Tizen Native API
6.5
|
The TTS API provides functions for synthesizing voice from text and playing synthesized sound data.
Required Header
#include <tts.h>
Overview
You can use Text-To-Speech (TTS) API to read sound data transformed by the engine from input texts. Applications can add input-text to queue for reading continuously and control the player that can play, pause, and stop sound data synthesized from text. To use TTS, follow these steps:
1. Create a handle
2. Register callback functions for notifications
3. Prepare tts-daemon asynchronously
4. Add text to queue for speech
5. Start synthesizing voice from text and play synthesized sound data
6. Pause the player
7. Resume the player
8. Stop the player
9. Destroy a handle
The TTS API also notifies you (by callback mechanism) when the state of TTS is changed, utterance is started and completed, default voice is changed or an error occurred. An application should register callback functions: tts_state_changed_cb(), tts_utterance_started_cb(), tts_utterance_completed_cb(), tts_default_voice_changed_cb(), tts_error_cb(), tts_engine_changed_cb().
State Diagram
The following diagram shows the life cycle and the states of the TTS. This state diagram shows that the state can be changed from 'None' to 'Created' by calling the tts_create() function. Similarly, you can know the relation between the states and API functions. You need to know the current state before calling the API, because the TTS API is based on state machine. The current state can be known from the state changed callback.
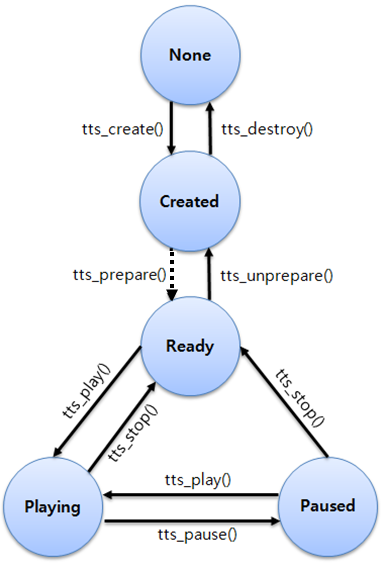
State Transitions
The following table shows previous state and post state of main function. It can be known what precondition is and which synchronous type is. Please check both previous state and post state from the table carefully. In case of tts_prepare() function, state doesn't be changed at once, but asynchronously. From the above state diagram, you can see dotted line as asynchronous api. In this case, the state changed callback is only way to know state transition as ready.
FUNCTION | PRE-STATE | POST-STATE | SYNC TYPE |
---|---|---|---|
tts_prepare() | Created | Ready | ASYNC |
tts_play() | Ready, Paused | Playing | SYNC |
tts_stop() | Ready, Playing, Paused | Ready | SYNC |
tts_pause() | Playing | Paused | SYNC |
State Dependent Function Calls
The following table shows state-dependent function calls. It is forbidden to call functions listed below in wrong states. Violation of this rule may result in an unpredictable behavior.
FUNCTION | VALID STATES | DESCRIPTION |
---|---|---|
tts_create() | None | All functions must be called after tts_create() |
tts_destroy() | Created, Ready, Playing, Paused | |
tts_set_mode() | Created | The application should set mode to use TTS for screen reader or notification like driving mode. |
tts_get_mode() | Created | |
tts_prepare() | Created | This function works asynchronously. If daemon fork is failed, application gets the error callback. |
tts_unprepare() | Ready | |
tts_foreach_supported_voices() | Created, Ready, Playing, Paused | |
tts_get_default_voice() | Created, Ready, Playing, Paused | |
tts_get_state() | Created, Ready, Playing, Paused | |
tts_get_max_text_size() | Ready | |
tts_get_speed_range() | Ready | |
tts_set_credential() | Created, Ready | |
tts_set_private_data() tts_get_private_data() | Ready | |
tts_get_error_message() | Created, Ready, Recording, Processing | This function should be called during TTS error callback. |
tts_add_text() | Ready, Playing, Paused | |
tts_play() | Ready, Paused | |
tts_stop() | Ready, Playing, Paused | |
tts_pause() | Playing | |
tts_set_state_changed_cb() tts_unset_state_changed_cb() tts_set_utterance_started_cb() tts_unset_utterance_started_cb() tts_set_utterance_completed_cb() tts_unset_utterance_completed_cb() tts_set_default_voice_changed_cb() tts_unset_default_voice_changed_cb() tts_set_error_cb() tts_unset_error_cb() tts_set_engine_changed_cb() tts_unset_engine_changed_cb() | Created | All callback function should be registered in Created state |
Related Features
This API is related with the following features:
- http://tizen.org/feature/speech.synthesis
It is recommended to design feature related codes in your application for reliability.
