Tizen Native API
6.5
|
The Player API provides functions for media playback and controlling media playback attributes.
Required Header
#include <player.h>
Overview
The Player API provides a way to play multimedia content. Content can be played from a file, from the network, or from memory. It gives the ability to start/stop/pause/mute, set the playback position (that is, seek), perform various status queries, and control the display.
Additional functions allow registering notifications via callback functions for various state change events.
This API also enables collaboration with the GUI service to present a video.
State Diagram
Playback of multimedia content is controlled by a state machine. The following diagram shows the life cycle and states of the Player.
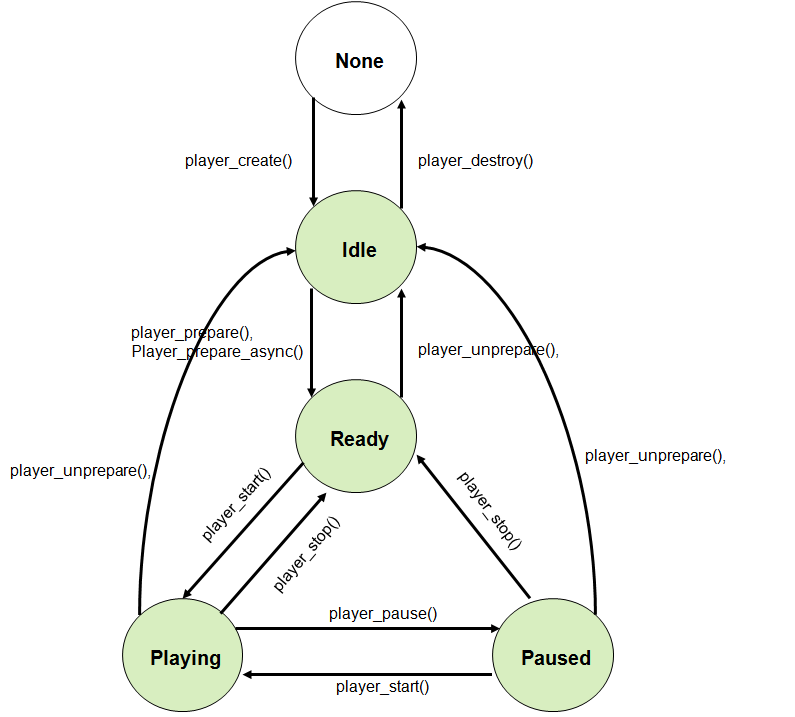
State Transitions
FUNCTION | PRE-STATE | POST-STATE | SYNC TYPE |
---|---|---|---|
player_create() | NONE | IDLE | SYNC |
player_destroy() | IDLE | NONE | SYNC |
player_prepare() | IDLE | READY | SYNC |
player_prepare_async() | IDLE | READY | ASYNC |
player_unprepare() | READY, PLAYING or PAUSED | IDLE | SYNC |
player_start() | READY or PAUSED | PLAYING | SYNC |
player_stop() | PLAYING | READY | SYNC |
player_pause() | PLAYING | PAUSED | SYNC |
State Dependent Function Calls
The following table shows state-dependent function calls. It is forbidden to call the functions listed below in wrong states. Violation of this rule may result in unpredictable behavior.
Asynchronous Operations
All functions that change the player state are synchronous except player_prepare_async(), player_set_play_position(), and player_capture_video(). Thus the result is passed to the application via the callback mechanism.
Callback(Event) Operations
Related Features
This API is related with the following features:
- http://tizen.org/feature/opengles.version.2_0
- http://tizen.org/feature/multimedia.player.spherical_video These features are required for 360 video playback.
- http://tizen.org/feature/multimedia.player.audio_offload This feature is required for hardware-offloaded audio processing.
It is recommended to design feature related codes in your application for reliability. You can check if a device supports the related features for this API by using System Information, thereby controlling the procedure of your application. To ensure your application is only running on the device with specific features, please define the features in your manifest file using the manifest editor in the SDK. More details on featuring your application can be found from Feature Element.
Functions | |
int | player_create (player_h *player) |
Creates a player handle for playing multimedia content. | |
int | player_destroy (player_h player) |
Destroys the media player handle and releases all its resources. | |
int | player_prepare (player_h player) |
Prepares the media player for playback. | |
int | player_prepare_async (player_h player, player_prepared_cb callback, void *user_data) |
Prepares the media player for playback, asynchronously. | |
int | player_unprepare (player_h player) |
Resets the media player. | |
int | player_set_uri (player_h player, const char *uri) |
Sets the data source (file-path, HTTP or RTSP URI) to use. | |
int | player_set_memory_buffer (player_h player, const void *data, int size) |
Sets memory as the data source. | |
int | player_get_state (player_h player, player_state_e *state) |
Gets the player's current state. | |
int | player_set_volume (player_h player, float left, float right) |
Sets the player's volume. | |
int | player_get_volume (player_h player, float *left, float *right) |
Gets the player's current volume factor. | |
int | player_set_sound_stream_info (player_h player, sound_stream_info_h stream_info) |
Sets the player's sound manager stream information. | |
int | player_set_audio_latency_mode (player_h player, audio_latency_mode_e latency_mode) |
Sets the audio latency mode. | |
int | player_get_audio_latency_mode (player_h player, audio_latency_mode_e *latency_mode) |
Gets the current audio latency mode. | |
int | player_start (player_h player) |
Starts or resumes playback. | |
int | player_stop (player_h player) |
Stops playing media content. | |
int | player_pause (player_h player) |
Pauses the player. | |
int | player_set_play_position (player_h player, int milliseconds, bool accurate, player_seek_completed_cb callback, void *user_data) |
Sets the seek position for playback, asynchronously. | |
int | player_set_play_position_nsec (player_h player, int64_t nanoseconds, bool accurate, player_seek_completed_cb callback, void *user_data) |
Sets the seek position in nanoseconds for playback, asynchronously. | |
int | player_get_play_position (player_h player, int *milliseconds) |
Gets the current position in milliseconds. | |
int | player_get_play_position_nsec (player_h player, int64_t *nanoseconds) |
Gets the current position in nanoseconds. | |
int | player_set_mute (player_h player, bool muted) |
Sets the player's mute status. | |
int | player_is_muted (player_h player, bool *muted) |
Gets the player's mute status. | |
int | player_set_looping (player_h player, bool looping) |
Sets the player's looping status. | |
int | player_is_looping (player_h player, bool *looping) |
Gets the player's looping status. | |
int | player_set_display (player_h player, player_display_type_e type, player_display_h display) |
Sets the video display. | |
int | player_set_media_packet_video_frame_decoded_cb (player_h player, player_media_packet_video_decoded_cb callback, void *user_data) |
Sets a callback function for getting the decoded video frame. | |
int | player_unset_media_packet_video_frame_decoded_cb (player_h player) |
Unsets the callback notifying the decoded video frame. | |
int | player_set_media_packet_audio_frame_decoded_cb (player_h player, media_format_h format, player_audio_extract_option_e opt, player_media_packet_audio_decoded_cb callback, void *user_data) |
Sets a callback function for getting the decoded audio data. | |
int | player_unset_media_packet_audio_frame_decoded_cb (player_h player) |
Unsets the callback notifying the decoded audio data. | |
int | player_push_media_stream (player_h player, media_packet_h packet) |
Pushes elementary stream to decode audio or video. | |
int | player_foreach_media_stream_supported_format (player_h player, player_supported_media_format_cb callback, void *user_data) |
Retrieves all supported media formats for the playback of external media stream. | |
int | player_set_media_stream_info (player_h player, player_stream_type_e type, media_format_h format) |
Sets contents information for media stream. | |
int | player_set_media_stream_buffer_status_cb (player_h player, player_stream_type_e type, player_media_stream_buffer_status_cb callback, void *user_data) |
Sets a callback function to be invoked when buffer underrun or overflow is occurred. | |
int | player_unset_media_stream_buffer_status_cb (player_h player, player_stream_type_e type) |
Unsets the buffer status callback function. | |
int | player_set_media_stream_seek_cb (player_h player, player_stream_type_e type, player_media_stream_seek_cb callback, void *user_data) |
Sets a callback function to be invoked when seeking is occurred. | |
int | player_unset_media_stream_seek_cb (player_h player, player_stream_type_e type) |
Unsets the seek callback function. | |
int | player_set_media_stream_buffer_max_size (player_h player, player_stream_type_e type, unsigned long long max_size) |
Sets the max size bytes of buffer. | |
int | player_get_media_stream_buffer_max_size (player_h player, player_stream_type_e type, unsigned long long *max_size) |
