Tizen Native API
6.5
|
The Camera API provides functions for camera preview, capture, and focusing.
Required Header
#include <camera.h>
Overview
The Camera API allows application developers to support using the camera. It includes functions that capture photos and support setting up notifications for state changes of capturing, previewing, focusing, information about resolution and binary data format, and functions for artistic picture manipulations like sepia, negative, sketch, and many more.
The Camera API allows creation of components required in taking pictures including:
- selecting proper exposure parameters
- selecting a proper output format
- displaying a photo preview
- triggering the capture phase
- controlling and displaying the camera state
- getting supported formats and picture resolutions
- getting a preview format and a resolution
The Camera API also notifies you (by callback mechanism) when a significant picture parameter changes e.g. focus.
State Diagram
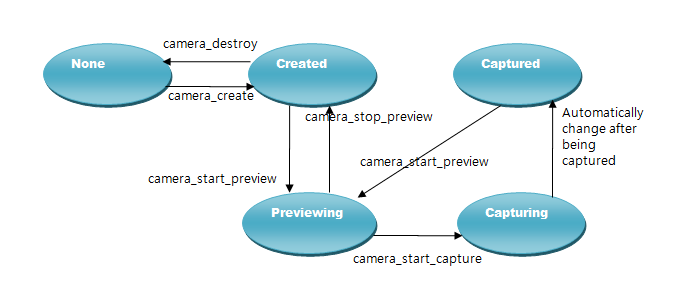
State Transitions
FUNCTION | PRE-STATE | POST-STATE | SYNC TYPE |
---|---|---|---|
camera_create() | NONE | CREATED | SYNC |
camera_destroy() | CREATED | NONE | SYNC |
camera_start_preview() | CREATED/CAPTURED | PREVIEW | SYNC |
camera_stop_preview() | PREVIEW | CREATED | SYNC |
camera_start_capture() | PREVIEW | CAPTURING -> CAPTURED* | SYNC |
(*) The transition from the CAPTURING state to the CAPTURED state will be processed automatically.
Callback(Event) Operations
The callback mechanism is used to notify the application about significant camera events.
REGISTER | UNREGISTER | CALLBACK | DESCRIPTION |
---|---|---|---|
camera_set_preview_cb() | camera_unset_preview_cb() | camera_preview_cb() | This callback is used to display the preview (it delivers copies of previous frames) |
camera_set_state_changed_cb() | camera_unset_state_changed_cb() | camera_state_changed_cb() | This callback is used to notify that the camera state has changed. Not executed in the recorder mode |
camera_set_focus_changed_cb() | camera_unset_focus_changed_cb() | camera_focus_changed_cb() | This callback is used to notify that the camera has changed focus (and it is ready to shoot) |
camera_set_error_cb() | camera_unset_error_cb() | camera_error_cb() | This callback is used to notify that an error has occurred |
Foreach Operations
FOREACH | CALLBACK | DESCRIPTION |
---|---|---|
camera_foreach_supported_preview_resolution() | camera_supported_preview_resolution_cb() | Supported preview resolutions |
camera_foreach_supported_capture_resolution() | camera_supported_capture_resolution_cb() | Supported camera capture resolutions |
camera_foreach_supported_capture_format() | camera_supported_capture_format_cb() | Supported camera capture formats |
camera_foreach_supported_preview_format() | camera_supported_preview_format_cb() | Supported camera preview format |
Related Features
This API is related with the following features:
- http://tizen.org/feature/camera
- http://tizen.org/feature/camera.back
- http://tizen.org/feature/camera.back.flash
- http://tizen.org/feature/camera.front
- http://tizen.org/feature/camera.front.flash
It is recommended to design feature related codes in your application for reliability.
You can check if a device supports the related features for this API by using System Information, thereby controlling the procedure of your application.
To ensure your application is only running on the device with specific features, please define the features in your manifest file using the manifest editor in the SDK.
More details on featuring your application can be found from Feature Element.