You can check if a device supports the related features for this API by using System Information, thereby controlling the procedure of your application.
To ensure your application is only running on the device with specific features, please define the features in your manifest file using the manifest editor in the SDK.
More details on featuring your application can be found from Feature Element.
Functions | |
int | tts_create (tts_h *tts) |
Creates a handle for TTS. | |
int | tts_destroy (tts_h tts) |
Destroys the handle and disconnects the daemon. | |
int | tts_set_mode (tts_h tts, tts_mode_e mode) |
Sets the TTS mode. | |
int | tts_get_mode (tts_h tts, tts_mode_e *mode) |
Gets the TTS mode. | |
int | tts_set_credential (tts_h tts, const char *credential) |
Sets the app credential. | |
int | tts_prepare (tts_h tts) |
Connects the daemon asynchronously. | |
int | tts_unprepare (tts_h tts) |
Disconnects the daemon. | |
int | tts_foreach_supported_voices (tts_h tts, tts_supported_voice_cb callback, void *user_data) |
Retrieves all supported voices of the current engine using callback function. | |
int | tts_get_default_voice (tts_h tts, char **language, int *voice_type) |
Gets the default voice set by the user. | |
int | tts_set_private_data (tts_h tts, const char *key, const char *data) |
Sets the private data to tts engine. | |
int | tts_get_private_data (tts_h tts, const char *key, char **data) |
Gets the private data from tts engine. | |
int | tts_get_max_text_size (tts_h tts, unsigned int *size) |
Gets the maximum byte size for text. | |
int | tts_get_state (tts_h tts, tts_state_e *state) |
Gets the current state of TTS. | |
int | tts_get_speed_range (tts_h tts, int *min, int *normal, int *max) |
Gets the speed range. | |
int | tts_get_error_message (tts_h tts, char **err_msg) |
Gets the current error message. | |
int | tts_check_screen_reader_on (tts_h tts, bool *is_on) |
Checks whether screen reader is turned on or not. | |
int | tts_add_text (tts_h tts, const char *text, const char *language, int voice_type, int speed, int *utt_id) |
Adds a text to the queue. | |
int | tts_play (tts_h tts) |
Starts synthesizing voice from the text and plays the synthesized audio data. | |
int | tts_stop (tts_h tts) |
Stops playing the utterance and clears the queue. | |
int | tts_pause (tts_h tts) |
Pauses the currently playing utterance. | |
int | tts_repeat (tts_h tts, char **text_repeat, int *utt_id) |
Repeats the last added text. | |
int | tts_set_state_changed_cb (tts_h tts, tts_state_changed_cb callback, void *user_data) |
Registers a callback function to be called when the TTS state changes. | |
int | tts_unset_state_changed_cb (tts_h tts) |
Unregisters the callback function. | |
int | tts_set_utterance_started_cb (tts_h tts, tts_utterance_started_cb callback, void *user_data) |
Registers a callback function to detect utterance start. | |
int | tts_unset_utterance_started_cb (tts_h tts) |
Unregisters the callback function. | |
int | tts_set_utterance_completed_cb (tts_h tts, tts_utterance_completed_cb callback, void *user_data) |
Registers a callback function to detect utterance completion. | |
int | tts_unset_utterance_completed_cb (tts_h tts) |
Unregisters the callback function. | |
int | tts_set_error_cb (tts_h tts, tts_error_cb callback, void *user_data) |
Registers a callback function to detect errors. | |
int | tts_unset_error_cb (tts_h tts) |
Unregisters the callback function. | |
int | tts_set_default_voice_changed_cb (tts_h tts, tts_default_voice_changed_cb callback, void *user_data) |
Registers a callback function to detect default voice change. | |
int | tts_unset_default_voice_changed_cb (tts_h tts) |
Unregisters the callback function. | |
int | tts_set_engine_changed_cb (tts_h tts, tts_engine_changed_cb callback, void *user_data) |
Registers a callback function to detect the engine change. | |
int | tts_unset_engine_changed_cb (tts_h tts) |
Unregisters the callback function. | |
int | tts_set_screen_reader_changed_cb (tts_h tts, tts_screen_reader_changed_cb callback, void *user_data) |