Gets the max size bytes of buffer. | |
int | player_set_media_stream_buffer_min_threshold (player_h player, player_stream_type_e type, unsigned int percent) |
Sets the buffer threshold percent of buffer. | |
int | player_get_media_stream_buffer_min_threshold (player_h player, player_stream_type_e type, unsigned int *percent) |
Gets the buffer threshold percent of buffer. | |
int | player_capture_video (player_h player, player_video_captured_cb callback, void *user_data) |
Captures the video frame, asynchronously. | |
int | player_set_completed_cb (player_h player, player_completed_cb callback, void *user_data) |
Sets a callback function to be invoked when the playback is finished. | |
int | player_unset_completed_cb (player_h player) |
Unsets the playback completed callback function. | |
int | player_set_interrupted_cb (player_h player, player_interrupted_cb callback, void *user_data) |
Sets a callback function to be invoked when the playback is interrupted or the interrupt is completed. | |
int | player_unset_interrupted_cb (player_h player) |
Unsets the interrupted callback function. | |
int | player_set_error_cb (player_h player, player_error_cb callback, void *user_data) |
Sets a callback function to be invoked when an error occurs. | |
int | player_unset_error_cb (player_h player) |
Unsets the error callback function. | |
int | player_set_playback_rate (player_h player, float rate) |
Sets the playback rate. | |
int | player_get_current_track (player_h player, player_stream_type_e type, int *index) |
Gets current track index. | |
int | player_get_track_language_code (player_h player, player_stream_type_e type, int index, char **code) |
Gets language code of a track. | |
int | player_get_track_count (player_h player, player_stream_type_e type, int *count) |
Gets the track count. | |
int | player_select_track (player_h player, player_stream_type_e type, int index) |
Selects a track to play. | |
int | player_set_audio_only (player_h player, bool audio_only) |
Sets the audio only mode. | |
int | player_is_audio_only (player_h player, bool *audio_only) |
Gets the audio only mode status. | |
int | player_set_replaygain_enabled (player_h player, bool enabled) |
Sets the player's replaygain status. | |
int | player_is_replaygain_enabled (player_h player, bool *enabled) |
Gets the player's replaygain status. | |
int | player_set_video_roi_area (player_h player, double x_scale, double y_scale, double w_scale, double h_scale) |
Sets the ROI (Region Of Interest) area of the content video source. | |
int | player_get_video_roi_area (player_h player, double *x_scale, double *y_scale, double *w_scale, double *h_scale) |
Gets the ROI (Region Of Interest) area of the content video source. | |
int | player_audio_pitch_set_enabled (player_h player, bool enabled) |
Enables or disables controlling the pitch of audio. | |
int | player_audio_pitch_is_enabled (player_h player, bool *enabled) |
Gets the status of controlling the pitch of audio. | |
int | player_audio_pitch_set_value (player_h player, float value) |
Sets the pitch of audio. | |
int | player_audio_pitch_get_value (player_h player, float *value) |
Gets the pitch of audio. | |
int | player_audio_offload_foreach_supported_format (player_h player, player_supported_media_format_cb callback, void *user_data) |
Retrieves all supported media formats for audio offload. | |
int | player_audio_offload_set_enabled (player_h player, bool enabled) |
Enables or disables the audio offload. | |
int | player_audio_offload_is_enabled (player_h player, bool *enabled) |
Gets the enabling status of audio offload. | |
int | player_audio_offload_is_activated (player_h player, bool *activated) |
Gets the activation status of audio offload. | |
int | player_set_audio_codec_type (player_h player, player_codec_type_e codec_type) |
Sets the default codec type of the audio decoder. | |
int | player_get_audio_codec_type (player_h player, player_codec_type_e *codec_type) |
Gets the default codec type of the audio decoder. | |
Typedefs | |
typedef struct player_s * | player_h |
The media player's type handle. | |
typedef void(* | player_prepared_cb )(void *user_data) |
Called when the media player is prepared. | |
typedef void(* | player_completed_cb )(void *user_data) |
Called when the media player is completed. | |
typedef void(* | player_seek_completed_cb )(void *user_data) |
Called when the seek operation is completed. | |
typedef void(* | player_interrupted_cb )(player_interrupted_code_e code, void *user_data) |
Called when the media player is interrupted. | |
typedef void(* | player_error_cb )(int error_code, void *user_data) |
Called when an error occurs in the media player. | |
typedef void(* | player_video_captured_cb )(unsigned char *captured_data, int width, int height, unsigned int size, void *user_data) |
Called when the video is captured. | |
typedef void(* | player_media_packet_video_decoded_cb )(media_packet_h packet, void *user_data) |
Called to register for notifications about delivering media packet when each video frame is decoded. | |
typedef void(* | player_media_packet_audio_decoded_cb )(media_packet_h packet, void *user_data) |
Called to register for notifications about delivering media packet when audio data is decoded. | |
typedef void(* | player_media_stream_buffer_status_cb )(player_media_stream_buffer_status_e status, void *user_data) |
Called when the buffer level drops below the threshold of max size or no free space in buffer. | |
typedef void(* | player_media_stream_seek_cb )(unsigned long long offset, void *user_data) |
Called to notify the next push-buffer offset when seeking is occurred. | |
typedef bool(* | player_supported_media_format_cb )(media_format_mimetype_e format, void *user_data) |
Called to inform about the supported media format MIME type. |
Typedef Documentation
typedef void(* player_completed_cb)(void *user_data) |
Called when the media player is completed.
It will be invoked when player has reached the end of the stream.
- Since :
- 2.3
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- Precondition:
- It will be invoked when the playback is completed if you register this callback using player_set_completed_cb().
typedef void(* player_error_cb)(int error_code, void *user_data) |
Called when an error occurs in the media player.
Following error codes can be delivered. PLAYER_ERROR_INVALID_OPERATION PLAYER_ERROR_INVALID_STATE PLAYER_ERROR_INVALID_URI PLAYER_ERROR_CONNECTION_FAILED PLAYER_ERROR_DRM_NOT_PERMITTED PLAYER_ERROR_FILE_NO_SPACE_ON_DEVICE PLAYER_ERROR_NOT_SUPPORTED_FILE PLAYER_ERROR_SEEK_FAILED PLAYER_ERROR_SERVICE_DISCONNECTED PLAYER_ERROR_NOT_SUPPORTED_AUDIO_CODEC (Since 4.0) PLAYER_ERROR_NOT_SUPPORTED_VIDEO_CODEC (Since 4.0) PLAYER_ERROR_NOT_SUPPORTED_SUBTITLE (Since 4.0)
- Since :
- 2.3
- Parameters:
-
[in] error_code The error code [in] user_data The user data passed from the callback registration function
typedef struct player_s* player_h |
The media player's type handle.
- Since :
- 2.3
typedef void(* player_interrupted_cb)(player_interrupted_code_e code, void *user_data) |
Called when the media player is interrupted.
If the code is PLAYER_INTERRUPTED_BY_RESOURCE_CONFLICT, the player state will be one of PLAYER_STATE_IDLE, PLAYER_STATE_READY, or PLAYER_STATE_PAUSED. Application should get exact state by calling player_get_state().
- Since :
- 2.3
- Parameters:
-
[in] code The interrupted error code [in] user_data The user data passed from the callback registration function
typedef void(* player_media_packet_audio_decoded_cb)(media_packet_h packet, void *user_data) |
Called to register for notifications about delivering media packet when audio data is decoded.
