Tizen Native API
6.5
|
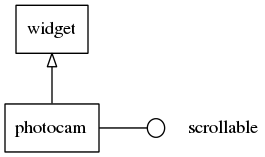
Photocam is a widget meant specifically for displaying high-resolution digital camera photos, giving speedy feedback (fast load), zooming and panning as well as fitting logic, all with low memory footprint. It is entirely focused on jpeg images, and takes advantage of properties of the jpeg format (via Evas loader features in the jpeg loader).
Signals that you can add callbacks for are:
"clicked"
- This is called when a user has clicked the photo without dragging around."press"
- This is called when a user has pressed down on the photo."longpressed"
- This is called when a user has pressed down on the photo for a long time without dragging around."clicked,double"
- This is called when a user has double-clicked the photo."load"
- Photo load begins."loaded"
- This is called when the image file load is complete for the first view (low resolution blurry version)."load,detail"
- Photo detailed data load begins."loaded,detail"
- This is called when the image file load is complete for the detailed image data (full resolution needed)."zoom,start"
- Zoom animation started."zoom,stop"
- Zoom animation stopped."zoom,change"
- Zoom changed when using an auto zoom mode."scroll"
- the content has been scrolled (moved)"scroll,anim,start"
- scrolling animation has started"scroll,anim,stop"
- scrolling animation has stopped"scroll,drag,start"
- dragging the contents around has started"scroll,drag,stop"
- dragging the contents around has stopped"focused"
- When the photocam has received focus. (since 1.8)"unfocused"
- When the photocam has lost focus. (since 1.8)
This widget implements the elm-scrollable-interface interface, so that all (non-deprecated) functions for the base Scroller widget also work for photocam objects.
Some calls on the photocam's API are marked as deprecated, as they just wrap the scrollable widgets counterpart functions. Use the ones we point you to, for each case of deprecation here, instead -- eventually the deprecated ones will be discarded (next major release).
Photocam example shows the API in action.
Functions | |
void | elm_photocam_gesture_enabled_set (Efl_Ui_Image_Zoomable *obj, Eina_Bool gesture) |
Set the gesture state for photocam. | |
Eina_Bool | elm_photocam_gesture_enabled_get (const Efl_Ui_Image_Zoomable *obj) |
Get the gesture state for photocam. | |
Evas_Object * | elm_photocam_add (Evas_Object *parent) |
Add a new Photocam object. | |
void | elm_photocam_image_region_bring_in (Evas_Object *obj, int x, int y, int w, int h) |
Bring in the viewed portion of the image. | |
Evas_Load_Error | elm_photocam_file_set (Evas_Object *obj, const char *file) |
Set the photo file to be shown. | |
const char * | elm_photocam_file_get (const Evas_Object *obj) |
Returns the path of the current image file. | |
void | elm_photocam_image_orient_set (Evas_Object *obj, Evas_Image_Orient orient) |
Set the photocam image orientation. | |
Evas_Image_Orient | elm_photocam_image_orient_get (const Evas_Object *obj) |
Get the photocam image orientation. | |
Evas_Object * | elm_photocam_internal_image_get (const Evas_Object *obj) |
Get the internal low-res image used for photocam. | |
void | elm_photocam_image_region_show (Evas_Object *obj, int x, int y, int w, int h) |
Set the viewed region of the image. | |
void | elm_photocam_image_size_get (const Evas_Object *obj, int *w, int *h) |
Get the current image pixel width and height. | |
Eina_Bool | elm_photocam_paused_get (const Evas_Object *obj) |
Get the paused state for photocam. | |
void | elm_photocam_paused_set (Evas_Object *obj, Eina_Bool paused) |
Set the paused state for photocam. | |
void | elm_photocam_zoom_set (Evas_Object *obj, double zoom) |
Set the zoom level of the photo. | |
double | elm_photocam_zoom_get (const Evas_Object *obj) |
Get the zoom level of the photo. | |
void | elm_photocam_zoom_mode_set (Evas_Object *obj, Elm_Photocam_Zoom_Mode mode) |
Set the zoom mode. | |
Elm_Photocam_Zoom_Mode | elm_photocam_zoom_mode_get (const Evas_Object *obj) |
Get the zoom mode. | |
Typedefs | |
typedef struct _Elm_Photocam_Progress | Elm_Photocam_Progress |
typedef struct _Elm_Photocam_Error | Elm_Photocam_Error |
Typedef Documentation
typedef struct _Elm_Photocam_Error Elm_Photocam_Error |
Structure associated with smart callback 'download,error'
- Since (EFL) :
- 1.8
typedef struct _Elm_Photocam_Progress Elm_Photocam_Progress |
Structure associated with smart callback 'download,progress'.
