can have, and the box will use it as the basis for all latter calculations. Elementary widgets set their own minimum size as needed, so there's rarely any need to use it manually.
evas_object_size_hint_weight_set(), when not in homogeneous mode, is used to tell whether the object will be allocated the minimum size it needs or if the space given to it should be expanded. It's important to realize that expanding the size given to the object is not the same thing as resizing the object. It could very well end being a small widget floating in a much larger empty space. If not set, the weight for objects will normally be 0.0 for both axis, meaning the widget will not be expanded. To take as much space possible, set the weight to EVAS_HINT_EXPAND (defined to 1.0) for the desired axis to expand.
Besides how much space each object is allocated, it's possible to control how the widget will be placed within that space using evas_object_size_hint_align_set(). By default, this value will be 0.5 for both axis, meaning the object will be centered, but any value from 0.0 (left or top, for the x
and y
axes, respectively) to 1.0 (right or bottom) can be used. The special value EVAS_HINT_FILL, which is -1.0, means the object will be resized to fill the entire space it was allocated.
In addition, customized functions to define the layout can be set, which allow the application developer to organize the objects within the box in any number of ways.
The special elm_box_layout_transition() function can be used to switch from one layout to another, animating the motion of the children of the box.
- Note:
- Objects should not be added to box objects using _add() calls.
Some examples on how to use boxes follow:
Functions | |
void | elm_box_layout_transition (Evas_Object *obj, Evas_Object_Box_Data *priv, void *data) |
Elm_Box_Transition * | elm_box_transition_new (const double duration, Evas_Object_Box_Layout start_layout, void *start_layout_data, Ecore_Cb start_layout_free_data, Evas_Object_Box_Layout end_layout, void *end_layout_data, Ecore_Cb end_layout_free_data, Ecore_Cb transition_end_cb, void *transition_end_data) |
void | elm_box_transition_free (void *data) |
void | elm_box_homogeneous_set (Elm_Box *obj, Eina_Bool homogeneous) |
Set the box to arrange its children homogeneously. | |
Eina_Bool | elm_box_homogeneous_get (const Elm_Box *obj) |
Get whether the box is using homogeneous mode or not ($true if it's homogeneous, false otherwise) | |
void | elm_box_align_set (Elm_Box *obj, double horizontal, double vertical) |
Set the alignment of the whole bounding box of contents. | |
void | elm_box_align_get (const Elm_Box *obj, double *horizontal, double *vertical) |
Get the alignment of the whole bounding box of contents. | |
void | elm_box_horizontal_set (Elm_Box *obj, Eina_Bool horizontal) |
Set the horizontal orientation. | |
Eina_Bool | elm_box_horizontal_get (const Elm_Box *obj) |
Get the horizontal orientation ($true if the box is set to horizontal mode, false otherwise) | |
void | elm_box_padding_set (Elm_Box *obj, int horizontal, int vertical) |
Set the space (padding) between the box's elements. | |
void | elm_box_padding_get (const Elm_Box *obj, int *horizontal, int *vertical) |
Get the space (padding) between the box's elements. | |
Eina_List * | elm_box_children_get (const Elm_Box *obj) |
Get a list of the objects packed into the box. | |
void | elm_box_pack_end (Elm_Box *obj, Efl_Canvas_Object *subobj) |
Add an object at the end of the pack list. | |
void | elm_box_unpack_all (Elm_Box *obj) |
Remove all items from the box, without deleting them. | |
void | elm_box_unpack (Elm_Box *obj, Efl_Canvas_Object *subobj) |
Unpack a box item. | |
void | elm_box_pack_after (Elm_Box *obj, Efl_Canvas_Object *subobj, Efl_Canvas_Object *after) |
Adds an object to the box after the indicated object. | |
void | elm_box_pack_start (Elm_Box *obj, Efl_Canvas_Object *subobj) |
Add an object to the beginning of the pack list. | |
void | elm_box_recalculate (Elm_Box *obj) |
Force the box to recalculate its children packing. | |
void | elm_box_pack_before (Elm_Box *obj, Efl_Canvas_Object *subobj, Efl_Canvas_Object *before) |
Adds an object to the box before the indicated object. | |
void | elm_box_clear (Elm_Box *obj) |
Clear the box of all children. | |
Evas_Object * | elm_box_add (Evas_Object *parent) |
void | elm_box_layout_set (Evas_Object *obj, Evas_Object_Box_Layout cb, const void *data, Ecore_Cb free_data) |
Set the layout defining function to be used by the box. | |
Typedefs | |
typedef struct _Elm_Box_Transition | Elm_Box_Transition |
Typedef Documentation
Opaque handler containing the parameters to perform an animated transition of the layout the box uses.