- Since :
- 5.5
- Remarks:
- The packet should be released by calling media_packet_destroy().
It is recommended to release it as soon as it is rendered, to avoid memory exhaustion.
- Parameters:
-
[in] packet Reference pointer to the media packet [in] user_data The user data passed from the callback registration function
typedef void(* player_media_packet_video_decoded_cb)(media_packet_h packet, void *user_data) |
Called to register for notifications about delivering media packet when each video frame is decoded.
- Since :
- 2.3
- Remarks:
- The UI update code must not be directly invoked.
-
The packet should be released using media_packet_destroy().
If not, the decoder will fail due to having insufficient buffer space for the decoded frame.
- Parameters:
-
[in] packet Reference pointer to the media packet [in] user_data The user data passed from the callback registration function
typedef void(* player_media_stream_buffer_status_cb)(player_media_stream_buffer_status_e status, void *user_data) |
Called when the buffer level drops below the threshold of max size or no free space in buffer.
- Since :
- 2.4
- Remarks:
- This function is used for media stream playback only.
- Parameters:
-
[in] status The buffer status [in] user_data The user data passed from the callback registration function
typedef void(* player_media_stream_seek_cb)(unsigned long long offset, void *user_data) |
Called to notify the next push-buffer offset when seeking is occurred.
The next push-buffer should produce buffers from the new offset.
- Since :
- 2.4
- Remarks:
- This function is used for media stream playback only.
- Parameters:
-
[in] offset The new byte position to seek [in] user_data The user data passed from the callback registration function
typedef void(* player_prepared_cb)(void *user_data) |
Called when the media player is prepared.
It will be invoked when player has reached the begin of stream.
- Since :
- 2.3
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- Precondition:
- player_prepare_async() will cause this callback.
- Postcondition:
- The player state will be PLAYER_STATE_READY.
- See also:
- player_prepare_async()
typedef void(* player_seek_completed_cb)(void *user_data) |
Called when the seek operation is completed.
- Since :
- 2.3
- Parameters:
-
[in] user_data The user data passed from the callback registration function
typedef bool(* player_supported_media_format_cb)(media_format_mimetype_e format, void *user_data) |
Called to inform about the supported media format MIME type.
The supported media format can vary depending on the device capabilities.
- Since :
- 5.5
- Parameters:
-
[in] format The supportable media format MIME type [in] user_data The user data passed from the callback registration function
- Returns:
true
to continue with the next iteration of the loop, otherwisefalse
to break out of the loop
typedef void(* player_video_captured_cb)(unsigned char *captured_data, int width, int height, unsigned int size, void *user_data) |
Called when the video is captured.
- Since :
- 2.3
- Remarks:
- The color space format of the captured image is IMAGE_UTIL_COLORSPACE_RGB888.
- The captured_data should not be released and it can be used only in the callback. To use outside, make a copy.
- Parameters:
-
[in] captured_data The captured image buffer [in] width The width of the captured image [in] height The height of the captured image [in] size The size of the captured image [in] user_data The user data passed from the callback registration function
- See also:
- player_capture_video()
Enumeration Type Documentation
enum audio_latency_mode_e |
Enumeration of audio extract option.
- Since :
- 5.5
- Enumerator:
enum player_codec_type_e |
Enumeration for display type.
- Since :
- 2.3
In case of using PLAYER_DISPLAY_TYPE_OVERLAY_SYNC_UI, the video is rendering in full scale in the rendering area.
To change the video rendering position, use UI API functions like ecore_wl2_subsurface_exported_surface_move(), ecore_wl2_subsurface_exported_surface_resize(), ecore_wl2_subsurface_exported_surface_transform_set(), ecore_wl2_subsurface_exported_surface_show() and ecore_wl2_subsurface_exported_surface_commit().
player_set_display_mode(), player_set_display_rotation() and player_set_display_roi_area() cannot be used and PLAYER_ERROR_NOT_AVAILABLE is returned if they are used.
Exported shell handle must be obtained by ecore_wl2_subsurface_exported_surface_handle_get() from Ecore_Wl2_Subsurface.
Then the exported shell handle should be set with player_set_display().
To render the first video buffer, commit by ecore_wl2_window_commit() after player_prepare() of Ecore_Wl2_Subsurface.
Always commit with ecore_wl2_window_commit() with parent surface when the UI changes and the video needs to be synchronized.
- Enumerator:
enum player_error_e |
Enumeration for media player's error codes.
- Since :
- 2.3
- Enumerator:
Enumeration of media stream buffer status.
- Since :
- 2.4
enum player_state_e |
Enumeration for media player state.
- Since :
- 2.3
enum player_stream_type_e |
Function Documentation
int player_audio_offload_foreach_supported_format | ( | player_h | player, |
player_supported_media_format_cb | callback, | ||
void * | user_data | ||
) |
Retrieves all supported media formats for audio offload.
The supported media format can vary depending on the device capabilities.
- Since :
- 5.5
- Remarks:
- This function is related to the following feature:
http://tizen.org/feature/multimedia.player.audio_offload
- Parameters:
-
[in] player The handle to the media player [in] callback The iteration callback function [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_FEATURE_NOT_SUPPORTED_ON_DEVICE Unsupported feature
int player_audio_offload_is_activated | ( | player_h | player, |
bool * | activated | ||
) |
Gets the activation status of audio offload.
Audio offload could be inactivated depending on the audio device capability even if the audio offload feature is supported.
- Since :
- 5.5
- Remarks:
- This function is related to the following feature:
http://tizen.org/feature/multimedia.player.audio_offload
- Parameters:
-
[in] player The handle to the media player [out] activated The activation status
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_STATE Invalid state PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_FEATURE_NOT_SUPPORTED_ON_DEVICE Unsupported feature
- Precondition:
- The player state must be one of PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED
int player_audio_offload_is_enabled | ( | player_h | player, |
bool * | enabled | ||
) |
Gets the enabling status of audio offload.
- Since :
- 5.5
- Remarks:
- This function is related to the following feature:
http://tizen.org/feature/multimedia.player.audio_offload
- Parameters:
-
[in] player The handle to the media player [out] enabled The enabling status (default: false)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_FEATURE_NOT_SUPPORTED_ON_DEVICE Unsupported feature
int player_audio_offload_set_enabled | ( | player_h | player, |
bool | enabled | ||
) |
Enables or disables the audio offload.
The player lets the hardware decode and render the sound if the audio offload is enabled. This will reduce the power consumption, but will disable the ability to handle output PCM. Please check the remarks for a list of functions which will not work if offloading is enabled.
- Since :
- 5.5
- Remarks:
- This function is related to the following feature:
http://tizen.org/feature/multimedia.player.audio_offload
-
The sound stream type of the player should be SOUND_STREAM_TYPE_MEDIA.
-
If audio offload is enabled, functions for audio effect are not available.
-
If audio offload is enabled, the following functions will return PLAYER_ERROR_NOT_AVAILABLE and they will not work at all even if they were called before enabling offload. :
player_set_media_packet_audio_frame_decoded_cb()
player_unset_media_packet_audio_frame_decoded_cb()
player_set_audio_latency_mode()
player_get_audio_latency_mode()
player_set_playback_rate()
player_get_current_track()
player_get_track_language_code()
player_get_track_count()
player_select_track()
player_set_replaygain_enabled()
player_is_replaygain_enabled()
player_audio_pitch_set_enabled()
player_audio_pitch_is_enabled()
player_audio_pitch_set_value()
player_audio_pitch_get_value()
- Parameters:
-
[in] player The handle to the media player [in] enabled The new audio offload status (default: false)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_FEATURE_NOT_SUPPORTED_ON_DEVICE Unsupported feature
- Precondition:
- The player state must be PLAYER_STATE_IDLE.
int player_audio_pitch_get_value | ( | player_h | player, |
float * | value | ||
) |
Gets the pitch of audio.
- Since :
- 5.5
- Remarks:
- This function is used for audio content only.