- Since (EFL) :
- 1.8
Function Documentation
Evas_Object* elm_photocam_add | ( | Evas_Object * | parent | ) |
Add a new Photocam object.
- Parameters:
-
parent The parent object
- Returns:
- The new object or NULL if it cannot be created
- Since :
- 2.3
- Examples:
- photocam_example_01.c.
const char* elm_photocam_file_get | ( | const Evas_Object * | obj | ) |
Returns the path of the current image file.
- Returns:
- Returns the path
- See also:
- elm_photocam_file_set()
- Since :
- 2.3
Evas_Load_Error elm_photocam_file_set | ( | Evas_Object * | obj, |
const char * | file | ||
) |
Set the photo file to be shown.
- Returns:
- The return error (see EVAS_LOAD_ERROR_NONE, EVAS_LOAD_ERROR_GENERIC etc.)
This sets (and shows) the specified file (with a relative or absolute path) and will return a load error (same error that evas_object_image_load_error_get() will return). The image will change and adjust its size at this point and begin a background load process for this photo that at some time in the future will be displayed at the full quality needed.
- Parameters:
-
[in] file The photo file
- Since :
- 2.3
- Examples:
- photocam_example_01.c.
Eina_Bool elm_photocam_gesture_enabled_get | ( | const Efl_Ui_Image_Zoomable * | obj | ) |
Get the gesture state for photocam.
This gets the current gesture state for the photocam object.
- Parameters:
-
[in] obj The object.
- Returns:
- The gesture state.
- Since :
- 2.3
void elm_photocam_gesture_enabled_set | ( | Efl_Ui_Image_Zoomable * | obj, |
Eina_Bool | gesture | ||
) |
Set the gesture state for photocam.
This sets the gesture state to on or off for photocam. The default is off. This will start multi touch zooming.
- Parameters:
-
[in] obj The object. [in] gesture The gesture state.
- Since :
- 2.3
Evas_Image_Orient elm_photocam_image_orient_get | ( | const Evas_Object * | obj | ) |
Get the photocam image orientation.
- Returns:
- The photocam image orientation Evas_Image_Orient. Default is EVAS_IMAGE_ORIENT_NONE.
- Since (EFL) :
- 1.14
- Since :
- 3.0
void elm_photocam_image_orient_set | ( | Evas_Object * | obj, |
Evas_Image_Orient | orient | ||
) |
Set the photocam image orientation.
This function allows to rotate or flip the photocam image.
- Parameters:
-
[in] orient The photocam image orientation Evas_Image_Orient. Default is EVAS_IMAGE_ORIENT_NONE.
- Since (EFL) :
- 1.14
- Since :
- 3.0
- Examples:
- photocam_example_01.c.
void elm_photocam_image_region_bring_in | ( | Evas_Object * | obj, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h | ||
) |
Bring in the viewed portion of the image.
- Parameters:
-
obj The photocam object x X-coordinate of region in image original pixels y Y-coordinate of region in image original pixels w Width of region in image original pixels h Height of region in image original pixels
This shows the region of the image using animation.
- Since :
- 2.3
- Examples:
- photocam_example_01.c.
void elm_photocam_image_region_show | ( | Evas_Object * | obj, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h | ||
) |
Set the viewed region of the image.
This shows the region of the image without using animation.