Function Documentation
Evas_Object* elm_box_add | ( | Evas_Object * | parent | ) |
Add a new box to the parent
By default, the box will be in vertical mode and non-homogeneous.
- Parameters:
-
parent The parent object
- Returns:
- The new object or NULL if it cannot be created
- Since :
- 2.3
- Examples:
- actionslider_example_01.c, bg_example_03.c, box_example_02.c, button_example_01.c, calendar_example_04.c, calendar_example_06.c, clock_example.c, colorselector_example_01.c, combobox_example_01.c, conformant_example_01.c, conformant_example_02.c, datetime_example.c, dayselector_example.c, diskselector_example_01.c, diskselector_example_02.c, entry_example.c, fileselector_button_example.c, fileselector_entry_example.c, fileselector_example.c, flipselector_example.c, general_funcs_example.c, gengrid_example.c, genlist_example_02.c, genlist_example_03.c, genlist_example_04.c, genlist_example_05.c, glview_example_01.c, index_example_01.c, inwin_example.c, list_example_01.c, list_example_03.c, map_example_01.c, map_example_02.c, map_example_03.c, mapbuf_example.c, notify_example_01.c, panel_example_01.c, progressbar_example.c, radio_example_01.c, separator_example_01.c, slider_example.c, slideshow_example.c, spinner_example.c, theme_example_01.c, theme_example_02.c, toolbar_example_01.c, toolbar_example_02.c, toolbar_example_03.c, transit_example_03.c, transit_example_04.c, web_example_02.c, and win_example.c.
void elm_box_align_get | ( | const Elm_Box * | obj, |
double * | horizontal, | ||
double * | vertical | ||
) |
Get the alignment of the whole bounding box of contents.
See also elm_box_align_set.
- Parameters:
-
[in] obj The object. [out] horizontal The horizontal alignment of elements [out] vertical The vertical alignment of elements
- Since :
- 2.3
void elm_box_align_set | ( | Elm_Box * | obj, |
double | horizontal, | ||
double | vertical | ||
) |
Set the alignment of the whole bounding box of contents.
Sets how the bounding box containing all the elements of the box, after their sizes and position has been calculated, will be aligned within the space given for the whole box widget.
- Parameters:
-
[in] obj The object. [in] horizontal The horizontal alignment of elements [in] vertical The vertical alignment of elements
- Since :
- 2.3
Eina_List* elm_box_children_get | ( | const Elm_Box * | obj | ) |
Get a list of the objects packed into the box.
Returns a new list
with a pointer to Evas_Object
in its nodes. The order of the list corresponds to the packing order the box uses.
You must free this list with eina_list_free() once you are done with it.
- Parameters:
-
[in] obj The object.
- Returns:
- List of children
- Since :
- 2.3
- Examples:
- box_example_02.c.
void elm_box_clear | ( | Elm_Box * | obj | ) |
Clear the box of all children.
Remove all the elements contained by the box, deleting the respective objects.
See also elm_box_unpack, elm_box_unpack_all.
- Parameters:
-
[in] obj The object.
- Since :
- 2.3
- Examples:
- box_example_02.c.
Eina_Bool elm_box_homogeneous_get | ( | const Elm_Box * | obj | ) |
Get whether the box is using homogeneous mode or not ($true if it's homogeneous, false
otherwise)
- Parameters:
-
[in] obj The object.
