Tizen Native API
6.5
|
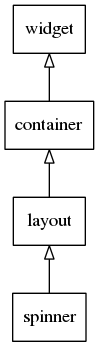
A spinner is a widget which allows the user to increase or decrease numeric values using arrow buttons, or edit values directly, clicking over it and typing the new value.
By default the spinner will not wrap and has a label of "%.0f" (just showing the integer value of the double).
A spinner has a label that is formatted with floating point values and thus accepts a printf-style format string, like “%1.2f units”.
It also allows specific values to be replaced by pre-defined labels.
This widget inherits from the Layout one, so that all the functions acting on it also work for spinner objects.
This widget emits the following signals, besides the ones sent from Layout :
"changed"
- Whenever the spinner value is changed."delay,changed"
- A short time after the value is changed by the user. This will be called only when the user stops dragging for a very short period or when they release their finger/mouse, so it avoids possibly expensive reactions to the value change."language,changed"
- the program's language changed"focused"
- When the spinner has received focus. (since 1.8)"unfocused"
- When the spinner has lost focus. (since 1.8)"spinner,drag,start"
- When dragging has started. (since 1.8)"spinner,drag,stop"
- When dragging has stopped. (since 1.8)
Available styles for it:
"default"
;"vertical"
: up/down buttons at the right side and text left aligned.
Supported elm_object common APIs.
- elm_object_signal_emit
- elm_object_signal_callback_add
- elm_object_signal_callback_del
- elm_object_disabled_set
- elm_object_disabled_get
Here is an example on its usage: Spinner widget example
Functions | |
void | elm_spinner_wrap_set (Elm_Spinner *obj, Eina_Bool wrap) |
Control whether the spinner should wrap when it reaches its minimum or maximum value. | |
Eina_Bool | elm_spinner_wrap_get (const Elm_Spinner *obj) |
Control whether the spinner should wrap when it reaches its minimum or maximum value. | |
void | elm_spinner_interval_set (Elm_Spinner *obj, double interval) |
Control the interval on time updates for a user mouse button hold on spinner widgets' arrows. | |
double | elm_spinner_interval_get (const Elm_Spinner *obj) |
Control the interval on time updates for a user mouse button hold on spinner widgets' arrows. | |
void | elm_spinner_round_set (Elm_Spinner *obj, int rnd) |
Control the round value for rounding. | |
int | elm_spinner_round_get (const Elm_Spinner *obj) |
Control the round value for rounding. | |
void | elm_spinner_editable_set (Elm_Spinner *obj, Eina_Bool editable) |
Control whether the spinner can be directly edited by the user or not. | |
Eina_Bool | elm_spinner_editable_get (const Elm_Spinner *obj) |
Control whether the spinner can be directly edited by the user or not. | |
void | elm_spinner_base_set (Elm_Spinner *obj, double base) |
Control the base for rounding. | |
double | elm_spinner_base_get (const Elm_Spinner *obj) |
Control the base for rounding. | |
void | elm_spinner_label_format_set (Elm_Spinner *obj, const char *fmt) |
Control the format string of the displayed label. | |
const char * | elm_spinner_label_format_get (const Elm_Spinner *obj) |
Control the format string of the displayed label. | |
void | elm_spinner_special_value_add (Elm_Spinner *obj, double value, const char *label) |
Control special string to display in the place of the numerical value. | |
Evas_Object * | elm_spinner_add (Evas_Object *parent) |
void | elm_spinner_special_value_del (Evas_Object *obj, double value) |
const char * | elm_spinner_special_value_get (Evas_Object *obj, double value) |
void | elm_spinner_min_max_set (Evas_Object *obj, double min, double max) |
Control the minimum and maximum values for the spinner. | |
void | elm_spinner_min_max_get (const Evas_Object *obj, double *min, double *max) |
Control the minimum and maximum values for the spinner. | |
void | elm_spinner_step_set (Evas_Object *obj, double step) |
Control the step used to increment or decrement the spinner value. | |
double | elm_spinner_step_get (const Evas_Object *obj) |
Control the step used to increment or decrement the spinner value. | |
void | elm_spinner_value_set (Evas_Object *obj, double val) |
Control the value the spinner displays. | |
double | elm_spinner_value_get (const Evas_Object *obj) |
Control the value the spinner displays. |
Function Documentation
Evas_Object* elm_spinner_add | ( | Evas_Object * | parent | ) |
Add a new spinner widget to the given parent Elementary (container) object.