Registers a callback function to detect the option of screen reader is changed or not. | |
int | tts_unset_screen_reader_changed_cb (tts_h tts) |
Unregisters the callback function to detect the option of screen reader is changed or not. | |
Typedefs | |
typedef struct tts_s * | tts_h |
The TTS handle. | |
typedef void(* | tts_state_changed_cb )(tts_h tts, tts_state_e previous, tts_state_e current, void *user_data) |
Called when the state of TTS is changed. | |
typedef void(* | tts_utterance_started_cb )(tts_h tts, int utt_id, void *user_data) |
Called when utterance has started. | |
typedef void(* | tts_utterance_completed_cb )(tts_h tts, int utt_id, void *user_data) |
Called when utterance is finished. | |
typedef void(* | tts_error_cb )(tts_h tts, int utt_id, tts_error_e reason, void *user_data) |
Called when an error occurs. | |
typedef bool(* | tts_supported_voice_cb )(tts_h tts, const char *language, int voice_type, void *user_data) |
Called to retrieve the supported voice. | |
typedef void(* | tts_default_voice_changed_cb )(tts_h tts, const char *previous_language, int previous_voice_type, const char *current_language, int current_voice_type, void *user_data) |
Called when the default voice is changed. | |
typedef void(* | tts_engine_changed_cb )(tts_h tts, const char *engine_id, const char *language, int voice_type, bool need_credential, void *user_data) |
Called when the engine is changed. | |
typedef void(* | tts_screen_reader_changed_cb )(tts_h tts, bool is_on, void *user_data) |
Called when the option of screen reader is changed. | |
Defines | |
#define | TTS_SPEED_AUTO 0 |
Definition for automatic speaking speed. | |
#define | TTS_VOICE_TYPE_AUTO 0 |
Definition for automatic voice type. | |
#define | TTS_VOICE_TYPE_MALE 1 |
Definition for male voice type. | |
#define | TTS_VOICE_TYPE_FEMALE 2 |
Definition for female voice type. | |
#define | TTS_VOICE_TYPE_CHILD 3 |
Definition for child voice type. |
Define Documentation
#define TTS_SPEED_AUTO 0 |
Definition for automatic speaking speed.
- Since :
- 2.3
#define TTS_VOICE_TYPE_AUTO 0 |
Definition for automatic voice type.
- Since :
- 2.3
#define TTS_VOICE_TYPE_CHILD 3 |
Definition for child voice type.
- Since :
- 2.3
#define TTS_VOICE_TYPE_FEMALE 2 |
Definition for female voice type.
- Since :
- 2.3
#define TTS_VOICE_TYPE_MALE 1 |
Definition for male voice type.
- Since :
- 2.3
Typedef Documentation
typedef void(* tts_default_voice_changed_cb)(tts_h tts, const char *previous_language, int previous_voice_type, const char *current_language, int current_voice_type, void *user_data) |
Called when the default voice is changed.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [in] previous_language The previous language [in] previous_voice_type The previous voice type [in] current_language The current language [in] current_voice_type The current voice type [in] user_data The user data passed from the callback registration function
- See also:
- tts_set_default_voice_changed_cb()
typedef void(* tts_engine_changed_cb)(tts_h tts, const char *engine_id, const char *language, int voice_type, bool need_credential, void *user_data) |
Called when the engine is changed.
- Since :
- 3.0
- Parameters:
-
[in] tts The TTS handle [in] engine_id Engine ID [in] language The default language specified as an ISO 3166 alpha-2 two letter country-code followed by ISO 639-1 for the two-letter language code (for example, "ko_KR" for Korean, "en_US" for American English) [in] voice_type The default voice type [in] need_credential The necessity of credential [in] user_data The user data passed from the callback registration function
- See also:
- tts_set_engine_changed_cb()
typedef void(* tts_error_cb)(tts_h tts, int utt_id, tts_error_e reason, void *user_data) |
Called when an error occurs.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [in] utt_id The utterance ID passed from the add text function [in] reason The error code [in] user_data The user data passed from the callback registration function
- Precondition:
- An application registers this callback using tts_set_error_cb() to detect error.
typedef struct tts_s* tts_h |
The TTS handle.