- Parameters:
-
[in] x X-coordinate of region in image original pixels [in] y Y-coordinate of region in image original pixels [in] w Width of region in image original pixels [in] h Height of region in image original pixels
- Since :
- 2.3
void elm_photocam_image_size_get | ( | const Evas_Object * | obj, |
int * | w, | ||
int * | h | ||
) |
Get the current image pixel width and height.
This gets the current photo pixel width and height (for the original). The size will be returned in the integers w
and h
that are pointed to.
- Parameters:
-
[out] w A pointer to the width return [out] h A pointer to the height return
- Since :
- 2.3
- Examples:
- photocam_example_01.c.
Evas_Object* elm_photocam_internal_image_get | ( | const Evas_Object * | obj | ) |
Get the internal low-res image used for photocam.
This gets the internal image object inside photocam. Do not modify it. It is for inspection only, and hooking callbacks to. Nothing else. It may be deleted at any time as well.
- Returns:
- The internal image object handle or
null
- Since :
- 2.3
Eina_Bool elm_photocam_paused_get | ( | const Evas_Object * | obj | ) |
Get the paused state for photocam.
This gets the current paused state for the photocam object.
- Returns:
- The pause state.
- Since :
- 2.3
void elm_photocam_paused_set | ( | Evas_Object * | obj, |
Eina_Bool | paused | ||
) |
Set the paused state for photocam.
This sets the paused state to on or off for photocam. The default is off. This will stop zooming using animation on zoom level changes and change instantly. This will stop any existing animations that are running.
- Parameters:
-
[in] paused The pause state.
- Since :
- 2.3
double elm_photocam_zoom_get | ( | const Evas_Object * | obj | ) |
Get the zoom level of the photo.
This returns the current zoom level of the photocam object. Note that if you set the fill mode to other than #ELM_PHOTOCAM_ZOOM_MODE_MANUAL (which is the default), the zoom level may be changed at any time by the photocam object itself to account for photo size and photocam viewport size.
- Returns:
- The zoom level to set
- Since :
- 2.3
Elm_Photocam_Zoom_Mode elm_photocam_zoom_mode_get | ( | const Evas_Object * | obj | ) |
Get the zoom mode.
This gets the current zoom mode of the photocam object.
- Returns:
- The zoom mode.
- Since :
- 2.3
void elm_photocam_zoom_mode_set | ( | Evas_Object * | obj, |
Elm_Photocam_Zoom_Mode | mode | ||
) |
Set the zoom mode.
This sets the zoom mode to manual or one of several automatic levels. Manual (ELM_PHOTOCAM_ZOOM_MODE_MANUAL) means that zoom is set manually by elm_photocam_zoom_mode_set and will stay at that level until changed by code or until zoom mode is changed. This is the default mode. The Automatic modes will allow the photocam object to automatically adjust zoom mode based on properties.
#ELM_PHOTOCAM_ZOOM_MODE_AUTO_FIT) will adjust zoom so the photo fits EXACTLY inside the scroll frame with no pixels outside this region. #ELM_PHOTOCAM_ZOOM_MODE_AUTO_FILL will be similar but ensure no pixels within the frame are left unfilled.
- Parameters:
-
[in] mode The zoom mode.
- Since :
- 2.3
- Examples:
- photocam_example_01.c.
void elm_photocam_zoom_set | ( | Evas_Object * | obj, |
double | zoom | ||
) |
Set the zoom level of the photo.
This sets the zoom level. If zoom
is 1, it means no zoom. If it's smaller than 1, it means zoom in. If it's bigger than 1, it means zoom out. For example, zoom
1 will be 1:1 pixel for pixel. zoom
2 will be 2:1 (that is 2x2 photo pixels will display as 1 on-screen pixel) which is a zoom out. 4:1 will be 4x4 photo pixels as 1 screen pixel, and so on. The zoom
parameter must be greater than 0. It is suggested to stick to powers of 2. (1, 2, 4, 8, 16, 32, etc.).
- Parameters:
-
[in] zoom The zoom level to set
- Since :
- 2.3
- Examples:
- photocam_example_01.c.