Tizen Native API
7.0
|
Objects generate events when they are moved, resized, when their visibility change, when they are deleted and so on. These methods allow one to be notified about and to handle such events.
Objects also generate events on input (keyboard and mouse), if they accept them (are visible, focused, etc).
For each of those events, Evas provides a way for one to register callback functions to be issued just after they happen.
The following figure illustrates some Evas (event) callbacks:
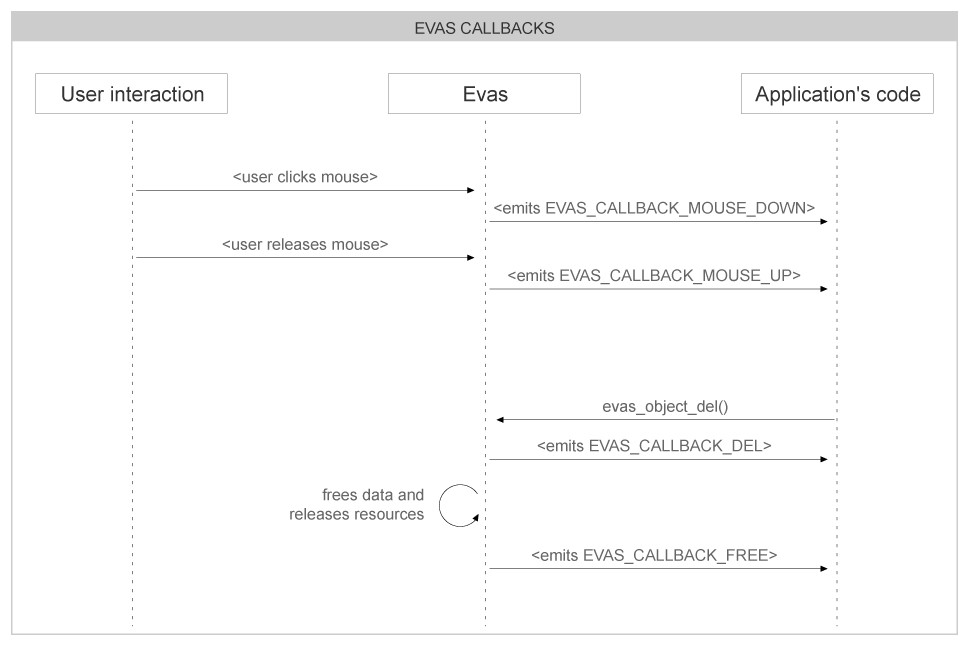
These events have their values in the #Evas_Callback_Type enumeration, which has also ones happening on the canvas level (see Canvas Events ).
Examples on this group of functions can be found here and here.
Function Documentation
void evas_object_event_callback_add | ( | Evas_Object * | obj, |
Evas_Callback_Type | type, | ||
Evas_Object_Event_Cb | func, | ||
const void * | data | ||
) |
Add (register) a callback function to a given Evas object event.
- Parameters:
-
obj Object to attach a callback to type The type of event that will trigger the callback func The function to be called when the event is triggered data The data pointer to be passed to func
This function adds a function callback to an object when the event of type type
occurs on object obj
. The function is func
.
In the event of a memory allocation error during addition of the callback to the object, evas_alloc_error() should be used to determine the nature of the error, if any, and the program should sensibly try and recover.
A callback function must have the ::Evas_Object_Event_Cb prototype definition. The first parameter (data
) in this definition will have the same value passed to evas_object_event_callback_add() as the data
parameter, at runtime. The second parameter e
is the canvas pointer on which the event occurred. The third parameter is a pointer to the object on which event occurred. Finally, the fourth parameter event_info
is a pointer to a data structure that may or may not be passed to the callback, depending on the event type that triggered the callback. This is so because some events don't carry extra context with them, but others do.