- Depending on audio codec type or by enabling audio offload, this function could be unavailable and this will return PLAYER_ERROR_NOT_AVAILABLE.
- Parameters:
-
[in] player The handle to the media player [out] value The audio stream pitch value
Valid range is 0.5~2. Default value is 1.
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_NOT_AVAILABLE Not available
int player_audio_pitch_is_enabled | ( | player_h | player, |
bool * | enabled | ||
) |
Gets the status of controlling the pitch of audio.
- Since :
- 5.5
- Remarks:
- This function is used for audio content only.
- Depending on audio codec type or by enabling audio offload, this function could be unavailable and this will return PLAYER_ERROR_NOT_AVAILABLE.
- Parameters:
-
[in] player The handle to the media player [out] enabled The audio pitch control status (default: false)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_NOT_AVAILABLE Not available
int player_audio_pitch_set_enabled | ( | player_h | player, |
bool | enabled | ||
) |
Enables or disables controlling the pitch of audio.
- Since :
- 5.5
- Remarks:
- This function is used for audio content only.
- Enabling pitch control could increase the CPU usage on some devices.
- If audio offload is enabled by calling player_audio_offload_set_enabled(), this function will return PLAYER_ERROR_NOT_AVAILABLE and this will not work at all even if this was called before enabling offload.
- This function could be unavailable depending on the audio codec type and this will return PLAYER_ERROR_NOT_AVAILABLE.
- Parameters:
-
[in] player The handle to the media player [in] enabled The new audio pitch control status (default: false)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_NOT_AVAILABLE Not available
- Precondition:
- The player state must be PLAYER_STATE_IDLE.
int player_audio_pitch_set_value | ( | player_h | player, |
float | value | ||
) |
Sets the pitch of audio.
- Since :
- 5.5
- Remarks:
- This function is used for audio content only.
- If audio offload is enabled by calling player_audio_offload_set_enabled(), this function will return PLAYER_ERROR_NOT_AVAILABLE and this will not work at all even if this was called before enabling offload.
- This function could be unavailable depending on the audio codec type and this will return PLAYER_ERROR_NOT_AVAILABLE.
- Parameters:
-
[in] player The handle to the media player [in] value The audio stream pitch value
Valid range is 0.5~2. Default value is 1.
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_NOT_AVAILABLE Not available
- Precondition:
- The pitch control must be enabled by calling player_audio_pitch_set_enabled() function.
int player_capture_video | ( | player_h | player, |
player_video_captured_cb | callback, | ||
void * | user_data | ||
) |
Captures the video frame, asynchronously.
- Since :
- 2.3
- Remarks:
- If the content is encrypted or there are copyright issues with it, this function could be unsupported depending on the DRM policy. In case the function is not supported by the DRM policy, it will return an error.
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_PLAYING by calling player_start() or set to PLAYER_STATE_PAUSED by calling player_pause().
- Video display type should be set by calling player_set_display() otherwise, audio stream is only processed even though video file is set.
- Postcondition:
- It invokes player_video_captured_cb() when capture completes, if you set a callback.
- See also:
- player_video_captured_cb()
int player_create | ( | player_h * | player | ) |
Creates a player handle for playing multimedia content.
- Since :
- 2.3
- Remarks:
- You must release player by using player_destroy().
Although you can create multiple player handles at the same time, the player cannot guarantee proper operation because of limited resources, such as audio or display device.
- Parameters:
-
[out] player A new handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_OUT_OF_MEMORY Out of memory PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_RESOURCE_LIMIT Cannot create more instance due to resource(socket, thread, etc) limitation on system.
- Postcondition:
- The player state will be PLAYER_STATE_IDLE.
- See also:
- player_destroy()
int player_destroy | ( | player_h | player | ) |
Destroys the media player handle and releases all its resources.
- Since :
- 2.3
- Remarks:
- To completely shutdown player operation, call this function with a valid player handle from any player state.
- Parameters:
-
[in] player The handle to the media player to be destroyed
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- Precondition:
- The player state must be one of PLAYER_STATE_IDLE, PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- Postcondition:
- The player state will be PLAYER_STATE_NONE.
- See also:
- player_create()
int player_foreach_media_stream_supported_format | ( | player_h | player, |
player_supported_media_format_cb | callback, | ||
void * | user_data | ||
) |
Retrieves all supported media formats for the playback of external media stream.
The supported media format can vary depending on the device capabilities.
- Since :
- 5.5
- Parameters:
-
[in] player The handle to the media player [in] callback The iteration callback function [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
int player_get_audio_codec_type | ( | player_h | player, |
player_codec_type_e * | codec_type | ||
) |
Gets the default codec type of the audio decoder.
- Since :
- 5.5
- Parameters:
-
[in] player The handle to the media player [out] codec_type The default codec type
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_audio_codec_type()
int player_get_audio_latency_mode | ( | player_h | player, |
audio_latency_mode_e * | latency_mode | ||
) |
Gets the current audio latency mode.
- Since :
- 2.3
- Remarks:
- If audio offload is enabled by calling player_audio_offload_set_enabled(), this function will return PLAYER_ERROR_NOT_AVAILABLE. (Since 5.5)
- Parameters:
-
[in] player The handle to the media player [out] latency_mode The latency mode to get from the audio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_NOT_AVAILABLE Not available (Since 5.5)
int player_get_current_track | ( | player_h | player, |
player_stream_type_e | type, | ||
int * | index | ||
) |
Gets current track index.
Index starts from 0.
- Since :
- 2.4
- Remarks:
- If audio offload is enabled by calling player_audio_offload_set_enabled(), this function will return PLAYER_ERROR_NOT_AVAILABLE. (Since 5.5)
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream which is PLAYER_STREAM_TYPE_AUDIO or PLAYER_STREAM_TYPE_TEXT [out] index The index of track
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_NOT_AVAILABLE Not available (Since 5.5)
- Precondition:
- The player state must be one of PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED
- See also:
- player_audio_offload_set_enabled()
int player_get_media_stream_buffer_max_size | ( | player_h | player, |
player_stream_type_e | type, | ||
unsigned long long * | max_size | ||
) |
Gets the max size bytes of buffer.
- Since :
- 2.4
- Remarks:
- This function is used for media stream playback only.
- If the buffer level over the max size, player_media_stream_buffer_status_cb() will be invoked with overflow status.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [out] max_size The max bytes of buffer
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
int player_get_media_stream_buffer_min_threshold | ( | player_h | player, |
player_stream_type_e | type, | ||
unsigned int * | percent | ||
) |
Gets the buffer threshold percent of buffer.
- Since :
- 2.4
- Remarks:
- This function is used for media stream playback only.
- If the buffer level drops below the percent value, player_media_stream_buffer_status_cb() will be invoked with underrun status.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [out] percent The minimum threshold(0~100) of buffer
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
int player_get_play_position | ( | player_h | player, |
int * | milliseconds | ||
) |
Gets the current position in milliseconds.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [out] milliseconds The current position in milliseconds
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_SEEK_FAILED Seek operation failure
int player_get_play_position_nsec | ( | player_h | player, |
int64_t * | nanoseconds | ||
) |
Gets the current position in nanoseconds.
- Since :
- 5.0
- Parameters:
-
[in] player The handle to the media player [out] nanoseconds The current position in nanoseconds
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_SEEK_FAILED Seek operation failure
int player_get_state | ( | player_h | player, |
player_state_e * | state | ||
) |
Gets the player's current state.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [out] state The current state of the player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- See also:
- player_state_e
int player_get_track_count | ( | player_h | player, |
player_stream_type_e | type, | ||
int * | count | ||
) |
Gets the track count.
- Since :
- 2.4
- Remarks:
- If audio offload is enabled by calling player_audio_offload_set_enabled(), this function will return PLAYER_ERROR_NOT_AVAILABLE. (Since 5.5)
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream which is PLAYER_STREAM_TYPE_AUDIO or PLAYER_STREAM_TYPE_TEXT [out] count The number of track
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_NOT_AVAILABLE Not available (Since 5.5)
- Precondition:
- The player state must be one of PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED
- See also:
- player_audio_offload_set_enabled()
int player_get_track_language_code | ( | player_h | player, |
player_stream_type_e | type, | ||
int | index, | ||
char ** | code | ||
) |
Gets language code of a track.
