Tizen Native API
7.0
|
The Screen Mirroring API provides functions for screen mirroring as sink.
Required Header
#include <scmirroring_sink.h>
Overview
The Screen Mirroring API allows you to implement screen mirroring application as sink. It gives the ability to connect to and disconnect from a screen mirroring source, and start, pause, and resume the screen mirroring sink, set the resolution or display, register state change callback function.
State Diagram
The following diagram shows the life cycle and states of the screen mirroring sink.
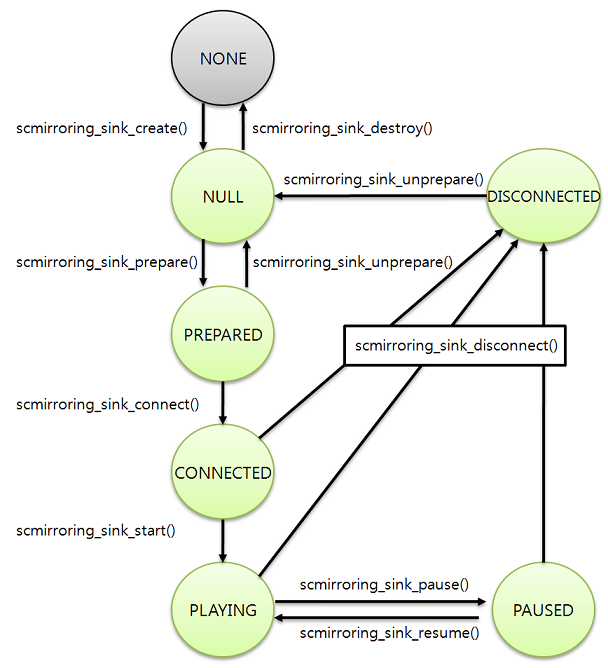
State Transitions
FUNCTION | PRE-STATE | POST-STATE | SYNC TYPE |
---|---|---|---|
scmirroring_sink_create() | NONE | NULL | SYNC |
scmirroring_sink_destroy() | NULL | NONE | SYNC |
scmirroring_sink_prepare() | NULL | PREPARED | SYNC |
scmirroring_sink_unprepare() | PREPARED, DISCONNECTED | NULL | SYNC |
scmirroring_sink_connect() | PREPARED | CONNECTED | ASYNC |
scmirroring_sink_start() | CONNECTED | PLAYING | ASYNC |
scmirroring_sink_disconnect() | CONNECTED, PAUSED or PLAYING | DISCONNECTED | SYNC |
scmirroring_sink_pause() | PLAYING | PAUSED | ASYNC |
scmirroring_sink_resume() | PAUSED | PLAYING | ASYNC |
State Dependent Function Calls
The following table shows state-dependent function calls. It is forbidden to call the functions listed below in wrong state. Violation of this rule may result in unpredictable behavior.
Asynchronous Operations
All functions that change the state are synchronous except scmirroring_sink_connect(), scmirroring_sink_start(), scmirroring_sink_pause(), and scmirroring_sink_resume(). Thus the result is passed to the application via the callback mechanism.
Callback(Event) Operations
REGISTER | UNREGISTER | CALLBACK | DESCRIPTION |
---|---|---|---|
scmirroring_sink_set_state_changed_cb() | scmirroring_sink_unset_state_changed_cb() | scmirroring_sink_state_cb() | This callback is called for state and error of screen mirroring. |
Related Features
This API is related with the following feature:
It is recommended to design feature related codes in your application for reliability. You can check if a device supports the related features for this API by using System Information, thereby controlling the procedure of your application. To ensure your application is only running on the device with specific features, please define the features in your manifest file using the manifest editor in the SDK. More details on featuring your application can be found from Feature Element.