- Since :
- 2.3
typedef void(* tts_screen_reader_changed_cb)(tts_h tts, bool is_on, void *user_data) |
Called when the option of screen reader is changed.
- Since :
- 6.5
- Parameters:
-
[in] tts The TTS handle [in] is_on The status of screen reader. If is_on is true
, screen reader is turned on. If not, it is turned off.[in] user_data The user data passed from the callback registration function
- See also:
- tts_set_screen_reader_changed_cb()
typedef void(* tts_state_changed_cb)(tts_h tts, tts_state_e previous, tts_state_e current, void *user_data) |
Called when the state of TTS is changed.
- Since :
- 2.3
If the daemon must stop player because of changing engine and the daemon must pause player because of other requests, this callback function is called.
- Parameters:
-
[in] tts The TTS handle [in] previous The previous state [in] current The current state [in] user_data The user data passed from the callback registration function
- Precondition:
- An application registers this callback using tts_set_state_changed_cb() to detect changing state.
typedef bool(* tts_supported_voice_cb)(tts_h tts, const char *language, int voice_type, void *user_data) |
Called to retrieve the supported voice.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [in] language Language specified as an ISO 3166 alpha-2 two letter country-code followed by ISO 639-1 for the two-letter language code (for example, "ko_KR" for Korean, "en_US" for American English) [in] voice_type A voice type (e.g. TTS_VOICE_TYPE_MALE, TTS_VOICE_TYPE_FEMALE) [in] user_data The user data passed from the foreach function
- Returns:
true
to continue with the next iteration of the loop,false
to break out of the loop
- Precondition:
- tts_foreach_supported_voices() will invoke this callback function.
- See also:
- tts_foreach_supported_voices()
typedef void(* tts_utterance_completed_cb)(tts_h tts, int utt_id, void *user_data) |
Called when utterance is finished.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [in] utt_id The utterance ID passed from the add text function [in] user_data The user data passed from the callback registration function
- Precondition:
- An application registers this callback using tts_set_utterance_completed_cb() to detect utterance completed.
typedef void(* tts_utterance_started_cb)(tts_h tts, int utt_id, void *user_data) |
Called when utterance has started.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [in] utt_id The utterance ID passed from the add text function [in] user_data The user data passed from the callback registration function
- Precondition:
- An application registers this callback using tts_set_utterance_started_cb() to detect utterance started.
Enumeration Type Documentation
enum tts_error_e |
Enumeration for error code.
- Since :
- 2.3
- Enumerator:
enum tts_mode_e |
enum tts_state_e |
Function Documentation
int tts_add_text | ( | tts_h | tts, |
const char * | text, | ||
const char * | language, | ||
int | voice_type, | ||
int | speed, | ||
int * | utt_id | ||
) |
Adds a text to the queue.
- Since :
- 2.3
- Remarks:
- Locale(e.g. setlocale()) MUST be set for utf8 text validation check.
- Parameters:
-
[in] tts The TTS handle [in] text An input text based utf8 [in] language The language selected from the tts_foreach_supported_voices() (e.g. 'NULL'(Automatic), 'en_US') [in] voice_type The voice type selected from the tts_foreach_supported_voices() (e.g. TTS_VOICE_TYPE_AUTO, TTS_VOICE_TYPE_FEMALE) [in] speed A speaking speed (e.g. TTS_SPEED_AUTO or the value from tts_get_speed_range()) [out] utt_id The utterance ID passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_PERMISSION_DENIED Permission denied TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state TTS_ERROR_INVALID_VOICE Invalid voice about language, voice type TTS_ERROR_OPERATION_FAILED Operation failure TTS_ERROR_SCREEN_READER_OFF Screen reader is turned off
- Precondition:
- The state should be TTS_STATE_READY, TTS_STATE_PLAYING or TTS_STATE_PAUSED.
int tts_check_screen_reader_on | ( | tts_h | tts, |
bool * | is_on | ||
) |
Checks whether screen reader is turned on or not.