- Returns:
- The homogeneous flag
- Since :
- 2.3
void elm_box_homogeneous_set | ( | Elm_Box * | obj, |
Eina_Bool | homogeneous | ||
) |
Set the box to arrange its children homogeneously.
If enabled, homogeneous layout makes all items the same size, according to the size of the largest of its children.
- Note:
- This flag is ignored if a custom layout function is set.
- Parameters:
-
[in] obj The object. [in] homogeneous The homogeneous flag
- Since :
- 2.3
Eina_Bool elm_box_horizontal_get | ( | const Elm_Box * | obj | ) |
Get the horizontal orientation ($true if the box is set to horizontal mode, false
otherwise)
- Parameters:
-
[in] obj The object.
- Returns:
- The horizontal flag
- Since :
- 2.3
void elm_box_horizontal_set | ( | Elm_Box * | obj, |
Eina_Bool | horizontal | ||
) |
Set the horizontal orientation.
By default, box object arranges their contents vertically from top to bottom. By calling this function with horizontal
as true
, the box will become horizontal, arranging contents from left to right.
- Note:
- This flag is ignored if a custom layout function is set.
- Parameters:
-
[in] obj The object. [in] horizontal The horizontal flag
- Since :
- 2.3
- Examples:
- bg_example_03.c, box_example_02.c, button_example_01.c, datetime_example.c, diskselector_example_02.c, entry_example.c, fileselector_button_example.c, fileselector_entry_example.c, fileselector_example.c, general_funcs_example.c, gengrid_example.c, genlist_example_02.c, genlist_example_03.c, index_example_01.c, list_example_03.c, map_example_03.c, mapbuf_example.c, notify_example_01.c, panel_example_01.c, progressbar_example.c, radio_example_01.c, separator_example_01.c, slideshow_example.c, transit_example_03.c, transit_example_04.c, web_example_02.c, and win_example.c.
void elm_box_layout_set | ( | Evas_Object * | obj, |
Evas_Object_Box_Layout | cb, | ||
const void * | data, | ||
Ecore_Cb | free_data | ||
) |
Set the layout defining function to be used by the box.
Whenever anything changes that requires the box in obj
to recalculate the size and position of its elements, the function cb
will be called to determine what the layout of the children will be.
Once a custom function is set, everything about the children layout is defined by it. The flags set by elm_box_horizontal_set and elm_box_homogeneous_set no longer have any meaning, and the values given by elm_box_padding_set and elm_box_align_set are up to this layout function to decide if they are used and how. These last two will be found in the priv
parameter, of type Evas_Object_Box_Data
, passed to cb
. The Evas_Object
the function receives is not the Elementary widget, but the internal Evas Box it uses, so none of the functions described here can be used on it.
Any of the layout functions in Evas
can be used here, as well as the special elm_box_layout_transition.
The final data
argument received by cb
is the same data
passed here, and the free_data
function will be called to free it whenever the box is destroyed or another layout function is set.
Setting cb
to null
will revert back to the default layout function.
See also elm_box_layout_transition.
- Parameters:
-
[in] cb The callback function used for layout [in] data Data that will be passed to layout function [in] free_data Function called to free data
- Since :
- 2.3
- Examples:
- box_example_02.c, genlist_example_04.c, and genlist_example_05.c.
void elm_box_layout_transition | ( | Evas_Object * | obj, |
Evas_Object_Box_Data * | priv, | ||
void * | data | ||
) |
Special layout function that animates the transition from one layout to another
- Parameters:
-
obj The object. priv The smart object instance data. data Data will be passed to function.
Normally, when switching the layout function for a box, this will be reflected immediately on screen on the next render, but it's also possible to do this through an animated transition.
This is done by creating an Elm_Box_Transition and setting the box layout to this function.
For example:
Elm_Box_Transition *t = elm_box_transition_new(1.0, evas_object_box_layout_vertical, // start NULL, // data for initial layout NULL, // free function for initial data evas_object_box_layout_horizontal, // end NULL, // data for final layout NULL, // free function for final data anim_end, // will be called when animation ends NULL); // data for anim_end function elm_box_layout_set(box, elm_box_layout_transition, t, elm_box_transition_free);
- Note:
- This function can only be used with elm_box_layout_set(). Calling it directly will not have the expected results.
