Tizen Native API
6.5
|
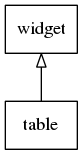
A container widget to arrange other widgets in a table where items can span multiple columns or rows - even overlap (and then be raised or lowered accordingly to adjust stacking if they do overlap).
The row and column count is not fixed. The table widget adjusts itself when subobjects are added to it dynamically.
The most common way to use a table is:
table = elm_table_add(win); evas_object_show(table); elm_table_padding_set(table, space_between_columns, space_between_rows); elm_table_pack(table, table_content_object, column, row, colspan, rowspan); elm_table_pack(table, table_content_object, next_column, next_row, colspan, rowspan); elm_table_pack(table, table_content_object, other_column, other_row, colspan, rowspan);
The following are examples of how to use a table:
Functions | |
void | elm_table_homogeneous_set (Elm_Table *obj, Eina_Bool homogeneous) |
Control the homogeneous state in a table. | |
Eina_Bool | elm_table_homogeneous_get (const Elm_Table *obj) |
Control the homogeneous state in a table. | |
void | elm_table_padding_set (Elm_Table *obj, int horizontal, int vertical) |
Set padding between cells. Default value is 0. | |
void | elm_table_padding_get (const Elm_Table *obj, int *horizontal, int *vertical) |
Get padding between cells. | |
void | elm_table_align_set (Elm_Table *obj, double horizontal, double vertical) |
Set alignment of table. Default value is 0.5. | |
void | elm_table_align_get (const Elm_Table *obj, double *horizontal, double *vertical) |
Get alignment of table. | |
void | elm_table_clear (Elm_Table *obj, Eina_Bool clear) |
Faster way to remove all child objects from a table object. | |
Efl_Canvas_Object * | elm_table_child_get (const Elm_Table *obj, int col, int row) |
Get child object of table at given coordinates. | |
void | elm_table_unpack (Elm_Table *obj, Efl_Canvas_Object *subobj) |
Remove child from table. | |
void | elm_table_pack (Elm_Table *obj, Efl_Canvas_Object *subobj, int column, int row, int colspan, int rowspan) |
Add a subobject on the table with the coordinates passed. | |
Evas_Object * | elm_table_add (Evas_Object *parent) |
Add a new table to the parent. | |
void | elm_table_pack_set (Evas_Object *subobj, int col, int row, int colspan, int rowspan) |
Set the packing location of an existing child of the table. | |
void | elm_table_pack_get (Evas_Object *subobj, int *col, int *row, int *colspan, int *rowspan) |
Get the packing location of an existing child of the table. |
Function Documentation
Evas_Object* elm_table_add | ( | Evas_Object * | parent | ) |
Add a new table to the parent.
- Parameters:
-
parent The parent object
- Returns:
- The new object or NULL if it cannot be created
- Since :
- 2.3
- Examples:
- mapbuf_example.c, table_example_01.c, and table_example_02.c.
void elm_table_align_get | ( | const Elm_Table * | obj, |
double * | horizontal, | ||
double * | vertical | ||
) |
Get alignment of table.
- Parameters:
-
[in] obj The object. [out] horizontal The horizontal alignment. [out] vertical The vertical alignment.
- Since (EFL) :
- 1.13
- Since :
- 3.0
void elm_table_align_set | ( | Elm_Table * | obj, |
double | horizontal, | ||
double | vertical | ||
) |
Set alignment of table. Default value is 0.5.
- Parameters:
-
[in] obj The object. [in] horizontal The horizontal alignment. [in] vertical The vertical alignment.
- Since (EFL) :
- 1.13
- Since :
- 3.0
Efl_Canvas_Object* elm_table_child_get | ( | const Elm_Table * | obj, |
int | col, | ||
int | row | ||
) |
Get child object of table at given coordinates.
- Parameters:
-
[in] obj The object. [in] col Column number of child object. [in] row Row number of child object.