The event type type
to trigger the function may be one of #EVAS_CALLBACK_MOUSE_IN, #EVAS_CALLBACK_MOUSE_OUT, #EVAS_CALLBACK_MOUSE_DOWN, #EVAS_CALLBACK_MOUSE_UP, #EVAS_CALLBACK_MOUSE_MOVE, #EVAS_CALLBACK_MOUSE_WHEEL, #EVAS_CALLBACK_MULTI_DOWN, #EVAS_CALLBACK_MULTI_UP, #EVAS_CALLBACK_AXIS_UPDATE, #EVAS_CALLBACK_MULTI_MOVE, #EVAS_CALLBACK_FREE, #EVAS_CALLBACK_KEY_DOWN, #EVAS_CALLBACK_KEY_UP, #EVAS_CALLBACK_FOCUS_IN, #EVAS_CALLBACK_FOCUS_OUT, #EVAS_CALLBACK_SHOW, #EVAS_CALLBACK_HIDE, #EVAS_CALLBACK_MOVE, #EVAS_CALLBACK_RESIZE, #EVAS_CALLBACK_RESTACK, #EVAS_CALLBACK_DEL, #EVAS_CALLBACK_HOLD, #EVAS_CALLBACK_CHANGED_SIZE_HINTS, #EVAS_CALLBACK_IMAGE_PRELOADED or #EVAS_CALLBACK_IMAGE_UNLOADED.
This determines the kind of event that will trigger the callback. What follows is a list explaining better the nature of each type of event, along with their associated event_info
pointers:
- #EVAS_CALLBACK_MOUSE_IN:
event_info
is a pointer to an Evas_Event_Mouse_In struct
This event is triggered when the mouse pointer enters the area (not shaded by other objects) of the objectobj
. This may occur by the mouse pointer being moved by evas_event_feed_mouse_move() calls, or by the object being shown, raised, moved, resized, or other objects being moved out of the way, hidden or lowered, whatever may cause the mouse pointer to get on top ofobj
, having been on top of another object previously.
- #EVAS_CALLBACK_MOUSE_OUT:
event_info
is a pointer to an Evas_Event_Mouse_Out struct
This event is triggered exactly like #EVAS_CALLBACK_MOUSE_IN is, but it occurs when the mouse pointer exits an object's area. Note that no mouse out events will be reported if the mouse pointer is implicitly grabbed to an object (mouse buttons are down, having been pressed while the pointer was over that object). In these cases, mouse out events will be reported once all buttons are released, if the mouse pointer has left the object's area. The indirect ways of taking off the mouse pointer from an object, like cited above, for #EVAS_CALLBACK_MOUSE_IN, also apply here, naturally.
- #EVAS_CALLBACK_MOUSE_DOWN:
event_info
is a pointer to an Evas_Event_Mouse_Down struct
This event is triggered by a mouse button being pressed while the mouse pointer is over an object. If the pointer mode for Evas is EVAS_OBJECT_POINTER_MODE_AUTOGRAB (default), this causes this object to passively grab the mouse until all mouse buttons have been released: all future mouse events will be reported to only this object until no buttons are down. That includes mouse move events, mouse in and mouse out events, and further button presses. When all buttons are released, event propagation will occur as normal (see Evas_Object_Pointer_Mode).
- #EVAS_CALLBACK_MOUSE_UP:
event_info
is a pointer to an Evas_Event_Mouse_Up struct
This event is triggered by a mouse button being released while the mouse pointer is over an object's area (or when passively grabbed to an object).
- #EVAS_CALLBACK_MOUSE_MOVE:
event_info
is a pointer to an Evas_Event_Mouse_Move struct
This event is triggered by the mouse pointer being moved while over an object's area (or while passively grabbed to an object).
- #EVAS_CALLBACK_MOUSE_WHEEL:
event_info
is a pointer to an Evas_Event_Mouse_Wheel struct
This event is triggered by the mouse wheel being rolled while the mouse pointer is over an object (or passively grabbed to an object).
- #EVAS_CALLBACK_MULTI_DOWN:
event_info
is a pointer to an Evas_Event_Multi_Down struct
- #EVAS_CALLBACK_MULTI_UP:
event_info
is a pointer to an Evas_Event_Multi_Up struct
- #EVAS_CALLBACK_MULTI_MOVE:
event_info
is a pointer to an Evas_Event_Multi_Move struct
- #EVAS_CALLBACK_AXIS_UPDATE:
event_info
is a pointer to an Evas_Event_Axis_Update struct
- #EVAS_CALLBACK_FREE:
event_info
isNULL
This event is triggered just before Evas is about to free all memory used by an object and remove all references to it. This is useful for programs to use if they attached data to an object and want to free it when the object is deleted. The object is still valid when this callback is called, but after it returns, there is no guarantee on the object's validity.