Functions | |
int | camera_create (camera_device_e device, camera_h *camera) |
Creates a new camera handle for controlling a camera. | |
int | camera_change_device (camera_h camera, camera_device_e device) |
Changes the camera device. | |
int | camera_destroy (camera_h camera) |
Destroys the camera handle and releases all its resources. | |
int | camera_start_preview (camera_h camera) |
Starts capturing and drawing preview frames on the screen. | |
int | camera_stop_preview (camera_h camera) |
Stops capturing and drawing preview frames. | |
int | camera_start_capture (camera_h camera, camera_capturing_cb capturing_cb, camera_capture_completed_cb completed_cb, void *user_data) |
Starts capturing of still images. | |
int | camera_start_continuous_capture (camera_h camera, int count, int interval, camera_capturing_cb capturing_cb, camera_capture_completed_cb completed_cb, void *user_data) |
Starts continuously capturing still images. | |
int | camera_stop_continuous_capture (camera_h camera) |
Aborts continuous capturing. | |
int | camera_get_state (camera_h camera, camera_state_e *state) |
Gets the state of the camera. | |
int | camera_start_focusing (camera_h camera, bool continuous) |
Starts camera auto-focusing, asynchronously. | |
int | camera_cancel_focusing (camera_h camera) |
Stops camera auto focusing. | |
int | camera_set_display (camera_h camera, camera_display_type_e type, camera_display_h display) |
Sets the display handle to show preview images. | |
int | camera_set_preview_resolution (camera_h camera, int width, int height) |
Sets the resolution of the preview. | |
int | camera_get_preview_resolution (camera_h camera, int *width, int *height) |
Gets the resolution of the preview. | |
int | camera_get_recommended_preview_resolution (camera_h camera, int *width, int *height) |
Gets the recommended preview resolution. | |
int | camera_start_face_detection (camera_h camera, camera_face_detected_cb callback, void *user_data) |
Starts face detection. | |
int | camera_stop_face_detection (camera_h camera) |
Stops face detection. | |
int | camera_set_display_reuse_hint (camera_h camera, bool hint) |
Sets the hint for display reuse. | |
int | camera_get_display_reuse_hint (camera_h camera, bool *hint) |
Gets the hint for display reuse. | |
int | camera_set_capture_resolution (camera_h camera, int width, int height) |
Sets the resolution of the captured image. | |
int | camera_get_capture_resolution (camera_h camera, int *width, int *height) |
Gets the resolution of the captured image. | |
int | camera_set_capture_format (camera_h camera, camera_pixel_format_e format) |
Sets the format of an image to be captured. | |
int | camera_get_capture_format (camera_h camera, camera_pixel_format_e *format) |
Gets the format of the image to be captured. | |
int | camera_set_preview_format (camera_h camera, camera_pixel_format_e format) |
Sets the preview data format. | |
int | camera_get_preview_format (camera_h camera, camera_pixel_format_e *format) |
Gets the format of the preview stream. | |
int | camera_get_facing_direction (camera_h camera, camera_facing_direction_e *facing_direction) |
Gets the facing direction of camera module. | |
int | camera_get_flash_state (camera_device_e device, camera_flash_state_e *state) |
Gets the camera's flash state. | |
int | camera_set_preview_cb (camera_h camera, camera_preview_cb callback, void *user_data) |
Registers a callback function to be called once per frame when previewing. | |
int | camera_unset_preview_cb (camera_h camera) |
Unregisters the callback function. | |
int | camera_set_media_packet_preview_cb (camera_h camera, camera_media_packet_preview_cb callback, void *user_data) |
Registers a media packet callback function to be called once per frame when previewing. | |
int | camera_unset_media_packet_preview_cb (camera_h camera) |
Unregisters the media packet callback function. | |
int | camera_set_state_changed_cb (camera_h camera, camera_state_changed_cb callback, void *user_data) |
Registers a callback function to be called when the camera state changes. | |
int | camera_unset_state_changed_cb (camera_h camera) |
Unregisters the callback function. | |
int | camera_set_interrupted_cb (camera_h camera, camera_interrupted_cb callback, void *user_data) |
Registers a callback function to be called when the camera is interrupted by policy. | |
int | camera_unset_interrupted_cb (camera_h camera) |
Unregisters the callback function. | |
int | camera_set_interrupt_started_cb (camera_h camera, camera_interrupt_started_cb callback, void *user_data) |
Registers a callback function to be called when the camera interrupt is started by policy. | |
int | camera_unset_interrupt_started_cb (camera_h camera) |
Unregisters the callback function. | |
int | camera_set_focus_changed_cb (camera_h camera, camera_focus_changed_cb callback, void *user_data) |
Registers a callback function to be called when the auto-focus state changes. | |
int | camera_unset_focus_changed_cb (camera_h camera) |
Unregisters the callback function. | |
int | camera_set_error_cb (camera_h camera, camera_error_cb callback, void *user_data) |
Registers a callback function to be called when an asynchronous operation error occurs. | |
int | camera_unset_error_cb (camera_h camera) |
Unregisters the callback function. | |
int | camera_get_device_state (camera_device_e device, camera_device_state_e *state) |