- Since :
- 2.4
- Remarks:
- code must be released with
free()
by caller - If audio offload is enabled by calling player_audio_offload_set_enabled(), this function will return PLAYER_ERROR_NOT_AVAILABLE. (Since 5.5)
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream which is PLAYER_STREAM_TYPE_AUDIO or PLAYER_STREAM_TYPE_TEXT [in] index The index of track [out] code A language code in ISO 639-1. "und" will be returned if the language is undefined.
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_NOT_AVAILABLE Not available (Since 5.5)
- Precondition:
- The player state must be one of PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED
- See also:
- player_audio_offload_set_enabled()
int player_get_video_roi_area | ( | player_h | player, |
double * | x_scale, | ||
double * | y_scale, | ||
double * | w_scale, | ||
double * | h_scale | ||
) |
Gets the ROI (Region Of Interest) area of the content video source.
- Since :
- 5.0
- Remarks:
- This function gets the ratio value of the each coordinate and size based on the video resolution size.
- The ROI area is valid only in PLAYER_DISPLAY_TYPE_OVERLAY.
- Parameters:
-
[in] player The handle to the media player [out] x_scale The current X coordinate ratio value of the video source area based on the video width size [out] y_scale The current Y coordinate ratio value of the video source area based on the video height size [out] w_scale The current width ratio value of the video source area based on the video width size [out] h_scale The current height ratio value of the video source area based on the video height size
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
int player_get_volume | ( | player_h | player, |
float * | left, | ||
float * | right | ||
) |
Gets the player's current volume factor.
The range of left and right is from 0
to 1.0
, inclusive (1.0 = 100%). This function gets the player volume, not the system volume. To get the system volume, use the Sound Manager API.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [out] left The current left volume scalar [out] right The current right volume scalar
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_volume()
int player_is_audio_only | ( | player_h | player, |
bool * | audio_only | ||
) |
Gets the audio only mode status.
- Since :
- 4.0
- Parameters:
-
[in] player The handle to the media player [out] audio_only The current audio only status: ( true
= audio only enabled,false
= audio only disabled)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_audio_only()
int player_is_looping | ( | player_h | player, |
bool * | looping | ||
) |
Gets the player's looping status.
If the looping status is true
, playback automatically restarts upon finishing. If it is false
, it won't.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [out] looping The looping status: ( true
= looping,false
= non-looping )
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_looping()
int player_is_muted | ( | player_h | player, |
bool * | muted | ||
) |
Gets the player's mute status.
If the mute status is true
, no sounds are played. If it is false
, sounds are played at the previously set volume level.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [out] muted The current mute status: ( true
= mute,false
= not muted)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_mute()
int player_is_replaygain_enabled | ( | player_h | player, |
bool * | enabled | ||
) |
Gets the player's replaygain status.
- Since :
- 5.0
- Remarks:
- Depending on audio codec type or by enabling audio offload, this function could be unavailable and this will return PLAYER_ERROR_NOT_AVAILABLE. (Since 5.5)
- Parameters:
-
[in] player The handle to the media player [out] enabled Pointer to store current replaygain status: ( true
= enabled replaygain,false
= disabled replaygain)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_NOT_AVAILABLE Not available (Since 5.5)
int player_pause | ( | player_h | player | ) |
Pauses the player.
- Since :
- 2.3
- Remarks:
- You can resume playback using player_start().
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid state PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_SOUND_POLICY Sound policy error
- Precondition:
- The player state must be set to PLAYER_STATE_PLAYING.
- Postcondition:
- The player state will be PLAYER_STATE_PAUSED.
- See also:
- player_start()
int player_prepare | ( | player_h | player | ) |
Prepares the media player for playback.
- Since :
- 2.3
- Remarks:
- The mediastorage privilege(http://tizen.org/privilege/mediastorage) must be added if any video/audio files are used to play located in the internal storage.
- The externalstorage privilege(http://tizen.org/privilege/externalstorage) must be added if any video/audio files are used to play located in the external storage.
- The internet privilege(http://tizen.org/privilege/internet) must be added if any URLs are used to play from network.
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_URI Invalid URI PLAYER_ERROR_NO_SUCH_FILE File not found PLAYER_ERROR_NOT_SUPPORTED_FILE File not supported PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_PERMISSION_DENIED Permission denied PLAYER_ERROR_NOT_SUPPORTED_AUDIO_CODEC Not support audio codec format (Since 4.0) PLAYER_ERROR_NOT_SUPPORTED_VIDEO_CODEC Not support video codec format (Since 4.0)
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare(). After that, call player_set_uri() to load the media content you want to play.
- Postcondition:
- The player state will be PLAYER_STATE_READY.
int player_prepare_async | ( | player_h | player, |
player_prepared_cb | callback, | ||
void * | user_data | ||
) |
Prepares the media player for playback, asynchronously.
- Since :
- 2.3
- Remarks:
- The mediastorage privilege(http://tizen.org/privilege/mediastorage) must be added if any video/audio files are used to play located in the internal storage. The externalstorage privilege(http://tizen.org/privilege/externalstorage) must be added if any video/audio files are used to play located in the external storage. The internet privilege(http://tizen.org/privilege/internet) must be added if any URLs are used to play from network.
Since 5.0: To cancel the asynchronous preparing, call player_unprepare() even in PLAYER_STATE_IDLE state.
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_URI Invalid URI PLAYER_ERROR_NO_SUCH_FILE File not found PLAYER_ERROR_NOT_SUPPORTED_FILE File not supported PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare(). After that, call player_set_uri() to load the media content you want to play.
- Postcondition:
- It invokes player_prepared_cb() when playback is prepared.
int player_push_media_stream | ( | player_h | player, |
media_packet_h | packet | ||
) |
Pushes elementary stream to decode audio or video.
- Since :
- 2.4
- Remarks:
- player_set_media_stream_info() must be called before using this function.
- The available buffer size can be set by calling player_set_media_stream_buffer_max_size(). If there is no available buffer space, this function will return error since 3.0.
- Parameters:
-
[in] player The handle to media player [in] packet The media packet to decode
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid state PLAYER_ERROR_NOT_SUPPORTED_FILE File not supported PLAYER_ERROR_BUFFER_SPACE No buffer space available (since 3.0) PLAYER_ERROR_INVALID_OPERATION Invalid operation (since 3.0)
- Precondition:
- The player state must be one of PLAYER_STATE_READY, PLAYER_STATE_PLAYING or PLAYER_STATE_PAUSED.
int player_select_track | ( | player_h | player, |
player_stream_type_e | type, | ||
int | index | ||
) |
Selects a track to play.
- Since :
- 2.4
- Remarks:
- If audio offload is enabled by calling player_audio_offload_set_enabled(), this function will return PLAYER_ERROR_NOT_AVAILABLE. (Since 5.5)
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream which is PLAYER_STREAM_TYPE_AUDIO or PLAYER_STREAM_TYPE_TEXT [in] index The index of track
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_NOT_AVAILABLE Not available (Since 5.5)
- Precondition:
- The player state must be one of PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED
int player_set_audio_codec_type | ( | player_h | player, |
player_codec_type_e | codec_type | ||
) |
Sets the default codec type of the audio decoder.
- Since :
- 5.5
- Remarks:
- The default codec type could be different depending on the device capability. S/W codec type is supported basically.
-
If selected H/W audio codec type does not support in some cases, S/W audio codec type could be used instead.