- Since :
- 2.3
- Examples:
- box_example_02.c.
void elm_box_pack_after | ( | Elm_Box * | obj, |
Efl_Canvas_Object * | subobj, | ||
Efl_Canvas_Object * | after | ||
) |
Adds an object to the box after the indicated object.
This will add the subobj
to the box indicated after the object indicated with after
. If after
is not already in the box, results are undefined. After means either to the right of the indicated object or below it depending on orientation.
See also elm_box_pack_start, elm_box_pack_end, elm_box_pack_before, elm_box_unpack, elm_box_unpack_all, elm_box_clear.
- Parameters:
-
[in] obj The object. [in] subobj The object to add to the box [in] after The object after which to add it
- Since :
- 2.3
- Examples:
- box_example_02.c, and web_example_02.c.
void elm_box_pack_before | ( | Elm_Box * | obj, |
Efl_Canvas_Object * | subobj, | ||
Efl_Canvas_Object * | before | ||
) |
Adds an object to the box before the indicated object.
This will add the subobj
to the box indicated before the object indicated with before
. If before
is not already in the box, results are undefined. Before means either to the left of the indicated object or above it depending on orientation.
See also elm_box_pack_start, elm_box_pack_end, elm_box_pack_after, elm_box_unpack, elm_box_unpack_all, elm_box_clear.
- Parameters:
-
[in] obj The object. [in] subobj The object to add to the box [in] before The object before which to add it
- Since :
- 2.3
void elm_box_pack_end | ( | Elm_Box * | obj, |
Efl_Canvas_Object * | subobj | ||
) |
Add an object at the end of the pack list.
Pack subobj
into the box obj
, placing it last in the list of children objects. The actual position the object will get on screen depends on the layout used. If no custom layout is set, it will be at the bottom or right, depending if the box is vertical or horizontal, respectively.
See also elm_box_pack_start, elm_box_pack_before, elm_box_pack_after, elm_box_unpack, elm_box_unpack_all, elm_box_clear.
- Parameters:
-
[in] obj The object. [in] subobj The object to add to the box
- Since :
- 2.3
- Examples:
- actionslider_example_01.c, bg_example_03.c, box_example_02.c, button_example_01.c, calendar_example_04.c, calendar_example_06.c, clock_example.c, colorselector_example_01.c, combobox_example_01.c, conformant_example_01.c, conformant_example_02.c, datetime_example.c, dayselector_example.c, diskselector_example_01.c, diskselector_example_02.c, entry_example.c, fileselector_button_example.c, fileselector_entry_example.c, fileselector_example.c, flipselector_example.c, general_funcs_example.c, gengrid_example.c, genlist_example_02.c, genlist_example_03.c, genlist_example_04.c, genlist_example_05.c, glview_example_01.c, index_example_01.c, inwin_example.c, list_example_01.c, list_example_03.c, map_example_01.c, map_example_02.c, map_example_03.c, mapbuf_example.c, notify_example_01.c, panel_example_01.c, progressbar_example.c, radio_example_01.c, separator_example_01.c, slider_example.c, slideshow_example.c, spinner_example.c, theme_example_01.c, theme_example_02.c, toolbar_example_01.c, toolbar_example_02.c, toolbar_example_03.c, transit_example_03.c, transit_example_04.c, web_example_02.c, and win_example.c.
void elm_box_pack_start | ( | Elm_Box * | obj, |
Efl_Canvas_Object * | subobj | ||
) |
Add an object to the beginning of the pack list.
Pack subobj
into the box obj
, placing it first in the list of children objects. The actual position the object will get on screen depends on the layout used. If no custom layout is set, it will be at the top or left, depending if the box is vertical or horizontal, respectively.
See also elm_box_pack_end, elm_box_pack_before, elm_box_pack_after, elm_box_unpack, elm_box_unpack_all, elm_box_clear.