Functions | |
int | scmirroring_sink_create (scmirroring_sink_h *scmirroring_sink) |
Creates a new screen mirroring sink handle. | |
int | scmirroring_sink_set_state_changed_cb (scmirroring_sink_h scmirroring_sink, scmirroring_sink_state_cb callback, void *user_data) |
Registers a callback function to be called when state change happens. | |
int | scmirroring_sink_set_ip_and_port (scmirroring_sink_h scmirroring_sink, const char *ip, const char *port) |
Sets server IP and port. | |
int | scmirroring_sink_set_display (scmirroring_sink_h scmirroring_sink, scmirroring_display_type_e type, void *display_surface) |
Pass window handle created by application and surface type(x11/evas). | |
int | scmirroring_sink_set_resolution (scmirroring_sink_h scmirroring_sink, int resolution) |
Sets resolutions of screen mirroring sink. | |
int | scmirroring_sink_prepare (scmirroring_sink_h scmirroring_sink) |
Prepares the screen mirroring sink handle and allocates specific resources. | |
int | scmirroring_sink_connect (scmirroring_sink_h scmirroring_sink) |
Creates connection and prepare for receiving data from SCMIRRORING source. | |
int | scmirroring_sink_start (scmirroring_sink_h scmirroring_sink) |
Starts receiving data from the SCMIRRORING source and display it(mirror). | |
int | scmirroring_sink_pause (scmirroring_sink_h scmirroring_sink) |
Pauses receiving data from the SCMIRRORING source. | |
int | scmirroring_sink_resume (scmirroring_sink_h scmirroring_sink) |
Resumes receiving data from the SCMIRRORING source. | |
int | scmirroring_sink_disconnect (scmirroring_sink_h scmirroring_sink) |
Disconnects and stops receiving data from the SCMIRRORING source. | |
int | scmirroring_sink_unprepare (scmirroring_sink_h scmirroring_sink) |
Unprepares screen mirroring. | |
int | scmirroring_sink_unset_state_changed_cb (scmirroring_sink_h scmirroring_sink) |
Unregisters the callback function user registered. | |
int | scmirroring_sink_destroy (scmirroring_sink_h scmirroring_sink) |
Destroys screen mirroring sink handle. | |
int | scmirroring_sink_get_negotiated_video_codec (scmirroring_sink_h *scmirroring_sink, scmirroring_video_codec_e *codec) |
Gets negotiated video codec of screen mirroring sink. | |
int | scmirroring_sink_get_negotiated_video_resolution (scmirroring_sink_h *scmirroring_sink, int *width, int *height) |
Gets negotiated video resolution of screen mirroring sink. | |
int | scmirroring_sink_get_negotiated_video_frame_rate (scmirroring_sink_h *scmirroring_sink, int *frame_rate) |
Gets negotiated frame rate of screen mirroring sink. | |
int | scmirroring_sink_get_negotiated_audio_codec (scmirroring_sink_h *scmirroring_sink, scmirroring_audio_codec_e *codec) |
Gets negotiated audio codec of screen mirroring sink. | |
int | scmirroring_sink_get_negotiated_audio_channel (scmirroring_sink_h *scmirroring_sink, int *channel) |
Gets negotiated audio channel of screen mirroring sink. | |
int | scmirroring_sink_get_negotiated_audio_sample_rate (scmirroring_sink_h *scmirroring_sink, int *sample_rate) |
Gets negotiated audio sample rate of screen mirroring sink. | |
int | scmirroring_sink_get_negotiated_audio_bitwidth (scmirroring_sink_h *scmirroring_sink, int *bitwidth) |
Gets negotiated audio bitwidth of screen mirroring sink. | |
int | scmirroring_sink_get_current_state (scmirroring_sink_h scmirroring_sink, scmirroring_sink_state_e *state) |
Gets the current state of screen mirroring sink. | |
Typedefs | |
typedef void * | scmirroring_sink_h |
The handle to the screen mirroring sink. | |
typedef void(* | scmirroring_sink_state_cb )(scmirroring_error_e error, scmirroring_sink_state_e state, void *user_data) |
Called when each status is changed. |
Typedef Documentation
typedef void* scmirroring_sink_h |
The handle to the screen mirroring sink.
- Since :
- 2.4
typedef void(* scmirroring_sink_state_cb)(scmirroring_error_e error, scmirroring_sink_state_e state, void *user_data) |
Called when each status is changed.
- Since :
- 2.4
This callback is called for state and error of screen mirroring sink
- Parameters:
-
[in] error The error code [in] state The screen mirroring sink state [in] user_data The user data passed from the scmirroring_sink_set_state_cb() function
- Precondition:
- scmirroring_sink_create()
- See also:
- scmirroring_sink_create()
Enumeration Type Documentation
enum scmirroring_error_e |
Enumeration for screen mirroring error.
- Since :
- 2.4
- Enumerator:
Ability to send to multisink.
- Since :
- 3.0
Enumeration for screen mirroring resolution.
- Since :
- 2.4
- Enumerator:
Enumeration for screen mirroring sink state.