- #EVAS_CALLBACK_KEY_DOWN:
event_info
is a pointer to an Evas_Event_Key_Down struct
This callback is called when a key is pressed and the focus is on the object, or a key has been grabbed to a particular object which wants to intercept the key press regardless of what object has the focus.
- #EVAS_CALLBACK_KEY_UP:
event_info
is a pointer to an Evas_Event_Key_Up struct
This callback is called when a key is released and the focus is on the object, or a key has been grabbed to a particular object which wants to intercept the key release regardless of what object has the focus.
- #EVAS_CALLBACK_FOCUS_IN:
event_info
isNULL
This event is called when an object gains the focus. When it is called the object has already gained the focus.
- #EVAS_CALLBACK_FOCUS_OUT:
event_info
isNULL
This event is triggered when an object loses the focus. When it is called the object has already lost the focus.
- #EVAS_CALLBACK_SHOW:
event_info
isNULL
This event is triggered by the object being shown by evas_object_show().
- #EVAS_CALLBACK_HIDE:
event_info
isNULL
This event is triggered by an object being hidden by evas_object_hide().
- #EVAS_CALLBACK_MOVE:
event_info
isNULL
This event is triggered by an object being moved. evas_object_move() can trigger this, as can any object-specific manipulations that would mean the object's origin could move.
- #EVAS_CALLBACK_RESIZE:
event_info
isNULL
This event is triggered by an object being resized. Resizes can be triggered by evas_object_resize() or by any object-specific calls that may cause the object to resize.
- #EVAS_CALLBACK_RESTACK:
event_info
isNULL
This event is triggered by an object being re-stacked. Stacking changes can be triggered by evas_object_stack_below()/evas_object_stack_above() and others.
- #EVAS_CALLBACK_DEL:
event_info
isNULL
.
- #EVAS_CALLBACK_HOLD:
event_info
is a pointer to an Evas_Event_Hold struct
- #EVAS_CALLBACK_CHANGED_SIZE_HINTS:
event_info
isNULL
.
- #EVAS_CALLBACK_IMAGE_PRELOADED:
event_info
isNULL
.
- #EVAS_CALLBACK_IMAGE_UNLOADED:
event_info
isNULL
.
- Note:
- Be careful not to add the same callback multiple times, if that's not what you want, because Evas won't check if a callback existed before exactly as the one being registered (and thus, call it more than once on the event, in this case). This would make sense if you passed different functions and/or callback data, only.
Example:
evas_object_event_callback_add( d.bg, EVAS_CALLBACK_KEY_DOWN, _on_keydown, NULL); if (evas_alloc_error() != EVAS_ALLOC_ERROR_NONE) { fprintf(stderr, "ERROR: Callback registering failed! Aborting.\n"); goto panic; }
See the full example here.
- Since :
- 2.3
- Examples:
- ctxpopup_example_01.c, ecore_evas_object_example.c, ecore_evas_window_sizes_example.c, ecore_imf_example.c, edje-basic.c, edje-box.c, edje-box2.c, edje-color-class.c, edje-drag.c, edje-perspective.c, edje-signals-messages.c, edje-table.c, edje-text.c, efl_thread_4.c, efl_thread_5.c, entry_example.c, evas-aspect-hints.c, evas-box.c, evas-events.c, evas-hints.c, evas-images.c, evas-images2.c, evas-map-utils.c, evas-object-manipulation.c, evas-smart-interface.c, evas-smart-object.c, evas-stacking.c, evas-text.c, glview_example_01.c, menu_example_01.c, slideshow_example.c, and web_example_02.c.
void* evas_object_event_callback_del | ( | Evas_Object * | obj, |
Evas_Callback_Type | type, | ||
Evas_Object_Event_Cb | func | ||
) |
Delete a callback function from an object
- Parameters:
-
obj Object to remove a callback from type The type of event that was triggering the callback func The function that was to be called when the event was triggered
- Returns:
- The data pointer that was to be passed to the callback
This function removes the most recently added callback from the object obj
which was triggered by the event type type
and was calling the function func
when triggered. If the removal is successful it will also return the data pointer that was passed to evas_object_event_callback_add() when the callback was added to the object. If not successful NULL
will be returned.