Gets the state of camera device. | |
int | camera_add_device_state_changed_cb (camera_device_state_changed_cb callback, void *user_data, int *cb_id) |
Registers a callback function to be called when the camera device state changes. | |
int | camera_remove_device_state_changed_cb (int cb_id) |
Unregisters the callback function. | |
Typedefs | |
typedef struct camera_cli_s * | camera_h |
The Camera handle. | |
typedef void * | camera_display_h |
The Camera display handle. | |
typedef void(* | camera_state_changed_cb )(camera_state_e previous, camera_state_e current, bool by_policy, void *user_data) |
Called when the camera state is changed. | |
typedef void(* | camera_device_state_changed_cb )(camera_device_e device, camera_device_state_e state, void *user_data) |
Called when the camera device state is changed. | |
typedef void(* | camera_interrupted_cb )(camera_policy_e policy, camera_state_e previous, camera_state_e current, void *user_data) |
Called when the camera is interrupted by policy. | |
typedef void(* | camera_interrupt_started_cb )(camera_policy_e policy, camera_state_e state, void *user_data) |
Called when the camera interrupt is started by policy. | |
typedef void(* | camera_focus_changed_cb )(camera_focus_state_e state, void *user_data) |
Called when the camera focus state is changed. | |
typedef void(* | camera_preview_cb )(camera_preview_data_s *frame, void *user_data) |
Called to register for notifications about delivering a copy of the new preview frame when every preview frame is displayed. | |
typedef void(* | camera_media_packet_preview_cb )(media_packet_h pkt, void *user_data) |
Called to register for notifications about delivering media packet when every preview frame is displayed. | |
typedef void(* | camera_capturing_cb )(camera_image_data_s *image, camera_image_data_s *postview, camera_image_data_s *thumbnail, void *user_data) |
Called to get information about image data taken by the camera once per frame while capturing. | |
typedef void(* | camera_capture_completed_cb )(void *user_data) |
Called when the camera capturing completes. | |
typedef void(* | camera_error_cb )(camera_error_e error, camera_state_e current_state, void *user_data) |
Called when an error occurs. | |
typedef void(* | camera_face_detected_cb )(camera_detected_face_s *faces, int count, void *user_data) |
Called when a face is detected in the preview frame. | |
Defines | |
#define | GET_DISPLAY(x) (void*)(x) |
Gets a display handle. |
Define Documentation
#define GET_DISPLAY | ( | x | ) | (void*)(x) |
Gets a display handle.
- Since :
- 2.3
Typedef Documentation
typedef void(* camera_capture_completed_cb)(void *user_data) |
Called when the camera capturing completes.
- Since :
- 2.3
- Remarks:
- The callback is called after camera_capturing_cb() is completed.
If you want to show the user a preview after capturing is finished,
an application can use camera_start_preview() after calling this callback.
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- Precondition:
- This callback function is invoked if it is registered using camera_start_capture() or camera_start_continuous_capture().
typedef void(* camera_capturing_cb)(camera_image_data_s *image, camera_image_data_s *postview, camera_image_data_s *thumbnail, void *user_data) |
Called to get information about image data taken by the camera once per frame while capturing.
- Since :
- 2.3
- Remarks:
- This function is issued in the context of internal framework so the UI update code should not be directly invoked. You must not call camera_start_preview() within this callback.
- Parameters:
-
[in] image The image data of the captured picture [in] postview The image data of the postview [in] thumbnail The image data of the thumbnail (it should be NULL
if the available thumbnail data does not exist)[in] user_data The user data passed from the callback registration function
- Precondition:
- camera_start_capture() or camera_start_continuous_capture() will invoke this callback function if it is registered using camera_start_capture() or camera_start_continuous_capture().
typedef void(* camera_device_state_changed_cb)(camera_device_e device, camera_device_state_e state, void *user_data) |
Called when the camera device state is changed.
- Since :
- 3.0
- Parameters:
-
[in] device The hardware camera type [in] state The state of the camera device [in] user_data The user data passed from the callback registration function
- See also:
- camera_add_device_state_changed_cb()
typedef void* camera_display_h |
The Camera display handle.
- Since :
- 2.3
typedef void(* camera_error_cb)(camera_error_e error, camera_state_e current_state, void *user_data) |
Called when an error occurs.
- Since :
- 2.3
- Remarks:
- This callback informs about a critical error situation.
When this callback is invoked, the user should release the resource and terminate the application.
In case of errors, one of these codes occur:
CAMERA_ERROR_DEVICE,
CAMERA_ERROR_INVALID_OPERATION,
CAMERA_ERROR_OUT_OF_MEMORY.
- Parameters:
-
[in] error The error code [in] current_state The current state of the camera [in] user_data The user data passed from the callback registration function
- Precondition:
- This callback function is invoked if it is registered using camera_set_error_cb().
typedef void(* camera_face_detected_cb)(camera_detected_face_s *faces, int count, void *user_data) |
Called when a face is detected in the preview frame.