- Since :
- 2.4
- Enumerator:
Function Documentation
int scmirroring_sink_connect | ( | scmirroring_sink_h | scmirroring_sink | ) |
Creates connection and prepare for receiving data from SCMIRRORING source.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/internet
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_OUT_OF_MEMORY Out of memory SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_PERMISSION_DENIED Permission denied SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- Register user callback by calling scmirroring_sink_set_state_changed_cb().
- Call scmirroring_sink_prepare()
- The screen mirroring state should be SCMIRRORING_SINK_STATE_PREPARED
- Postcondition:
- The screen mirroring state will be SCMIRRORING_SINK_STATE_CONNECTED
int scmirroring_sink_create | ( | scmirroring_sink_h * | scmirroring_sink | ) |
Creates a new screen mirroring sink handle.
- Since :
- 2.4
- Remarks:
- You must release scmirroring_sink using scmirroring_sink_destroy().
- Parameters:
-
[out] scmirroring_sink A newly returned handle to the screen mirroring sink
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_OUT_OF_MEMORY Out of memory SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Postcondition:
- The screen mirroring state will be SCMIRRORING_SINK_STATE_NULL
- See also:
- scmirroring_sink_destroy()
int scmirroring_sink_destroy | ( | scmirroring_sink_h | scmirroring_sink | ) |
Destroys screen mirroring sink handle.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_PERMISSION_DENIED Permission denied SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- The screen mirroring state should be SCMIRRORING_SINK_STATE_NULL
- See also:
- scmirroring_sink_create()
int scmirroring_sink_disconnect | ( | scmirroring_sink_h | scmirroring_sink | ) |
Disconnects and stops receiving data from the SCMIRRORING source.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/internet
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_OUT_OF_MEMORY Out of memory SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_PERMISSION_DENIED Permission denied SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- The screen mirroring state should be SCMIRRORING_SINK_STATE_CONNECTED or SCMIRRORING_SINK_STATE_PLAYING or SCMIRRORING_SINK_STATE_PAUSED
- Postcondition:
- The screen mirroring state will be SCMIRRORING_SINK_STATE_DISCONNECTED
int scmirroring_sink_get_current_state | ( | scmirroring_sink_h | scmirroring_sink, |
scmirroring_sink_state_e * | state | ||
) |
Gets the current state of screen mirroring sink.
The current state of screen mirroring sink is changed by calling CAPIs. And it provides the state of screen mirroring sink the time this api is called.
- Since :
- 5.0
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink [out] state The current state of screen mirroring sink
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
int scmirroring_sink_get_negotiated_audio_bitwidth | ( | scmirroring_sink_h * | scmirroring_sink, |
int * | bitwidth | ||
) |
Gets negotiated audio bitwidth of screen mirroring sink.
The audio bitwidth is negotiated by screen mirroring source.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink [out] bitwidth Bitwidth of audio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- Register user callback by calling scmirroring_sink_set_state_changed_cb().
- Call scmirroring_sink_prepare()
- Call scmirroring_sink_connect()
- The screen mirroring state must be SCMIRRORING_SINK_STATE_CONNECTED or SCMIRRORING_SINK_STATE_PLAYING
int scmirroring_sink_get_negotiated_audio_channel | ( | scmirroring_sink_h * | scmirroring_sink, |
int * | channel | ||
) |
Gets negotiated audio channel of screen mirroring sink.
The audio channel is negotiated by screen mirroring source.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink [out] channel Channel of audio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- Register user callback by calling scmirroring_sink_set_state_changed_cb().
- Call scmirroring_sink_prepare()
- Call scmirroring_sink_connect()
- The screen mirroring state must be SCMIRRORING_SINK_STATE_CONNECTED or SCMIRRORING_SINK_STATE_PLAYING
int scmirroring_sink_get_negotiated_audio_codec | ( | scmirroring_sink_h * | scmirroring_sink, |
scmirroring_audio_codec_e * | codec | ||
) |
Gets negotiated audio codec of screen mirroring sink.
The audio codec is negotiated by screen mirroring source.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink [out] codec Codec of audio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- Register user callback by calling scmirroring_sink_set_state_changed_cb().