- Since :
- 6.5
- Remarks:
- If TTS mode is TTS_MODE_SCREEN_READER, you should call this function to check whether screen reader is turned on or not, before calling 'tts_prepare()'. If TTS mode is TTS_MODE_SCREEN_READER and is_on is
false
, all other functions will return TTS_ERROR_SCREEN_READER_OFF. The is_on must be released using free() when it is no longer required.
- Parameters:
-
[in] tts The TTS handle [out] is_on The current status of screen reader
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter
int tts_create | ( | tts_h * | tts | ) |
Creates a handle for TTS.
- Since :
- 2.3
- Remarks:
- If the function succeeds, tts handle must be released with tts_destroy().
- Parameters:
-
[out] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_OUT_OF_MEMORY Out of memory TTS_ERROR_ENGINE_NOT_FOUND Engine not found TTS_ERROR_OPERATION_FAILED Operation failure
- Postcondition:
- If this function is called, the TTS state will be TTS_STATE_CREATED.
- See also:
- tts_destroy()
int tts_destroy | ( | tts_h | tts | ) |
Destroys the handle and disconnects the daemon.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_OPERATION_FAILED Operation failure
- See also:
- tts_create()
int tts_foreach_supported_voices | ( | tts_h | tts, |
tts_supported_voice_cb | callback, | ||
void * | user_data | ||
) |
Retrieves all supported voices of the current engine using callback function.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [in] callback The callback function to invoke [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_ENGINE_NOT_FOUND Engine not found TTS_ERROR_OPERATION_FAILED Operation failure
- Postcondition:
- This function invokes tts_supported_voice_cb() repeatedly for getting voices.
- See also:
- tts_get_default_voice()
int tts_get_default_voice | ( | tts_h | tts, |
char ** | language, | ||
int * | voice_type | ||
) |
Gets the default voice set by the user.
- Since :
- 2.3
- Remarks:
- If the function succeeds, language must be released with free().
- Parameters:
-
[in] tts The TTS handle [out] language Language specified as an ISO 3166 alpha-2 two letter country-code followed by ISO 639-1 for the two-letter language code (for example, "ko_KR" for Korean, "en_US" for American English) [out] voice_type The voice type
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_ENGINE_NOT_FOUND Engine not found TTS_ERROR_OPERATION_FAILED Operation failure
- See also:
- tts_foreach_supported_voices()
int tts_get_error_message | ( | tts_h | tts, |
char ** | err_msg | ||
) |
Gets the current error message.
- Since :
- 3.0
- Remarks:
- This function should be called from a tts error callback. Calling in any other context will result in an Operation failed error. A successful call will allocate err_msg, which must be released by calling free() when it is no longer required.
- Parameters:
-
[in] tts The TTS handle [out] err_msg The current error message
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_OPERATION_FAILED Operation failure
- See also:
- tts_set_error_cb()
- tts_unset_error_cb()
int tts_get_max_text_size | ( | tts_h | tts, |
unsigned int * | size | ||
) |
Gets the maximum byte size for text.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [out] size The maximum byte size for text
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state TTS_ERROR_OPERATION_FAILED Operation failure
- Precondition:
- The state should be TTS_STATE_READY.
- See also:
- tts_add_text()
int tts_get_mode | ( | tts_h | tts, |
tts_mode_e * | mode | ||
) |
Gets the TTS mode.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [out] mode The mode
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
- See also:
- tts_set_mode()
int tts_get_private_data | ( | tts_h | tts, |
const char * | key, | ||
char ** | data | ||
) |
Gets the private data from tts engine.
The private data is the information provided by the engine. Using this API, the application can get the private data which corresponds to the key from the engine.
- Since :
- 3.0
- Remarks:
- The data must be released using free() when it is no longer required. If the engine is replaced with the other engine, the key may be ignored.
- Parameters:
-
[in] tts The TTS handle [in] key The field name of private data [out] data The data field of private data
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state TTS_ERROR_ENGINE_NOT_FOUND Engine not found TTS_ERROR_OPERATION_FAILED Operation failure
- Precondition:
- The state should be TTS_STATE_READY.