- Parameters:
-
parent The parent object.
- Returns:
- a new spinner widget handle or
NULL
, on errors.
This function inserts a new spinner widget on the canvas.
- Since :
- 2.3
- Examples:
- bg_example_03.c, gengrid_example.c, slideshow_example.c, spinner_example.c, and transit_example_03.c.
double elm_spinner_base_get | ( | const Elm_Spinner * | obj | ) |
Control the base for rounding.
Rounding works as follows:
rounded_val = base + (double)(((value - base) / round) * round)
Where rounded_val, value and base are doubles, and round is an integer.
This means that things will be rounded to increments (or decrements) of "round" starting from value base
. The default base for rounding is 0.
Example: round = 3, base = 2 Values: ..., -2, 0, 2, 5, 8, 11, 14, ...
Example: round = 2, base = 5.5 Values: ..., -0.5, 1.5, 3.5, 5.5, 7.5, 9.5, 11.5, ...
- Parameters:
-
[in] obj The object.
- Returns:
- The base value
- Since :
- 2.3
void elm_spinner_base_set | ( | Elm_Spinner * | obj, |
double | base | ||
) |
Control the base for rounding.
Rounding works as follows:
rounded_val = base + (double)(((value - base) / round) * round)
Where rounded_val, value and base are doubles, and round is an integer.
This means that things will be rounded to increments (or decrements) of "round" starting from value base
. The default base for rounding is 0.
Example: round = 3, base = 2 Values: ..., -2, 0, 2, 5, 8, 11, 14, ...
Example: round = 2, base = 5.5 Values: ..., -0.5, 1.5, 3.5, 5.5, 7.5, 9.5, 11.5, ...
- Parameters:
-
[in] obj The object. [in] base The base value
- Since :
- 2.3
Eina_Bool elm_spinner_editable_get | ( | const Elm_Spinner * | obj | ) |
Control whether the spinner can be directly edited by the user or not.
Spinner objects can have edition disabled, in which state they will be changed only by arrows. Useful for contexts where you don't want your users to interact with it writing the value. Specially when using special values, the user can see real value instead of special label on edition.
It's enabled by default.
- Parameters:
-
[in] obj The object.
- Returns:
true
to allow users to edit it orfalse
to don't allow users to edit it directly.
- Since :
- 2.3
void elm_spinner_editable_set | ( | Elm_Spinner * | obj, |
Eina_Bool | editable | ||
) |
Control whether the spinner can be directly edited by the user or not.
Spinner objects can have edition disabled, in which state they will be changed only by arrows. Useful for contexts where you don't want your users to interact with it writing the value. Specially when using special values, the user can see real value instead of special label on edition.
It's enabled by default.
- Parameters:
-
[in] obj The object. [in] editable true
to allow users to edit it orfalse
to don't allow users to edit it directly.
- Since :
- 2.3
- Examples:
- bg_example_03.c, spinner_example.c, and transit_example_03.c.
double elm_spinner_interval_get | ( | const Elm_Spinner * | obj | ) |
Control the interval on time updates for a user mouse button hold on spinner widgets' arrows.
This interval value is decreased while the user holds the mouse pointer either incrementing or decrementing spinner's value.
This helps the user to get to a given value distant from the current one easier/faster, as it will start to change quicker and quicker on mouse button holds.
The calculation for the next change interval value, starting from the one set with this call, is the previous interval divided by $1.05, so it decreases a little bit.
The default starting interval value for automatic changes is $0.85 seconds.
- Parameters:
-
[in] obj The object.
- Returns:
- The (first) interval value in seconds.
- Since :
- 2.3
void elm_spinner_interval_set | ( | Elm_Spinner * | obj, |
double | interval | ||
) |
Control the interval on time updates for a user mouse button hold on spinner widgets' arrows.
This interval value is decreased while the user holds the mouse pointer either incrementing or decrementing spinner's value.
This helps the user to get to a given value distant from the current one easier/faster, as it will start to change quicker and quicker on mouse button holds.
The calculation for the next change interval value, starting from the one set with this call, is the previous interval divided by $1.05, so it decreases a little bit.