- Call scmirroring_sink_prepare()
- Call scmirroring_sink_connect()
- The screen mirroring state must be SCMIRRORING_SINK_STATE_CONNECTED or SCMIRRORING_SINK_STATE_PLAYING
int scmirroring_sink_get_negotiated_audio_sample_rate | ( | scmirroring_sink_h * | scmirroring_sink, |
int * | sample_rate | ||
) |
Gets negotiated audio sample rate of screen mirroring sink.
The audio sample rate is negotiated by screen mirroring source.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink [out] sample_rate Sample rate of audio
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- Register user callback by calling scmirroring_sink_set_state_changed_cb().
- Call scmirroring_sink_prepare()
- Call scmirroring_sink_connect()
- The screen mirroring state must be SCMIRRORING_SINK_STATE_CONNECTED or SCMIRRORING_SINK_STATE_PLAYING
int scmirroring_sink_get_negotiated_video_codec | ( | scmirroring_sink_h * | scmirroring_sink, |
scmirroring_video_codec_e * | codec | ||
) |
Gets negotiated video codec of screen mirroring sink.
The video codec is negotiated by screen mirroring source.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink [out] codec Codec of video
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- Register user callback by calling scmirroring_sink_set_state_changed_cb().
- Call scmirroring_sink_prepare()
- Call scmirroring_sink_connect()
- The screen mirroring state must be SCMIRRORING_SINK_STATE_CONNECTED or SCMIRRORING_SINK_STATE_PLAYING
int scmirroring_sink_get_negotiated_video_frame_rate | ( | scmirroring_sink_h * | scmirroring_sink, |
int * | frame_rate | ||
) |
Gets negotiated frame rate of screen mirroring sink.
The video frame rate is negotiated by screen mirroring source.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink [out] frame_rate Frame rate of video
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- Register user callback by calling scmirroring_sink_set_state_changed_cb().
- Call scmirroring_sink_prepare()
- Call scmirroring_sink_connect()
- The screen mirroring state must be SCMIRRORING_SINK_STATE_CONNECTED or SCMIRRORING_SINK_STATE_PLAYING
int scmirroring_sink_get_negotiated_video_resolution | ( | scmirroring_sink_h * | scmirroring_sink, |
int * | width, | ||
int * | height | ||
) |
Gets negotiated video resolution of screen mirroring sink.
The video resolution is negotiated by screen mirroring source.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink [out] width Width of video [out] height Height of video
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- Register user callback by calling scmirroring_sink_set_state_changed_cb().
- Call scmirroring_sink_prepare()
- Call scmirroring_sink_connect()
- The screen mirroring state must be SCMIRRORING_SINK_STATE_CONNECTED or SCMIRRORING_SINK_STATE_PLAYING
int scmirroring_sink_pause | ( | scmirroring_sink_h | scmirroring_sink | ) |
Pauses receiving data from the SCMIRRORING source.
This function pauses receiving data from the SCMIRRORING source, which means it sends RTSP PAUSE message to source.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/internet
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_OUT_OF_MEMORY Out of memory SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_PERMISSION_DENIED Permission denied SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- The screen mirroring state should be SCMIRRORING_SINK_STATE_PLAYING
- Postcondition:
- The screen mirroring state will be SCMIRRORING_SINK_STATE_PAUSED
int scmirroring_sink_prepare | ( | scmirroring_sink_h | scmirroring_sink | ) |
Prepares the screen mirroring sink handle and allocates specific resources.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_OUT_OF_MEMORY Out of memory SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- Register user callback by calling scmirroring_sink_set_state_changed_cb().
- The screen mirroring state should be SCMIRRORING_SINK_STATE_NULL
- Postcondition:
- The screen mirroring state will be SCMIRRORING_SINK_STATE_PREPARED
int scmirroring_sink_resume | ( | scmirroring_sink_h | scmirroring_sink | ) |
Resumes receiving data from the SCMIRRORING source.
This function pauses receiving data from the SCMIRRORING source, which means it sends RTSP PLAY message to source.
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/internet
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_OUT_OF_MEMORY Out of memory SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_PERMISSION_DENIED Permission denied SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- The screen mirroring state should be SCMIRRORING_SINK_STATE_PAUSED
- Postcondition:
- The screen mirroring state will be SCMIRRORING_SINK_STATE_PLAYING
- See also:
- scmirroring_sink_pause()
int scmirroring_sink_set_display | ( | scmirroring_sink_h | scmirroring_sink, |
scmirroring_display_type_e | type, | ||
void * | display_surface | ||
) |
Pass window handle created by application and surface type(x11/evas).