- Since :
- 2.3
- Parameters:
-
[in] faces The detected face array [in] count The length of the array [in] user_data The user data passed from the callback registration function
- See also:
- camera_start_face_detection()
typedef void(* camera_focus_changed_cb)(camera_focus_state_e state, void *user_data) |
Called when the camera focus state is changed.
When the camera auto focus completes or a change to the focus state occurs, this callback is invoked.
Changes of focus state are as follows:
CAMERA_FOCUS_STATE_RELEASED -> start focusing -> CAMERA_FOCUS_STATE_ONGOING -> working -> CAMERA_FOCUS_STATE_FOCUSED or CAMERA_FOCUS_STATE_FAILED.
- Since :
- 2.3
- Parameters:
-
[in] state The current state of the auto-focus [in] user_data The user data passed from the callback registration function
- Precondition:
- camera_start_focusing() will invoke this callback if you register it using camera_set_focus_changed_cb().
typedef struct camera_cli_s* camera_h |
typedef void(* camera_interrupt_started_cb)(camera_policy_e policy, camera_state_e state, void *user_data) |
Called when the camera interrupt is started by policy.
- Since :
- 4.0
- Remarks:
- This callback is called before interrupt handling is started.
- Parameters:
-
[in] policy The policy that is interrupting the camera [in] state The current state of the camera [in] user_data The user data passed from the callback registration function
- See also:
- camera_set_interrupt_started_cb()
typedef void(* camera_interrupted_cb)(camera_policy_e policy, camera_state_e previous, camera_state_e current, void *user_data) |
Called when the camera is interrupted by policy.
- Since :
- 2.3
- Remarks:
- This callback is called after interrupt handling is completed.
- Parameters:
-
[in] policy The policy that interrupted the camera [in] previous The previous state of the camera [in] current The current state of the camera [in] user_data The user data passed from the callback registration function
- See also:
- camera_set_interrupted_cb()
typedef void(* camera_media_packet_preview_cb)(media_packet_h pkt, void *user_data) |
Called to register for notifications about delivering media packet when every preview frame is displayed.
- Since :
- 2.3
- Remarks:
- This function is issued in the context of internal framework so the UI update code should not be directly invoked.
If the camera is used as a recorder then this callback function won't be called.
and the packet should be released by media_packet_destroy() after use.
- Parameters:
-
[in] pkt Reference pointer to media packet [in] user_data The user data passed from the callback registration function
- Precondition:
- camera_start_preview() will invoke this callback function if you register this callback using camera_set_media_packet_preview_cb().
typedef void(* camera_preview_cb)(camera_preview_data_s *frame, void *user_data) |
Called to register for notifications about delivering a copy of the new preview frame when every preview frame is displayed.
- Since :
- 2.3
- Remarks:
- This function is issued in the context of internal framework so the UI update code should not be directly invoked.
If the camera is used as a recorder then this callback function won't be called. - The frame should not be released and it's available until the callback returns.
- Parameters:
-
[in] frame The reference pointer to preview stream data [in] user_data The user data passed from the callback registration function
- Precondition:
- camera_start_preview() will invoke this callback function if you register this callback using camera_set_preview_cb().
typedef void(* camera_state_changed_cb)(camera_state_e previous, camera_state_e current, bool by_policy, void *user_data) |
Called when the camera state is changed.
- Since :
- 2.3
- Parameters:
-
[in] previous The previous state of the camera [in] current The current state of the camera [in] by_policy If true
the state is changed by policy, otherwisefalse
[in] user_data The user data passed from the callback registration function
- Precondition:
- camera_start_preview(), camera_start_capture() or camera_stop_preview() will invoke this callback if you register this callback using camera_set_state_changed_cb().
- See also:
- camera_set_state_changed_cb()
Enumeration Type Documentation
enum camera_device_e |
Enumeration for the camera device.
- Since :
- 2.3
- Enumerator:
enum camera_error_e |
Enumeration for the error codes of Camera.
- Since :
- 2.3
- Enumerator:
enum camera_flash_state_e |
enum camera_flip_e |
enum camera_focus_state_e |
Enumeration for the camera pixel format.
- Since :
- 2.3
- Remarks:
- If CAMERA_PIXEL_FORMAT_INVZ is set, the type of display should be CAMERA_DISPLAY_TYPE_NONE.
Otherwise, camera_start_preview() will return CAMERA_ERROR_INVALID_OPERATION. (Since 5.0)
- Enumerator:
enum camera_policy_e |
enum camera_rotation_e |
enum camera_state_e |
Function Documentation
int camera_add_device_state_changed_cb | ( | camera_device_state_changed_cb | callback, |
void * | user_data, | ||
int * | cb_id | ||
) |
Registers a callback function to be called when the camera device state changes.
