Tizen Native API
7.0
|
The Radio APIs provide functions for accessing the radio.
Required Header
#include <radio.h>
Overview
The Radio API provides support for using the Radio. The API allows :
- Starting and stopping the radio
- Seeking radio frequency
- Scanning radio signals
- Getting the state of the radio
A radio handle (radio_h) is created by calling the radio_create() function and can be started by using the radio_start() function. It provides functions to start (radio_scan_start()) and stop (radio_scan_stop()) radio signal scanning. The radio frequency seek up and seek down can be done asynchronously by calling the radio_seek_up() and radio_seek_down() functions respectively. It also provides functions to get (radio_get_frequency()) and set (radio_set_frequency()) frequency for the given radio handle.
State Diagram
The radio API is controlled by a state machine. The following diagram shows the life cycle and the states of the Radio.
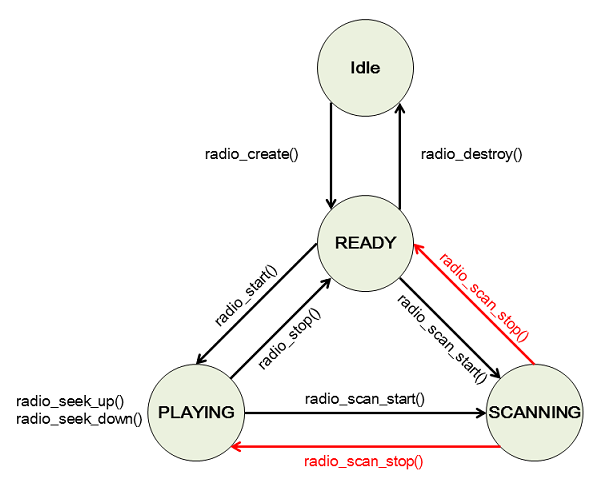
State Transitions
FUNCTION | PRE-STATE | POST-STATE | SYNC TYPE |
---|---|---|---|
radio_create() | NONE | READY | SYNC |
radio_destroy() | READY | NONE | SYNC |
radio_start() | READY | PLAYING | SYNC |
radio_stop() | PLAYING | READY | SYNC |
radio_scan_start() | READY or PLAYING | SCANNING | ASYNC |
radio_scan_stop() | SCANNING | READY or PLAYING | ASYNC |
radio_seek_up() | PLAYING | PLAYING | ASYNC |
radio_seek_down() | PLAYING | PLAYING | ASYNC |
This API also gives notifications for radio's state change events by a callback mechanism.
Callback(Event) Operations
The callback mechanism is used to notify the application about radio events.
REGISTER | UNREGISTER | CALLBACK | DESCRIPTION |
---|---|---|---|
radio_set_scan_completed_cb() | radio_unset_scan_completed_cb() | radio_scan_completed_cb() | This callback is invoked when the scan is completed |
radio_set_interrupted_cb() | radio_unset_interrupted_cb() | radio_interrupted_cb() | This callback is used to notify when the radio is interrupted |
Related Features
This API is related with the following features:
- http://tizen.org/feature/fmradio
It is recommended to design feature related codes in your application for reliability.
You can check if a device supports the related features for this API by using System Information, thereby controlling the procedure of your application.
To ensure your application is only running on the device with specific features, please define the features in your manifest file using the manifest editor in the SDK.
More details on featuring your application can be found from Feature Element.