The default starting interval value for automatic changes is $0.85 seconds.
- Parameters:
-
[in] obj The object. [in] interval The (first) interval value in seconds.
- Since :
- 2.3
- Examples:
- spinner_example.c.
const char* elm_spinner_label_format_get | ( | const Elm_Spinner * | obj | ) |
Control the format string of the displayed label.
If NULL
, this sets the format to "%.0f". If not it sets the format string for the label text. The label text is provided a floating point value, so the label text can display up to 1 floating point value. Note that this is optional.
Use a format string such as "%1.2f meters" for example, and it will display values like: "3.14 meters" for a value equal to 3.14159.
Default is "%0.f".
- Parameters:
-
[in] obj The object.
- Returns:
- The format string for the label display.
- Since :
- 2.3
void elm_spinner_label_format_set | ( | Elm_Spinner * | obj, |
const char * | fmt | ||
) |
Control the format string of the displayed label.
If NULL
, this sets the format to "%.0f". If not it sets the format string for the label text. The label text is provided a floating point value, so the label text can display up to 1 floating point value. Note that this is optional.
Use a format string such as "%1.2f meters" for example, and it will display values like: "3.14 meters" for a value equal to 3.14159.
Default is "%0.f".
- Parameters:
-
[in] obj The object. [in] fmt The format string for the label display.
- Since :
- 2.3
- Examples:
- bg_example_03.c, gengrid_example.c, slideshow_example.c, spinner_example.c, and transit_example_03.c.
void elm_spinner_min_max_get | ( | const Evas_Object * | obj, |
double * | min, | ||
double * | max | ||
) |
Control the minimum and maximum values for the spinner.
Define the allowed range of values to be selected by the user.
If actual value is less than min
, it will be updated to min
. If it is bigger then max
, will be updated to max
. Actual value can be get with elm_obj_spinner_value_get.
By default, min is equal to 0, and max is equal to 100.
- Warning:
- Maximum must be greater than minimum.
- Parameters:
-
[out] min The minimum value. [out] max The maximum value.
- Since :
- 2.3
void elm_spinner_min_max_set | ( | Evas_Object * | obj, |
double | min, | ||
double | max | ||
) |
Control the minimum and maximum values for the spinner.
Define the allowed range of values to be selected by the user.
If actual value is less than min
, it will be updated to min
. If it is bigger then max
, will be updated to max
. Actual value can be get with elm_obj_spinner_value_get.
By default, min is equal to 0, and max is equal to 100.
- Warning:
- Maximum must be greater than minimum.
- Parameters:
-
[in] min The minimum value. [in] max The maximum value.
- Since :
- 2.3
- Examples:
- bg_example_03.c, gengrid_example.c, slideshow_example.c, spinner_example.c, and transit_example_03.c.
int elm_spinner_round_get | ( | const Elm_Spinner * | obj | ) |
Control the round value for rounding.
Sets the rounding value used for value rounding in the spinner.
- Parameters:
-
[in] obj The object.
- Returns:
- The rounding value
- Since :
- 2.3
void elm_spinner_round_set | ( | Elm_Spinner * | obj, |
int | rnd | ||
) |
Control the round value for rounding.
Sets the rounding value used for value rounding in the spinner.
- Parameters:
-
[in] obj The object. [in] rnd The rounding value
- Since :
- 2.3
void elm_spinner_special_value_add | ( | Elm_Spinner * | obj, |
double | value, | ||
const char * | label | ||
) |
Control special string to display in the place of the numerical value.
It's useful for cases when a user should select an item that is better indicated by a label than a value. For example, weekdays or months.
- Note:
- If another label was previously set to
value
, it will be replaced by the new label.
- Parameters:
-
[in] obj The object. [in] value The value to be replaced. [in] label The label to be used.
- Since :
- 2.3
- Examples:
- bg_example_03.c, spinner_example.c, and transit_example_03.c.
void elm_spinner_special_value_del | ( | Evas_Object * | obj, |
double | value | ||
) |
Delete the special string display in the place of the numerical value.
- Parameters:
-
obj The spinner object. value The replaced value.
It will remove a previously added special value. After this, the spinner will display the value itself instead of a label.
- See also:
- elm_spinner_special_value_add() for more details.