- Since :
- 3.0
- Parameters:
-
[in] callback The callback function to register [in] user_data The user data to be passed to the callback function [out] cb_id The id of registered callback
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_OUT_OF_MEMORY Out of memory CAMERA_ERROR_NOT_SUPPORTED The feature is not supported
- Postcondition:
- This function will invoke camera_device_state_changed_cb() when the camera device's state changes.
int camera_cancel_focusing | ( | camera_h | camera | ) |
Stops camera auto focusing.
- Since :
- 2.3
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/camera
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_PREVIEW.
int camera_change_device | ( | camera_h | camera, |
camera_device_e | device | ||
) |
Changes the camera device.
- Since :
- 3.0
- Remarks:
- This function can be used to change camera device simply without camera_destroy() and camera_create().
If display reuse hint is set by camera_set_display_reuse_hint() before stopping the preview,
display handle will be reused and last frame on display can be kept even though camera device is changed.
- Parameters:
-
[in] camera The handle to the camera [in] device The hardware camera to access
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_OUT_OF_MEMORY Out of memory CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_CREATED.
- Postcondition:
- If it succeeds, the camera attributes and settings will be reset.
int camera_create | ( | camera_device_e | device, |
camera_h * | camera | ||
) |
Creates a new camera handle for controlling a camera.
- Since :
- 2.3
- Remarks:
- Multiple handles on a context at the same time are allowed to be created. However, camera cannot guarantee proper operation because of limited resources, such as camera device, audio device, and display device.
. A camera must be released using camera_destroy(). -
The privilege http://tizen.org/privilege/camera is not required since 4.0,
but it is required in all earlier versions.
- Parameters:
-
[in] device The hardware camera to access [out] camera A newly returned handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_OUT_OF_MEMORY Out of memory CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported
- Postcondition:
- If it succeeds, the camera state will be CAMERA_STATE_CREATED.
- See also:
- camera_destroy()
int camera_destroy | ( | camera_h | camera | ) |
Destroys the camera handle and releases all its resources.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported
- See also:
- camera_create()
int camera_get_capture_format | ( | camera_h | camera, |
camera_pixel_format_e * | format | ||
) |
Gets the format of the image to be captured.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera [out] format The format of the image to be captured
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int camera_get_capture_resolution | ( | camera_h | camera, |
int * | width, | ||
int * | height | ||
) |
Gets the resolution of the captured image.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera [out] width The capture width [out] height The capture height
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int camera_get_device_state | ( | camera_device_e | device, |
camera_device_state_e * | state | ||
) |
Gets the state of camera device.
- Since :
- 3.0
- Parameters:
-
[in] device The hardware camera type [out] state The current state of the device
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_NOT_SUPPORTED The feature is not supported
int camera_get_display_reuse_hint | ( | camera_h | camera, |
bool * | hint | ||
) |
Gets the hint for display reuse.
- Since :
- 3.0
- Remarks:
- If the current display type is CAMERA_DISPLAY_TYPE_NONE, this function will return CAMERA_ERROR_INVALID_OPERATION.
- Parameters:
-
[in] camera The handle to the camera [out] hint The hint for display reuse; true - reuse the display, false - do not reuse
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_OPERATION Internal error CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int camera_get_facing_direction | ( | camera_h | camera, |
camera_facing_direction_e * | facing_direction | ||
) |
Gets the facing direction of camera module.
- Since :
- 3.0
- Parameters:
-
[in] camera The handle to the camera [out] facing_direction The facing direction of camera module
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_OPERATION Internal error CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int camera_get_flash_state | ( | camera_device_e | device, |
camera_flash_state_e * | state | ||
) |
Gets the camera's flash state.
- Since :
- 3.0
- Parameters:
-
[in] device The hardware camera to access [out] state The current flash state
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_OPERATION Internal error CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_NOT_SUPPORTED The feature is not supported
int camera_get_preview_format | ( | camera_h | camera, |
camera_pixel_format_e * | format | ||
) |
Gets the format of the preview stream.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera [out] format The preview data format
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int camera_get_preview_resolution | ( | camera_h | camera, |
int * | width, | ||
int * | height | ||
) |
Gets the resolution of the preview.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera [out] width The preview width [out] height The preview height
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int camera_get_recommended_preview_resolution | ( | camera_h | camera, |
int * | width, | ||
int * | height | ||
) |
Gets the recommended preview resolution.
- Since :
- 2.3
- Remarks:
- Depending on the capture resolution aspect ratio and display resolution, the recommended preview resolution is determined.
- Parameters:
-
[in] camera The handle to the camera [out] width The preview width [out] height The preview height
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int camera_get_state | ( | camera_h | camera, |
camera_state_e * | state | ||
) |
Gets the state of the camera.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera [out] state The current state of the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int camera_remove_device_state_changed_cb | ( | int | cb_id | ) |
Unregisters the callback function.