Functions | |
int | radio_create (radio_h *radio) |
Creates a radio handle. | |
int | radio_destroy (radio_h radio) |
Destroys the radio handle and releases all its resources. | |
int | radio_get_state (radio_h radio, radio_state_e *state) |
Gets the radio's current state. | |
int | radio_start (radio_h radio) |
Starts playing the radio. | |
int | radio_stop (radio_h radio) |
Stops playing the radio. | |
int | radio_seek_up (radio_h radio, radio_seek_completed_cb callback, void *user_data) |
Seeks up the effective frequency of the radio, asynchronously. | |
int | radio_seek_down (radio_h radio, radio_seek_completed_cb callback, void *user_data) |
Seeks down the effective frequency of the radio, asynchronously. | |
int | radio_set_frequency (radio_h radio, int frequency) |
Sets the radio frequency. | |
int | radio_get_frequency (radio_h radio, int *frequency) |
Gets the current frequency of the radio. | |
int | radio_get_signal_strength (radio_h radio, int *strength) |
Gets the current signal strength of the radio. | |
int | radio_scan_start (radio_h radio, radio_scan_updated_cb callback, void *user_data) |
Starts scanning radio signals, asynchronously. | |
int | radio_scan_stop (radio_h radio, radio_scan_stopped_cb callback, void *user_data) |
Stops scanning radio signals, asynchronously. | |
int | radio_set_mute (radio_h radio, bool muted) |
Sets the radio's mute status. | |
int | radio_is_muted (radio_h radio, bool *muted) |
Gets the radio's mute status. | |
int | radio_set_scan_completed_cb (radio_h radio, radio_scan_completed_cb callback, void *user_data) |
Sets a callback function to be invoked when the scan finishes. | |
int | radio_unset_scan_completed_cb (radio_h radio) |
Unsets the scan completed callback function. | |
int | radio_set_interrupted_cb (radio_h radio, radio_interrupted_cb callback, void *user_data) |
Sets a callback function to be invoked when the radio is interrupted. | |
int | radio_unset_interrupted_cb (radio_h radio) |
Unsets the interrupted callback function. | |
int | radio_get_frequency_range (radio_h radio, int *min_freq, int *max_freq) |
Gets the min, max frequency of the region. | |
int | radio_get_channel_spacing (radio_h radio, int *channel_spacing) |
Gets channel spacing. | |
int | radio_set_volume (radio_h radio, float volume) |
Sets the radio's volume. | |
int | radio_get_volume (radio_h radio, float *volume) |
Gets the radio's current volume. | |
Typedefs | |
typedef struct radio_s * | radio_h |
Radio type handle. | |
typedef void(* | radio_scan_updated_cb )(int frequency, void *user_data) |
Called when the scan information is updated. | |
typedef void(* | radio_scan_stopped_cb )(void *user_data) |
Called when the radio scan is stopped. | |
typedef void(* | radio_scan_completed_cb )(void *user_data) |
Called when the radio scan is completed. | |
typedef void(* | radio_seek_completed_cb )(int frequency, void *user_data) |
Called when the radio seek is completed. | |
typedef void(* | radio_interrupted_cb )(radio_interrupted_code_e code, void *user_data) |
Called when the radio is interrupted. |
Typedef Documentation
typedef struct radio_s* radio_h |
Radio type handle.
- Since :
- 2.3
typedef void(* radio_interrupted_cb)(radio_interrupted_code_e code, void *user_data) |
Called when the radio is interrupted.
- Since :
- 2.3
- Parameters:
-
[in] code The interrupted error code [in] user_data The user data passed from the callback registration function
typedef void(* radio_scan_completed_cb)(void *user_data) |
Called when the radio scan is completed.
- Since :
- 2.3
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- Precondition:
- It will be invoked when the scan is completed by registering this callback using radio_set_scan_completed_cb().
typedef void(* radio_scan_stopped_cb)(void *user_data) |
Called when the radio scan is stopped.
- Since :
- 2.3
- Parameters:
-
[in] user_data The user data passed from the callback registration function
- Precondition:
- It will be invoked when the scan is stopped by radio_scan_stop().
- See also:
- radio_scan_stop()
typedef void(* radio_scan_updated_cb)(int frequency, void *user_data) |
Called when the scan information is updated.
- Since :
- 2.3
- Parameters:
-
[in] frequency The tuned radio frequency [87500 ~ 108000] (kHz) [in] user_data The user data passed from the callback registration function
- Precondition:
- It will be invoked by radio_scan_start().
- See also:
- radio_scan_start()
typedef void(* radio_seek_completed_cb)(int frequency, void *user_data) |
Called when the radio seek is completed.
- Since :
- 2.3
- Parameters:
-
[in] frequency The current frequency [87500 ~ 108000] (kHz) [in] user_data The user data passed from the callback registration function
- Precondition:
- It will be invoked when the radio seek is completed by registering this callback using radio_seek_up() or radio_seek_down().
