Tizen Native API
6.5
|
The Frame Component API provides functions for handling Frame Component state changes or system events, and get information about the Frame Component.
Required Header
#include <frame_component.h>
Overview
The Frame Component API provides functions for handling Frame Component of Component Based Application state changes or system events.
Registering Callbacks for Frame Component State Change Events
The frame component state change events include the following:
State | Description |
---|---|
frame_component_create_cb() | The callback function called after the frame component instance is created. |
frame_component_start_cb() | The callback function called when the frame component instance is started. |
frame_component_resume_cb() | The callback function called when the frame component becomes visible. |
frame_component_pause_cb() | The callback function called when the frame component becomes invisible. |
frame_component_stop_cb() | The callback function called before the frame component instance is stopped. |
frame_component_destroy_cb() | The callback function called before the frame component instance is destroyed. |
frame_component_restore_content_cb() | The callback function called when the content information of the frame component instance is restored. |
frame_component_save_content_cb() | The callback function called before the content information of the frame component instance is saved. |
frame_component_action_cb() | The callback function called when another application sends a launch request to the component. |
frame_component_device_orientation_changed_cb() | The callback function called when the device orientation is changed. |
frame_component_language_changed_cb() | The callback function called when the system language is changed. |
frame_component_region_format_changed_cb() | The callback function called when the system region format is changed. |
frame_component_low_battery_cb() | The callback function called when the battery status is changed. |
frame_component_low_memory_cb() | The callback function called when the memory status is changed. |
frame_component_suspended_state_changed_cb() | The callback function called when the suspended state of the frame component is changed. |
Please refer to the following state diagram to see the possible transitions and callbacks that are called while transition.
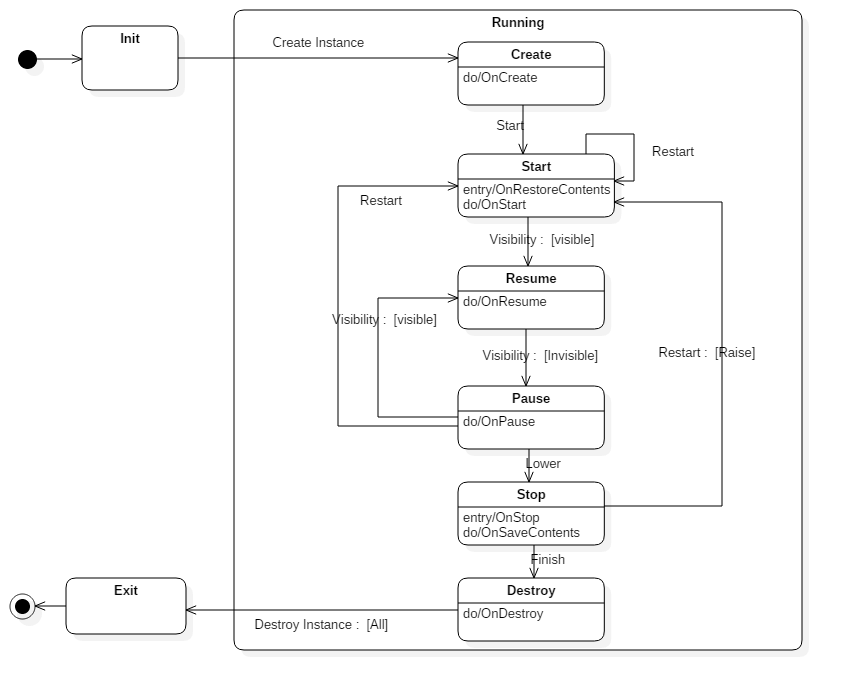
Functions | |
int | frame_component_get_display_status (component_h context, component_display_status_e *display_status) |
Gets the display status. | |
int | frame_component_get_window (component_h context, Evas_Object **window) |
Gets an Evas object for the frame component. | |
Typedefs | |
typedef Evas_Object *(* | frame_component_create_cb )(component_h context, void *user_data) |
Called when the frame component instance is created. | |
typedef void(* | frame_component_start_cb )(component_h context, app_control_h app_control, bool restarted, void *user_data) |
Called when the frame component instance is started. | |
typedef void(* | frame_component_resume_cb )(component_h context, void *user_data) |
Called when the frame component becomes visible. | |
typedef void(* | frame_component_pause_cb )(component_h context, void *user_data) |
Called when the frame component becomes invisible. | |
typedef void(* | frame_component_stop_cb )(component_h context, void *user_data) |
Called before the frame component instance is stopped. | |
typedef void(* | frame_component_destroy_cb )(component_h context, void *user_data) |
Called before the frame component instance is destroyed. | |
typedef void(* | frame_component_restore_content_cb )(component_h context, bundle *content, void *user_data) |
Called after the content information of the frame component instance is restored. | |
typedef void(* | frame_component_save_content_cb )(component_h context, bundle *content, void *user_data) |
Called before the content information of the frame component instance is saved. | |
typedef void(* | frame_component_action_cb )(component_h context, const char *action, app_control_h app_control, void *user_data) |
Called when another application sends a launch request to the component. | |
typedef void(* | frame_component_device_orientation_changed_cb )(component_h context, component_device_orientation_e orientation, void *user_data) |
Called when the device orientation is changed. | |
typedef void(* | frame_component_language_changed_cb )(component_h context, const char *language, void *user_data) |
Called when the system language is changed. | |
typedef void(* | frame_component_region_format_changed_cb )(component_h context, const char *region, void *user_data) |