-
If application use the H/W audio codec type by default, following functions should be called after setting codec type because the availability could be changed depending on the codec capability. :
player_audio_effect_equalizer_is_available()
player_set_media_packet_audio_frame_decoded_cb()
player_unset_media_packet_audio_frame_decoded_cb()
player_set_replaygain_enabled()
player_is_replaygain_enabled()
player_audio_pitch_set_enabled()
player_audio_pitch_is_enabled()
player_audio_pitch_set_value()
player_audio_pitch_get_value()
- Parameters:
-
[in] player The handle to the media player [in] codec_type The default codec type
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_NOT_SUPPORTED_AUDIO_CODEC Not support audio codec type
- Precondition:
- The player state must be PLAYER_STATE_IDLE by player_create() or player_unprepare().
- See also:
- player_get_audio_codec_type()
int player_set_audio_latency_mode | ( | player_h | player, |
audio_latency_mode_e | latency_mode | ||
) |
Sets the audio latency mode.
- Since :
- 2.3
- Remarks:
- The default audio latency mode of the player is AUDIO_LATENCY_MODE_MID. To get the current audio latency mode, use player_get_audio_latency_mode(). If it's high mode, audio output interval can be increased so, it can keep more audio data to play. But, state transition like pause or resume can be more slower than default(mid) mode.
- If audio offload is enabled by calling player_audio_offload_set_enabled(), this function will return PLAYER_ERROR_NOT_AVAILABLE and this will not work at all even if it was called before enabling offload. (Since 5.5)
- Parameters:
-
[in] player The handle to the media player [in] latency_mode The latency mode to be applied to the audio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_NOT_AVAILABLE Not available (Since 5.5)
int player_set_audio_only | ( | player_h | player, |
bool | audio_only | ||
) |
Sets the audio only mode.
This function is used to disable or enable video rendering during playback.
- Since :
- 4.0
- Parameters:
-
[in] player The handle to the media player [in] audio_only The new audio only status: ( true
= enable audio only,false
= disable audio only)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be one of: PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- See also:
- player_is_audio_only()
int player_set_completed_cb | ( | player_h | player, |
player_completed_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to be invoked when the playback is finished.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- Postcondition:
- player_completed_cb() will be invoked.
int player_set_display | ( | player_h | player, |
player_display_type_e | type, | ||
player_display_h | display | ||
) |
Sets the video display.
- Since :
- 2.3
- Remarks:
- To get display to set, use GET_DISPLAY().
- We are not supporting changing display.
-
This function must be called before calling the player_prepare() or player_prepare_async()
to reflect the display type. - This function must be called in main thread of application. Otherwise, it will return PLAYER_ERROR_INVALID_OPERATION by internal restriction. To avoid PLAYER_ERROR_INVALID_OPERATION in sub thread, ecore_thread_main_loop_begin() and ecore_thread_main_loop_end() can be used, but deadlock can be also occurred if main thread is busy. So, it's not recommended to use them. (since 5.0)
- Parameters:
-
[in] player The handle to the media player [in] type The display type [in] display The handle to display
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
int player_set_error_cb | ( | player_h | player, |
player_error_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to be invoked when an error occurs.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- Postcondition:
- player_error_cb() will be invoked.
- See also:
- player_unset_error_cb()
- player_error_cb()
int player_set_interrupted_cb | ( | player_h | player, |
player_interrupted_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to be invoked when the playback is interrupted or the interrupt is completed.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- Postcondition:
- player_interrupted_cb() will be invoked.
int player_set_looping | ( | player_h | player, |
bool | looping | ||
) |
Sets the player's looping status.
If the looping status is true
, playback automatically restarts upon finishing. If it is false
, it won't. The default value is false
.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] looping The new looping status: ( true
= looping,false
= non-looping )
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_is_looping()
int player_set_media_packet_audio_frame_decoded_cb | ( | player_h | player, |
media_format_h | format, | ||
player_audio_extract_option_e | opt, | ||
player_media_packet_audio_decoded_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function for getting the decoded audio data.
This function is used to get audio PCM data of input media content via registered callback.
An application can specify the output PCM format by Media Format API.
- Since :
- 5.5
- Remarks:
- This function must be called before calling player_prepare() or player_prepare_async().
A registered callback is called in a separate thread (not in the main loop).
The audio PCM data can be retrieved using a registered callback as a media packet and it is available until it's destroyed by media_packet_destroy().
The packet has to be destroyed as quickly as possible after rendering the data
and all the packets have to be destroyed before player_unprepare() is called.
- If audio offload is enabled by calling player_audio_offload_set_enabled(), this function will return PLAYER_ERROR_NOT_AVAILABLE and this will not work at all even if this was called before enabling offload.
- This function could be unavailable depending on the audio codec type and this will return PLAYER_ERROR_NOT_AVAILABLE.
- If the content is encrypted or there are copyright issues with it, this function could be unsupported depending on the DRM policy.
- Parameters:
-
[in] player The handle to the media player [in] format The media format handle about required audio PCM specification. This format has to include PCM MIME type, audio channel and sampling rate. If the format is NULL, the original PCM format or platform default PCM format will be applied. [in] opt The audio extract option [in] callback The callback function to be registered [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid state PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_NOT_AVAILABLE Not available
- Precondition:
- The player's state must be PLAYER_STATE_IDLE.
- See also:
- player_unset_media_packet_audio_frame_decoded_cb()
- player_audio_offload_set_enabled()
- player_audio_offload_is_enabled()
- player_set_audio_codec_type()
- player_get_audio_codec_type()
- Example
#include <player.h> #include <media_format.h> ... player_h player = NULL; media_format_h a_format = NULL; ... media_format_create(&a_format); media_format_set_audio_mime(a_format, MEDIA_FORMAT_PCM_F32LE); media_format_set_audio_channel(a_format, 2); media_format_set_audio_samplerate(a_format, 44100); player_set_media_packet_audio_frame_decoded_cb(player, a_format, PLAYER_AUDIO_EXTRACT_DEFAULT, _audio_pcm_cb, udata); media_format_unref(a_format); ...
int player_set_media_packet_video_frame_decoded_cb | ( | player_h | player, |
player_media_packet_video_decoded_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function for getting the decoded video frame.
- Since :
- 2.3
- Remarks:
- This function must be called before calling player_prepare() or player_prepare_async().
A registered callback is called in a separate thread (not in the main loop).
A video frame can be retrieved using a registered callback as a media packet.
The callback function holds the same buffer that will be drawn on the display device.
So if you change the media packet in a registered callback, it will be displayed on the device
and the media packet is available until it's destroyed by media_packet_destroy().
The packet have to be destroyed as quickly as possible after rendering the packet
and all the packets have to be destroyed before player_unprepare() is called.
- If the content is encrypted or there are copyright issues with it, this function could be unsupported depending on the DRM policy.
- Parameters:
-
[in] player The handle to the media player [in] callback The callback function to be registered [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid state
- Precondition:
- The player's state must be PLAYER_STATE_IDLE. And, PLAYER_DISPLAY_TYPE_NONE must be set by calling player_set_display().
int player_set_media_stream_buffer_max_size | ( | player_h | player, |
player_stream_type_e | type, | ||
unsigned long long | max_size | ||
) |
Sets the max size bytes of buffer.
- Since :
- 2.4
- Remarks:
- This function is used for media stream playback only.
- If the buffer level over the max size, player_media_stream_buffer_status_cb() will be invoked with overflow status.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [in] max_size The max bytes of buffer, it has to be bigger than zero. (default: 200000)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
int player_set_media_stream_buffer_min_threshold | ( | player_h | player, |
player_stream_type_e | type, | ||
unsigned int | percent | ||
) |
Sets the buffer threshold percent of buffer.
- Since :
- 2.4
- Remarks:
- This function is used for media stream playback only.
- If the buffer level drops below the percent value, player_media_stream_buffer_status_cb() will be invoked with underrun status.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [in] percent The minimum threshold(0~100) of buffer (default: 0)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
int player_set_media_stream_buffer_status_cb | ( | player_h | player, |
player_stream_type_e | type, | ||
player_media_stream_buffer_status_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to be invoked when buffer underrun or overflow is occurred.
- Since :
- 2.4
- Remarks:
- This function is used for media stream playback only.
- The callback is called in a separate thread (not in the main loop).