- See also:
- radio_seek_up()
- radio_seek_down()
Enumeration Type Documentation
enum radio_error_e |
Enumeration of error codes for the radio.
- Since :
- 2.3
- Enumerator:
enum radio_state_e |
Function Documentation
int radio_create | ( | radio_h * | radio | ) |
Creates a radio handle.
- Since :
- 2.3
- Remarks:
- You must release radio using radio_destroy().
- Parameters:
-
[out] radio A new handle to radio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_OUT_OF_MEMORY Out of memory RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
- See also:
- radio_destroy()
int radio_destroy | ( | radio_h | radio | ) |
Destroys the radio handle and releases all its resources.
- Since :
- 2.3
- Remarks:
- To completely shutdown the radio operation, call this function with a valid radio handle.
- Parameters:
-
[in] radio The handle to radio to be destroyed
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
- See also:
- radio_create()
int radio_get_channel_spacing | ( | radio_h | radio, |
int * | channel_spacing | ||
) |
Gets channel spacing.
- Since :
- 2.4
- Parameters:
-
[in] radio The handle to radio [out] channel_spacing The channel spacing value
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
int radio_get_frequency | ( | radio_h | radio, |
int * | frequency | ||
) |
Gets the current frequency of the radio.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio [out] frequency The current frequency [87500 ~ 108000] (kHz)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
- See also:
- radio_set_frequency()
int radio_get_frequency_range | ( | radio_h | radio, |
int * | min_freq, | ||
int * | max_freq | ||
) |
Gets the min, max frequency of the region.
- Since :
- 2.4
- Parameters:
-
[in] radio The handle to radio [out] min_freq The min frequency [87500 ~ 108000] (kHz) [out] max_freq The max frequency [87500 ~ 108000] (kHz)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
int radio_get_signal_strength | ( | radio_h | radio, |
int * | strength | ||
) |
Gets the current signal strength of the radio.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio [out] strength The current signal strength [-128 ~ 128] (dBm)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
int radio_get_state | ( | radio_h | radio, |
radio_state_e * | state | ||
) |
Gets the radio's current state.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio [out] state The current state of the radio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_NOT_SUPPORTED Not supported
int radio_get_volume | ( | radio_h | radio, |
float * | volume | ||
) |
Gets the radio's current volume.
The range of volume is from 0
to 1.0
, inclusive (1.0 = 100%). This function gets the radio volume, not the system volume. To get the system volume, use the Sound Manager API.
- Since :
- 3.0
- Parameters:
-
[in] radio The handle to radio [out] volume The current volume [0.0 ~ 1.0](1.0 = 100%)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
- See also:
- radio_set_volume()
int radio_is_muted | ( | radio_h | radio, |
bool * | muted | ||
) |
Gets the radio's mute status.
If the mute status is true
, no sounds are played. If false
, sounds are played.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio [out] muted The current mute status: ( true
= mute,false
= not muted)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
- See also:
- radio_set_mute()
int radio_scan_start | ( | radio_h | radio, |
radio_scan_updated_cb | callback, | ||
void * | user_data | ||
) |
Starts scanning radio signals, asynchronously.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_INVALID_STATE Invalid radio state RADIO_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The radio state must be set to RADIO_STATE_READY or RADIO_STATE_PLAYING.
- Postcondition:
- The radio state will be RADIO_STATE_SCANNING during a search. After the scan is completed, the radio state will be set back to the state from before the function call(RADIO_STATE_READY or RADIO_STATE_PLAYING).
- It invokes radio_scan_updated_cb() when the scan information updates.
- It invokes radio_scan_completed_cb() when the scan completes, if you set a callback with radio_set_scan_completed_cb().
int radio_scan_stop | ( | radio_h | radio, |
radio_scan_stopped_cb | callback, | ||
void * | user_data | ||
) |
Stops scanning radio signals, asynchronously.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid state RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_INVALID_STATE Invalid radio state RADIO_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The radio state must be set to RADIO_STATE_SCANNING by calling radio_scan_start().
- Postcondition:
- It invokes radio_scan_stopped_cb() when the scan stops.
- After the scan stopped, the radio state will be set back to the state from before the radio_scan_start() function call(RADIO_STATE_READY or RADIO_STATE_PLAYING).