Called when the system region format is changed. | |
typedef void(* | frame_component_low_battery_cb )(component_h context, component_low_battery_status_e status, void *user_data) |
Called when the battery status is changed. | |
typedef void(* | frame_component_low_memory_cb )(component_h context, component_low_memory_status_e status, void *user_data) |
Called when the memory status is changed. | |
typedef void(* | frame_component_suspended_state_changed_cb )(component_h context, component_suspended_state_e state, void *user_data) |
Called when the suspended state of the frame component is changed. |
Typedef Documentation
typedef void(* frame_component_action_cb)(component_h context, const char *action, app_control_h app_control, void *user_data) |
Called when another application sends a launch request to the component.
Before calling frame_component_start_cb(), this callback function is called.
- Since :
- 5.5
- Remarks:
- After this callback function returns, the handle of the app_control is released. Therefore, if you want to use the handle after returning this callback function, you MUST copy it by using app_control_clone() function.
- The action must not be deallocated by the component. The action is managed by the platform and will be released when the app_control action is unregistered.
- The context should not be released. The context can be used only in the callback.
- The app_control should not be released. The app_control can be used only in the callback. To use outside, make a copy using app_control_clone().
- Parameters:
-
[in] context The context of the frame component instance [in] action The name of the app_control action [in] app_control The handle of the app_control [in] user_data The user data passed from component_based_app_add_frame_component() function
typedef Evas_Object*(* frame_component_create_cb)(component_h context, void *user_data) |
Called when the frame component instance is created.
The returned Evas_Object MUST NOT be released using evas_object_del(). The platform frees the window when the frame component instance is destroyed.
- Since :
- 5.5
- Remarks:
- The context should not be released. The context can be used only in the callback.
- Parameters:
-
[in] context The context of the frame component instance [in] user_data The user data passed from component_based_app_add_frame_component() function
- Returns:
- The Evas object on success, otherwise a null pointer on failure.
- See also:
- frame_component_get_window()
typedef void(* frame_component_destroy_cb)(component_h context, void *user_data) |
Called before the frame component instance is destroyed.
- Since :
- 5.5
- Remarks:
- The context should not be released. The context can be used only in the callback.
- Parameters:
-
[in] context The context of the frame component instance [in] user_data The user data passed from component_based_app_add_frame_component() function
typedef void(* frame_component_device_orientation_changed_cb)(component_h context, component_device_orientation_e orientation, void *user_data) |
Called when the device orientation is changed.
- Since :
- 5.5
- Remarks:
- The context should not be released. The context can be used only in the callback.
- Parameters:
-
[in] context The context of the frame component instance [in] orientation The device orientation [in] user_data The user data passed from component_based_app_add_frame_component() function
typedef void(* frame_component_language_changed_cb)(component_h context, const char *language, void *user_data) |
Called when the system language is changed.
- Since :
- 5.5
- Remarks:
- The context should not be released. The context can be used only in the callback.
- The language must not be deallocated by the component. The language can be used only in the callback.
- Parameters:
-
[in] context The context of the frame component instance [in] language The language [in] user_data The user data passed from component_based_app_add_frame_component() function
typedef void(* frame_component_low_battery_cb)(component_h context, component_low_battery_status_e status, void *user_data) |
Called when the battery status is changed.
- Since :
- 5.5
- Remarks:
- The context should not be released. The context can be used only in the callback.
- Parameters:
-
[in] context The context of the frame component instance [in] status The low battery status [in] user_data The user data passed from component_based_app_add_frame_component() function
typedef void(* frame_component_low_memory_cb)(component_h context, component_low_memory_status_e status, void *user_data) |
Called when the memory status is changed.