- Since :
- 3.0
- Parameters:
-
[in] cb_id The id of registered callback
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_NOT_SUPPORTED The feature is not supported
- See also:
- camera_add_device_state_changed_cb()
int camera_set_capture_format | ( | camera_h | camera, |
camera_pixel_format_e | format | ||
) |
Sets the format of an image to be captured.
- Since :
- 2.3
- Remarks:
- This function should be called before capturing (see camera_start_capture()).
- Parameters:
-
[in] camera The handle to the camera [out] format The format of the image to be captured
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_CREATED or CAMERA_STATE_PREVIEW.
int camera_set_capture_resolution | ( | camera_h | camera, |
int | width, | ||
int | height | ||
) |
Sets the resolution of the captured image.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera [in] width The capture width [in] height The capture height
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_CREATED or CAMERA_STATE_PREVIEW.
int camera_set_display | ( | camera_h | camera, |
camera_display_type_e | type, | ||
camera_display_h | display | ||
) |
Sets the display handle to show preview images.
- Since :
- 2.3
- Remarks:
- This function must be called before previewing (see camera_start_preview()). In Custom ROI display mode, camera_attr_set_display_roi_area() function must be called before calling this function.
- This function must be called in main thread of the application. Otherwise, it will return CAMERA_ERROR_INVALID_OPERATION by internal restriction. To avoid CAMERA_ERROR_INVALID_OPERATION in sub thread, ecore_thread_main_loop_begin() and ecore_thread_main_loop_end() can be used, but deadlock can occur if the main thread is busy. So, it's not recommended to use them. (Since 5.0)
- Parameters:
-
[in] camera The handle to the camera [in] type The display type [in] display The display handle from GET_DISPLAY
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_CREATED.
int camera_set_display_reuse_hint | ( | camera_h | camera, |
bool | hint | ||
) |
Sets the hint for display reuse.
If the hint is set to true, the display will be reused when the camera device is changed with camera_change_device().
- Since :
- 3.0
- Remarks:
- If the current display type is CAMERA_DISPLAY_TYPE_NONE, this function will return CAMERA_ERROR_INVALID_OPERATION.
- Parameters:
-
[in] camera The handle to the camera [in] hint The hint for display reuse; true - reuse the display, false - do not reuse
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_INVALID_OPERATION Internal error CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_PREVIEW.
int camera_set_error_cb | ( | camera_h | camera, |
camera_error_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when an asynchronous operation error occurs.
- Since :
- 2.3
- Remarks:
- This callback informs about a critical error situation.
When this callback is invoked, the user should release the resource and terminate the application.
In case of errors, one of the following codes will occur:
CAMERA_ERROR_DEVICE,
CAMERA_ERROR_INVALID_OPERATION,
CAMERA_ERROR_OUT_OF_MEMORY.
- Parameters:
-
[in] camera The handle to the camera [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Postcondition:
- This function will invoke camera_error_cb() when an asynchronous operation error occurs.
- See also:
- camera_unset_error_cb()
- camera_error_cb()
int camera_set_focus_changed_cb | ( | camera_h | camera, |
camera_focus_changed_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when the auto-focus state changes.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Postcondition:
- This function will invoke camera_focus_changed_cb() when the auto-focus state changes.
int camera_set_interrupt_started_cb | ( | camera_h | camera, |
camera_interrupt_started_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when the camera interrupt is started by policy.
- Since :
- 4.0
- Parameters:
-
[in] camera The handle to the camera [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter
int camera_set_interrupted_cb | ( | camera_h | camera, |
camera_interrupted_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when the camera is interrupted by policy.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
int camera_set_media_packet_preview_cb | ( | camera_h | camera, |
camera_media_packet_preview_cb | callback, | ||
void * | user_data | ||
) |
Registers a media packet callback function to be called once per frame when previewing.
- Since :
- 2.3
- Remarks:
- This callback does not work in video recorder mode.
This function should be called before previewing (see camera_start_preview())
A registered callback is called on the internal thread of the camera.
A video frame can be retrieved using a registered callback as a media packet.
The callback function holds the same buffer that will be drawn on the display device.
So if you change the media packet in a registered callback, it will be displayed on the device
and the media packet is available until it's destroyed by media_packet_destroy().
- Parameters:
-
[in] camera The handle to the camera [in] callback The callback function to be registered [in] user_data The user data to be passed to the callback function
- Returns:
- 0 on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera's state should be CAMERA_STATE_CREATED.
int camera_set_preview_cb | ( | camera_h | camera, |
camera_preview_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called once per frame when previewing.
- Since :
- 2.3
- Remarks:
- This callback does not work in the video recorder mode.