- See also:
- tts_set_private_data()
int tts_get_speed_range | ( | tts_h | tts, |
int * | min, | ||
int * | normal, | ||
int * | max | ||
) |
Gets the speed range.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [out] min The minimum speed value [out] normal The normal speed value [out] max The maximum speed value
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state TTS_ERROR_OPERATION_FAILED Operation failure
- Precondition:
- The state should be TTS_STATE_CREATED.
- See also:
- tts_add_text()
int tts_get_state | ( | tts_h | tts, |
tts_state_e * | state | ||
) |
Gets the current state of TTS.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [out] state The current state of TTS
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter
- See also:
- tts_play()
- tts_stop()
- tts_pause()
Pauses the currently playing utterance.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state TTS_ERROR_OPERATION_FAILED Operation failure TTS_ERROR_SCREEN_READER_OFF Screen reader is turned off
- Precondition:
- The TTS state should be TTS_STATE_PLAYING.
- Postcondition:
- If this function succeeds, the TTS state will be TTS_STATE_PAUSED.
- See also:
- tts_play()
- tts_stop()
- tts_error_cb()
Starts synthesizing voice from the text and plays the synthesized audio data.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_PERMISSION_DENIED Permission denied TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_OUT_OF_NETWORK Out of network TTS_ERROR_INVALID_STATE Invalid state TTS_ERROR_OPERATION_FAILED Operation failure TTS_ERROR_SCREEN_READER_OFF Screen reader is turned off
- Precondition:
- The current state should be TTS_STATE_READY or TTS_STATE_PAUSED.
- Postcondition:
- If this function succeeds, the TTS state will be TTS_STATE_PLAYING.
int tts_prepare | ( | tts_h | tts | ) |
Connects the daemon asynchronously.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state TTS_ERROR_SCREEN_READER_OFF Screen reader is turned off
- Precondition:
- The state should be TTS_STATE_CREATED.
- Postcondition:
- If this function is successful, the TTS state will be TTS_STATE_READY. If this function is failed, the error callback is called. (e.g. TTS_ERROR_ENGINE_NOT_FOUND)
- See also:
- tts_unprepare()
int tts_repeat | ( | tts_h | tts, |
char ** | text_repeat, | ||
int * | utt_id | ||
) |
Repeats the last added text.
- Since :
- 5.0
- Remarks:
- This function repeats the last added text once again. If there is no previously added text, this function will not work. If the language is changed, the last added text is removed from the service. Before calling this function, please call 'tts_stop()' in order to stop playing the previous one. If this function succeeds, text_repeat must be released with free().
- Parameters:
-
[in] tts The TTS handle [out] text_repeat Texts to be played repeatedly [out] utt_id The utterance ID passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state TTS_ERROR_OPERATION_FAILED Operation failure TTS_ERROR_SCREEN_READER_OFF Screen reader is turned off
- Precondition:
- The state should be TTS_STATE_READY.
- Postcondition:
- If this function succeeds, the TTS state will be TTS_STATE_PLAYING.
- See also:
- tts_add_text()
- tts_stop()
int tts_set_credential | ( | tts_h | tts, |
const char * | credential | ||
) |
Sets the app credential.
Using this API, the application can set a credential. The credential is a key to verify the authorization about using the engine. If the application sets the credential, it will be able to use functions of the engine entirely.
- Since :
- 3.0
- Remarks:
- The necessity of the credential depends on the engine. In case of the engine which is basically embedded in Tizen, the credential is not necessary so far. However, if the user wants to apply the 3rd party's engine, the credential may be necessary. In that case, please follow the policy provided by the corresponding engine.
- Parameters:
-
[in] tts The TTS handle [in] credential The app credential
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Success TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED or TTS_STATE_READY.
- See also:
- tts_play()
int tts_set_default_voice_changed_cb | ( | tts_h | tts, |
tts_default_voice_changed_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to detect default voice change.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
int tts_set_engine_changed_cb | ( | tts_h | tts, |
tts_engine_changed_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to detect the engine change.