- Since :
- 5.5
- Remarks:
- The context should not be released. The context can be used only in the callback.
- Parameters:
-
[in] context The context of the frame component instance [in] status The low memory status [in] user_data The user data passed from component_based_app_add_frame_component() function
typedef void(* frame_component_pause_cb)(component_h context, void *user_data) |
Called when the frame component becomes invisible.
- Since :
- 5.5
- Remarks:
- The context should not be released. The context can be used only in the callback.
- Parameters:
-
[in] context The context of the frame component instance [in] user_data The user data passed from component_based_app_add_frame_component() function
typedef void(* frame_component_region_format_changed_cb)(component_h context, const char *region, void *user_data) |
Called when the system region format is changed.
- Since :
- 5.5
- Remarks:
- The context should not be released. The context can be used only in the callback.
- The region must not be deallocated by the component. The region can be used only in the callback.
- Parameters:
-
[in] context The context of the frame component instance [in] region The region format [in] user_data The user data passed from component_based_app_add_frame_component() function
typedef void(* frame_component_restore_content_cb)(component_h context, bundle *content, void *user_data) |
Called after the content information of the frame component instance is restored.
- Since :
- 5.5
- Remarks:
- The content should not be released. The content can be used only in the callback. To use outside, make a copy using bundle_dup().
- Parameters:
-
[in] context The context of the frame component instance [in] content The content information [in] user_data The user data passed from component_based_app_add_frame_component() function
typedef void(* frame_component_resume_cb)(component_h context, void *user_data) |
Called when the frame component becomes visible.
- Since :
- 5.5
- Remarks:
- The context should not be released. The context can be used only in the callback.
- Parameters:
-
[in] context The context of the frame component instance [in] user_data The user data passed from component_based_app_add_frame_component() function
typedef void(* frame_component_save_content_cb)(component_h context, bundle *content, void *user_data) |
Called before the content information of the frame component instance is saved.
- Since :
- 5.5
- Remarks:
- The content should not be released. The content can be used only in the callback. To use outside, make a copy using bundle_dup().
- Parameters:
-
[in] context The context of the frame component instance [in] content The content information [in] user_data The user data passed from component_based_app_add_frame_component() function
typedef void(* frame_component_start_cb)(component_h context, app_control_h app_control, bool restarted, void *user_data) |
Called when the frame component instance is started.
- Since :
- 5.5
- Remarks:
- The context should not be released. The context can be used only in the callback.
- The app_control should not be released. The app_control can be used only in the callback. To use outside, make a copy using app_control_clone().
- Parameters:
-
[in] context The context of the frame component instance [in] app_control The app control handle [in] restarted true
, if the instance is restarted[in] user_data The user data passed from component_based_app_add_frame_component() function
typedef void(* frame_component_stop_cb)(component_h context, void *user_data) |
Called before the frame component instance is stopped.
- Since :
- 5.5
- Remarks:
- The context should not be released. The context can be used only in the callback.
- Parameters:
-
[in] context The context of the frame component instance [in] user_data The user data passed from component_based_app_add_frame_component() function
typedef void(* frame_component_suspended_state_changed_cb)(component_h context, component_suspended_state_e state, void *user_data) |
Called when the suspended state of the frame component is changed.
- Since :
- 5.5
- Remarks:
- The context should not be released. The context can be used only in the callback.
- Parameters:
-
[in] context The context of the frame component instance [in] state The suspended state [in] user_data The user data passed from component_based_app_add_frame_component() function
Function Documentation
int frame_component_get_display_status | ( | component_h | context, |
component_display_status_e * | display_status | ||
) |
Gets the display status.
- Since :
- 5.5
- Parameters:
-
[in] context The context of the frame component instance [out] display_status The display status
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
COMPONENT_ERROR_NONE Successful COMPONENT_ERROR_NOT_SUPPORTED Not supported COMPONENT_ERROR_INVALID_PARAMETER Invalid parameter COMPONENT_ERROR_INVALID_CONTEXT The display is in an unknown state.
int frame_component_get_window | ( | component_h | context, |
Evas_Object ** | window | ||
) |
Gets an Evas object for the frame component.
- Since :
- 5.5
- Remarks:
- The
window
MUST NOT be released using evas_object_del(). The platform frees window when the frame component instance is destroyed.
- Parameters:
-
[in] context The context of the frame component instance [out] window The evas object for window
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
COMPONENT_ERROR_NONE Successful COMPONENT_ERROR_INVALID_PARAMETER Invalid parameter