- Parameters:
-
[in] obj The object. [in] subobj The object to add to the box
- Since :
- 2.3
void elm_box_padding_get | ( | const Elm_Box * | obj, |
int * | horizontal, | ||
int * | vertical | ||
) |
Get the space (padding) between the box's elements.
See also elm_box_padding_set.
- Parameters:
-
[in] obj The object. [out] horizontal The horizontal space between elements [out] vertical The vertical space between elements
- Since :
- 2.3
void elm_box_padding_set | ( | Elm_Box * | obj, |
int | horizontal, | ||
int | vertical | ||
) |
Set the space (padding) between the box's elements.
Extra space in pixels that will be added between a box child and its neighbors after its containing cell has been calculated. This padding is set for all elements in the box, besides any possible padding that individual elements may have through their size hints.
- Parameters:
-
[in] obj The object. [in] horizontal The horizontal space between elements [in] vertical The vertical space between elements
- Since :
- 2.3
void elm_box_recalculate | ( | Elm_Box * | obj | ) |
Force the box to recalculate its children packing.
If any children was added or removed, box will not calculate the values immediately rather leaving it to the next main loop iteration. While this is great as it would save lots of recalculation, whenever you need to get the position of a just added item you must force recalculate before doing so.
- Parameters:
-
[in] obj The object.
- Since :
- 2.3
void elm_box_transition_free | ( | void * | data | ) |
Free a Elm_Box_Transition instance created with elm_box_transition_new().
This function is mostly useful as the free_data
parameter in elm_box_layout_set() when elm_box_layout_transition().
- Parameters:
-
data The Elm_Box_Transition instance to be freed.
- Since :
- 2.3
- Examples:
- box_example_02.c.
Elm_Box_Transition* elm_box_transition_new | ( | const double | duration, |
Evas_Object_Box_Layout | start_layout, | ||
void * | start_layout_data, | ||
Ecore_Cb | start_layout_free_data, | ||
Evas_Object_Box_Layout | end_layout, | ||
void * | end_layout_data, | ||
Ecore_Cb | end_layout_free_data, | ||
Ecore_Cb | transition_end_cb, | ||
void * | transition_end_data | ||
) |
Create a new Elm_Box_Transition to animate the switch of layouts
If you want to animate the change from one layout to another, you need to set the layout function of the box to elm_box_layout_transition(), passing as user data to it an instance of Elm_Box_Transition with the necessary information to perform this animation. The free function to set for the layout is elm_box_transition_free().
The parameters to create an Elm_Box_Transition sum up to how long will it be, in seconds, a layout function to describe the initial point, another for the final position of the children and one function to be called when the whole animation ends. This last function is useful to set the definitive layout for the box, usually the same as the end layout for the animation, but could be used to start another transition.
- Parameters:
-
duration The duration of the transition in seconds start_layout The layout function that will be used to start the animation start_layout_data The data to be passed the start_layout
functionstart_layout_free_data Function to free start_layout_data
end_layout The layout function that will be used to end the animation end_layout_data Data param passed to end_layout
end_layout_free_data The data to be passed the end_layout
functionend_layout_free_data Function to free end_layout_data
transition_end_cb Callback function called when animation ends transition_end_data Data to be passed to transition_end_cb
- Returns:
- An instance of Elm_Box_Transition
- Since :
- 2.3
- Examples:
- box_example_02.c.
void elm_box_unpack | ( | Elm_Box * | obj, |
Efl_Canvas_Object * | subobj | ||
) |
Unpack a box item.
Remove the object given by subobj
from the box obj
without deleting it.
See also elm_box_unpack_all, elm_box_clear.
- Parameters:
-
[in] obj The object. [in] subobj The object to unpack
- Since :
- 2.3
- Examples:
- box_example_02.c.
void elm_box_unpack_all | ( | Elm_Box * | obj | ) |
Remove all items from the box, without deleting them.
Clear the box from all children, but don't delete the respective objects. If no other references of the box children exist, the objects will never be deleted, and thus the application will leak the memory. Make sure when using this function that you hold a reference to all the objects in the box obj
.
See also elm_box_clear, elm_box_unpack.
- Parameters:
-
[in] obj The object.
- Since :
- 2.3