- See also:
- radio_scan_start()
int radio_seek_down | ( | radio_h | radio, |
radio_seek_completed_cb | callback, | ||
void * | user_data | ||
) |
Seeks down the effective frequency of the radio, asynchronously.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_INVALID_STATE Invalid radio state RADIO_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The radio state must be set to RADIO_STATE_PLAYING by calling radio_start().
- Postcondition:
- It invokes radio_seek_completed_cb() when the seek completes.
- See also:
- radio_seek_up()
int radio_seek_up | ( | radio_h | radio, |
radio_seek_completed_cb | callback, | ||
void * | user_data | ||
) |
Seeks up the effective frequency of the radio, asynchronously.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_INVALID_STATE Invalid radio state RADIO_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The radio state must be set to RADIO_STATE_PLAYING by calling radio_start().
- Postcondition:
- It invokes radio_seek_completed_cb() when the seek completes.
- See also:
- radio_seek_down()
int radio_set_frequency | ( | radio_h | radio, |
int | frequency | ||
) |
Sets the radio frequency.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio [in] frequency The frequency to set [87500 ~ 108000] (kHz)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
- See also:
- radio_get_frequency()
int radio_set_interrupted_cb | ( | radio_h | radio, |
radio_interrupted_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to be invoked when the radio is interrupted.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
- Postcondition:
- radio_interrupted_cb() will be invoked.
int radio_set_mute | ( | radio_h | radio, |
bool | muted | ||
) |
Sets the radio's mute status.
If the mute status is true
, no sounds will be played. If false
, sounds will be played. Until this function is called, by default the radio is not muted.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio [in] muted The new mute status: ( true
= mute,false
= not muted)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
- See also:
- radio_is_muted()
int radio_set_scan_completed_cb | ( | radio_h | radio, |
radio_scan_completed_cb | callback, | ||
void * | user_data | ||
) |
Sets a callback function to be invoked when the scan finishes.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio [in] callback The callback function to register [in] user_data The user data to be passed to the callback function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
- Postcondition:
- radio_scan_completed_cb() will be invoked.
int radio_set_volume | ( | radio_h | radio, |
float | volume | ||
) |
Sets the radio's volume.
Setting this volume adjusts the radio's instance volume, not the system volume. The valid range is from 0 to 1.0, inclusive (1.0 = 100%). Default value is 1.0. To change system volume, use the Sound Manager API. Finally, it does not support to set other value into each channel currently.
- Since :
- 3.0
- Parameters:
-
[in] radio The handle to radio [in] volume The volume to set [0.0 ~ 1.0](1.0 = 100%)
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
- See also:
- radio_get_volume()
int radio_start | ( | radio_h | radio | ) |
Starts playing the radio.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_STATE Invalid radio state RADIO_ERROR_SOUND_POLICY Sound policy error RADIO_ERROR_NOT_SUPPORTED Not supported RADIO_ERROR_NO_ANTENNA No Antenna error
- Precondition:
- The radio state must be set to RADIO_STATE_READY by calling radio_create().
- Postcondition:
- The radio state will be RADIO_STATE_PLAYING.
- See also:
- radio_stop()
int radio_stop | ( | radio_h | radio | ) |
Stops playing the radio.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid state RADIO_ERROR_INVALID_STATE Invalid radio state RADIO_ERROR_NOT_SUPPORTED Not supported
- Precondition:
- The radio state must be set to RADIO_STATE_PLAYING by calling radio_start().
- Postcondition:
- The radio state will be RADIO_STATE_READY.
- See also:
- radio_start()
- radio_scan_start()
int radio_unset_interrupted_cb | ( | radio_h | radio | ) |
Unsets the interrupted callback function.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
- See also:
- radio_set_interrupted_cb()
int radio_unset_scan_completed_cb | ( | radio_h | radio | ) |
Unsets the scan completed callback function.
- Since :
- 2.3
- Parameters:
-
[in] radio The handle to radio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
RADIO_ERROR_NONE Successful RADIO_ERROR_INVALID_PARAMETER Invalid parameter RADIO_ERROR_INVALID_OPERATION Invalid operation RADIO_ERROR_NOT_SUPPORTED Not supported
- See also:
- radio_set_scan_completed_cb()