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [in] callback The buffer status callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
- Postcondition:
- player_media_stream_buffer_status_cb() will be invoked.
int player_set_media_stream_info | ( | player_h | player, |
player_stream_type_e | type, | ||
media_format_h | format | ||
) |
Sets contents information for media stream.
- Since :
- 2.4
- Remarks:
- AV format must be set before pushing elementary stream with player_push_media_stream().
- This function must be called before calling the player_prepare() or player_prepare_async() to reflect the media information.
- The supported media format MIME type can be checked by calling player_foreach_media_stream_supported_format(). (Since 5.5)
- Parameters:
-
[in] player The handle to media player [in] type The type of target stream [in] format The media format to set media information
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid state PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_NOT_SUPPORTED_FORMAT Not supported format (Since 5.5)
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
int player_set_media_stream_seek_cb | ( | player_h | player, |
player_stream_type_e | type, | ||
player_media_stream_seek_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to be invoked when seeking is occurred.
- Since :
- 2.4
- Remarks:
- This function is used for media stream playback only.
- The callback is called in a separate thread (not in the main loop).
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
- Postcondition:
- player_media_stream_seek_cb() will be invoked.
int player_set_memory_buffer | ( | player_h | player, |
const void * | data, | ||
int | size | ||
) |
Sets memory as the data source.
Associates media content, cached in memory, with the player. Unlike the case of player_set_uri(), the media resides in memory. If the function call is successful, subsequent calls to player_prepare() and player_start() will start playing the media.
- Since :
- 2.3
- Remarks:
- If you provide an invalid data, you won't receive an error message until you call player_start().
- This function must be called before calling the player_prepare() or player_prepare_async() to build the player based on the data.
- Parameters:
-
[in] player The handle to the media player [in] data The memory pointer of media data [in] size The size of media data
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
- See also:
- player_set_uri()
int player_set_mute | ( | player_h | player, |
bool | muted | ||
) |
Sets the player's mute status.
If the mute status is true
, no sounds are played. If it is false
, sounds are played at the previously set volume level. Until this function is called, by default the player is not muted.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] muted The new mute status: ( true
= mute,false
= not muted)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_is_muted()
int player_set_play_position | ( | player_h | player, |
int | milliseconds, | ||
bool | accurate, | ||
player_seek_completed_cb | callback, | ||
void * | user_data | ||
) |
Sets the seek position for playback, asynchronously.
- Since :
- 2.3
- Remarks:
- This function will trigger the seeking operation on player instance like in the case of player_set_play_position_nsec(). Normally application needs to wait for player_seek_completed_cb() before calling setting position function again. Otherwise it will return PLAYER_ERROR_SEEK_FAILED.
- Calling player_pause() or player_start() before player_seek_completed_cb() is invoked will cause PLAYER_ERROR_INVALID_OPERATION to be returned.
- Please note that if application is playing external media data via player_set_media_stream_info(), then consecutive calling of this function will always succeed and there is no need to wait for player_seek_completed_cb() before next calling of this function.(Since 3.0)
- Even if you don't set visible to true by calling player_set_display_visible(), the video will be shown when the player_seek_completed_cb() is invoked.
- In case of non-seekable content, the function will return PLAYER_ERROR_INVALID_OPERATION and the player will keep playing without changing the play position.
- Parameters:
-
[in] player The handle to the media player [in] milliseconds The position in milliseconds from the start to the seek point [in] accurate If true
the nearest frame position is returned, but this might be considerably slow, iffalse
the nearest key frame position is returned, this might be faster but less accurate.[in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_SEEK_FAILED Seek operation failure
- Precondition:
- The player state must be one of PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- Postcondition:
- It invokes player_seek_completed_cb() when seek operation completes, if you set a callback.
int player_set_play_position_nsec | ( | player_h | player, |
int64_t | nanoseconds, | ||
bool | accurate, | ||
player_seek_completed_cb | callback, | ||
void * | user_data | ||
) |
Sets the seek position in nanoseconds for playback, asynchronously.
- Since :
- 5.0
- Remarks:
- This function will trigger the seeking operation on player instance like in the case of player_set_play_position(). Normally application needs to wait for player_seek_completed_cb() before calling setting position function again. Otherwise it will return PLAYER_ERROR_SEEK_FAILED.
- Calling player_pause() or player_start() before player_seek_completed_cb() is invoked will cause PLAYER_ERROR_INVALID_OPERATION to be returned.
- Please note that if application is playing external media data via player_set_media_stream_info(), then consecutive calling of this function will always succeed and there is no need to wait for player_seek_completed_cb() before next calling of this function.
- Even if you don't set visible to true by calling player_set_display_visible(), the video will be shown when the player_seek_completed_cb() is invoked.
- In case of non-seekable content, the function will return PLAYER_ERROR_INVALID_OPERATION and the player will keep playing without changing the play position.
- Parameters:
-
[in] player The handle to the media player [in] nanoseconds The position in nanoseconds from the start to the seek point [in] accurate If true
the nearest frame position is returned, but this might be considerably slow, iffalse
the nearest key frame position is returned, this might be faster but less accurate.[in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_SEEK_FAILED Seek operation failure
- Precondition:
- The player state must be one of PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- Postcondition:
- It invokes player_seek_completed_cb() when seek operation completes, if you set a callback.
int player_set_playback_rate | ( | player_h | player, |
float | rate | ||
) |
Sets the playback rate.
The default value is 1.0
.
- Since :
- 2.3
- Remarks:
- PLAYER_ERROR_INVALID_OPERATION occurs when streaming playback.
-
No operation is performed, if rate is
0
. -
The sound is muted, when playback rate is under
0.0
and over2.0
. - If audio offload is enabled by calling player_audio_offload_set_enabled(), this function will return PLAYER_ERROR_NOT_AVAILABLE. (Since 5.5)
- Parameters:
-
[in] player The handle to the media player [in] rate The playback rate (-5.0x ~ 5.0x)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_NOT_AVAILABLE Not available (Since 5.5)
- Precondition:
- The player state must be one of PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- See also:
- player_audio_offload_set_enabled()
int player_set_replaygain_enabled | ( | player_h | player, |
bool | enabled | ||
) |
Sets the player's replaygain status.
If the replaygain status is true
, replaygain is applied (if contents has a replaygain tag). If it is false
, the replaygain isn't affected by tag and properties.
- Since :
- 5.0
- Remarks:
- If audio offload is enabled by calling player_audio_offload_set_enabled(), this function will return PLAYER_ERROR_NOT_AVAILABLE and this will not work at all even if this was called before enabling offload. (Since 5.5)
- This function could be unavailable depending on the audio codec type and this will return PLAYER_ERROR_NOT_AVAILABLE. (Since 5.5)
- Parameters:
-
[in] player The handle to the media player [in] enabled The new replaygain status: ( true
= enable,false
= disable)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_NOT_AVAILABLE Not available (Since 5.5)
int player_set_sound_stream_info | ( | player_h | player, |
sound_stream_info_h | stream_info | ||
) |
Sets the player's sound manager stream information.
- Since :
- 3.0
- Remarks:
- You can set sound stream information including audio routing and volume type. For more details, please refer to sound_manager.h
- This function must be called before calling the player_prepare() or player_prepare_async() to reflect the sound stream information when the player is building.
-
This function is related to the following feature:
http://tizen.org/feature/multimedia.player.stream_info
If this feature is not supported, the stream_type of the player is fixed to the SOUND_STREAM_TYPE_MEDIA.
- Parameters:
-
[in] player The handle to the media player [in] stream_info The sound manager info type
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_FEATURE_NOT_SUPPORTED_ON_DEVICE Unsupported feature
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create().
- See also:
- sound_stream_info_h
- sound_manager_create_stream_information()
- sound_manager_destroy_stream_information()
- Example
#include <player.h> #include <sound_manager.h> ... player_h player = NULL; sound_stream_info_h stream_info = NULL; sound_stream_type_e stream_type = SOUND_STREAM_TYPE_MEDIA; ... sound_manager_create_stream_information (stream_type, _focus_callback, use_data, &stream_info); player_create (&player); player_set_sound_stream_info (player, stream_info); ... player_prepare_async (player, _prepared_cb, udata); ...
int player_set_uri | ( | player_h | player, |
const char * | uri | ||
) |
Sets the data source (file-path, HTTP or RTSP URI) to use.