- Since (EFL) :
- 1.8
- Since :
- 2.3
const char* elm_spinner_special_value_get | ( | Evas_Object * | obj, |
double | value | ||
) |
Get the special string display in the place of the numerical value.
- Parameters:
-
obj The spinner object. value The replaced value.
- Returns:
- The used label.
- See also:
- elm_spinner_special_value_add() for more details.
- Since (EFL) :
- 1.8
- Since :
- 2.3
double elm_spinner_step_get | ( | const Evas_Object * | obj | ) |
Control the step used to increment or decrement the spinner value.
This value will be incremented or decremented to the displayed value. It will be incremented while the user keep right or top arrow pressed, and will be decremented while the user keep left or bottom arrow pressed.
The interval to increment / decrement can be set with elm_obj_spinner_interval_set.
By default step value is equal to 1.
- Returns:
- The step value.
- Since :
- 2.3
void elm_spinner_step_set | ( | Evas_Object * | obj, |
double | step | ||
) |
Control the step used to increment or decrement the spinner value.
This value will be incremented or decremented to the displayed value. It will be incremented while the user keep right or top arrow pressed, and will be decremented while the user keep left or bottom arrow pressed.
The interval to increment / decrement can be set with elm_obj_spinner_interval_set.
By default step value is equal to 1.
- Parameters:
-
[in] step The step value.
- Since :
- 2.3
- Examples:
- slideshow_example.c, and spinner_example.c.
double elm_spinner_value_get | ( | const Evas_Object * | obj | ) |
Control the value the spinner displays.
Value will be presented on the label following format specified with elm_spinner_format_set().
Warning The value must to be between min and max values. This values are set by elm_spinner_min_max_set().
- Returns:
- The value to be displayed.
- Since :
- 2.3
- Examples:
- bg_example_03.c, gengrid_example.c, slideshow_example.c, spinner_example.c, and transit_example_03.c.
void elm_spinner_value_set | ( | Evas_Object * | obj, |
double | val | ||
) |
Control the value the spinner displays.
Value will be presented on the label following format specified with elm_spinner_format_set().
Warning The value must to be between min and max values. This values are set by elm_spinner_min_max_set().
- Parameters:
-
[in] val The value to be displayed.
- Since :
- 2.3
- Examples:
- gengrid_example.c, and slideshow_example.c.
Eina_Bool elm_spinner_wrap_get | ( | const Elm_Spinner * | obj | ) |
Control whether the spinner should wrap when it reaches its minimum or maximum value.
Disabled by default. If disabled, when the user tries to increment the value, but displayed value plus step value is bigger than maximum value, the new value will be the maximum value. The same happens when the user tries to decrement it, but the value less step is less than minimum value. In this case, the new displayed value will be the minimum value.
When wrap is enabled, when the user tries to increment the value, but displayed value plus step value is bigger than maximum value, the new value will be the minimum value. When the the user tries to decrement it, but the value less step is less than minimum value, the new displayed value will be the maximum value.
E.g.: min
= 10 max
= 50 step
= 20 displayed
= 20
When the user decrement value (using left or bottom arrow), it will displays $50.
- Parameters:
-
[in] obj The object.
- Returns:
true
to enable wrap orfalse
to disable it.
- Since :
- 2.3
void elm_spinner_wrap_set | ( | Elm_Spinner * | obj, |
Eina_Bool | wrap | ||
) |
Control whether the spinner should wrap when it reaches its minimum or maximum value.
Disabled by default. If disabled, when the user tries to increment the value, but displayed value plus step value is bigger than maximum value, the new value will be the maximum value. The same happens when the user tries to decrement it, but the value less step is less than minimum value. In this case, the new displayed value will be the minimum value.
When wrap is enabled, when the user tries to increment the value, but displayed value plus step value is bigger than maximum value, the new value will be the minimum value. When the the user tries to decrement it, but the value less step is less than minimum value, the new displayed value will be the maximum value.
E.g.: min
= 10 max
= 50 step
= 20 displayed
= 20
When the user decrement value (using left or bottom arrow), it will displays $50.
- Parameters:
-
[in] obj The object. [in] wrap true
to enable wrap orfalse
to disable it.
- Since :
- 2.3
- Examples:
- spinner_example.c.