This function will use handle created by the application to set the overlay & display on the surface passed by the application
- Since :
- 2.4
- Remarks:
- This function must be called in main thread of application. Otherwise, it will return SCMIRRORING_ERROR_INVALID_OPERATION by internal restriction. (since tizen 5.0)
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink [in] type Surface type(x11/evas) [in] display_surface The display_surface created by application to force sink to display content over it
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_OUT_OF_MEMORY Out of memory SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_PERMISSION_DENIED Permission denied SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- See also:
- scmirroring_sink_create()
int scmirroring_sink_set_ip_and_port | ( | scmirroring_sink_h | scmirroring_sink, |
const char * | ip, | ||
const char * | port | ||
) |
Sets server IP and port.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink [in] ip The server IP address to connect to [in] port The server port to connect to
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_OUT_OF_MEMORY Out of memory SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_PERMISSION_DENIED Permission denied SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- See also:
- scmirroring_sink_create()
int scmirroring_sink_set_resolution | ( | scmirroring_sink_h | scmirroring_sink, |
int | resolution | ||
) |
Sets resolutions of screen mirroring sink.
This function sets resolutions of screen mirroring sink using scmirroring_resolution_e as following. (ex. SCMIRRORING_RESOLUTION_1920x1080_P30 | SCMIRRORING_RESOLUTION_1280x720_P30) Use it only when you want to set specific resolutions but if screen mirroring source does not support the resolutions which you set, the screen mirroring sink will be disconnected.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink [in] resolution Resolution of screen mirroring sink
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_OUT_OF_MEMORY Out of memory SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_PERMISSION_DENIED Permission denied SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- See also:
- scmirroring_sink_create()
int scmirroring_sink_set_state_changed_cb | ( | scmirroring_sink_h | scmirroring_sink, |
scmirroring_sink_state_cb | callback, | ||
void * | user_data | ||
) |
Registers a callback function to be called when state change happens.
This function registers user callback and this callback is called when each status is changed.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink [in] callback The callback function to invoke [in] user_data The user data passed to the callback registration function
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_OUT_OF_MEMORY Out of memory SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_PERMISSION_DENIED Permission denied SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- See also:
- scmirroring_sink_create()
int scmirroring_sink_start | ( | scmirroring_sink_h | scmirroring_sink | ) |
Starts receiving data from the SCMIRRORING source and display it(mirror).
- Since :
- 2.4
- Privilege Level:
- public
- Privilege:
- http://tizen.org/privilege/internet
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_OUT_OF_MEMORY Out of memory SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_PERMISSION_DENIED Permission denied SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- Register user callback by calling scmirroring_sink_set_state_changed_cb().
- Call scmirroring_sink_prepare()
- Call scmirroring_sink_connect()
- The screen mirroring state should be SCMIRRORING_SINK_STATE_CONNECTED
- Postcondition:
- The screen mirroring state will be SCMIRRORING_SINK_STATE_PLAYING
int scmirroring_sink_unprepare | ( | scmirroring_sink_h | scmirroring_sink | ) |
Unprepares screen mirroring.
This function unprepares screen mirroring, which closes specific resources.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_OUT_OF_MEMORY Out of memory SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- Register user callback by calling scmirroring_sink_set_state_changed_cb().
- Call scmirroring_sink_prepare()
- Postcondition:
- The screen mirroring state will be SCMIRRORING_SINK_STATE_NULL
int scmirroring_sink_unset_state_changed_cb | ( | scmirroring_sink_h | scmirroring_sink | ) |
Unregisters the callback function user registered.
- Since :
- 2.4
- Parameters:
-
[in] scmirroring_sink The handle to the screen mirroring sink
- Returns:
0
on success, otherwise a negative error value
- Return values:
-
SCMIRRORING_ERROR_NONE Successful SCMIRRORING_ERROR_INVALID_PARAMETER Invalid parameter SCMIRRORING_ERROR_INVALID_OPERATION Invalid operation SCMIRRORING_ERROR_PERMISSION_DENIED Permission denied SCMIRRORING_ERROR_NOT_SUPPORTED Not supported SCMIRRORING_ERROR_UNKNOWN Unknown Error
- Precondition:
- Create a screen mirroring sink handle by calling scmirroring_sink_create().
- Register user callback by calling scmirroring_sink_set_state_changed_cb().