Before 4.0, the only allowed state for calling this function was CAMERA_STATE_CREATED.
Since 4.0, CAMERA_STATE_PREVIEW has been added as an allowed state,
so that this function could be called before previewing or even while previewing.
A registered callback is called on the internal thread of the camera.
A video frame can be retrieved using a registered callback,
and the buffer is only available in a registered callback.
Since tizen 3.0, if you change the buffer in a registered callback,
it could not be displayed on the device in case of copied buffer.
and if camera_is_supported_media_packet_preview_cb() returns false,
it's copied buffer case.
- Parameters:
-
[in] camera The handle to the camera [in] callback The callback function to be registered [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- Before 4.0 : The camera state must be set to CAMERA_STATE_CREATED.
Since 4.0 : The camera state must be set to CAMERA_STATE_CREATED or CAMERA_STATE_PREVIEW.
int camera_set_preview_format | ( | camera_h | camera, |
camera_pixel_format_e | format | ||
) |
Sets the preview data format.
- Since :
- 2.3
- Remarks:
- This function should be called before previewing (see camera_start_preview()).
- Parameters:
-
[in] camera The handle to the camera [in] format The preview data format
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_CREATED.
int camera_set_preview_resolution | ( | camera_h | camera, |
int | width, | ||
int | height | ||
) |
Sets the resolution of the preview.
- Since :
- 2.3
- Remarks:
- This function should be called before previewing (camera_start_preview()).
- Parameters:
-
[in] camera The handle to the camera [in] width The preview width [in] height The preview height
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_CREATED or CAMERA_STATE_PREVIEW.
int camera_set_state_changed_cb | ( | camera_h | camera, |
camera_state_changed_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when the camera state changes.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Postcondition:
- This function will invoke camera_state_changed_cb() when the camera state changes.
int camera_start_capture | ( | camera_h | camera, |
camera_capturing_cb | capturing_cb, | ||
camera_capture_completed_cb | completed_cb, | ||
void * | user_data | ||
) |
Starts capturing of still images.
- Since :
- 2.3
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/camera
- Remarks:
- This function causes the transition of the camera state from CAMERA_STATE_CAPTURING to CAMERA_STATE_CAPTURED automatically
and the corresponding callback function camera_capturing_cb() and camera_capture_completed_cb() will be invoked
The captured image will be delivered through camera_capturing_cb().
camera_capture_completed_cb() callback notifies about completion of camera_capturing_cb().
The camera's preview should be restarted by calling camera_start_preview().
- Parameters:
-
[in] camera The handle to the camera [in] capturing_cb The callback for capturing data [in] completed_cb The callback for notification of completion [in] user_data The user data
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_PREVIEW.
If needed, modify capture resolution(camera_set_capture_resolution()), capture format(camera_set_capture_format()), or image quality(camera_attr_set_image_quality()).
- Postcondition:
- If it succeeds the camera state will be CAMERA_STATE_CAPTURED.
- See also:
- camera_start_preview()
- camera_start_continuous_capture();
- camera_foreach_supported_capture_resolution()
- camera_set_capture_resolution()
- camera_get_capture_resolution()
- camera_foreach_supported_capture_format()
- camera_set_capture_format()
- camera_get_capture_format()
- camera_attr_set_image_quality()
- camera_attr_get_image_quality()
int camera_start_continuous_capture | ( | camera_h | camera, |
int | count, | ||
int | interval, | ||
camera_capturing_cb | capturing_cb, | ||
camera_capture_completed_cb | completed_cb, | ||
void * | user_data | ||
) |
Starts continuously capturing still images.
- Since :
- 2.3
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/camera
- Remarks:
- If this is not supported zero shutter lag occurs. The capture resolution could be changed to the preview resolution.
This function causes the transition of the camera state from CAMERA_STATE_CAPTURING to CAMERA_STATE_CAPTURED automatically
and the corresponding callback function camera_capturing_cb() and camera_capture_completed_cb() will be invoked
Each Captured image will be delivered through camera_capturing_cb().
The camera_capture_completed_cb() callback notifies about the completion of an entire capture.
The camera's preview should be restarted by calling camera_start_preview().
.
- Parameters:
-
[in] camera The handle to the camera [in] count The number of still images [in] interval The interval of the capture (millisecond) [in] capturing_cb The callback for capturing data [in] completed_cb The callback for notification of completion [in] user_data The user data
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Postcondition:
- If it succeeds the camera state will be CAMERA_STATE_CAPTURED.
int camera_start_face_detection | ( | camera_h | camera, |
camera_face_detected_cb | callback, | ||
void * | user_data | ||
) |
Starts face detection.
- Since :
- 2.3
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/camera
- Remarks:
- This should be called after the preview is started.