Example:
extern Evas_Object *object; void *my_data; void up_callback(void *data, Evas *e, Evas_Object *obj, void *event_info); my_data = evas_object_event_callback_del(object, EVAS_CALLBACK_MOUSE_UP, up_callback);
- Since :
- 2.3
- Examples:
- evas-smart-interface.c, and evas-smart-object.c.
void* evas_object_event_callback_del_full | ( | Evas_Object * | obj, |
Evas_Callback_Type | type, | ||
Evas_Object_Event_Cb | func, | ||
const void * | data | ||
) |
Delete (unregister) a callback function registered to a given Evas object event.
- Parameters:
-
obj Object to remove a callback from type The type of event that was triggering the callback func The function that was to be called when the event was triggered data The data pointer that was to be passed to the callback
- Returns:
- The data pointer that was to be passed to the callback
This function removes the most recently added callback from the object obj
, which was triggered by the event type type
and was calling the function func
with data data
, when triggered. If the removal is successful it will also return the data pointer that was passed to evas_object_event_callback_add() (that will be the same as the parameter) when the callback was added to the object. In errors, NULL
will be returned.
- Note:
- For deletion of Evas object events callbacks filtering by just type and function pointer, use evas_object_event_callback_del().
Example:
extern Evas_Object *object; void *my_data; void up_callback(void *data, Evas *e, Evas_Object *obj, void *event_info); my_data = evas_object_event_callback_del_full(object, EVAS_CALLBACK_MOUSE_UP, up_callback, data);
- Since :
- 2.3
void evas_object_event_callback_priority_add | ( | Evas_Object * | obj, |
Evas_Callback_Type | type, | ||
Evas_Callback_Priority | priority, | ||
Evas_Object_Event_Cb | func, | ||
const void * | data | ||
) |
Add (register) a callback function to a given Evas object event with a non-default priority set. Except for the priority field, it's exactly the same as evas_object_event_callback_add
- Parameters:
-
obj Object to attach a callback to type The type of event that will trigger the callback priority The priority of the callback, lower values called first. func The function to be called when the event is triggered data The data pointer to be passed to func
- See also:
- evas_object_event_callback_add
- Since (EFL) :
- 1.1
- Since :
- 2.3
Eina_Bool evas_object_key_grab | ( | Evas_Object * | obj, |
const char * | keyname, | ||
Evas_Modifier_Mask | modifiers, | ||
Evas_Modifier_Mask | not_modifiers, | ||
Eina_Bool | exclusive | ||
) |
Requests keyname
key events be directed to obj
.
Key grabs allow one or more objects to receive key events for specific key strokes even if other objects have focus. Whenever a key is grabbed, only the objects grabbing it will get the events for the given keys.
keyname
is a platform dependent symbolic name for the key pressed (see Key Input Functions for more information).
modifiers
and not_modifiers
are bit masks of all the modifiers that must and mustn't, respectively, be pressed along with keyname
key in order to trigger this new key grab. Modifiers can be things such as Shift and Ctrl as well as user defined types via evas_key_modifier_add. Retrieve them with evas_key_modifier_mask_get or use 0 for empty masks.
exclusive
will make the given object the only one permitted to grab the given key. If given true
, subsequent calls on this function with different obj
arguments will fail, unless the key is ungrabbed again.
- Warning:
- Providing impossible modifier sets creates undefined behavior.
See also evas_object_key_ungrab, evas_object_focus_get, evas_object_focus_set, evas_focus_get, evas_key_modifier_add.
- Parameters:
-
[in] keyname The key to request events for. [in] modifiers A mask of modifiers that must be present to trigger the event. [in] not_modifiers A mask of modifiers that must not be present to trigger the event. [in] exclusive Request that the obj
is the only object receiving thekeyname
events.
- Returns:
true
if the call succeeded,false
otherwise.
- Since :
- 2.3
- Examples:
- evas-events.c, and web_example_02.c.
void evas_object_key_ungrab | ( | Evas_Object * | obj, |
const char * | keyname, | ||
Evas_Modifier_Mask | modifiers, | ||
Evas_Modifier_Mask | not_modifiers | ||
) |
Removes the grab on keyname
key events by obj
.
Removes a key grab on obj
if keyname
, modifiers
, and not_modifiers
match.
See also evas_object_key_grab, evas_object_focus_get, evas_object_focus_set, evas_focus_get.
- Parameters:
-
[in] keyname The key the grab is set for. [in] modifiers A mask of modifiers that must be present to trigger the event. [in] not_modifiers A mask of modifiers that mus not not be present to trigger the event.
- Since :
- 2.3
- Examples:
- evas-events.c.