Associates media contents, referred to by the URI, with the player. If the function call is successful, subsequent calls to player_prepare() and player_start() will start playing the media.
- Since :
- 2.3
- Remarks:
- If you use HTTP or RTSP, URI must start with "http://" or "rtsp://". The default protocol is "file://". If you provide an invalid URI, you won't receive an error message until you call player_start().
- This function must be called before calling the player_prepare() or player_prepare_async() to build the player based on the URI information.
- The mediastorage privilege(http://tizen.org/privilege/mediastorage) must be added if any video/audio files are used to play located in the internal storage.
- The externalstorage privilege(http://tizen.org/privilege/externalstorage) must be added if any video/audio files are used to play located in the external storage.
- The internet privilege(http://tizen.org/privilege/internet) must be added if any URLs are used to play from network.
- Parameters:
-
[in] player The handle to the media player [in] uri The content location, such as the file path, the URI of the HTTP or RTSP stream you want to play
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_PERMISSION_DENIED Permission denied
- Precondition:
- The player state must be set to PLAYER_STATE_IDLE by calling player_create() or player_unprepare().
- See also:
- player_set_memory_buffer()
int player_set_video_roi_area | ( | player_h | player, |
double | x_scale, | ||
double | y_scale, | ||
double | w_scale, | ||
double | h_scale | ||
) |
Sets the ROI (Region Of Interest) area of the content video source.
This function is to set the ROI area of video content to render it on PLAYER_DISPLAY_TYPE_OVERLAY display with current display mode. It can be regarded as zooming operation because the selected video area will be rendered fit to the display.
- Since :
- 5.0
- Remarks:
- This function requires the ratio value of the each coordinate and size based on the video resolution size to consider the dynamic resolution video content.
- This function have to be called after calling player_set_display() and the ROI area is valid only in PLAYER_DISPLAY_TYPE_OVERLAY.
- Parameters:
-
[in] player The handle to the media player [in] x_scale X coordinate ratio value of the video source area based on the video width size
Valid range is 0.0~1.0.[in] y_scale Y coordinate ratio value of the video source area based on the video height size
Valid range is 0.0~1.0.[in] w_scale Width ratio value of the video source area based on the video width size
Valid range is from greater than 0.0 to 1.0.[in] h_scale Height ratio value of the video source area based on the video height size
Valid range is from greater than 0.0 to 1.0.
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_display_type_e
- player_set_display()
- player_set_display_mode()
- player_get_video_size()
- player_get_video_roi_area()
- Example
#include <player.h> ... player_h player = NULL; player_display_h display = NULL; int width = 0, height = 0; ... player_set_display (player, PLAYER_DISPLAY_TYPE_OVERLAY, display); player_get_video_size (player, &width, &height); ... player_set_video_roi_area (player, 30/(double)width, 30/(double)height, 480/(double)width, 270/(double)height); ...
int player_set_volume | ( | player_h | player, |
float | left, | ||
float | right | ||
) |
Sets the player's volume.
Setting this volume adjusts the player's instance volume, not the system volume. The valid range is from 0 to 1.0, inclusive (1.0 = 100%). Default value is 1.0. To change system volume, use the Sound Manager API. Finally, it does not support to set other value into each channel currently.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player [in] left The left volume scalar [in] right The right volume scalar
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_get_volume()
int player_start | ( | player_h | player | ) |
Starts or resumes playback.
Plays current media content, or resumes playback if the player is paused or buffering during HTTP streaming play.
- Since :
- 2.3
- Remarks:
- Even if you don't set visible to true by calling player_set_display_visible(), the video will be shown on PLAYER_STATE_PLAYING state.
-
Since 3.0:
Sound can be mixed with other sounds, if you don't control the stream focus in sound-manager module.
You can refer to Sound Manager. -
Since 5.0:
In case of HTTP streaming playback, the player could be internally paused for buffering. If the application calls this function during the buffering, the playback will be resumed by force and the buffering message posting by player_buffering_cb() will be stopped.
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_CONNECTION_FAILED Network connection failed PLAYER_ERROR_SOUND_POLICY Sound policy error
- Precondition:
- Before 5.0: The player state must be PLAYER_STATE_READY or PLAYER_STATE_PAUSED.
- Since 5.0: The player state must be one of PLAYER_STATE_READY, PLAYER_STATE_PLAYING, or PLAYER_STATE_PAUSED.
- Postcondition:
- The player state will be PLAYER_STATE_PLAYING.
- It invokes player_completed_cb() when playback completes, if you set a callback with player_set_completed_cb().
int player_stop | ( | player_h | player | ) |
Stops playing media content.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid state PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state PLAYER_ERROR_SOUND_POLICY Sound policy error
- Precondition:
- The player state must be set to PLAYER_STATE_PLAYING by calling player_start() or set to PLAYER_STATE_PAUSED by calling player_pause().
- Postcondition:
- The player state will be PLAYER_STATE_READY.
- See also:
- player_start()
- player_pause()
int player_unprepare | ( | player_h | player | ) |
Resets the media player.
The most recently used media is reset and no longer associated with the player. Playback is no longer possible. If you want to use the player again, you must set the data URI and call player_prepare() again.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation PLAYER_ERROR_INVALID_STATE Invalid player state
- Precondition:
- Before 5.0: The player state must be one of: PLAYER_STATE_READY, PLAYER_STATE_PLAYING, PLAYER_STATE_PAUSED.
- Since 5.0: The player state must be one of: PLAYER_STATE_IDLE, PLAYER_STATE_READY, PLAYER_STATE_PLAYING, PLAYER_STATE_PAUSED. PLAYER_STATE_IDLE is allowed only if player preparation was started with player_prepare_async().
- Postcondition:
- The player state will be PLAYER_STATE_IDLE.
- See also:
- player_prepare()
int player_unset_completed_cb | ( | player_h | player | ) |
Unsets the playback completed callback function.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_completed_cb()
int player_unset_error_cb | ( | player_h | player | ) |
Unsets the error callback function.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_error_cb()
int player_unset_interrupted_cb | ( | player_h | player | ) |
Unsets the interrupted callback function.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_OPERATION Invalid operation
- See also:
- player_set_interrupted_cb()
int player_unset_media_packet_audio_frame_decoded_cb | ( | player_h | player | ) |
Unsets the callback notifying the decoded audio data.
- Since :
- 5.5
- Remarks:
- Depending on the audio codec type or by enabling audio offload, this function could be unavailable and this will return PLAYER_ERROR_NOT_AVAILABLE.
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter PLAYER_ERROR_INVALID_STATE Invalid state PLAYER_ERROR_NOT_AVAILABLE Not available
- Precondition:
- The player's state must be PLAYER_STATE_READY or PLAYER_STATE_IDLE
int player_unset_media_packet_video_frame_decoded_cb | ( | player_h | player | ) |
Unsets the callback notifying the decoded video frame.
- Since :
- 2.3
- Parameters:
-
[in] player The handle to the media player
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- Precondition:
- The player's state must be PLAYER_STATE_READY or PLAYER_STATE_IDLE
int player_unset_media_stream_buffer_status_cb | ( | player_h | player, |
player_stream_type_e | type | ||
) |
Unsets the buffer status callback function.
- Since :
- 2.4
- Remarks:
- This function is used for media stream playback only.
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
int player_unset_media_stream_seek_cb | ( | player_h | player, |
player_stream_type_e | type | ||
) |
Unsets the seek callback function.
- Since :
- 2.4
- Parameters:
-
[in] player The handle to the media player [in] type The type of target stream
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
PLAYER_ERROR_NONE Successful PLAYER_ERROR_INVALID_PARAMETER Invalid parameter
- See also:
- player_set_media_stream_seek_cb()