This callback will be invoked when the face is detected in the preview frame.
Internally it starts continuous focus and focusing on the detected face.
When face detection is running, the camera_start_focusing(), camera_cancel_focusing(), camera_attr_set_af_mode(), camera_attr_set_af_area(), camera_attr_set_exposure_mode(), and camera_attr_set_whitebalance() settings are ignored.
If camera_stop_preview() is invoked, face detection is stopped and then preview is resumed using camera_start_preview(), this method should be called again to resume face detection.
- Parameters:
-
[in] camera The handle to the camera [in] callback The callback to notify face detection [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Not preview state CAMERA_ERROR_INVALID_OPERATION Not supported this feature CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be CAMERA_STATE_PREVIEW.
int camera_start_focusing | ( | camera_h | camera, |
bool | continuous | ||
) |
Starts camera auto-focusing, asynchronously.
- Since :
- 2.3
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/camera
- Remarks:
- If continuous status is
true
, the camera continuously tries to focus.
- Parameters:
-
[in] camera The handle to the camera [in] continuous The status of continuous focusing
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_PREVIEW.
- Postcondition:
- The camera focus state will be CAMERA_FOCUS_STATE_ONGOING.
int camera_start_preview | ( | camera_h | camera | ) |
Starts capturing and drawing preview frames on the screen.
- Since :
- 2.3
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/camera
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_RESOURCE_CONFLICT Resource conflict error CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_DEVICE_BUSY The device is being used in another application or is performing other operations CAMERA_ERROR_DEVICE_NOT_FOUND No camera device CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_CREATED or CAMERA_STATE_CAPTURED.
You must set the display handle.
If needed, modify preview FPS(camera_attr_set_preview_fps()), preview resolution(camera_set_preview_resolution()), or preview format(camera_set_preview_format()).
- Postcondition:
- If it succeeds, the camera state will be CAMERA_STATE_PREVIEW.
camera_preview_cb() will be called when preview image data becomes available.
- See also:
- camera_stop_preview()
- camera_set_display()
- camera_set_preview_cb()
- camera_set_media_packet_preview_cb()
- camera_foreach_supported_preview_resolution()
- camera_set_preview_resolution()
- camera_get_preview_resolution()
- camera_foreach_supported_preview_format()
- camera_set_preview_format()
- camera_get_preview_format()
- camera_attr_foreach_supported_fps()
- camera_attr_set_preview_fps()
- camera_attr_get_preview_fps()
int camera_stop_continuous_capture | ( | camera_h | camera | ) |
Aborts continuous capturing.
- Since :
- 2.3
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/camera
- Remarks:
- The camera state will be changed to CAMERA_STATE_CAPTURED.
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_PREVIEW.
- See also:
- camera_start_continuous_capture()
int camera_stop_face_detection | ( | camera_h | camera | ) |
Stops face detection.
- Since :
- 2.3
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/camera
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- This should be called after face detection is started.
int camera_stop_preview | ( | camera_h | camera | ) |
Stops capturing and drawing preview frames.
- Since :
- 2.3
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/camera
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_INVALID_STATE Invalid state CAMERA_ERROR_INVALID_OPERATION Invalid operation CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- Precondition:
- The camera state must be set to CAMERA_STATE_PREVIEW.
- Postcondition:
- The camera state will be CAMERA_STATE_CREATED.
int camera_unset_error_cb | ( | camera_h | camera | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- See also:
- camera_set_error_cb()
int camera_unset_focus_changed_cb | ( | camera_h | camera | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- See also:
- camera_set_focus_changed_cb()
int camera_unset_interrupt_started_cb | ( | camera_h | camera | ) |
Unregisters the callback function.
- Since :
- 4.0
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter
- See also:
- camera_set_interrupt_started_cb()
int camera_unset_interrupted_cb | ( | camera_h | camera | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- See also:
- camera_set_interrupted_cb()
int camera_unset_media_packet_preview_cb | ( | camera_h | camera | ) |
Unregisters the media packet callback function.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- See also:
- camera_set_media_packet_preview_cb()
int camera_unset_preview_cb | ( | camera_h | camera | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- See also:
- camera_set_preview_cb()
int camera_unset_state_changed_cb | ( | camera_h | camera | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] camera The handle to the camera
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
CAMERA_ERROR_NONE Successful CAMERA_ERROR_INVALID_PARAMETER Invalid parameter CAMERA_ERROR_PERMISSION_DENIED The access to the resources can not be granted CAMERA_ERROR_NOT_SUPPORTED The feature is not supported CAMERA_ERROR_SERVICE_DISCONNECTED The socket to multimedia server is disconnected
- See also:
- camera_set_state_changed_cb()