- Since :
- 3.0
- Parameters:
-
[in] tts The TTS handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
int tts_set_error_cb | ( | tts_h | tts, |
tts_error_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to detect errors.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
- See also:
- tts_error_cb()
- tts_unset_error_cb()
int tts_set_mode | ( | tts_h | tts, |
tts_mode_e | mode | ||
) |
Sets the TTS mode.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [in] mode The mode
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state TTS_ERROR_OPERATION_FAILED Operation failure
- Precondition:
- The state should be TTS_STATE_CREATED.
- See also:
- tts_get_mode()
int tts_set_private_data | ( | tts_h | tts, |
const char * | key, | ||
const char * | data | ||
) |
Sets the private data to tts engine.
The private data is the setting parameter for applying keys provided by the engine. Using this API, the application can set the private data and use the corresponding key of the engine. For example, if the engine provides 'girl's voice' as a voice type, the application can set the private data as the following. int ret = tts_set_private_data(tts_h, "voice_type", "GIRL");
- Since :
- 3.0
- Remarks:
- If the engine is replaced with the other engine, the key may be ignored.
- Parameters:
-
[in] tts The TTS handle [in] key The field name of private data [in] data The data for set
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state TTS_ERROR_ENGINE_NOT_FOUND Engine not found TTS_ERROR_OPERATION_FAILED Operation failure
- Precondition:
- The state should be TTS_STATE_READY.
- See also:
- tts_get_private_data()
int tts_set_screen_reader_changed_cb | ( | tts_h | tts, |
tts_screen_reader_changed_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to detect the option of screen reader is changed or not.
- Since :
- 6.5
- Remarks:
- If TTS mode is TTS_MODE_SCREEN_READER, you should set the callback to check the option of screen reader is changed or not.
- Parameters:
-
[in] tts The TTS handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
int tts_set_state_changed_cb | ( | tts_h | tts, |
tts_state_changed_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when the TTS state changes.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
int tts_set_utterance_completed_cb | ( | tts_h | tts, |
tts_utterance_completed_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to detect utterance completion.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
int tts_set_utterance_started_cb | ( | tts_h | tts, |
tts_utterance_started_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to detect utterance start.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
Stops playing the utterance and clears the queue.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state TTS_ERROR_OPERATION_FAILED Operation failure TTS_ERROR_SCREEN_READER_OFF Screen reader is turned off
- Precondition:
- The TTS state should be TTS_STATE_READY or TTS_STATE_PLAYING or TTS_STATE_PAUSED.
- Postcondition:
- If this function succeeds, the TTS state will be TTS_STATE_READY. This function will remove all text via tts_add_text() and synthesized sound data.
- See also:
- tts_play()
- tts_pause()
int tts_unprepare | ( | tts_h | tts | ) |
Disconnects the daemon.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_READY.
- Postcondition:
- If this function is called, the TTS state will be TTS_STATE_CREATED.
- See also:
- tts_prepare()
int tts_unset_default_voice_changed_cb | ( | tts_h | tts | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
- See also:
- tts_set_default_voice_changed_cb()
int tts_unset_engine_changed_cb | ( | tts_h | tts | ) |
Unregisters the callback function.
- Since :
- 3.0
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
- See also:
- tts_set_engine_changed_cb()
int tts_unset_error_cb | ( | tts_h | tts | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
- See also:
- tts_set_error_cb()
int tts_unset_screen_reader_changed_cb | ( | tts_h | tts | ) |
Unregisters the callback function to detect the option of screen reader is changed or not.
- Since :
- 6.5
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
- See also:
- tts_set_screen_reader_changed_cb()
int tts_unset_state_changed_cb | ( | tts_h | tts | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
- See also:
- tts_set_state_changed_cb()
int tts_unset_utterance_completed_cb | ( | tts_h | tts | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
- See also:
- tts_set_utterance_completed_cb()
int tts_unset_utterance_started_cb | ( | tts_h | tts | ) |
Unregisters the callback function.
- Since :
- 2.3
- Parameters:
-
[in] tts The TTS handle
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
TTS_ERROR_NONE Successful TTS_ERROR_NOT_SUPPORTED TTS NOT supported TTS_ERROR_INVALID_PARAMETER Invalid parameter TTS_ERROR_INVALID_STATE Invalid state
- Precondition:
- The state should be TTS_STATE_CREATED.
- See also:
- tts_set_utterance_started_cb()