- Returns:
- Child of object if find if not return
null
.
- Since :
- 3.0
void elm_table_clear | ( | Elm_Table * | obj, |
Eina_Bool | clear | ||
) |
Faster way to remove all child objects from a table object.
- Parameters:
-
[in] obj The object. [in] clear If true
, will delete children, else just remove from table.
- Since :
- 2.3
Eina_Bool elm_table_homogeneous_get | ( | const Elm_Table * | obj | ) |
Control the homogeneous state in a table.
- Parameters:
-
[in] obj The object.
- Returns:
- A boolean to set if the layout is homogeneous in the table.
- Since :
- 2.3
void elm_table_homogeneous_set | ( | Elm_Table * | obj, |
Eina_Bool | homogeneous | ||
) |
Control the homogeneous state in a table.
- Parameters:
-
[in] obj The object. [in] homogeneous A boolean to set if the layout is homogeneous in the table.
- Since :
- 2.3
- Examples:
- table_example_01.c, and table_example_02.c.
void elm_table_pack | ( | Elm_Table * | obj, |
Efl_Canvas_Object * | subobj, | ||
int | column, | ||
int | row, | ||
int | colspan, | ||
int | rowspan | ||
) |
Add a subobject on the table with the coordinates passed.
- Note:
- All positioning inside the table is relative to rows and columns, so a value of 0 for x and y, means the top left cell of the table, and a value of 1 for w and h means
subobj
only takes that 1 cell. - Columns and rows only guarantee 16bit unsigned values at best. That means that col + colspan AND row + rowspan must fit inside 16bit unsigned values cleanly. You will be warned once values exceed 15bit storage, and attempting to use values not able to fit in 16bits will result in failure.
- Parameters:
-
[in] obj The object. [in] subobj The subobject to be added to the table. [in] column Column number. [in] row Row number. [in] colspan Columns span. [in] rowspan Rows span.
- Since :
- 2.3
- Examples:
- mapbuf_example.c, table_example_01.c, and table_example_02.c.
void elm_table_pack_get | ( | Evas_Object * | subobj, |
int * | col, | ||
int * | row, | ||
int * | colspan, | ||
int * | rowspan | ||
) |
Get the packing location of an existing child of the table.
- Parameters:
-
subobj The subobject to be modified in the table col Column number row Row number colspan colspan rowspan rowspan
- See also:
- elm_table_pack_set()
- Since :
- 2.3
void elm_table_pack_set | ( | Evas_Object * | subobj, |
int | col, | ||
int | row, | ||
int | colspan, | ||
int | rowspan | ||
) |
Set the packing location of an existing child of the table.
- Parameters:
-
subobj The subobject to be modified in the table col Column number row Row number colspan colspan rowspan rowspan
Modifies the position of an object already in the table.
- Note:
- All positioning inside the table is relative to rows and columns, so a value of 0 for col and row, means the top left cell of the table, and a value of 1 for colspan and rowspan means
subobj
only takes that 1 cell.
- Since :
- 2.3
void elm_table_padding_get | ( | const Elm_Table * | obj, |
int * | horizontal, | ||
int * | vertical | ||
) |
Get padding between cells.
- Parameters:
-
[in] obj The object. [out] horizontal The horizontal padding. [out] vertical The vertical padding.
- Since :
- 2.3
void elm_table_padding_set | ( | Elm_Table * | obj, |
int | horizontal, | ||
int | vertical | ||
) |
Set padding between cells. Default value is 0.
- Parameters:
-
[in] obj The object. [in] horizontal The horizontal padding. [in] vertical The vertical padding.
- Since :
- 2.3
- Examples:
- table_example_01.c.
void elm_table_unpack | ( | Elm_Table * | obj, |
Efl_Canvas_Object * | subobj | ||
) |
Remove child from table.
- Parameters:
-
[in] obj The object. [in] subobj The subobject.
- Since :
- 2.3